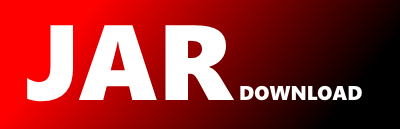
software.amazon.awssdk.services.location.DefaultLocationClient Maven / Gradle / Ivy
Show all versions of location Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.location;
import java.util.Collections;
import java.util.List;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.awscore.client.handler.AwsSyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.ApiName;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.client.handler.SyncClientHandler;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.util.VersionInfo;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.services.location.model.AccessDeniedException;
import software.amazon.awssdk.services.location.model.AssociateTrackerConsumerRequest;
import software.amazon.awssdk.services.location.model.AssociateTrackerConsumerResponse;
import software.amazon.awssdk.services.location.model.BatchDeleteDevicePositionHistoryRequest;
import software.amazon.awssdk.services.location.model.BatchDeleteDevicePositionHistoryResponse;
import software.amazon.awssdk.services.location.model.BatchDeleteGeofenceRequest;
import software.amazon.awssdk.services.location.model.BatchDeleteGeofenceResponse;
import software.amazon.awssdk.services.location.model.BatchEvaluateGeofencesRequest;
import software.amazon.awssdk.services.location.model.BatchEvaluateGeofencesResponse;
import software.amazon.awssdk.services.location.model.BatchGetDevicePositionRequest;
import software.amazon.awssdk.services.location.model.BatchGetDevicePositionResponse;
import software.amazon.awssdk.services.location.model.BatchPutGeofenceRequest;
import software.amazon.awssdk.services.location.model.BatchPutGeofenceResponse;
import software.amazon.awssdk.services.location.model.BatchUpdateDevicePositionRequest;
import software.amazon.awssdk.services.location.model.BatchUpdateDevicePositionResponse;
import software.amazon.awssdk.services.location.model.CalculateRouteMatrixRequest;
import software.amazon.awssdk.services.location.model.CalculateRouteMatrixResponse;
import software.amazon.awssdk.services.location.model.CalculateRouteRequest;
import software.amazon.awssdk.services.location.model.CalculateRouteResponse;
import software.amazon.awssdk.services.location.model.ConflictException;
import software.amazon.awssdk.services.location.model.CreateGeofenceCollectionRequest;
import software.amazon.awssdk.services.location.model.CreateGeofenceCollectionResponse;
import software.amazon.awssdk.services.location.model.CreateMapRequest;
import software.amazon.awssdk.services.location.model.CreateMapResponse;
import software.amazon.awssdk.services.location.model.CreatePlaceIndexRequest;
import software.amazon.awssdk.services.location.model.CreatePlaceIndexResponse;
import software.amazon.awssdk.services.location.model.CreateRouteCalculatorRequest;
import software.amazon.awssdk.services.location.model.CreateRouteCalculatorResponse;
import software.amazon.awssdk.services.location.model.CreateTrackerRequest;
import software.amazon.awssdk.services.location.model.CreateTrackerResponse;
import software.amazon.awssdk.services.location.model.DeleteGeofenceCollectionRequest;
import software.amazon.awssdk.services.location.model.DeleteGeofenceCollectionResponse;
import software.amazon.awssdk.services.location.model.DeleteMapRequest;
import software.amazon.awssdk.services.location.model.DeleteMapResponse;
import software.amazon.awssdk.services.location.model.DeletePlaceIndexRequest;
import software.amazon.awssdk.services.location.model.DeletePlaceIndexResponse;
import software.amazon.awssdk.services.location.model.DeleteRouteCalculatorRequest;
import software.amazon.awssdk.services.location.model.DeleteRouteCalculatorResponse;
import software.amazon.awssdk.services.location.model.DeleteTrackerRequest;
import software.amazon.awssdk.services.location.model.DeleteTrackerResponse;
import software.amazon.awssdk.services.location.model.DescribeGeofenceCollectionRequest;
import software.amazon.awssdk.services.location.model.DescribeGeofenceCollectionResponse;
import software.amazon.awssdk.services.location.model.DescribeMapRequest;
import software.amazon.awssdk.services.location.model.DescribeMapResponse;
import software.amazon.awssdk.services.location.model.DescribePlaceIndexRequest;
import software.amazon.awssdk.services.location.model.DescribePlaceIndexResponse;
import software.amazon.awssdk.services.location.model.DescribeRouteCalculatorRequest;
import software.amazon.awssdk.services.location.model.DescribeRouteCalculatorResponse;
import software.amazon.awssdk.services.location.model.DescribeTrackerRequest;
import software.amazon.awssdk.services.location.model.DescribeTrackerResponse;
import software.amazon.awssdk.services.location.model.DisassociateTrackerConsumerRequest;
import software.amazon.awssdk.services.location.model.DisassociateTrackerConsumerResponse;
import software.amazon.awssdk.services.location.model.GetDevicePositionHistoryRequest;
import software.amazon.awssdk.services.location.model.GetDevicePositionHistoryResponse;
import software.amazon.awssdk.services.location.model.GetDevicePositionRequest;
import software.amazon.awssdk.services.location.model.GetDevicePositionResponse;
import software.amazon.awssdk.services.location.model.GetGeofenceRequest;
import software.amazon.awssdk.services.location.model.GetGeofenceResponse;
import software.amazon.awssdk.services.location.model.GetMapGlyphsRequest;
import software.amazon.awssdk.services.location.model.GetMapGlyphsResponse;
import software.amazon.awssdk.services.location.model.GetMapSpritesRequest;
import software.amazon.awssdk.services.location.model.GetMapSpritesResponse;
import software.amazon.awssdk.services.location.model.GetMapStyleDescriptorRequest;
import software.amazon.awssdk.services.location.model.GetMapStyleDescriptorResponse;
import software.amazon.awssdk.services.location.model.GetMapTileRequest;
import software.amazon.awssdk.services.location.model.GetMapTileResponse;
import software.amazon.awssdk.services.location.model.GetPlaceRequest;
import software.amazon.awssdk.services.location.model.GetPlaceResponse;
import software.amazon.awssdk.services.location.model.InternalServerException;
import software.amazon.awssdk.services.location.model.ListDevicePositionsRequest;
import software.amazon.awssdk.services.location.model.ListDevicePositionsResponse;
import software.amazon.awssdk.services.location.model.ListGeofenceCollectionsRequest;
import software.amazon.awssdk.services.location.model.ListGeofenceCollectionsResponse;
import software.amazon.awssdk.services.location.model.ListGeofencesRequest;
import software.amazon.awssdk.services.location.model.ListGeofencesResponse;
import software.amazon.awssdk.services.location.model.ListMapsRequest;
import software.amazon.awssdk.services.location.model.ListMapsResponse;
import software.amazon.awssdk.services.location.model.ListPlaceIndexesRequest;
import software.amazon.awssdk.services.location.model.ListPlaceIndexesResponse;
import software.amazon.awssdk.services.location.model.ListRouteCalculatorsRequest;
import software.amazon.awssdk.services.location.model.ListRouteCalculatorsResponse;
import software.amazon.awssdk.services.location.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.location.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.location.model.ListTrackerConsumersRequest;
import software.amazon.awssdk.services.location.model.ListTrackerConsumersResponse;
import software.amazon.awssdk.services.location.model.ListTrackersRequest;
import software.amazon.awssdk.services.location.model.ListTrackersResponse;
import software.amazon.awssdk.services.location.model.LocationException;
import software.amazon.awssdk.services.location.model.LocationRequest;
import software.amazon.awssdk.services.location.model.PutGeofenceRequest;
import software.amazon.awssdk.services.location.model.PutGeofenceResponse;
import software.amazon.awssdk.services.location.model.ResourceNotFoundException;
import software.amazon.awssdk.services.location.model.SearchPlaceIndexForPositionRequest;
import software.amazon.awssdk.services.location.model.SearchPlaceIndexForPositionResponse;
import software.amazon.awssdk.services.location.model.SearchPlaceIndexForSuggestionsRequest;
import software.amazon.awssdk.services.location.model.SearchPlaceIndexForSuggestionsResponse;
import software.amazon.awssdk.services.location.model.SearchPlaceIndexForTextRequest;
import software.amazon.awssdk.services.location.model.SearchPlaceIndexForTextResponse;
import software.amazon.awssdk.services.location.model.ServiceQuotaExceededException;
import software.amazon.awssdk.services.location.model.TagResourceRequest;
import software.amazon.awssdk.services.location.model.TagResourceResponse;
import software.amazon.awssdk.services.location.model.ThrottlingException;
import software.amazon.awssdk.services.location.model.UntagResourceRequest;
import software.amazon.awssdk.services.location.model.UntagResourceResponse;
import software.amazon.awssdk.services.location.model.UpdateGeofenceCollectionRequest;
import software.amazon.awssdk.services.location.model.UpdateGeofenceCollectionResponse;
import software.amazon.awssdk.services.location.model.UpdateMapRequest;
import software.amazon.awssdk.services.location.model.UpdateMapResponse;
import software.amazon.awssdk.services.location.model.UpdatePlaceIndexRequest;
import software.amazon.awssdk.services.location.model.UpdatePlaceIndexResponse;
import software.amazon.awssdk.services.location.model.UpdateRouteCalculatorRequest;
import software.amazon.awssdk.services.location.model.UpdateRouteCalculatorResponse;
import software.amazon.awssdk.services.location.model.UpdateTrackerRequest;
import software.amazon.awssdk.services.location.model.UpdateTrackerResponse;
import software.amazon.awssdk.services.location.model.ValidationException;
import software.amazon.awssdk.services.location.paginators.GetDevicePositionHistoryIterable;
import software.amazon.awssdk.services.location.paginators.ListDevicePositionsIterable;
import software.amazon.awssdk.services.location.paginators.ListGeofenceCollectionsIterable;
import software.amazon.awssdk.services.location.paginators.ListGeofencesIterable;
import software.amazon.awssdk.services.location.paginators.ListMapsIterable;
import software.amazon.awssdk.services.location.paginators.ListPlaceIndexesIterable;
import software.amazon.awssdk.services.location.paginators.ListRouteCalculatorsIterable;
import software.amazon.awssdk.services.location.paginators.ListTrackerConsumersIterable;
import software.amazon.awssdk.services.location.paginators.ListTrackersIterable;
import software.amazon.awssdk.services.location.transform.AssociateTrackerConsumerRequestMarshaller;
import software.amazon.awssdk.services.location.transform.BatchDeleteDevicePositionHistoryRequestMarshaller;
import software.amazon.awssdk.services.location.transform.BatchDeleteGeofenceRequestMarshaller;
import software.amazon.awssdk.services.location.transform.BatchEvaluateGeofencesRequestMarshaller;
import software.amazon.awssdk.services.location.transform.BatchGetDevicePositionRequestMarshaller;
import software.amazon.awssdk.services.location.transform.BatchPutGeofenceRequestMarshaller;
import software.amazon.awssdk.services.location.transform.BatchUpdateDevicePositionRequestMarshaller;
import software.amazon.awssdk.services.location.transform.CalculateRouteMatrixRequestMarshaller;
import software.amazon.awssdk.services.location.transform.CalculateRouteRequestMarshaller;
import software.amazon.awssdk.services.location.transform.CreateGeofenceCollectionRequestMarshaller;
import software.amazon.awssdk.services.location.transform.CreateMapRequestMarshaller;
import software.amazon.awssdk.services.location.transform.CreatePlaceIndexRequestMarshaller;
import software.amazon.awssdk.services.location.transform.CreateRouteCalculatorRequestMarshaller;
import software.amazon.awssdk.services.location.transform.CreateTrackerRequestMarshaller;
import software.amazon.awssdk.services.location.transform.DeleteGeofenceCollectionRequestMarshaller;
import software.amazon.awssdk.services.location.transform.DeleteMapRequestMarshaller;
import software.amazon.awssdk.services.location.transform.DeletePlaceIndexRequestMarshaller;
import software.amazon.awssdk.services.location.transform.DeleteRouteCalculatorRequestMarshaller;
import software.amazon.awssdk.services.location.transform.DeleteTrackerRequestMarshaller;
import software.amazon.awssdk.services.location.transform.DescribeGeofenceCollectionRequestMarshaller;
import software.amazon.awssdk.services.location.transform.DescribeMapRequestMarshaller;
import software.amazon.awssdk.services.location.transform.DescribePlaceIndexRequestMarshaller;
import software.amazon.awssdk.services.location.transform.DescribeRouteCalculatorRequestMarshaller;
import software.amazon.awssdk.services.location.transform.DescribeTrackerRequestMarshaller;
import software.amazon.awssdk.services.location.transform.DisassociateTrackerConsumerRequestMarshaller;
import software.amazon.awssdk.services.location.transform.GetDevicePositionHistoryRequestMarshaller;
import software.amazon.awssdk.services.location.transform.GetDevicePositionRequestMarshaller;
import software.amazon.awssdk.services.location.transform.GetGeofenceRequestMarshaller;
import software.amazon.awssdk.services.location.transform.GetMapGlyphsRequestMarshaller;
import software.amazon.awssdk.services.location.transform.GetMapSpritesRequestMarshaller;
import software.amazon.awssdk.services.location.transform.GetMapStyleDescriptorRequestMarshaller;
import software.amazon.awssdk.services.location.transform.GetMapTileRequestMarshaller;
import software.amazon.awssdk.services.location.transform.GetPlaceRequestMarshaller;
import software.amazon.awssdk.services.location.transform.ListDevicePositionsRequestMarshaller;
import software.amazon.awssdk.services.location.transform.ListGeofenceCollectionsRequestMarshaller;
import software.amazon.awssdk.services.location.transform.ListGeofencesRequestMarshaller;
import software.amazon.awssdk.services.location.transform.ListMapsRequestMarshaller;
import software.amazon.awssdk.services.location.transform.ListPlaceIndexesRequestMarshaller;
import software.amazon.awssdk.services.location.transform.ListRouteCalculatorsRequestMarshaller;
import software.amazon.awssdk.services.location.transform.ListTagsForResourceRequestMarshaller;
import software.amazon.awssdk.services.location.transform.ListTrackerConsumersRequestMarshaller;
import software.amazon.awssdk.services.location.transform.ListTrackersRequestMarshaller;
import software.amazon.awssdk.services.location.transform.PutGeofenceRequestMarshaller;
import software.amazon.awssdk.services.location.transform.SearchPlaceIndexForPositionRequestMarshaller;
import software.amazon.awssdk.services.location.transform.SearchPlaceIndexForSuggestionsRequestMarshaller;
import software.amazon.awssdk.services.location.transform.SearchPlaceIndexForTextRequestMarshaller;
import software.amazon.awssdk.services.location.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.location.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.location.transform.UpdateGeofenceCollectionRequestMarshaller;
import software.amazon.awssdk.services.location.transform.UpdateMapRequestMarshaller;
import software.amazon.awssdk.services.location.transform.UpdatePlaceIndexRequestMarshaller;
import software.amazon.awssdk.services.location.transform.UpdateRouteCalculatorRequestMarshaller;
import software.amazon.awssdk.services.location.transform.UpdateTrackerRequestMarshaller;
import software.amazon.awssdk.utils.Logger;
/**
* Internal implementation of {@link LocationClient}.
*
* @see LocationClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultLocationClient implements LocationClient {
private static final Logger log = Logger.loggerFor(DefaultLocationClient.class);
private final SyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultLocationClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsSyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
*
* Creates an association between a geofence collection and a tracker resource. This allows the tracker resource to
* communicate location data to the linked geofence collection.
*
*
* You can associate up to five geofence collections to each tracker resource.
*
*
*
* Currently not supported — Cross-account configurations, such as creating associations between a tracker resource
* in one account and a geofence collection in another account.
*
*
*
* @param associateTrackerConsumerRequest
* @return Result of the AssociateTrackerConsumer operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws ConflictException
* The request was unsuccessful because of a conflict.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ServiceQuotaExceededException
* The operation was denied because the request would exceed the maximum quota set for
* Amazon Location Service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.AssociateTrackerConsumer
* @see AWS API Documentation
*/
@Override
public AssociateTrackerConsumerResponse associateTrackerConsumer(
AssociateTrackerConsumerRequest associateTrackerConsumerRequest) throws InternalServerException,
ResourceNotFoundException, ConflictException, AccessDeniedException, ValidationException,
ServiceQuotaExceededException, ThrottlingException, AwsServiceException, SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AssociateTrackerConsumerResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, associateTrackerConsumerRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateTrackerConsumer");
String hostPrefix = "tracking.";
String resolvedHostExpression = "tracking.";
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AssociateTrackerConsumer").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(associateTrackerConsumerRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new AssociateTrackerConsumerRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes the position history of one or more devices from a tracker resource.
*
*
* @param batchDeleteDevicePositionHistoryRequest
* @return Result of the BatchDeleteDevicePositionHistory operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.BatchDeleteDevicePositionHistory
* @see AWS API Documentation
*/
@Override
public BatchDeleteDevicePositionHistoryResponse batchDeleteDevicePositionHistory(
BatchDeleteDevicePositionHistoryRequest batchDeleteDevicePositionHistoryRequest) throws InternalServerException,
ResourceNotFoundException, AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException,
SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchDeleteDevicePositionHistoryResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
batchDeleteDevicePositionHistoryRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchDeleteDevicePositionHistory");
String hostPrefix = "tracking.";
String resolvedHostExpression = "tracking.";
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchDeleteDevicePositionHistory").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(batchDeleteDevicePositionHistoryRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new BatchDeleteDevicePositionHistoryRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a batch of geofences from a geofence collection.
*
*
*
* This operation deletes the resource permanently.
*
*
*
* @param batchDeleteGeofenceRequest
* @return Result of the BatchDeleteGeofence operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.BatchDeleteGeofence
* @see AWS
* API Documentation
*/
@Override
public BatchDeleteGeofenceResponse batchDeleteGeofence(BatchDeleteGeofenceRequest batchDeleteGeofenceRequest)
throws InternalServerException, ResourceNotFoundException, AccessDeniedException, ValidationException,
ThrottlingException, AwsServiceException, SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchDeleteGeofenceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchDeleteGeofenceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchDeleteGeofence");
String hostPrefix = "geofencing.";
String resolvedHostExpression = "geofencing.";
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("BatchDeleteGeofence").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(batchDeleteGeofenceRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new BatchDeleteGeofenceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Evaluates device positions against the geofence geometries from a given geofence collection.
*
*
* This operation always returns an empty response because geofences are asynchronously evaluated. The evaluation
* determines if the device has entered or exited a geofenced area, and then publishes one of the following events
* to Amazon EventBridge:
*
*
* -
*
* ENTER
if Amazon Location determines that the tracked device has entered a geofenced area.
*
*
* -
*
* EXIT
if Amazon Location determines that the tracked device has exited a geofenced area.
*
*
*
*
*
* The last geofence that a device was observed within is tracked for 30 days after the most recent device position
* update.
*
*
*
* Geofence evaluation uses the given device position. It does not account for the optional Accuracy
of
* a DevicePositionUpdate
.
*
*
*
* The DeviceID
is used as a string to represent the device. You do not need to have a
* Tracker
associated with the DeviceID
.
*
*
*
* @param batchEvaluateGeofencesRequest
* @return Result of the BatchEvaluateGeofences operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.BatchEvaluateGeofences
* @see AWS API Documentation
*/
@Override
public BatchEvaluateGeofencesResponse batchEvaluateGeofences(BatchEvaluateGeofencesRequest batchEvaluateGeofencesRequest)
throws InternalServerException, ResourceNotFoundException, AccessDeniedException, ValidationException,
ThrottlingException, AwsServiceException, SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchEvaluateGeofencesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchEvaluateGeofencesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchEvaluateGeofences");
String hostPrefix = "geofencing.";
String resolvedHostExpression = "geofencing.";
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchEvaluateGeofences").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(batchEvaluateGeofencesRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new BatchEvaluateGeofencesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists the latest device positions for requested devices.
*
*
* @param batchGetDevicePositionRequest
* @return Result of the BatchGetDevicePosition operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.BatchGetDevicePosition
* @see AWS API Documentation
*/
@Override
public BatchGetDevicePositionResponse batchGetDevicePosition(BatchGetDevicePositionRequest batchGetDevicePositionRequest)
throws InternalServerException, ResourceNotFoundException, AccessDeniedException, ValidationException,
ThrottlingException, AwsServiceException, SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchGetDevicePositionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchGetDevicePositionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchGetDevicePosition");
String hostPrefix = "tracking.";
String resolvedHostExpression = "tracking.";
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchGetDevicePosition").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(batchGetDevicePositionRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new BatchGetDevicePositionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* A batch request for storing geofence geometries into a given geofence collection, or updates the geometry of an
* existing geofence if a geofence ID is included in the request.
*
*
* @param batchPutGeofenceRequest
* @return Result of the BatchPutGeofence operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.BatchPutGeofence
* @see AWS API
* Documentation
*/
@Override
public BatchPutGeofenceResponse batchPutGeofence(BatchPutGeofenceRequest batchPutGeofenceRequest)
throws InternalServerException, ResourceNotFoundException, AccessDeniedException, ValidationException,
ThrottlingException, AwsServiceException, SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
BatchPutGeofenceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchPutGeofenceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchPutGeofence");
String hostPrefix = "geofencing.";
String resolvedHostExpression = "geofencing.";
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("BatchPutGeofence").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(batchPutGeofenceRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new BatchPutGeofenceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Uploads position update data for one or more devices to a tracker resource. Amazon Location uses the data when it
* reports the last known device position and position history. Amazon Location retains location data for 30 days.
*
*
*
* Position updates are handled based on the PositionFiltering
property of the tracker. When
* PositionFiltering
is set to TimeBased
, updates are evaluated against linked geofence
* collections, and location data is stored at a maximum of one position per 30 second interval. If your update
* frequency is more often than every 30 seconds, only one update per 30 seconds is stored for each unique device
* ID.
*
*
* When PositionFiltering
is set to DistanceBased
filtering, location data is stored and
* evaluated against linked geofence collections only if the device has moved more than 30 m (98.4 ft).
*
*
* When PositionFiltering
is set to AccuracyBased
filtering, location data is stored and
* evaluated against linked geofence collections only if the device has moved more than the measured accuracy. For
* example, if two consecutive updates from a device have a horizontal accuracy of 5 m and 10 m, the second update
* is neither stored or evaluated if the device has moved less than 15 m. If PositionFiltering
is set
* to AccuracyBased
filtering, Amazon Location uses the default value { "Horizontal": 0}
* when accuracy is not provided on a DevicePositionUpdate
.
*
*
*
* @param batchUpdateDevicePositionRequest
* @return Result of the BatchUpdateDevicePosition operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.BatchUpdateDevicePosition
* @see AWS API Documentation
*/
@Override
public BatchUpdateDevicePositionResponse batchUpdateDevicePosition(
BatchUpdateDevicePositionRequest batchUpdateDevicePositionRequest) throws InternalServerException,
ResourceNotFoundException, AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException,
SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchUpdateDevicePositionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchUpdateDevicePositionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchUpdateDevicePosition");
String hostPrefix = "tracking.";
String resolvedHostExpression = "tracking.";
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchUpdateDevicePosition").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(batchUpdateDevicePositionRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new BatchUpdateDevicePositionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Calculates a route
* given the following required parameters: DeparturePosition
and DestinationPosition
.
* Requires that you first create a
* route calculator resource.
*
*
* By default, a request that doesn't specify a departure time uses the best time of day to travel with the best
* traffic conditions when calculating the route.
*
*
* Additional options include:
*
*
* -
*
* Specifying a departure
* time using either DepartureTime
or DepartNow
. This calculates a route based on
* predictive traffic data at the given time.
*
*
*
* You can't specify both DepartureTime
and DepartNow
in a single request. Specifying both
* parameters returns a validation error.
*
*
* -
*
* Specifying a travel
* mode using TravelMode sets the transportation mode used to calculate the routes. This also lets you specify
* additional route preferences in CarModeOptions
if traveling by Car
, or
* TruckModeOptions
if traveling by Truck
.
*
*
*
* If you specify walking
for the travel mode and your data provider is Esri, the start and destination
* must be within 40km.
*
*
*
*
* @param calculateRouteRequest
* @return Result of the CalculateRoute operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.CalculateRoute
* @see AWS API
* Documentation
*/
@Override
public CalculateRouteResponse calculateRoute(CalculateRouteRequest calculateRouteRequest) throws InternalServerException,
ResourceNotFoundException, AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException,
SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CalculateRouteResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, calculateRouteRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CalculateRoute");
String hostPrefix = "routes.";
String resolvedHostExpression = "routes.";
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CalculateRoute").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(calculateRouteRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CalculateRouteRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Calculates a
* route matrix given the following required parameters: DeparturePositions
and
* DestinationPositions
. CalculateRouteMatrix
calculates routes and returns the travel
* time and travel distance from each departure position to each destination position in the request. For example,
* given departure positions A and B, and destination positions X and Y, CalculateRouteMatrix
will
* return time and distance for routes from A to X, A to Y, B to X, and B to Y (in that order). The number of
* results returned (and routes calculated) will be the number of DeparturePositions
times the number
* of DestinationPositions
.
*
*
*
* Your account is charged for each route calculated, not the number of requests.
*
*
*
* Requires that you first create a
* route calculator resource.
*
*
* By default, a request that doesn't specify a departure time uses the best time of day to travel with the best
* traffic conditions when calculating routes.
*
*
* Additional options include:
*
*
* -
*
* Specifying a departure
* time using either DepartureTime
or DepartNow
. This calculates routes based on
* predictive traffic data at the given time.
*
*
*
* You can't specify both DepartureTime
and DepartNow
in a single request. Specifying both
* parameters returns a validation error.
*
*
* -
*
* Specifying a travel
* mode using TravelMode sets the transportation mode used to calculate the routes. This also lets you specify
* additional route preferences in CarModeOptions
if traveling by Car
, or
* TruckModeOptions
if traveling by Truck
.
*
*
*
*
* @param calculateRouteMatrixRequest
* @return Result of the CalculateRouteMatrix operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.CalculateRouteMatrix
* @see AWS
* API Documentation
*/
@Override
public CalculateRouteMatrixResponse calculateRouteMatrix(CalculateRouteMatrixRequest calculateRouteMatrixRequest)
throws InternalServerException, ResourceNotFoundException, AccessDeniedException, ValidationException,
ThrottlingException, AwsServiceException, SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CalculateRouteMatrixResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, calculateRouteMatrixRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CalculateRouteMatrix");
String hostPrefix = "routes.";
String resolvedHostExpression = "routes.";
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CalculateRouteMatrix").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(calculateRouteMatrixRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CalculateRouteMatrixRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a geofence collection, which manages and stores geofences.
*
*
* @param createGeofenceCollectionRequest
* @return Result of the CreateGeofenceCollection operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ConflictException
* The request was unsuccessful because of a conflict.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.CreateGeofenceCollection
* @see AWS API Documentation
*/
@Override
public CreateGeofenceCollectionResponse createGeofenceCollection(
CreateGeofenceCollectionRequest createGeofenceCollectionRequest) throws InternalServerException, ConflictException,
AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException, SdkClientException,
LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateGeofenceCollectionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createGeofenceCollectionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateGeofenceCollection");
String hostPrefix = "geofencing.";
String resolvedHostExpression = "geofencing.";
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateGeofenceCollection").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(createGeofenceCollectionRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateGeofenceCollectionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a map resource in your AWS account, which provides map tiles of different styles sourced from global
* location data providers.
*
*
*
* If your application is tracking or routing assets you use in your business, such as delivery vehicles or
* employees, you may only use HERE as your geolocation provider. See section 82 of the AWS service terms for more details.
*
*
*
* @param createMapRequest
* @return Result of the CreateMap operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ConflictException
* The request was unsuccessful because of a conflict.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.CreateMap
* @see AWS API
* Documentation
*/
@Override
public CreateMapResponse createMap(CreateMapRequest createMapRequest) throws InternalServerException, ConflictException,
AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException, SdkClientException,
LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateMapResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createMapRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateMap");
String hostPrefix = "maps.";
String resolvedHostExpression = "maps.";
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateMap").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(createMapRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateMapRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a place index resource in your AWS account. Use a place index resource to geocode addresses and other
* text queries by using the SearchPlaceIndexForText
operation, and reverse geocode coordinates by
* using the SearchPlaceIndexForPosition
operation, and enable autosuggestions by using the
* SearchPlaceIndexForSuggestions
operation.
*
*
*
* If your application is tracking or routing assets you use in your business, such as delivery vehicles or
* employees, you may only use HERE as your geolocation provider. See section 82 of the AWS service terms for more details.
*
*
*
* @param createPlaceIndexRequest
* @return Result of the CreatePlaceIndex operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ConflictException
* The request was unsuccessful because of a conflict.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.CreatePlaceIndex
* @see AWS API
* Documentation
*/
@Override
public CreatePlaceIndexResponse createPlaceIndex(CreatePlaceIndexRequest createPlaceIndexRequest)
throws InternalServerException, ConflictException, AccessDeniedException, ValidationException, ThrottlingException,
AwsServiceException, SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreatePlaceIndexResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createPlaceIndexRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreatePlaceIndex");
String hostPrefix = "places.";
String resolvedHostExpression = "places.";
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreatePlaceIndex").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(createPlaceIndexRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreatePlaceIndexRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a route calculator resource in your AWS account.
*
*
* You can send requests to a route calculator resource to estimate travel time, distance, and get directions. A
* route calculator sources traffic and road network data from your chosen data provider.
*
*
*
* If your application is tracking or routing assets you use in your business, such as delivery vehicles or
* employees, you may only use HERE as your geolocation provider. See section 82 of the AWS service terms for more details.
*
*
*
* @param createRouteCalculatorRequest
* @return Result of the CreateRouteCalculator operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ConflictException
* The request was unsuccessful because of a conflict.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.CreateRouteCalculator
* @see AWS API Documentation
*/
@Override
public CreateRouteCalculatorResponse createRouteCalculator(CreateRouteCalculatorRequest createRouteCalculatorRequest)
throws InternalServerException, ConflictException, AccessDeniedException, ValidationException, ThrottlingException,
AwsServiceException, SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateRouteCalculatorResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createRouteCalculatorRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateRouteCalculator");
String hostPrefix = "routes.";
String resolvedHostExpression = "routes.";
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateRouteCalculator").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(createRouteCalculatorRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateRouteCalculatorRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a tracker resource in your AWS account, which lets you retrieve current and historical location of
* devices.
*
*
* @param createTrackerRequest
* @return Result of the CreateTracker operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ConflictException
* The request was unsuccessful because of a conflict.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.CreateTracker
* @see AWS API
* Documentation
*/
@Override
public CreateTrackerResponse createTracker(CreateTrackerRequest createTrackerRequest) throws InternalServerException,
ConflictException, AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException,
SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateTrackerResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createTrackerRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateTracker");
String hostPrefix = "tracking.";
String resolvedHostExpression = "tracking.";
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateTracker").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(createTrackerRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateTrackerRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a geofence collection from your AWS account.
*
*
*
* This operation deletes the resource permanently. If the geofence collection is the target of a tracker resource,
* the devices will no longer be monitored.
*
*
*
* @param deleteGeofenceCollectionRequest
* @return Result of the DeleteGeofenceCollection operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.DeleteGeofenceCollection
* @see AWS API Documentation
*/
@Override
public DeleteGeofenceCollectionResponse deleteGeofenceCollection(
DeleteGeofenceCollectionRequest deleteGeofenceCollectionRequest) throws InternalServerException,
ResourceNotFoundException, AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException,
SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteGeofenceCollectionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteGeofenceCollectionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteGeofenceCollection");
String hostPrefix = "geofencing.";
String resolvedHostExpression = "geofencing.";
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteGeofenceCollection").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(deleteGeofenceCollectionRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteGeofenceCollectionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a map resource from your AWS account.
*
*
*
* This operation deletes the resource permanently. If the map is being used in an application, the map may not
* render.
*
*
*
* @param deleteMapRequest
* @return Result of the DeleteMap operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.DeleteMap
* @see AWS API
* Documentation
*/
@Override
public DeleteMapResponse deleteMap(DeleteMapRequest deleteMapRequest) throws InternalServerException,
ResourceNotFoundException, AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException,
SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteMapResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteMapRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteMap");
String hostPrefix = "maps.";
String resolvedHostExpression = "maps.";
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteMap").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(deleteMapRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteMapRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a place index resource from your AWS account.
*
*
*
* This operation deletes the resource permanently.
*
*
*
* @param deletePlaceIndexRequest
* @return Result of the DeletePlaceIndex operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.DeletePlaceIndex
* @see AWS API
* Documentation
*/
@Override
public DeletePlaceIndexResponse deletePlaceIndex(DeletePlaceIndexRequest deletePlaceIndexRequest)
throws InternalServerException, ResourceNotFoundException, AccessDeniedException, ValidationException,
ThrottlingException, AwsServiceException, SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeletePlaceIndexResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deletePlaceIndexRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeletePlaceIndex");
String hostPrefix = "places.";
String resolvedHostExpression = "places.";
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeletePlaceIndex").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(deletePlaceIndexRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeletePlaceIndexRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a route calculator resource from your AWS account.
*
*
*
* This operation deletes the resource permanently.
*
*
*
* @param deleteRouteCalculatorRequest
* @return Result of the DeleteRouteCalculator operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.DeleteRouteCalculator
* @see AWS API Documentation
*/
@Override
public DeleteRouteCalculatorResponse deleteRouteCalculator(DeleteRouteCalculatorRequest deleteRouteCalculatorRequest)
throws InternalServerException, ResourceNotFoundException, AccessDeniedException, ValidationException,
ThrottlingException, AwsServiceException, SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteRouteCalculatorResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteRouteCalculatorRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteRouteCalculator");
String hostPrefix = "routes.";
String resolvedHostExpression = "routes.";
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteRouteCalculator").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(deleteRouteCalculatorRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteRouteCalculatorRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a tracker resource from your AWS account.
*
*
*
* This operation deletes the resource permanently. If the tracker resource is in use, you may encounter an error.
* Make sure that the target resource isn't a dependency for your applications.
*
*
*
* @param deleteTrackerRequest
* @return Result of the DeleteTracker operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.DeleteTracker
* @see AWS API
* Documentation
*/
@Override
public DeleteTrackerResponse deleteTracker(DeleteTrackerRequest deleteTrackerRequest) throws InternalServerException,
ResourceNotFoundException, AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException,
SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteTrackerResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteTrackerRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteTracker");
String hostPrefix = "tracking.";
String resolvedHostExpression = "tracking.";
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteTracker").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(deleteTrackerRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteTrackerRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves the geofence collection details.
*
*
* @param describeGeofenceCollectionRequest
* @return Result of the DescribeGeofenceCollection operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.DescribeGeofenceCollection
* @see AWS API Documentation
*/
@Override
public DescribeGeofenceCollectionResponse describeGeofenceCollection(
DescribeGeofenceCollectionRequest describeGeofenceCollectionRequest) throws InternalServerException,
ResourceNotFoundException, AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException,
SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeGeofenceCollectionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeGeofenceCollectionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeGeofenceCollection");
String hostPrefix = "geofencing.";
String resolvedHostExpression = "geofencing.";
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeGeofenceCollection").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(describeGeofenceCollectionRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeGeofenceCollectionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves the map resource details.
*
*
* @param describeMapRequest
* @return Result of the DescribeMap operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.DescribeMap
* @see AWS API
* Documentation
*/
@Override
public DescribeMapResponse describeMap(DescribeMapRequest describeMapRequest) throws InternalServerException,
ResourceNotFoundException, AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException,
SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeMapResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeMapRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeMap");
String hostPrefix = "maps.";
String resolvedHostExpression = "maps.";
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeMap").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(describeMapRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeMapRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves the place index resource details.
*
*
* @param describePlaceIndexRequest
* @return Result of the DescribePlaceIndex operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.DescribePlaceIndex
* @see AWS
* API Documentation
*/
@Override
public DescribePlaceIndexResponse describePlaceIndex(DescribePlaceIndexRequest describePlaceIndexRequest)
throws InternalServerException, ResourceNotFoundException, AccessDeniedException, ValidationException,
ThrottlingException, AwsServiceException, SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribePlaceIndexResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describePlaceIndexRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribePlaceIndex");
String hostPrefix = "places.";
String resolvedHostExpression = "places.";
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribePlaceIndex").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(describePlaceIndexRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribePlaceIndexRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves the route calculator resource details.
*
*
* @param describeRouteCalculatorRequest
* @return Result of the DescribeRouteCalculator operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.DescribeRouteCalculator
* @see AWS API Documentation
*/
@Override
public DescribeRouteCalculatorResponse describeRouteCalculator(DescribeRouteCalculatorRequest describeRouteCalculatorRequest)
throws InternalServerException, ResourceNotFoundException, AccessDeniedException, ValidationException,
ThrottlingException, AwsServiceException, SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeRouteCalculatorResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeRouteCalculatorRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeRouteCalculator");
String hostPrefix = "routes.";
String resolvedHostExpression = "routes.";
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeRouteCalculator").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(describeRouteCalculatorRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeRouteCalculatorRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves the tracker resource details.
*
*
* @param describeTrackerRequest
* @return Result of the DescribeTracker operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.DescribeTracker
* @see AWS API
* Documentation
*/
@Override
public DescribeTrackerResponse describeTracker(DescribeTrackerRequest describeTrackerRequest) throws InternalServerException,
ResourceNotFoundException, AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException,
SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeTrackerResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeTrackerRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeTracker");
String hostPrefix = "tracking.";
String resolvedHostExpression = "tracking.";
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeTracker").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(describeTrackerRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeTrackerRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Removes the association between a tracker resource and a geofence collection.
*
*
*
* Once you unlink a tracker resource from a geofence collection, the tracker positions will no longer be
* automatically evaluated against geofences.
*
*
*
* @param disassociateTrackerConsumerRequest
* @return Result of the DisassociateTrackerConsumer operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.DisassociateTrackerConsumer
* @see AWS API Documentation
*/
@Override
public DisassociateTrackerConsumerResponse disassociateTrackerConsumer(
DisassociateTrackerConsumerRequest disassociateTrackerConsumerRequest) throws InternalServerException,
ResourceNotFoundException, AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException,
SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DisassociateTrackerConsumerResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, disassociateTrackerConsumerRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisassociateTrackerConsumer");
String hostPrefix = "tracking.";
String resolvedHostExpression = "tracking.";
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisassociateTrackerConsumer").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(disassociateTrackerConsumerRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DisassociateTrackerConsumerRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves a device's most recent position according to its sample time.
*
*
*
* Device positions are deleted after 30 days.
*
*
*
* @param getDevicePositionRequest
* @return Result of the GetDevicePosition operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.GetDevicePosition
* @see AWS
* API Documentation
*/
@Override
public GetDevicePositionResponse getDevicePosition(GetDevicePositionRequest getDevicePositionRequest)
throws InternalServerException, ResourceNotFoundException, AccessDeniedException, ValidationException,
ThrottlingException, AwsServiceException, SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetDevicePositionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getDevicePositionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetDevicePosition");
String hostPrefix = "tracking.";
String resolvedHostExpression = "tracking.";
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetDevicePosition").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(getDevicePositionRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetDevicePositionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves the device position history from a tracker resource within a specified range of time.
*
*
*
* Device positions are deleted after 30 days.
*
*
*
* @param getDevicePositionHistoryRequest
* @return Result of the GetDevicePositionHistory operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.GetDevicePositionHistory
* @see AWS API Documentation
*/
@Override
public GetDevicePositionHistoryResponse getDevicePositionHistory(
GetDevicePositionHistoryRequest getDevicePositionHistoryRequest) throws InternalServerException,
ResourceNotFoundException, AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException,
SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetDevicePositionHistoryResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getDevicePositionHistoryRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetDevicePositionHistory");
String hostPrefix = "tracking.";
String resolvedHostExpression = "tracking.";
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetDevicePositionHistory").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(getDevicePositionHistoryRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetDevicePositionHistoryRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves the device position history from a tracker resource within a specified range of time.
*
*
*
* Device positions are deleted after 30 days.
*
*
*
* This is a variant of
* {@link #getDevicePositionHistory(software.amazon.awssdk.services.location.model.GetDevicePositionHistoryRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.location.paginators.GetDevicePositionHistoryIterable responses = client.getDevicePositionHistoryPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.location.paginators.GetDevicePositionHistoryIterable responses = client
* .getDevicePositionHistoryPaginator(request);
* for (software.amazon.awssdk.services.location.model.GetDevicePositionHistoryResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.location.paginators.GetDevicePositionHistoryIterable responses = client.getDevicePositionHistoryPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getDevicePositionHistory(software.amazon.awssdk.services.location.model.GetDevicePositionHistoryRequest)}
* operation.
*
*
* @param getDevicePositionHistoryRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.GetDevicePositionHistory
* @see AWS API Documentation
*/
@Override
public GetDevicePositionHistoryIterable getDevicePositionHistoryPaginator(
GetDevicePositionHistoryRequest getDevicePositionHistoryRequest) throws InternalServerException,
ResourceNotFoundException, AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException,
SdkClientException, LocationException {
return new GetDevicePositionHistoryIterable(this, applyPaginatorUserAgent(getDevicePositionHistoryRequest));
}
/**
*
* Retrieves the geofence details from a geofence collection.
*
*
* @param getGeofenceRequest
* @return Result of the GetGeofence operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.GetGeofence
* @see AWS API
* Documentation
*/
@Override
public GetGeofenceResponse getGeofence(GetGeofenceRequest getGeofenceRequest) throws InternalServerException,
ResourceNotFoundException, AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException,
SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetGeofenceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getGeofenceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetGeofence");
String hostPrefix = "geofencing.";
String resolvedHostExpression = "geofencing.";
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetGeofence").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(getGeofenceRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetGeofenceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves glyphs used to display labels on a map.
*
*
* @param getMapGlyphsRequest
* @return Result of the GetMapGlyphs operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.GetMapGlyphs
* @see AWS API
* Documentation
*/
@Override
public GetMapGlyphsResponse getMapGlyphs(GetMapGlyphsRequest getMapGlyphsRequest) throws InternalServerException,
ResourceNotFoundException, AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException,
SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(false).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetMapGlyphsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getMapGlyphsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetMapGlyphs");
String hostPrefix = "maps.";
String resolvedHostExpression = "maps.";
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetMapGlyphs").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(getMapGlyphsRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetMapGlyphsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves the sprite sheet corresponding to a map resource. The sprite sheet is a PNG image paired with a JSON
* document describing the offsets of individual icons that will be displayed on a rendered map.
*
*
* @param getMapSpritesRequest
* @return Result of the GetMapSprites operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.GetMapSprites
* @see AWS API
* Documentation
*/
@Override
public GetMapSpritesResponse getMapSprites(GetMapSpritesRequest getMapSpritesRequest) throws InternalServerException,
ResourceNotFoundException, AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException,
SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(false).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetMapSpritesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getMapSpritesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetMapSprites");
String hostPrefix = "maps.";
String resolvedHostExpression = "maps.";
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetMapSprites").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(getMapSpritesRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetMapSpritesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves the map style descriptor from a map resource.
*
*
* The style descriptor contains specifications on how features render on a map. For example, what data to display,
* what order to display the data in, and the style for the data. Style descriptors follow the Mapbox Style
* Specification.
*
*
* @param getMapStyleDescriptorRequest
* @return Result of the GetMapStyleDescriptor operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.GetMapStyleDescriptor
* @see AWS API Documentation
*/
@Override
public GetMapStyleDescriptorResponse getMapStyleDescriptor(GetMapStyleDescriptorRequest getMapStyleDescriptorRequest)
throws InternalServerException, ResourceNotFoundException, AccessDeniedException, ValidationException,
ThrottlingException, AwsServiceException, SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(false).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetMapStyleDescriptorResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getMapStyleDescriptorRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetMapStyleDescriptor");
String hostPrefix = "maps.";
String resolvedHostExpression = "maps.";
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetMapStyleDescriptor").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(getMapStyleDescriptorRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetMapStyleDescriptorRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves a vector data tile from the map resource. Map tiles are used by clients to render a map. they're
* addressed using a grid arrangement with an X coordinate, Y coordinate, and Z (zoom) level.
*
*
* The origin (0, 0) is the top left of the map. Increasing the zoom level by 1 doubles both the X and Y dimensions,
* so a tile containing data for the entire world at (0/0/0) will be split into 4 tiles at zoom 1 (1/0/0, 1/0/1,
* 1/1/0, 1/1/1).
*
*
* @param getMapTileRequest
* @return Result of the GetMapTile operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.GetMapTile
* @see AWS API
* Documentation
*/
@Override
public GetMapTileResponse getMapTile(GetMapTileRequest getMapTileRequest) throws InternalServerException,
ResourceNotFoundException, AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException,
SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(false).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetMapTileResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getMapTileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetMapTile");
String hostPrefix = "maps.";
String resolvedHostExpression = "maps.";
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetMapTile").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(getMapTileRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetMapTileRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Finds a place by its unique ID. A PlaceId
is returned by other search operations.
*
*
*
* A PlaceId is valid only if all of the following are the same in the original search request and the call to
* GetPlace
.
*
*
* -
*
* Customer AWS account
*
*
* -
*
* AWS Region
*
*
* -
*
* Data provider specified in the place index resource
*
*
*
*
*
* @param getPlaceRequest
* @return Result of the GetPlace operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.GetPlace
* @see AWS API
* Documentation
*/
@Override
public GetPlaceResponse getPlace(GetPlaceRequest getPlaceRequest) throws InternalServerException, ResourceNotFoundException,
AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException, SdkClientException,
LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetPlaceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getPlaceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetPlace");
String hostPrefix = "places.";
String resolvedHostExpression = "places.";
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetPlace").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(getPlaceRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetPlaceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* A batch request to retrieve all device positions.
*
*
* @param listDevicePositionsRequest
* @return Result of the ListDevicePositions operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.ListDevicePositions
* @see AWS
* API Documentation
*/
@Override
public ListDevicePositionsResponse listDevicePositions(ListDevicePositionsRequest listDevicePositionsRequest)
throws InternalServerException, AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException,
SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListDevicePositionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listDevicePositionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListDevicePositions");
String hostPrefix = "tracking.";
String resolvedHostExpression = "tracking.";
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListDevicePositions").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(listDevicePositionsRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListDevicePositionsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* A batch request to retrieve all device positions.
*
*
*
* This is a variant of
* {@link #listDevicePositions(software.amazon.awssdk.services.location.model.ListDevicePositionsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.location.paginators.ListDevicePositionsIterable responses = client.listDevicePositionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.location.paginators.ListDevicePositionsIterable responses = client
* .listDevicePositionsPaginator(request);
* for (software.amazon.awssdk.services.location.model.ListDevicePositionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.location.paginators.ListDevicePositionsIterable responses = client.listDevicePositionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDevicePositions(software.amazon.awssdk.services.location.model.ListDevicePositionsRequest)}
* operation.
*
*
* @param listDevicePositionsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.ListDevicePositions
* @see AWS
* API Documentation
*/
@Override
public ListDevicePositionsIterable listDevicePositionsPaginator(ListDevicePositionsRequest listDevicePositionsRequest)
throws InternalServerException, AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException,
SdkClientException, LocationException {
return new ListDevicePositionsIterable(this, applyPaginatorUserAgent(listDevicePositionsRequest));
}
/**
*
* Lists geofence collections in your AWS account.
*
*
* @param listGeofenceCollectionsRequest
* @return Result of the ListGeofenceCollections operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.ListGeofenceCollections
* @see AWS API Documentation
*/
@Override
public ListGeofenceCollectionsResponse listGeofenceCollections(ListGeofenceCollectionsRequest listGeofenceCollectionsRequest)
throws InternalServerException, AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException,
SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListGeofenceCollectionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listGeofenceCollectionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListGeofenceCollections");
String hostPrefix = "geofencing.";
String resolvedHostExpression = "geofencing.";
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListGeofenceCollections").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(listGeofenceCollectionsRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListGeofenceCollectionsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists geofence collections in your AWS account.
*
*
*
* This is a variant of
* {@link #listGeofenceCollections(software.amazon.awssdk.services.location.model.ListGeofenceCollectionsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.location.paginators.ListGeofenceCollectionsIterable responses = client.listGeofenceCollectionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.location.paginators.ListGeofenceCollectionsIterable responses = client
* .listGeofenceCollectionsPaginator(request);
* for (software.amazon.awssdk.services.location.model.ListGeofenceCollectionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.location.paginators.ListGeofenceCollectionsIterable responses = client.listGeofenceCollectionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listGeofenceCollections(software.amazon.awssdk.services.location.model.ListGeofenceCollectionsRequest)}
* operation.
*
*
* @param listGeofenceCollectionsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.ListGeofenceCollections
* @see AWS API Documentation
*/
@Override
public ListGeofenceCollectionsIterable listGeofenceCollectionsPaginator(
ListGeofenceCollectionsRequest listGeofenceCollectionsRequest) throws InternalServerException, AccessDeniedException,
ValidationException, ThrottlingException, AwsServiceException, SdkClientException, LocationException {
return new ListGeofenceCollectionsIterable(this, applyPaginatorUserAgent(listGeofenceCollectionsRequest));
}
/**
*
* Lists geofences stored in a given geofence collection.
*
*
* @param listGeofencesRequest
* @return Result of the ListGeofences operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.ListGeofences
* @see AWS API
* Documentation
*/
@Override
public ListGeofencesResponse listGeofences(ListGeofencesRequest listGeofencesRequest) throws InternalServerException,
ResourceNotFoundException, AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException,
SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListGeofencesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listGeofencesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListGeofences");
String hostPrefix = "geofencing.";
String resolvedHostExpression = "geofencing.";
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListGeofences").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(listGeofencesRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListGeofencesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists geofences stored in a given geofence collection.
*
*
*
* This is a variant of {@link #listGeofences(software.amazon.awssdk.services.location.model.ListGeofencesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.location.paginators.ListGeofencesIterable responses = client.listGeofencesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.location.paginators.ListGeofencesIterable responses = client.listGeofencesPaginator(request);
* for (software.amazon.awssdk.services.location.model.ListGeofencesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.location.paginators.ListGeofencesIterable responses = client.listGeofencesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listGeofences(software.amazon.awssdk.services.location.model.ListGeofencesRequest)} operation.
*
*
* @param listGeofencesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.ListGeofences
* @see AWS API
* Documentation
*/
@Override
public ListGeofencesIterable listGeofencesPaginator(ListGeofencesRequest listGeofencesRequest)
throws InternalServerException, ResourceNotFoundException, AccessDeniedException, ValidationException,
ThrottlingException, AwsServiceException, SdkClientException, LocationException {
return new ListGeofencesIterable(this, applyPaginatorUserAgent(listGeofencesRequest));
}
/**
*
* Lists map resources in your AWS account.
*
*
* @param listMapsRequest
* @return Result of the ListMaps operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.ListMaps
* @see AWS API
* Documentation
*/
@Override
public ListMapsResponse listMaps(ListMapsRequest listMapsRequest) throws InternalServerException, AccessDeniedException,
ValidationException, ThrottlingException, AwsServiceException, SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListMapsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listMapsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListMaps");
String hostPrefix = "maps.";
String resolvedHostExpression = "maps.";
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListMaps").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(listMapsRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListMapsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists map resources in your AWS account.
*
*
*
* This is a variant of {@link #listMaps(software.amazon.awssdk.services.location.model.ListMapsRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.location.paginators.ListMapsIterable responses = client.listMapsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.location.paginators.ListMapsIterable responses = client.listMapsPaginator(request);
* for (software.amazon.awssdk.services.location.model.ListMapsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.location.paginators.ListMapsIterable responses = client.listMapsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listMaps(software.amazon.awssdk.services.location.model.ListMapsRequest)} operation.
*
*
* @param listMapsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.ListMaps
* @see AWS API
* Documentation
*/
@Override
public ListMapsIterable listMapsPaginator(ListMapsRequest listMapsRequest) throws InternalServerException,
AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException, SdkClientException,
LocationException {
return new ListMapsIterable(this, applyPaginatorUserAgent(listMapsRequest));
}
/**
*
* Lists place index resources in your AWS account.
*
*
* @param listPlaceIndexesRequest
* @return Result of the ListPlaceIndexes operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.ListPlaceIndexes
* @see AWS API
* Documentation
*/
@Override
public ListPlaceIndexesResponse listPlaceIndexes(ListPlaceIndexesRequest listPlaceIndexesRequest)
throws InternalServerException, AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException,
SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListPlaceIndexesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listPlaceIndexesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListPlaceIndexes");
String hostPrefix = "places.";
String resolvedHostExpression = "places.";
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListPlaceIndexes").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(listPlaceIndexesRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListPlaceIndexesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists place index resources in your AWS account.
*
*
*
* This is a variant of
* {@link #listPlaceIndexes(software.amazon.awssdk.services.location.model.ListPlaceIndexesRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.location.paginators.ListPlaceIndexesIterable responses = client.listPlaceIndexesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.location.paginators.ListPlaceIndexesIterable responses = client
* .listPlaceIndexesPaginator(request);
* for (software.amazon.awssdk.services.location.model.ListPlaceIndexesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.location.paginators.ListPlaceIndexesIterable responses = client.listPlaceIndexesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPlaceIndexes(software.amazon.awssdk.services.location.model.ListPlaceIndexesRequest)} operation.
*
*
* @param listPlaceIndexesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.ListPlaceIndexes
* @see AWS API
* Documentation
*/
@Override
public ListPlaceIndexesIterable listPlaceIndexesPaginator(ListPlaceIndexesRequest listPlaceIndexesRequest)
throws InternalServerException, AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException,
SdkClientException, LocationException {
return new ListPlaceIndexesIterable(this, applyPaginatorUserAgent(listPlaceIndexesRequest));
}
/**
*
* Lists route calculator resources in your AWS account.
*
*
* @param listRouteCalculatorsRequest
* @return Result of the ListRouteCalculators operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.ListRouteCalculators
* @see AWS
* API Documentation
*/
@Override
public ListRouteCalculatorsResponse listRouteCalculators(ListRouteCalculatorsRequest listRouteCalculatorsRequest)
throws InternalServerException, AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException,
SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListRouteCalculatorsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listRouteCalculatorsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListRouteCalculators");
String hostPrefix = "routes.";
String resolvedHostExpression = "routes.";
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListRouteCalculators").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(listRouteCalculatorsRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListRouteCalculatorsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists route calculator resources in your AWS account.
*
*
*
* This is a variant of
* {@link #listRouteCalculators(software.amazon.awssdk.services.location.model.ListRouteCalculatorsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.location.paginators.ListRouteCalculatorsIterable responses = client.listRouteCalculatorsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.location.paginators.ListRouteCalculatorsIterable responses = client
* .listRouteCalculatorsPaginator(request);
* for (software.amazon.awssdk.services.location.model.ListRouteCalculatorsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.location.paginators.ListRouteCalculatorsIterable responses = client.listRouteCalculatorsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listRouteCalculators(software.amazon.awssdk.services.location.model.ListRouteCalculatorsRequest)}
* operation.
*
*
* @param listRouteCalculatorsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.ListRouteCalculators
* @see AWS
* API Documentation
*/
@Override
public ListRouteCalculatorsIterable listRouteCalculatorsPaginator(ListRouteCalculatorsRequest listRouteCalculatorsRequest)
throws InternalServerException, AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException,
SdkClientException, LocationException {
return new ListRouteCalculatorsIterable(this, applyPaginatorUserAgent(listRouteCalculatorsRequest));
}
/**
*
* Returns a list of tags that are applied to the specified Amazon Location resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.ListTagsForResource
* @see AWS
* API Documentation
*/
@Override
public ListTagsForResourceResponse listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest)
throws InternalServerException, ResourceNotFoundException, AccessDeniedException, ValidationException,
ThrottlingException, AwsServiceException, SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListTagsForResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listTagsForResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListTagsForResource");
String hostPrefix = "metadata.";
String resolvedHostExpression = "metadata.";
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListTagsForResource").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(listTagsForResourceRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListTagsForResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists geofence collections currently associated to the given tracker resource.
*
*
* @param listTrackerConsumersRequest
* @return Result of the ListTrackerConsumers operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.ListTrackerConsumers
* @see AWS
* API Documentation
*/
@Override
public ListTrackerConsumersResponse listTrackerConsumers(ListTrackerConsumersRequest listTrackerConsumersRequest)
throws InternalServerException, ResourceNotFoundException, AccessDeniedException, ValidationException,
ThrottlingException, AwsServiceException, SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListTrackerConsumersResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listTrackerConsumersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListTrackerConsumers");
String hostPrefix = "tracking.";
String resolvedHostExpression = "tracking.";
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListTrackerConsumers").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(listTrackerConsumersRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListTrackerConsumersRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists geofence collections currently associated to the given tracker resource.
*
*
*
* This is a variant of
* {@link #listTrackerConsumers(software.amazon.awssdk.services.location.model.ListTrackerConsumersRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.location.paginators.ListTrackerConsumersIterable responses = client.listTrackerConsumersPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.location.paginators.ListTrackerConsumersIterable responses = client
* .listTrackerConsumersPaginator(request);
* for (software.amazon.awssdk.services.location.model.ListTrackerConsumersResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.location.paginators.ListTrackerConsumersIterable responses = client.listTrackerConsumersPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTrackerConsumers(software.amazon.awssdk.services.location.model.ListTrackerConsumersRequest)}
* operation.
*
*
* @param listTrackerConsumersRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.ListTrackerConsumers
* @see AWS
* API Documentation
*/
@Override
public ListTrackerConsumersIterable listTrackerConsumersPaginator(ListTrackerConsumersRequest listTrackerConsumersRequest)
throws InternalServerException, ResourceNotFoundException, AccessDeniedException, ValidationException,
ThrottlingException, AwsServiceException, SdkClientException, LocationException {
return new ListTrackerConsumersIterable(this, applyPaginatorUserAgent(listTrackerConsumersRequest));
}
/**
*
* Lists tracker resources in your AWS account.
*
*
* @param listTrackersRequest
* @return Result of the ListTrackers operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.ListTrackers
* @see AWS API
* Documentation
*/
@Override
public ListTrackersResponse listTrackers(ListTrackersRequest listTrackersRequest) throws InternalServerException,
AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException, SdkClientException,
LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListTrackersResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listTrackersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListTrackers");
String hostPrefix = "tracking.";
String resolvedHostExpression = "tracking.";
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListTrackers").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(listTrackersRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListTrackersRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists tracker resources in your AWS account.
*
*
*
* This is a variant of {@link #listTrackers(software.amazon.awssdk.services.location.model.ListTrackersRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.location.paginators.ListTrackersIterable responses = client.listTrackersPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.location.paginators.ListTrackersIterable responses = client.listTrackersPaginator(request);
* for (software.amazon.awssdk.services.location.model.ListTrackersResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.location.paginators.ListTrackersIterable responses = client.listTrackersPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTrackers(software.amazon.awssdk.services.location.model.ListTrackersRequest)} operation.
*
*
* @param listTrackersRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.ListTrackers
* @see AWS API
* Documentation
*/
@Override
public ListTrackersIterable listTrackersPaginator(ListTrackersRequest listTrackersRequest) throws InternalServerException,
AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException, SdkClientException,
LocationException {
return new ListTrackersIterable(this, applyPaginatorUserAgent(listTrackersRequest));
}
/**
*
* Stores a geofence geometry in a given geofence collection, or updates the geometry of an existing geofence if a
* geofence ID is included in the request.
*
*
* @param putGeofenceRequest
* @return Result of the PutGeofence operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws ConflictException
* The request was unsuccessful because of a conflict.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.PutGeofence
* @see AWS API
* Documentation
*/
@Override
public PutGeofenceResponse putGeofence(PutGeofenceRequest putGeofenceRequest) throws InternalServerException,
ResourceNotFoundException, ConflictException, AccessDeniedException, ValidationException, ThrottlingException,
AwsServiceException, SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
PutGeofenceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, putGeofenceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutGeofence");
String hostPrefix = "geofencing.";
String resolvedHostExpression = "geofencing.";
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("PutGeofence").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(putGeofenceRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new PutGeofenceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Reverse geocodes a given coordinate and returns a legible address. Allows you to search for Places or points of
* interest near a given position.
*
*
* @param searchPlaceIndexForPositionRequest
* @return Result of the SearchPlaceIndexForPosition operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.SearchPlaceIndexForPosition
* @see AWS API Documentation
*/
@Override
public SearchPlaceIndexForPositionResponse searchPlaceIndexForPosition(
SearchPlaceIndexForPositionRequest searchPlaceIndexForPositionRequest) throws InternalServerException,
ResourceNotFoundException, AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException,
SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, SearchPlaceIndexForPositionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, searchPlaceIndexForPositionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "SearchPlaceIndexForPosition");
String hostPrefix = "places.";
String resolvedHostExpression = "places.";
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("SearchPlaceIndexForPosition").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(searchPlaceIndexForPositionRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new SearchPlaceIndexForPositionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Generates suggestions for addresses and points of interest based on partial or misspelled free-form text. This
* operation is also known as autocomplete, autosuggest, or fuzzy matching.
*
*
* Optional parameters let you narrow your search results by bounding box or country, or bias your search toward a
* specific position on the globe.
*
*
*
* You can search for suggested place names near a specified position by using BiasPosition
, or filter
* results within a bounding box by using FilterBBox
. These parameters are mutually exclusive; using
* both BiasPosition
and FilterBBox
in the same command returns an error.
*
*
*
* @param searchPlaceIndexForSuggestionsRequest
* @return Result of the SearchPlaceIndexForSuggestions operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.SearchPlaceIndexForSuggestions
* @see AWS API Documentation
*/
@Override
public SearchPlaceIndexForSuggestionsResponse searchPlaceIndexForSuggestions(
SearchPlaceIndexForSuggestionsRequest searchPlaceIndexForSuggestionsRequest) throws InternalServerException,
ResourceNotFoundException, AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException,
SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, SearchPlaceIndexForSuggestionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
searchPlaceIndexForSuggestionsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "SearchPlaceIndexForSuggestions");
String hostPrefix = "places.";
String resolvedHostExpression = "places.";
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("SearchPlaceIndexForSuggestions").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(searchPlaceIndexForSuggestionsRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new SearchPlaceIndexForSuggestionsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Geocodes free-form text, such as an address, name, city, or region to allow you to search for Places or points of
* interest.
*
*
* Optional parameters let you narrow your search results by bounding box or country, or bias your search toward a
* specific position on the globe.
*
*
*
* You can search for places near a given position using BiasPosition
, or filter results within a
* bounding box using FilterBBox
. Providing both parameters simultaneously returns an error.
*
*
*
* Search results are returned in order of highest to lowest relevance.
*
*
* @param searchPlaceIndexForTextRequest
* @return Result of the SearchPlaceIndexForText operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.SearchPlaceIndexForText
* @see AWS API Documentation
*/
@Override
public SearchPlaceIndexForTextResponse searchPlaceIndexForText(SearchPlaceIndexForTextRequest searchPlaceIndexForTextRequest)
throws InternalServerException, ResourceNotFoundException, AccessDeniedException, ValidationException,
ThrottlingException, AwsServiceException, SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, SearchPlaceIndexForTextResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, searchPlaceIndexForTextRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "SearchPlaceIndexForText");
String hostPrefix = "places.";
String resolvedHostExpression = "places.";
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("SearchPlaceIndexForText").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(searchPlaceIndexForTextRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new SearchPlaceIndexForTextRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Assigns one or more tags (key-value pairs) to the specified Amazon Location Service resource.
*
*
*
* <p>Tags can help you organize and categorize your resources. You can also use them to scope user permissions, by granting a user permission to access or change only resources with certain tag values.</p> <p>You can use the <code>TagResource</code> operation with an Amazon Location Service resource that already has tags. If you specify a new tag key for the resource, this tag is appended to the tags already associated with the resource. If you specify a tag key that's already associated with the resource, the new tag value that you specify replaces the previous value for that tag. </p> <p>You can associate up to 50 tags with a resource.</p>
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.TagResource
* @see AWS API
* Documentation
*/
@Override
public TagResourceResponse tagResource(TagResourceRequest tagResourceRequest) throws InternalServerException,
ResourceNotFoundException, AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException,
SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
TagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, tagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "TagResource");
String hostPrefix = "metadata.";
String resolvedHostExpression = "metadata.";
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("TagResource").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(tagResourceRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new TagResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Removes one or more tags from the specified Amazon Location resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.UntagResource
* @see AWS API
* Documentation
*/
@Override
public UntagResourceResponse untagResource(UntagResourceRequest untagResourceRequest) throws InternalServerException,
ResourceNotFoundException, AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException,
SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UntagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, untagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UntagResource");
String hostPrefix = "metadata.";
String resolvedHostExpression = "metadata.";
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UntagResource").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(untagResourceRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UntagResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Updates the specified properties of a given geofence collection.
*
*
* @param updateGeofenceCollectionRequest
* @return Result of the UpdateGeofenceCollection operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.UpdateGeofenceCollection
* @see AWS API Documentation
*/
@Override
public UpdateGeofenceCollectionResponse updateGeofenceCollection(
UpdateGeofenceCollectionRequest updateGeofenceCollectionRequest) throws InternalServerException,
ResourceNotFoundException, AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException,
SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, UpdateGeofenceCollectionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateGeofenceCollectionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateGeofenceCollection");
String hostPrefix = "geofencing.";
String resolvedHostExpression = "geofencing.";
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("UpdateGeofenceCollection").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(updateGeofenceCollectionRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UpdateGeofenceCollectionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Updates the specified properties of a given map resource.
*
*
* @param updateMapRequest
* @return Result of the UpdateMap operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.UpdateMap
* @see AWS API
* Documentation
*/
@Override
public UpdateMapResponse updateMap(UpdateMapRequest updateMapRequest) throws InternalServerException,
ResourceNotFoundException, AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException,
SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UpdateMapResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateMapRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateMap");
String hostPrefix = "maps.";
String resolvedHostExpression = "maps.";
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UpdateMap").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(updateMapRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UpdateMapRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Updates the specified properties of a given place index resource.
*
*
* @param updatePlaceIndexRequest
* @return Result of the UpdatePlaceIndex operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.UpdatePlaceIndex
* @see AWS API
* Documentation
*/
@Override
public UpdatePlaceIndexResponse updatePlaceIndex(UpdatePlaceIndexRequest updatePlaceIndexRequest)
throws InternalServerException, ResourceNotFoundException, AccessDeniedException, ValidationException,
ThrottlingException, AwsServiceException, SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UpdatePlaceIndexResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updatePlaceIndexRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdatePlaceIndex");
String hostPrefix = "places.";
String resolvedHostExpression = "places.";
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UpdatePlaceIndex").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(updatePlaceIndexRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UpdatePlaceIndexRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Updates the specified properties for a given route calculator resource.
*
*
* @param updateRouteCalculatorRequest
* @return Result of the UpdateRouteCalculator operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.UpdateRouteCalculator
* @see AWS API Documentation
*/
@Override
public UpdateRouteCalculatorResponse updateRouteCalculator(UpdateRouteCalculatorRequest updateRouteCalculatorRequest)
throws InternalServerException, ResourceNotFoundException, AccessDeniedException, ValidationException,
ThrottlingException, AwsServiceException, SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, UpdateRouteCalculatorResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateRouteCalculatorRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateRouteCalculator");
String hostPrefix = "routes.";
String resolvedHostExpression = "routes.";
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UpdateRouteCalculator").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(updateRouteCalculatorRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UpdateRouteCalculatorRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Updates the specified properties of a given tracker resource.
*
*
* @param updateTrackerRequest
* @return Result of the UpdateTracker operation returned by the service.
* @throws InternalServerException
* The request has failed to process because of an unknown server error, exception, or failure.
* @throws ResourceNotFoundException
* The resource that you've entered was not found in your AWS account.
* @throws AccessDeniedException
* The request was denied because of insufficient access or permissions. Check with an administrator to
* verify your permissions.
* @throws ValidationException
* The input failed to meet the constraints specified by the AWS service.
* @throws ThrottlingException
* The request was denied because of request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LocationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LocationClient.UpdateTracker
* @see AWS API
* Documentation
*/
@Override
public UpdateTrackerResponse updateTracker(UpdateTrackerRequest updateTrackerRequest) throws InternalServerException,
ResourceNotFoundException, AccessDeniedException, ValidationException, ThrottlingException, AwsServiceException,
SdkClientException, LocationException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UpdateTrackerResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateTrackerRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Location");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateTracker");
String hostPrefix = "tracking.";
String resolvedHostExpression = "tracking.";
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UpdateTracker").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).hostPrefixExpression(resolvedHostExpression)
.withInput(updateTrackerRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UpdateTrackerRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
private static List resolveMetricPublishers(SdkClientConfiguration clientConfiguration,
RequestOverrideConfiguration requestOverrideConfiguration) {
List publishers = null;
if (requestOverrideConfiguration != null) {
publishers = requestOverrideConfiguration.metricPublishers();
}
if (publishers == null || publishers.isEmpty()) {
publishers = clientConfiguration.option(SdkClientOption.METRIC_PUBLISHERS);
}
if (publishers == null) {
publishers = Collections.emptyList();
}
return publishers;
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata) {
return protocolFactory.createErrorResponseHandler(operationMetadata);
}
private > T init(T builder) {
return builder
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(LocationException::builder)
.protocol(AwsJsonProtocol.REST_JSON)
.protocolVersion("1.1")
.registerModeledException(
ExceptionMetadata.builder().errorCode("ThrottlingException")
.exceptionBuilderSupplier(ThrottlingException::builder).httpStatusCode(429).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ServiceQuotaExceededException")
.exceptionBuilderSupplier(ServiceQuotaExceededException::builder).httpStatusCode(402).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InternalServerException")
.exceptionBuilderSupplier(InternalServerException::builder).httpStatusCode(500).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("AccessDeniedException")
.exceptionBuilderSupplier(AccessDeniedException::builder).httpStatusCode(403).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ConflictException")
.exceptionBuilderSupplier(ConflictException::builder).httpStatusCode(409).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ResourceNotFoundException")
.exceptionBuilderSupplier(ResourceNotFoundException::builder).httpStatusCode(404).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ValidationException")
.exceptionBuilderSupplier(ValidationException::builder).httpStatusCode(400).build());
}
@Override
public void close() {
clientHandler.close();
}
private T applyPaginatorUserAgent(T request) {
Consumer userAgentApplier = b -> b.addApiName(ApiName.builder()
.version(VersionInfo.SDK_VERSION).name("PAGINATED").build());
AwsRequestOverrideConfiguration overrideConfiguration = request.overrideConfiguration()
.map(c -> c.toBuilder().applyMutation(userAgentApplier).build())
.orElse((AwsRequestOverrideConfiguration.builder().applyMutation(userAgentApplier).build()));
return (T) request.toBuilder().overrideConfiguration(overrideConfiguration).build();
}
}