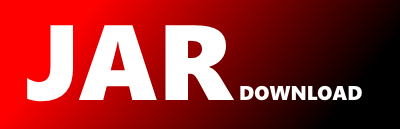
software.amazon.awssdk.services.location.model.CalculateRouteSummary Maven / Gradle / Ivy
Show all versions of location Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.location.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* A summary of the calculated route.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class CalculateRouteSummary implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField DATA_SOURCE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DataSource").getter(getter(CalculateRouteSummary::dataSource)).setter(setter(Builder::dataSource))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DataSource").build()).build();
private static final SdkField DISTANCE_FIELD = SdkField. builder(MarshallingType.DOUBLE)
.memberName("Distance").getter(getter(CalculateRouteSummary::distance)).setter(setter(Builder::distance))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Distance").build()).build();
private static final SdkField DISTANCE_UNIT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DistanceUnit").getter(getter(CalculateRouteSummary::distanceUnitAsString))
.setter(setter(Builder::distanceUnit))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DistanceUnit").build()).build();
private static final SdkField DURATION_SECONDS_FIELD = SdkField. builder(MarshallingType.DOUBLE)
.memberName("DurationSeconds").getter(getter(CalculateRouteSummary::durationSeconds))
.setter(setter(Builder::durationSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DurationSeconds").build()).build();
private static final SdkField> ROUTE_B_BOX_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("RouteBBox")
.getter(getter(CalculateRouteSummary::routeBBox))
.setter(setter(Builder::routeBBox))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RouteBBox").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.DOUBLE)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DATA_SOURCE_FIELD,
DISTANCE_FIELD, DISTANCE_UNIT_FIELD, DURATION_SECONDS_FIELD, ROUTE_B_BOX_FIELD));
private static final long serialVersionUID = 1L;
private final String dataSource;
private final Double distance;
private final String distanceUnit;
private final Double durationSeconds;
private final List routeBBox;
private CalculateRouteSummary(BuilderImpl builder) {
this.dataSource = builder.dataSource;
this.distance = builder.distance;
this.distanceUnit = builder.distanceUnit;
this.durationSeconds = builder.durationSeconds;
this.routeBBox = builder.routeBBox;
}
/**
*
* The data provider of traffic and road network data used to calculate the route. Indicates one of the available
* providers:
*
*
* -
*
* Esri
*
*
* -
*
* Grab
*
*
* -
*
* Here
*
*
*
*
* For more information about data providers, see Amazon Location
* Service data providers.
*
*
* @return The data provider of traffic and road network data used to calculate the route. Indicates one of the
* available providers:
*
* -
*
* Esri
*
*
* -
*
* Grab
*
*
* -
*
* Here
*
*
*
*
* For more information about data providers, see Amazon
* Location Service data providers.
*/
public final String dataSource() {
return dataSource;
}
/**
*
* The total distance covered by the route. The sum of the distance travelled between every stop on the route.
*
*
*
* If Esri is the data source for the route calculator, the route distance can’t be greater than 400 km. If the
* route exceeds 400 km, the response is a 400 RoutesValidationException
error.
*
*
*
* @return The total distance covered by the route. The sum of the distance travelled between every stop on the
* route.
*
* If Esri is the data source for the route calculator, the route distance can’t be greater than 400 km. If
* the route exceeds 400 km, the response is a 400 RoutesValidationException
error.
*
*/
public final Double distance() {
return distance;
}
/**
*
* The unit of measurement for route distances.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #distanceUnit} will
* return {@link DistanceUnit#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #distanceUnitAsString}.
*
*
* @return The unit of measurement for route distances.
* @see DistanceUnit
*/
public final DistanceUnit distanceUnit() {
return DistanceUnit.fromValue(distanceUnit);
}
/**
*
* The unit of measurement for route distances.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #distanceUnit} will
* return {@link DistanceUnit#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #distanceUnitAsString}.
*
*
* @return The unit of measurement for route distances.
* @see DistanceUnit
*/
public final String distanceUnitAsString() {
return distanceUnit;
}
/**
*
* The total travel time for the route measured in seconds. The sum of the travel time between every stop on the
* route.
*
*
* @return The total travel time for the route measured in seconds. The sum of the travel time between every stop on
* the route.
*/
public final Double durationSeconds() {
return durationSeconds;
}
/**
* For responses, this returns true if the service returned a value for the RouteBBox property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasRouteBBox() {
return routeBBox != null && !(routeBBox instanceof SdkAutoConstructList);
}
/**
*
* Specifies a geographical box surrounding a route. Used to zoom into a route when displaying it in a map. For
* example, [min x, min y, max x, max y]
.
*
*
* The first 2 bbox
parameters describe the lower southwest corner:
*
*
* -
*
* The first bbox
position is the X coordinate or longitude of the lower southwest corner.
*
*
* -
*
* The second bbox
position is the Y coordinate or latitude of the lower southwest corner.
*
*
*
*
* The next 2 bbox
parameters describe the upper northeast corner:
*
*
* -
*
* The third bbox
position is the X coordinate, or longitude of the upper northeast corner.
*
*
* -
*
* The fourth bbox
position is the Y coordinate, or latitude of the upper northeast corner.
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasRouteBBox} method.
*
*
* @return Specifies a geographical box surrounding a route. Used to zoom into a route when displaying it in a map.
* For example, [min x, min y, max x, max y]
.
*
* The first 2 bbox
parameters describe the lower southwest corner:
*
*
* -
*
* The first bbox
position is the X coordinate or longitude of the lower southwest corner.
*
*
* -
*
* The second bbox
position is the Y coordinate or latitude of the lower southwest corner.
*
*
*
*
* The next 2 bbox
parameters describe the upper northeast corner:
*
*
* -
*
* The third bbox
position is the X coordinate, or longitude of the upper northeast corner.
*
*
* -
*
* The fourth bbox
position is the Y coordinate, or latitude of the upper northeast corner.
*
*
*/
public final List routeBBox() {
return routeBBox;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(dataSource());
hashCode = 31 * hashCode + Objects.hashCode(distance());
hashCode = 31 * hashCode + Objects.hashCode(distanceUnitAsString());
hashCode = 31 * hashCode + Objects.hashCode(durationSeconds());
hashCode = 31 * hashCode + Objects.hashCode(hasRouteBBox() ? routeBBox() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CalculateRouteSummary)) {
return false;
}
CalculateRouteSummary other = (CalculateRouteSummary) obj;
return Objects.equals(dataSource(), other.dataSource()) && Objects.equals(distance(), other.distance())
&& Objects.equals(distanceUnitAsString(), other.distanceUnitAsString())
&& Objects.equals(durationSeconds(), other.durationSeconds()) && hasRouteBBox() == other.hasRouteBBox()
&& Objects.equals(routeBBox(), other.routeBBox());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CalculateRouteSummary").add("DataSource", dataSource()).add("Distance", distance())
.add("DistanceUnit", distanceUnitAsString()).add("DurationSeconds", durationSeconds())
.add("RouteBBox", routeBBox() == null ? null : "*** Sensitive Data Redacted ***").build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "DataSource":
return Optional.ofNullable(clazz.cast(dataSource()));
case "Distance":
return Optional.ofNullable(clazz.cast(distance()));
case "DistanceUnit":
return Optional.ofNullable(clazz.cast(distanceUnitAsString()));
case "DurationSeconds":
return Optional.ofNullable(clazz.cast(durationSeconds()));
case "RouteBBox":
return Optional.ofNullable(clazz.cast(routeBBox()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function