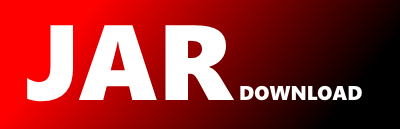
software.amazon.awssdk.services.lookoutvision.LookoutVisionClient Maven / Gradle / Ivy
Show all versions of lookoutvision Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.lookoutvision;
import java.nio.file.Path;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.sync.RequestBody;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.lookoutvision.model.AccessDeniedException;
import software.amazon.awssdk.services.lookoutvision.model.ConflictException;
import software.amazon.awssdk.services.lookoutvision.model.CreateDatasetRequest;
import software.amazon.awssdk.services.lookoutvision.model.CreateDatasetResponse;
import software.amazon.awssdk.services.lookoutvision.model.CreateModelRequest;
import software.amazon.awssdk.services.lookoutvision.model.CreateModelResponse;
import software.amazon.awssdk.services.lookoutvision.model.CreateProjectRequest;
import software.amazon.awssdk.services.lookoutvision.model.CreateProjectResponse;
import software.amazon.awssdk.services.lookoutvision.model.DeleteDatasetRequest;
import software.amazon.awssdk.services.lookoutvision.model.DeleteDatasetResponse;
import software.amazon.awssdk.services.lookoutvision.model.DeleteModelRequest;
import software.amazon.awssdk.services.lookoutvision.model.DeleteModelResponse;
import software.amazon.awssdk.services.lookoutvision.model.DeleteProjectRequest;
import software.amazon.awssdk.services.lookoutvision.model.DeleteProjectResponse;
import software.amazon.awssdk.services.lookoutvision.model.DescribeDatasetRequest;
import software.amazon.awssdk.services.lookoutvision.model.DescribeDatasetResponse;
import software.amazon.awssdk.services.lookoutvision.model.DescribeModelRequest;
import software.amazon.awssdk.services.lookoutvision.model.DescribeModelResponse;
import software.amazon.awssdk.services.lookoutvision.model.DescribeProjectRequest;
import software.amazon.awssdk.services.lookoutvision.model.DescribeProjectResponse;
import software.amazon.awssdk.services.lookoutvision.model.DetectAnomaliesRequest;
import software.amazon.awssdk.services.lookoutvision.model.DetectAnomaliesResponse;
import software.amazon.awssdk.services.lookoutvision.model.InternalServerException;
import software.amazon.awssdk.services.lookoutvision.model.ListDatasetEntriesRequest;
import software.amazon.awssdk.services.lookoutvision.model.ListDatasetEntriesResponse;
import software.amazon.awssdk.services.lookoutvision.model.ListModelsRequest;
import software.amazon.awssdk.services.lookoutvision.model.ListModelsResponse;
import software.amazon.awssdk.services.lookoutvision.model.ListProjectsRequest;
import software.amazon.awssdk.services.lookoutvision.model.ListProjectsResponse;
import software.amazon.awssdk.services.lookoutvision.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.lookoutvision.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.lookoutvision.model.LookoutVisionException;
import software.amazon.awssdk.services.lookoutvision.model.ResourceNotFoundException;
import software.amazon.awssdk.services.lookoutvision.model.ServiceQuotaExceededException;
import software.amazon.awssdk.services.lookoutvision.model.StartModelRequest;
import software.amazon.awssdk.services.lookoutvision.model.StartModelResponse;
import software.amazon.awssdk.services.lookoutvision.model.StopModelRequest;
import software.amazon.awssdk.services.lookoutvision.model.StopModelResponse;
import software.amazon.awssdk.services.lookoutvision.model.TagResourceRequest;
import software.amazon.awssdk.services.lookoutvision.model.TagResourceResponse;
import software.amazon.awssdk.services.lookoutvision.model.ThrottlingException;
import software.amazon.awssdk.services.lookoutvision.model.UntagResourceRequest;
import software.amazon.awssdk.services.lookoutvision.model.UntagResourceResponse;
import software.amazon.awssdk.services.lookoutvision.model.UpdateDatasetEntriesRequest;
import software.amazon.awssdk.services.lookoutvision.model.UpdateDatasetEntriesResponse;
import software.amazon.awssdk.services.lookoutvision.model.ValidationException;
import software.amazon.awssdk.services.lookoutvision.paginators.ListDatasetEntriesIterable;
import software.amazon.awssdk.services.lookoutvision.paginators.ListModelsIterable;
import software.amazon.awssdk.services.lookoutvision.paginators.ListProjectsIterable;
/**
* Service client for accessing Amazon Lookout for Vision. This can be created using the static {@link #builder()}
* method.
*
*
* This is the Amazon Lookout for Vision API Reference. It provides descriptions of actions, data types, common
* parameters, and common errors.
*
*
* Amazon Lookout for Vision enables you to find visual defects in industrial products, accurately and at scale. It uses
* computer vision to identify missing components in an industrial product, damage to vehicles or structures,
* irregularities in production lines, and even minuscule defects in silicon wafers — or any other physical item where
* quality is important such as a missing capacitor on printed circuit boards.
*
*/
@Generated("software.amazon.awssdk:codegen")
public interface LookoutVisionClient extends SdkClient {
String SERVICE_NAME = "lookoutvision";
/**
* Create a {@link LookoutVisionClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static LookoutVisionClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link LookoutVisionClient}.
*/
static LookoutVisionClientBuilder builder() {
return new DefaultLookoutVisionClientBuilder();
}
/**
*
* Creates a new dataset in an Amazon Lookout for Vision project. CreateDataset
can create a training
* or a test dataset from a valid dataset source (DatasetSource
).
*
*
* If you want a single dataset project, specify train
for the value of DatasetType
.
*
*
* To have a project with separate training and test datasets, call CreateDataset
twice. On the first
* call, specify train
for the value of DatasetType
. On the second call, specify
* test
for the value of DatasetType
.
*
*
* This operation requires permissions to perform the lookoutvision:CreateDataset
operation.
*
*
* @param createDatasetRequest
* @return Result of the CreateDataset operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws ServiceQuotaExceededException
* A service quota was exceeded the allowed limit. For more information, see Limits in Amazon Lookout for
* Vision in the Amazon Lookout for Vision Developer Guide.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.CreateDataset
* @see AWS
* API Documentation
*/
default CreateDatasetResponse createDataset(CreateDatasetRequest createDatasetRequest) throws AccessDeniedException,
InternalServerException, ValidationException, ConflictException, ResourceNotFoundException, ThrottlingException,
ServiceQuotaExceededException, AwsServiceException, SdkClientException, LookoutVisionException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new dataset in an Amazon Lookout for Vision project. CreateDataset
can create a training
* or a test dataset from a valid dataset source (DatasetSource
).
*
*
* If you want a single dataset project, specify train
for the value of DatasetType
.
*
*
* To have a project with separate training and test datasets, call CreateDataset
twice. On the first
* call, specify train
for the value of DatasetType
. On the second call, specify
* test
for the value of DatasetType
.
*
*
* This operation requires permissions to perform the lookoutvision:CreateDataset
operation.
*
*
*
* This is a convenience which creates an instance of the {@link CreateDatasetRequest.Builder} avoiding the need to
* create one manually via {@link CreateDatasetRequest#builder()}
*
*
* @param createDatasetRequest
* A {@link Consumer} that will call methods on {@link CreateDatasetRequest.Builder} to create a request.
* @return Result of the CreateDataset operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws ServiceQuotaExceededException
* A service quota was exceeded the allowed limit. For more information, see Limits in Amazon Lookout for
* Vision in the Amazon Lookout for Vision Developer Guide.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.CreateDataset
* @see AWS
* API Documentation
*/
default CreateDatasetResponse createDataset(Consumer createDatasetRequest)
throws AccessDeniedException, InternalServerException, ValidationException, ConflictException,
ResourceNotFoundException, ThrottlingException, ServiceQuotaExceededException, AwsServiceException,
SdkClientException, LookoutVisionException {
return createDataset(CreateDatasetRequest.builder().applyMutation(createDatasetRequest).build());
}
/**
*
* Creates a new version of a model within an an Amazon Lookout for Vision project. CreateModel
is an
* asynchronous operation in which Amazon Lookout for Vision trains, tests, and evaluates a new version of a model.
*
*
* To get the current status, check the Status
field returned in the response from
* DescribeModel.
*
*
* If the project has a single dataset, Amazon Lookout for Vision internally splits the dataset to create a training
* and a test dataset. If the project has a training and a test dataset, Lookout for Vision uses the respective
* datasets to train and test the model.
*
*
* After training completes, the evaluation metrics are stored at the location specified in
* OutputConfig
.
*
*
* This operation requires permissions to perform the lookoutvision:CreateModel
operation. If you want
* to tag your model, you also require permission to the lookoutvision:TagResource
operation.
*
*
* @param createModelRequest
* @return Result of the CreateModel operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws ServiceQuotaExceededException
* A service quota was exceeded the allowed limit. For more information, see Limits in Amazon Lookout for
* Vision in the Amazon Lookout for Vision Developer Guide.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.CreateModel
* @see AWS API
* Documentation
*/
default CreateModelResponse createModel(CreateModelRequest createModelRequest) throws AccessDeniedException,
InternalServerException, ValidationException, ConflictException, ResourceNotFoundException, ThrottlingException,
ServiceQuotaExceededException, AwsServiceException, SdkClientException, LookoutVisionException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new version of a model within an an Amazon Lookout for Vision project. CreateModel
is an
* asynchronous operation in which Amazon Lookout for Vision trains, tests, and evaluates a new version of a model.
*
*
* To get the current status, check the Status
field returned in the response from
* DescribeModel.
*
*
* If the project has a single dataset, Amazon Lookout for Vision internally splits the dataset to create a training
* and a test dataset. If the project has a training and a test dataset, Lookout for Vision uses the respective
* datasets to train and test the model.
*
*
* After training completes, the evaluation metrics are stored at the location specified in
* OutputConfig
.
*
*
* This operation requires permissions to perform the lookoutvision:CreateModel
operation. If you want
* to tag your model, you also require permission to the lookoutvision:TagResource
operation.
*
*
*
* This is a convenience which creates an instance of the {@link CreateModelRequest.Builder} avoiding the need to
* create one manually via {@link CreateModelRequest#builder()}
*
*
* @param createModelRequest
* A {@link Consumer} that will call methods on {@link CreateModelRequest.Builder} to create a request.
* @return Result of the CreateModel operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws ServiceQuotaExceededException
* A service quota was exceeded the allowed limit. For more information, see Limits in Amazon Lookout for
* Vision in the Amazon Lookout for Vision Developer Guide.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.CreateModel
* @see AWS API
* Documentation
*/
default CreateModelResponse createModel(Consumer createModelRequest)
throws AccessDeniedException, InternalServerException, ValidationException, ConflictException,
ResourceNotFoundException, ThrottlingException, ServiceQuotaExceededException, AwsServiceException,
SdkClientException, LookoutVisionException {
return createModel(CreateModelRequest.builder().applyMutation(createModelRequest).build());
}
/**
*
* Creates an empty Amazon Lookout for Vision project. After you create the project, add a dataset by calling
* CreateDataset.
*
*
* This operation requires permissions to perform the lookoutvision:CreateProject
operation.
*
*
* @param createProjectRequest
* @return Result of the CreateProject operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws ServiceQuotaExceededException
* A service quota was exceeded the allowed limit. For more information, see Limits in Amazon Lookout for
* Vision in the Amazon Lookout for Vision Developer Guide.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.CreateProject
* @see AWS
* API Documentation
*/
default CreateProjectResponse createProject(CreateProjectRequest createProjectRequest) throws AccessDeniedException,
InternalServerException, ValidationException, ConflictException, ResourceNotFoundException, ThrottlingException,
ServiceQuotaExceededException, AwsServiceException, SdkClientException, LookoutVisionException {
throw new UnsupportedOperationException();
}
/**
*
* Creates an empty Amazon Lookout for Vision project. After you create the project, add a dataset by calling
* CreateDataset.
*
*
* This operation requires permissions to perform the lookoutvision:CreateProject
operation.
*
*
*
* This is a convenience which creates an instance of the {@link CreateProjectRequest.Builder} avoiding the need to
* create one manually via {@link CreateProjectRequest#builder()}
*
*
* @param createProjectRequest
* A {@link Consumer} that will call methods on {@link CreateProjectRequest.Builder} to create a request.
* @return Result of the CreateProject operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws ServiceQuotaExceededException
* A service quota was exceeded the allowed limit. For more information, see Limits in Amazon Lookout for
* Vision in the Amazon Lookout for Vision Developer Guide.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.CreateProject
* @see AWS
* API Documentation
*/
default CreateProjectResponse createProject(Consumer createProjectRequest)
throws AccessDeniedException, InternalServerException, ValidationException, ConflictException,
ResourceNotFoundException, ThrottlingException, ServiceQuotaExceededException, AwsServiceException,
SdkClientException, LookoutVisionException {
return createProject(CreateProjectRequest.builder().applyMutation(createProjectRequest).build());
}
/**
*
* Deletes an existing Amazon Lookout for Vision dataset
.
*
*
* If your the project has a single dataset, you must create a new dataset before you can create a model.
*
*
* If you project has a training dataset and a test dataset consider the following.
*
*
* -
*
* If you delete the test dataset, your project reverts to a single dataset project. If you then train the model,
* Amazon Lookout for Vision internally splits the remaining dataset into a training and test dataset.
*
*
* -
*
* If you delete the training dataset, you must create a training dataset before you can create a model.
*
*
*
*
* This operation requires permissions to perform the lookoutvision:DeleteDataset
operation.
*
*
* @param deleteDatasetRequest
* @return Result of the DeleteDataset operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.DeleteDataset
* @see AWS
* API Documentation
*/
default DeleteDatasetResponse deleteDataset(DeleteDatasetRequest deleteDatasetRequest) throws AccessDeniedException,
InternalServerException, ValidationException, ConflictException, ResourceNotFoundException, ThrottlingException,
AwsServiceException, SdkClientException, LookoutVisionException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an existing Amazon Lookout for Vision dataset
.
*
*
* If your the project has a single dataset, you must create a new dataset before you can create a model.
*
*
* If you project has a training dataset and a test dataset consider the following.
*
*
* -
*
* If you delete the test dataset, your project reverts to a single dataset project. If you then train the model,
* Amazon Lookout for Vision internally splits the remaining dataset into a training and test dataset.
*
*
* -
*
* If you delete the training dataset, you must create a training dataset before you can create a model.
*
*
*
*
* This operation requires permissions to perform the lookoutvision:DeleteDataset
operation.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteDatasetRequest.Builder} avoiding the need to
* create one manually via {@link DeleteDatasetRequest#builder()}
*
*
* @param deleteDatasetRequest
* A {@link Consumer} that will call methods on {@link DeleteDatasetRequest.Builder} to create a request.
* @return Result of the DeleteDataset operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.DeleteDataset
* @see AWS
* API Documentation
*/
default DeleteDatasetResponse deleteDataset(Consumer deleteDatasetRequest)
throws AccessDeniedException, InternalServerException, ValidationException, ConflictException,
ResourceNotFoundException, ThrottlingException, AwsServiceException, SdkClientException, LookoutVisionException {
return deleteDataset(DeleteDatasetRequest.builder().applyMutation(deleteDatasetRequest).build());
}
/**
*
* Deletes an Amazon Lookout for Vision model. You can't delete a running model. To stop a running model, use the
* StopModel operation.
*
*
* It might take a few seconds to delete a model. To determine if a model has been deleted, call ListProjects
* and check if the version of the model (ModelVersion
) is in the Models
array.
*
*
* This operation requires permissions to perform the lookoutvision:DeleteModel
operation.
*
*
* @param deleteModelRequest
* @return Result of the DeleteModel operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.DeleteModel
* @see AWS API
* Documentation
*/
default DeleteModelResponse deleteModel(DeleteModelRequest deleteModelRequest) throws AccessDeniedException,
InternalServerException, ValidationException, ConflictException, ResourceNotFoundException, ThrottlingException,
AwsServiceException, SdkClientException, LookoutVisionException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an Amazon Lookout for Vision model. You can't delete a running model. To stop a running model, use the
* StopModel operation.
*
*
* It might take a few seconds to delete a model. To determine if a model has been deleted, call ListProjects
* and check if the version of the model (ModelVersion
) is in the Models
array.
*
*
* This operation requires permissions to perform the lookoutvision:DeleteModel
operation.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteModelRequest.Builder} avoiding the need to
* create one manually via {@link DeleteModelRequest#builder()}
*
*
* @param deleteModelRequest
* A {@link Consumer} that will call methods on {@link DeleteModelRequest.Builder} to create a request.
* @return Result of the DeleteModel operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.DeleteModel
* @see AWS API
* Documentation
*/
default DeleteModelResponse deleteModel(Consumer deleteModelRequest)
throws AccessDeniedException, InternalServerException, ValidationException, ConflictException,
ResourceNotFoundException, ThrottlingException, AwsServiceException, SdkClientException, LookoutVisionException {
return deleteModel(DeleteModelRequest.builder().applyMutation(deleteModelRequest).build());
}
/**
*
* Deletes an Amazon Lookout for Vision project.
*
*
* To delete a project, you must first delete each version of the model associated with the project. To delete a
* model use the DeleteModel operation.
*
*
* You also have to delete the dataset(s) associated with the model. For more information, see DeleteDataset.
* The images referenced by the training and test datasets aren't deleted.
*
*
* This operation requires permissions to perform the lookoutvision:DeleteProject
operation.
*
*
* @param deleteProjectRequest
* @return Result of the DeleteProject operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.DeleteProject
* @see AWS
* API Documentation
*/
default DeleteProjectResponse deleteProject(DeleteProjectRequest deleteProjectRequest) throws AccessDeniedException,
InternalServerException, ValidationException, ConflictException, ResourceNotFoundException, ThrottlingException,
AwsServiceException, SdkClientException, LookoutVisionException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an Amazon Lookout for Vision project.
*
*
* To delete a project, you must first delete each version of the model associated with the project. To delete a
* model use the DeleteModel operation.
*
*
* You also have to delete the dataset(s) associated with the model. For more information, see DeleteDataset.
* The images referenced by the training and test datasets aren't deleted.
*
*
* This operation requires permissions to perform the lookoutvision:DeleteProject
operation.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteProjectRequest.Builder} avoiding the need to
* create one manually via {@link DeleteProjectRequest#builder()}
*
*
* @param deleteProjectRequest
* A {@link Consumer} that will call methods on {@link DeleteProjectRequest.Builder} to create a request.
* @return Result of the DeleteProject operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.DeleteProject
* @see AWS
* API Documentation
*/
default DeleteProjectResponse deleteProject(Consumer deleteProjectRequest)
throws AccessDeniedException, InternalServerException, ValidationException, ConflictException,
ResourceNotFoundException, ThrottlingException, AwsServiceException, SdkClientException, LookoutVisionException {
return deleteProject(DeleteProjectRequest.builder().applyMutation(deleteProjectRequest).build());
}
/**
*
* Describe an Amazon Lookout for Vision dataset.
*
*
* This operation requires permissions to perform the lookoutvision:DescribeDataset
operation.
*
*
* @param describeDatasetRequest
* @return Result of the DescribeDataset operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.DescribeDataset
* @see AWS
* API Documentation
*/
default DescribeDatasetResponse describeDataset(DescribeDatasetRequest describeDatasetRequest) throws AccessDeniedException,
InternalServerException, ValidationException, ConflictException, ResourceNotFoundException, ThrottlingException,
AwsServiceException, SdkClientException, LookoutVisionException {
throw new UnsupportedOperationException();
}
/**
*
* Describe an Amazon Lookout for Vision dataset.
*
*
* This operation requires permissions to perform the lookoutvision:DescribeDataset
operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeDatasetRequest.Builder} avoiding the need
* to create one manually via {@link DescribeDatasetRequest#builder()}
*
*
* @param describeDatasetRequest
* A {@link Consumer} that will call methods on {@link DescribeDatasetRequest.Builder} to create a request.
* @return Result of the DescribeDataset operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.DescribeDataset
* @see AWS
* API Documentation
*/
default DescribeDatasetResponse describeDataset(Consumer describeDatasetRequest)
throws AccessDeniedException, InternalServerException, ValidationException, ConflictException,
ResourceNotFoundException, ThrottlingException, AwsServiceException, SdkClientException, LookoutVisionException {
return describeDataset(DescribeDatasetRequest.builder().applyMutation(describeDatasetRequest).build());
}
/**
*
* Describes a version of an Amazon Lookout for Vision model.
*
*
* This operation requires permissions to perform the lookoutvision:DescribeModel
operation.
*
*
* @param describeModelRequest
* @return Result of the DescribeModel operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.DescribeModel
* @see AWS
* API Documentation
*/
default DescribeModelResponse describeModel(DescribeModelRequest describeModelRequest) throws AccessDeniedException,
InternalServerException, ValidationException, ConflictException, ResourceNotFoundException, ThrottlingException,
AwsServiceException, SdkClientException, LookoutVisionException {
throw new UnsupportedOperationException();
}
/**
*
* Describes a version of an Amazon Lookout for Vision model.
*
*
* This operation requires permissions to perform the lookoutvision:DescribeModel
operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeModelRequest.Builder} avoiding the need to
* create one manually via {@link DescribeModelRequest#builder()}
*
*
* @param describeModelRequest
* A {@link Consumer} that will call methods on {@link DescribeModelRequest.Builder} to create a request.
* @return Result of the DescribeModel operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.DescribeModel
* @see AWS
* API Documentation
*/
default DescribeModelResponse describeModel(Consumer describeModelRequest)
throws AccessDeniedException, InternalServerException, ValidationException, ConflictException,
ResourceNotFoundException, ThrottlingException, AwsServiceException, SdkClientException, LookoutVisionException {
return describeModel(DescribeModelRequest.builder().applyMutation(describeModelRequest).build());
}
/**
*
* Describes an Amazon Lookout for Vision project.
*
*
* This operation requires permissions to perform the lookoutvision:DescribeProject
operation.
*
*
* @param describeProjectRequest
* @return Result of the DescribeProject operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.DescribeProject
* @see AWS
* API Documentation
*/
default DescribeProjectResponse describeProject(DescribeProjectRequest describeProjectRequest) throws AccessDeniedException,
InternalServerException, ValidationException, ConflictException, ResourceNotFoundException, ThrottlingException,
AwsServiceException, SdkClientException, LookoutVisionException {
throw new UnsupportedOperationException();
}
/**
*
* Describes an Amazon Lookout for Vision project.
*
*
* This operation requires permissions to perform the lookoutvision:DescribeProject
operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeProjectRequest.Builder} avoiding the need
* to create one manually via {@link DescribeProjectRequest#builder()}
*
*
* @param describeProjectRequest
* A {@link Consumer} that will call methods on {@link DescribeProjectRequest.Builder} to create a request.
* @return Result of the DescribeProject operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.DescribeProject
* @see AWS
* API Documentation
*/
default DescribeProjectResponse describeProject(Consumer describeProjectRequest)
throws AccessDeniedException, InternalServerException, ValidationException, ConflictException,
ResourceNotFoundException, ThrottlingException, AwsServiceException, SdkClientException, LookoutVisionException {
return describeProject(DescribeProjectRequest.builder().applyMutation(describeProjectRequest).build());
}
/**
*
* Detects anomalies in an image that you supply.
*
*
* The response from DetectAnomalies
includes a boolean prediction that the image contains one or more
* anomalies and a confidence value for the prediction.
*
*
*
* Before calling DetectAnomalies
, you must first start your model with the StartModel
* operation. You are charged for the amount of time, in minutes, that a model runs and for the number of anomaly
* detection units that your model uses. If you are not using a model, use the StopModel operation to stop
* your model.
*
*
*
* This operation requires permissions to perform the lookoutvision:DetectAnomalies
operation.
*
*
* @param detectAnomaliesRequest
* @param requestBody
* The content to send to the service. A {@link RequestBody} can be created using one of several factory
* methods for various sources of data. For example, to create a request body from a file you can do the
* following.
*
*
* {@code RequestBody.fromFile(new File("myfile.txt"))}
*
*
* See documentation in {@link RequestBody} for additional details and which sources of data are supported.
* The service documentation for the request content is as follows '
*
* The unencrypted image bytes that you want to analyze.
*
* '
* @return Result of the DetectAnomalies operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.DetectAnomalies
* @see AWS
* API Documentation
*/
default DetectAnomaliesResponse detectAnomalies(DetectAnomaliesRequest detectAnomaliesRequest, RequestBody requestBody)
throws AccessDeniedException, InternalServerException, ValidationException, ConflictException,
ResourceNotFoundException, ThrottlingException, AwsServiceException, SdkClientException, LookoutVisionException {
throw new UnsupportedOperationException();
}
/**
*
* Detects anomalies in an image that you supply.
*
*
* The response from DetectAnomalies
includes a boolean prediction that the image contains one or more
* anomalies and a confidence value for the prediction.
*
*
*
* Before calling DetectAnomalies
, you must first start your model with the StartModel
* operation. You are charged for the amount of time, in minutes, that a model runs and for the number of anomaly
* detection units that your model uses. If you are not using a model, use the StopModel operation to stop
* your model.
*
*
*
* This operation requires permissions to perform the lookoutvision:DetectAnomalies
operation.
*
*
*
* This is a convenience which creates an instance of the {@link DetectAnomaliesRequest.Builder} avoiding the need
* to create one manually via {@link DetectAnomaliesRequest#builder()}
*
*
* @param detectAnomaliesRequest
* A {@link Consumer} that will call methods on {@link DetectAnomaliesRequest.Builder} to create a request.
* @param requestBody
* The content to send to the service. A {@link RequestBody} can be created using one of several factory
* methods for various sources of data. For example, to create a request body from a file you can do the
* following.
*
*
* {@code RequestBody.fromFile(new File("myfile.txt"))}
*
*
* See documentation in {@link RequestBody} for additional details and which sources of data are supported.
* The service documentation for the request content is as follows '
*
* The unencrypted image bytes that you want to analyze.
*
* '
* @return Result of the DetectAnomalies operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.DetectAnomalies
* @see AWS
* API Documentation
*/
default DetectAnomaliesResponse detectAnomalies(Consumer detectAnomaliesRequest,
RequestBody requestBody) throws AccessDeniedException, InternalServerException, ValidationException,
ConflictException, ResourceNotFoundException, ThrottlingException, AwsServiceException, SdkClientException,
LookoutVisionException {
return detectAnomalies(DetectAnomaliesRequest.builder().applyMutation(detectAnomaliesRequest).build(), requestBody);
}
/**
*
* Detects anomalies in an image that you supply.
*
*
* The response from DetectAnomalies
includes a boolean prediction that the image contains one or more
* anomalies and a confidence value for the prediction.
*
*
*
* Before calling DetectAnomalies
, you must first start your model with the StartModel
* operation. You are charged for the amount of time, in minutes, that a model runs and for the number of anomaly
* detection units that your model uses. If you are not using a model, use the StopModel operation to stop
* your model.
*
*
*
* This operation requires permissions to perform the lookoutvision:DetectAnomalies
operation.
*
*
* @param detectAnomaliesRequest
* @param sourcePath
* {@link Path} to file containing data to send to the service. File will be read entirely and may be read
* multiple times in the event of a retry. If the file does not exist or the current user does not have
* access to read it then an exception will be thrown. The service documentation for the request content is
* as follows '
*
* The unencrypted image bytes that you want to analyze.
*
* '
* @return Result of the DetectAnomalies operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.DetectAnomalies
* @see #detectAnomalies(DetectAnomaliesRequest, RequestBody)
* @see AWS
* API Documentation
*/
default DetectAnomaliesResponse detectAnomalies(DetectAnomaliesRequest detectAnomaliesRequest, Path sourcePath)
throws AccessDeniedException, InternalServerException, ValidationException, ConflictException,
ResourceNotFoundException, ThrottlingException, AwsServiceException, SdkClientException, LookoutVisionException {
return detectAnomalies(detectAnomaliesRequest, RequestBody.fromFile(sourcePath));
}
/**
*
* Detects anomalies in an image that you supply.
*
*
* The response from DetectAnomalies
includes a boolean prediction that the image contains one or more
* anomalies and a confidence value for the prediction.
*
*
*
* Before calling DetectAnomalies
, you must first start your model with the StartModel
* operation. You are charged for the amount of time, in minutes, that a model runs and for the number of anomaly
* detection units that your model uses. If you are not using a model, use the StopModel operation to stop
* your model.
*
*
*
* This operation requires permissions to perform the lookoutvision:DetectAnomalies
operation.
*
*
*
* This is a convenience which creates an instance of the {@link DetectAnomaliesRequest.Builder} avoiding the need
* to create one manually via {@link DetectAnomaliesRequest#builder()}
*
*
* @param detectAnomaliesRequest
* A {@link Consumer} that will call methods on {@link DetectAnomaliesRequest.Builder} to create a request.
* @param sourcePath
* {@link Path} to file containing data to send to the service. File will be read entirely and may be read
* multiple times in the event of a retry. If the file does not exist or the current user does not have
* access to read it then an exception will be thrown. The service documentation for the request content is
* as follows '
*
* The unencrypted image bytes that you want to analyze.
*
* '
* @return Result of the DetectAnomalies operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.DetectAnomalies
* @see #detectAnomalies(DetectAnomaliesRequest, RequestBody)
* @see AWS
* API Documentation
*/
default DetectAnomaliesResponse detectAnomalies(Consumer detectAnomaliesRequest,
Path sourcePath) throws AccessDeniedException, InternalServerException, ValidationException, ConflictException,
ResourceNotFoundException, ThrottlingException, AwsServiceException, SdkClientException, LookoutVisionException {
return detectAnomalies(DetectAnomaliesRequest.builder().applyMutation(detectAnomaliesRequest).build(), sourcePath);
}
/**
*
* Lists the JSON Lines within a dataset. An Amazon Lookout for Vision JSON Line contains the anomaly information
* for a single image, including the image location and the assigned label.
*
*
* This operation requires permissions to perform the lookoutvision:ListDatasetEntries
operation.
*
*
* @param listDatasetEntriesRequest
* @return Result of the ListDatasetEntries operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.ListDatasetEntries
* @see AWS API Documentation
*/
default ListDatasetEntriesResponse listDatasetEntries(ListDatasetEntriesRequest listDatasetEntriesRequest)
throws AccessDeniedException, InternalServerException, ValidationException, ConflictException,
ResourceNotFoundException, ThrottlingException, AwsServiceException, SdkClientException, LookoutVisionException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the JSON Lines within a dataset. An Amazon Lookout for Vision JSON Line contains the anomaly information
* for a single image, including the image location and the assigned label.
*
*
* This operation requires permissions to perform the lookoutvision:ListDatasetEntries
operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListDatasetEntriesRequest.Builder} avoiding the
* need to create one manually via {@link ListDatasetEntriesRequest#builder()}
*
*
* @param listDatasetEntriesRequest
* A {@link Consumer} that will call methods on {@link ListDatasetEntriesRequest.Builder} to create a
* request.
* @return Result of the ListDatasetEntries operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.ListDatasetEntries
* @see AWS API Documentation
*/
default ListDatasetEntriesResponse listDatasetEntries(Consumer listDatasetEntriesRequest)
throws AccessDeniedException, InternalServerException, ValidationException, ConflictException,
ResourceNotFoundException, ThrottlingException, AwsServiceException, SdkClientException, LookoutVisionException {
return listDatasetEntries(ListDatasetEntriesRequest.builder().applyMutation(listDatasetEntriesRequest).build());
}
/**
*
* Lists the JSON Lines within a dataset. An Amazon Lookout for Vision JSON Line contains the anomaly information
* for a single image, including the image location and the assigned label.
*
*
* This operation requires permissions to perform the lookoutvision:ListDatasetEntries
operation.
*
*
*
* This is a variant of
* {@link #listDatasetEntries(software.amazon.awssdk.services.lookoutvision.model.ListDatasetEntriesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.lookoutvision.paginators.ListDatasetEntriesIterable responses = client.listDatasetEntriesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.lookoutvision.paginators.ListDatasetEntriesIterable responses = client
* .listDatasetEntriesPaginator(request);
* for (software.amazon.awssdk.services.lookoutvision.model.ListDatasetEntriesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.lookoutvision.paginators.ListDatasetEntriesIterable responses = client.listDatasetEntriesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDatasetEntries(software.amazon.awssdk.services.lookoutvision.model.ListDatasetEntriesRequest)}
* operation.
*
*
* @param listDatasetEntriesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.ListDatasetEntries
* @see AWS API Documentation
*/
default ListDatasetEntriesIterable listDatasetEntriesPaginator(ListDatasetEntriesRequest listDatasetEntriesRequest)
throws AccessDeniedException, InternalServerException, ValidationException, ConflictException,
ResourceNotFoundException, ThrottlingException, AwsServiceException, SdkClientException, LookoutVisionException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the JSON Lines within a dataset. An Amazon Lookout for Vision JSON Line contains the anomaly information
* for a single image, including the image location and the assigned label.
*
*
* This operation requires permissions to perform the lookoutvision:ListDatasetEntries
operation.
*
*
*
* This is a variant of
* {@link #listDatasetEntries(software.amazon.awssdk.services.lookoutvision.model.ListDatasetEntriesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.lookoutvision.paginators.ListDatasetEntriesIterable responses = client.listDatasetEntriesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.lookoutvision.paginators.ListDatasetEntriesIterable responses = client
* .listDatasetEntriesPaginator(request);
* for (software.amazon.awssdk.services.lookoutvision.model.ListDatasetEntriesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.lookoutvision.paginators.ListDatasetEntriesIterable responses = client.listDatasetEntriesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDatasetEntries(software.amazon.awssdk.services.lookoutvision.model.ListDatasetEntriesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListDatasetEntriesRequest.Builder} avoiding the
* need to create one manually via {@link ListDatasetEntriesRequest#builder()}
*
*
* @param listDatasetEntriesRequest
* A {@link Consumer} that will call methods on {@link ListDatasetEntriesRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.ListDatasetEntries
* @see AWS API Documentation
*/
default ListDatasetEntriesIterable listDatasetEntriesPaginator(
Consumer listDatasetEntriesRequest) throws AccessDeniedException,
InternalServerException, ValidationException, ConflictException, ResourceNotFoundException, ThrottlingException,
AwsServiceException, SdkClientException, LookoutVisionException {
return listDatasetEntriesPaginator(ListDatasetEntriesRequest.builder().applyMutation(listDatasetEntriesRequest).build());
}
/**
*
* Lists the versions of a model in an Amazon Lookout for Vision project.
*
*
* This operation requires permissions to perform the lookoutvision:ListModels
operation.
*
*
* @param listModelsRequest
* @return Result of the ListModels operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.ListModels
* @see AWS API
* Documentation
*/
default ListModelsResponse listModels(ListModelsRequest listModelsRequest) throws AccessDeniedException,
InternalServerException, ValidationException, ConflictException, ResourceNotFoundException, ThrottlingException,
AwsServiceException, SdkClientException, LookoutVisionException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the versions of a model in an Amazon Lookout for Vision project.
*
*
* This operation requires permissions to perform the lookoutvision:ListModels
operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListModelsRequest.Builder} avoiding the need to
* create one manually via {@link ListModelsRequest#builder()}
*
*
* @param listModelsRequest
* A {@link Consumer} that will call methods on {@link ListModelsRequest.Builder} to create a request.
* @return Result of the ListModels operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.ListModels
* @see AWS API
* Documentation
*/
default ListModelsResponse listModels(Consumer listModelsRequest) throws AccessDeniedException,
InternalServerException, ValidationException, ConflictException, ResourceNotFoundException, ThrottlingException,
AwsServiceException, SdkClientException, LookoutVisionException {
return listModels(ListModelsRequest.builder().applyMutation(listModelsRequest).build());
}
/**
*
* Lists the versions of a model in an Amazon Lookout for Vision project.
*
*
* This operation requires permissions to perform the lookoutvision:ListModels
operation.
*
*
*
* This is a variant of {@link #listModels(software.amazon.awssdk.services.lookoutvision.model.ListModelsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.lookoutvision.paginators.ListModelsIterable responses = client.listModelsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.lookoutvision.paginators.ListModelsIterable responses = client.listModelsPaginator(request);
* for (software.amazon.awssdk.services.lookoutvision.model.ListModelsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.lookoutvision.paginators.ListModelsIterable responses = client.listModelsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listModels(software.amazon.awssdk.services.lookoutvision.model.ListModelsRequest)} operation.
*
*
* @param listModelsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.ListModels
* @see AWS API
* Documentation
*/
default ListModelsIterable listModelsPaginator(ListModelsRequest listModelsRequest) throws AccessDeniedException,
InternalServerException, ValidationException, ConflictException, ResourceNotFoundException, ThrottlingException,
AwsServiceException, SdkClientException, LookoutVisionException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the versions of a model in an Amazon Lookout for Vision project.
*
*
* This operation requires permissions to perform the lookoutvision:ListModels
operation.
*
*
*
* This is a variant of {@link #listModels(software.amazon.awssdk.services.lookoutvision.model.ListModelsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.lookoutvision.paginators.ListModelsIterable responses = client.listModelsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.lookoutvision.paginators.ListModelsIterable responses = client.listModelsPaginator(request);
* for (software.amazon.awssdk.services.lookoutvision.model.ListModelsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.lookoutvision.paginators.ListModelsIterable responses = client.listModelsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listModels(software.amazon.awssdk.services.lookoutvision.model.ListModelsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListModelsRequest.Builder} avoiding the need to
* create one manually via {@link ListModelsRequest#builder()}
*
*
* @param listModelsRequest
* A {@link Consumer} that will call methods on {@link ListModelsRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.ListModels
* @see AWS API
* Documentation
*/
default ListModelsIterable listModelsPaginator(Consumer listModelsRequest)
throws AccessDeniedException, InternalServerException, ValidationException, ConflictException,
ResourceNotFoundException, ThrottlingException, AwsServiceException, SdkClientException, LookoutVisionException {
return listModelsPaginator(ListModelsRequest.builder().applyMutation(listModelsRequest).build());
}
/**
*
* Lists the Amazon Lookout for Vision projects in your AWS account.
*
*
* This operation requires permissions to perform the lookoutvision:ListProjects
operation.
*
*
* @param listProjectsRequest
* @return Result of the ListProjects operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.ListProjects
* @see AWS API
* Documentation
*/
default ListProjectsResponse listProjects(ListProjectsRequest listProjectsRequest) throws AccessDeniedException,
InternalServerException, ValidationException, ConflictException, ResourceNotFoundException, ThrottlingException,
AwsServiceException, SdkClientException, LookoutVisionException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the Amazon Lookout for Vision projects in your AWS account.
*
*
* This operation requires permissions to perform the lookoutvision:ListProjects
operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListProjectsRequest.Builder} avoiding the need to
* create one manually via {@link ListProjectsRequest#builder()}
*
*
* @param listProjectsRequest
* A {@link Consumer} that will call methods on {@link ListProjectsRequest.Builder} to create a request.
* @return Result of the ListProjects operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.ListProjects
* @see AWS API
* Documentation
*/
default ListProjectsResponse listProjects(Consumer listProjectsRequest)
throws AccessDeniedException, InternalServerException, ValidationException, ConflictException,
ResourceNotFoundException, ThrottlingException, AwsServiceException, SdkClientException, LookoutVisionException {
return listProjects(ListProjectsRequest.builder().applyMutation(listProjectsRequest).build());
}
/**
*
* Lists the Amazon Lookout for Vision projects in your AWS account.
*
*
* This operation requires permissions to perform the lookoutvision:ListProjects
operation.
*
*
*
* This is a variant of
* {@link #listProjects(software.amazon.awssdk.services.lookoutvision.model.ListProjectsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.lookoutvision.paginators.ListProjectsIterable responses = client.listProjectsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.lookoutvision.paginators.ListProjectsIterable responses = client
* .listProjectsPaginator(request);
* for (software.amazon.awssdk.services.lookoutvision.model.ListProjectsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.lookoutvision.paginators.ListProjectsIterable responses = client.listProjectsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listProjects(software.amazon.awssdk.services.lookoutvision.model.ListProjectsRequest)} operation.
*
*
* @param listProjectsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.ListProjects
* @see AWS API
* Documentation
*/
default ListProjectsIterable listProjectsPaginator(ListProjectsRequest listProjectsRequest) throws AccessDeniedException,
InternalServerException, ValidationException, ConflictException, ResourceNotFoundException, ThrottlingException,
AwsServiceException, SdkClientException, LookoutVisionException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the Amazon Lookout for Vision projects in your AWS account.
*
*
* This operation requires permissions to perform the lookoutvision:ListProjects
operation.
*
*
*
* This is a variant of
* {@link #listProjects(software.amazon.awssdk.services.lookoutvision.model.ListProjectsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.lookoutvision.paginators.ListProjectsIterable responses = client.listProjectsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.lookoutvision.paginators.ListProjectsIterable responses = client
* .listProjectsPaginator(request);
* for (software.amazon.awssdk.services.lookoutvision.model.ListProjectsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.lookoutvision.paginators.ListProjectsIterable responses = client.listProjectsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listProjects(software.amazon.awssdk.services.lookoutvision.model.ListProjectsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListProjectsRequest.Builder} avoiding the need to
* create one manually via {@link ListProjectsRequest#builder()}
*
*
* @param listProjectsRequest
* A {@link Consumer} that will call methods on {@link ListProjectsRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.ListProjects
* @see AWS API
* Documentation
*/
default ListProjectsIterable listProjectsPaginator(Consumer listProjectsRequest)
throws AccessDeniedException, InternalServerException, ValidationException, ConflictException,
ResourceNotFoundException, ThrottlingException, AwsServiceException, SdkClientException, LookoutVisionException {
return listProjectsPaginator(ListProjectsRequest.builder().applyMutation(listProjectsRequest).build());
}
/**
*
* Returns a list of tags attached to the specified Amazon Lookout for Vision model.
*
*
* This operation requires permissions to perform the lookoutvision:ListTagsForResource
operation.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.ListTagsForResource
* @see AWS API Documentation
*/
default ListTagsForResourceResponse listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest)
throws AccessDeniedException, InternalServerException, ValidationException, ConflictException,
ResourceNotFoundException, ThrottlingException, AwsServiceException, SdkClientException, LookoutVisionException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of tags attached to the specified Amazon Lookout for Vision model.
*
*
* This operation requires permissions to perform the lookoutvision:ListTagsForResource
operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on {@link ListTagsForResourceRequest.Builder} to create a
* request.
* @return Result of the ListTagsForResource operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.ListTagsForResource
* @see AWS API Documentation
*/
default ListTagsForResourceResponse listTagsForResource(
Consumer listTagsForResourceRequest) throws AccessDeniedException,
InternalServerException, ValidationException, ConflictException, ResourceNotFoundException, ThrottlingException,
AwsServiceException, SdkClientException, LookoutVisionException {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Starts the running of the version of an Amazon Lookout for Vision model. Starting a model takes a while to
* complete. To check the current state of the model, use DescribeModel.
*
*
* A model is ready to use when its status is HOSTED
.
*
*
* Once the model is running, you can detect custom labels in new images by calling DetectAnomalies.
*
*
*
* You are charged for the amount of time that the model is running. To stop a running model, call StopModel.
*
*
*
* This operation requires permissions to perform the lookoutvision:StartModel
operation.
*
*
* @param startModelRequest
* @return Result of the StartModel operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws ServiceQuotaExceededException
* A service quota was exceeded the allowed limit. For more information, see Limits in Amazon Lookout for
* Vision in the Amazon Lookout for Vision Developer Guide.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.StartModel
* @see AWS API
* Documentation
*/
default StartModelResponse startModel(StartModelRequest startModelRequest) throws AccessDeniedException,
InternalServerException, ValidationException, ConflictException, ResourceNotFoundException, ThrottlingException,
ServiceQuotaExceededException, AwsServiceException, SdkClientException, LookoutVisionException {
throw new UnsupportedOperationException();
}
/**
*
* Starts the running of the version of an Amazon Lookout for Vision model. Starting a model takes a while to
* complete. To check the current state of the model, use DescribeModel.
*
*
* A model is ready to use when its status is HOSTED
.
*
*
* Once the model is running, you can detect custom labels in new images by calling DetectAnomalies.
*
*
*
* You are charged for the amount of time that the model is running. To stop a running model, call StopModel.
*
*
*
* This operation requires permissions to perform the lookoutvision:StartModel
operation.
*
*
*
* This is a convenience which creates an instance of the {@link StartModelRequest.Builder} avoiding the need to
* create one manually via {@link StartModelRequest#builder()}
*
*
* @param startModelRequest
* A {@link Consumer} that will call methods on {@link StartModelRequest.Builder} to create a request.
* @return Result of the StartModel operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws ServiceQuotaExceededException
* A service quota was exceeded the allowed limit. For more information, see Limits in Amazon Lookout for
* Vision in the Amazon Lookout for Vision Developer Guide.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.StartModel
* @see AWS API
* Documentation
*/
default StartModelResponse startModel(Consumer startModelRequest) throws AccessDeniedException,
InternalServerException, ValidationException, ConflictException, ResourceNotFoundException, ThrottlingException,
ServiceQuotaExceededException, AwsServiceException, SdkClientException, LookoutVisionException {
return startModel(StartModelRequest.builder().applyMutation(startModelRequest).build());
}
/**
*
* Stops the hosting of a running model. The operation might take a while to complete. To check the current status,
* call DescribeModel.
*
*
* After the model hosting stops, the Status
of the model is TRAINED
.
*
*
* This operation requires permissions to perform the lookoutvision:StopModel
operation.
*
*
* @param stopModelRequest
* @return Result of the StopModel operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.StopModel
* @see AWS API
* Documentation
*/
default StopModelResponse stopModel(StopModelRequest stopModelRequest) throws AccessDeniedException, InternalServerException,
ValidationException, ConflictException, ResourceNotFoundException, ThrottlingException, AwsServiceException,
SdkClientException, LookoutVisionException {
throw new UnsupportedOperationException();
}
/**
*
* Stops the hosting of a running model. The operation might take a while to complete. To check the current status,
* call DescribeModel.
*
*
* After the model hosting stops, the Status
of the model is TRAINED
.
*
*
* This operation requires permissions to perform the lookoutvision:StopModel
operation.
*
*
*
* This is a convenience which creates an instance of the {@link StopModelRequest.Builder} avoiding the need to
* create one manually via {@link StopModelRequest#builder()}
*
*
* @param stopModelRequest
* A {@link Consumer} that will call methods on {@link StopModelRequest.Builder} to create a request.
* @return Result of the StopModel operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.StopModel
* @see AWS API
* Documentation
*/
default StopModelResponse stopModel(Consumer stopModelRequest) throws AccessDeniedException,
InternalServerException, ValidationException, ConflictException, ResourceNotFoundException, ThrottlingException,
AwsServiceException, SdkClientException, LookoutVisionException {
return stopModel(StopModelRequest.builder().applyMutation(stopModelRequest).build());
}
/**
*
* Adds one or more key-value tags to an Amazon Lookout for Vision model. For more information, see Tagging a
* model in the Amazon Lookout for Vision Developer Guide.
*
*
* This operation requires permissions to perform the lookoutvision:TagResource
operation.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws ServiceQuotaExceededException
* A service quota was exceeded the allowed limit. For more information, see Limits in Amazon Lookout for
* Vision in the Amazon Lookout for Vision Developer Guide.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.TagResource
* @see AWS API
* Documentation
*/
default TagResourceResponse tagResource(TagResourceRequest tagResourceRequest) throws AccessDeniedException,
InternalServerException, ValidationException, ConflictException, ResourceNotFoundException, ThrottlingException,
ServiceQuotaExceededException, AwsServiceException, SdkClientException, LookoutVisionException {
throw new UnsupportedOperationException();
}
/**
*
* Adds one or more key-value tags to an Amazon Lookout for Vision model. For more information, see Tagging a
* model in the Amazon Lookout for Vision Developer Guide.
*
*
* This operation requires permissions to perform the lookoutvision:TagResource
operation.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on {@link TagResourceRequest.Builder} to create a request.
* @return Result of the TagResource operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws ServiceQuotaExceededException
* A service quota was exceeded the allowed limit. For more information, see Limits in Amazon Lookout for
* Vision in the Amazon Lookout for Vision Developer Guide.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.TagResource
* @see AWS API
* Documentation
*/
default TagResourceResponse tagResource(Consumer tagResourceRequest)
throws AccessDeniedException, InternalServerException, ValidationException, ConflictException,
ResourceNotFoundException, ThrottlingException, ServiceQuotaExceededException, AwsServiceException,
SdkClientException, LookoutVisionException {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Removes one or more tags from an Amazon Lookout for Vision model. For more information, see Tagging a
* model in the Amazon Lookout for Vision Developer Guide.
*
*
* This operation requires permissions to perform the lookoutvision:UntagResource
operation.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.UntagResource
* @see AWS
* API Documentation
*/
default UntagResourceResponse untagResource(UntagResourceRequest untagResourceRequest) throws AccessDeniedException,
InternalServerException, ValidationException, ConflictException, ResourceNotFoundException, ThrottlingException,
AwsServiceException, SdkClientException, LookoutVisionException {
throw new UnsupportedOperationException();
}
/**
*
* Removes one or more tags from an Amazon Lookout for Vision model. For more information, see Tagging a
* model in the Amazon Lookout for Vision Developer Guide.
*
*
* This operation requires permissions to perform the lookoutvision:UntagResource
operation.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on {@link UntagResourceRequest.Builder} to create a request.
* @return Result of the UntagResource operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.UntagResource
* @see AWS
* API Documentation
*/
default UntagResourceResponse untagResource(Consumer untagResourceRequest)
throws AccessDeniedException, InternalServerException, ValidationException, ConflictException,
ResourceNotFoundException, ThrottlingException, AwsServiceException, SdkClientException, LookoutVisionException {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
*
* Adds one or more JSON Line entries to a dataset. A JSON Line includes information about an image used for
* training or testing an Amazon Lookout for Vision model. The following is an example JSON Line.
*
*
* Updating a dataset might take a while to complete. To check the current status, call DescribeDataset and
* check the Status
field in the response.
*
*
* This operation requires permissions to perform the lookoutvision:UpdateDatasetEntries
operation.
*
*
* @param updateDatasetEntriesRequest
* @return Result of the UpdateDatasetEntries operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.UpdateDatasetEntries
* @see AWS API Documentation
*/
default UpdateDatasetEntriesResponse updateDatasetEntries(UpdateDatasetEntriesRequest updateDatasetEntriesRequest)
throws AccessDeniedException, InternalServerException, ValidationException, ConflictException,
ResourceNotFoundException, ThrottlingException, AwsServiceException, SdkClientException, LookoutVisionException {
throw new UnsupportedOperationException();
}
/**
*
* Adds one or more JSON Line entries to a dataset. A JSON Line includes information about an image used for
* training or testing an Amazon Lookout for Vision model. The following is an example JSON Line.
*
*
* Updating a dataset might take a while to complete. To check the current status, call DescribeDataset and
* check the Status
field in the response.
*
*
* This operation requires permissions to perform the lookoutvision:UpdateDatasetEntries
operation.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateDatasetEntriesRequest.Builder} avoiding the
* need to create one manually via {@link UpdateDatasetEntriesRequest#builder()}
*
*
* @param updateDatasetEntriesRequest
* A {@link Consumer} that will call methods on {@link UpdateDatasetEntriesRequest.Builder} to create a
* request.
* @return Result of the UpdateDatasetEntries operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws LookoutVisionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample LookoutVisionClient.UpdateDatasetEntries
* @see AWS API Documentation
*/
default UpdateDatasetEntriesResponse updateDatasetEntries(
Consumer updateDatasetEntriesRequest) throws AccessDeniedException,
InternalServerException, ValidationException, ConflictException, ResourceNotFoundException, ThrottlingException,
AwsServiceException, SdkClientException, LookoutVisionException {
return updateDatasetEntries(UpdateDatasetEntriesRequest.builder().applyMutation(updateDatasetEntriesRequest).build());
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of("lookoutvision");
}
}