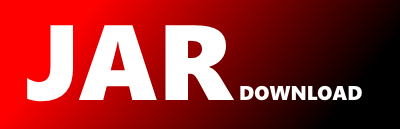
software.amazon.awssdk.services.lookoutvision.model.ModelMetadata Maven / Gradle / Ivy
Show all versions of lookoutvision Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.lookoutvision.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes an Amazon Lookout for Vision model.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ModelMetadata implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField CREATION_TIMESTAMP_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreationTimestamp").getter(getter(ModelMetadata::creationTimestamp))
.setter(setter(Builder::creationTimestamp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreationTimestamp").build()).build();
private static final SdkField MODEL_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ModelVersion").getter(getter(ModelMetadata::modelVersion)).setter(setter(Builder::modelVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ModelVersion").build()).build();
private static final SdkField MODEL_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ModelArn").getter(getter(ModelMetadata::modelArn)).setter(setter(Builder::modelArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ModelArn").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Description").getter(getter(ModelMetadata::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(ModelMetadata::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField STATUS_MESSAGE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StatusMessage").getter(getter(ModelMetadata::statusMessage)).setter(setter(Builder::statusMessage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StatusMessage").build()).build();
private static final SdkField PERFORMANCE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("Performance")
.getter(getter(ModelMetadata::performance)).setter(setter(Builder::performance))
.constructor(ModelPerformance::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Performance").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CREATION_TIMESTAMP_FIELD,
MODEL_VERSION_FIELD, MODEL_ARN_FIELD, DESCRIPTION_FIELD, STATUS_FIELD, STATUS_MESSAGE_FIELD, PERFORMANCE_FIELD));
private static final long serialVersionUID = 1L;
private final Instant creationTimestamp;
private final String modelVersion;
private final String modelArn;
private final String description;
private final String status;
private final String statusMessage;
private final ModelPerformance performance;
private ModelMetadata(BuilderImpl builder) {
this.creationTimestamp = builder.creationTimestamp;
this.modelVersion = builder.modelVersion;
this.modelArn = builder.modelArn;
this.description = builder.description;
this.status = builder.status;
this.statusMessage = builder.statusMessage;
this.performance = builder.performance;
}
/**
*
* The unix timestamp for the date and time that the model was created.
*
*
* @return The unix timestamp for the date and time that the model was created.
*/
public final Instant creationTimestamp() {
return creationTimestamp;
}
/**
*
* The version of the model.
*
*
* @return The version of the model.
*/
public final String modelVersion() {
return modelVersion;
}
/**
*
* The Amazon Resource Name (ARN) of the model.
*
*
* @return The Amazon Resource Name (ARN) of the model.
*/
public final String modelArn() {
return modelArn;
}
/**
*
* The description for the model.
*
*
* @return The description for the model.
*/
public final String description() {
return description;
}
/**
*
* The status of the model.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ModelStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The status of the model.
* @see ModelStatus
*/
public final ModelStatus status() {
return ModelStatus.fromValue(status);
}
/**
*
* The status of the model.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ModelStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The status of the model.
* @see ModelStatus
*/
public final String statusAsString() {
return status;
}
/**
*
* The status message for the model.
*
*
* @return The status message for the model.
*/
public final String statusMessage() {
return statusMessage;
}
/**
*
* Performance metrics for the model. Not available until training has successfully completed.
*
*
* @return Performance metrics for the model. Not available until training has successfully completed.
*/
public final ModelPerformance performance() {
return performance;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(creationTimestamp());
hashCode = 31 * hashCode + Objects.hashCode(modelVersion());
hashCode = 31 * hashCode + Objects.hashCode(modelArn());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(statusMessage());
hashCode = 31 * hashCode + Objects.hashCode(performance());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ModelMetadata)) {
return false;
}
ModelMetadata other = (ModelMetadata) obj;
return Objects.equals(creationTimestamp(), other.creationTimestamp())
&& Objects.equals(modelVersion(), other.modelVersion()) && Objects.equals(modelArn(), other.modelArn())
&& Objects.equals(description(), other.description()) && Objects.equals(statusAsString(), other.statusAsString())
&& Objects.equals(statusMessage(), other.statusMessage()) && Objects.equals(performance(), other.performance());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ModelMetadata").add("CreationTimestamp", creationTimestamp())
.add("ModelVersion", modelVersion()).add("ModelArn", modelArn()).add("Description", description())
.add("Status", statusAsString()).add("StatusMessage", statusMessage()).add("Performance", performance()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "CreationTimestamp":
return Optional.ofNullable(clazz.cast(creationTimestamp()));
case "ModelVersion":
return Optional.ofNullable(clazz.cast(modelVersion()));
case "ModelArn":
return Optional.ofNullable(clazz.cast(modelArn()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "Status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "StatusMessage":
return Optional.ofNullable(clazz.cast(statusMessage()));
case "Performance":
return Optional.ofNullable(clazz.cast(performance()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function