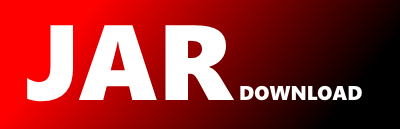
software.amazon.awssdk.services.lookoutvision.DefaultLookoutVisionAsyncClient Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.lookoutvision;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.auth.signer.AsyncAws4Signer;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.ApiName;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.async.AsyncRequestBody;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.runtime.transform.AsyncStreamingRequestMarshaller;
import software.amazon.awssdk.core.signer.Signer;
import software.amazon.awssdk.core.util.VersionInfo;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.services.lookoutvision.model.AccessDeniedException;
import software.amazon.awssdk.services.lookoutvision.model.ConflictException;
import software.amazon.awssdk.services.lookoutvision.model.CreateDatasetRequest;
import software.amazon.awssdk.services.lookoutvision.model.CreateDatasetResponse;
import software.amazon.awssdk.services.lookoutvision.model.CreateModelRequest;
import software.amazon.awssdk.services.lookoutvision.model.CreateModelResponse;
import software.amazon.awssdk.services.lookoutvision.model.CreateProjectRequest;
import software.amazon.awssdk.services.lookoutvision.model.CreateProjectResponse;
import software.amazon.awssdk.services.lookoutvision.model.DeleteDatasetRequest;
import software.amazon.awssdk.services.lookoutvision.model.DeleteDatasetResponse;
import software.amazon.awssdk.services.lookoutvision.model.DeleteModelRequest;
import software.amazon.awssdk.services.lookoutvision.model.DeleteModelResponse;
import software.amazon.awssdk.services.lookoutvision.model.DeleteProjectRequest;
import software.amazon.awssdk.services.lookoutvision.model.DeleteProjectResponse;
import software.amazon.awssdk.services.lookoutvision.model.DescribeDatasetRequest;
import software.amazon.awssdk.services.lookoutvision.model.DescribeDatasetResponse;
import software.amazon.awssdk.services.lookoutvision.model.DescribeModelRequest;
import software.amazon.awssdk.services.lookoutvision.model.DescribeModelResponse;
import software.amazon.awssdk.services.lookoutvision.model.DescribeProjectRequest;
import software.amazon.awssdk.services.lookoutvision.model.DescribeProjectResponse;
import software.amazon.awssdk.services.lookoutvision.model.DetectAnomaliesRequest;
import software.amazon.awssdk.services.lookoutvision.model.DetectAnomaliesResponse;
import software.amazon.awssdk.services.lookoutvision.model.InternalServerException;
import software.amazon.awssdk.services.lookoutvision.model.ListDatasetEntriesRequest;
import software.amazon.awssdk.services.lookoutvision.model.ListDatasetEntriesResponse;
import software.amazon.awssdk.services.lookoutvision.model.ListModelsRequest;
import software.amazon.awssdk.services.lookoutvision.model.ListModelsResponse;
import software.amazon.awssdk.services.lookoutvision.model.ListProjectsRequest;
import software.amazon.awssdk.services.lookoutvision.model.ListProjectsResponse;
import software.amazon.awssdk.services.lookoutvision.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.lookoutvision.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.lookoutvision.model.LookoutVisionException;
import software.amazon.awssdk.services.lookoutvision.model.LookoutVisionRequest;
import software.amazon.awssdk.services.lookoutvision.model.ResourceNotFoundException;
import software.amazon.awssdk.services.lookoutvision.model.ServiceQuotaExceededException;
import software.amazon.awssdk.services.lookoutvision.model.StartModelRequest;
import software.amazon.awssdk.services.lookoutvision.model.StartModelResponse;
import software.amazon.awssdk.services.lookoutvision.model.StopModelRequest;
import software.amazon.awssdk.services.lookoutvision.model.StopModelResponse;
import software.amazon.awssdk.services.lookoutvision.model.TagResourceRequest;
import software.amazon.awssdk.services.lookoutvision.model.TagResourceResponse;
import software.amazon.awssdk.services.lookoutvision.model.ThrottlingException;
import software.amazon.awssdk.services.lookoutvision.model.UntagResourceRequest;
import software.amazon.awssdk.services.lookoutvision.model.UntagResourceResponse;
import software.amazon.awssdk.services.lookoutvision.model.UpdateDatasetEntriesRequest;
import software.amazon.awssdk.services.lookoutvision.model.UpdateDatasetEntriesResponse;
import software.amazon.awssdk.services.lookoutvision.model.ValidationException;
import software.amazon.awssdk.services.lookoutvision.paginators.ListDatasetEntriesPublisher;
import software.amazon.awssdk.services.lookoutvision.paginators.ListModelsPublisher;
import software.amazon.awssdk.services.lookoutvision.paginators.ListProjectsPublisher;
import software.amazon.awssdk.services.lookoutvision.transform.CreateDatasetRequestMarshaller;
import software.amazon.awssdk.services.lookoutvision.transform.CreateModelRequestMarshaller;
import software.amazon.awssdk.services.lookoutvision.transform.CreateProjectRequestMarshaller;
import software.amazon.awssdk.services.lookoutvision.transform.DeleteDatasetRequestMarshaller;
import software.amazon.awssdk.services.lookoutvision.transform.DeleteModelRequestMarshaller;
import software.amazon.awssdk.services.lookoutvision.transform.DeleteProjectRequestMarshaller;
import software.amazon.awssdk.services.lookoutvision.transform.DescribeDatasetRequestMarshaller;
import software.amazon.awssdk.services.lookoutvision.transform.DescribeModelRequestMarshaller;
import software.amazon.awssdk.services.lookoutvision.transform.DescribeProjectRequestMarshaller;
import software.amazon.awssdk.services.lookoutvision.transform.DetectAnomaliesRequestMarshaller;
import software.amazon.awssdk.services.lookoutvision.transform.ListDatasetEntriesRequestMarshaller;
import software.amazon.awssdk.services.lookoutvision.transform.ListModelsRequestMarshaller;
import software.amazon.awssdk.services.lookoutvision.transform.ListProjectsRequestMarshaller;
import software.amazon.awssdk.services.lookoutvision.transform.ListTagsForResourceRequestMarshaller;
import software.amazon.awssdk.services.lookoutvision.transform.StartModelRequestMarshaller;
import software.amazon.awssdk.services.lookoutvision.transform.StopModelRequestMarshaller;
import software.amazon.awssdk.services.lookoutvision.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.lookoutvision.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.lookoutvision.transform.UpdateDatasetEntriesRequestMarshaller;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link LookoutVisionAsyncClient}.
*
* @see LookoutVisionAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultLookoutVisionAsyncClient implements LookoutVisionAsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultLookoutVisionAsyncClient.class);
private final AsyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultLookoutVisionAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
*
* Creates a new dataset in an Amazon Lookout for Vision project. CreateDataset
can create a training
* or a test dataset from a valid dataset source (DatasetSource
).
*
*
* If you want a single dataset project, specify train
for the value of DatasetType
.
*
*
* To have a project with separate training and test datasets, call CreateDataset
twice. On the first
* call, specify train
for the value of DatasetType
. On the second call, specify
* test
for the value of DatasetType
.
*
*
* This operation requires permissions to perform the lookoutvision:CreateDataset
operation.
*
*
* @param createDatasetRequest
* @return A Java Future containing the result of the CreateDataset operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You are not authorized to perform the action.
* - InternalServerException Amazon Lookout for Vision experienced a service issue. Try your call again.
* - ValidationException An input validation error occured. For example, invalid characters in a project
* name, or if a pagination token is invalid.
* - ConflictException The update or deletion of a resource caused an inconsistent state.
* - ResourceNotFoundException The resource could not be found.
* - ThrottlingException Amazon Lookout for Vision is temporarily unable to process the request. Try your
* call again.
* - ServiceQuotaExceededException A service quota was exceeded the allowed limit. For more information,
* see Limits in Amazon Lookout for Vision in the Amazon Lookout for Vision Developer Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - LookoutVisionException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample LookoutVisionAsyncClient.CreateDataset
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture createDataset(CreateDatasetRequest createDatasetRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createDatasetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "LookoutVision");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateDataset");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateDatasetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateDataset")
.withMarshaller(new CreateDatasetRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createDatasetRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = createDatasetRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new version of a model within an an Amazon Lookout for Vision project. CreateModel
is an
* asynchronous operation in which Amazon Lookout for Vision trains, tests, and evaluates a new version of a model.
*
*
* To get the current status, check the Status
field returned in the response from
* DescribeModel.
*
*
* If the project has a single dataset, Amazon Lookout for Vision internally splits the dataset to create a training
* and a test dataset. If the project has a training and a test dataset, Lookout for Vision uses the respective
* datasets to train and test the model.
*
*
* After training completes, the evaluation metrics are stored at the location specified in
* OutputConfig
.
*
*
* This operation requires permissions to perform the lookoutvision:CreateModel
operation. If you want
* to tag your model, you also require permission to the lookoutvision:TagResource
operation.
*
*
* @param createModelRequest
* @return A Java Future containing the result of the CreateModel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You are not authorized to perform the action.
* - InternalServerException Amazon Lookout for Vision experienced a service issue. Try your call again.
* - ValidationException An input validation error occured. For example, invalid characters in a project
* name, or if a pagination token is invalid.
* - ConflictException The update or deletion of a resource caused an inconsistent state.
* - ResourceNotFoundException The resource could not be found.
* - ThrottlingException Amazon Lookout for Vision is temporarily unable to process the request. Try your
* call again.
* - ServiceQuotaExceededException A service quota was exceeded the allowed limit. For more information,
* see Limits in Amazon Lookout for Vision in the Amazon Lookout for Vision Developer Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - LookoutVisionException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample LookoutVisionAsyncClient.CreateModel
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createModel(CreateModelRequest createModelRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createModelRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "LookoutVision");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateModel");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateModelResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateModel").withMarshaller(new CreateModelRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createModelRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = createModelRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates an empty Amazon Lookout for Vision project. After you create the project, add a dataset by calling
* CreateDataset.
*
*
* This operation requires permissions to perform the lookoutvision:CreateProject
operation.
*
*
* @param createProjectRequest
* @return A Java Future containing the result of the CreateProject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You are not authorized to perform the action.
* - InternalServerException Amazon Lookout for Vision experienced a service issue. Try your call again.
* - ValidationException An input validation error occured. For example, invalid characters in a project
* name, or if a pagination token is invalid.
* - ConflictException The update or deletion of a resource caused an inconsistent state.
* - ResourceNotFoundException The resource could not be found.
* - ThrottlingException Amazon Lookout for Vision is temporarily unable to process the request. Try your
* call again.
* - ServiceQuotaExceededException A service quota was exceeded the allowed limit. For more information,
* see Limits in Amazon Lookout for Vision in the Amazon Lookout for Vision Developer Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - LookoutVisionException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample LookoutVisionAsyncClient.CreateProject
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture createProject(CreateProjectRequest createProjectRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createProjectRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "LookoutVision");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateProject");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateProjectResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateProject")
.withMarshaller(new CreateProjectRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createProjectRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = createProjectRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes an existing Amazon Lookout for Vision dataset
.
*
*
* If your the project has a single dataset, you must create a new dataset before you can create a model.
*
*
* If you project has a training dataset and a test dataset consider the following.
*
*
* -
*
* If you delete the test dataset, your project reverts to a single dataset project. If you then train the model,
* Amazon Lookout for Vision internally splits the remaining dataset into a training and test dataset.
*
*
* -
*
* If you delete the training dataset, you must create a training dataset before you can create a model.
*
*
*
*
* This operation requires permissions to perform the lookoutvision:DeleteDataset
operation.
*
*
* @param deleteDatasetRequest
* @return A Java Future containing the result of the DeleteDataset operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You are not authorized to perform the action.
* - InternalServerException Amazon Lookout for Vision experienced a service issue. Try your call again.
* - ValidationException An input validation error occured. For example, invalid characters in a project
* name, or if a pagination token is invalid.
* - ConflictException The update or deletion of a resource caused an inconsistent state.
* - ResourceNotFoundException The resource could not be found.
* - ThrottlingException Amazon Lookout for Vision is temporarily unable to process the request. Try your
* call again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - LookoutVisionException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample LookoutVisionAsyncClient.DeleteDataset
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteDataset(DeleteDatasetRequest deleteDatasetRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteDatasetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "LookoutVision");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteDataset");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteDatasetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteDataset")
.withMarshaller(new DeleteDatasetRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteDatasetRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteDatasetRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes an Amazon Lookout for Vision model. You can't delete a running model. To stop a running model, use the
* StopModel operation.
*
*
* It might take a few seconds to delete a model. To determine if a model has been deleted, call ListProjects
* and check if the version of the model (ModelVersion
) is in the Models
array.
*
*
* This operation requires permissions to perform the lookoutvision:DeleteModel
operation.
*
*
* @param deleteModelRequest
* @return A Java Future containing the result of the DeleteModel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You are not authorized to perform the action.
* - InternalServerException Amazon Lookout for Vision experienced a service issue. Try your call again.
* - ValidationException An input validation error occured. For example, invalid characters in a project
* name, or if a pagination token is invalid.
* - ConflictException The update or deletion of a resource caused an inconsistent state.
* - ResourceNotFoundException The resource could not be found.
* - ThrottlingException Amazon Lookout for Vision is temporarily unable to process the request. Try your
* call again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - LookoutVisionException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample LookoutVisionAsyncClient.DeleteModel
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteModel(DeleteModelRequest deleteModelRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteModelRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "LookoutVision");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteModel");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteModelResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteModel").withMarshaller(new DeleteModelRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteModelRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteModelRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes an Amazon Lookout for Vision project.
*
*
* To delete a project, you must first delete each version of the model associated with the project. To delete a
* model use the DeleteModel operation.
*
*
* You also have to delete the dataset(s) associated with the model. For more information, see DeleteDataset.
* The images referenced by the training and test datasets aren't deleted.
*
*
* This operation requires permissions to perform the lookoutvision:DeleteProject
operation.
*
*
* @param deleteProjectRequest
* @return A Java Future containing the result of the DeleteProject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You are not authorized to perform the action.
* - InternalServerException Amazon Lookout for Vision experienced a service issue. Try your call again.
* - ValidationException An input validation error occured. For example, invalid characters in a project
* name, or if a pagination token is invalid.
* - ConflictException The update or deletion of a resource caused an inconsistent state.
* - ResourceNotFoundException The resource could not be found.
* - ThrottlingException Amazon Lookout for Vision is temporarily unable to process the request. Try your
* call again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - LookoutVisionException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample LookoutVisionAsyncClient.DeleteProject
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteProject(DeleteProjectRequest deleteProjectRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteProjectRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "LookoutVision");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteProject");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteProjectResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteProject")
.withMarshaller(new DeleteProjectRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteProjectRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteProjectRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describe an Amazon Lookout for Vision dataset.
*
*
* This operation requires permissions to perform the lookoutvision:DescribeDataset
operation.
*
*
* @param describeDatasetRequest
* @return A Java Future containing the result of the DescribeDataset operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You are not authorized to perform the action.
* - InternalServerException Amazon Lookout for Vision experienced a service issue. Try your call again.
* - ValidationException An input validation error occured. For example, invalid characters in a project
* name, or if a pagination token is invalid.
* - ConflictException The update or deletion of a resource caused an inconsistent state.
* - ResourceNotFoundException The resource could not be found.
* - ThrottlingException Amazon Lookout for Vision is temporarily unable to process the request. Try your
* call again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - LookoutVisionException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample LookoutVisionAsyncClient.DescribeDataset
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describeDataset(DescribeDatasetRequest describeDatasetRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeDatasetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "LookoutVision");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeDataset");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeDatasetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeDataset")
.withMarshaller(new DescribeDatasetRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeDatasetRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = describeDatasetRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes a version of an Amazon Lookout for Vision model.
*
*
* This operation requires permissions to perform the lookoutvision:DescribeModel
operation.
*
*
* @param describeModelRequest
* @return A Java Future containing the result of the DescribeModel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You are not authorized to perform the action.
* - InternalServerException Amazon Lookout for Vision experienced a service issue. Try your call again.
* - ValidationException An input validation error occured. For example, invalid characters in a project
* name, or if a pagination token is invalid.
* - ConflictException The update or deletion of a resource caused an inconsistent state.
* - ResourceNotFoundException The resource could not be found.
* - ThrottlingException Amazon Lookout for Vision is temporarily unable to process the request. Try your
* call again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - LookoutVisionException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample LookoutVisionAsyncClient.DescribeModel
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describeModel(DescribeModelRequest describeModelRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeModelRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "LookoutVision");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeModel");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeModelResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeModel")
.withMarshaller(new DescribeModelRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeModelRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = describeModelRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes an Amazon Lookout for Vision project.
*
*
* This operation requires permissions to perform the lookoutvision:DescribeProject
operation.
*
*
* @param describeProjectRequest
* @return A Java Future containing the result of the DescribeProject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You are not authorized to perform the action.
* - InternalServerException Amazon Lookout for Vision experienced a service issue. Try your call again.
* - ValidationException An input validation error occured. For example, invalid characters in a project
* name, or if a pagination token is invalid.
* - ConflictException The update or deletion of a resource caused an inconsistent state.
* - ResourceNotFoundException The resource could not be found.
* - ThrottlingException Amazon Lookout for Vision is temporarily unable to process the request. Try your
* call again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - LookoutVisionException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample LookoutVisionAsyncClient.DescribeProject
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describeProject(DescribeProjectRequest describeProjectRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeProjectRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "LookoutVision");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeProject");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeProjectResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeProject")
.withMarshaller(new DescribeProjectRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeProjectRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = describeProjectRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Detects anomalies in an image that you supply.
*
*
* The response from DetectAnomalies
includes a boolean prediction that the image contains one or more
* anomalies and a confidence value for the prediction.
*
*
*
* Before calling DetectAnomalies
, you must first start your model with the StartModel
* operation. You are charged for the amount of time, in minutes, that a model runs and for the number of anomaly
* detection units that your model uses. If you are not using a model, use the StopModel operation to stop
* your model.
*
*
*
* This operation requires permissions to perform the lookoutvision:DetectAnomalies
operation.
*
*
* @param detectAnomaliesRequest
* @param requestBody
* Functional interface that can be implemented to produce the request content in a non-blocking manner. The
* size of the content is expected to be known up front. See {@link AsyncRequestBody} for specific details on
* implementing this interface as well as links to precanned implementations for common scenarios like
* uploading from a file. The service documentation for the request content is as follows '
*
* The unencrypted image bytes that you want to analyze.
*
* '
* @return A Java Future containing the result of the DetectAnomalies operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You are not authorized to perform the action.
* - InternalServerException Amazon Lookout for Vision experienced a service issue. Try your call again.
* - ValidationException An input validation error occured. For example, invalid characters in a project
* name, or if a pagination token is invalid.
* - ConflictException The update or deletion of a resource caused an inconsistent state.
* - ResourceNotFoundException The resource could not be found.
* - ThrottlingException Amazon Lookout for Vision is temporarily unable to process the request. Try your
* call again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - LookoutVisionException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample LookoutVisionAsyncClient.DetectAnomalies
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture detectAnomalies(DetectAnomaliesRequest detectAnomaliesRequest,
AsyncRequestBody requestBody) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, detectAnomaliesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "LookoutVision");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DetectAnomalies");
if (!isSignerOverridden(clientConfiguration)) {
detectAnomaliesRequest = applySignerOverride(detectAnomaliesRequest, AsyncAws4Signer.create());
}
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DetectAnomaliesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DetectAnomalies")
.withMarshaller(
AsyncStreamingRequestMarshaller.builder()
.delegateMarshaller(new DetectAnomaliesRequestMarshaller(protocolFactory))
.asyncRequestBody(requestBody).requiresLength(true).build())
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withAsyncRequestBody(requestBody)
.withInput(detectAnomaliesRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = detectAnomaliesRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the JSON Lines within a dataset. An Amazon Lookout for Vision JSON Line contains the anomaly information
* for a single image, including the image location and the assigned label.
*
*
* This operation requires permissions to perform the lookoutvision:ListDatasetEntries
operation.
*
*
* @param listDatasetEntriesRequest
* @return A Java Future containing the result of the ListDatasetEntries operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You are not authorized to perform the action.
* - InternalServerException Amazon Lookout for Vision experienced a service issue. Try your call again.
* - ValidationException An input validation error occured. For example, invalid characters in a project
* name, or if a pagination token is invalid.
* - ConflictException The update or deletion of a resource caused an inconsistent state.
* - ResourceNotFoundException The resource could not be found.
* - ThrottlingException Amazon Lookout for Vision is temporarily unable to process the request. Try your
* call again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - LookoutVisionException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample LookoutVisionAsyncClient.ListDatasetEntries
* @see AWS API Documentation
*/
@Override
public CompletableFuture listDatasetEntries(ListDatasetEntriesRequest listDatasetEntriesRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listDatasetEntriesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "LookoutVision");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListDatasetEntries");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListDatasetEntriesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListDatasetEntries")
.withMarshaller(new ListDatasetEntriesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listDatasetEntriesRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = listDatasetEntriesRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the JSON Lines within a dataset. An Amazon Lookout for Vision JSON Line contains the anomaly information
* for a single image, including the image location and the assigned label.
*
*
* This operation requires permissions to perform the lookoutvision:ListDatasetEntries
operation.
*
*
*
* This is a variant of
* {@link #listDatasetEntries(software.amazon.awssdk.services.lookoutvision.model.ListDatasetEntriesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.lookoutvision.paginators.ListDatasetEntriesPublisher publisher = client.listDatasetEntriesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.lookoutvision.paginators.ListDatasetEntriesPublisher publisher = client.listDatasetEntriesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.lookoutvision.model.ListDatasetEntriesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDatasetEntries(software.amazon.awssdk.services.lookoutvision.model.ListDatasetEntriesRequest)}
* operation.
*
*
* @param listDatasetEntriesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You are not authorized to perform the action.
* - InternalServerException Amazon Lookout for Vision experienced a service issue. Try your call again.
* - ValidationException An input validation error occured. For example, invalid characters in a project
* name, or if a pagination token is invalid.
* - ConflictException The update or deletion of a resource caused an inconsistent state.
* - ResourceNotFoundException The resource could not be found.
* - ThrottlingException Amazon Lookout for Vision is temporarily unable to process the request. Try your
* call again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - LookoutVisionException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample LookoutVisionAsyncClient.ListDatasetEntries
* @see AWS API Documentation
*/
public ListDatasetEntriesPublisher listDatasetEntriesPaginator(ListDatasetEntriesRequest listDatasetEntriesRequest) {
return new ListDatasetEntriesPublisher(this, applyPaginatorUserAgent(listDatasetEntriesRequest));
}
/**
*
* Lists the versions of a model in an Amazon Lookout for Vision project.
*
*
* This operation requires permissions to perform the lookoutvision:ListModels
operation.
*
*
* @param listModelsRequest
* @return A Java Future containing the result of the ListModels operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You are not authorized to perform the action.
* - InternalServerException Amazon Lookout for Vision experienced a service issue. Try your call again.
* - ValidationException An input validation error occured. For example, invalid characters in a project
* name, or if a pagination token is invalid.
* - ConflictException The update or deletion of a resource caused an inconsistent state.
* - ResourceNotFoundException The resource could not be found.
* - ThrottlingException Amazon Lookout for Vision is temporarily unable to process the request. Try your
* call again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - LookoutVisionException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample LookoutVisionAsyncClient.ListModels
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listModels(ListModelsRequest listModelsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listModelsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "LookoutVision");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListModels");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListModelsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("ListModels")
.withMarshaller(new ListModelsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listModelsRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = listModelsRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the versions of a model in an Amazon Lookout for Vision project.
*
*
* This operation requires permissions to perform the lookoutvision:ListModels
operation.
*
*
*
* This is a variant of {@link #listModels(software.amazon.awssdk.services.lookoutvision.model.ListModelsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.lookoutvision.paginators.ListModelsPublisher publisher = client.listModelsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.lookoutvision.paginators.ListModelsPublisher publisher = client.listModelsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.lookoutvision.model.ListModelsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listModels(software.amazon.awssdk.services.lookoutvision.model.ListModelsRequest)} operation.
*
*
* @param listModelsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You are not authorized to perform the action.
* - InternalServerException Amazon Lookout for Vision experienced a service issue. Try your call again.
* - ValidationException An input validation error occured. For example, invalid characters in a project
* name, or if a pagination token is invalid.
* - ConflictException The update or deletion of a resource caused an inconsistent state.
* - ResourceNotFoundException The resource could not be found.
* - ThrottlingException Amazon Lookout for Vision is temporarily unable to process the request. Try your
* call again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - LookoutVisionException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample LookoutVisionAsyncClient.ListModels
* @see AWS API
* Documentation
*/
public ListModelsPublisher listModelsPaginator(ListModelsRequest listModelsRequest) {
return new ListModelsPublisher(this, applyPaginatorUserAgent(listModelsRequest));
}
/**
*
* Lists the Amazon Lookout for Vision projects in your AWS account.
*
*
* This operation requires permissions to perform the lookoutvision:ListProjects
operation.
*
*
* @param listProjectsRequest
* @return A Java Future containing the result of the ListProjects operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You are not authorized to perform the action.
* - InternalServerException Amazon Lookout for Vision experienced a service issue. Try your call again.
* - ValidationException An input validation error occured. For example, invalid characters in a project
* name, or if a pagination token is invalid.
* - ConflictException The update or deletion of a resource caused an inconsistent state.
* - ResourceNotFoundException The resource could not be found.
* - ThrottlingException Amazon Lookout for Vision is temporarily unable to process the request. Try your
* call again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - LookoutVisionException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample LookoutVisionAsyncClient.ListProjects
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listProjects(ListProjectsRequest listProjectsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listProjectsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "LookoutVision");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListProjects");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListProjectsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListProjects").withMarshaller(new ListProjectsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listProjectsRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = listProjectsRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the Amazon Lookout for Vision projects in your AWS account.
*
*
* This operation requires permissions to perform the lookoutvision:ListProjects
operation.
*
*
*
* This is a variant of
* {@link #listProjects(software.amazon.awssdk.services.lookoutvision.model.ListProjectsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.lookoutvision.paginators.ListProjectsPublisher publisher = client.listProjectsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.lookoutvision.paginators.ListProjectsPublisher publisher = client.listProjectsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.lookoutvision.model.ListProjectsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listProjects(software.amazon.awssdk.services.lookoutvision.model.ListProjectsRequest)} operation.
*
*
* @param listProjectsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You are not authorized to perform the action.
* - InternalServerException Amazon Lookout for Vision experienced a service issue. Try your call again.
* - ValidationException An input validation error occured. For example, invalid characters in a project
* name, or if a pagination token is invalid.
* - ConflictException The update or deletion of a resource caused an inconsistent state.
* - ResourceNotFoundException The resource could not be found.
* - ThrottlingException Amazon Lookout for Vision is temporarily unable to process the request. Try your
* call again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - LookoutVisionException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample LookoutVisionAsyncClient.ListProjects
* @see AWS
* API Documentation
*/
public ListProjectsPublisher listProjectsPaginator(ListProjectsRequest listProjectsRequest) {
return new ListProjectsPublisher(this, applyPaginatorUserAgent(listProjectsRequest));
}
/**
*
* Returns a list of tags attached to the specified Amazon Lookout for Vision model.
*
*
* This operation requires permissions to perform the lookoutvision:ListTagsForResource
operation.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You are not authorized to perform the action.
* - InternalServerException Amazon Lookout for Vision experienced a service issue. Try your call again.
* - ValidationException An input validation error occured. For example, invalid characters in a project
* name, or if a pagination token is invalid.
* - ConflictException The update or deletion of a resource caused an inconsistent state.
* - ResourceNotFoundException The resource could not be found.
* - ThrottlingException Amazon Lookout for Vision is temporarily unable to process the request. Try your
* call again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - LookoutVisionException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample LookoutVisionAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
@Override
public CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listTagsForResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "LookoutVision");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListTagsForResource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListTagsForResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListTagsForResource")
.withMarshaller(new ListTagsForResourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listTagsForResourceRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = listTagsForResourceRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Starts the running of the version of an Amazon Lookout for Vision model. Starting a model takes a while to
* complete. To check the current state of the model, use DescribeModel.
*
*
* A model is ready to use when its status is HOSTED
.
*
*
* Once the model is running, you can detect custom labels in new images by calling DetectAnomalies.
*
*
*
* You are charged for the amount of time that the model is running. To stop a running model, call StopModel.
*
*
*
* This operation requires permissions to perform the lookoutvision:StartModel
operation.
*
*
* @param startModelRequest
* @return A Java Future containing the result of the StartModel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You are not authorized to perform the action.
* - InternalServerException Amazon Lookout for Vision experienced a service issue. Try your call again.
* - ValidationException An input validation error occured. For example, invalid characters in a project
* name, or if a pagination token is invalid.
* - ConflictException The update or deletion of a resource caused an inconsistent state.
* - ResourceNotFoundException The resource could not be found.
* - ThrottlingException Amazon Lookout for Vision is temporarily unable to process the request. Try your
* call again.
* - ServiceQuotaExceededException A service quota was exceeded the allowed limit. For more information,
* see Limits in Amazon Lookout for Vision in the Amazon Lookout for Vision Developer Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - LookoutVisionException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample LookoutVisionAsyncClient.StartModel
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture startModel(StartModelRequest startModelRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, startModelRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "LookoutVision");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StartModel");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
StartModelResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("StartModel")
.withMarshaller(new StartModelRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(startModelRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = startModelRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Stops the hosting of a running model. The operation might take a while to complete. To check the current status,
* call DescribeModel.
*
*
* After the model hosting stops, the Status
of the model is TRAINED
.
*
*
* This operation requires permissions to perform the lookoutvision:StopModel
operation.
*
*
* @param stopModelRequest
* @return A Java Future containing the result of the StopModel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You are not authorized to perform the action.
* - InternalServerException Amazon Lookout for Vision experienced a service issue. Try your call again.
* - ValidationException An input validation error occured. For example, invalid characters in a project
* name, or if a pagination token is invalid.
* - ConflictException The update or deletion of a resource caused an inconsistent state.
* - ResourceNotFoundException The resource could not be found.
* - ThrottlingException Amazon Lookout for Vision is temporarily unable to process the request. Try your
* call again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - LookoutVisionException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample LookoutVisionAsyncClient.StopModel
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture stopModel(StopModelRequest stopModelRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, stopModelRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "LookoutVision");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StopModel");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
StopModelResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("StopModel")
.withMarshaller(new StopModelRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withMetricCollector(apiCallMetricCollector)
.withInput(stopModelRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = stopModelRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Adds one or more key-value tags to an Amazon Lookout for Vision model. For more information, see Tagging a
* model in the Amazon Lookout for Vision Developer Guide.
*
*
* This operation requires permissions to perform the lookoutvision:TagResource
operation.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You are not authorized to perform the action.
* - InternalServerException Amazon Lookout for Vision experienced a service issue. Try your call again.
* - ValidationException An input validation error occured. For example, invalid characters in a project
* name, or if a pagination token is invalid.
* - ConflictException The update or deletion of a resource caused an inconsistent state.
* - ResourceNotFoundException The resource could not be found.
* - ThrottlingException Amazon Lookout for Vision is temporarily unable to process the request. Try your
* call again.
* - ServiceQuotaExceededException A service quota was exceeded the allowed limit. For more information,
* see Limits in Amazon Lookout for Vision in the Amazon Lookout for Vision Developer Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - LookoutVisionException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample LookoutVisionAsyncClient.TagResource
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, tagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "LookoutVision");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "TagResource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
TagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("TagResource").withMarshaller(new TagResourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(tagResourceRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = tagResourceRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Removes one or more tags from an Amazon Lookout for Vision model. For more information, see Tagging a
* model in the Amazon Lookout for Vision Developer Guide.
*
*
* This operation requires permissions to perform the lookoutvision:UntagResource
operation.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You are not authorized to perform the action.
* - InternalServerException Amazon Lookout for Vision experienced a service issue. Try your call again.
* - ValidationException An input validation error occured. For example, invalid characters in a project
* name, or if a pagination token is invalid.
* - ConflictException The update or deletion of a resource caused an inconsistent state.
* - ResourceNotFoundException The resource could not be found.
* - ThrottlingException Amazon Lookout for Vision is temporarily unable to process the request. Try your
* call again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - LookoutVisionException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample LookoutVisionAsyncClient.UntagResource
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, untagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "LookoutVision");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UntagResource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UntagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("UntagResource")
.withMarshaller(new UntagResourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(untagResourceRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = untagResourceRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Adds one or more JSON Line entries to a dataset. A JSON Line includes information about an image used for
* training or testing an Amazon Lookout for Vision model. The following is an example JSON Line.
*
*
* Updating a dataset might take a while to complete. To check the current status, call DescribeDataset and
* check the Status
field in the response.
*
*
* This operation requires permissions to perform the lookoutvision:UpdateDatasetEntries
operation.
*
*
* @param updateDatasetEntriesRequest
* @return A Java Future containing the result of the UpdateDatasetEntries operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You are not authorized to perform the action.
* - InternalServerException Amazon Lookout for Vision experienced a service issue. Try your call again.
* - ValidationException An input validation error occured. For example, invalid characters in a project
* name, or if a pagination token is invalid.
* - ConflictException The update or deletion of a resource caused an inconsistent state.
* - ResourceNotFoundException The resource could not be found.
* - ThrottlingException Amazon Lookout for Vision is temporarily unable to process the request. Try your
* call again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - LookoutVisionException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample LookoutVisionAsyncClient.UpdateDatasetEntries
* @see AWS API Documentation
*/
@Override
public CompletableFuture updateDatasetEntries(
UpdateDatasetEntriesRequest updateDatasetEntriesRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateDatasetEntriesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "LookoutVision");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateDatasetEntries");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, UpdateDatasetEntriesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("UpdateDatasetEntries")
.withMarshaller(new UpdateDatasetEntriesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(updateDatasetEntriesRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = updateDatasetEntriesRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
@Override
public void close() {
clientHandler.close();
}
private > T init(T builder) {
return builder
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(LookoutVisionException::builder)
.protocol(AwsJsonProtocol.REST_JSON)
.protocolVersion("1.1")
.registerModeledException(
ExceptionMetadata.builder().errorCode("AccessDeniedException")
.exceptionBuilderSupplier(AccessDeniedException::builder).httpStatusCode(403).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ConflictException")
.exceptionBuilderSupplier(ConflictException::builder).httpStatusCode(409).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ResourceNotFoundException")
.exceptionBuilderSupplier(ResourceNotFoundException::builder).httpStatusCode(404).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ThrottlingException")
.exceptionBuilderSupplier(ThrottlingException::builder).httpStatusCode(429).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ValidationException")
.exceptionBuilderSupplier(ValidationException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ServiceQuotaExceededException")
.exceptionBuilderSupplier(ServiceQuotaExceededException::builder).httpStatusCode(402).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InternalServerException")
.exceptionBuilderSupplier(InternalServerException::builder).httpStatusCode(500).build());
}
private static List resolveMetricPublishers(SdkClientConfiguration clientConfiguration,
RequestOverrideConfiguration requestOverrideConfiguration) {
List publishers = null;
if (requestOverrideConfiguration != null) {
publishers = requestOverrideConfiguration.metricPublishers();
}
if (publishers == null || publishers.isEmpty()) {
publishers = clientConfiguration.option(SdkClientOption.METRIC_PUBLISHERS);
}
if (publishers == null) {
publishers = Collections.emptyList();
}
return publishers;
}
private T applyPaginatorUserAgent(T request) {
Consumer userAgentApplier = b -> b.addApiName(ApiName.builder()
.version(VersionInfo.SDK_VERSION).name("PAGINATED").build());
AwsRequestOverrideConfiguration overrideConfiguration = request.overrideConfiguration()
.map(c -> c.toBuilder().applyMutation(userAgentApplier).build())
.orElse((AwsRequestOverrideConfiguration.builder().applyMutation(userAgentApplier).build()));
return (T) request.toBuilder().overrideConfiguration(overrideConfiguration).build();
}
private T applySignerOverride(T request, Signer signer) {
if (request.overrideConfiguration().flatMap(c -> c.signer()).isPresent()) {
return request;
}
Consumer signerOverride = b -> b.signer(signer).build();
AwsRequestOverrideConfiguration overrideConfiguration = request.overrideConfiguration()
.map(c -> c.toBuilder().applyMutation(signerOverride).build())
.orElse((AwsRequestOverrideConfiguration.builder().applyMutation(signerOverride).build()));
return (T) request.toBuilder().overrideConfiguration(overrideConfiguration).build();
}
private static boolean isSignerOverridden(SdkClientConfiguration clientConfiguration) {
return Boolean.TRUE.equals(clientConfiguration.option(SdkClientOption.SIGNER_OVERRIDDEN));
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata) {
return protocolFactory.createErrorResponseHandler(operationMetadata);
}
}