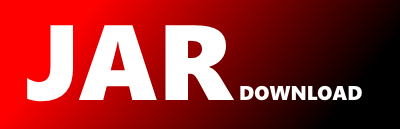
software.amazon.awssdk.services.lookoutvision.model.DetectAnomalyResult Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.lookoutvision.model;
import java.io.Serializable;
import java.nio.ByteBuffer;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkBytes;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The prediction results from a call to DetectAnomalies. DetectAnomalyResult
includes
* classification information for the prediction (IsAnomalous
and Confidence
). If the model
* you use is an image segementation model, DetectAnomalyResult
also includes segmentation information (
* Anomalies
and AnomalyMask
). Classification information is calculated separately from
* segmentation information and you shouldn't assume a relationship between them.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DetectAnomalyResult implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField SOURCE_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("Source").getter(getter(DetectAnomalyResult::source)).setter(setter(Builder::source))
.constructor(ImageSource::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Source").build()).build();
private static final SdkField IS_ANOMALOUS_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("IsAnomalous").getter(getter(DetectAnomalyResult::isAnomalous)).setter(setter(Builder::isAnomalous))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IsAnomalous").build()).build();
private static final SdkField CONFIDENCE_FIELD = SdkField. builder(MarshallingType.FLOAT)
.memberName("Confidence").getter(getter(DetectAnomalyResult::confidence)).setter(setter(Builder::confidence))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Confidence").build()).build();
private static final SdkField> ANOMALIES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Anomalies")
.getter(getter(DetectAnomalyResult::anomalies))
.setter(setter(Builder::anomalies))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Anomalies").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Anomaly::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField ANOMALY_MASK_FIELD = SdkField. builder(MarshallingType.SDK_BYTES)
.memberName("AnomalyMask").getter(getter(DetectAnomalyResult::anomalyMask)).setter(setter(Builder::anomalyMask))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AnomalyMask").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(SOURCE_FIELD,
IS_ANOMALOUS_FIELD, CONFIDENCE_FIELD, ANOMALIES_FIELD, ANOMALY_MASK_FIELD));
private static final long serialVersionUID = 1L;
private final ImageSource source;
private final Boolean isAnomalous;
private final Float confidence;
private final List anomalies;
private final SdkBytes anomalyMask;
private DetectAnomalyResult(BuilderImpl builder) {
this.source = builder.source;
this.isAnomalous = builder.isAnomalous;
this.confidence = builder.confidence;
this.anomalies = builder.anomalies;
this.anomalyMask = builder.anomalyMask;
}
/**
*
* The source of the image that was analyzed. direct
means that the images was supplied from the local
* computer. No other values are supported.
*
*
* @return The source of the image that was analyzed. direct
means that the images was supplied from
* the local computer. No other values are supported.
*/
public final ImageSource source() {
return source;
}
/**
*
* True if Amazon Lookout for Vision classifies the image as containing an anomaly, otherwise false.
*
*
* @return True if Amazon Lookout for Vision classifies the image as containing an anomaly, otherwise false.
*/
public final Boolean isAnomalous() {
return isAnomalous;
}
/**
*
* The confidence that Lookout for Vision has in the accuracy of the classification in IsAnomalous
.
*
*
* @return The confidence that Lookout for Vision has in the accuracy of the classification in
* IsAnomalous
.
*/
public final Float confidence() {
return confidence;
}
/**
* For responses, this returns true if the service returned a value for the Anomalies property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasAnomalies() {
return anomalies != null && !(anomalies instanceof SdkAutoConstructList);
}
/**
*
* If the model is an image segmentation model, Anomalies
contains a list of anomaly types found in the
* image. There is one entry for each type of anomaly found (even if multiple instances of an anomaly type exist on
* the image). The first element in the list is always an anomaly type representing the image background
* ('background') and shouldn't be considered an anomaly. Amazon Lookout for Vision automatically add the background
* anomaly type to the response, and you don't need to declare a background anomaly type in your dataset.
*
*
* If the list has one entry ('background'), no anomalies were found on the image.
*
*
*
* An image classification model doesn't return an Anomalies
list.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAnomalies} method.
*
*
* @return If the model is an image segmentation model, Anomalies
contains a list of anomaly types
* found in the image. There is one entry for each type of anomaly found (even if multiple instances of an
* anomaly type exist on the image). The first element in the list is always an anomaly type representing
* the image background ('background') and shouldn't be considered an anomaly. Amazon Lookout for Vision
* automatically add the background anomaly type to the response, and you don't need to declare a background
* anomaly type in your dataset.
*
* If the list has one entry ('background'), no anomalies were found on the image.
*
*
*
* An image classification model doesn't return an Anomalies
list.
*/
public final List anomalies() {
return anomalies;
}
/**
*
* If the model is an image segmentation model, AnomalyMask
contains pixel masks that covers all
* anomaly types found on the image. Each anomaly type has a different mask color. To map a color to an anomaly
* type, see the color
field of the PixelAnomaly object.
*
*
* An image classification model doesn't return an Anomalies
list.
*
*
* @return If the model is an image segmentation model, AnomalyMask
contains pixel masks that covers
* all anomaly types found on the image. Each anomaly type has a different mask color. To map a color to an
* anomaly type, see the color
field of the PixelAnomaly object.
*
* An image classification model doesn't return an Anomalies
list.
*/
public final SdkBytes anomalyMask() {
return anomalyMask;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(source());
hashCode = 31 * hashCode + Objects.hashCode(isAnomalous());
hashCode = 31 * hashCode + Objects.hashCode(confidence());
hashCode = 31 * hashCode + Objects.hashCode(hasAnomalies() ? anomalies() : null);
hashCode = 31 * hashCode + Objects.hashCode(anomalyMask());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DetectAnomalyResult)) {
return false;
}
DetectAnomalyResult other = (DetectAnomalyResult) obj;
return Objects.equals(source(), other.source()) && Objects.equals(isAnomalous(), other.isAnomalous())
&& Objects.equals(confidence(), other.confidence()) && hasAnomalies() == other.hasAnomalies()
&& Objects.equals(anomalies(), other.anomalies()) && Objects.equals(anomalyMask(), other.anomalyMask());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DetectAnomalyResult").add("Source", source()).add("IsAnomalous", isAnomalous())
.add("Confidence", confidence()).add("Anomalies", hasAnomalies() ? anomalies() : null)
.add("AnomalyMask", anomalyMask()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Source":
return Optional.ofNullable(clazz.cast(source()));
case "IsAnomalous":
return Optional.ofNullable(clazz.cast(isAnomalous()));
case "Confidence":
return Optional.ofNullable(clazz.cast(confidence()));
case "Anomalies":
return Optional.ofNullable(clazz.cast(anomalies()));
case "AnomalyMask":
return Optional.ofNullable(clazz.cast(anomalyMask()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function