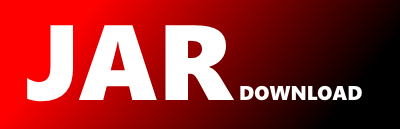
software.amazon.awssdk.services.lookoutvision.model.ModelPackagingDescription Maven / Gradle / Ivy
Show all versions of lookoutvision Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.lookoutvision.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Information about a model packaging job. For more information, see DescribeModelPackagingJob.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ModelPackagingDescription implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField JOB_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("JobName").getter(getter(ModelPackagingDescription::jobName)).setter(setter(Builder::jobName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("JobName").build()).build();
private static final SdkField PROJECT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ProjectName").getter(getter(ModelPackagingDescription::projectName))
.setter(setter(Builder::projectName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ProjectName").build()).build();
private static final SdkField MODEL_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ModelVersion").getter(getter(ModelPackagingDescription::modelVersion))
.setter(setter(Builder::modelVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ModelVersion").build()).build();
private static final SdkField MODEL_PACKAGING_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("ModelPackagingConfiguration")
.getter(getter(ModelPackagingDescription::modelPackagingConfiguration))
.setter(setter(Builder::modelPackagingConfiguration))
.constructor(ModelPackagingConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ModelPackagingConfiguration")
.build()).build();
private static final SdkField MODEL_PACKAGING_JOB_DESCRIPTION_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ModelPackagingJobDescription")
.getter(getter(ModelPackagingDescription::modelPackagingJobDescription))
.setter(setter(Builder::modelPackagingJobDescription))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ModelPackagingJobDescription")
.build()).build();
private static final SdkField MODEL_PACKAGING_METHOD_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ModelPackagingMethod").getter(getter(ModelPackagingDescription::modelPackagingMethod))
.setter(setter(Builder::modelPackagingMethod))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ModelPackagingMethod").build())
.build();
private static final SdkField MODEL_PACKAGING_OUTPUT_DETAILS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("ModelPackagingOutputDetails")
.getter(getter(ModelPackagingDescription::modelPackagingOutputDetails))
.setter(setter(Builder::modelPackagingOutputDetails))
.constructor(ModelPackagingOutputDetails::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ModelPackagingOutputDetails")
.build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(ModelPackagingDescription::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField STATUS_MESSAGE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StatusMessage").getter(getter(ModelPackagingDescription::statusMessage))
.setter(setter(Builder::statusMessage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StatusMessage").build()).build();
private static final SdkField CREATION_TIMESTAMP_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreationTimestamp").getter(getter(ModelPackagingDescription::creationTimestamp))
.setter(setter(Builder::creationTimestamp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreationTimestamp").build()).build();
private static final SdkField LAST_UPDATED_TIMESTAMP_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("LastUpdatedTimestamp").getter(getter(ModelPackagingDescription::lastUpdatedTimestamp))
.setter(setter(Builder::lastUpdatedTimestamp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastUpdatedTimestamp").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(JOB_NAME_FIELD,
PROJECT_NAME_FIELD, MODEL_VERSION_FIELD, MODEL_PACKAGING_CONFIGURATION_FIELD, MODEL_PACKAGING_JOB_DESCRIPTION_FIELD,
MODEL_PACKAGING_METHOD_FIELD, MODEL_PACKAGING_OUTPUT_DETAILS_FIELD, STATUS_FIELD, STATUS_MESSAGE_FIELD,
CREATION_TIMESTAMP_FIELD, LAST_UPDATED_TIMESTAMP_FIELD));
private static final long serialVersionUID = 1L;
private final String jobName;
private final String projectName;
private final String modelVersion;
private final ModelPackagingConfiguration modelPackagingConfiguration;
private final String modelPackagingJobDescription;
private final String modelPackagingMethod;
private final ModelPackagingOutputDetails modelPackagingOutputDetails;
private final String status;
private final String statusMessage;
private final Instant creationTimestamp;
private final Instant lastUpdatedTimestamp;
private ModelPackagingDescription(BuilderImpl builder) {
this.jobName = builder.jobName;
this.projectName = builder.projectName;
this.modelVersion = builder.modelVersion;
this.modelPackagingConfiguration = builder.modelPackagingConfiguration;
this.modelPackagingJobDescription = builder.modelPackagingJobDescription;
this.modelPackagingMethod = builder.modelPackagingMethod;
this.modelPackagingOutputDetails = builder.modelPackagingOutputDetails;
this.status = builder.status;
this.statusMessage = builder.statusMessage;
this.creationTimestamp = builder.creationTimestamp;
this.lastUpdatedTimestamp = builder.lastUpdatedTimestamp;
}
/**
*
* The name of the model packaging job.
*
*
* @return The name of the model packaging job.
*/
public final String jobName() {
return jobName;
}
/**
*
* The name of the project that's associated with a model that's in the model package.
*
*
* @return The name of the project that's associated with a model that's in the model package.
*/
public final String projectName() {
return projectName;
}
/**
*
* The version of the model used in the model packaging job.
*
*
* @return The version of the model used in the model packaging job.
*/
public final String modelVersion() {
return modelVersion;
}
/**
*
* The configuration information used in the model packaging job.
*
*
* @return The configuration information used in the model packaging job.
*/
public final ModelPackagingConfiguration modelPackagingConfiguration() {
return modelPackagingConfiguration;
}
/**
*
* The description for the model packaging job.
*
*
* @return The description for the model packaging job.
*/
public final String modelPackagingJobDescription() {
return modelPackagingJobDescription;
}
/**
*
* The AWS service used to package the job. Currently Lookout for Vision can package jobs with AWS IoT Greengrass.
*
*
* @return The AWS service used to package the job. Currently Lookout for Vision can package jobs with AWS IoT
* Greengrass.
*/
public final String modelPackagingMethod() {
return modelPackagingMethod;
}
/**
*
* Information about the output of the model packaging job. For more information, see
* DescribeModelPackagingJob.
*
*
* @return Information about the output of the model packaging job. For more information, see
* DescribeModelPackagingJob.
*/
public final ModelPackagingOutputDetails modelPackagingOutputDetails() {
return modelPackagingOutputDetails;
}
/**
*
* The status of the model packaging job.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ModelPackagingJobStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return The status of the model packaging job.
* @see ModelPackagingJobStatus
*/
public final ModelPackagingJobStatus status() {
return ModelPackagingJobStatus.fromValue(status);
}
/**
*
* The status of the model packaging job.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ModelPackagingJobStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return The status of the model packaging job.
* @see ModelPackagingJobStatus
*/
public final String statusAsString() {
return status;
}
/**
*
* The status message for the model packaging job.
*
*
* @return The status message for the model packaging job.
*/
public final String statusMessage() {
return statusMessage;
}
/**
*
* The Unix timestamp for the time and date that the model packaging job was created.
*
*
* @return The Unix timestamp for the time and date that the model packaging job was created.
*/
public final Instant creationTimestamp() {
return creationTimestamp;
}
/**
*
* The Unix timestamp for the time and date that the model packaging job was last updated.
*
*
* @return The Unix timestamp for the time and date that the model packaging job was last updated.
*/
public final Instant lastUpdatedTimestamp() {
return lastUpdatedTimestamp;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(jobName());
hashCode = 31 * hashCode + Objects.hashCode(projectName());
hashCode = 31 * hashCode + Objects.hashCode(modelVersion());
hashCode = 31 * hashCode + Objects.hashCode(modelPackagingConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(modelPackagingJobDescription());
hashCode = 31 * hashCode + Objects.hashCode(modelPackagingMethod());
hashCode = 31 * hashCode + Objects.hashCode(modelPackagingOutputDetails());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(statusMessage());
hashCode = 31 * hashCode + Objects.hashCode(creationTimestamp());
hashCode = 31 * hashCode + Objects.hashCode(lastUpdatedTimestamp());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ModelPackagingDescription)) {
return false;
}
ModelPackagingDescription other = (ModelPackagingDescription) obj;
return Objects.equals(jobName(), other.jobName()) && Objects.equals(projectName(), other.projectName())
&& Objects.equals(modelVersion(), other.modelVersion())
&& Objects.equals(modelPackagingConfiguration(), other.modelPackagingConfiguration())
&& Objects.equals(modelPackagingJobDescription(), other.modelPackagingJobDescription())
&& Objects.equals(modelPackagingMethod(), other.modelPackagingMethod())
&& Objects.equals(modelPackagingOutputDetails(), other.modelPackagingOutputDetails())
&& Objects.equals(statusAsString(), other.statusAsString())
&& Objects.equals(statusMessage(), other.statusMessage())
&& Objects.equals(creationTimestamp(), other.creationTimestamp())
&& Objects.equals(lastUpdatedTimestamp(), other.lastUpdatedTimestamp());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ModelPackagingDescription").add("JobName", jobName()).add("ProjectName", projectName())
.add("ModelVersion", modelVersion()).add("ModelPackagingConfiguration", modelPackagingConfiguration())
.add("ModelPackagingJobDescription", modelPackagingJobDescription())
.add("ModelPackagingMethod", modelPackagingMethod())
.add("ModelPackagingOutputDetails", modelPackagingOutputDetails()).add("Status", statusAsString())
.add("StatusMessage", statusMessage()).add("CreationTimestamp", creationTimestamp())
.add("LastUpdatedTimestamp", lastUpdatedTimestamp()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "JobName":
return Optional.ofNullable(clazz.cast(jobName()));
case "ProjectName":
return Optional.ofNullable(clazz.cast(projectName()));
case "ModelVersion":
return Optional.ofNullable(clazz.cast(modelVersion()));
case "ModelPackagingConfiguration":
return Optional.ofNullable(clazz.cast(modelPackagingConfiguration()));
case "ModelPackagingJobDescription":
return Optional.ofNullable(clazz.cast(modelPackagingJobDescription()));
case "ModelPackagingMethod":
return Optional.ofNullable(clazz.cast(modelPackagingMethod()));
case "ModelPackagingOutputDetails":
return Optional.ofNullable(clazz.cast(modelPackagingOutputDetails()));
case "Status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "StatusMessage":
return Optional.ofNullable(clazz.cast(statusMessage()));
case "CreationTimestamp":
return Optional.ofNullable(clazz.cast(creationTimestamp()));
case "LastUpdatedTimestamp":
return Optional.ofNullable(clazz.cast(lastUpdatedTimestamp()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function