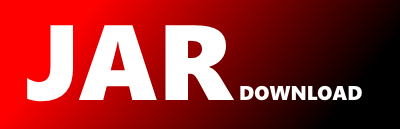
software.amazon.awssdk.services.lookoutvision.model.TargetPlatform Maven / Gradle / Ivy
Show all versions of lookoutvision Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.lookoutvision.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The platform on which a model runs on an AWS IoT Greengrass core device.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class TargetPlatform implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField OS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Os")
.getter(getter(TargetPlatform::osAsString)).setter(setter(Builder::os))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Os").build()).build();
private static final SdkField ARCH_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Arch")
.getter(getter(TargetPlatform::archAsString)).setter(setter(Builder::arch))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Arch").build()).build();
private static final SdkField ACCELERATOR_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Accelerator").getter(getter(TargetPlatform::acceleratorAsString)).setter(setter(Builder::accelerator))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Accelerator").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(OS_FIELD, ARCH_FIELD,
ACCELERATOR_FIELD));
private static final long serialVersionUID = 1L;
private final String os;
private final String arch;
private final String accelerator;
private TargetPlatform(BuilderImpl builder) {
this.os = builder.os;
this.arch = builder.arch;
this.accelerator = builder.accelerator;
}
/**
*
* The target operating system for the model. Linux is the only operating system that is currently supported.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #os} will return
* {@link TargetPlatformOs#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #osAsString}.
*
*
* @return The target operating system for the model. Linux is the only operating system that is currently
* supported.
* @see TargetPlatformOs
*/
public final TargetPlatformOs os() {
return TargetPlatformOs.fromValue(os);
}
/**
*
* The target operating system for the model. Linux is the only operating system that is currently supported.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #os} will return
* {@link TargetPlatformOs#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #osAsString}.
*
*
* @return The target operating system for the model. Linux is the only operating system that is currently
* supported.
* @see TargetPlatformOs
*/
public final String osAsString() {
return os;
}
/**
*
* The target architecture for the model. The currently supported architectures are X86_64 (64-bit version of the
* x86 instruction set) and ARM_64 (ARMv8 64-bit CPU).
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #arch} will return
* {@link TargetPlatformArch#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #archAsString}.
*
*
* @return The target architecture for the model. The currently supported architectures are X86_64 (64-bit version
* of the x86 instruction set) and ARM_64 (ARMv8 64-bit CPU).
* @see TargetPlatformArch
*/
public final TargetPlatformArch arch() {
return TargetPlatformArch.fromValue(arch);
}
/**
*
* The target architecture for the model. The currently supported architectures are X86_64 (64-bit version of the
* x86 instruction set) and ARM_64 (ARMv8 64-bit CPU).
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #arch} will return
* {@link TargetPlatformArch#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #archAsString}.
*
*
* @return The target architecture for the model. The currently supported architectures are X86_64 (64-bit version
* of the x86 instruction set) and ARM_64 (ARMv8 64-bit CPU).
* @see TargetPlatformArch
*/
public final String archAsString() {
return arch;
}
/**
*
* The target accelerator for the model. Currently, Amazon Lookout for Vision only supports NVIDIA (Nvidia graphics
* processing unit) and CPU accelerators. If you specify NVIDIA as an accelerator, you must also specify the
* gpu-code
, trt-ver
, and cuda-ver
compiler options. If you don't specify an
* accelerator, Lookout for Vision uses the CPU for compilation and we highly recommend that you use the
* GreengrassConfiguration$CompilerOptions field. For example, you can use the following compiler options for
* CPU:
*
*
* -
*
* mcpu
: CPU micro-architecture. For example, {'mcpu': 'skylake-avx512'}
*
*
* -
*
* mattr
: CPU flags. For example, {'mattr': ['+neon', '+vfpv4']}
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #accelerator} will
* return {@link TargetPlatformAccelerator#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #acceleratorAsString}.
*
*
* @return The target accelerator for the model. Currently, Amazon Lookout for Vision only supports NVIDIA (Nvidia
* graphics processing unit) and CPU accelerators. If you specify NVIDIA as an accelerator, you must also
* specify the gpu-code
, trt-ver
, and cuda-ver
compiler options. If
* you don't specify an accelerator, Lookout for Vision uses the CPU for compilation and we highly recommend
* that you use the GreengrassConfiguration$CompilerOptions field. For example, you can use the
* following compiler options for CPU:
*
* -
*
* mcpu
: CPU micro-architecture. For example, {'mcpu': 'skylake-avx512'}
*
*
* -
*
* mattr
: CPU flags. For example, {'mattr': ['+neon', '+vfpv4']}
*
*
* @see TargetPlatformAccelerator
*/
public final TargetPlatformAccelerator accelerator() {
return TargetPlatformAccelerator.fromValue(accelerator);
}
/**
*
* The target accelerator for the model. Currently, Amazon Lookout for Vision only supports NVIDIA (Nvidia graphics
* processing unit) and CPU accelerators. If you specify NVIDIA as an accelerator, you must also specify the
* gpu-code
, trt-ver
, and cuda-ver
compiler options. If you don't specify an
* accelerator, Lookout for Vision uses the CPU for compilation and we highly recommend that you use the
* GreengrassConfiguration$CompilerOptions field. For example, you can use the following compiler options for
* CPU:
*
*
* -
*
* mcpu
: CPU micro-architecture. For example, {'mcpu': 'skylake-avx512'}
*
*
* -
*
* mattr
: CPU flags. For example, {'mattr': ['+neon', '+vfpv4']}
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #accelerator} will
* return {@link TargetPlatformAccelerator#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #acceleratorAsString}.
*
*
* @return The target accelerator for the model. Currently, Amazon Lookout for Vision only supports NVIDIA (Nvidia
* graphics processing unit) and CPU accelerators. If you specify NVIDIA as an accelerator, you must also
* specify the gpu-code
, trt-ver
, and cuda-ver
compiler options. If
* you don't specify an accelerator, Lookout for Vision uses the CPU for compilation and we highly recommend
* that you use the GreengrassConfiguration$CompilerOptions field. For example, you can use the
* following compiler options for CPU:
*
* -
*
* mcpu
: CPU micro-architecture. For example, {'mcpu': 'skylake-avx512'}
*
*
* -
*
* mattr
: CPU flags. For example, {'mattr': ['+neon', '+vfpv4']}
*
*
* @see TargetPlatformAccelerator
*/
public final String acceleratorAsString() {
return accelerator;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(osAsString());
hashCode = 31 * hashCode + Objects.hashCode(archAsString());
hashCode = 31 * hashCode + Objects.hashCode(acceleratorAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof TargetPlatform)) {
return false;
}
TargetPlatform other = (TargetPlatform) obj;
return Objects.equals(osAsString(), other.osAsString()) && Objects.equals(archAsString(), other.archAsString())
&& Objects.equals(acceleratorAsString(), other.acceleratorAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("TargetPlatform").add("Os", osAsString()).add("Arch", archAsString())
.add("Accelerator", acceleratorAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Os":
return Optional.ofNullable(clazz.cast(osAsString()));
case "Arch":
return Optional.ofNullable(clazz.cast(archAsString()));
case "Accelerator":
return Optional.ofNullable(clazz.cast(acceleratorAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function