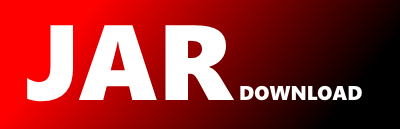
software.amazon.awssdk.services.lookoutvision.model.CreateDatasetRequest Maven / Gradle / Ivy
Show all versions of lookoutvision Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.lookoutvision.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.DefaultValueTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateDatasetRequest extends LookoutVisionRequest implements
ToCopyableBuilder {
private static final SdkField PROJECT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ProjectName").getter(getter(CreateDatasetRequest::projectName)).setter(setter(Builder::projectName))
.traits(LocationTrait.builder().location(MarshallLocation.PATH).locationName("projectName").build()).build();
private static final SdkField DATASET_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DatasetType").getter(getter(CreateDatasetRequest::datasetType)).setter(setter(Builder::datasetType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DatasetType").build()).build();
private static final SdkField DATASET_SOURCE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("DatasetSource")
.getter(getter(CreateDatasetRequest::datasetSource)).setter(setter(Builder::datasetSource))
.constructor(DatasetSource::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DatasetSource").build()).build();
private static final SdkField CLIENT_TOKEN_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ClientToken")
.getter(getter(CreateDatasetRequest::clientToken))
.setter(setter(Builder::clientToken))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("X-Amzn-Client-Token").build(),
DefaultValueTrait.idempotencyToken()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(PROJECT_NAME_FIELD,
DATASET_TYPE_FIELD, DATASET_SOURCE_FIELD, CLIENT_TOKEN_FIELD));
private final String projectName;
private final String datasetType;
private final DatasetSource datasetSource;
private final String clientToken;
private CreateDatasetRequest(BuilderImpl builder) {
super(builder);
this.projectName = builder.projectName;
this.datasetType = builder.datasetType;
this.datasetSource = builder.datasetSource;
this.clientToken = builder.clientToken;
}
/**
*
* The name of the project in which you want to create a dataset.
*
*
* @return The name of the project in which you want to create a dataset.
*/
public final String projectName() {
return projectName;
}
/**
*
* The type of the dataset. Specify train
for a training dataset. Specify test
for a test
* dataset.
*
*
* @return The type of the dataset. Specify train
for a training dataset. Specify test
for
* a test dataset.
*/
public final String datasetType() {
return datasetType;
}
/**
*
* The location of the manifest file that Amazon Lookout for Vision uses to create the dataset.
*
*
* If you don't specify DatasetSource
, an empty dataset is created and the operation synchronously
* returns. Later, you can add JSON Lines by calling UpdateDatasetEntries.
*
*
* If you specify a value for DataSource
, the manifest at the S3 location is validated and used to
* create the dataset. The call to CreateDataset
is asynchronous and might take a while to complete. To
* find out the current status, Check the value of Status
returned in a call to DescribeDataset.
*
*
* @return The location of the manifest file that Amazon Lookout for Vision uses to create the dataset.
*
* If you don't specify DatasetSource
, an empty dataset is created and the operation
* synchronously returns. Later, you can add JSON Lines by calling UpdateDatasetEntries.
*
*
* If you specify a value for DataSource
, the manifest at the S3 location is validated and used
* to create the dataset. The call to CreateDataset
is asynchronous and might take a while to
* complete. To find out the current status, Check the value of Status
returned in a call to
* DescribeDataset.
*/
public final DatasetSource datasetSource() {
return datasetSource;
}
/**
*
* ClientToken is an idempotency token that ensures a call to CreateDataset
completes only once. You
* choose the value to pass. For example, An issue might prevent you from getting a response from
* CreateDataset
. In this case, safely retry your call to CreateDataset
by using the same
* ClientToken
parameter value.
*
*
* If you don't supply a value for ClientToken
, the AWS SDK you are using inserts a value for you. This
* prevents retries after a network error from making multiple dataset creation requests. You'll need to provide
* your own value for other use cases.
*
*
* An error occurs if the other input parameters are not the same as in the first request. Using a different value
* for ClientToken
is considered a new call to CreateDataset
. An idempotency token is
* active for 8 hours.
*
*
* @return ClientToken is an idempotency token that ensures a call to CreateDataset
completes only
* once. You choose the value to pass. For example, An issue might prevent you from getting a response from
* CreateDataset
. In this case, safely retry your call to CreateDataset
by using
* the same ClientToken
parameter value.
*
* If you don't supply a value for ClientToken
, the AWS SDK you are using inserts a value for
* you. This prevents retries after a network error from making multiple dataset creation requests. You'll
* need to provide your own value for other use cases.
*
*
* An error occurs if the other input parameters are not the same as in the first request. Using a different
* value for ClientToken
is considered a new call to CreateDataset
. An idempotency
* token is active for 8 hours.
*/
public final String clientToken() {
return clientToken;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(projectName());
hashCode = 31 * hashCode + Objects.hashCode(datasetType());
hashCode = 31 * hashCode + Objects.hashCode(datasetSource());
hashCode = 31 * hashCode + Objects.hashCode(clientToken());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateDatasetRequest)) {
return false;
}
CreateDatasetRequest other = (CreateDatasetRequest) obj;
return Objects.equals(projectName(), other.projectName()) && Objects.equals(datasetType(), other.datasetType())
&& Objects.equals(datasetSource(), other.datasetSource()) && Objects.equals(clientToken(), other.clientToken());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateDatasetRequest").add("ProjectName", projectName()).add("DatasetType", datasetType())
.add("DatasetSource", datasetSource()).add("ClientToken", clientToken()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ProjectName":
return Optional.ofNullable(clazz.cast(projectName()));
case "DatasetType":
return Optional.ofNullable(clazz.cast(datasetType()));
case "DatasetSource":
return Optional.ofNullable(clazz.cast(datasetSource()));
case "ClientToken":
return Optional.ofNullable(clazz.cast(clientToken()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* If you don't specify DatasetSource
, an empty dataset is created and the operation
* synchronously returns. Later, you can add JSON Lines by calling UpdateDatasetEntries.
*
*
* If you specify a value for DataSource
, the manifest at the S3 location is validated and
* used to create the dataset. The call to CreateDataset
is asynchronous and might take a
* while to complete. To find out the current status, Check the value of Status
returned in
* a call to DescribeDataset.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder datasetSource(DatasetSource datasetSource);
/**
*
* The location of the manifest file that Amazon Lookout for Vision uses to create the dataset.
*
*
* If you don't specify DatasetSource
, an empty dataset is created and the operation synchronously
* returns. Later, you can add JSON Lines by calling UpdateDatasetEntries.
*
*
* If you specify a value for DataSource
, the manifest at the S3 location is validated and used to
* create the dataset. The call to CreateDataset
is asynchronous and might take a while to
* complete. To find out the current status, Check the value of Status
returned in a call to
* DescribeDataset.
*
* This is a convenience method that creates an instance of the {@link DatasetSource.Builder} avoiding the need
* to create one manually via {@link DatasetSource#builder()}.
*
*
* When the {@link Consumer} completes, {@link DatasetSource.Builder#build()} is called immediately and its
* result is passed to {@link #datasetSource(DatasetSource)}.
*
* @param datasetSource
* a consumer that will call methods on {@link DatasetSource.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #datasetSource(DatasetSource)
*/
default Builder datasetSource(Consumer datasetSource) {
return datasetSource(DatasetSource.builder().applyMutation(datasetSource).build());
}
/**
*
* ClientToken is an idempotency token that ensures a call to CreateDataset
completes only once.
* You choose the value to pass. For example, An issue might prevent you from getting a response from
* CreateDataset
. In this case, safely retry your call to CreateDataset
by using the
* same ClientToken
parameter value.
*
*
* If you don't supply a value for ClientToken
, the AWS SDK you are using inserts a value for you.
* This prevents retries after a network error from making multiple dataset creation requests. You'll need to
* provide your own value for other use cases.
*
*
* An error occurs if the other input parameters are not the same as in the first request. Using a different
* value for ClientToken
is considered a new call to CreateDataset
. An idempotency
* token is active for 8 hours.
*
*
* @param clientToken
* ClientToken is an idempotency token that ensures a call to CreateDataset
completes only
* once. You choose the value to pass. For example, An issue might prevent you from getting a response
* from CreateDataset
. In this case, safely retry your call to CreateDataset
by
* using the same ClientToken
parameter value.
*
* If you don't supply a value for ClientToken
, the AWS SDK you are using inserts a value
* for you. This prevents retries after a network error from making multiple dataset creation requests.
* You'll need to provide your own value for other use cases.
*
*
* An error occurs if the other input parameters are not the same as in the first request. Using a
* different value for ClientToken
is considered a new call to CreateDataset
.
* An idempotency token is active for 8 hours.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder clientToken(String clientToken);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends LookoutVisionRequest.BuilderImpl implements Builder {
private String projectName;
private String datasetType;
private DatasetSource datasetSource;
private String clientToken;
private BuilderImpl() {
}
private BuilderImpl(CreateDatasetRequest model) {
super(model);
projectName(model.projectName);
datasetType(model.datasetType);
datasetSource(model.datasetSource);
clientToken(model.clientToken);
}
public final String getProjectName() {
return projectName;
}
public final void setProjectName(String projectName) {
this.projectName = projectName;
}
@Override
public final Builder projectName(String projectName) {
this.projectName = projectName;
return this;
}
public final String getDatasetType() {
return datasetType;
}
public final void setDatasetType(String datasetType) {
this.datasetType = datasetType;
}
@Override
public final Builder datasetType(String datasetType) {
this.datasetType = datasetType;
return this;
}
public final DatasetSource.Builder getDatasetSource() {
return datasetSource != null ? datasetSource.toBuilder() : null;
}
public final void setDatasetSource(DatasetSource.BuilderImpl datasetSource) {
this.datasetSource = datasetSource != null ? datasetSource.build() : null;
}
@Override
public final Builder datasetSource(DatasetSource datasetSource) {
this.datasetSource = datasetSource;
return this;
}
public final String getClientToken() {
return clientToken;
}
public final void setClientToken(String clientToken) {
this.clientToken = clientToken;
}
@Override
public final Builder clientToken(String clientToken) {
this.clientToken = clientToken;
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public CreateDatasetRequest build() {
return new CreateDatasetRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}