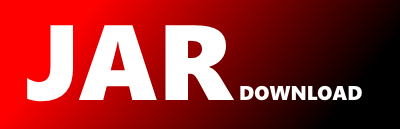
software.amazon.awssdk.services.m2.M2AsyncClient Maven / Gradle / Ivy
Show all versions of m2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.m2;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.services.m2.model.CancelBatchJobExecutionRequest;
import software.amazon.awssdk.services.m2.model.CancelBatchJobExecutionResponse;
import software.amazon.awssdk.services.m2.model.CreateApplicationRequest;
import software.amazon.awssdk.services.m2.model.CreateApplicationResponse;
import software.amazon.awssdk.services.m2.model.CreateDataSetImportTaskRequest;
import software.amazon.awssdk.services.m2.model.CreateDataSetImportTaskResponse;
import software.amazon.awssdk.services.m2.model.CreateDeploymentRequest;
import software.amazon.awssdk.services.m2.model.CreateDeploymentResponse;
import software.amazon.awssdk.services.m2.model.CreateEnvironmentRequest;
import software.amazon.awssdk.services.m2.model.CreateEnvironmentResponse;
import software.amazon.awssdk.services.m2.model.DeleteApplicationFromEnvironmentRequest;
import software.amazon.awssdk.services.m2.model.DeleteApplicationFromEnvironmentResponse;
import software.amazon.awssdk.services.m2.model.DeleteApplicationRequest;
import software.amazon.awssdk.services.m2.model.DeleteApplicationResponse;
import software.amazon.awssdk.services.m2.model.DeleteEnvironmentRequest;
import software.amazon.awssdk.services.m2.model.DeleteEnvironmentResponse;
import software.amazon.awssdk.services.m2.model.GetApplicationRequest;
import software.amazon.awssdk.services.m2.model.GetApplicationResponse;
import software.amazon.awssdk.services.m2.model.GetApplicationVersionRequest;
import software.amazon.awssdk.services.m2.model.GetApplicationVersionResponse;
import software.amazon.awssdk.services.m2.model.GetBatchJobExecutionRequest;
import software.amazon.awssdk.services.m2.model.GetBatchJobExecutionResponse;
import software.amazon.awssdk.services.m2.model.GetDataSetDetailsRequest;
import software.amazon.awssdk.services.m2.model.GetDataSetDetailsResponse;
import software.amazon.awssdk.services.m2.model.GetDataSetImportTaskRequest;
import software.amazon.awssdk.services.m2.model.GetDataSetImportTaskResponse;
import software.amazon.awssdk.services.m2.model.GetDeploymentRequest;
import software.amazon.awssdk.services.m2.model.GetDeploymentResponse;
import software.amazon.awssdk.services.m2.model.GetEnvironmentRequest;
import software.amazon.awssdk.services.m2.model.GetEnvironmentResponse;
import software.amazon.awssdk.services.m2.model.ListApplicationVersionsRequest;
import software.amazon.awssdk.services.m2.model.ListApplicationVersionsResponse;
import software.amazon.awssdk.services.m2.model.ListApplicationsRequest;
import software.amazon.awssdk.services.m2.model.ListApplicationsResponse;
import software.amazon.awssdk.services.m2.model.ListBatchJobDefinitionsRequest;
import software.amazon.awssdk.services.m2.model.ListBatchJobDefinitionsResponse;
import software.amazon.awssdk.services.m2.model.ListBatchJobExecutionsRequest;
import software.amazon.awssdk.services.m2.model.ListBatchJobExecutionsResponse;
import software.amazon.awssdk.services.m2.model.ListDataSetImportHistoryRequest;
import software.amazon.awssdk.services.m2.model.ListDataSetImportHistoryResponse;
import software.amazon.awssdk.services.m2.model.ListDataSetsRequest;
import software.amazon.awssdk.services.m2.model.ListDataSetsResponse;
import software.amazon.awssdk.services.m2.model.ListDeploymentsRequest;
import software.amazon.awssdk.services.m2.model.ListDeploymentsResponse;
import software.amazon.awssdk.services.m2.model.ListEngineVersionsRequest;
import software.amazon.awssdk.services.m2.model.ListEngineVersionsResponse;
import software.amazon.awssdk.services.m2.model.ListEnvironmentsRequest;
import software.amazon.awssdk.services.m2.model.ListEnvironmentsResponse;
import software.amazon.awssdk.services.m2.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.m2.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.m2.model.StartApplicationRequest;
import software.amazon.awssdk.services.m2.model.StartApplicationResponse;
import software.amazon.awssdk.services.m2.model.StartBatchJobRequest;
import software.amazon.awssdk.services.m2.model.StartBatchJobResponse;
import software.amazon.awssdk.services.m2.model.StopApplicationRequest;
import software.amazon.awssdk.services.m2.model.StopApplicationResponse;
import software.amazon.awssdk.services.m2.model.TagResourceRequest;
import software.amazon.awssdk.services.m2.model.TagResourceResponse;
import software.amazon.awssdk.services.m2.model.UntagResourceRequest;
import software.amazon.awssdk.services.m2.model.UntagResourceResponse;
import software.amazon.awssdk.services.m2.model.UpdateApplicationRequest;
import software.amazon.awssdk.services.m2.model.UpdateApplicationResponse;
import software.amazon.awssdk.services.m2.model.UpdateEnvironmentRequest;
import software.amazon.awssdk.services.m2.model.UpdateEnvironmentResponse;
import software.amazon.awssdk.services.m2.paginators.ListApplicationVersionsPublisher;
import software.amazon.awssdk.services.m2.paginators.ListApplicationsPublisher;
import software.amazon.awssdk.services.m2.paginators.ListBatchJobDefinitionsPublisher;
import software.amazon.awssdk.services.m2.paginators.ListBatchJobExecutionsPublisher;
import software.amazon.awssdk.services.m2.paginators.ListDataSetImportHistoryPublisher;
import software.amazon.awssdk.services.m2.paginators.ListDataSetsPublisher;
import software.amazon.awssdk.services.m2.paginators.ListDeploymentsPublisher;
import software.amazon.awssdk.services.m2.paginators.ListEngineVersionsPublisher;
import software.amazon.awssdk.services.m2.paginators.ListEnvironmentsPublisher;
/**
* Service client for accessing AWSMainframeModernization asynchronously. This can be created using the static
* {@link #builder()} method.
*
*
* Amazon Web Services Mainframe Modernization provides tools and resources to help you plan and implement migration and
* modernization from mainframes to Amazon Web Services managed runtime environments. It provides tools for analyzing
* existing mainframe applications, developing or updating mainframe applications using COBOL or PL/I, and implementing
* an automated pipeline for continuous integration and continuous delivery (CI/CD) of the applications.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface M2AsyncClient extends SdkClient {
String SERVICE_NAME = "m2";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "m2";
/**
* Create a {@link M2AsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static M2AsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link M2AsyncClient}.
*/
static M2AsyncClientBuilder builder() {
return new DefaultM2AsyncClientBuilder();
}
/**
*
* Cancels the running of a specific batch job execution.
*
*
* @param cancelBatchJobExecutionRequest
* @return A Java Future containing the result of the CancelBatchJobExecution operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.CancelBatchJobExecution
* @see AWS
* API Documentation
*/
default CompletableFuture cancelBatchJobExecution(
CancelBatchJobExecutionRequest cancelBatchJobExecutionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Cancels the running of a specific batch job execution.
*
*
*
* This is a convenience which creates an instance of the {@link CancelBatchJobExecutionRequest.Builder} avoiding
* the need to create one manually via {@link CancelBatchJobExecutionRequest#builder()}
*
*
* @param cancelBatchJobExecutionRequest
* A {@link Consumer} that will call methods on {@link CancelBatchJobExecutionRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CancelBatchJobExecution operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.CancelBatchJobExecution
* @see AWS
* API Documentation
*/
default CompletableFuture cancelBatchJobExecution(
Consumer cancelBatchJobExecutionRequest) {
return cancelBatchJobExecution(CancelBatchJobExecutionRequest.builder().applyMutation(cancelBatchJobExecutionRequest)
.build());
}
/**
*
* Creates a new application with given parameters. Requires an existing environment and application definition
* file.
*
*
* @param createApplicationRequest
* @return A Java Future containing the result of the CreateApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - ServiceQuotaExceededException One or more quotas for Amazon Web Services Mainframe Modernization
* exceeds the limit.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.CreateApplication
* @see AWS API
* Documentation
*/
default CompletableFuture createApplication(CreateApplicationRequest createApplicationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new application with given parameters. Requires an existing environment and application definition
* file.
*
*
*
* This is a convenience which creates an instance of the {@link CreateApplicationRequest.Builder} avoiding the need
* to create one manually via {@link CreateApplicationRequest#builder()}
*
*
* @param createApplicationRequest
* A {@link Consumer} that will call methods on {@link CreateApplicationRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - ServiceQuotaExceededException One or more quotas for Amazon Web Services Mainframe Modernization
* exceeds the limit.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.CreateApplication
* @see AWS API
* Documentation
*/
default CompletableFuture createApplication(
Consumer createApplicationRequest) {
return createApplication(CreateApplicationRequest.builder().applyMutation(createApplicationRequest).build());
}
/**
*
* Starts a data set import task for a specific application.
*
*
* @param createDataSetImportTaskRequest
* @return A Java Future containing the result of the CreateDataSetImportTask operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - ServiceQuotaExceededException One or more quotas for Amazon Web Services Mainframe Modernization
* exceeds the limit.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.CreateDataSetImportTask
* @see AWS
* API Documentation
*/
default CompletableFuture createDataSetImportTask(
CreateDataSetImportTaskRequest createDataSetImportTaskRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Starts a data set import task for a specific application.
*
*
*
* This is a convenience which creates an instance of the {@link CreateDataSetImportTaskRequest.Builder} avoiding
* the need to create one manually via {@link CreateDataSetImportTaskRequest#builder()}
*
*
* @param createDataSetImportTaskRequest
* A {@link Consumer} that will call methods on {@link CreateDataSetImportTaskRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateDataSetImportTask operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - ServiceQuotaExceededException One or more quotas for Amazon Web Services Mainframe Modernization
* exceeds the limit.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.CreateDataSetImportTask
* @see AWS
* API Documentation
*/
default CompletableFuture createDataSetImportTask(
Consumer createDataSetImportTaskRequest) {
return createDataSetImportTask(CreateDataSetImportTaskRequest.builder().applyMutation(createDataSetImportTaskRequest)
.build());
}
/**
*
* Creates and starts a deployment to deploy an application into an environment.
*
*
* @param createDeploymentRequest
* @return A Java Future containing the result of the CreateDeployment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - ServiceQuotaExceededException One or more quotas for Amazon Web Services Mainframe Modernization
* exceeds the limit.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.CreateDeployment
* @see AWS API
* Documentation
*/
default CompletableFuture createDeployment(CreateDeploymentRequest createDeploymentRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates and starts a deployment to deploy an application into an environment.
*
*
*
* This is a convenience which creates an instance of the {@link CreateDeploymentRequest.Builder} avoiding the need
* to create one manually via {@link CreateDeploymentRequest#builder()}
*
*
* @param createDeploymentRequest
* A {@link Consumer} that will call methods on {@link CreateDeploymentRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateDeployment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - ServiceQuotaExceededException One or more quotas for Amazon Web Services Mainframe Modernization
* exceeds the limit.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.CreateDeployment
* @see AWS API
* Documentation
*/
default CompletableFuture createDeployment(
Consumer createDeploymentRequest) {
return createDeployment(CreateDeploymentRequest.builder().applyMutation(createDeploymentRequest).build());
}
/**
*
* Creates a runtime environment for a given runtime engine.
*
*
* @param createEnvironmentRequest
* @return A Java Future containing the result of the CreateEnvironment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - ServiceQuotaExceededException One or more quotas for Amazon Web Services Mainframe Modernization
* exceeds the limit.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.CreateEnvironment
* @see AWS API
* Documentation
*/
default CompletableFuture createEnvironment(CreateEnvironmentRequest createEnvironmentRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a runtime environment for a given runtime engine.
*
*
*
* This is a convenience which creates an instance of the {@link CreateEnvironmentRequest.Builder} avoiding the need
* to create one manually via {@link CreateEnvironmentRequest#builder()}
*
*
* @param createEnvironmentRequest
* A {@link Consumer} that will call methods on {@link CreateEnvironmentRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateEnvironment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - ServiceQuotaExceededException One or more quotas for Amazon Web Services Mainframe Modernization
* exceeds the limit.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.CreateEnvironment
* @see AWS API
* Documentation
*/
default CompletableFuture createEnvironment(
Consumer createEnvironmentRequest) {
return createEnvironment(CreateEnvironmentRequest.builder().applyMutation(createEnvironmentRequest).build());
}
/**
*
* Deletes a specific application. You cannot delete a running application.
*
*
* @param deleteApplicationRequest
* @return A Java Future containing the result of the DeleteApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.DeleteApplication
* @see AWS API
* Documentation
*/
default CompletableFuture deleteApplication(DeleteApplicationRequest deleteApplicationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a specific application. You cannot delete a running application.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteApplicationRequest.Builder} avoiding the need
* to create one manually via {@link DeleteApplicationRequest#builder()}
*
*
* @param deleteApplicationRequest
* A {@link Consumer} that will call methods on {@link DeleteApplicationRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.DeleteApplication
* @see AWS API
* Documentation
*/
default CompletableFuture deleteApplication(
Consumer deleteApplicationRequest) {
return deleteApplication(DeleteApplicationRequest.builder().applyMutation(deleteApplicationRequest).build());
}
/**
*
* Deletes a specific application from a specified environment where it has been previously deployed. You cannot
* delete an environment using DeleteEnvironment, if any application has ever been deployed to it. This API removes
* the association of the application with the environment so you can delete the environment smoothly.
*
*
* @param deleteApplicationFromEnvironmentRequest
* @return A Java Future containing the result of the DeleteApplicationFromEnvironment operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.DeleteApplicationFromEnvironment
* @see AWS API Documentation
*/
default CompletableFuture deleteApplicationFromEnvironment(
DeleteApplicationFromEnvironmentRequest deleteApplicationFromEnvironmentRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a specific application from a specified environment where it has been previously deployed. You cannot
* delete an environment using DeleteEnvironment, if any application has ever been deployed to it. This API removes
* the association of the application with the environment so you can delete the environment smoothly.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteApplicationFromEnvironmentRequest.Builder}
* avoiding the need to create one manually via {@link DeleteApplicationFromEnvironmentRequest#builder()}
*
*
* @param deleteApplicationFromEnvironmentRequest
* A {@link Consumer} that will call methods on {@link DeleteApplicationFromEnvironmentRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DeleteApplicationFromEnvironment operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.DeleteApplicationFromEnvironment
* @see AWS API Documentation
*/
default CompletableFuture deleteApplicationFromEnvironment(
Consumer deleteApplicationFromEnvironmentRequest) {
return deleteApplicationFromEnvironment(DeleteApplicationFromEnvironmentRequest.builder()
.applyMutation(deleteApplicationFromEnvironmentRequest).build());
}
/**
*
* Deletes a specific environment. The environment cannot contain deployed applications. If it does, you must delete
* those applications before you delete the environment.
*
*
* @param deleteEnvironmentRequest
* @return A Java Future containing the result of the DeleteEnvironment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.DeleteEnvironment
* @see AWS API
* Documentation
*/
default CompletableFuture deleteEnvironment(DeleteEnvironmentRequest deleteEnvironmentRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a specific environment. The environment cannot contain deployed applications. If it does, you must delete
* those applications before you delete the environment.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteEnvironmentRequest.Builder} avoiding the need
* to create one manually via {@link DeleteEnvironmentRequest#builder()}
*
*
* @param deleteEnvironmentRequest
* A {@link Consumer} that will call methods on {@link DeleteEnvironmentRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteEnvironment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.DeleteEnvironment
* @see AWS API
* Documentation
*/
default CompletableFuture deleteEnvironment(
Consumer deleteEnvironmentRequest) {
return deleteEnvironment(DeleteEnvironmentRequest.builder().applyMutation(deleteEnvironmentRequest).build());
}
/**
*
* Describes the details of a specific application.
*
*
* @param getApplicationRequest
* @return A Java Future containing the result of the GetApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.GetApplication
* @see AWS API
* Documentation
*/
default CompletableFuture getApplication(GetApplicationRequest getApplicationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the details of a specific application.
*
*
*
* This is a convenience which creates an instance of the {@link GetApplicationRequest.Builder} avoiding the need to
* create one manually via {@link GetApplicationRequest#builder()}
*
*
* @param getApplicationRequest
* A {@link Consumer} that will call methods on {@link GetApplicationRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.GetApplication
* @see AWS API
* Documentation
*/
default CompletableFuture getApplication(Consumer getApplicationRequest) {
return getApplication(GetApplicationRequest.builder().applyMutation(getApplicationRequest).build());
}
/**
*
* Returns details about a specific version of a specific application.
*
*
* @param getApplicationVersionRequest
* @return A Java Future containing the result of the GetApplicationVersion operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.GetApplicationVersion
* @see AWS API
* Documentation
*/
default CompletableFuture getApplicationVersion(
GetApplicationVersionRequest getApplicationVersionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns details about a specific version of a specific application.
*
*
*
* This is a convenience which creates an instance of the {@link GetApplicationVersionRequest.Builder} avoiding the
* need to create one manually via {@link GetApplicationVersionRequest#builder()}
*
*
* @param getApplicationVersionRequest
* A {@link Consumer} that will call methods on {@link GetApplicationVersionRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetApplicationVersion operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.GetApplicationVersion
* @see AWS API
* Documentation
*/
default CompletableFuture getApplicationVersion(
Consumer getApplicationVersionRequest) {
return getApplicationVersion(GetApplicationVersionRequest.builder().applyMutation(getApplicationVersionRequest).build());
}
/**
*
* Gets the details of a specific batch job execution for a specific application.
*
*
* @param getBatchJobExecutionRequest
* @return A Java Future containing the result of the GetBatchJobExecution operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.GetBatchJobExecution
* @see AWS API
* Documentation
*/
default CompletableFuture getBatchJobExecution(
GetBatchJobExecutionRequest getBatchJobExecutionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the details of a specific batch job execution for a specific application.
*
*
*
* This is a convenience which creates an instance of the {@link GetBatchJobExecutionRequest.Builder} avoiding the
* need to create one manually via {@link GetBatchJobExecutionRequest#builder()}
*
*
* @param getBatchJobExecutionRequest
* A {@link Consumer} that will call methods on {@link GetBatchJobExecutionRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetBatchJobExecution operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.GetBatchJobExecution
* @see AWS API
* Documentation
*/
default CompletableFuture getBatchJobExecution(
Consumer getBatchJobExecutionRequest) {
return getBatchJobExecution(GetBatchJobExecutionRequest.builder().applyMutation(getBatchJobExecutionRequest).build());
}
/**
*
* Gets the details of a specific data set.
*
*
* @param getDataSetDetailsRequest
* @return A Java Future containing the result of the GetDataSetDetails operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.GetDataSetDetails
* @see AWS API
* Documentation
*/
default CompletableFuture getDataSetDetails(GetDataSetDetailsRequest getDataSetDetailsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the details of a specific data set.
*
*
*
* This is a convenience which creates an instance of the {@link GetDataSetDetailsRequest.Builder} avoiding the need
* to create one manually via {@link GetDataSetDetailsRequest#builder()}
*
*
* @param getDataSetDetailsRequest
* A {@link Consumer} that will call methods on {@link GetDataSetDetailsRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetDataSetDetails operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.GetDataSetDetails
* @see AWS API
* Documentation
*/
default CompletableFuture getDataSetDetails(
Consumer getDataSetDetailsRequest) {
return getDataSetDetails(GetDataSetDetailsRequest.builder().applyMutation(getDataSetDetailsRequest).build());
}
/**
*
* Gets the status of a data set import task initiated with the CreateDataSetImportTask operation.
*
*
* @param getDataSetImportTaskRequest
* @return A Java Future containing the result of the GetDataSetImportTask operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.GetDataSetImportTask
* @see AWS API
* Documentation
*/
default CompletableFuture getDataSetImportTask(
GetDataSetImportTaskRequest getDataSetImportTaskRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the status of a data set import task initiated with the CreateDataSetImportTask operation.
*
*
*
* This is a convenience which creates an instance of the {@link GetDataSetImportTaskRequest.Builder} avoiding the
* need to create one manually via {@link GetDataSetImportTaskRequest#builder()}
*
*
* @param getDataSetImportTaskRequest
* A {@link Consumer} that will call methods on {@link GetDataSetImportTaskRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetDataSetImportTask operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.GetDataSetImportTask
* @see AWS API
* Documentation
*/
default CompletableFuture getDataSetImportTask(
Consumer getDataSetImportTaskRequest) {
return getDataSetImportTask(GetDataSetImportTaskRequest.builder().applyMutation(getDataSetImportTaskRequest).build());
}
/**
*
* Gets details of a specific deployment with a given deployment identifier.
*
*
* @param getDeploymentRequest
* @return A Java Future containing the result of the GetDeployment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.GetDeployment
* @see AWS API
* Documentation
*/
default CompletableFuture getDeployment(GetDeploymentRequest getDeploymentRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets details of a specific deployment with a given deployment identifier.
*
*
*
* This is a convenience which creates an instance of the {@link GetDeploymentRequest.Builder} avoiding the need to
* create one manually via {@link GetDeploymentRequest#builder()}
*
*
* @param getDeploymentRequest
* A {@link Consumer} that will call methods on {@link GetDeploymentRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetDeployment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.GetDeployment
* @see AWS API
* Documentation
*/
default CompletableFuture getDeployment(Consumer getDeploymentRequest) {
return getDeployment(GetDeploymentRequest.builder().applyMutation(getDeploymentRequest).build());
}
/**
*
* Describes a specific runtime environment.
*
*
* @param getEnvironmentRequest
* @return A Java Future containing the result of the GetEnvironment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.GetEnvironment
* @see AWS API
* Documentation
*/
default CompletableFuture getEnvironment(GetEnvironmentRequest getEnvironmentRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes a specific runtime environment.
*
*
*
* This is a convenience which creates an instance of the {@link GetEnvironmentRequest.Builder} avoiding the need to
* create one manually via {@link GetEnvironmentRequest#builder()}
*
*
* @param getEnvironmentRequest
* A {@link Consumer} that will call methods on {@link GetEnvironmentRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetEnvironment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.GetEnvironment
* @see AWS API
* Documentation
*/
default CompletableFuture getEnvironment(Consumer getEnvironmentRequest) {
return getEnvironment(GetEnvironmentRequest.builder().applyMutation(getEnvironmentRequest).build());
}
/**
*
* Returns a list of the application versions for a specific application.
*
*
* @param listApplicationVersionsRequest
* @return A Java Future containing the result of the ListApplicationVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListApplicationVersions
* @see AWS
* API Documentation
*/
default CompletableFuture listApplicationVersions(
ListApplicationVersionsRequest listApplicationVersionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of the application versions for a specific application.
*
*
*
* This is a convenience which creates an instance of the {@link ListApplicationVersionsRequest.Builder} avoiding
* the need to create one manually via {@link ListApplicationVersionsRequest#builder()}
*
*
* @param listApplicationVersionsRequest
* A {@link Consumer} that will call methods on {@link ListApplicationVersionsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListApplicationVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListApplicationVersions
* @see AWS
* API Documentation
*/
default CompletableFuture listApplicationVersions(
Consumer listApplicationVersionsRequest) {
return listApplicationVersions(ListApplicationVersionsRequest.builder().applyMutation(listApplicationVersionsRequest)
.build());
}
/**
*
* Returns a list of the application versions for a specific application.
*
*
*
* This is a variant of
* {@link #listApplicationVersions(software.amazon.awssdk.services.m2.model.ListApplicationVersionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.m2.paginators.ListApplicationVersionsPublisher publisher = client.listApplicationVersionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.m2.paginators.ListApplicationVersionsPublisher publisher = client.listApplicationVersionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.m2.model.ListApplicationVersionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listApplicationVersions(software.amazon.awssdk.services.m2.model.ListApplicationVersionsRequest)}
* operation.
*
*
* @param listApplicationVersionsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListApplicationVersions
* @see AWS
* API Documentation
*/
default ListApplicationVersionsPublisher listApplicationVersionsPaginator(
ListApplicationVersionsRequest listApplicationVersionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of the application versions for a specific application.
*
*
*
* This is a variant of
* {@link #listApplicationVersions(software.amazon.awssdk.services.m2.model.ListApplicationVersionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.m2.paginators.ListApplicationVersionsPublisher publisher = client.listApplicationVersionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.m2.paginators.ListApplicationVersionsPublisher publisher = client.listApplicationVersionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.m2.model.ListApplicationVersionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listApplicationVersions(software.amazon.awssdk.services.m2.model.ListApplicationVersionsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListApplicationVersionsRequest.Builder} avoiding
* the need to create one manually via {@link ListApplicationVersionsRequest#builder()}
*
*
* @param listApplicationVersionsRequest
* A {@link Consumer} that will call methods on {@link ListApplicationVersionsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListApplicationVersions
* @see AWS
* API Documentation
*/
default ListApplicationVersionsPublisher listApplicationVersionsPaginator(
Consumer listApplicationVersionsRequest) {
return listApplicationVersionsPaginator(ListApplicationVersionsRequest.builder()
.applyMutation(listApplicationVersionsRequest).build());
}
/**
*
* Lists the applications associated with a specific Amazon Web Services account. You can provide the unique
* identifier of a specific environment in a query parameter to see all applications associated with that
* environment.
*
*
* @param listApplicationsRequest
* @return A Java Future containing the result of the ListApplications operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListApplications
* @see AWS API
* Documentation
*/
default CompletableFuture listApplications(ListApplicationsRequest listApplicationsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the applications associated with a specific Amazon Web Services account. You can provide the unique
* identifier of a specific environment in a query parameter to see all applications associated with that
* environment.
*
*
*
* This is a convenience which creates an instance of the {@link ListApplicationsRequest.Builder} avoiding the need
* to create one manually via {@link ListApplicationsRequest#builder()}
*
*
* @param listApplicationsRequest
* A {@link Consumer} that will call methods on {@link ListApplicationsRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListApplications operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListApplications
* @see AWS API
* Documentation
*/
default CompletableFuture listApplications(
Consumer listApplicationsRequest) {
return listApplications(ListApplicationsRequest.builder().applyMutation(listApplicationsRequest).build());
}
/**
*
* Lists the applications associated with a specific Amazon Web Services account. You can provide the unique
* identifier of a specific environment in a query parameter to see all applications associated with that
* environment.
*
*
*
* This is a variant of {@link #listApplications(software.amazon.awssdk.services.m2.model.ListApplicationsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.m2.paginators.ListApplicationsPublisher publisher = client.listApplicationsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.m2.paginators.ListApplicationsPublisher publisher = client.listApplicationsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.m2.model.ListApplicationsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listApplications(software.amazon.awssdk.services.m2.model.ListApplicationsRequest)} operation.
*
*
* @param listApplicationsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListApplications
* @see AWS API
* Documentation
*/
default ListApplicationsPublisher listApplicationsPaginator(ListApplicationsRequest listApplicationsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the applications associated with a specific Amazon Web Services account. You can provide the unique
* identifier of a specific environment in a query parameter to see all applications associated with that
* environment.
*
*
*
* This is a variant of {@link #listApplications(software.amazon.awssdk.services.m2.model.ListApplicationsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.m2.paginators.ListApplicationsPublisher publisher = client.listApplicationsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.m2.paginators.ListApplicationsPublisher publisher = client.listApplicationsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.m2.model.ListApplicationsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listApplications(software.amazon.awssdk.services.m2.model.ListApplicationsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListApplicationsRequest.Builder} avoiding the need
* to create one manually via {@link ListApplicationsRequest#builder()}
*
*
* @param listApplicationsRequest
* A {@link Consumer} that will call methods on {@link ListApplicationsRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListApplications
* @see AWS API
* Documentation
*/
default ListApplicationsPublisher listApplicationsPaginator(Consumer listApplicationsRequest) {
return listApplicationsPaginator(ListApplicationsRequest.builder().applyMutation(listApplicationsRequest).build());
}
/**
*
* Lists all the available batch job definitions based on the batch job resources uploaded during the application
* creation. The listed batch job definitions can then be used to start a batch job.
*
*
* @param listBatchJobDefinitionsRequest
* @return A Java Future containing the result of the ListBatchJobDefinitions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListBatchJobDefinitions
* @see AWS
* API Documentation
*/
default CompletableFuture listBatchJobDefinitions(
ListBatchJobDefinitionsRequest listBatchJobDefinitionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all the available batch job definitions based on the batch job resources uploaded during the application
* creation. The listed batch job definitions can then be used to start a batch job.
*
*
*
* This is a convenience which creates an instance of the {@link ListBatchJobDefinitionsRequest.Builder} avoiding
* the need to create one manually via {@link ListBatchJobDefinitionsRequest#builder()}
*
*
* @param listBatchJobDefinitionsRequest
* A {@link Consumer} that will call methods on {@link ListBatchJobDefinitionsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListBatchJobDefinitions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListBatchJobDefinitions
* @see AWS
* API Documentation
*/
default CompletableFuture listBatchJobDefinitions(
Consumer listBatchJobDefinitionsRequest) {
return listBatchJobDefinitions(ListBatchJobDefinitionsRequest.builder().applyMutation(listBatchJobDefinitionsRequest)
.build());
}
/**
*
* Lists all the available batch job definitions based on the batch job resources uploaded during the application
* creation. The listed batch job definitions can then be used to start a batch job.
*
*
*
* This is a variant of
* {@link #listBatchJobDefinitions(software.amazon.awssdk.services.m2.model.ListBatchJobDefinitionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.m2.paginators.ListBatchJobDefinitionsPublisher publisher = client.listBatchJobDefinitionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.m2.paginators.ListBatchJobDefinitionsPublisher publisher = client.listBatchJobDefinitionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.m2.model.ListBatchJobDefinitionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listBatchJobDefinitions(software.amazon.awssdk.services.m2.model.ListBatchJobDefinitionsRequest)}
* operation.
*
*
* @param listBatchJobDefinitionsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListBatchJobDefinitions
* @see AWS
* API Documentation
*/
default ListBatchJobDefinitionsPublisher listBatchJobDefinitionsPaginator(
ListBatchJobDefinitionsRequest listBatchJobDefinitionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all the available batch job definitions based on the batch job resources uploaded during the application
* creation. The listed batch job definitions can then be used to start a batch job.
*
*
*
* This is a variant of
* {@link #listBatchJobDefinitions(software.amazon.awssdk.services.m2.model.ListBatchJobDefinitionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.m2.paginators.ListBatchJobDefinitionsPublisher publisher = client.listBatchJobDefinitionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.m2.paginators.ListBatchJobDefinitionsPublisher publisher = client.listBatchJobDefinitionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.m2.model.ListBatchJobDefinitionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listBatchJobDefinitions(software.amazon.awssdk.services.m2.model.ListBatchJobDefinitionsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListBatchJobDefinitionsRequest.Builder} avoiding
* the need to create one manually via {@link ListBatchJobDefinitionsRequest#builder()}
*
*
* @param listBatchJobDefinitionsRequest
* A {@link Consumer} that will call methods on {@link ListBatchJobDefinitionsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListBatchJobDefinitions
* @see AWS
* API Documentation
*/
default ListBatchJobDefinitionsPublisher listBatchJobDefinitionsPaginator(
Consumer listBatchJobDefinitionsRequest) {
return listBatchJobDefinitionsPaginator(ListBatchJobDefinitionsRequest.builder()
.applyMutation(listBatchJobDefinitionsRequest).build());
}
/**
*
* Lists historical, current, and scheduled batch job executions for a specific application.
*
*
* @param listBatchJobExecutionsRequest
* @return A Java Future containing the result of the ListBatchJobExecutions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListBatchJobExecutions
* @see AWS API
* Documentation
*/
default CompletableFuture listBatchJobExecutions(
ListBatchJobExecutionsRequest listBatchJobExecutionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists historical, current, and scheduled batch job executions for a specific application.
*
*
*
* This is a convenience which creates an instance of the {@link ListBatchJobExecutionsRequest.Builder} avoiding the
* need to create one manually via {@link ListBatchJobExecutionsRequest#builder()}
*
*
* @param listBatchJobExecutionsRequest
* A {@link Consumer} that will call methods on {@link ListBatchJobExecutionsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListBatchJobExecutions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListBatchJobExecutions
* @see AWS API
* Documentation
*/
default CompletableFuture listBatchJobExecutions(
Consumer listBatchJobExecutionsRequest) {
return listBatchJobExecutions(ListBatchJobExecutionsRequest.builder().applyMutation(listBatchJobExecutionsRequest)
.build());
}
/**
*
* Lists historical, current, and scheduled batch job executions for a specific application.
*
*
*
* This is a variant of
* {@link #listBatchJobExecutions(software.amazon.awssdk.services.m2.model.ListBatchJobExecutionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.m2.paginators.ListBatchJobExecutionsPublisher publisher = client.listBatchJobExecutionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.m2.paginators.ListBatchJobExecutionsPublisher publisher = client.listBatchJobExecutionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.m2.model.ListBatchJobExecutionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listBatchJobExecutions(software.amazon.awssdk.services.m2.model.ListBatchJobExecutionsRequest)}
* operation.
*
*
* @param listBatchJobExecutionsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListBatchJobExecutions
* @see AWS API
* Documentation
*/
default ListBatchJobExecutionsPublisher listBatchJobExecutionsPaginator(
ListBatchJobExecutionsRequest listBatchJobExecutionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists historical, current, and scheduled batch job executions for a specific application.
*
*
*
* This is a variant of
* {@link #listBatchJobExecutions(software.amazon.awssdk.services.m2.model.ListBatchJobExecutionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.m2.paginators.ListBatchJobExecutionsPublisher publisher = client.listBatchJobExecutionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.m2.paginators.ListBatchJobExecutionsPublisher publisher = client.listBatchJobExecutionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.m2.model.ListBatchJobExecutionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listBatchJobExecutions(software.amazon.awssdk.services.m2.model.ListBatchJobExecutionsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListBatchJobExecutionsRequest.Builder} avoiding the
* need to create one manually via {@link ListBatchJobExecutionsRequest#builder()}
*
*
* @param listBatchJobExecutionsRequest
* A {@link Consumer} that will call methods on {@link ListBatchJobExecutionsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListBatchJobExecutions
* @see AWS API
* Documentation
*/
default ListBatchJobExecutionsPublisher listBatchJobExecutionsPaginator(
Consumer listBatchJobExecutionsRequest) {
return listBatchJobExecutionsPaginator(ListBatchJobExecutionsRequest.builder()
.applyMutation(listBatchJobExecutionsRequest).build());
}
/**
*
* Lists the data set imports for the specified application.
*
*
* @param listDataSetImportHistoryRequest
* @return A Java Future containing the result of the ListDataSetImportHistory operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListDataSetImportHistory
* @see AWS
* API Documentation
*/
default CompletableFuture listDataSetImportHistory(
ListDataSetImportHistoryRequest listDataSetImportHistoryRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the data set imports for the specified application.
*
*
*
* This is a convenience which creates an instance of the {@link ListDataSetImportHistoryRequest.Builder} avoiding
* the need to create one manually via {@link ListDataSetImportHistoryRequest#builder()}
*
*
* @param listDataSetImportHistoryRequest
* A {@link Consumer} that will call methods on {@link ListDataSetImportHistoryRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListDataSetImportHistory operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListDataSetImportHistory
* @see AWS
* API Documentation
*/
default CompletableFuture listDataSetImportHistory(
Consumer listDataSetImportHistoryRequest) {
return listDataSetImportHistory(ListDataSetImportHistoryRequest.builder().applyMutation(listDataSetImportHistoryRequest)
.build());
}
/**
*
* Lists the data set imports for the specified application.
*
*
*
* This is a variant of
* {@link #listDataSetImportHistory(software.amazon.awssdk.services.m2.model.ListDataSetImportHistoryRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.m2.paginators.ListDataSetImportHistoryPublisher publisher = client.listDataSetImportHistoryPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.m2.paginators.ListDataSetImportHistoryPublisher publisher = client.listDataSetImportHistoryPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.m2.model.ListDataSetImportHistoryResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDataSetImportHistory(software.amazon.awssdk.services.m2.model.ListDataSetImportHistoryRequest)}
* operation.
*
*
* @param listDataSetImportHistoryRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListDataSetImportHistory
* @see AWS
* API Documentation
*/
default ListDataSetImportHistoryPublisher listDataSetImportHistoryPaginator(
ListDataSetImportHistoryRequest listDataSetImportHistoryRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the data set imports for the specified application.
*
*
*
* This is a variant of
* {@link #listDataSetImportHistory(software.amazon.awssdk.services.m2.model.ListDataSetImportHistoryRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.m2.paginators.ListDataSetImportHistoryPublisher publisher = client.listDataSetImportHistoryPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.m2.paginators.ListDataSetImportHistoryPublisher publisher = client.listDataSetImportHistoryPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.m2.model.ListDataSetImportHistoryResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDataSetImportHistory(software.amazon.awssdk.services.m2.model.ListDataSetImportHistoryRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListDataSetImportHistoryRequest.Builder} avoiding
* the need to create one manually via {@link ListDataSetImportHistoryRequest#builder()}
*
*
* @param listDataSetImportHistoryRequest
* A {@link Consumer} that will call methods on {@link ListDataSetImportHistoryRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListDataSetImportHistory
* @see AWS
* API Documentation
*/
default ListDataSetImportHistoryPublisher listDataSetImportHistoryPaginator(
Consumer listDataSetImportHistoryRequest) {
return listDataSetImportHistoryPaginator(ListDataSetImportHistoryRequest.builder()
.applyMutation(listDataSetImportHistoryRequest).build());
}
/**
*
* Lists the data sets imported for a specific application. In Amazon Web Services Mainframe Modernization, data
* sets are associated with applications deployed on environments. This is known as importing data sets. Currently,
* Amazon Web Services Mainframe Modernization can import data sets into catalogs using CreateDataSetImportTask.
*
*
* @param listDataSetsRequest
* @return A Java Future containing the result of the ListDataSets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListDataSets
* @see AWS API
* Documentation
*/
default CompletableFuture listDataSets(ListDataSetsRequest listDataSetsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the data sets imported for a specific application. In Amazon Web Services Mainframe Modernization, data
* sets are associated with applications deployed on environments. This is known as importing data sets. Currently,
* Amazon Web Services Mainframe Modernization can import data sets into catalogs using CreateDataSetImportTask.
*
*
*
* This is a convenience which creates an instance of the {@link ListDataSetsRequest.Builder} avoiding the need to
* create one manually via {@link ListDataSetsRequest#builder()}
*
*
* @param listDataSetsRequest
* A {@link Consumer} that will call methods on {@link ListDataSetsRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListDataSets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListDataSets
* @see AWS API
* Documentation
*/
default CompletableFuture listDataSets(Consumer listDataSetsRequest) {
return listDataSets(ListDataSetsRequest.builder().applyMutation(listDataSetsRequest).build());
}
/**
*
* Lists the data sets imported for a specific application. In Amazon Web Services Mainframe Modernization, data
* sets are associated with applications deployed on environments. This is known as importing data sets. Currently,
* Amazon Web Services Mainframe Modernization can import data sets into catalogs using CreateDataSetImportTask.
*
*
*
* This is a variant of {@link #listDataSets(software.amazon.awssdk.services.m2.model.ListDataSetsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.m2.paginators.ListDataSetsPublisher publisher = client.listDataSetsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.m2.paginators.ListDataSetsPublisher publisher = client.listDataSetsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.m2.model.ListDataSetsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDataSets(software.amazon.awssdk.services.m2.model.ListDataSetsRequest)} operation.
*
*
* @param listDataSetsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListDataSets
* @see AWS API
* Documentation
*/
default ListDataSetsPublisher listDataSetsPaginator(ListDataSetsRequest listDataSetsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the data sets imported for a specific application. In Amazon Web Services Mainframe Modernization, data
* sets are associated with applications deployed on environments. This is known as importing data sets. Currently,
* Amazon Web Services Mainframe Modernization can import data sets into catalogs using CreateDataSetImportTask.
*
*
*
* This is a variant of {@link #listDataSets(software.amazon.awssdk.services.m2.model.ListDataSetsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.m2.paginators.ListDataSetsPublisher publisher = client.listDataSetsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.m2.paginators.ListDataSetsPublisher publisher = client.listDataSetsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.m2.model.ListDataSetsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDataSets(software.amazon.awssdk.services.m2.model.ListDataSetsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListDataSetsRequest.Builder} avoiding the need to
* create one manually via {@link ListDataSetsRequest#builder()}
*
*
* @param listDataSetsRequest
* A {@link Consumer} that will call methods on {@link ListDataSetsRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListDataSets
* @see AWS API
* Documentation
*/
default ListDataSetsPublisher listDataSetsPaginator(Consumer listDataSetsRequest) {
return listDataSetsPaginator(ListDataSetsRequest.builder().applyMutation(listDataSetsRequest).build());
}
/**
*
* Returns a list of all deployments of a specific application. A deployment is a combination of a specific
* application and a specific version of that application. Each deployment is mapped to a particular application
* version.
*
*
* @param listDeploymentsRequest
* @return A Java Future containing the result of the ListDeployments operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListDeployments
* @see AWS API
* Documentation
*/
default CompletableFuture listDeployments(ListDeploymentsRequest listDeploymentsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of all deployments of a specific application. A deployment is a combination of a specific
* application and a specific version of that application. Each deployment is mapped to a particular application
* version.
*
*
*
* This is a convenience which creates an instance of the {@link ListDeploymentsRequest.Builder} avoiding the need
* to create one manually via {@link ListDeploymentsRequest#builder()}
*
*
* @param listDeploymentsRequest
* A {@link Consumer} that will call methods on {@link ListDeploymentsRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListDeployments operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListDeployments
* @see AWS API
* Documentation
*/
default CompletableFuture listDeployments(
Consumer listDeploymentsRequest) {
return listDeployments(ListDeploymentsRequest.builder().applyMutation(listDeploymentsRequest).build());
}
/**
*
* Returns a list of all deployments of a specific application. A deployment is a combination of a specific
* application and a specific version of that application. Each deployment is mapped to a particular application
* version.
*
*
*
* This is a variant of {@link #listDeployments(software.amazon.awssdk.services.m2.model.ListDeploymentsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.m2.paginators.ListDeploymentsPublisher publisher = client.listDeploymentsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.m2.paginators.ListDeploymentsPublisher publisher = client.listDeploymentsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.m2.model.ListDeploymentsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDeployments(software.amazon.awssdk.services.m2.model.ListDeploymentsRequest)} operation.
*
*
* @param listDeploymentsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListDeployments
* @see AWS API
* Documentation
*/
default ListDeploymentsPublisher listDeploymentsPaginator(ListDeploymentsRequest listDeploymentsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of all deployments of a specific application. A deployment is a combination of a specific
* application and a specific version of that application. Each deployment is mapped to a particular application
* version.
*
*
*
* This is a variant of {@link #listDeployments(software.amazon.awssdk.services.m2.model.ListDeploymentsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.m2.paginators.ListDeploymentsPublisher publisher = client.listDeploymentsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.m2.paginators.ListDeploymentsPublisher publisher = client.listDeploymentsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.m2.model.ListDeploymentsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDeployments(software.amazon.awssdk.services.m2.model.ListDeploymentsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListDeploymentsRequest.Builder} avoiding the need
* to create one manually via {@link ListDeploymentsRequest#builder()}
*
*
* @param listDeploymentsRequest
* A {@link Consumer} that will call methods on {@link ListDeploymentsRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListDeployments
* @see AWS API
* Documentation
*/
default ListDeploymentsPublisher listDeploymentsPaginator(Consumer listDeploymentsRequest) {
return listDeploymentsPaginator(ListDeploymentsRequest.builder().applyMutation(listDeploymentsRequest).build());
}
/**
*
* Lists the available engine versions.
*
*
* @param listEngineVersionsRequest
* @return A Java Future containing the result of the ListEngineVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListEngineVersions
* @see AWS API
* Documentation
*/
default CompletableFuture listEngineVersions(ListEngineVersionsRequest listEngineVersionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the available engine versions.
*
*
*
* This is a convenience which creates an instance of the {@link ListEngineVersionsRequest.Builder} avoiding the
* need to create one manually via {@link ListEngineVersionsRequest#builder()}
*
*
* @param listEngineVersionsRequest
* A {@link Consumer} that will call methods on {@link ListEngineVersionsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListEngineVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListEngineVersions
* @see AWS API
* Documentation
*/
default CompletableFuture listEngineVersions(
Consumer listEngineVersionsRequest) {
return listEngineVersions(ListEngineVersionsRequest.builder().applyMutation(listEngineVersionsRequest).build());
}
/**
*
* Lists the available engine versions.
*
*
*
* This is a variant of
* {@link #listEngineVersions(software.amazon.awssdk.services.m2.model.ListEngineVersionsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.m2.paginators.ListEngineVersionsPublisher publisher = client.listEngineVersionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.m2.paginators.ListEngineVersionsPublisher publisher = client.listEngineVersionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.m2.model.ListEngineVersionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listEngineVersions(software.amazon.awssdk.services.m2.model.ListEngineVersionsRequest)} operation.
*
*
* @param listEngineVersionsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListEngineVersions
* @see AWS API
* Documentation
*/
default ListEngineVersionsPublisher listEngineVersionsPaginator(ListEngineVersionsRequest listEngineVersionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the available engine versions.
*
*
*
* This is a variant of
* {@link #listEngineVersions(software.amazon.awssdk.services.m2.model.ListEngineVersionsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.m2.paginators.ListEngineVersionsPublisher publisher = client.listEngineVersionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.m2.paginators.ListEngineVersionsPublisher publisher = client.listEngineVersionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.m2.model.ListEngineVersionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listEngineVersions(software.amazon.awssdk.services.m2.model.ListEngineVersionsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListEngineVersionsRequest.Builder} avoiding the
* need to create one manually via {@link ListEngineVersionsRequest#builder()}
*
*
* @param listEngineVersionsRequest
* A {@link Consumer} that will call methods on {@link ListEngineVersionsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListEngineVersions
* @see AWS API
* Documentation
*/
default ListEngineVersionsPublisher listEngineVersionsPaginator(
Consumer listEngineVersionsRequest) {
return listEngineVersionsPaginator(ListEngineVersionsRequest.builder().applyMutation(listEngineVersionsRequest).build());
}
/**
*
* Lists the runtime environments.
*
*
* @param listEnvironmentsRequest
* @return A Java Future containing the result of the ListEnvironments operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListEnvironments
* @see AWS API
* Documentation
*/
default CompletableFuture listEnvironments(ListEnvironmentsRequest listEnvironmentsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the runtime environments.
*
*
*
* This is a convenience which creates an instance of the {@link ListEnvironmentsRequest.Builder} avoiding the need
* to create one manually via {@link ListEnvironmentsRequest#builder()}
*
*
* @param listEnvironmentsRequest
* A {@link Consumer} that will call methods on {@link ListEnvironmentsRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListEnvironments operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListEnvironments
* @see AWS API
* Documentation
*/
default CompletableFuture listEnvironments(
Consumer listEnvironmentsRequest) {
return listEnvironments(ListEnvironmentsRequest.builder().applyMutation(listEnvironmentsRequest).build());
}
/**
*
* Lists the runtime environments.
*
*
*
* This is a variant of {@link #listEnvironments(software.amazon.awssdk.services.m2.model.ListEnvironmentsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.m2.paginators.ListEnvironmentsPublisher publisher = client.listEnvironmentsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.m2.paginators.ListEnvironmentsPublisher publisher = client.listEnvironmentsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.m2.model.ListEnvironmentsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listEnvironments(software.amazon.awssdk.services.m2.model.ListEnvironmentsRequest)} operation.
*
*
* @param listEnvironmentsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListEnvironments
* @see AWS API
* Documentation
*/
default ListEnvironmentsPublisher listEnvironmentsPaginator(ListEnvironmentsRequest listEnvironmentsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the runtime environments.
*
*
*
* This is a variant of {@link #listEnvironments(software.amazon.awssdk.services.m2.model.ListEnvironmentsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.m2.paginators.ListEnvironmentsPublisher publisher = client.listEnvironmentsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.m2.paginators.ListEnvironmentsPublisher publisher = client.listEnvironmentsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.m2.model.ListEnvironmentsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listEnvironments(software.amazon.awssdk.services.m2.model.ListEnvironmentsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListEnvironmentsRequest.Builder} avoiding the need
* to create one manually via {@link ListEnvironmentsRequest#builder()}
*
*
* @param listEnvironmentsRequest
* A {@link Consumer} that will call methods on {@link ListEnvironmentsRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListEnvironments
* @see AWS API
* Documentation
*/
default ListEnvironmentsPublisher listEnvironmentsPaginator(Consumer listEnvironmentsRequest) {
return listEnvironmentsPaginator(ListEnvironmentsRequest.builder().applyMutation(listEnvironmentsRequest).build());
}
/**
*
* Lists the tags for the specified resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListTagsForResource
* @see AWS API
* Documentation
*/
default CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the tags for the specified resource.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on {@link ListTagsForResourceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListTagsForResource
* @see AWS API
* Documentation
*/
default CompletableFuture listTagsForResource(
Consumer listTagsForResourceRequest) {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Starts an application that is currently stopped.
*
*
* @param startApplicationRequest
* @return A Java Future containing the result of the StartApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.StartApplication
* @see AWS API
* Documentation
*/
default CompletableFuture startApplication(StartApplicationRequest startApplicationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Starts an application that is currently stopped.
*
*
*
* This is a convenience which creates an instance of the {@link StartApplicationRequest.Builder} avoiding the need
* to create one manually via {@link StartApplicationRequest#builder()}
*
*
* @param startApplicationRequest
* A {@link Consumer} that will call methods on {@link StartApplicationRequest.Builder} to create a request.
* @return A Java Future containing the result of the StartApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.StartApplication
* @see AWS API
* Documentation
*/
default CompletableFuture startApplication(
Consumer startApplicationRequest) {
return startApplication(StartApplicationRequest.builder().applyMutation(startApplicationRequest).build());
}
/**
*
* Starts a batch job and returns the unique identifier of this execution of the batch job. The associated
* application must be running in order to start the batch job.
*
*
* @param startBatchJobRequest
* @return A Java Future containing the result of the StartBatchJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.StartBatchJob
* @see AWS API
* Documentation
*/
default CompletableFuture startBatchJob(StartBatchJobRequest startBatchJobRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Starts a batch job and returns the unique identifier of this execution of the batch job. The associated
* application must be running in order to start the batch job.
*
*
*
* This is a convenience which creates an instance of the {@link StartBatchJobRequest.Builder} avoiding the need to
* create one manually via {@link StartBatchJobRequest#builder()}
*
*
* @param startBatchJobRequest
* A {@link Consumer} that will call methods on {@link StartBatchJobRequest.Builder} to create a request.
* @return A Java Future containing the result of the StartBatchJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.StartBatchJob
* @see AWS API
* Documentation
*/
default CompletableFuture startBatchJob(Consumer startBatchJobRequest) {
return startBatchJob(StartBatchJobRequest.builder().applyMutation(startBatchJobRequest).build());
}
/**
*
* Stops a running application.
*
*
* @param stopApplicationRequest
* @return A Java Future containing the result of the StopApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.StopApplication
* @see AWS API
* Documentation
*/
default CompletableFuture stopApplication(StopApplicationRequest stopApplicationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Stops a running application.
*
*
*
* This is a convenience which creates an instance of the {@link StopApplicationRequest.Builder} avoiding the need
* to create one manually via {@link StopApplicationRequest#builder()}
*
*
* @param stopApplicationRequest
* A {@link Consumer} that will call methods on {@link StopApplicationRequest.Builder} to create a request.
* @return A Java Future containing the result of the StopApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.StopApplication
* @see AWS API
* Documentation
*/
default CompletableFuture stopApplication(
Consumer stopApplicationRequest) {
return stopApplication(StopApplicationRequest.builder().applyMutation(stopApplicationRequest).build());
}
/**
*
* Adds one or more tags to the specified resource.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - ServiceQuotaExceededException One or more quotas for Amazon Web Services Mainframe Modernization
* exceeds the limit.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds one or more tags to the specified resource.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on {@link TagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - ServiceQuotaExceededException One or more quotas for Amazon Web Services Mainframe Modernization
* exceeds the limit.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(Consumer tagResourceRequest) {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Removes one or more tags from the specified resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes one or more tags from the specified resource.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on {@link UntagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(Consumer untagResourceRequest) {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
*
* Updates an application and creates a new version.
*
*
* @param updateApplicationRequest
* @return A Java Future containing the result of the UpdateApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.UpdateApplication
* @see AWS API
* Documentation
*/
default CompletableFuture updateApplication(UpdateApplicationRequest updateApplicationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates an application and creates a new version.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateApplicationRequest.Builder} avoiding the need
* to create one manually via {@link UpdateApplicationRequest#builder()}
*
*
* @param updateApplicationRequest
* A {@link Consumer} that will call methods on {@link UpdateApplicationRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.UpdateApplication
* @see AWS API
* Documentation
*/
default CompletableFuture updateApplication(
Consumer updateApplicationRequest) {
return updateApplication(UpdateApplicationRequest.builder().applyMutation(updateApplicationRequest).build());
}
/**
*
* Updates the configuration details for a specific environment.
*
*
* @param updateEnvironmentRequest
* @return A Java Future containing the result of the UpdateEnvironment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - ServiceQuotaExceededException One or more quotas for Amazon Web Services Mainframe Modernization
* exceeds the limit.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.UpdateEnvironment
* @see AWS API
* Documentation
*/
default CompletableFuture updateEnvironment(UpdateEnvironmentRequest updateEnvironmentRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the configuration details for a specific environment.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateEnvironmentRequest.Builder} avoiding the need
* to create one manually via {@link UpdateEnvironmentRequest#builder()}
*
*
* @param updateEnvironmentRequest
* A {@link Consumer} that will call methods on {@link UpdateEnvironmentRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateEnvironment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException One or more parameters provided in the request is not valid.
* - ServiceQuotaExceededException One or more quotas for Amazon Web Services Mainframe Modernization
* exceeds the limit.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ThrottlingException The number of requests made exceeds the limit.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.UpdateEnvironment
* @see AWS API
* Documentation
*/
default CompletableFuture updateEnvironment(
Consumer updateEnvironmentRequest) {
return updateEnvironment(UpdateEnvironmentRequest.builder().applyMutation(updateEnvironmentRequest).build());
}
}