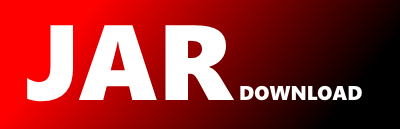
software.amazon.awssdk.services.m2.model.VsamDetailAttributes Maven / Gradle / Ivy
Show all versions of m2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.m2.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The attributes of a VSAM type data set.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class VsamDetailAttributes implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField> ALTERNATE_KEYS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("alternateKeys")
.getter(getter(VsamDetailAttributes::alternateKeys))
.setter(setter(Builder::alternateKeys))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("alternateKeys").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(AlternateKey::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField CACHE_AT_STARTUP_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("cacheAtStartup").getter(getter(VsamDetailAttributes::cacheAtStartup))
.setter(setter(Builder::cacheAtStartup))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("cacheAtStartup").build()).build();
private static final SdkField COMPRESSED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("compressed").getter(getter(VsamDetailAttributes::compressed)).setter(setter(Builder::compressed))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("compressed").build()).build();
private static final SdkField ENCODING_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("encoding").getter(getter(VsamDetailAttributes::encoding)).setter(setter(Builder::encoding))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("encoding").build()).build();
private static final SdkField PRIMARY_KEY_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("primaryKey").getter(getter(VsamDetailAttributes::primaryKey)).setter(setter(Builder::primaryKey))
.constructor(PrimaryKey::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("primaryKey").build()).build();
private static final SdkField RECORD_FORMAT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("recordFormat").getter(getter(VsamDetailAttributes::recordFormat)).setter(setter(Builder::recordFormat))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("recordFormat").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ALTERNATE_KEYS_FIELD,
CACHE_AT_STARTUP_FIELD, COMPRESSED_FIELD, ENCODING_FIELD, PRIMARY_KEY_FIELD, RECORD_FORMAT_FIELD));
private static final long serialVersionUID = 1L;
private final List alternateKeys;
private final Boolean cacheAtStartup;
private final Boolean compressed;
private final String encoding;
private final PrimaryKey primaryKey;
private final String recordFormat;
private VsamDetailAttributes(BuilderImpl builder) {
this.alternateKeys = builder.alternateKeys;
this.cacheAtStartup = builder.cacheAtStartup;
this.compressed = builder.compressed;
this.encoding = builder.encoding;
this.primaryKey = builder.primaryKey;
this.recordFormat = builder.recordFormat;
}
/**
* For responses, this returns true if the service returned a value for the AlternateKeys property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasAlternateKeys() {
return alternateKeys != null && !(alternateKeys instanceof SdkAutoConstructList);
}
/**
*
* The alternate key definitions, if any. A legacy dataset might not have any alternate key defined, but if those
* alternate keys definitions exist, provide them as some applications will make use of them.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAlternateKeys} method.
*
*
* @return The alternate key definitions, if any. A legacy dataset might not have any alternate key defined, but if
* those alternate keys definitions exist, provide them as some applications will make use of them.
*/
public final List alternateKeys() {
return alternateKeys;
}
/**
*
* If set to True, enforces loading the data set into cache before it’s used by the application.
*
*
* @return If set to True, enforces loading the data set into cache before it’s used by the application.
*/
public final Boolean cacheAtStartup() {
return cacheAtStartup;
}
/**
*
* Indicates whether indexes for this dataset are stored as compressed values. If you have a large data set
* (typically > 100 Mb), consider setting this flag to True.
*
*
* @return Indicates whether indexes for this dataset are stored as compressed values. If you have a large data set
* (typically > 100 Mb), consider setting this flag to True.
*/
public final Boolean compressed() {
return compressed;
}
/**
*
* The character set used by the data set. Can be ASCII, EBCDIC, or unknown.
*
*
* @return The character set used by the data set. Can be ASCII, EBCDIC, or unknown.
*/
public final String encoding() {
return encoding;
}
/**
*
* The primary key of the data set.
*
*
* @return The primary key of the data set.
*/
public final PrimaryKey primaryKey() {
return primaryKey;
}
/**
*
* The record format of the data set.
*
*
* @return The record format of the data set.
*/
public final String recordFormat() {
return recordFormat;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(hasAlternateKeys() ? alternateKeys() : null);
hashCode = 31 * hashCode + Objects.hashCode(cacheAtStartup());
hashCode = 31 * hashCode + Objects.hashCode(compressed());
hashCode = 31 * hashCode + Objects.hashCode(encoding());
hashCode = 31 * hashCode + Objects.hashCode(primaryKey());
hashCode = 31 * hashCode + Objects.hashCode(recordFormat());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof VsamDetailAttributes)) {
return false;
}
VsamDetailAttributes other = (VsamDetailAttributes) obj;
return hasAlternateKeys() == other.hasAlternateKeys() && Objects.equals(alternateKeys(), other.alternateKeys())
&& Objects.equals(cacheAtStartup(), other.cacheAtStartup()) && Objects.equals(compressed(), other.compressed())
&& Objects.equals(encoding(), other.encoding()) && Objects.equals(primaryKey(), other.primaryKey())
&& Objects.equals(recordFormat(), other.recordFormat());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("VsamDetailAttributes").add("AlternateKeys", hasAlternateKeys() ? alternateKeys() : null)
.add("CacheAtStartup", cacheAtStartup()).add("Compressed", compressed()).add("Encoding", encoding())
.add("PrimaryKey", primaryKey()).add("RecordFormat", recordFormat()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "alternateKeys":
return Optional.ofNullable(clazz.cast(alternateKeys()));
case "cacheAtStartup":
return Optional.ofNullable(clazz.cast(cacheAtStartup()));
case "compressed":
return Optional.ofNullable(clazz.cast(compressed()));
case "encoding":
return Optional.ofNullable(clazz.cast(encoding()));
case "primaryKey":
return Optional.ofNullable(clazz.cast(primaryKey()));
case "recordFormat":
return Optional.ofNullable(clazz.cast(recordFormat()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function