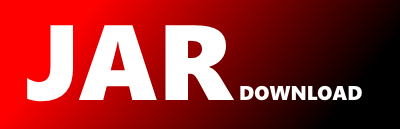
software.amazon.awssdk.services.m2.model.UpdateEnvironmentRequest Maven / Gradle / Ivy
Show all versions of m2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.m2.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class UpdateEnvironmentRequest extends M2Request implements
ToCopyableBuilder {
private static final SdkField APPLY_DURING_MAINTENANCE_WINDOW_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("applyDuringMaintenanceWindow")
.getter(getter(UpdateEnvironmentRequest::applyDuringMaintenanceWindow))
.setter(setter(Builder::applyDuringMaintenanceWindow))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("applyDuringMaintenanceWindow")
.build()).build();
private static final SdkField DESIRED_CAPACITY_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("desiredCapacity").getter(getter(UpdateEnvironmentRequest::desiredCapacity))
.setter(setter(Builder::desiredCapacity))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("desiredCapacity").build()).build();
private static final SdkField ENGINE_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("engineVersion").getter(getter(UpdateEnvironmentRequest::engineVersion))
.setter(setter(Builder::engineVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("engineVersion").build()).build();
private static final SdkField ENVIRONMENT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("environmentId").getter(getter(UpdateEnvironmentRequest::environmentId))
.setter(setter(Builder::environmentId))
.traits(LocationTrait.builder().location(MarshallLocation.PATH).locationName("environmentId").build()).build();
private static final SdkField FORCE_UPDATE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("forceUpdate").getter(getter(UpdateEnvironmentRequest::forceUpdate)).setter(setter(Builder::forceUpdate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("forceUpdate").build()).build();
private static final SdkField INSTANCE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("instanceType").getter(getter(UpdateEnvironmentRequest::instanceType))
.setter(setter(Builder::instanceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("instanceType").build()).build();
private static final SdkField PREFERRED_MAINTENANCE_WINDOW_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("preferredMaintenanceWindow")
.getter(getter(UpdateEnvironmentRequest::preferredMaintenanceWindow))
.setter(setter(Builder::preferredMaintenanceWindow))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("preferredMaintenanceWindow").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(
APPLY_DURING_MAINTENANCE_WINDOW_FIELD, DESIRED_CAPACITY_FIELD, ENGINE_VERSION_FIELD, ENVIRONMENT_ID_FIELD,
FORCE_UPDATE_FIELD, INSTANCE_TYPE_FIELD, PREFERRED_MAINTENANCE_WINDOW_FIELD));
private final Boolean applyDuringMaintenanceWindow;
private final Integer desiredCapacity;
private final String engineVersion;
private final String environmentId;
private final Boolean forceUpdate;
private final String instanceType;
private final String preferredMaintenanceWindow;
private UpdateEnvironmentRequest(BuilderImpl builder) {
super(builder);
this.applyDuringMaintenanceWindow = builder.applyDuringMaintenanceWindow;
this.desiredCapacity = builder.desiredCapacity;
this.engineVersion = builder.engineVersion;
this.environmentId = builder.environmentId;
this.forceUpdate = builder.forceUpdate;
this.instanceType = builder.instanceType;
this.preferredMaintenanceWindow = builder.preferredMaintenanceWindow;
}
/**
*
* Indicates whether to update the runtime environment during the maintenance window. The default is false.
* Currently, Amazon Web Services Mainframe Modernization accepts the engineVersion
parameter only if
* applyDuringMaintenanceWindow
is true. If any parameter other than engineVersion
is
* provided in UpdateEnvironmentRequest
, it will fail if applyDuringMaintenanceWindow
is
* set to true.
*
*
* @return Indicates whether to update the runtime environment during the maintenance window. The default is false.
* Currently, Amazon Web Services Mainframe Modernization accepts the engineVersion
parameter
* only if applyDuringMaintenanceWindow
is true. If any parameter other than
* engineVersion
is provided in UpdateEnvironmentRequest
, it will fail if
* applyDuringMaintenanceWindow
is set to true.
*/
public final Boolean applyDuringMaintenanceWindow() {
return applyDuringMaintenanceWindow;
}
/**
*
* The desired capacity for the runtime environment to update. The minimum possible value is 0 and the maximum is
* 100.
*
*
* @return The desired capacity for the runtime environment to update. The minimum possible value is 0 and the
* maximum is 100.
*/
public final Integer desiredCapacity() {
return desiredCapacity;
}
/**
*
* The version of the runtime engine for the runtime environment.
*
*
* @return The version of the runtime engine for the runtime environment.
*/
public final String engineVersion() {
return engineVersion;
}
/**
*
* The unique identifier of the runtime environment that you want to update.
*
*
* @return The unique identifier of the runtime environment that you want to update.
*/
public final String environmentId() {
return environmentId;
}
/**
*
* Forces the updates on the environment. This option is needed if the applications in the environment are not
* stopped or if there are ongoing application-related activities in the environment.
*
*
* If you use this option, be aware that it could lead to data corruption in the applications, and that you might
* need to perform repair and recovery procedures for the applications.
*
*
* This option is not needed if the attribute being updated is preferredMaintenanceWindow
.
*
*
* @return Forces the updates on the environment. This option is needed if the applications in the environment are
* not stopped or if there are ongoing application-related activities in the environment.
*
* If you use this option, be aware that it could lead to data corruption in the applications, and that you
* might need to perform repair and recovery procedures for the applications.
*
*
* This option is not needed if the attribute being updated is preferredMaintenanceWindow
.
*/
public final Boolean forceUpdate() {
return forceUpdate;
}
/**
*
* The instance type for the runtime environment to update.
*
*
* @return The instance type for the runtime environment to update.
*/
public final String instanceType() {
return instanceType;
}
/**
*
* Configures the maintenance window that you want for the runtime environment. The maintenance window must have the
* format ddd:hh24:mi-ddd:hh24:mi
and must be less than 24 hours. The following two examples are valid
* maintenance windows: sun:23:45-mon:00:15
or sat:01:00-sat:03:00
.
*
*
* If you do not provide a value, a random system-generated value will be assigned.
*
*
* @return Configures the maintenance window that you want for the runtime environment. The maintenance window must
* have the format ddd:hh24:mi-ddd:hh24:mi
and must be less than 24 hours. The following two
* examples are valid maintenance windows: sun:23:45-mon:00:15
or
* sat:01:00-sat:03:00
.
*
* If you do not provide a value, a random system-generated value will be assigned.
*/
public final String preferredMaintenanceWindow() {
return preferredMaintenanceWindow;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(applyDuringMaintenanceWindow());
hashCode = 31 * hashCode + Objects.hashCode(desiredCapacity());
hashCode = 31 * hashCode + Objects.hashCode(engineVersion());
hashCode = 31 * hashCode + Objects.hashCode(environmentId());
hashCode = 31 * hashCode + Objects.hashCode(forceUpdate());
hashCode = 31 * hashCode + Objects.hashCode(instanceType());
hashCode = 31 * hashCode + Objects.hashCode(preferredMaintenanceWindow());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UpdateEnvironmentRequest)) {
return false;
}
UpdateEnvironmentRequest other = (UpdateEnvironmentRequest) obj;
return Objects.equals(applyDuringMaintenanceWindow(), other.applyDuringMaintenanceWindow())
&& Objects.equals(desiredCapacity(), other.desiredCapacity())
&& Objects.equals(engineVersion(), other.engineVersion())
&& Objects.equals(environmentId(), other.environmentId()) && Objects.equals(forceUpdate(), other.forceUpdate())
&& Objects.equals(instanceType(), other.instanceType())
&& Objects.equals(preferredMaintenanceWindow(), other.preferredMaintenanceWindow());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("UpdateEnvironmentRequest").add("ApplyDuringMaintenanceWindow", applyDuringMaintenanceWindow())
.add("DesiredCapacity", desiredCapacity()).add("EngineVersion", engineVersion())
.add("EnvironmentId", environmentId()).add("ForceUpdate", forceUpdate()).add("InstanceType", instanceType())
.add("PreferredMaintenanceWindow", preferredMaintenanceWindow()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "applyDuringMaintenanceWindow":
return Optional.ofNullable(clazz.cast(applyDuringMaintenanceWindow()));
case "desiredCapacity":
return Optional.ofNullable(clazz.cast(desiredCapacity()));
case "engineVersion":
return Optional.ofNullable(clazz.cast(engineVersion()));
case "environmentId":
return Optional.ofNullable(clazz.cast(environmentId()));
case "forceUpdate":
return Optional.ofNullable(clazz.cast(forceUpdate()));
case "instanceType":
return Optional.ofNullable(clazz.cast(instanceType()));
case "preferredMaintenanceWindow":
return Optional.ofNullable(clazz.cast(preferredMaintenanceWindow()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* If you use this option, be aware that it could lead to data corruption in the applications, and that
* you might need to perform repair and recovery procedures for the applications.
*
*
* This option is not needed if the attribute being updated is preferredMaintenanceWindow
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder forceUpdate(Boolean forceUpdate);
/**
*
* The instance type for the runtime environment to update.
*
*
* @param instanceType
* The instance type for the runtime environment to update.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder instanceType(String instanceType);
/**
*
* Configures the maintenance window that you want for the runtime environment. The maintenance window must have
* the format ddd:hh24:mi-ddd:hh24:mi
and must be less than 24 hours. The following two examples
* are valid maintenance windows: sun:23:45-mon:00:15
or sat:01:00-sat:03:00
.
*
*
* If you do not provide a value, a random system-generated value will be assigned.
*
*
* @param preferredMaintenanceWindow
* Configures the maintenance window that you want for the runtime environment. The maintenance window
* must have the format ddd:hh24:mi-ddd:hh24:mi
and must be less than 24 hours. The
* following two examples are valid maintenance windows: sun:23:45-mon:00:15
or
* sat:01:00-sat:03:00
.
*
* If you do not provide a value, a random system-generated value will be assigned.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder preferredMaintenanceWindow(String preferredMaintenanceWindow);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends M2Request.BuilderImpl implements Builder {
private Boolean applyDuringMaintenanceWindow;
private Integer desiredCapacity;
private String engineVersion;
private String environmentId;
private Boolean forceUpdate;
private String instanceType;
private String preferredMaintenanceWindow;
private BuilderImpl() {
}
private BuilderImpl(UpdateEnvironmentRequest model) {
super(model);
applyDuringMaintenanceWindow(model.applyDuringMaintenanceWindow);
desiredCapacity(model.desiredCapacity);
engineVersion(model.engineVersion);
environmentId(model.environmentId);
forceUpdate(model.forceUpdate);
instanceType(model.instanceType);
preferredMaintenanceWindow(model.preferredMaintenanceWindow);
}
public final Boolean getApplyDuringMaintenanceWindow() {
return applyDuringMaintenanceWindow;
}
public final void setApplyDuringMaintenanceWindow(Boolean applyDuringMaintenanceWindow) {
this.applyDuringMaintenanceWindow = applyDuringMaintenanceWindow;
}
@Override
public final Builder applyDuringMaintenanceWindow(Boolean applyDuringMaintenanceWindow) {
this.applyDuringMaintenanceWindow = applyDuringMaintenanceWindow;
return this;
}
public final Integer getDesiredCapacity() {
return desiredCapacity;
}
public final void setDesiredCapacity(Integer desiredCapacity) {
this.desiredCapacity = desiredCapacity;
}
@Override
public final Builder desiredCapacity(Integer desiredCapacity) {
this.desiredCapacity = desiredCapacity;
return this;
}
public final String getEngineVersion() {
return engineVersion;
}
public final void setEngineVersion(String engineVersion) {
this.engineVersion = engineVersion;
}
@Override
public final Builder engineVersion(String engineVersion) {
this.engineVersion = engineVersion;
return this;
}
public final String getEnvironmentId() {
return environmentId;
}
public final void setEnvironmentId(String environmentId) {
this.environmentId = environmentId;
}
@Override
public final Builder environmentId(String environmentId) {
this.environmentId = environmentId;
return this;
}
public final Boolean getForceUpdate() {
return forceUpdate;
}
public final void setForceUpdate(Boolean forceUpdate) {
this.forceUpdate = forceUpdate;
}
@Override
public final Builder forceUpdate(Boolean forceUpdate) {
this.forceUpdate = forceUpdate;
return this;
}
public final String getInstanceType() {
return instanceType;
}
public final void setInstanceType(String instanceType) {
this.instanceType = instanceType;
}
@Override
public final Builder instanceType(String instanceType) {
this.instanceType = instanceType;
return this;
}
public final String getPreferredMaintenanceWindow() {
return preferredMaintenanceWindow;
}
public final void setPreferredMaintenanceWindow(String preferredMaintenanceWindow) {
this.preferredMaintenanceWindow = preferredMaintenanceWindow;
}
@Override
public final Builder preferredMaintenanceWindow(String preferredMaintenanceWindow) {
this.preferredMaintenanceWindow = preferredMaintenanceWindow;
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public UpdateEnvironmentRequest build() {
return new UpdateEnvironmentRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}