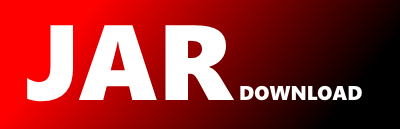
software.amazon.awssdk.services.m2.DefaultM2AsyncClient Maven / Gradle / Ivy
Show all versions of m2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.m2;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import java.util.function.Function;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.AwsProtocolMetadata;
import software.amazon.awssdk.awscore.internal.AwsServiceProtocol;
import software.amazon.awssdk.awscore.retry.AwsRetryStrategy;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkPlugin;
import software.amazon.awssdk.core.SdkRequest;
import software.amazon.awssdk.core.client.config.ClientOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.retry.RetryMode;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.retries.api.RetryStrategy;
import software.amazon.awssdk.services.m2.internal.M2ServiceClientConfigurationBuilder;
import software.amazon.awssdk.services.m2.model.AccessDeniedException;
import software.amazon.awssdk.services.m2.model.CancelBatchJobExecutionRequest;
import software.amazon.awssdk.services.m2.model.CancelBatchJobExecutionResponse;
import software.amazon.awssdk.services.m2.model.ConflictException;
import software.amazon.awssdk.services.m2.model.CreateApplicationRequest;
import software.amazon.awssdk.services.m2.model.CreateApplicationResponse;
import software.amazon.awssdk.services.m2.model.CreateDataSetImportTaskRequest;
import software.amazon.awssdk.services.m2.model.CreateDataSetImportTaskResponse;
import software.amazon.awssdk.services.m2.model.CreateDeploymentRequest;
import software.amazon.awssdk.services.m2.model.CreateDeploymentResponse;
import software.amazon.awssdk.services.m2.model.CreateEnvironmentRequest;
import software.amazon.awssdk.services.m2.model.CreateEnvironmentResponse;
import software.amazon.awssdk.services.m2.model.DeleteApplicationFromEnvironmentRequest;
import software.amazon.awssdk.services.m2.model.DeleteApplicationFromEnvironmentResponse;
import software.amazon.awssdk.services.m2.model.DeleteApplicationRequest;
import software.amazon.awssdk.services.m2.model.DeleteApplicationResponse;
import software.amazon.awssdk.services.m2.model.DeleteEnvironmentRequest;
import software.amazon.awssdk.services.m2.model.DeleteEnvironmentResponse;
import software.amazon.awssdk.services.m2.model.ExecutionTimeoutException;
import software.amazon.awssdk.services.m2.model.GetApplicationRequest;
import software.amazon.awssdk.services.m2.model.GetApplicationResponse;
import software.amazon.awssdk.services.m2.model.GetApplicationVersionRequest;
import software.amazon.awssdk.services.m2.model.GetApplicationVersionResponse;
import software.amazon.awssdk.services.m2.model.GetBatchJobExecutionRequest;
import software.amazon.awssdk.services.m2.model.GetBatchJobExecutionResponse;
import software.amazon.awssdk.services.m2.model.GetDataSetDetailsRequest;
import software.amazon.awssdk.services.m2.model.GetDataSetDetailsResponse;
import software.amazon.awssdk.services.m2.model.GetDataSetImportTaskRequest;
import software.amazon.awssdk.services.m2.model.GetDataSetImportTaskResponse;
import software.amazon.awssdk.services.m2.model.GetDeploymentRequest;
import software.amazon.awssdk.services.m2.model.GetDeploymentResponse;
import software.amazon.awssdk.services.m2.model.GetEnvironmentRequest;
import software.amazon.awssdk.services.m2.model.GetEnvironmentResponse;
import software.amazon.awssdk.services.m2.model.GetSignedBluinsightsUrlRequest;
import software.amazon.awssdk.services.m2.model.GetSignedBluinsightsUrlResponse;
import software.amazon.awssdk.services.m2.model.InternalServerException;
import software.amazon.awssdk.services.m2.model.ListApplicationVersionsRequest;
import software.amazon.awssdk.services.m2.model.ListApplicationVersionsResponse;
import software.amazon.awssdk.services.m2.model.ListApplicationsRequest;
import software.amazon.awssdk.services.m2.model.ListApplicationsResponse;
import software.amazon.awssdk.services.m2.model.ListBatchJobDefinitionsRequest;
import software.amazon.awssdk.services.m2.model.ListBatchJobDefinitionsResponse;
import software.amazon.awssdk.services.m2.model.ListBatchJobExecutionsRequest;
import software.amazon.awssdk.services.m2.model.ListBatchJobExecutionsResponse;
import software.amazon.awssdk.services.m2.model.ListBatchJobRestartPointsRequest;
import software.amazon.awssdk.services.m2.model.ListBatchJobRestartPointsResponse;
import software.amazon.awssdk.services.m2.model.ListDataSetImportHistoryRequest;
import software.amazon.awssdk.services.m2.model.ListDataSetImportHistoryResponse;
import software.amazon.awssdk.services.m2.model.ListDataSetsRequest;
import software.amazon.awssdk.services.m2.model.ListDataSetsResponse;
import software.amazon.awssdk.services.m2.model.ListDeploymentsRequest;
import software.amazon.awssdk.services.m2.model.ListDeploymentsResponse;
import software.amazon.awssdk.services.m2.model.ListEngineVersionsRequest;
import software.amazon.awssdk.services.m2.model.ListEngineVersionsResponse;
import software.amazon.awssdk.services.m2.model.ListEnvironmentsRequest;
import software.amazon.awssdk.services.m2.model.ListEnvironmentsResponse;
import software.amazon.awssdk.services.m2.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.m2.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.m2.model.M2Exception;
import software.amazon.awssdk.services.m2.model.ResourceNotFoundException;
import software.amazon.awssdk.services.m2.model.ServiceQuotaExceededException;
import software.amazon.awssdk.services.m2.model.ServiceUnavailableException;
import software.amazon.awssdk.services.m2.model.StartApplicationRequest;
import software.amazon.awssdk.services.m2.model.StartApplicationResponse;
import software.amazon.awssdk.services.m2.model.StartBatchJobRequest;
import software.amazon.awssdk.services.m2.model.StartBatchJobResponse;
import software.amazon.awssdk.services.m2.model.StopApplicationRequest;
import software.amazon.awssdk.services.m2.model.StopApplicationResponse;
import software.amazon.awssdk.services.m2.model.TagResourceRequest;
import software.amazon.awssdk.services.m2.model.TagResourceResponse;
import software.amazon.awssdk.services.m2.model.ThrottlingException;
import software.amazon.awssdk.services.m2.model.UntagResourceRequest;
import software.amazon.awssdk.services.m2.model.UntagResourceResponse;
import software.amazon.awssdk.services.m2.model.UpdateApplicationRequest;
import software.amazon.awssdk.services.m2.model.UpdateApplicationResponse;
import software.amazon.awssdk.services.m2.model.UpdateEnvironmentRequest;
import software.amazon.awssdk.services.m2.model.UpdateEnvironmentResponse;
import software.amazon.awssdk.services.m2.model.ValidationException;
import software.amazon.awssdk.services.m2.transform.CancelBatchJobExecutionRequestMarshaller;
import software.amazon.awssdk.services.m2.transform.CreateApplicationRequestMarshaller;
import software.amazon.awssdk.services.m2.transform.CreateDataSetImportTaskRequestMarshaller;
import software.amazon.awssdk.services.m2.transform.CreateDeploymentRequestMarshaller;
import software.amazon.awssdk.services.m2.transform.CreateEnvironmentRequestMarshaller;
import software.amazon.awssdk.services.m2.transform.DeleteApplicationFromEnvironmentRequestMarshaller;
import software.amazon.awssdk.services.m2.transform.DeleteApplicationRequestMarshaller;
import software.amazon.awssdk.services.m2.transform.DeleteEnvironmentRequestMarshaller;
import software.amazon.awssdk.services.m2.transform.GetApplicationRequestMarshaller;
import software.amazon.awssdk.services.m2.transform.GetApplicationVersionRequestMarshaller;
import software.amazon.awssdk.services.m2.transform.GetBatchJobExecutionRequestMarshaller;
import software.amazon.awssdk.services.m2.transform.GetDataSetDetailsRequestMarshaller;
import software.amazon.awssdk.services.m2.transform.GetDataSetImportTaskRequestMarshaller;
import software.amazon.awssdk.services.m2.transform.GetDeploymentRequestMarshaller;
import software.amazon.awssdk.services.m2.transform.GetEnvironmentRequestMarshaller;
import software.amazon.awssdk.services.m2.transform.GetSignedBluinsightsUrlRequestMarshaller;
import software.amazon.awssdk.services.m2.transform.ListApplicationVersionsRequestMarshaller;
import software.amazon.awssdk.services.m2.transform.ListApplicationsRequestMarshaller;
import software.amazon.awssdk.services.m2.transform.ListBatchJobDefinitionsRequestMarshaller;
import software.amazon.awssdk.services.m2.transform.ListBatchJobExecutionsRequestMarshaller;
import software.amazon.awssdk.services.m2.transform.ListBatchJobRestartPointsRequestMarshaller;
import software.amazon.awssdk.services.m2.transform.ListDataSetImportHistoryRequestMarshaller;
import software.amazon.awssdk.services.m2.transform.ListDataSetsRequestMarshaller;
import software.amazon.awssdk.services.m2.transform.ListDeploymentsRequestMarshaller;
import software.amazon.awssdk.services.m2.transform.ListEngineVersionsRequestMarshaller;
import software.amazon.awssdk.services.m2.transform.ListEnvironmentsRequestMarshaller;
import software.amazon.awssdk.services.m2.transform.ListTagsForResourceRequestMarshaller;
import software.amazon.awssdk.services.m2.transform.StartApplicationRequestMarshaller;
import software.amazon.awssdk.services.m2.transform.StartBatchJobRequestMarshaller;
import software.amazon.awssdk.services.m2.transform.StopApplicationRequestMarshaller;
import software.amazon.awssdk.services.m2.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.m2.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.m2.transform.UpdateApplicationRequestMarshaller;
import software.amazon.awssdk.services.m2.transform.UpdateEnvironmentRequestMarshaller;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link M2AsyncClient}.
*
* @see M2AsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultM2AsyncClient implements M2AsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultM2AsyncClient.class);
private static final AwsProtocolMetadata protocolMetadata = AwsProtocolMetadata.builder()
.serviceProtocol(AwsServiceProtocol.REST_JSON).build();
private final AsyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultM2AsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration.toBuilder().option(SdkClientOption.SDK_CLIENT, this).build();
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
/**
*
* Cancels the running of a specific batch job execution.
*
*
* @param cancelBatchJobExecutionRequest
* @return A Java Future containing the result of the CancelBatchJobExecution operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The number of requests made exceeds the limit.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.CancelBatchJobExecution
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture cancelBatchJobExecution(
CancelBatchJobExecutionRequest cancelBatchJobExecutionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(cancelBatchJobExecutionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, cancelBatchJobExecutionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "m2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CancelBatchJobExecution");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CancelBatchJobExecutionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CancelBatchJobExecution").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CancelBatchJobExecutionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(cancelBatchJobExecutionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new application with given parameters. Requires an existing runtime environment and application
* definition file.
*
*
* @param createApplicationRequest
* @return A Java Future containing the result of the CreateApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServiceQuotaExceededException One or more quotas for Amazon Web Services Mainframe Modernization
* exceeds the limit.
* - ThrottlingException The number of requests made exceeds the limit.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.CreateApplication
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createApplication(CreateApplicationRequest createApplicationRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createApplicationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createApplicationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "m2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateApplication");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateApplicationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateApplication").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateApplicationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createApplicationRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Starts a data set import task for a specific application.
*
*
* @param createDataSetImportTaskRequest
* @return A Java Future containing the result of the CreateDataSetImportTask operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - ServiceQuotaExceededException One or more quotas for Amazon Web Services Mainframe Modernization
* exceeds the limit.
* - ThrottlingException The number of requests made exceeds the limit.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.CreateDataSetImportTask
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture createDataSetImportTask(
CreateDataSetImportTaskRequest createDataSetImportTaskRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createDataSetImportTaskRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createDataSetImportTaskRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "m2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateDataSetImportTask");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateDataSetImportTaskResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateDataSetImportTask").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateDataSetImportTaskRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createDataSetImportTaskRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates and starts a deployment to deploy an application into a runtime environment.
*
*
* @param createDeploymentRequest
* @return A Java Future containing the result of the CreateDeployment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - ServiceQuotaExceededException One or more quotas for Amazon Web Services Mainframe Modernization
* exceeds the limit.
* - ThrottlingException The number of requests made exceeds the limit.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.CreateDeployment
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createDeployment(CreateDeploymentRequest createDeploymentRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createDeploymentRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createDeploymentRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "m2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateDeployment");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateDeploymentResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateDeployment").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateDeploymentRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createDeploymentRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a runtime environment for a given runtime engine.
*
*
* @param createEnvironmentRequest
* @return A Java Future containing the result of the CreateEnvironment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServiceQuotaExceededException One or more quotas for Amazon Web Services Mainframe Modernization
* exceeds the limit.
* - ThrottlingException The number of requests made exceeds the limit.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.CreateEnvironment
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createEnvironment(CreateEnvironmentRequest createEnvironmentRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createEnvironmentRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createEnvironmentRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "m2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateEnvironment");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateEnvironmentResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateEnvironment").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateEnvironmentRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createEnvironmentRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a specific application. You cannot delete a running application.
*
*
* @param deleteApplicationRequest
* @return A Java Future containing the result of the DeleteApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The number of requests made exceeds the limit.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.DeleteApplication
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteApplication(DeleteApplicationRequest deleteApplicationRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteApplicationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteApplicationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "m2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteApplication");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteApplicationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteApplication").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteApplicationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteApplicationRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a specific application from the specific runtime environment where it was previously deployed. You cannot
* delete a runtime environment using DeleteEnvironment if any application has ever been deployed to it. This API
* removes the association of the application with the runtime environment so you can delete the environment
* smoothly.
*
*
* @param deleteApplicationFromEnvironmentRequest
* @return A Java Future containing the result of the DeleteApplicationFromEnvironment operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The number of requests made exceeds the limit.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.DeleteApplicationFromEnvironment
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteApplicationFromEnvironment(
DeleteApplicationFromEnvironmentRequest deleteApplicationFromEnvironmentRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteApplicationFromEnvironmentRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
deleteApplicationFromEnvironmentRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "m2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteApplicationFromEnvironment");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, DeleteApplicationFromEnvironmentResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteApplicationFromEnvironment").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteApplicationFromEnvironmentRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteApplicationFromEnvironmentRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a specific runtime environment. The environment cannot contain deployed applications. If it does, you
* must delete those applications before you delete the environment.
*
*
* @param deleteEnvironmentRequest
* @return A Java Future containing the result of the DeleteEnvironment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The number of requests made exceeds the limit.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.DeleteEnvironment
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteEnvironment(DeleteEnvironmentRequest deleteEnvironmentRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteEnvironmentRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteEnvironmentRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "m2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteEnvironment");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteEnvironmentResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteEnvironment").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteEnvironmentRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteEnvironmentRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the details of a specific application.
*
*
* @param getApplicationRequest
* @return A Java Future containing the result of the GetApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The number of requests made exceeds the limit.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.GetApplication
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getApplication(GetApplicationRequest getApplicationRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getApplicationRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getApplicationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "m2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetApplication");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetApplicationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetApplication").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetApplicationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getApplicationRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns details about a specific version of a specific application.
*
*
* @param getApplicationVersionRequest
* @return A Java Future containing the result of the GetApplicationVersion operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The number of requests made exceeds the limit.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.GetApplicationVersion
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getApplicationVersion(
GetApplicationVersionRequest getApplicationVersionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getApplicationVersionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getApplicationVersionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "m2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetApplicationVersion");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetApplicationVersionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetApplicationVersion").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetApplicationVersionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getApplicationVersionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets the details of a specific batch job execution for a specific application.
*
*
* @param getBatchJobExecutionRequest
* @return A Java Future containing the result of the GetBatchJobExecution operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The number of requests made exceeds the limit.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.GetBatchJobExecution
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getBatchJobExecution(
GetBatchJobExecutionRequest getBatchJobExecutionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getBatchJobExecutionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getBatchJobExecutionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "m2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetBatchJobExecution");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetBatchJobExecutionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetBatchJobExecution").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetBatchJobExecutionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getBatchJobExecutionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets the details of a specific data set.
*
*
* @param getDataSetDetailsRequest
* @return A Java Future containing the result of the GetDataSetDetails operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - ExecutionTimeoutException Failed to connect to server, or didn’t receive response within expected
* time period.
* - ServiceUnavailableException Server cannot process the request at the moment.
* - ThrottlingException The number of requests made exceeds the limit.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.GetDataSetDetails
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getDataSetDetails(GetDataSetDetailsRequest getDataSetDetailsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getDataSetDetailsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getDataSetDetailsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "m2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetDataSetDetails");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetDataSetDetailsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetDataSetDetails").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetDataSetDetailsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getDataSetDetailsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets the status of a data set import task initiated with the CreateDataSetImportTask operation.
*
*
* @param getDataSetImportTaskRequest
* @return A Java Future containing the result of the GetDataSetImportTask operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The number of requests made exceeds the limit.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.GetDataSetImportTask
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getDataSetImportTask(
GetDataSetImportTaskRequest getDataSetImportTaskRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getDataSetImportTaskRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getDataSetImportTaskRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "m2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetDataSetImportTask");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetDataSetImportTaskResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetDataSetImportTask").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetDataSetImportTaskRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getDataSetImportTaskRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets details of a specific deployment with a given deployment identifier.
*
*
* @param getDeploymentRequest
* @return A Java Future containing the result of the GetDeployment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The number of requests made exceeds the limit.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.GetDeployment
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getDeployment(GetDeploymentRequest getDeploymentRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getDeploymentRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getDeploymentRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "m2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetDeployment");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetDeploymentResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetDeployment").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetDeploymentRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getDeploymentRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes a specific runtime environment.
*
*
* @param getEnvironmentRequest
* @return A Java Future containing the result of the GetEnvironment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The number of requests made exceeds the limit.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.GetEnvironment
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getEnvironment(GetEnvironmentRequest getEnvironmentRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getEnvironmentRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getEnvironmentRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "m2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetEnvironment");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetEnvironmentResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetEnvironment").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetEnvironmentRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getEnvironmentRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets a single sign-on URL that can be used to connect to AWS Blu Insights.
*
*
* @param getSignedBluinsightsUrlRequest
* @return A Java Future containing the result of the GetSignedBluinsightsUrl operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The number of requests made exceeds the limit.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.GetSignedBluinsightsUrl
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture getSignedBluinsightsUrl(
GetSignedBluinsightsUrlRequest getSignedBluinsightsUrlRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getSignedBluinsightsUrlRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getSignedBluinsightsUrlRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "m2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetSignedBluinsightsUrl");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetSignedBluinsightsUrlResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetSignedBluinsightsUrl").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetSignedBluinsightsUrlRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getSignedBluinsightsUrlRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of the application versions for a specific application.
*
*
* @param listApplicationVersionsRequest
* @return A Java Future containing the result of the ListApplicationVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The number of requests made exceeds the limit.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListApplicationVersions
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listApplicationVersions(
ListApplicationVersionsRequest listApplicationVersionsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listApplicationVersionsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listApplicationVersionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "m2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListApplicationVersions");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListApplicationVersionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListApplicationVersions").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListApplicationVersionsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listApplicationVersionsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the applications associated with a specific Amazon Web Services account. You can provide the unique
* identifier of a specific runtime environment in a query parameter to see all applications associated with that
* environment.
*
*
* @param listApplicationsRequest
* @return A Java Future containing the result of the ListApplications operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The number of requests made exceeds the limit.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListApplications
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listApplications(ListApplicationsRequest listApplicationsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listApplicationsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listApplicationsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "m2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListApplications");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListApplicationsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListApplications").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListApplicationsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listApplicationsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists all the available batch job definitions based on the batch job resources uploaded during the application
* creation. You can use the batch job definitions in the list to start a batch job.
*
*
* @param listBatchJobDefinitionsRequest
* @return A Java Future containing the result of the ListBatchJobDefinitions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The number of requests made exceeds the limit.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListBatchJobDefinitions
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listBatchJobDefinitions(
ListBatchJobDefinitionsRequest listBatchJobDefinitionsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listBatchJobDefinitionsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listBatchJobDefinitionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "m2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListBatchJobDefinitions");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListBatchJobDefinitionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListBatchJobDefinitions").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListBatchJobDefinitionsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listBatchJobDefinitionsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists historical, current, and scheduled batch job executions for a specific application.
*
*
* @param listBatchJobExecutionsRequest
* @return A Java Future containing the result of the ListBatchJobExecutions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The number of requests made exceeds the limit.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListBatchJobExecutions
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listBatchJobExecutions(
ListBatchJobExecutionsRequest listBatchJobExecutionsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listBatchJobExecutionsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listBatchJobExecutionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "m2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListBatchJobExecutions");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListBatchJobExecutionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListBatchJobExecutions").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListBatchJobExecutionsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listBatchJobExecutionsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists all the job steps for a JCL file to restart a batch job. This is only applicable for Micro Focus engine
* with versions 8.0.6 and above.
*
*
* @param listBatchJobRestartPointsRequest
* @return A Java Future containing the result of the ListBatchJobRestartPoints operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The number of requests made exceeds the limit.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListBatchJobRestartPoints
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listBatchJobRestartPoints(
ListBatchJobRestartPointsRequest listBatchJobRestartPointsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listBatchJobRestartPointsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listBatchJobRestartPointsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "m2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListBatchJobRestartPoints");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListBatchJobRestartPointsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListBatchJobRestartPoints").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListBatchJobRestartPointsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listBatchJobRestartPointsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the data set imports for the specified application.
*
*
* @param listDataSetImportHistoryRequest
* @return A Java Future containing the result of the ListDataSetImportHistory operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The number of requests made exceeds the limit.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListDataSetImportHistory
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listDataSetImportHistory(
ListDataSetImportHistoryRequest listDataSetImportHistoryRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listDataSetImportHistoryRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listDataSetImportHistoryRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "m2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListDataSetImportHistory");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListDataSetImportHistoryResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListDataSetImportHistory").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListDataSetImportHistoryRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listDataSetImportHistoryRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the data sets imported for a specific application. In Amazon Web Services Mainframe Modernization, data
* sets are associated with applications deployed on runtime environments. This is known as importing data sets.
* Currently, Amazon Web Services Mainframe Modernization can import data sets into catalogs using CreateDataSetImportTask.
*
*
* @param listDataSetsRequest
* @return A Java Future containing the result of the ListDataSets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - ExecutionTimeoutException Failed to connect to server, or didn’t receive response within expected
* time period.
* - ServiceUnavailableException Server cannot process the request at the moment.
* - ThrottlingException The number of requests made exceeds the limit.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListDataSets
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listDataSets(ListDataSetsRequest listDataSetsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listDataSetsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listDataSetsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "m2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListDataSets");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListDataSetsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListDataSets").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListDataSetsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listDataSetsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of all deployments of a specific application. A deployment is a combination of a specific
* application and a specific version of that application. Each deployment is mapped to a particular application
* version.
*
*
* @param listDeploymentsRequest
* @return A Java Future containing the result of the ListDeployments operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The number of requests made exceeds the limit.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListDeployments
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listDeployments(ListDeploymentsRequest listDeploymentsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listDeploymentsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listDeploymentsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "m2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListDeployments");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListDeploymentsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListDeployments").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListDeploymentsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listDeploymentsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the available engine versions.
*
*
* @param listEngineVersionsRequest
* @return A Java Future containing the result of the ListEngineVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The number of requests made exceeds the limit.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListEngineVersions
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listEngineVersions(ListEngineVersionsRequest listEngineVersionsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listEngineVersionsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listEngineVersionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "m2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListEngineVersions");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListEngineVersionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListEngineVersions").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListEngineVersionsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listEngineVersionsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the runtime environments.
*
*
* @param listEnvironmentsRequest
* @return A Java Future containing the result of the ListEnvironments operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The number of requests made exceeds the limit.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListEnvironments
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listEnvironments(ListEnvironmentsRequest listEnvironmentsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listEnvironmentsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listEnvironmentsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "m2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListEnvironments");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListEnvironmentsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListEnvironments").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListEnvironmentsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listEnvironmentsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the tags for the specified resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The number of requests made exceeds the limit.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.ListTagsForResource
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listTagsForResourceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listTagsForResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "m2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListTagsForResource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListTagsForResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListTagsForResource").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListTagsForResourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listTagsForResourceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Starts an application that is currently stopped.
*
*
* @param startApplicationRequest
* @return A Java Future containing the result of the StartApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The number of requests made exceeds the limit.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.StartApplication
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture startApplication(StartApplicationRequest startApplicationRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(startApplicationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, startApplicationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "m2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StartApplication");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, StartApplicationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("StartApplication").withProtocolMetadata(protocolMetadata)
.withMarshaller(new StartApplicationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(startApplicationRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Starts a batch job and returns the unique identifier of this execution of the batch job. The associated
* application must be running in order to start the batch job.
*
*
* @param startBatchJobRequest
* @return A Java Future containing the result of the StartBatchJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The number of requests made exceeds the limit.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.StartBatchJob
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture startBatchJob(StartBatchJobRequest startBatchJobRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(startBatchJobRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, startBatchJobRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "m2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StartBatchJob");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
StartBatchJobResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("StartBatchJob").withProtocolMetadata(protocolMetadata)
.withMarshaller(new StartBatchJobRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(startBatchJobRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Stops a running application.
*
*
* @param stopApplicationRequest
* @return A Java Future containing the result of the StopApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The number of requests made exceeds the limit.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.StopApplication
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture stopApplication(StopApplicationRequest stopApplicationRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(stopApplicationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, stopApplicationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "m2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StopApplication");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, StopApplicationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("StopApplication").withProtocolMetadata(protocolMetadata)
.withMarshaller(new StopApplicationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(stopApplicationRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Adds one or more tags to the specified resource.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - ServiceQuotaExceededException One or more quotas for Amazon Web Services Mainframe Modernization
* exceeds the limit.
* - ThrottlingException The number of requests made exceeds the limit.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.TagResource
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(tagResourceRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, tagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "m2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "TagResource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
TagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("TagResource").withProtocolMetadata(protocolMetadata)
.withMarshaller(new TagResourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(tagResourceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Removes one or more tags from the specified resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The number of requests made exceeds the limit.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.UntagResource
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(untagResourceRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, untagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "m2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UntagResource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UntagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("UntagResource").withProtocolMetadata(protocolMetadata)
.withMarshaller(new UntagResourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(untagResourceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Updates an application and creates a new version.
*
*
* @param updateApplicationRequest
* @return A Java Future containing the result of the UpdateApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The number of requests made exceeds the limit.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.UpdateApplication
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture updateApplication(UpdateApplicationRequest updateApplicationRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(updateApplicationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateApplicationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "m2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateApplication");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, UpdateApplicationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("UpdateApplication").withProtocolMetadata(protocolMetadata)
.withMarshaller(new UpdateApplicationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(updateApplicationRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Updates the configuration details for a specific runtime environment.
*
*
* @param updateEnvironmentRequest
* @return A Java Future containing the result of the UpdateEnvironment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - ServiceQuotaExceededException One or more quotas for Amazon Web Services Mainframe Modernization
* exceeds the limit.
* - ThrottlingException The number of requests made exceeds the limit.
* - AccessDeniedException The account or role doesn't have the right permissions to make the request.
* - ConflictException The parameters provided in the request conflict with existing resources.
* - ValidationException One or more parameters provided in the request is not valid.
* - InternalServerException An unexpected error occurred during the processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - M2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample M2AsyncClient.UpdateEnvironment
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture updateEnvironment(UpdateEnvironmentRequest updateEnvironmentRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(updateEnvironmentRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateEnvironmentRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "m2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateEnvironment");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, UpdateEnvironmentResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("UpdateEnvironment").withProtocolMetadata(protocolMetadata)
.withMarshaller(new UpdateEnvironmentRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(updateEnvironmentRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
@Override
public final M2ServiceClientConfiguration serviceClientConfiguration() {
return new M2ServiceClientConfigurationBuilder(this.clientConfiguration.toBuilder()).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
private > T init(T builder) {
return builder
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(M2Exception::builder)
.protocol(AwsJsonProtocol.REST_JSON)
.protocolVersion("1.1")
.registerModeledException(
ExceptionMetadata.builder().errorCode("ThrottlingException")
.exceptionBuilderSupplier(ThrottlingException::builder).httpStatusCode(429).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ServiceQuotaExceededException")
.exceptionBuilderSupplier(ServiceQuotaExceededException::builder).httpStatusCode(402).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InternalServerException")
.exceptionBuilderSupplier(InternalServerException::builder).httpStatusCode(500).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("AccessDeniedException")
.exceptionBuilderSupplier(AccessDeniedException::builder).httpStatusCode(403).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ConflictException")
.exceptionBuilderSupplier(ConflictException::builder).httpStatusCode(409).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ResourceNotFoundException")
.exceptionBuilderSupplier(ResourceNotFoundException::builder).httpStatusCode(404).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ValidationException")
.exceptionBuilderSupplier(ValidationException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ServiceUnavailableException")
.exceptionBuilderSupplier(ServiceUnavailableException::builder).httpStatusCode(503).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ExecutionTimeoutException")
.exceptionBuilderSupplier(ExecutionTimeoutException::builder).httpStatusCode(504).build());
}
private static List resolveMetricPublishers(SdkClientConfiguration clientConfiguration,
RequestOverrideConfiguration requestOverrideConfiguration) {
List publishers = null;
if (requestOverrideConfiguration != null) {
publishers = requestOverrideConfiguration.metricPublishers();
}
if (publishers == null || publishers.isEmpty()) {
publishers = clientConfiguration.option(SdkClientOption.METRIC_PUBLISHERS);
}
if (publishers == null) {
publishers = Collections.emptyList();
}
return publishers;
}
private void updateRetryStrategyClientConfiguration(SdkClientConfiguration.Builder configuration) {
ClientOverrideConfiguration.Builder builder = configuration.asOverrideConfigurationBuilder();
RetryMode retryMode = builder.retryMode();
if (retryMode != null) {
configuration.option(SdkClientOption.RETRY_STRATEGY, AwsRetryStrategy.forRetryMode(retryMode));
} else {
Consumer> configurator = builder.retryStrategyConfigurator();
if (configurator != null) {
RetryStrategy.Builder, ?> defaultBuilder = AwsRetryStrategy.defaultRetryStrategy().toBuilder();
configurator.accept(defaultBuilder);
configuration.option(SdkClientOption.RETRY_STRATEGY, defaultBuilder.build());
} else {
RetryStrategy retryStrategy = builder.retryStrategy();
if (retryStrategy != null) {
configuration.option(SdkClientOption.RETRY_STRATEGY, retryStrategy);
}
}
}
configuration.option(SdkClientOption.CONFIGURED_RETRY_MODE, null);
configuration.option(SdkClientOption.CONFIGURED_RETRY_STRATEGY, null);
configuration.option(SdkClientOption.CONFIGURED_RETRY_CONFIGURATOR, null);
}
private SdkClientConfiguration updateSdkClientConfiguration(SdkRequest request, SdkClientConfiguration clientConfiguration) {
List plugins = request.overrideConfiguration().map(c -> c.plugins()).orElse(Collections.emptyList());
SdkClientConfiguration.Builder configuration = clientConfiguration.toBuilder();
if (plugins.isEmpty()) {
return configuration.build();
}
M2ServiceClientConfigurationBuilder serviceConfigBuilder = new M2ServiceClientConfigurationBuilder(configuration);
for (SdkPlugin plugin : plugins) {
plugin.configureClient(serviceConfigBuilder);
}
updateRetryStrategyClientConfiguration(configuration);
return configuration.build();
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata) {
return protocolFactory.createErrorResponseHandler(operationMetadata);
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata, Function> exceptionMetadataMapper) {
return protocolFactory.createErrorResponseHandler(operationMetadata, exceptionMetadataMapper);
}
@Override
public void close() {
clientHandler.close();
}
}