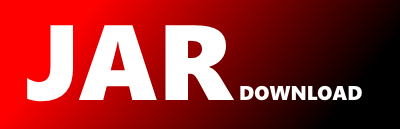
software.amazon.awssdk.services.machinelearning.model.CreateDataSourceFromS3Request Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.machinelearning.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateDataSourceFromS3Request extends MachineLearningRequest implements
ToCopyableBuilder {
private static final SdkField DATA_SOURCE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DataSourceId").getter(getter(CreateDataSourceFromS3Request::dataSourceId))
.setter(setter(Builder::dataSourceId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DataSourceId").build()).build();
private static final SdkField DATA_SOURCE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DataSourceName").getter(getter(CreateDataSourceFromS3Request::dataSourceName))
.setter(setter(Builder::dataSourceName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DataSourceName").build()).build();
private static final SdkField DATA_SPEC_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("DataSpec").getter(getter(CreateDataSourceFromS3Request::dataSpec)).setter(setter(Builder::dataSpec))
.constructor(S3DataSpec::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DataSpec").build()).build();
private static final SdkField COMPUTE_STATISTICS_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("ComputeStatistics").getter(getter(CreateDataSourceFromS3Request::computeStatistics))
.setter(setter(Builder::computeStatistics))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ComputeStatistics").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DATA_SOURCE_ID_FIELD,
DATA_SOURCE_NAME_FIELD, DATA_SPEC_FIELD, COMPUTE_STATISTICS_FIELD));
private final String dataSourceId;
private final String dataSourceName;
private final S3DataSpec dataSpec;
private final Boolean computeStatistics;
private CreateDataSourceFromS3Request(BuilderImpl builder) {
super(builder);
this.dataSourceId = builder.dataSourceId;
this.dataSourceName = builder.dataSourceName;
this.dataSpec = builder.dataSpec;
this.computeStatistics = builder.computeStatistics;
}
/**
*
* A user-supplied identifier that uniquely identifies the DataSource
.
*
*
* @return A user-supplied identifier that uniquely identifies the DataSource
.
*/
public final String dataSourceId() {
return dataSourceId;
}
/**
*
* A user-supplied name or description of the DataSource
.
*
*
* @return A user-supplied name or description of the DataSource
.
*/
public final String dataSourceName() {
return dataSourceName;
}
/**
*
* The data specification of a DataSource
:
*
*
* -
*
* DataLocationS3 - The Amazon S3 location of the observation data.
*
*
* -
*
* DataSchemaLocationS3 - The Amazon S3 location of the DataSchema
.
*
*
* -
*
* DataSchema - A JSON string representing the schema. This is not required if DataSchemaUri
is
* specified.
*
*
* -
*
* DataRearrangement - A JSON string that represents the splitting and rearrangement requirements for the
* Datasource
.
*
*
* Sample - "{\"splitting\":{\"percentBegin\":10,\"percentEnd\":60}}"
*
*
*
*
* @return The data specification of a DataSource
:
*
* -
*
* DataLocationS3 - The Amazon S3 location of the observation data.
*
*
* -
*
* DataSchemaLocationS3 - The Amazon S3 location of the DataSchema
.
*
*
* -
*
* DataSchema - A JSON string representing the schema. This is not required if DataSchemaUri
is
* specified.
*
*
* -
*
* DataRearrangement - A JSON string that represents the splitting and rearrangement requirements for the
* Datasource
.
*
*
* Sample - "{\"splitting\":{\"percentBegin\":10,\"percentEnd\":60}}"
*
*
*/
public final S3DataSpec dataSpec() {
return dataSpec;
}
/**
*
* The compute statistics for a DataSource
. The statistics are generated from the observation data
* referenced by a DataSource
. Amazon ML uses the statistics internally during MLModel
* training. This parameter must be set to true
if the
*
DataSource
needs to be used for MLModel
training.
*
*
* @return The compute statistics for a DataSource
. The statistics are generated from the observation
* data referenced by a DataSource
. Amazon ML uses the statistics internally during
* MLModel
training. This parameter must be set to true
if the
*
DataSource
needs to be used for MLModel
training.
*/
public final Boolean computeStatistics() {
return computeStatistics;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(dataSourceId());
hashCode = 31 * hashCode + Objects.hashCode(dataSourceName());
hashCode = 31 * hashCode + Objects.hashCode(dataSpec());
hashCode = 31 * hashCode + Objects.hashCode(computeStatistics());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateDataSourceFromS3Request)) {
return false;
}
CreateDataSourceFromS3Request other = (CreateDataSourceFromS3Request) obj;
return Objects.equals(dataSourceId(), other.dataSourceId()) && Objects.equals(dataSourceName(), other.dataSourceName())
&& Objects.equals(dataSpec(), other.dataSpec()) && Objects.equals(computeStatistics(), other.computeStatistics());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateDataSourceFromS3Request").add("DataSourceId", dataSourceId())
.add("DataSourceName", dataSourceName()).add("DataSpec", dataSpec())
.add("ComputeStatistics", computeStatistics()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "DataSourceId":
return Optional.ofNullable(clazz.cast(dataSourceId()));
case "DataSourceName":
return Optional.ofNullable(clazz.cast(dataSourceName()));
case "DataSpec":
return Optional.ofNullable(clazz.cast(dataSpec()));
case "ComputeStatistics":
return Optional.ofNullable(clazz.cast(computeStatistics()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function