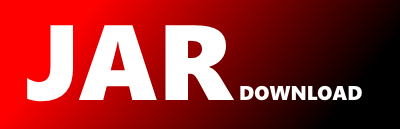
software.amazon.awssdk.services.machinelearning.model.GetDataSourceResponse Maven / Gradle / Ivy
Show all versions of machinelearning Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.machinelearning.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents the output of a GetDataSource
operation and describes a DataSource
.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class GetDataSourceResponse extends MachineLearningResponse implements
ToCopyableBuilder {
private static final SdkField DATA_SOURCE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DataSourceId").getter(getter(GetDataSourceResponse::dataSourceId)).setter(setter(Builder::dataSourceId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DataSourceId").build()).build();
private static final SdkField DATA_LOCATION_S3_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DataLocationS3").getter(getter(GetDataSourceResponse::dataLocationS3))
.setter(setter(Builder::dataLocationS3))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DataLocationS3").build()).build();
private static final SdkField DATA_REARRANGEMENT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DataRearrangement").getter(getter(GetDataSourceResponse::dataRearrangement))
.setter(setter(Builder::dataRearrangement))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DataRearrangement").build()).build();
private static final SdkField CREATED_BY_IAM_USER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CreatedByIamUser").getter(getter(GetDataSourceResponse::createdByIamUser))
.setter(setter(Builder::createdByIamUser))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreatedByIamUser").build()).build();
private static final SdkField CREATED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreatedAt").getter(getter(GetDataSourceResponse::createdAt)).setter(setter(Builder::createdAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreatedAt").build()).build();
private static final SdkField LAST_UPDATED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("LastUpdatedAt").getter(getter(GetDataSourceResponse::lastUpdatedAt))
.setter(setter(Builder::lastUpdatedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastUpdatedAt").build()).build();
private static final SdkField DATA_SIZE_IN_BYTES_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("DataSizeInBytes").getter(getter(GetDataSourceResponse::dataSizeInBytes))
.setter(setter(Builder::dataSizeInBytes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DataSizeInBytes").build()).build();
private static final SdkField NUMBER_OF_FILES_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("NumberOfFiles").getter(getter(GetDataSourceResponse::numberOfFiles))
.setter(setter(Builder::numberOfFiles))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NumberOfFiles").build()).build();
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Name")
.getter(getter(GetDataSourceResponse::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Name").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(GetDataSourceResponse::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField LOG_URI_FIELD = SdkField. builder(MarshallingType.STRING).memberName("LogUri")
.getter(getter(GetDataSourceResponse::logUri)).setter(setter(Builder::logUri))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LogUri").build()).build();
private static final SdkField MESSAGE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Message")
.getter(getter(GetDataSourceResponse::message)).setter(setter(Builder::message))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Message").build()).build();
private static final SdkField REDSHIFT_METADATA_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("RedshiftMetadata")
.getter(getter(GetDataSourceResponse::redshiftMetadata)).setter(setter(Builder::redshiftMetadata))
.constructor(RedshiftMetadata::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RedshiftMetadata").build()).build();
private static final SdkField RDS_METADATA_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("RDSMetadata").getter(getter(GetDataSourceResponse::rdsMetadata)).setter(setter(Builder::rdsMetadata))
.constructor(RDSMetadata::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RDSMetadata").build()).build();
private static final SdkField ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RoleARN").getter(getter(GetDataSourceResponse::roleARN)).setter(setter(Builder::roleARN))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RoleARN").build()).build();
private static final SdkField COMPUTE_STATISTICS_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("ComputeStatistics").getter(getter(GetDataSourceResponse::computeStatistics))
.setter(setter(Builder::computeStatistics))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ComputeStatistics").build()).build();
private static final SdkField COMPUTE_TIME_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("ComputeTime").getter(getter(GetDataSourceResponse::computeTime)).setter(setter(Builder::computeTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ComputeTime").build()).build();
private static final SdkField FINISHED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("FinishedAt").getter(getter(GetDataSourceResponse::finishedAt)).setter(setter(Builder::finishedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FinishedAt").build()).build();
private static final SdkField STARTED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("StartedAt").getter(getter(GetDataSourceResponse::startedAt)).setter(setter(Builder::startedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StartedAt").build()).build();
private static final SdkField DATA_SOURCE_SCHEMA_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DataSourceSchema").getter(getter(GetDataSourceResponse::dataSourceSchema))
.setter(setter(Builder::dataSourceSchema))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DataSourceSchema").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DATA_SOURCE_ID_FIELD,
DATA_LOCATION_S3_FIELD, DATA_REARRANGEMENT_FIELD, CREATED_BY_IAM_USER_FIELD, CREATED_AT_FIELD, LAST_UPDATED_AT_FIELD,
DATA_SIZE_IN_BYTES_FIELD, NUMBER_OF_FILES_FIELD, NAME_FIELD, STATUS_FIELD, LOG_URI_FIELD, MESSAGE_FIELD,
REDSHIFT_METADATA_FIELD, RDS_METADATA_FIELD, ROLE_ARN_FIELD, COMPUTE_STATISTICS_FIELD, COMPUTE_TIME_FIELD,
FINISHED_AT_FIELD, STARTED_AT_FIELD, DATA_SOURCE_SCHEMA_FIELD));
private final String dataSourceId;
private final String dataLocationS3;
private final String dataRearrangement;
private final String createdByIamUser;
private final Instant createdAt;
private final Instant lastUpdatedAt;
private final Long dataSizeInBytes;
private final Long numberOfFiles;
private final String name;
private final String status;
private final String logUri;
private final String message;
private final RedshiftMetadata redshiftMetadata;
private final RDSMetadata rdsMetadata;
private final String roleARN;
private final Boolean computeStatistics;
private final Long computeTime;
private final Instant finishedAt;
private final Instant startedAt;
private final String dataSourceSchema;
private GetDataSourceResponse(BuilderImpl builder) {
super(builder);
this.dataSourceId = builder.dataSourceId;
this.dataLocationS3 = builder.dataLocationS3;
this.dataRearrangement = builder.dataRearrangement;
this.createdByIamUser = builder.createdByIamUser;
this.createdAt = builder.createdAt;
this.lastUpdatedAt = builder.lastUpdatedAt;
this.dataSizeInBytes = builder.dataSizeInBytes;
this.numberOfFiles = builder.numberOfFiles;
this.name = builder.name;
this.status = builder.status;
this.logUri = builder.logUri;
this.message = builder.message;
this.redshiftMetadata = builder.redshiftMetadata;
this.rdsMetadata = builder.rdsMetadata;
this.roleARN = builder.roleARN;
this.computeStatistics = builder.computeStatistics;
this.computeTime = builder.computeTime;
this.finishedAt = builder.finishedAt;
this.startedAt = builder.startedAt;
this.dataSourceSchema = builder.dataSourceSchema;
}
/**
*
* The ID assigned to the DataSource
at creation. This value should be identical to the value of the
* DataSourceId
in the request.
*
*
* @return The ID assigned to the DataSource
at creation. This value should be identical to the value
* of the DataSourceId
in the request.
*/
public final String dataSourceId() {
return dataSourceId;
}
/**
*
* The location of the data file or directory in Amazon Simple Storage Service (Amazon S3).
*
*
* @return The location of the data file or directory in Amazon Simple Storage Service (Amazon S3).
*/
public final String dataLocationS3() {
return dataLocationS3;
}
/**
*
* A JSON string that represents the splitting and rearrangement requirement used when this DataSource
* was created.
*
*
* @return A JSON string that represents the splitting and rearrangement requirement used when this
* DataSource
was created.
*/
public final String dataRearrangement() {
return dataRearrangement;
}
/**
*
* The AWS user account from which the DataSource
was created. The account type can be either an AWS
* root account or an AWS Identity and Access Management (IAM) user account.
*
*
* @return The AWS user account from which the DataSource
was created. The account type can be either
* an AWS root account or an AWS Identity and Access Management (IAM) user account.
*/
public final String createdByIamUser() {
return createdByIamUser;
}
/**
*
* The time that the DataSource
was created. The time is expressed in epoch time.
*
*
* @return The time that the DataSource
was created. The time is expressed in epoch time.
*/
public final Instant createdAt() {
return createdAt;
}
/**
*
* The time of the most recent edit to the DataSource
. The time is expressed in epoch time.
*
*
* @return The time of the most recent edit to the DataSource
. The time is expressed in epoch time.
*/
public final Instant lastUpdatedAt() {
return lastUpdatedAt;
}
/**
*
* The total size of observations in the data files.
*
*
* @return The total size of observations in the data files.
*/
public final Long dataSizeInBytes() {
return dataSizeInBytes;
}
/**
*
* The number of data files referenced by the DataSource
.
*
*
* @return The number of data files referenced by the DataSource
.
*/
public final Long numberOfFiles() {
return numberOfFiles;
}
/**
*
* A user-supplied name or description of the DataSource
.
*
*
* @return A user-supplied name or description of the DataSource
.
*/
public final String name() {
return name;
}
/**
*
* The current status of the DataSource
. This element can have one of the following values:
*
*
* -
*
* PENDING
- Amazon ML submitted a request to create a DataSource
.
*
*
* -
*
* INPROGRESS
- The creation process is underway.
*
*
* -
*
* FAILED
- The request to create a DataSource
did not run to completion. It is not
* usable.
*
*
* -
*
* COMPLETED
- The creation process completed successfully.
*
*
* -
*
* DELETED
- The DataSource
is marked as deleted. It is not usable.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link EntityStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The current status of the DataSource
. This element can have one of the following values:
*
* -
*
* PENDING
- Amazon ML submitted a request to create a DataSource
.
*
*
* -
*
* INPROGRESS
- The creation process is underway.
*
*
* -
*
* FAILED
- The request to create a DataSource
did not run to completion. It is
* not usable.
*
*
* -
*
* COMPLETED
- The creation process completed successfully.
*
*
* -
*
* DELETED
- The DataSource
is marked as deleted. It is not usable.
*
*
* @see EntityStatus
*/
public final EntityStatus status() {
return EntityStatus.fromValue(status);
}
/**
*
* The current status of the DataSource
. This element can have one of the following values:
*
*
* -
*
* PENDING
- Amazon ML submitted a request to create a DataSource
.
*
*
* -
*
* INPROGRESS
- The creation process is underway.
*
*
* -
*
* FAILED
- The request to create a DataSource
did not run to completion. It is not
* usable.
*
*
* -
*
* COMPLETED
- The creation process completed successfully.
*
*
* -
*
* DELETED
- The DataSource
is marked as deleted. It is not usable.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link EntityStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The current status of the DataSource
. This element can have one of the following values:
*
* -
*
* PENDING
- Amazon ML submitted a request to create a DataSource
.
*
*
* -
*
* INPROGRESS
- The creation process is underway.
*
*
* -
*
* FAILED
- The request to create a DataSource
did not run to completion. It is
* not usable.
*
*
* -
*
* COMPLETED
- The creation process completed successfully.
*
*
* -
*
* DELETED
- The DataSource
is marked as deleted. It is not usable.
*
*
* @see EntityStatus
*/
public final String statusAsString() {
return status;
}
/**
*
* A link to the file containing logs of CreateDataSourceFrom*
operations.
*
*
* @return A link to the file containing logs of CreateDataSourceFrom*
operations.
*/
public final String logUri() {
return logUri;
}
/**
*
* The user-supplied description of the most recent details about creating the DataSource
.
*
*
* @return The user-supplied description of the most recent details about creating the DataSource
.
*/
public final String message() {
return message;
}
/**
* Returns the value of the RedshiftMetadata property for this object.
*
* @return The value of the RedshiftMetadata property for this object.
*/
public final RedshiftMetadata redshiftMetadata() {
return redshiftMetadata;
}
/**
* Returns the value of the RDSMetadata property for this object.
*
* @return The value of the RDSMetadata property for this object.
*/
public final RDSMetadata rdsMetadata() {
return rdsMetadata;
}
/**
* Returns the value of the RoleARN property for this object.
*
* @return The value of the RoleARN property for this object.
*/
public final String roleARN() {
return roleARN;
}
/**
*
* The parameter is true
if statistics need to be generated from the observation data.
*
*
* @return The parameter is true
if statistics need to be generated from the observation data.
*/
public final Boolean computeStatistics() {
return computeStatistics;
}
/**
*
* The approximate CPU time in milliseconds that Amazon Machine Learning spent processing the
* DataSource
, normalized and scaled on computation resources. ComputeTime
is only
* available if the DataSource
is in the COMPLETED
state and the
* ComputeStatistics
is set to true.
*
*
* @return The approximate CPU time in milliseconds that Amazon Machine Learning spent processing the
* DataSource
, normalized and scaled on computation resources. ComputeTime
is only
* available if the DataSource
is in the COMPLETED
state and the
* ComputeStatistics
is set to true.
*/
public final Long computeTime() {
return computeTime;
}
/**
*
* The epoch time when Amazon Machine Learning marked the DataSource
as COMPLETED
or
* FAILED
. FinishedAt
is only available when the DataSource
is in the
* COMPLETED
or FAILED
state.
*
*
* @return The epoch time when Amazon Machine Learning marked the DataSource
as COMPLETED
* or FAILED
. FinishedAt
is only available when the DataSource
is in
* the COMPLETED
or FAILED
state.
*/
public final Instant finishedAt() {
return finishedAt;
}
/**
*
* The epoch time when Amazon Machine Learning marked the DataSource
as INPROGRESS
.
* StartedAt
isn't available if the DataSource
is in the PENDING
state.
*
*
* @return The epoch time when Amazon Machine Learning marked the DataSource
as INPROGRESS
* . StartedAt
isn't available if the DataSource
is in the PENDING
* state.
*/
public final Instant startedAt() {
return startedAt;
}
/**
*
* The schema used by all of the data files of this DataSource
.
*
*
* Note: This parameter is provided as part of the verbose format.
*
*
* @return The schema used by all of the data files of this DataSource
.
*
* Note: This parameter is provided as part of the verbose format.
*/
public final String dataSourceSchema() {
return dataSourceSchema;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(dataSourceId());
hashCode = 31 * hashCode + Objects.hashCode(dataLocationS3());
hashCode = 31 * hashCode + Objects.hashCode(dataRearrangement());
hashCode = 31 * hashCode + Objects.hashCode(createdByIamUser());
hashCode = 31 * hashCode + Objects.hashCode(createdAt());
hashCode = 31 * hashCode + Objects.hashCode(lastUpdatedAt());
hashCode = 31 * hashCode + Objects.hashCode(dataSizeInBytes());
hashCode = 31 * hashCode + Objects.hashCode(numberOfFiles());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(logUri());
hashCode = 31 * hashCode + Objects.hashCode(message());
hashCode = 31 * hashCode + Objects.hashCode(redshiftMetadata());
hashCode = 31 * hashCode + Objects.hashCode(rdsMetadata());
hashCode = 31 * hashCode + Objects.hashCode(roleARN());
hashCode = 31 * hashCode + Objects.hashCode(computeStatistics());
hashCode = 31 * hashCode + Objects.hashCode(computeTime());
hashCode = 31 * hashCode + Objects.hashCode(finishedAt());
hashCode = 31 * hashCode + Objects.hashCode(startedAt());
hashCode = 31 * hashCode + Objects.hashCode(dataSourceSchema());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GetDataSourceResponse)) {
return false;
}
GetDataSourceResponse other = (GetDataSourceResponse) obj;
return Objects.equals(dataSourceId(), other.dataSourceId()) && Objects.equals(dataLocationS3(), other.dataLocationS3())
&& Objects.equals(dataRearrangement(), other.dataRearrangement())
&& Objects.equals(createdByIamUser(), other.createdByIamUser()) && Objects.equals(createdAt(), other.createdAt())
&& Objects.equals(lastUpdatedAt(), other.lastUpdatedAt())
&& Objects.equals(dataSizeInBytes(), other.dataSizeInBytes())
&& Objects.equals(numberOfFiles(), other.numberOfFiles()) && Objects.equals(name(), other.name())
&& Objects.equals(statusAsString(), other.statusAsString()) && Objects.equals(logUri(), other.logUri())
&& Objects.equals(message(), other.message()) && Objects.equals(redshiftMetadata(), other.redshiftMetadata())
&& Objects.equals(rdsMetadata(), other.rdsMetadata()) && Objects.equals(roleARN(), other.roleARN())
&& Objects.equals(computeStatistics(), other.computeStatistics())
&& Objects.equals(computeTime(), other.computeTime()) && Objects.equals(finishedAt(), other.finishedAt())
&& Objects.equals(startedAt(), other.startedAt()) && Objects.equals(dataSourceSchema(), other.dataSourceSchema());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("GetDataSourceResponse").add("DataSourceId", dataSourceId())
.add("DataLocationS3", dataLocationS3()).add("DataRearrangement", dataRearrangement())
.add("CreatedByIamUser", createdByIamUser()).add("CreatedAt", createdAt()).add("LastUpdatedAt", lastUpdatedAt())
.add("DataSizeInBytes", dataSizeInBytes()).add("NumberOfFiles", numberOfFiles()).add("Name", name())
.add("Status", statusAsString()).add("LogUri", logUri()).add("Message", message())
.add("RedshiftMetadata", redshiftMetadata()).add("RDSMetadata", rdsMetadata()).add("RoleARN", roleARN())
.add("ComputeStatistics", computeStatistics()).add("ComputeTime", computeTime()).add("FinishedAt", finishedAt())
.add("StartedAt", startedAt()).add("DataSourceSchema", dataSourceSchema()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "DataSourceId":
return Optional.ofNullable(clazz.cast(dataSourceId()));
case "DataLocationS3":
return Optional.ofNullable(clazz.cast(dataLocationS3()));
case "DataRearrangement":
return Optional.ofNullable(clazz.cast(dataRearrangement()));
case "CreatedByIamUser":
return Optional.ofNullable(clazz.cast(createdByIamUser()));
case "CreatedAt":
return Optional.ofNullable(clazz.cast(createdAt()));
case "LastUpdatedAt":
return Optional.ofNullable(clazz.cast(lastUpdatedAt()));
case "DataSizeInBytes":
return Optional.ofNullable(clazz.cast(dataSizeInBytes()));
case "NumberOfFiles":
return Optional.ofNullable(clazz.cast(numberOfFiles()));
case "Name":
return Optional.ofNullable(clazz.cast(name()));
case "Status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "LogUri":
return Optional.ofNullable(clazz.cast(logUri()));
case "Message":
return Optional.ofNullable(clazz.cast(message()));
case "RedshiftMetadata":
return Optional.ofNullable(clazz.cast(redshiftMetadata()));
case "RDSMetadata":
return Optional.ofNullable(clazz.cast(rdsMetadata()));
case "RoleARN":
return Optional.ofNullable(clazz.cast(roleARN()));
case "ComputeStatistics":
return Optional.ofNullable(clazz.cast(computeStatistics()));
case "ComputeTime":
return Optional.ofNullable(clazz.cast(computeTime()));
case "FinishedAt":
return Optional.ofNullable(clazz.cast(finishedAt()));
case "StartedAt":
return Optional.ofNullable(clazz.cast(startedAt()));
case "DataSourceSchema":
return Optional.ofNullable(clazz.cast(dataSourceSchema()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* -
*
* PENDING
- Amazon ML submitted a request to create a DataSource
.
*
*
* -
*
* INPROGRESS
- The creation process is underway.
*
*
* -
*
* FAILED
- The request to create a DataSource
did not run to completion. It is
* not usable.
*
*
* -
*
* COMPLETED
- The creation process completed successfully.
*
*
* -
*
* DELETED
- The DataSource
is marked as deleted. It is not usable.
*
*
* @see EntityStatus
* @return Returns a reference to this object so that method calls can be chained together.
* @see EntityStatus
*/
Builder status(String status);
/**
*
* The current status of the DataSource
. This element can have one of the following values:
*
*
* -
*
* PENDING
- Amazon ML submitted a request to create a DataSource
.
*
*
* -
*
* INPROGRESS
- The creation process is underway.
*
*
* -
*
* FAILED
- The request to create a DataSource
did not run to completion. It is not
* usable.
*
*
* -
*
* COMPLETED
- The creation process completed successfully.
*
*
* -
*
* DELETED
- The DataSource
is marked as deleted. It is not usable.
*
*
*
*
* @param status
* The current status of the DataSource
. This element can have one of the following
* values:
*
* -
*
* PENDING
- Amazon ML submitted a request to create a DataSource
.
*
*
* -
*
* INPROGRESS
- The creation process is underway.
*
*
* -
*
* FAILED
- The request to create a DataSource
did not run to completion. It is
* not usable.
*
*
* -
*
* COMPLETED
- The creation process completed successfully.
*
*
* -
*
* DELETED
- The DataSource
is marked as deleted. It is not usable.
*
*
* @see EntityStatus
* @return Returns a reference to this object so that method calls can be chained together.
* @see EntityStatus
*/
Builder status(EntityStatus status);
/**
*
* A link to the file containing logs of CreateDataSourceFrom*
operations.
*
*
* @param logUri
* A link to the file containing logs of CreateDataSourceFrom*
operations.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder logUri(String logUri);
/**
*
* The user-supplied description of the most recent details about creating the DataSource
.
*
*
* @param message
* The user-supplied description of the most recent details about creating the DataSource
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder message(String message);
/**
* Sets the value of the RedshiftMetadata property for this object.
*
* @param redshiftMetadata
* The new value for the RedshiftMetadata property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder redshiftMetadata(RedshiftMetadata redshiftMetadata);
/**
* Sets the value of the RedshiftMetadata property for this object.
*
* This is a convenience method that creates an instance of the {@link RedshiftMetadata.Builder} avoiding the
* need to create one manually via {@link RedshiftMetadata#builder()}.
*
*
* When the {@link Consumer} completes, {@link RedshiftMetadata.Builder#build()} is called immediately and its
* result is passed to {@link #redshiftMetadata(RedshiftMetadata)}.
*
* @param redshiftMetadata
* a consumer that will call methods on {@link RedshiftMetadata.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #redshiftMetadata(RedshiftMetadata)
*/
default Builder redshiftMetadata(Consumer redshiftMetadata) {
return redshiftMetadata(RedshiftMetadata.builder().applyMutation(redshiftMetadata).build());
}
/**
* Sets the value of the RDSMetadata property for this object.
*
* @param rdsMetadata
* The new value for the RDSMetadata property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder rdsMetadata(RDSMetadata rdsMetadata);
/**
* Sets the value of the RDSMetadata property for this object.
*
* This is a convenience method that creates an instance of the {@link RDSMetadata.Builder} avoiding the need to
* create one manually via {@link RDSMetadata#builder()}.
*
*
* When the {@link Consumer} completes, {@link RDSMetadata.Builder#build()} is called immediately and its result
* is passed to {@link #rdsMetadata(RDSMetadata)}.
*
* @param rdsMetadata
* a consumer that will call methods on {@link RDSMetadata.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #rdsMetadata(RDSMetadata)
*/
default Builder rdsMetadata(Consumer rdsMetadata) {
return rdsMetadata(RDSMetadata.builder().applyMutation(rdsMetadata).build());
}
/**
* Sets the value of the RoleARN property for this object.
*
* @param roleARN
* The new value for the RoleARN property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder roleARN(String roleARN);
/**
*
* The parameter is true
if statistics need to be generated from the observation data.
*
*
* @param computeStatistics
* The parameter is true
if statistics need to be generated from the observation data.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder computeStatistics(Boolean computeStatistics);
/**
*
* The approximate CPU time in milliseconds that Amazon Machine Learning spent processing the
* DataSource
, normalized and scaled on computation resources. ComputeTime
is only
* available if the DataSource
is in the COMPLETED
state and the
* ComputeStatistics
is set to true.
*
*
* @param computeTime
* The approximate CPU time in milliseconds that Amazon Machine Learning spent processing the
* DataSource
, normalized and scaled on computation resources. ComputeTime
is
* only available if the DataSource
is in the COMPLETED
state and the
* ComputeStatistics
is set to true.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder computeTime(Long computeTime);
/**
*
* The epoch time when Amazon Machine Learning marked the DataSource
as COMPLETED
or
* FAILED
. FinishedAt
is only available when the DataSource
is in the
* COMPLETED
or FAILED
state.
*
*
* @param finishedAt
* The epoch time when Amazon Machine Learning marked the DataSource
as
* COMPLETED
or FAILED
. FinishedAt
is only available when the
* DataSource
is in the COMPLETED
or FAILED
state.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder finishedAt(Instant finishedAt);
/**
*
* The epoch time when Amazon Machine Learning marked the DataSource
as INPROGRESS
.
* StartedAt
isn't available if the DataSource
is in the PENDING
state.
*
*
* @param startedAt
* The epoch time when Amazon Machine Learning marked the DataSource
as
* INPROGRESS
. StartedAt
isn't available if the DataSource
is in
* the PENDING
state.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder startedAt(Instant startedAt);
/**
*
* The schema used by all of the data files of this DataSource
.
*
*
* Note: This parameter is provided as part of the verbose format.
*
*
* @param dataSourceSchema
* The schema used by all of the data files of this DataSource
.
*
* Note: This parameter is provided as part of the verbose format.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder dataSourceSchema(String dataSourceSchema);
}
static final class BuilderImpl extends MachineLearningResponse.BuilderImpl implements Builder {
private String dataSourceId;
private String dataLocationS3;
private String dataRearrangement;
private String createdByIamUser;
private Instant createdAt;
private Instant lastUpdatedAt;
private Long dataSizeInBytes;
private Long numberOfFiles;
private String name;
private String status;
private String logUri;
private String message;
private RedshiftMetadata redshiftMetadata;
private RDSMetadata rdsMetadata;
private String roleARN;
private Boolean computeStatistics;
private Long computeTime;
private Instant finishedAt;
private Instant startedAt;
private String dataSourceSchema;
private BuilderImpl() {
}
private BuilderImpl(GetDataSourceResponse model) {
super(model);
dataSourceId(model.dataSourceId);
dataLocationS3(model.dataLocationS3);
dataRearrangement(model.dataRearrangement);
createdByIamUser(model.createdByIamUser);
createdAt(model.createdAt);
lastUpdatedAt(model.lastUpdatedAt);
dataSizeInBytes(model.dataSizeInBytes);
numberOfFiles(model.numberOfFiles);
name(model.name);
status(model.status);
logUri(model.logUri);
message(model.message);
redshiftMetadata(model.redshiftMetadata);
rdsMetadata(model.rdsMetadata);
roleARN(model.roleARN);
computeStatistics(model.computeStatistics);
computeTime(model.computeTime);
finishedAt(model.finishedAt);
startedAt(model.startedAt);
dataSourceSchema(model.dataSourceSchema);
}
public final String getDataSourceId() {
return dataSourceId;
}
public final void setDataSourceId(String dataSourceId) {
this.dataSourceId = dataSourceId;
}
@Override
public final Builder dataSourceId(String dataSourceId) {
this.dataSourceId = dataSourceId;
return this;
}
public final String getDataLocationS3() {
return dataLocationS3;
}
public final void setDataLocationS3(String dataLocationS3) {
this.dataLocationS3 = dataLocationS3;
}
@Override
public final Builder dataLocationS3(String dataLocationS3) {
this.dataLocationS3 = dataLocationS3;
return this;
}
public final String getDataRearrangement() {
return dataRearrangement;
}
public final void setDataRearrangement(String dataRearrangement) {
this.dataRearrangement = dataRearrangement;
}
@Override
public final Builder dataRearrangement(String dataRearrangement) {
this.dataRearrangement = dataRearrangement;
return this;
}
public final String getCreatedByIamUser() {
return createdByIamUser;
}
public final void setCreatedByIamUser(String createdByIamUser) {
this.createdByIamUser = createdByIamUser;
}
@Override
public final Builder createdByIamUser(String createdByIamUser) {
this.createdByIamUser = createdByIamUser;
return this;
}
public final Instant getCreatedAt() {
return createdAt;
}
public final void setCreatedAt(Instant createdAt) {
this.createdAt = createdAt;
}
@Override
public final Builder createdAt(Instant createdAt) {
this.createdAt = createdAt;
return this;
}
public final Instant getLastUpdatedAt() {
return lastUpdatedAt;
}
public final void setLastUpdatedAt(Instant lastUpdatedAt) {
this.lastUpdatedAt = lastUpdatedAt;
}
@Override
public final Builder lastUpdatedAt(Instant lastUpdatedAt) {
this.lastUpdatedAt = lastUpdatedAt;
return this;
}
public final Long getDataSizeInBytes() {
return dataSizeInBytes;
}
public final void setDataSizeInBytes(Long dataSizeInBytes) {
this.dataSizeInBytes = dataSizeInBytes;
}
@Override
public final Builder dataSizeInBytes(Long dataSizeInBytes) {
this.dataSizeInBytes = dataSizeInBytes;
return this;
}
public final Long getNumberOfFiles() {
return numberOfFiles;
}
public final void setNumberOfFiles(Long numberOfFiles) {
this.numberOfFiles = numberOfFiles;
}
@Override
public final Builder numberOfFiles(Long numberOfFiles) {
this.numberOfFiles = numberOfFiles;
return this;
}
public final String getName() {
return name;
}
public final void setName(String name) {
this.name = name;
}
@Override
public final Builder name(String name) {
this.name = name;
return this;
}
public final String getStatus() {
return status;
}
public final void setStatus(String status) {
this.status = status;
}
@Override
public final Builder status(String status) {
this.status = status;
return this;
}
@Override
public final Builder status(EntityStatus status) {
this.status(status == null ? null : status.toString());
return this;
}
public final String getLogUri() {
return logUri;
}
public final void setLogUri(String logUri) {
this.logUri = logUri;
}
@Override
public final Builder logUri(String logUri) {
this.logUri = logUri;
return this;
}
public final String getMessage() {
return message;
}
public final void setMessage(String message) {
this.message = message;
}
@Override
public final Builder message(String message) {
this.message = message;
return this;
}
public final RedshiftMetadata.Builder getRedshiftMetadata() {
return redshiftMetadata != null ? redshiftMetadata.toBuilder() : null;
}
public final void setRedshiftMetadata(RedshiftMetadata.BuilderImpl redshiftMetadata) {
this.redshiftMetadata = redshiftMetadata != null ? redshiftMetadata.build() : null;
}
@Override
public final Builder redshiftMetadata(RedshiftMetadata redshiftMetadata) {
this.redshiftMetadata = redshiftMetadata;
return this;
}
public final RDSMetadata.Builder getRdsMetadata() {
return rdsMetadata != null ? rdsMetadata.toBuilder() : null;
}
public final void setRdsMetadata(RDSMetadata.BuilderImpl rdsMetadata) {
this.rdsMetadata = rdsMetadata != null ? rdsMetadata.build() : null;
}
@Override
public final Builder rdsMetadata(RDSMetadata rdsMetadata) {
this.rdsMetadata = rdsMetadata;
return this;
}
public final String getRoleARN() {
return roleARN;
}
public final void setRoleARN(String roleARN) {
this.roleARN = roleARN;
}
@Override
public final Builder roleARN(String roleARN) {
this.roleARN = roleARN;
return this;
}
public final Boolean getComputeStatistics() {
return computeStatistics;
}
public final void setComputeStatistics(Boolean computeStatistics) {
this.computeStatistics = computeStatistics;
}
@Override
public final Builder computeStatistics(Boolean computeStatistics) {
this.computeStatistics = computeStatistics;
return this;
}
public final Long getComputeTime() {
return computeTime;
}
public final void setComputeTime(Long computeTime) {
this.computeTime = computeTime;
}
@Override
public final Builder computeTime(Long computeTime) {
this.computeTime = computeTime;
return this;
}
public final Instant getFinishedAt() {
return finishedAt;
}
public final void setFinishedAt(Instant finishedAt) {
this.finishedAt = finishedAt;
}
@Override
public final Builder finishedAt(Instant finishedAt) {
this.finishedAt = finishedAt;
return this;
}
public final Instant getStartedAt() {
return startedAt;
}
public final void setStartedAt(Instant startedAt) {
this.startedAt = startedAt;
}
@Override
public final Builder startedAt(Instant startedAt) {
this.startedAt = startedAt;
return this;
}
public final String getDataSourceSchema() {
return dataSourceSchema;
}
public final void setDataSourceSchema(String dataSourceSchema) {
this.dataSourceSchema = dataSourceSchema;
}
@Override
public final Builder dataSourceSchema(String dataSourceSchema) {
this.dataSourceSchema = dataSourceSchema;
return this;
}
@Override
public GetDataSourceResponse build() {
return new GetDataSourceResponse(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}