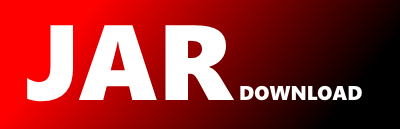
software.amazon.awssdk.services.machinelearning.model.CreateBatchPredictionRequest Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.machinelearning.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateBatchPredictionRequest extends MachineLearningRequest implements
ToCopyableBuilder {
private static final SdkField BATCH_PREDICTION_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("BatchPredictionId").getter(getter(CreateBatchPredictionRequest::batchPredictionId))
.setter(setter(Builder::batchPredictionId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BatchPredictionId").build()).build();
private static final SdkField BATCH_PREDICTION_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("BatchPredictionName").getter(getter(CreateBatchPredictionRequest::batchPredictionName))
.setter(setter(Builder::batchPredictionName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BatchPredictionName").build())
.build();
private static final SdkField ML_MODEL_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MLModelId").getter(getter(CreateBatchPredictionRequest::mlModelId)).setter(setter(Builder::mlModelId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MLModelId").build()).build();
private static final SdkField BATCH_PREDICTION_DATA_SOURCE_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("BatchPredictionDataSourceId")
.getter(getter(CreateBatchPredictionRequest::batchPredictionDataSourceId))
.setter(setter(Builder::batchPredictionDataSourceId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BatchPredictionDataSourceId")
.build()).build();
private static final SdkField OUTPUT_URI_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OutputUri").getter(getter(CreateBatchPredictionRequest::outputUri)).setter(setter(Builder::outputUri))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OutputUri").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(BATCH_PREDICTION_ID_FIELD,
BATCH_PREDICTION_NAME_FIELD, ML_MODEL_ID_FIELD, BATCH_PREDICTION_DATA_SOURCE_ID_FIELD, OUTPUT_URI_FIELD));
private final String batchPredictionId;
private final String batchPredictionName;
private final String mlModelId;
private final String batchPredictionDataSourceId;
private final String outputUri;
private CreateBatchPredictionRequest(BuilderImpl builder) {
super(builder);
this.batchPredictionId = builder.batchPredictionId;
this.batchPredictionName = builder.batchPredictionName;
this.mlModelId = builder.mlModelId;
this.batchPredictionDataSourceId = builder.batchPredictionDataSourceId;
this.outputUri = builder.outputUri;
}
/**
*
* A user-supplied ID that uniquely identifies the BatchPrediction
.
*
*
* @return A user-supplied ID that uniquely identifies the BatchPrediction
.
*/
public final String batchPredictionId() {
return batchPredictionId;
}
/**
*
* A user-supplied name or description of the BatchPrediction
. BatchPredictionName
can
* only use the UTF-8 character set.
*
*
* @return A user-supplied name or description of the BatchPrediction
. BatchPredictionName
* can only use the UTF-8 character set.
*/
public final String batchPredictionName() {
return batchPredictionName;
}
/**
*
* The ID of the MLModel
that will generate predictions for the group of observations.
*
*
* @return The ID of the MLModel
that will generate predictions for the group of observations.
*/
public final String mlModelId() {
return mlModelId;
}
/**
*
* The ID of the DataSource
that points to the group of observations to predict.
*
*
* @return The ID of the DataSource
that points to the group of observations to predict.
*/
public final String batchPredictionDataSourceId() {
return batchPredictionDataSourceId;
}
/**
*
* The location of an Amazon Simple Storage Service (Amazon S3) bucket or directory to store the batch prediction
* results. The following substrings are not allowed in the s3 key
portion of the
* outputURI
field: ':', '//', '/./', '/../'.
*
*
* Amazon ML needs permissions to store and retrieve the logs on your behalf. For information about how to set
* permissions, see the Amazon Machine Learning
* Developer Guide.
*
*
* @return The location of an Amazon Simple Storage Service (Amazon S3) bucket or directory to store the batch
* prediction results. The following substrings are not allowed in the s3 key
portion of the
* outputURI
field: ':', '//', '/./', '/../'.
*
* Amazon ML needs permissions to store and retrieve the logs on your behalf. For information about how to
* set permissions, see the Amazon Machine
* Learning Developer Guide.
*/
public final String outputUri() {
return outputUri;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(batchPredictionId());
hashCode = 31 * hashCode + Objects.hashCode(batchPredictionName());
hashCode = 31 * hashCode + Objects.hashCode(mlModelId());
hashCode = 31 * hashCode + Objects.hashCode(batchPredictionDataSourceId());
hashCode = 31 * hashCode + Objects.hashCode(outputUri());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateBatchPredictionRequest)) {
return false;
}
CreateBatchPredictionRequest other = (CreateBatchPredictionRequest) obj;
return Objects.equals(batchPredictionId(), other.batchPredictionId())
&& Objects.equals(batchPredictionName(), other.batchPredictionName())
&& Objects.equals(mlModelId(), other.mlModelId())
&& Objects.equals(batchPredictionDataSourceId(), other.batchPredictionDataSourceId())
&& Objects.equals(outputUri(), other.outputUri());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateBatchPredictionRequest").add("BatchPredictionId", batchPredictionId())
.add("BatchPredictionName", batchPredictionName()).add("MLModelId", mlModelId())
.add("BatchPredictionDataSourceId", batchPredictionDataSourceId()).add("OutputUri", outputUri()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "BatchPredictionId":
return Optional.ofNullable(clazz.cast(batchPredictionId()));
case "BatchPredictionName":
return Optional.ofNullable(clazz.cast(batchPredictionName()));
case "MLModelId":
return Optional.ofNullable(clazz.cast(mlModelId()));
case "BatchPredictionDataSourceId":
return Optional.ofNullable(clazz.cast(batchPredictionDataSourceId()));
case "OutputUri":
return Optional.ofNullable(clazz.cast(outputUri()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* Amazon ML needs permissions to store and retrieve the logs on your behalf. For information about how
* to set permissions, see the Amazon
* Machine Learning Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder outputUri(String outputUri);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends MachineLearningRequest.BuilderImpl implements Builder {
private String batchPredictionId;
private String batchPredictionName;
private String mlModelId;
private String batchPredictionDataSourceId;
private String outputUri;
private BuilderImpl() {
}
private BuilderImpl(CreateBatchPredictionRequest model) {
super(model);
batchPredictionId(model.batchPredictionId);
batchPredictionName(model.batchPredictionName);
mlModelId(model.mlModelId);
batchPredictionDataSourceId(model.batchPredictionDataSourceId);
outputUri(model.outputUri);
}
public final String getBatchPredictionId() {
return batchPredictionId;
}
public final void setBatchPredictionId(String batchPredictionId) {
this.batchPredictionId = batchPredictionId;
}
@Override
public final Builder batchPredictionId(String batchPredictionId) {
this.batchPredictionId = batchPredictionId;
return this;
}
public final String getBatchPredictionName() {
return batchPredictionName;
}
public final void setBatchPredictionName(String batchPredictionName) {
this.batchPredictionName = batchPredictionName;
}
@Override
public final Builder batchPredictionName(String batchPredictionName) {
this.batchPredictionName = batchPredictionName;
return this;
}
public final String getMlModelId() {
return mlModelId;
}
public final void setMlModelId(String mlModelId) {
this.mlModelId = mlModelId;
}
@Override
public final Builder mlModelId(String mlModelId) {
this.mlModelId = mlModelId;
return this;
}
public final String getBatchPredictionDataSourceId() {
return batchPredictionDataSourceId;
}
public final void setBatchPredictionDataSourceId(String batchPredictionDataSourceId) {
this.batchPredictionDataSourceId = batchPredictionDataSourceId;
}
@Override
public final Builder batchPredictionDataSourceId(String batchPredictionDataSourceId) {
this.batchPredictionDataSourceId = batchPredictionDataSourceId;
return this;
}
public final String getOutputUri() {
return outputUri;
}
public final void setOutputUri(String outputUri) {
this.outputUri = outputUri;
}
@Override
public final Builder outputUri(String outputUri) {
this.outputUri = outputUri;
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public CreateBatchPredictionRequest build() {
return new CreateBatchPredictionRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}