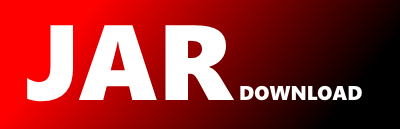
software.amazon.awssdk.services.machinelearning.model.GetEvaluationResponse Maven / Gradle / Ivy
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.machinelearning.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents the output of a GetEvaluation
operation and describes an Evaluation
.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class GetEvaluationResponse extends MachineLearningResponse implements
ToCopyableBuilder {
private static final SdkField EVALUATION_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(GetEvaluationResponse::evaluationId)).setter(setter(Builder::evaluationId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EvaluationId").build()).build();
private static final SdkField ML_MODEL_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(GetEvaluationResponse::mlModelId)).setter(setter(Builder::mlModelId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MLModelId").build()).build();
private static final SdkField EVALUATION_DATA_SOURCE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(GetEvaluationResponse::evaluationDataSourceId)).setter(setter(Builder::evaluationDataSourceId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EvaluationDataSourceId").build())
.build();
private static final SdkField INPUT_DATA_LOCATION_S3_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(GetEvaluationResponse::inputDataLocationS3)).setter(setter(Builder::inputDataLocationS3))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InputDataLocationS3").build())
.build();
private static final SdkField CREATED_BY_IAM_USER_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(GetEvaluationResponse::createdByIamUser)).setter(setter(Builder::createdByIamUser))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreatedByIamUser").build()).build();
private static final SdkField CREATED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(GetEvaluationResponse::createdAt)).setter(setter(Builder::createdAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreatedAt").build()).build();
private static final SdkField LAST_UPDATED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(GetEvaluationResponse::lastUpdatedAt)).setter(setter(Builder::lastUpdatedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastUpdatedAt").build()).build();
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(GetEvaluationResponse::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Name").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(GetEvaluationResponse::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField PERFORMANCE_METRICS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(GetEvaluationResponse::performanceMetrics))
.setter(setter(Builder::performanceMetrics)).constructor(PerformanceMetrics::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PerformanceMetrics").build())
.build();
private static final SdkField LOG_URI_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(GetEvaluationResponse::logUri)).setter(setter(Builder::logUri))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LogUri").build()).build();
private static final SdkField MESSAGE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(GetEvaluationResponse::message)).setter(setter(Builder::message))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Message").build()).build();
private static final SdkField COMPUTE_TIME_FIELD = SdkField. builder(MarshallingType.LONG)
.getter(getter(GetEvaluationResponse::computeTime)).setter(setter(Builder::computeTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ComputeTime").build()).build();
private static final SdkField FINISHED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(GetEvaluationResponse::finishedAt)).setter(setter(Builder::finishedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FinishedAt").build()).build();
private static final SdkField STARTED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(GetEvaluationResponse::startedAt)).setter(setter(Builder::startedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StartedAt").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(EVALUATION_ID_FIELD,
ML_MODEL_ID_FIELD, EVALUATION_DATA_SOURCE_ID_FIELD, INPUT_DATA_LOCATION_S3_FIELD, CREATED_BY_IAM_USER_FIELD,
CREATED_AT_FIELD, LAST_UPDATED_AT_FIELD, NAME_FIELD, STATUS_FIELD, PERFORMANCE_METRICS_FIELD, LOG_URI_FIELD,
MESSAGE_FIELD, COMPUTE_TIME_FIELD, FINISHED_AT_FIELD, STARTED_AT_FIELD));
private final String evaluationId;
private final String mlModelId;
private final String evaluationDataSourceId;
private final String inputDataLocationS3;
private final String createdByIamUser;
private final Instant createdAt;
private final Instant lastUpdatedAt;
private final String name;
private final String status;
private final PerformanceMetrics performanceMetrics;
private final String logUri;
private final String message;
private final Long computeTime;
private final Instant finishedAt;
private final Instant startedAt;
private GetEvaluationResponse(BuilderImpl builder) {
super(builder);
this.evaluationId = builder.evaluationId;
this.mlModelId = builder.mlModelId;
this.evaluationDataSourceId = builder.evaluationDataSourceId;
this.inputDataLocationS3 = builder.inputDataLocationS3;
this.createdByIamUser = builder.createdByIamUser;
this.createdAt = builder.createdAt;
this.lastUpdatedAt = builder.lastUpdatedAt;
this.name = builder.name;
this.status = builder.status;
this.performanceMetrics = builder.performanceMetrics;
this.logUri = builder.logUri;
this.message = builder.message;
this.computeTime = builder.computeTime;
this.finishedAt = builder.finishedAt;
this.startedAt = builder.startedAt;
}
/**
*
* The evaluation ID which is same as the EvaluationId
in the request.
*
*
* @return The evaluation ID which is same as the EvaluationId
in the request.
*/
public String evaluationId() {
return evaluationId;
}
/**
*
* The ID of the MLModel
that was the focus of the evaluation.
*
*
* @return The ID of the MLModel
that was the focus of the evaluation.
*/
public String mlModelId() {
return mlModelId;
}
/**
*
* The DataSource
used for this evaluation.
*
*
* @return The DataSource
used for this evaluation.
*/
public String evaluationDataSourceId() {
return evaluationDataSourceId;
}
/**
*
* The location of the data file or directory in Amazon Simple Storage Service (Amazon S3).
*
*
* @return The location of the data file or directory in Amazon Simple Storage Service (Amazon S3).
*/
public String inputDataLocationS3() {
return inputDataLocationS3;
}
/**
*
* The AWS user account that invoked the evaluation. The account type can be either an AWS root account or an AWS
* Identity and Access Management (IAM) user account.
*
*
* @return The AWS user account that invoked the evaluation. The account type can be either an AWS root account or
* an AWS Identity and Access Management (IAM) user account.
*/
public String createdByIamUser() {
return createdByIamUser;
}
/**
*
* The time that the Evaluation
was created. The time is expressed in epoch time.
*
*
* @return The time that the Evaluation
was created. The time is expressed in epoch time.
*/
public Instant createdAt() {
return createdAt;
}
/**
*
* The time of the most recent edit to the Evaluation
. The time is expressed in epoch time.
*
*
* @return The time of the most recent edit to the Evaluation
. The time is expressed in epoch time.
*/
public Instant lastUpdatedAt() {
return lastUpdatedAt;
}
/**
*
* A user-supplied name or description of the Evaluation
.
*
*
* @return A user-supplied name or description of the Evaluation
.
*/
public String name() {
return name;
}
/**
*
* The status of the evaluation. This element can have one of the following values:
*
*
* -
PENDING
- Amazon Machine Language (Amazon ML) submitted a request to evaluate an
* MLModel
.
* -
INPROGRESS
- The evaluation is underway.
* -
FAILED
- The request to evaluate an MLModel
did not run to completion. It is not
* usable.
* -
COMPLETED
- The evaluation process completed successfully.
* -
DELETED
- The Evaluation
is marked as deleted. It is not usable.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link EntityStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The status of the evaluation. This element can have one of the following values:
*
* -
PENDING
- Amazon Machine Language (Amazon ML) submitted a request to evaluate an
* MLModel
.
* -
INPROGRESS
- The evaluation is underway.
* -
FAILED
- The request to evaluate an MLModel
did not run to completion. It
* is not usable.
* -
COMPLETED
- The evaluation process completed successfully.
* -
DELETED
- The Evaluation
is marked as deleted. It is not usable.
* @see EntityStatus
*/
public EntityStatus status() {
return EntityStatus.fromValue(status);
}
/**
*
* The status of the evaluation. This element can have one of the following values:
*
*
* -
PENDING
- Amazon Machine Language (Amazon ML) submitted a request to evaluate an
* MLModel
.
* -
INPROGRESS
- The evaluation is underway.
* -
FAILED
- The request to evaluate an MLModel
did not run to completion. It is not
* usable.
* -
COMPLETED
- The evaluation process completed successfully.
* -
DELETED
- The Evaluation
is marked as deleted. It is not usable.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link EntityStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The status of the evaluation. This element can have one of the following values:
*
* -
PENDING
- Amazon Machine Language (Amazon ML) submitted a request to evaluate an
* MLModel
.
* -
INPROGRESS
- The evaluation is underway.
* -
FAILED
- The request to evaluate an MLModel
did not run to completion. It
* is not usable.
* -
COMPLETED
- The evaluation process completed successfully.
* -
DELETED
- The Evaluation
is marked as deleted. It is not usable.
* @see EntityStatus
*/
public String statusAsString() {
return status;
}
/**
*
* Measurements of how well the MLModel
performed using observations referenced by the
* DataSource
. One of the following metric is returned based on the type of the MLModel
:
*
*
* -
*
* BinaryAUC: A binary MLModel
uses the Area Under the Curve (AUC) technique to measure performance.
*
*
* -
*
* RegressionRMSE: A regression MLModel
uses the Root Mean Square Error (RMSE) technique to measure
* performance. RMSE measures the difference between predicted and actual values for a single variable.
*
*
* -
*
* MulticlassAvgFScore: A multiclass MLModel
uses the F1 score technique to measure performance.
*
*
*
*
* For more information about performance metrics, please see the Amazon Machine Learning Developer Guide.
*
*
* @return Measurements of how well the MLModel
performed using observations referenced by the
* DataSource
. One of the following metric is returned based on the type of the
* MLModel
:
*
* -
*
* BinaryAUC: A binary MLModel
uses the Area Under the Curve (AUC) technique to measure
* performance.
*
*
* -
*
* RegressionRMSE: A regression MLModel
uses the Root Mean Square Error (RMSE) technique to
* measure performance. RMSE measures the difference between predicted and actual values for a single
* variable.
*
*
* -
*
* MulticlassAvgFScore: A multiclass MLModel
uses the F1 score technique to measure
* performance.
*
*
*
*
* For more information about performance metrics, please see the Amazon Machine Learning Developer Guide.
*/
public PerformanceMetrics performanceMetrics() {
return performanceMetrics;
}
/**
*
* A link to the file that contains logs of the CreateEvaluation
operation.
*
*
* @return A link to the file that contains logs of the CreateEvaluation
operation.
*/
public String logUri() {
return logUri;
}
/**
*
* A description of the most recent details about evaluating the MLModel
.
*
*
* @return A description of the most recent details about evaluating the MLModel
.
*/
public String message() {
return message;
}
/**
*
* The approximate CPU time in milliseconds that Amazon Machine Learning spent processing the
* Evaluation
, normalized and scaled on computation resources. ComputeTime
is only
* available if the Evaluation
is in the COMPLETED
state.
*
*
* @return The approximate CPU time in milliseconds that Amazon Machine Learning spent processing the
* Evaluation
, normalized and scaled on computation resources. ComputeTime
is only
* available if the Evaluation
is in the COMPLETED
state.
*/
public Long computeTime() {
return computeTime;
}
/**
*
* The epoch time when Amazon Machine Learning marked the Evaluation
as COMPLETED
or
* FAILED
. FinishedAt
is only available when the Evaluation
is in the
* COMPLETED
or FAILED
state.
*
*
* @return The epoch time when Amazon Machine Learning marked the Evaluation
as COMPLETED
* or FAILED
. FinishedAt
is only available when the Evaluation
is in
* the COMPLETED
or FAILED
state.
*/
public Instant finishedAt() {
return finishedAt;
}
/**
*
* The epoch time when Amazon Machine Learning marked the Evaluation
as INPROGRESS
.
* StartedAt
isn't available if the Evaluation
is in the PENDING
state.
*
*
* @return The epoch time when Amazon Machine Learning marked the Evaluation
as INPROGRESS
* . StartedAt
isn't available if the Evaluation
is in the PENDING
* state.
*/
public Instant startedAt() {
return startedAt;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(evaluationId());
hashCode = 31 * hashCode + Objects.hashCode(mlModelId());
hashCode = 31 * hashCode + Objects.hashCode(evaluationDataSourceId());
hashCode = 31 * hashCode + Objects.hashCode(inputDataLocationS3());
hashCode = 31 * hashCode + Objects.hashCode(createdByIamUser());
hashCode = 31 * hashCode + Objects.hashCode(createdAt());
hashCode = 31 * hashCode + Objects.hashCode(lastUpdatedAt());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(performanceMetrics());
hashCode = 31 * hashCode + Objects.hashCode(logUri());
hashCode = 31 * hashCode + Objects.hashCode(message());
hashCode = 31 * hashCode + Objects.hashCode(computeTime());
hashCode = 31 * hashCode + Objects.hashCode(finishedAt());
hashCode = 31 * hashCode + Objects.hashCode(startedAt());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GetEvaluationResponse)) {
return false;
}
GetEvaluationResponse other = (GetEvaluationResponse) obj;
return Objects.equals(evaluationId(), other.evaluationId()) && Objects.equals(mlModelId(), other.mlModelId())
&& Objects.equals(evaluationDataSourceId(), other.evaluationDataSourceId())
&& Objects.equals(inputDataLocationS3(), other.inputDataLocationS3())
&& Objects.equals(createdByIamUser(), other.createdByIamUser()) && Objects.equals(createdAt(), other.createdAt())
&& Objects.equals(lastUpdatedAt(), other.lastUpdatedAt()) && Objects.equals(name(), other.name())
&& Objects.equals(statusAsString(), other.statusAsString())
&& Objects.equals(performanceMetrics(), other.performanceMetrics()) && Objects.equals(logUri(), other.logUri())
&& Objects.equals(message(), other.message()) && Objects.equals(computeTime(), other.computeTime())
&& Objects.equals(finishedAt(), other.finishedAt()) && Objects.equals(startedAt(), other.startedAt());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("GetEvaluationResponse").add("EvaluationId", evaluationId()).add("MLModelId", mlModelId())
.add("EvaluationDataSourceId", evaluationDataSourceId()).add("InputDataLocationS3", inputDataLocationS3())
.add("CreatedByIamUser", createdByIamUser()).add("CreatedAt", createdAt()).add("LastUpdatedAt", lastUpdatedAt())
.add("Name", name()).add("Status", statusAsString()).add("PerformanceMetrics", performanceMetrics())
.add("LogUri", logUri()).add("Message", message()).add("ComputeTime", computeTime())
.add("FinishedAt", finishedAt()).add("StartedAt", startedAt()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "EvaluationId":
return Optional.ofNullable(clazz.cast(evaluationId()));
case "MLModelId":
return Optional.ofNullable(clazz.cast(mlModelId()));
case "EvaluationDataSourceId":
return Optional.ofNullable(clazz.cast(evaluationDataSourceId()));
case "InputDataLocationS3":
return Optional.ofNullable(clazz.cast(inputDataLocationS3()));
case "CreatedByIamUser":
return Optional.ofNullable(clazz.cast(createdByIamUser()));
case "CreatedAt":
return Optional.ofNullable(clazz.cast(createdAt()));
case "LastUpdatedAt":
return Optional.ofNullable(clazz.cast(lastUpdatedAt()));
case "Name":
return Optional.ofNullable(clazz.cast(name()));
case "Status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "PerformanceMetrics":
return Optional.ofNullable(clazz.cast(performanceMetrics()));
case "LogUri":
return Optional.ofNullable(clazz.cast(logUri()));
case "Message":
return Optional.ofNullable(clazz.cast(message()));
case "ComputeTime":
return Optional.ofNullable(clazz.cast(computeTime()));
case "FinishedAt":
return Optional.ofNullable(clazz.cast(finishedAt()));
case "StartedAt":
return Optional.ofNullable(clazz.cast(startedAt()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function