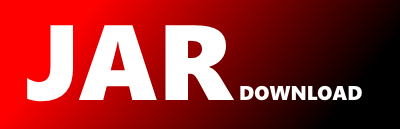
software.amazon.awssdk.services.machinelearning.model.RDSMetadata Maven / Gradle / Ivy
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.machinelearning.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The datasource details that are specific to Amazon RDS.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class RDSMetadata implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField DATABASE_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.getter(getter(RDSMetadata::database)).setter(setter(Builder::database)).constructor(RDSDatabase::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Database").build()).build();
private static final SdkField DATABASE_USER_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RDSMetadata::databaseUserName)).setter(setter(Builder::databaseUserName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DatabaseUserName").build()).build();
private static final SdkField SELECT_SQL_QUERY_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RDSMetadata::selectSqlQuery)).setter(setter(Builder::selectSqlQuery))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SelectSqlQuery").build()).build();
private static final SdkField RESOURCE_ROLE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RDSMetadata::resourceRole)).setter(setter(Builder::resourceRole))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceRole").build()).build();
private static final SdkField SERVICE_ROLE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RDSMetadata::serviceRole)).setter(setter(Builder::serviceRole))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServiceRole").build()).build();
private static final SdkField DATA_PIPELINE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RDSMetadata::dataPipelineId)).setter(setter(Builder::dataPipelineId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DataPipelineId").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DATABASE_FIELD,
DATABASE_USER_NAME_FIELD, SELECT_SQL_QUERY_FIELD, RESOURCE_ROLE_FIELD, SERVICE_ROLE_FIELD, DATA_PIPELINE_ID_FIELD));
private static final long serialVersionUID = 1L;
private final RDSDatabase database;
private final String databaseUserName;
private final String selectSqlQuery;
private final String resourceRole;
private final String serviceRole;
private final String dataPipelineId;
private RDSMetadata(BuilderImpl builder) {
this.database = builder.database;
this.databaseUserName = builder.databaseUserName;
this.selectSqlQuery = builder.selectSqlQuery;
this.resourceRole = builder.resourceRole;
this.serviceRole = builder.serviceRole;
this.dataPipelineId = builder.dataPipelineId;
}
/**
*
* The database details required to connect to an Amazon RDS.
*
*
* @return The database details required to connect to an Amazon RDS.
*/
public RDSDatabase database() {
return database;
}
/**
* Returns the value of the DatabaseUserName property for this object.
*
* @return The value of the DatabaseUserName property for this object.
*/
public String databaseUserName() {
return databaseUserName;
}
/**
*
* The SQL query that is supplied during CreateDataSourceFromRDS. Returns only if Verbose
is
* true in GetDataSourceInput
.
*
*
* @return The SQL query that is supplied during CreateDataSourceFromRDS. Returns only if
* Verbose
is true in GetDataSourceInput
.
*/
public String selectSqlQuery() {
return selectSqlQuery;
}
/**
*
* The role (DataPipelineDefaultResourceRole) assumed by an Amazon EC2 instance to carry out the copy task from
* Amazon RDS to Amazon S3. For more information, see Role templates for
* data pipelines.
*
*
* @return The role (DataPipelineDefaultResourceRole) assumed by an Amazon EC2 instance to carry out the copy task
* from Amazon RDS to Amazon S3. For more information, see Role templates
* for data pipelines.
*/
public String resourceRole() {
return resourceRole;
}
/**
*
* The role (DataPipelineDefaultRole) assumed by the Data Pipeline service to monitor the progress of the copy task
* from Amazon RDS to Amazon S3. For more information, see Role templates for
* data pipelines.
*
*
* @return The role (DataPipelineDefaultRole) assumed by the Data Pipeline service to monitor the progress of the
* copy task from Amazon RDS to Amazon S3. For more information, see Role templates
* for data pipelines.
*/
public String serviceRole() {
return serviceRole;
}
/**
*
* The ID of the Data Pipeline instance that is used to carry to copy data from Amazon RDS to Amazon S3. You can use
* the ID to find details about the instance in the Data Pipeline console.
*
*
* @return The ID of the Data Pipeline instance that is used to carry to copy data from Amazon RDS to Amazon S3. You
* can use the ID to find details about the instance in the Data Pipeline console.
*/
public String dataPipelineId() {
return dataPipelineId;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(database());
hashCode = 31 * hashCode + Objects.hashCode(databaseUserName());
hashCode = 31 * hashCode + Objects.hashCode(selectSqlQuery());
hashCode = 31 * hashCode + Objects.hashCode(resourceRole());
hashCode = 31 * hashCode + Objects.hashCode(serviceRole());
hashCode = 31 * hashCode + Objects.hashCode(dataPipelineId());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof RDSMetadata)) {
return false;
}
RDSMetadata other = (RDSMetadata) obj;
return Objects.equals(database(), other.database()) && Objects.equals(databaseUserName(), other.databaseUserName())
&& Objects.equals(selectSqlQuery(), other.selectSqlQuery())
&& Objects.equals(resourceRole(), other.resourceRole()) && Objects.equals(serviceRole(), other.serviceRole())
&& Objects.equals(dataPipelineId(), other.dataPipelineId());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("RDSMetadata").add("Database", database()).add("DatabaseUserName", databaseUserName())
.add("SelectSqlQuery", selectSqlQuery()).add("ResourceRole", resourceRole()).add("ServiceRole", serviceRole())
.add("DataPipelineId", dataPipelineId()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Database":
return Optional.ofNullable(clazz.cast(database()));
case "DatabaseUserName":
return Optional.ofNullable(clazz.cast(databaseUserName()));
case "SelectSqlQuery":
return Optional.ofNullable(clazz.cast(selectSqlQuery()));
case "ResourceRole":
return Optional.ofNullable(clazz.cast(resourceRole()));
case "ServiceRole":
return Optional.ofNullable(clazz.cast(serviceRole()));
case "DataPipelineId":
return Optional.ofNullable(clazz.cast(dataPipelineId()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function