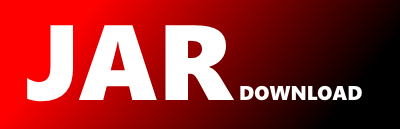
software.amazon.awssdk.services.marketplacemetering.model.RegisterUsageRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of marketplacemetering Show documentation
Show all versions of marketplacemetering Show documentation
The AWS Java SDK for AWS Marketplace Metering Service module holds the client classes that are used for
communicating with AWS Marketplace Metering Service.
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.marketplacemetering.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class RegisterUsageRequest extends MarketplaceMeteringRequest implements
ToCopyableBuilder {
private static final SdkField PRODUCT_CODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ProductCode").getter(getter(RegisterUsageRequest::productCode)).setter(setter(Builder::productCode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ProductCode").build()).build();
private static final SdkField PUBLIC_KEY_VERSION_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("PublicKeyVersion").getter(getter(RegisterUsageRequest::publicKeyVersion))
.setter(setter(Builder::publicKeyVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PublicKeyVersion").build()).build();
private static final SdkField NONCE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Nonce")
.getter(getter(RegisterUsageRequest::nonce)).setter(setter(Builder::nonce))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Nonce").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(PRODUCT_CODE_FIELD,
PUBLIC_KEY_VERSION_FIELD, NONCE_FIELD));
private final String productCode;
private final Integer publicKeyVersion;
private final String nonce;
private RegisterUsageRequest(BuilderImpl builder) {
super(builder);
this.productCode = builder.productCode;
this.publicKeyVersion = builder.publicKeyVersion;
this.nonce = builder.nonce;
}
/**
*
* Product code is used to uniquely identify a product in AWS Marketplace. The product code should be the same as
* the one used during the publishing of a new product.
*
*
* @return Product code is used to uniquely identify a product in AWS Marketplace. The product code should be the
* same as the one used during the publishing of a new product.
*/
public final String productCode() {
return productCode;
}
/**
*
* Public Key Version provided by AWS Marketplace
*
*
* @return Public Key Version provided by AWS Marketplace
*/
public final Integer publicKeyVersion() {
return publicKeyVersion;
}
/**
*
* (Optional) To scope down the registration to a specific running software instance and guard against replay
* attacks.
*
*
* @return (Optional) To scope down the registration to a specific running software instance and guard against
* replay attacks.
*/
public final String nonce() {
return nonce;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(productCode());
hashCode = 31 * hashCode + Objects.hashCode(publicKeyVersion());
hashCode = 31 * hashCode + Objects.hashCode(nonce());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof RegisterUsageRequest)) {
return false;
}
RegisterUsageRequest other = (RegisterUsageRequest) obj;
return Objects.equals(productCode(), other.productCode()) && Objects.equals(publicKeyVersion(), other.publicKeyVersion())
&& Objects.equals(nonce(), other.nonce());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("RegisterUsageRequest").add("ProductCode", productCode())
.add("PublicKeyVersion", publicKeyVersion()).add("Nonce", nonce()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ProductCode":
return Optional.ofNullable(clazz.cast(productCode()));
case "PublicKeyVersion":
return Optional.ofNullable(clazz.cast(publicKeyVersion()));
case "Nonce":
return Optional.ofNullable(clazz.cast(nonce()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy