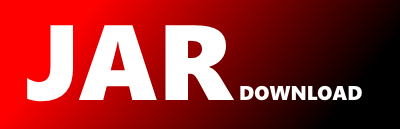
software.amazon.awssdk.services.mediaconvert.model.KantarWatermarkSettings Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.mediaconvert.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
* Use these settings only when you use Kantar watermarking. Specify the values that MediaConvert uses to generate and
* place Kantar watermarks in your output audio. These settings apply to every output in your job. In addition to
* specifying these values, you also need to store your Kantar credentials in AWS Secrets Manager. For more information,
* see https://docs.aws.amazon.com/mediaconvert/latest/ug/kantar-watermarking.html.
*/
@Generated("software.amazon.awssdk:codegen")
public final class KantarWatermarkSettings implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField CHANNEL_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ChannelName").getter(getter(KantarWatermarkSettings::channelName)).setter(setter(Builder::channelName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("channelName").build()).build();
private static final SdkField CONTENT_REFERENCE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ContentReference").getter(getter(KantarWatermarkSettings::contentReference))
.setter(setter(Builder::contentReference))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("contentReference").build()).build();
private static final SdkField CREDENTIALS_SECRET_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CredentialsSecretName").getter(getter(KantarWatermarkSettings::credentialsSecretName))
.setter(setter(Builder::credentialsSecretName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("credentialsSecretName").build())
.build();
private static final SdkField FILE_OFFSET_FIELD = SdkField. builder(MarshallingType.DOUBLE)
.memberName("FileOffset").getter(getter(KantarWatermarkSettings::fileOffset)).setter(setter(Builder::fileOffset))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("fileOffset").build()).build();
private static final SdkField KANTAR_LICENSE_ID_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("KantarLicenseId").getter(getter(KantarWatermarkSettings::kantarLicenseId))
.setter(setter(Builder::kantarLicenseId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("kantarLicenseId").build()).build();
private static final SdkField KANTAR_SERVER_URL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("KantarServerUrl").getter(getter(KantarWatermarkSettings::kantarServerUrl))
.setter(setter(Builder::kantarServerUrl))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("kantarServerUrl").build()).build();
private static final SdkField LOG_DESTINATION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LogDestination").getter(getter(KantarWatermarkSettings::logDestination))
.setter(setter(Builder::logDestination))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("logDestination").build()).build();
private static final SdkField METADATA3_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Metadata3").getter(getter(KantarWatermarkSettings::metadata3)).setter(setter(Builder::metadata3))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("metadata3").build()).build();
private static final SdkField METADATA4_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Metadata4").getter(getter(KantarWatermarkSettings::metadata4)).setter(setter(Builder::metadata4))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("metadata4").build()).build();
private static final SdkField METADATA5_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Metadata5").getter(getter(KantarWatermarkSettings::metadata5)).setter(setter(Builder::metadata5))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("metadata5").build()).build();
private static final SdkField METADATA6_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Metadata6").getter(getter(KantarWatermarkSettings::metadata6)).setter(setter(Builder::metadata6))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("metadata6").build()).build();
private static final SdkField METADATA7_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Metadata7").getter(getter(KantarWatermarkSettings::metadata7)).setter(setter(Builder::metadata7))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("metadata7").build()).build();
private static final SdkField METADATA8_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Metadata8").getter(getter(KantarWatermarkSettings::metadata8)).setter(setter(Builder::metadata8))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("metadata8").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CHANNEL_NAME_FIELD,
CONTENT_REFERENCE_FIELD, CREDENTIALS_SECRET_NAME_FIELD, FILE_OFFSET_FIELD, KANTAR_LICENSE_ID_FIELD,
KANTAR_SERVER_URL_FIELD, LOG_DESTINATION_FIELD, METADATA3_FIELD, METADATA4_FIELD, METADATA5_FIELD, METADATA6_FIELD,
METADATA7_FIELD, METADATA8_FIELD));
private static final long serialVersionUID = 1L;
private final String channelName;
private final String contentReference;
private final String credentialsSecretName;
private final Double fileOffset;
private final Integer kantarLicenseId;
private final String kantarServerUrl;
private final String logDestination;
private final String metadata3;
private final String metadata4;
private final String metadata5;
private final String metadata6;
private final String metadata7;
private final String metadata8;
private KantarWatermarkSettings(BuilderImpl builder) {
this.channelName = builder.channelName;
this.contentReference = builder.contentReference;
this.credentialsSecretName = builder.credentialsSecretName;
this.fileOffset = builder.fileOffset;
this.kantarLicenseId = builder.kantarLicenseId;
this.kantarServerUrl = builder.kantarServerUrl;
this.logDestination = builder.logDestination;
this.metadata3 = builder.metadata3;
this.metadata4 = builder.metadata4;
this.metadata5 = builder.metadata5;
this.metadata6 = builder.metadata6;
this.metadata7 = builder.metadata7;
this.metadata8 = builder.metadata8;
}
/**
* Provide an audio channel name from your Kantar audio license.
*
* @return Provide an audio channel name from your Kantar audio license.
*/
public final String channelName() {
return channelName;
}
/**
* Specify a unique identifier for Kantar to use for this piece of content.
*
* @return Specify a unique identifier for Kantar to use for this piece of content.
*/
public final String contentReference() {
return contentReference;
}
/**
* Provide the name of the AWS Secrets Manager secret where your Kantar credentials are stored. Note that your
* MediaConvert service role must provide access to this secret. For more information, see
* https://docs.aws.amazon.com
* /mediaconvert/latest/ug/granting-permissions-for-mediaconvert-to-access-secrets-manager-secret.html. For
* instructions on creating a secret, see
* https://docs.aws.amazon.com/secretsmanager/latest/userguide/tutorials_basic.html, in the AWS Secrets Manager User
* Guide.
*
* @return Provide the name of the AWS Secrets Manager secret where your Kantar credentials are stored. Note that
* your MediaConvert service role must provide access to this secret. For more information, see
* https://docs.
* aws.amazon.com/mediaconvert/latest/ug/granting-permissions-for-mediaconvert-to-access-secrets
* -manager-secret.html. For instructions on creating a secret, see
* https://docs.aws.amazon.com/secretsmanager/latest/userguide/tutorials_basic.html, in the AWS Secrets
* Manager User Guide.
*/
public final String credentialsSecretName() {
return credentialsSecretName;
}
/**
* Optional. Specify an offset, in whole seconds, from the start of your output and the beginning of the
* watermarking. When you don't specify an offset, Kantar defaults to zero.
*
* @return Optional. Specify an offset, in whole seconds, from the start of your output and the beginning of the
* watermarking. When you don't specify an offset, Kantar defaults to zero.
*/
public final Double fileOffset() {
return fileOffset;
}
/**
* Provide your Kantar license ID number. You should get this number from Kantar.
*
* @return Provide your Kantar license ID number. You should get this number from Kantar.
*/
public final Integer kantarLicenseId() {
return kantarLicenseId;
}
/**
* Provide the HTTPS endpoint to the Kantar server. You should get this endpoint from Kantar.
*
* @return Provide the HTTPS endpoint to the Kantar server. You should get this endpoint from Kantar.
*/
public final String kantarServerUrl() {
return kantarServerUrl;
}
/**
* Optional. Specify the Amazon S3 bucket where you want MediaConvert to store your Kantar watermark XML logs. When
* you don't specify a bucket, MediaConvert doesn't save these logs. Note that your MediaConvert service role must
* provide access to this location. For more information, see
* https://docs.aws.amazon.com/mediaconvert/latest/ug/iam-role.html
*
* @return Optional. Specify the Amazon S3 bucket where you want MediaConvert to store your Kantar watermark XML
* logs. When you don't specify a bucket, MediaConvert doesn't save these logs. Note that your MediaConvert
* service role must provide access to this location. For more information, see
* https://docs.aws.amazon.com/mediaconvert/latest/ug/iam-role.html
*/
public final String logDestination() {
return logDestination;
}
/**
* You can optionally use this field to specify the first timestamp that Kantar embeds during watermarking. Kantar
* suggests that you be very cautious when using this Kantar feature, and that you use it only on channels that are
* managed specifically for use with this feature by your Audience Measurement Operator. For more information about
* this feature, contact Kantar technical support.
*
* @return You can optionally use this field to specify the first timestamp that Kantar embeds during watermarking.
* Kantar suggests that you be very cautious when using this Kantar feature, and that you use it only on
* channels that are managed specifically for use with this feature by your Audience Measurement Operator.
* For more information about this feature, contact Kantar technical support.
*/
public final String metadata3() {
return metadata3;
}
/**
* Additional metadata that MediaConvert sends to Kantar. Maximum length is 50 characters.
*
* @return Additional metadata that MediaConvert sends to Kantar. Maximum length is 50 characters.
*/
public final String metadata4() {
return metadata4;
}
/**
* Additional metadata that MediaConvert sends to Kantar. Maximum length is 50 characters.
*
* @return Additional metadata that MediaConvert sends to Kantar. Maximum length is 50 characters.
*/
public final String metadata5() {
return metadata5;
}
/**
* Additional metadata that MediaConvert sends to Kantar. Maximum length is 50 characters.
*
* @return Additional metadata that MediaConvert sends to Kantar. Maximum length is 50 characters.
*/
public final String metadata6() {
return metadata6;
}
/**
* Additional metadata that MediaConvert sends to Kantar. Maximum length is 50 characters.
*
* @return Additional metadata that MediaConvert sends to Kantar. Maximum length is 50 characters.
*/
public final String metadata7() {
return metadata7;
}
/**
* Additional metadata that MediaConvert sends to Kantar. Maximum length is 50 characters.
*
* @return Additional metadata that MediaConvert sends to Kantar. Maximum length is 50 characters.
*/
public final String metadata8() {
return metadata8;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(channelName());
hashCode = 31 * hashCode + Objects.hashCode(contentReference());
hashCode = 31 * hashCode + Objects.hashCode(credentialsSecretName());
hashCode = 31 * hashCode + Objects.hashCode(fileOffset());
hashCode = 31 * hashCode + Objects.hashCode(kantarLicenseId());
hashCode = 31 * hashCode + Objects.hashCode(kantarServerUrl());
hashCode = 31 * hashCode + Objects.hashCode(logDestination());
hashCode = 31 * hashCode + Objects.hashCode(metadata3());
hashCode = 31 * hashCode + Objects.hashCode(metadata4());
hashCode = 31 * hashCode + Objects.hashCode(metadata5());
hashCode = 31 * hashCode + Objects.hashCode(metadata6());
hashCode = 31 * hashCode + Objects.hashCode(metadata7());
hashCode = 31 * hashCode + Objects.hashCode(metadata8());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof KantarWatermarkSettings)) {
return false;
}
KantarWatermarkSettings other = (KantarWatermarkSettings) obj;
return Objects.equals(channelName(), other.channelName()) && Objects.equals(contentReference(), other.contentReference())
&& Objects.equals(credentialsSecretName(), other.credentialsSecretName())
&& Objects.equals(fileOffset(), other.fileOffset()) && Objects.equals(kantarLicenseId(), other.kantarLicenseId())
&& Objects.equals(kantarServerUrl(), other.kantarServerUrl())
&& Objects.equals(logDestination(), other.logDestination()) && Objects.equals(metadata3(), other.metadata3())
&& Objects.equals(metadata4(), other.metadata4()) && Objects.equals(metadata5(), other.metadata5())
&& Objects.equals(metadata6(), other.metadata6()) && Objects.equals(metadata7(), other.metadata7())
&& Objects.equals(metadata8(), other.metadata8());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("KantarWatermarkSettings").add("ChannelName", channelName())
.add("ContentReference", contentReference()).add("CredentialsSecretName", credentialsSecretName())
.add("FileOffset", fileOffset()).add("KantarLicenseId", kantarLicenseId())
.add("KantarServerUrl", kantarServerUrl()).add("LogDestination", logDestination()).add("Metadata3", metadata3())
.add("Metadata4", metadata4()).add("Metadata5", metadata5()).add("Metadata6", metadata6())
.add("Metadata7", metadata7()).add("Metadata8", metadata8()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ChannelName":
return Optional.ofNullable(clazz.cast(channelName()));
case "ContentReference":
return Optional.ofNullable(clazz.cast(contentReference()));
case "CredentialsSecretName":
return Optional.ofNullable(clazz.cast(credentialsSecretName()));
case "FileOffset":
return Optional.ofNullable(clazz.cast(fileOffset()));
case "KantarLicenseId":
return Optional.ofNullable(clazz.cast(kantarLicenseId()));
case "KantarServerUrl":
return Optional.ofNullable(clazz.cast(kantarServerUrl()));
case "LogDestination":
return Optional.ofNullable(clazz.cast(logDestination()));
case "Metadata3":
return Optional.ofNullable(clazz.cast(metadata3()));
case "Metadata4":
return Optional.ofNullable(clazz.cast(metadata4()));
case "Metadata5":
return Optional.ofNullable(clazz.cast(metadata5()));
case "Metadata6":
return Optional.ofNullable(clazz.cast(metadata6()));
case "Metadata7":
return Optional.ofNullable(clazz.cast(metadata7()));
case "Metadata8":
return Optional.ofNullable(clazz.cast(metadata8()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy