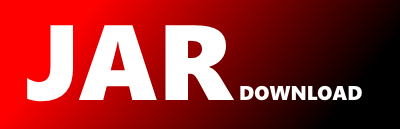
software.amazon.awssdk.services.mediaconvert.model.S3EncryptionSettings Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.mediaconvert.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
* Settings for how your job outputs are encrypted as they are uploaded to Amazon S3.
*/
@Generated("software.amazon.awssdk:codegen")
public final class S3EncryptionSettings implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField ENCRYPTION_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EncryptionType").getter(getter(S3EncryptionSettings::encryptionTypeAsString))
.setter(setter(Builder::encryptionType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("encryptionType").build()).build();
private static final SdkField KMS_ENCRYPTION_CONTEXT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("KmsEncryptionContext").getter(getter(S3EncryptionSettings::kmsEncryptionContext))
.setter(setter(Builder::kmsEncryptionContext))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("kmsEncryptionContext").build())
.build();
private static final SdkField KMS_KEY_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("KmsKeyArn").getter(getter(S3EncryptionSettings::kmsKeyArn)).setter(setter(Builder::kmsKeyArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("kmsKeyArn").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ENCRYPTION_TYPE_FIELD,
KMS_ENCRYPTION_CONTEXT_FIELD, KMS_KEY_ARN_FIELD));
private static final long serialVersionUID = 1L;
private final String encryptionType;
private final String kmsEncryptionContext;
private final String kmsKeyArn;
private S3EncryptionSettings(BuilderImpl builder) {
this.encryptionType = builder.encryptionType;
this.kmsEncryptionContext = builder.kmsEncryptionContext;
this.kmsKeyArn = builder.kmsKeyArn;
}
/**
* Specify how you want your data keys managed. AWS uses data keys to encrypt your content. AWS also encrypts the
* data keys themselves, using a customer master key (CMK), and then stores the encrypted data keys alongside your
* encrypted content. Use this setting to specify which AWS service manages the CMK. For simplest set up, choose
* Amazon S3. If you want your master key to be managed by AWS Key Management Service (KMS), choose AWS KMS. By
* default, when you choose AWS KMS, KMS uses the AWS managed customer master key (CMK) associated with Amazon S3 to
* encrypt your data keys. You can optionally choose to specify a different, customer managed CMK. Do so by
* specifying the Amazon Resource Name (ARN) of the key for the setting KMS ARN.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #encryptionType}
* will return {@link S3ServerSideEncryptionType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #encryptionTypeAsString}.
*
*
* @return Specify how you want your data keys managed. AWS uses data keys to encrypt your content. AWS also
* encrypts the data keys themselves, using a customer master key (CMK), and then stores the encrypted data
* keys alongside your encrypted content. Use this setting to specify which AWS service manages the CMK. For
* simplest set up, choose Amazon S3. If you want your master key to be managed by AWS Key Management
* Service (KMS), choose AWS KMS. By default, when you choose AWS KMS, KMS uses the AWS managed customer
* master key (CMK) associated with Amazon S3 to encrypt your data keys. You can optionally choose to
* specify a different, customer managed CMK. Do so by specifying the Amazon Resource Name (ARN) of the key
* for the setting KMS ARN.
* @see S3ServerSideEncryptionType
*/
public final S3ServerSideEncryptionType encryptionType() {
return S3ServerSideEncryptionType.fromValue(encryptionType);
}
/**
* Specify how you want your data keys managed. AWS uses data keys to encrypt your content. AWS also encrypts the
* data keys themselves, using a customer master key (CMK), and then stores the encrypted data keys alongside your
* encrypted content. Use this setting to specify which AWS service manages the CMK. For simplest set up, choose
* Amazon S3. If you want your master key to be managed by AWS Key Management Service (KMS), choose AWS KMS. By
* default, when you choose AWS KMS, KMS uses the AWS managed customer master key (CMK) associated with Amazon S3 to
* encrypt your data keys. You can optionally choose to specify a different, customer managed CMK. Do so by
* specifying the Amazon Resource Name (ARN) of the key for the setting KMS ARN.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #encryptionType}
* will return {@link S3ServerSideEncryptionType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #encryptionTypeAsString}.
*
*
* @return Specify how you want your data keys managed. AWS uses data keys to encrypt your content. AWS also
* encrypts the data keys themselves, using a customer master key (CMK), and then stores the encrypted data
* keys alongside your encrypted content. Use this setting to specify which AWS service manages the CMK. For
* simplest set up, choose Amazon S3. If you want your master key to be managed by AWS Key Management
* Service (KMS), choose AWS KMS. By default, when you choose AWS KMS, KMS uses the AWS managed customer
* master key (CMK) associated with Amazon S3 to encrypt your data keys. You can optionally choose to
* specify a different, customer managed CMK. Do so by specifying the Amazon Resource Name (ARN) of the key
* for the setting KMS ARN.
* @see S3ServerSideEncryptionType
*/
public final String encryptionTypeAsString() {
return encryptionType;
}
/**
* Optionally, specify the encryption context that you want to use alongside your KMS key. AWS KMS uses this
* encryption context as additional authenticated data (AAD) to support authenticated encryption. This value must be
* a base64-encoded UTF-8 string holding JSON which represents a string-string map. To use this setting, you must
* also set Server-side encryption to AWS KMS. For more information about encryption context, see:
* https://docs.aws.amazon.com/kms/latest/developerguide/concepts.html#encrypt_context.
*
* @return Optionally, specify the encryption context that you want to use alongside your KMS key. AWS KMS uses this
* encryption context as additional authenticated data (AAD) to support authenticated encryption. This value
* must be a base64-encoded UTF-8 string holding JSON which represents a string-string map. To use this
* setting, you must also set Server-side encryption to AWS KMS. For more information about encryption
* context, see: https://docs.aws.amazon.com/kms/latest/developerguide/concepts.html#encrypt_context.
*/
public final String kmsEncryptionContext() {
return kmsEncryptionContext;
}
/**
* Optionally, specify the customer master key (CMK) that you want to use to encrypt the data key that AWS uses to
* encrypt your output content. Enter the Amazon Resource Name (ARN) of the CMK. To use this setting, you must also
* set Server-side encryption to AWS KMS. If you set Server-side encryption to AWS KMS but don't specify a CMK here,
* AWS uses the AWS managed CMK associated with Amazon S3.
*
* @return Optionally, specify the customer master key (CMK) that you want to use to encrypt the data key that AWS
* uses to encrypt your output content. Enter the Amazon Resource Name (ARN) of the CMK. To use this
* setting, you must also set Server-side encryption to AWS KMS. If you set Server-side encryption to AWS
* KMS but don't specify a CMK here, AWS uses the AWS managed CMK associated with Amazon S3.
*/
public final String kmsKeyArn() {
return kmsKeyArn;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(encryptionTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(kmsEncryptionContext());
hashCode = 31 * hashCode + Objects.hashCode(kmsKeyArn());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof S3EncryptionSettings)) {
return false;
}
S3EncryptionSettings other = (S3EncryptionSettings) obj;
return Objects.equals(encryptionTypeAsString(), other.encryptionTypeAsString())
&& Objects.equals(kmsEncryptionContext(), other.kmsEncryptionContext())
&& Objects.equals(kmsKeyArn(), other.kmsKeyArn());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("S3EncryptionSettings").add("EncryptionType", encryptionTypeAsString())
.add("KmsEncryptionContext", kmsEncryptionContext()).add("KmsKeyArn", kmsKeyArn()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "EncryptionType":
return Optional.ofNullable(clazz.cast(encryptionTypeAsString()));
case "KmsEncryptionContext":
return Optional.ofNullable(clazz.cast(kmsEncryptionContext()));
case "KmsKeyArn":
return Optional.ofNullable(clazz.cast(kmsKeyArn()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy