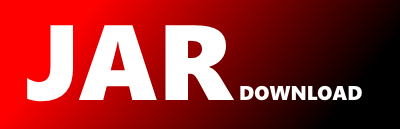
software.amazon.awssdk.services.mediaconvert.model.SpekeKeyProviderCmaf Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.mediaconvert.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
* If your output group type is CMAF, use these settings when doing DRM encryption with a SPEKE-compliant key provider.
* If your output group type is HLS, DASH, or Microsoft Smooth, use the SpekeKeyProvider settings instead.
*/
@Generated("software.amazon.awssdk:codegen")
public final class SpekeKeyProviderCmaf implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField CERTIFICATE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CertificateArn").getter(getter(SpekeKeyProviderCmaf::certificateArn))
.setter(setter(Builder::certificateArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("certificateArn").build()).build();
private static final SdkField> DASH_SIGNALED_SYSTEM_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("DashSignaledSystemIds")
.getter(getter(SpekeKeyProviderCmaf::dashSignaledSystemIds))
.setter(setter(Builder::dashSignaledSystemIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("dashSignaledSystemIds").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField ENCRYPTION_CONTRACT_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("EncryptionContractConfiguration")
.getter(getter(SpekeKeyProviderCmaf::encryptionContractConfiguration))
.setter(setter(Builder::encryptionContractConfiguration))
.constructor(EncryptionContractConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("encryptionContractConfiguration")
.build()).build();
private static final SdkField> HLS_SIGNALED_SYSTEM_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("HlsSignaledSystemIds")
.getter(getter(SpekeKeyProviderCmaf::hlsSignaledSystemIds))
.setter(setter(Builder::hlsSignaledSystemIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("hlsSignaledSystemIds").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField RESOURCE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ResourceId").getter(getter(SpekeKeyProviderCmaf::resourceId)).setter(setter(Builder::resourceId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("resourceId").build()).build();
private static final SdkField URL_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Url")
.getter(getter(SpekeKeyProviderCmaf::url)).setter(setter(Builder::url))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("url").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CERTIFICATE_ARN_FIELD,
DASH_SIGNALED_SYSTEM_IDS_FIELD, ENCRYPTION_CONTRACT_CONFIGURATION_FIELD, HLS_SIGNALED_SYSTEM_IDS_FIELD,
RESOURCE_ID_FIELD, URL_FIELD));
private static final long serialVersionUID = 1L;
private final String certificateArn;
private final List dashSignaledSystemIds;
private final EncryptionContractConfiguration encryptionContractConfiguration;
private final List hlsSignaledSystemIds;
private final String resourceId;
private final String url;
private SpekeKeyProviderCmaf(BuilderImpl builder) {
this.certificateArn = builder.certificateArn;
this.dashSignaledSystemIds = builder.dashSignaledSystemIds;
this.encryptionContractConfiguration = builder.encryptionContractConfiguration;
this.hlsSignaledSystemIds = builder.hlsSignaledSystemIds;
this.resourceId = builder.resourceId;
this.url = builder.url;
}
/**
* If you want your key provider to encrypt the content keys that it provides to MediaConvert, set up a certificate
* with a master key using AWS Certificate Manager. Specify the certificate's Amazon Resource Name (ARN) here.
*
* @return If you want your key provider to encrypt the content keys that it provides to MediaConvert, set up a
* certificate with a master key using AWS Certificate Manager. Specify the certificate's Amazon Resource
* Name (ARN) here.
*/
public final String certificateArn() {
return certificateArn;
}
/**
* For responses, this returns true if the service returned a value for the DashSignaledSystemIds property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasDashSignaledSystemIds() {
return dashSignaledSystemIds != null && !(dashSignaledSystemIds instanceof SdkAutoConstructList);
}
/**
* Specify the DRM system IDs that you want signaled in the DASH manifest that MediaConvert creates as part of this
* CMAF package. The DASH manifest can currently signal up to three system IDs. For more information, see
* https://dashif.org/identifiers/content_protection/.
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasDashSignaledSystemIds} method.
*
*
* @return Specify the DRM system IDs that you want signaled in the DASH manifest that MediaConvert creates as part
* of this CMAF package. The DASH manifest can currently signal up to three system IDs. For more
* information, see https://dashif.org/identifiers/content_protection/.
*/
public final List dashSignaledSystemIds() {
return dashSignaledSystemIds;
}
/**
* Specify the SPEKE version, either v1.0 or v2.0, that MediaConvert uses when encrypting your output. For more
* information, see: https://docs.aws.amazon.com/speke/latest/documentation/speke-api-specification.html To use
* SPEKE v1.0: Leave blank. To use SPEKE v2.0: Specify a SPEKE v2.0 video preset and a SPEKE v2.0 audio preset.
*
* @return Specify the SPEKE version, either v1.0 or v2.0, that MediaConvert uses when encrypting your output. For
* more information, see:
* https://docs.aws.amazon.com/speke/latest/documentation/speke-api-specification.html To use SPEKE v1.0:
* Leave blank. To use SPEKE v2.0: Specify a SPEKE v2.0 video preset and a SPEKE v2.0 audio preset.
*/
public final EncryptionContractConfiguration encryptionContractConfiguration() {
return encryptionContractConfiguration;
}
/**
* For responses, this returns true if the service returned a value for the HlsSignaledSystemIds property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasHlsSignaledSystemIds() {
return hlsSignaledSystemIds != null && !(hlsSignaledSystemIds instanceof SdkAutoConstructList);
}
/**
* Specify the DRM system ID that you want signaled in the HLS manifest that MediaConvert creates as part of this
* CMAF package. The HLS manifest can currently signal only one system ID. For more information, see
* https://dashif.org/identifiers/content_protection/.
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasHlsSignaledSystemIds} method.
*
*
* @return Specify the DRM system ID that you want signaled in the HLS manifest that MediaConvert creates as part of
* this CMAF package. The HLS manifest can currently signal only one system ID. For more information, see
* https://dashif.org/identifiers/content_protection/.
*/
public final List hlsSignaledSystemIds() {
return hlsSignaledSystemIds;
}
/**
* Specify the resource ID that your SPEKE-compliant key provider uses to identify this content.
*
* @return Specify the resource ID that your SPEKE-compliant key provider uses to identify this content.
*/
public final String resourceId() {
return resourceId;
}
/**
* Specify the URL to the key server that your SPEKE-compliant DRM key provider uses to provide keys for encrypting
* your content.
*
* @return Specify the URL to the key server that your SPEKE-compliant DRM key provider uses to provide keys for
* encrypting your content.
*/
public final String url() {
return url;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(certificateArn());
hashCode = 31 * hashCode + Objects.hashCode(hasDashSignaledSystemIds() ? dashSignaledSystemIds() : null);
hashCode = 31 * hashCode + Objects.hashCode(encryptionContractConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(hasHlsSignaledSystemIds() ? hlsSignaledSystemIds() : null);
hashCode = 31 * hashCode + Objects.hashCode(resourceId());
hashCode = 31 * hashCode + Objects.hashCode(url());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof SpekeKeyProviderCmaf)) {
return false;
}
SpekeKeyProviderCmaf other = (SpekeKeyProviderCmaf) obj;
return Objects.equals(certificateArn(), other.certificateArn())
&& hasDashSignaledSystemIds() == other.hasDashSignaledSystemIds()
&& Objects.equals(dashSignaledSystemIds(), other.dashSignaledSystemIds())
&& Objects.equals(encryptionContractConfiguration(), other.encryptionContractConfiguration())
&& hasHlsSignaledSystemIds() == other.hasHlsSignaledSystemIds()
&& Objects.equals(hlsSignaledSystemIds(), other.hlsSignaledSystemIds())
&& Objects.equals(resourceId(), other.resourceId()) && Objects.equals(url(), other.url());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("SpekeKeyProviderCmaf").add("CertificateArn", certificateArn())
.add("DashSignaledSystemIds", hasDashSignaledSystemIds() ? dashSignaledSystemIds() : null)
.add("EncryptionContractConfiguration", encryptionContractConfiguration())
.add("HlsSignaledSystemIds", hasHlsSignaledSystemIds() ? hlsSignaledSystemIds() : null)
.add("ResourceId", resourceId()).add("Url", url()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "CertificateArn":
return Optional.ofNullable(clazz.cast(certificateArn()));
case "DashSignaledSystemIds":
return Optional.ofNullable(clazz.cast(dashSignaledSystemIds()));
case "EncryptionContractConfiguration":
return Optional.ofNullable(clazz.cast(encryptionContractConfiguration()));
case "HlsSignaledSystemIds":
return Optional.ofNullable(clazz.cast(hlsSignaledSystemIds()));
case "ResourceId":
return Optional.ofNullable(clazz.cast(resourceId()));
case "Url":
return Optional.ofNullable(clazz.cast(url()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy