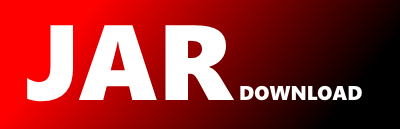
software.amazon.awssdk.services.mediaconvert.model.MotionImageInserter Maven / Gradle / Ivy
Show all versions of mediaconvert Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.mediaconvert.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
* Overlay motion graphics on top of your video. The motion graphics that you specify here appear on all outputs in all
* output groups. For more information, see
* https://docs.aws.amazon.com/mediaconvert/latest/ug/motion-graphic-overlay.html.
*/
@Generated("software.amazon.awssdk:codegen")
public final class MotionImageInserter implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField FRAMERATE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("Framerate")
.getter(getter(MotionImageInserter::framerate)).setter(setter(Builder::framerate))
.constructor(MotionImageInsertionFramerate::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("framerate").build()).build();
private static final SdkField INPUT_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Input")
.getter(getter(MotionImageInserter::input)).setter(setter(Builder::input))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("input").build()).build();
private static final SdkField INSERTION_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InsertionMode").getter(getter(MotionImageInserter::insertionModeAsString))
.setter(setter(Builder::insertionMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("insertionMode").build()).build();
private static final SdkField OFFSET_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("Offset")
.getter(getter(MotionImageInserter::offset)).setter(setter(Builder::offset))
.constructor(MotionImageInsertionOffset::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("offset").build()).build();
private static final SdkField PLAYBACK_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Playback").getter(getter(MotionImageInserter::playbackAsString)).setter(setter(Builder::playback))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("playback").build()).build();
private static final SdkField START_TIME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StartTime").getter(getter(MotionImageInserter::startTime)).setter(setter(Builder::startTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("startTime").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(FRAMERATE_FIELD, INPUT_FIELD,
INSERTION_MODE_FIELD, OFFSET_FIELD, PLAYBACK_FIELD, START_TIME_FIELD));
private static final long serialVersionUID = 1L;
private final MotionImageInsertionFramerate framerate;
private final String input;
private final String insertionMode;
private final MotionImageInsertionOffset offset;
private final String playback;
private final String startTime;
private MotionImageInserter(BuilderImpl builder) {
this.framerate = builder.framerate;
this.input = builder.input;
this.insertionMode = builder.insertionMode;
this.offset = builder.offset;
this.playback = builder.playback;
this.startTime = builder.startTime;
}
/**
* If your motion graphic asset is a .mov file, keep this setting unspecified. If your motion graphic asset is a
* series of .png files, specify the frame rate of the overlay in frames per second, as a fraction. For example,
* specify 24 fps as 24/1. Make sure that the number of images in your series matches the frame rate and your
* intended overlay duration. For example, if you want a 30-second overlay at 30 fps, you should have 900 .png
* images. This overlay frame rate doesn't need to match the frame rate of the underlying video.
*
* @return If your motion graphic asset is a .mov file, keep this setting unspecified. If your motion graphic asset
* is a series of .png files, specify the frame rate of the overlay in frames per second, as a fraction. For
* example, specify 24 fps as 24/1. Make sure that the number of images in your series matches the frame
* rate and your intended overlay duration. For example, if you want a 30-second overlay at 30 fps, you
* should have 900 .png images. This overlay frame rate doesn't need to match the frame rate of the
* underlying video.
*/
public final MotionImageInsertionFramerate framerate() {
return framerate;
}
/**
* Specify the .mov file or series of .png files that you want to overlay on your video. For .png files, provide the
* file name of the first file in the series. Make sure that the names of the .png files end with sequential numbers
* that specify the order that they are played in. For example, overlay_000.png, overlay_001.png, overlay_002.png,
* and so on. The sequence must start at zero, and each image file name must have the same number of digits. Pad
* your initial file names with enough zeros to complete the sequence. For example, if the first image is
* overlay_0.png, there can be only 10 images in the sequence, with the last image being overlay_9.png. But if the
* first image is overlay_00.png, there can be 100 images in the sequence.
*
* @return Specify the .mov file or series of .png files that you want to overlay on your video. For .png files,
* provide the file name of the first file in the series. Make sure that the names of the .png files end
* with sequential numbers that specify the order that they are played in. For example, overlay_000.png,
* overlay_001.png, overlay_002.png, and so on. The sequence must start at zero, and each image file name
* must have the same number of digits. Pad your initial file names with enough zeros to complete the
* sequence. For example, if the first image is overlay_0.png, there can be only 10 images in the sequence,
* with the last image being overlay_9.png. But if the first image is overlay_00.png, there can be 100
* images in the sequence.
*/
public final String input() {
return input;
}
/**
* Choose the type of motion graphic asset that you are providing for your overlay. You can choose either a .mov
* file or a series of .png files.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #insertionMode}
* will return {@link MotionImageInsertionMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #insertionModeAsString}.
*
*
* @return Choose the type of motion graphic asset that you are providing for your overlay. You can choose either a
* .mov file or a series of .png files.
* @see MotionImageInsertionMode
*/
public final MotionImageInsertionMode insertionMode() {
return MotionImageInsertionMode.fromValue(insertionMode);
}
/**
* Choose the type of motion graphic asset that you are providing for your overlay. You can choose either a .mov
* file or a series of .png files.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #insertionMode}
* will return {@link MotionImageInsertionMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #insertionModeAsString}.
*
*
* @return Choose the type of motion graphic asset that you are providing for your overlay. You can choose either a
* .mov file or a series of .png files.
* @see MotionImageInsertionMode
*/
public final String insertionModeAsString() {
return insertionMode;
}
/**
* Use Offset to specify the placement of your motion graphic overlay on the video frame. Specify in pixels, from
* the upper-left corner of the frame. If you don't specify an offset, the service scales your overlay to the full
* size of the frame. Otherwise, the service inserts the overlay at its native resolution and scales the size up or
* down with any video scaling.
*
* @return Use Offset to specify the placement of your motion graphic overlay on the video frame. Specify in pixels,
* from the upper-left corner of the frame. If you don't specify an offset, the service scales your overlay
* to the full size of the frame. Otherwise, the service inserts the overlay at its native resolution and
* scales the size up or down with any video scaling.
*/
public final MotionImageInsertionOffset offset() {
return offset;
}
/**
* Specify whether your motion graphic overlay repeats on a loop or plays only once.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #playback} will
* return {@link MotionImagePlayback#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #playbackAsString}.
*
*
* @return Specify whether your motion graphic overlay repeats on a loop or plays only once.
* @see MotionImagePlayback
*/
public final MotionImagePlayback playback() {
return MotionImagePlayback.fromValue(playback);
}
/**
* Specify whether your motion graphic overlay repeats on a loop or plays only once.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #playback} will
* return {@link MotionImagePlayback#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #playbackAsString}.
*
*
* @return Specify whether your motion graphic overlay repeats on a loop or plays only once.
* @see MotionImagePlayback
*/
public final String playbackAsString() {
return playback;
}
/**
* Specify when the motion overlay begins. Use timecode format (HH:MM:SS:FF or HH:MM:SS;FF). Make sure that the
* timecode you provide here takes into account how you have set up your timecode configuration under both job
* settings and input settings. The simplest way to do that is to set both to start at 0. If you need to set up your
* job to follow timecodes embedded in your source that don't start at zero, make sure that you specify a start time
* that is after the first embedded timecode. For more information, see
* https://docs.aws.amazon.com/mediaconvert/latest/ug/setting-up-timecode.html
*
* @return Specify when the motion overlay begins. Use timecode format (HH:MM:SS:FF or HH:MM:SS;FF). Make sure that
* the timecode you provide here takes into account how you have set up your timecode configuration under
* both job settings and input settings. The simplest way to do that is to set both to start at 0. If you
* need to set up your job to follow timecodes embedded in your source that don't start at zero, make sure
* that you specify a start time that is after the first embedded timecode. For more information, see
* https://docs.aws.amazon.com/mediaconvert/latest/ug/setting-up-timecode.html
*/
public final String startTime() {
return startTime;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(framerate());
hashCode = 31 * hashCode + Objects.hashCode(input());
hashCode = 31 * hashCode + Objects.hashCode(insertionModeAsString());
hashCode = 31 * hashCode + Objects.hashCode(offset());
hashCode = 31 * hashCode + Objects.hashCode(playbackAsString());
hashCode = 31 * hashCode + Objects.hashCode(startTime());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof MotionImageInserter)) {
return false;
}
MotionImageInserter other = (MotionImageInserter) obj;
return Objects.equals(framerate(), other.framerate()) && Objects.equals(input(), other.input())
&& Objects.equals(insertionModeAsString(), other.insertionModeAsString())
&& Objects.equals(offset(), other.offset()) && Objects.equals(playbackAsString(), other.playbackAsString())
&& Objects.equals(startTime(), other.startTime());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("MotionImageInserter").add("Framerate", framerate()).add("Input", input())
.add("InsertionMode", insertionModeAsString()).add("Offset", offset()).add("Playback", playbackAsString())
.add("StartTime", startTime()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Framerate":
return Optional.ofNullable(clazz.cast(framerate()));
case "Input":
return Optional.ofNullable(clazz.cast(input()));
case "InsertionMode":
return Optional.ofNullable(clazz.cast(insertionModeAsString()));
case "Offset":
return Optional.ofNullable(clazz.cast(offset()));
case "Playback":
return Optional.ofNullable(clazz.cast(playbackAsString()));
case "StartTime":
return Optional.ofNullable(clazz.cast(startTime()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function