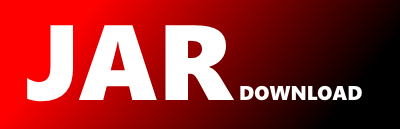
software.amazon.awssdk.services.mediaconvert.model.M3u8Settings Maven / Gradle / Ivy
Show all versions of mediaconvert Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.mediaconvert.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
* These settings relate to the MPEG-2 transport stream (MPEG2-TS) container for the MPEG2-TS segments in your HLS
* outputs.
*/
@Generated("software.amazon.awssdk:codegen")
public final class M3u8Settings implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField AUDIO_DURATION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AudioDuration").getter(getter(M3u8Settings::audioDurationAsString))
.setter(setter(Builder::audioDuration))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("audioDuration").build()).build();
private static final SdkField AUDIO_FRAMES_PER_PES_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("AudioFramesPerPes").getter(getter(M3u8Settings::audioFramesPerPes))
.setter(setter(Builder::audioFramesPerPes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("audioFramesPerPes").build()).build();
private static final SdkField> AUDIO_PIDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("AudioPids")
.getter(getter(M3u8Settings::audioPids))
.setter(setter(Builder::audioPids))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("audioPids").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.INTEGER)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField DATA_PTS_CONTROL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DataPTSControl").getter(getter(M3u8Settings::dataPTSControlAsString))
.setter(setter(Builder::dataPTSControl))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("dataPTSControl").build()).build();
private static final SdkField MAX_PCR_INTERVAL_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("MaxPcrInterval").getter(getter(M3u8Settings::maxPcrInterval)).setter(setter(Builder::maxPcrInterval))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("maxPcrInterval").build()).build();
private static final SdkField NIELSEN_ID3_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NielsenId3").getter(getter(M3u8Settings::nielsenId3AsString)).setter(setter(Builder::nielsenId3))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("nielsenId3").build()).build();
private static final SdkField PAT_INTERVAL_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("PatInterval").getter(getter(M3u8Settings::patInterval)).setter(setter(Builder::patInterval))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("patInterval").build()).build();
private static final SdkField PCR_CONTROL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PcrControl").getter(getter(M3u8Settings::pcrControlAsString)).setter(setter(Builder::pcrControl))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("pcrControl").build()).build();
private static final SdkField PCR_PID_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("PcrPid").getter(getter(M3u8Settings::pcrPid)).setter(setter(Builder::pcrPid))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("pcrPid").build()).build();
private static final SdkField PMT_INTERVAL_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("PmtInterval").getter(getter(M3u8Settings::pmtInterval)).setter(setter(Builder::pmtInterval))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("pmtInterval").build()).build();
private static final SdkField PMT_PID_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("PmtPid").getter(getter(M3u8Settings::pmtPid)).setter(setter(Builder::pmtPid))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("pmtPid").build()).build();
private static final SdkField PRIVATE_METADATA_PID_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("PrivateMetadataPid").getter(getter(M3u8Settings::privateMetadataPid))
.setter(setter(Builder::privateMetadataPid))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("privateMetadataPid").build())
.build();
private static final SdkField PROGRAM_NUMBER_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("ProgramNumber").getter(getter(M3u8Settings::programNumber)).setter(setter(Builder::programNumber))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("programNumber").build()).build();
private static final SdkField PTS_OFFSET_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("PtsOffset").getter(getter(M3u8Settings::ptsOffset)).setter(setter(Builder::ptsOffset))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ptsOffset").build()).build();
private static final SdkField PTS_OFFSET_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PtsOffsetMode").getter(getter(M3u8Settings::ptsOffsetModeAsString))
.setter(setter(Builder::ptsOffsetMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ptsOffsetMode").build()).build();
private static final SdkField SCTE35_PID_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("Scte35Pid").getter(getter(M3u8Settings::scte35Pid)).setter(setter(Builder::scte35Pid))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("scte35Pid").build()).build();
private static final SdkField SCTE35_SOURCE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Scte35Source").getter(getter(M3u8Settings::scte35SourceAsString)).setter(setter(Builder::scte35Source))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("scte35Source").build()).build();
private static final SdkField TIMED_METADATA_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TimedMetadata").getter(getter(M3u8Settings::timedMetadataAsString))
.setter(setter(Builder::timedMetadata))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("timedMetadata").build()).build();
private static final SdkField TIMED_METADATA_PID_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("TimedMetadataPid").getter(getter(M3u8Settings::timedMetadataPid))
.setter(setter(Builder::timedMetadataPid))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("timedMetadataPid").build()).build();
private static final SdkField TRANSPORT_STREAM_ID_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("TransportStreamId").getter(getter(M3u8Settings::transportStreamId))
.setter(setter(Builder::transportStreamId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("transportStreamId").build()).build();
private static final SdkField VIDEO_PID_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("VideoPid").getter(getter(M3u8Settings::videoPid)).setter(setter(Builder::videoPid))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("videoPid").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(AUDIO_DURATION_FIELD,
AUDIO_FRAMES_PER_PES_FIELD, AUDIO_PIDS_FIELD, DATA_PTS_CONTROL_FIELD, MAX_PCR_INTERVAL_FIELD, NIELSEN_ID3_FIELD,
PAT_INTERVAL_FIELD, PCR_CONTROL_FIELD, PCR_PID_FIELD, PMT_INTERVAL_FIELD, PMT_PID_FIELD, PRIVATE_METADATA_PID_FIELD,
PROGRAM_NUMBER_FIELD, PTS_OFFSET_FIELD, PTS_OFFSET_MODE_FIELD, SCTE35_PID_FIELD, SCTE35_SOURCE_FIELD,
TIMED_METADATA_FIELD, TIMED_METADATA_PID_FIELD, TRANSPORT_STREAM_ID_FIELD, VIDEO_PID_FIELD));
private static final long serialVersionUID = 1L;
private final String audioDuration;
private final Integer audioFramesPerPes;
private final List audioPids;
private final String dataPTSControl;
private final Integer maxPcrInterval;
private final String nielsenId3;
private final Integer patInterval;
private final String pcrControl;
private final Integer pcrPid;
private final Integer pmtInterval;
private final Integer pmtPid;
private final Integer privateMetadataPid;
private final Integer programNumber;
private final Integer ptsOffset;
private final String ptsOffsetMode;
private final Integer scte35Pid;
private final String scte35Source;
private final String timedMetadata;
private final Integer timedMetadataPid;
private final Integer transportStreamId;
private final Integer videoPid;
private M3u8Settings(BuilderImpl builder) {
this.audioDuration = builder.audioDuration;
this.audioFramesPerPes = builder.audioFramesPerPes;
this.audioPids = builder.audioPids;
this.dataPTSControl = builder.dataPTSControl;
this.maxPcrInterval = builder.maxPcrInterval;
this.nielsenId3 = builder.nielsenId3;
this.patInterval = builder.patInterval;
this.pcrControl = builder.pcrControl;
this.pcrPid = builder.pcrPid;
this.pmtInterval = builder.pmtInterval;
this.pmtPid = builder.pmtPid;
this.privateMetadataPid = builder.privateMetadataPid;
this.programNumber = builder.programNumber;
this.ptsOffset = builder.ptsOffset;
this.ptsOffsetMode = builder.ptsOffsetMode;
this.scte35Pid = builder.scte35Pid;
this.scte35Source = builder.scte35Source;
this.timedMetadata = builder.timedMetadata;
this.timedMetadataPid = builder.timedMetadataPid;
this.transportStreamId = builder.transportStreamId;
this.videoPid = builder.videoPid;
}
/**
* Specify this setting only when your output will be consumed by a downstream repackaging workflow that is
* sensitive to very small duration differences between video and audio. For this situation, choose Match video
* duration. In all other cases, keep the default value, Default codec duration. When you choose Match video
* duration, MediaConvert pads the output audio streams with silence or trims them to ensure that the total duration
* of each audio stream is at least as long as the total duration of the video stream. After padding or trimming,
* the audio stream duration is no more than one frame longer than the video stream. MediaConvert applies audio
* padding or trimming only to the end of the last segment of the output. For unsegmented outputs, MediaConvert adds
* padding only to the end of the file. When you keep the default value, any minor discrepancies between audio and
* video duration will depend on your output audio codec.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #audioDuration}
* will return {@link M3u8AudioDuration#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #audioDurationAsString}.
*
*
* @return Specify this setting only when your output will be consumed by a downstream repackaging workflow that is
* sensitive to very small duration differences between video and audio. For this situation, choose Match
* video duration. In all other cases, keep the default value, Default codec duration. When you choose Match
* video duration, MediaConvert pads the output audio streams with silence or trims them to ensure that the
* total duration of each audio stream is at least as long as the total duration of the video stream. After
* padding or trimming, the audio stream duration is no more than one frame longer than the video stream.
* MediaConvert applies audio padding or trimming only to the end of the last segment of the output. For
* unsegmented outputs, MediaConvert adds padding only to the end of the file. When you keep the default
* value, any minor discrepancies between audio and video duration will depend on your output audio codec.
* @see M3u8AudioDuration
*/
public final M3u8AudioDuration audioDuration() {
return M3u8AudioDuration.fromValue(audioDuration);
}
/**
* Specify this setting only when your output will be consumed by a downstream repackaging workflow that is
* sensitive to very small duration differences between video and audio. For this situation, choose Match video
* duration. In all other cases, keep the default value, Default codec duration. When you choose Match video
* duration, MediaConvert pads the output audio streams with silence or trims them to ensure that the total duration
* of each audio stream is at least as long as the total duration of the video stream. After padding or trimming,
* the audio stream duration is no more than one frame longer than the video stream. MediaConvert applies audio
* padding or trimming only to the end of the last segment of the output. For unsegmented outputs, MediaConvert adds
* padding only to the end of the file. When you keep the default value, any minor discrepancies between audio and
* video duration will depend on your output audio codec.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #audioDuration}
* will return {@link M3u8AudioDuration#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #audioDurationAsString}.
*
*
* @return Specify this setting only when your output will be consumed by a downstream repackaging workflow that is
* sensitive to very small duration differences between video and audio. For this situation, choose Match
* video duration. In all other cases, keep the default value, Default codec duration. When you choose Match
* video duration, MediaConvert pads the output audio streams with silence or trims them to ensure that the
* total duration of each audio stream is at least as long as the total duration of the video stream. After
* padding or trimming, the audio stream duration is no more than one frame longer than the video stream.
* MediaConvert applies audio padding or trimming only to the end of the last segment of the output. For
* unsegmented outputs, MediaConvert adds padding only to the end of the file. When you keep the default
* value, any minor discrepancies between audio and video duration will depend on your output audio codec.
* @see M3u8AudioDuration
*/
public final String audioDurationAsString() {
return audioDuration;
}
/**
* The number of audio frames to insert for each PES packet.
*
* @return The number of audio frames to insert for each PES packet.
*/
public final Integer audioFramesPerPes() {
return audioFramesPerPes;
}
/**
* For responses, this returns true if the service returned a value for the AudioPids property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasAudioPids() {
return audioPids != null && !(audioPids instanceof SdkAutoConstructList);
}
/**
* Packet Identifier (PID) of the elementary audio stream(s) in the transport stream. Multiple values are accepted,
* and can be entered in ranges and/or by comma separation.
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAudioPids} method.
*
*
* @return Packet Identifier (PID) of the elementary audio stream(s) in the transport stream. Multiple values are
* accepted, and can be entered in ranges and/or by comma separation.
*/
public final List audioPids() {
return audioPids;
}
/**
* If you select ALIGN_TO_VIDEO, MediaConvert writes captions and data packets with Presentation Timestamp (PTS)
* values greater than or equal to the first video packet PTS (MediaConvert drops captions and data packets with
* lesser PTS values). Keep the default value AUTO to allow all PTS values.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #dataPTSControl}
* will return {@link M3u8DataPtsControl#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #dataPTSControlAsString}.
*
*
* @return If you select ALIGN_TO_VIDEO, MediaConvert writes captions and data packets with Presentation Timestamp
* (PTS) values greater than or equal to the first video packet PTS (MediaConvert drops captions and data
* packets with lesser PTS values). Keep the default value AUTO to allow all PTS values.
* @see M3u8DataPtsControl
*/
public final M3u8DataPtsControl dataPTSControl() {
return M3u8DataPtsControl.fromValue(dataPTSControl);
}
/**
* If you select ALIGN_TO_VIDEO, MediaConvert writes captions and data packets with Presentation Timestamp (PTS)
* values greater than or equal to the first video packet PTS (MediaConvert drops captions and data packets with
* lesser PTS values). Keep the default value AUTO to allow all PTS values.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #dataPTSControl}
* will return {@link M3u8DataPtsControl#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #dataPTSControlAsString}.
*
*
* @return If you select ALIGN_TO_VIDEO, MediaConvert writes captions and data packets with Presentation Timestamp
* (PTS) values greater than or equal to the first video packet PTS (MediaConvert drops captions and data
* packets with lesser PTS values). Keep the default value AUTO to allow all PTS values.
* @see M3u8DataPtsControl
*/
public final String dataPTSControlAsString() {
return dataPTSControl;
}
/**
* Specify the maximum time, in milliseconds, between Program Clock References (PCRs) inserted into the transport
* stream.
*
* @return Specify the maximum time, in milliseconds, between Program Clock References (PCRs) inserted into the
* transport stream.
*/
public final Integer maxPcrInterval() {
return maxPcrInterval;
}
/**
* If INSERT, Nielsen inaudible tones for media tracking will be detected in the input audio and an equivalent ID3
* tag will be inserted in the output.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #nielsenId3} will
* return {@link M3u8NielsenId3#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #nielsenId3AsString}.
*
*
* @return If INSERT, Nielsen inaudible tones for media tracking will be detected in the input audio and an
* equivalent ID3 tag will be inserted in the output.
* @see M3u8NielsenId3
*/
public final M3u8NielsenId3 nielsenId3() {
return M3u8NielsenId3.fromValue(nielsenId3);
}
/**
* If INSERT, Nielsen inaudible tones for media tracking will be detected in the input audio and an equivalent ID3
* tag will be inserted in the output.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #nielsenId3} will
* return {@link M3u8NielsenId3#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #nielsenId3AsString}.
*
*
* @return If INSERT, Nielsen inaudible tones for media tracking will be detected in the input audio and an
* equivalent ID3 tag will be inserted in the output.
* @see M3u8NielsenId3
*/
public final String nielsenId3AsString() {
return nielsenId3;
}
/**
* The number of milliseconds between instances of this table in the output transport stream.
*
* @return The number of milliseconds between instances of this table in the output transport stream.
*/
public final Integer patInterval() {
return patInterval;
}
/**
* When set to PCR_EVERY_PES_PACKET a Program Clock Reference value is inserted for every Packetized Elementary
* Stream (PES) header. This parameter is effective only when the PCR PID is the same as the video or audio
* elementary stream.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #pcrControl} will
* return {@link M3u8PcrControl#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #pcrControlAsString}.
*
*
* @return When set to PCR_EVERY_PES_PACKET a Program Clock Reference value is inserted for every Packetized
* Elementary Stream (PES) header. This parameter is effective only when the PCR PID is the same as the
* video or audio elementary stream.
* @see M3u8PcrControl
*/
public final M3u8PcrControl pcrControl() {
return M3u8PcrControl.fromValue(pcrControl);
}
/**
* When set to PCR_EVERY_PES_PACKET a Program Clock Reference value is inserted for every Packetized Elementary
* Stream (PES) header. This parameter is effective only when the PCR PID is the same as the video or audio
* elementary stream.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #pcrControl} will
* return {@link M3u8PcrControl#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #pcrControlAsString}.
*
*
* @return When set to PCR_EVERY_PES_PACKET a Program Clock Reference value is inserted for every Packetized
* Elementary Stream (PES) header. This parameter is effective only when the PCR PID is the same as the
* video or audio elementary stream.
* @see M3u8PcrControl
*/
public final String pcrControlAsString() {
return pcrControl;
}
/**
* Packet Identifier (PID) of the Program Clock Reference (PCR) in the transport stream. When no value is given, the
* encoder will assign the same value as the Video PID.
*
* @return Packet Identifier (PID) of the Program Clock Reference (PCR) in the transport stream. When no value is
* given, the encoder will assign the same value as the Video PID.
*/
public final Integer pcrPid() {
return pcrPid;
}
/**
* The number of milliseconds between instances of this table in the output transport stream.
*
* @return The number of milliseconds between instances of this table in the output transport stream.
*/
public final Integer pmtInterval() {
return pmtInterval;
}
/**
* Packet Identifier (PID) for the Program Map Table (PMT) in the transport stream.
*
* @return Packet Identifier (PID) for the Program Map Table (PMT) in the transport stream.
*/
public final Integer pmtPid() {
return pmtPid;
}
/**
* Packet Identifier (PID) of the private metadata stream in the transport stream.
*
* @return Packet Identifier (PID) of the private metadata stream in the transport stream.
*/
public final Integer privateMetadataPid() {
return privateMetadataPid;
}
/**
* The value of the program number field in the Program Map Table.
*
* @return The value of the program number field in the Program Map Table.
*/
public final Integer programNumber() {
return programNumber;
}
/**
* Manually specify the initial PTS offset, in seconds, when you set PTS offset to Seconds. Enter an integer from 0
* to 3600. Leave blank to keep the default value 2.
*
* @return Manually specify the initial PTS offset, in seconds, when you set PTS offset to Seconds. Enter an integer
* from 0 to 3600. Leave blank to keep the default value 2.
*/
public final Integer ptsOffset() {
return ptsOffset;
}
/**
* Specify the initial presentation timestamp (PTS) offset for your transport stream output. To let MediaConvert
* automatically determine the initial PTS offset: Keep the default value, Auto. We recommend that you choose Auto
* for the widest player compatibility. The initial PTS will be at least two seconds and vary depending on your
* output's bitrate, HRD buffer size and HRD buffer initial fill percentage. To manually specify an initial PTS
* offset: Choose Seconds. Then specify the number of seconds with PTS offset.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #ptsOffsetMode}
* will return {@link TsPtsOffset#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #ptsOffsetModeAsString}.
*
*
* @return Specify the initial presentation timestamp (PTS) offset for your transport stream output. To let
* MediaConvert automatically determine the initial PTS offset: Keep the default value, Auto. We recommend
* that you choose Auto for the widest player compatibility. The initial PTS will be at least two seconds
* and vary depending on your output's bitrate, HRD buffer size and HRD buffer initial fill percentage. To
* manually specify an initial PTS offset: Choose Seconds. Then specify the number of seconds with PTS
* offset.
* @see TsPtsOffset
*/
public final TsPtsOffset ptsOffsetMode() {
return TsPtsOffset.fromValue(ptsOffsetMode);
}
/**
* Specify the initial presentation timestamp (PTS) offset for your transport stream output. To let MediaConvert
* automatically determine the initial PTS offset: Keep the default value, Auto. We recommend that you choose Auto
* for the widest player compatibility. The initial PTS will be at least two seconds and vary depending on your
* output's bitrate, HRD buffer size and HRD buffer initial fill percentage. To manually specify an initial PTS
* offset: Choose Seconds. Then specify the number of seconds with PTS offset.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #ptsOffsetMode}
* will return {@link TsPtsOffset#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #ptsOffsetModeAsString}.
*
*
* @return Specify the initial presentation timestamp (PTS) offset for your transport stream output. To let
* MediaConvert automatically determine the initial PTS offset: Keep the default value, Auto. We recommend
* that you choose Auto for the widest player compatibility. The initial PTS will be at least two seconds
* and vary depending on your output's bitrate, HRD buffer size and HRD buffer initial fill percentage. To
* manually specify an initial PTS offset: Choose Seconds. Then specify the number of seconds with PTS
* offset.
* @see TsPtsOffset
*/
public final String ptsOffsetModeAsString() {
return ptsOffsetMode;
}
/**
* Packet Identifier (PID) of the SCTE-35 stream in the transport stream.
*
* @return Packet Identifier (PID) of the SCTE-35 stream in the transport stream.
*/
public final Integer scte35Pid() {
return scte35Pid;
}
/**
* For SCTE-35 markers from your input-- Choose Passthrough if you want SCTE-35 markers that appear in your input to
* also appear in this output. Choose None if you don't want SCTE-35 markers in this output. For SCTE-35 markers
* from an ESAM XML document-- Choose None if you don't want manifest conditioning. Choose Passthrough and choose Ad
* markers if you do want manifest conditioning. In both cases, also provide the ESAM XML as a string in the setting
* Signal processing notification XML.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #scte35Source} will
* return {@link M3u8Scte35Source#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #scte35SourceAsString}.
*
*
* @return For SCTE-35 markers from your input-- Choose Passthrough if you want SCTE-35 markers that appear in your
* input to also appear in this output. Choose None if you don't want SCTE-35 markers in this output. For
* SCTE-35 markers from an ESAM XML document-- Choose None if you don't want manifest conditioning. Choose
* Passthrough and choose Ad markers if you do want manifest conditioning. In both cases, also provide the
* ESAM XML as a string in the setting Signal processing notification XML.
* @see M3u8Scte35Source
*/
public final M3u8Scte35Source scte35Source() {
return M3u8Scte35Source.fromValue(scte35Source);
}
/**
* For SCTE-35 markers from your input-- Choose Passthrough if you want SCTE-35 markers that appear in your input to
* also appear in this output. Choose None if you don't want SCTE-35 markers in this output. For SCTE-35 markers
* from an ESAM XML document-- Choose None if you don't want manifest conditioning. Choose Passthrough and choose Ad
* markers if you do want manifest conditioning. In both cases, also provide the ESAM XML as a string in the setting
* Signal processing notification XML.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #scte35Source} will
* return {@link M3u8Scte35Source#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #scte35SourceAsString}.
*
*
* @return For SCTE-35 markers from your input-- Choose Passthrough if you want SCTE-35 markers that appear in your
* input to also appear in this output. Choose None if you don't want SCTE-35 markers in this output. For
* SCTE-35 markers from an ESAM XML document-- Choose None if you don't want manifest conditioning. Choose
* Passthrough and choose Ad markers if you do want manifest conditioning. In both cases, also provide the
* ESAM XML as a string in the setting Signal processing notification XML.
* @see M3u8Scte35Source
*/
public final String scte35SourceAsString() {
return scte35Source;
}
/**
* Set ID3 metadata to Passthrough to include ID3 metadata in this output. This includes ID3 metadata from the
* following features: ID3 timestamp period, and Custom ID3 metadata inserter. To exclude this ID3 metadata in this
* output: set ID3 metadata to None or leave blank.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #timedMetadata}
* will return {@link TimedMetadata#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #timedMetadataAsString}.
*
*
* @return Set ID3 metadata to Passthrough to include ID3 metadata in this output. This includes ID3 metadata from
* the following features: ID3 timestamp period, and Custom ID3 metadata inserter. To exclude this ID3
* metadata in this output: set ID3 metadata to None or leave blank.
* @see TimedMetadata
*/
public final TimedMetadata timedMetadata() {
return TimedMetadata.fromValue(timedMetadata);
}
/**
* Set ID3 metadata to Passthrough to include ID3 metadata in this output. This includes ID3 metadata from the
* following features: ID3 timestamp period, and Custom ID3 metadata inserter. To exclude this ID3 metadata in this
* output: set ID3 metadata to None or leave blank.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #timedMetadata}
* will return {@link TimedMetadata#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #timedMetadataAsString}.
*
*
* @return Set ID3 metadata to Passthrough to include ID3 metadata in this output. This includes ID3 metadata from
* the following features: ID3 timestamp period, and Custom ID3 metadata inserter. To exclude this ID3
* metadata in this output: set ID3 metadata to None or leave blank.
* @see TimedMetadata
*/
public final String timedMetadataAsString() {
return timedMetadata;
}
/**
* Packet Identifier (PID) of the ID3 metadata stream in the transport stream.
*
* @return Packet Identifier (PID) of the ID3 metadata stream in the transport stream.
*/
public final Integer timedMetadataPid() {
return timedMetadataPid;
}
/**
* The value of the transport stream ID field in the Program Map Table.
*
* @return The value of the transport stream ID field in the Program Map Table.
*/
public final Integer transportStreamId() {
return transportStreamId;
}
/**
* Packet Identifier (PID) of the elementary video stream in the transport stream.
*
* @return Packet Identifier (PID) of the elementary video stream in the transport stream.
*/
public final Integer videoPid() {
return videoPid;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(audioDurationAsString());
hashCode = 31 * hashCode + Objects.hashCode(audioFramesPerPes());
hashCode = 31 * hashCode + Objects.hashCode(hasAudioPids() ? audioPids() : null);
hashCode = 31 * hashCode + Objects.hashCode(dataPTSControlAsString());
hashCode = 31 * hashCode + Objects.hashCode(maxPcrInterval());
hashCode = 31 * hashCode + Objects.hashCode(nielsenId3AsString());
hashCode = 31 * hashCode + Objects.hashCode(patInterval());
hashCode = 31 * hashCode + Objects.hashCode(pcrControlAsString());
hashCode = 31 * hashCode + Objects.hashCode(pcrPid());
hashCode = 31 * hashCode + Objects.hashCode(pmtInterval());
hashCode = 31 * hashCode + Objects.hashCode(pmtPid());
hashCode = 31 * hashCode + Objects.hashCode(privateMetadataPid());
hashCode = 31 * hashCode + Objects.hashCode(programNumber());
hashCode = 31 * hashCode + Objects.hashCode(ptsOffset());
hashCode = 31 * hashCode + Objects.hashCode(ptsOffsetModeAsString());
hashCode = 31 * hashCode + Objects.hashCode(scte35Pid());
hashCode = 31 * hashCode + Objects.hashCode(scte35SourceAsString());
hashCode = 31 * hashCode + Objects.hashCode(timedMetadataAsString());
hashCode = 31 * hashCode + Objects.hashCode(timedMetadataPid());
hashCode = 31 * hashCode + Objects.hashCode(transportStreamId());
hashCode = 31 * hashCode + Objects.hashCode(videoPid());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof M3u8Settings)) {
return false;
}
M3u8Settings other = (M3u8Settings) obj;
return Objects.equals(audioDurationAsString(), other.audioDurationAsString())
&& Objects.equals(audioFramesPerPes(), other.audioFramesPerPes()) && hasAudioPids() == other.hasAudioPids()
&& Objects.equals(audioPids(), other.audioPids())
&& Objects.equals(dataPTSControlAsString(), other.dataPTSControlAsString())
&& Objects.equals(maxPcrInterval(), other.maxPcrInterval())
&& Objects.equals(nielsenId3AsString(), other.nielsenId3AsString())
&& Objects.equals(patInterval(), other.patInterval())
&& Objects.equals(pcrControlAsString(), other.pcrControlAsString()) && Objects.equals(pcrPid(), other.pcrPid())
&& Objects.equals(pmtInterval(), other.pmtInterval()) && Objects.equals(pmtPid(), other.pmtPid())
&& Objects.equals(privateMetadataPid(), other.privateMetadataPid())
&& Objects.equals(programNumber(), other.programNumber()) && Objects.equals(ptsOffset(), other.ptsOffset())
&& Objects.equals(ptsOffsetModeAsString(), other.ptsOffsetModeAsString())
&& Objects.equals(scte35Pid(), other.scte35Pid())
&& Objects.equals(scte35SourceAsString(), other.scte35SourceAsString())
&& Objects.equals(timedMetadataAsString(), other.timedMetadataAsString())
&& Objects.equals(timedMetadataPid(), other.timedMetadataPid())
&& Objects.equals(transportStreamId(), other.transportStreamId()) && Objects.equals(videoPid(), other.videoPid());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("M3u8Settings").add("AudioDuration", audioDurationAsString())
.add("AudioFramesPerPes", audioFramesPerPes()).add("AudioPids", hasAudioPids() ? audioPids() : null)
.add("DataPTSControl", dataPTSControlAsString()).add("MaxPcrInterval", maxPcrInterval())
.add("NielsenId3", nielsenId3AsString()).add("PatInterval", patInterval())
.add("PcrControl", pcrControlAsString()).add("PcrPid", pcrPid()).add("PmtInterval", pmtInterval())
.add("PmtPid", pmtPid()).add("PrivateMetadataPid", privateMetadataPid()).add("ProgramNumber", programNumber())
.add("PtsOffset", ptsOffset()).add("PtsOffsetMode", ptsOffsetModeAsString()).add("Scte35Pid", scte35Pid())
.add("Scte35Source", scte35SourceAsString()).add("TimedMetadata", timedMetadataAsString())
.add("TimedMetadataPid", timedMetadataPid()).add("TransportStreamId", transportStreamId())
.add("VideoPid", videoPid()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AudioDuration":
return Optional.ofNullable(clazz.cast(audioDurationAsString()));
case "AudioFramesPerPes":
return Optional.ofNullable(clazz.cast(audioFramesPerPes()));
case "AudioPids":
return Optional.ofNullable(clazz.cast(audioPids()));
case "DataPTSControl":
return Optional.ofNullable(clazz.cast(dataPTSControlAsString()));
case "MaxPcrInterval":
return Optional.ofNullable(clazz.cast(maxPcrInterval()));
case "NielsenId3":
return Optional.ofNullable(clazz.cast(nielsenId3AsString()));
case "PatInterval":
return Optional.ofNullable(clazz.cast(patInterval()));
case "PcrControl":
return Optional.ofNullable(clazz.cast(pcrControlAsString()));
case "PcrPid":
return Optional.ofNullable(clazz.cast(pcrPid()));
case "PmtInterval":
return Optional.ofNullable(clazz.cast(pmtInterval()));
case "PmtPid":
return Optional.ofNullable(clazz.cast(pmtPid()));
case "PrivateMetadataPid":
return Optional.ofNullable(clazz.cast(privateMetadataPid()));
case "ProgramNumber":
return Optional.ofNullable(clazz.cast(programNumber()));
case "PtsOffset":
return Optional.ofNullable(clazz.cast(ptsOffset()));
case "PtsOffsetMode":
return Optional.ofNullable(clazz.cast(ptsOffsetModeAsString()));
case "Scte35Pid":
return Optional.ofNullable(clazz.cast(scte35Pid()));
case "Scte35Source":
return Optional.ofNullable(clazz.cast(scte35SourceAsString()));
case "TimedMetadata":
return Optional.ofNullable(clazz.cast(timedMetadataAsString()));
case "TimedMetadataPid":
return Optional.ofNullable(clazz.cast(timedMetadataPid()));
case "TransportStreamId":
return Optional.ofNullable(clazz.cast(transportStreamId()));
case "VideoPid":
return Optional.ofNullable(clazz.cast(videoPid()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function