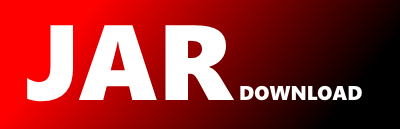
software.amazon.awssdk.services.mediaconvert.model.CmfcSettings Maven / Gradle / Ivy
Show all versions of mediaconvert Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.mediaconvert.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
* These settings relate to the fragmented MP4 container for the segments in your CMAF outputs.
*/
@Generated("software.amazon.awssdk:codegen")
public final class CmfcSettings implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField AUDIO_DURATION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AudioDuration").getter(getter(CmfcSettings::audioDurationAsString))
.setter(setter(Builder::audioDuration))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("audioDuration").build()).build();
private static final SdkField AUDIO_GROUP_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AudioGroupId").getter(getter(CmfcSettings::audioGroupId)).setter(setter(Builder::audioGroupId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("audioGroupId").build()).build();
private static final SdkField AUDIO_RENDITION_SETS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AudioRenditionSets").getter(getter(CmfcSettings::audioRenditionSets))
.setter(setter(Builder::audioRenditionSets))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("audioRenditionSets").build())
.build();
private static final SdkField AUDIO_TRACK_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AudioTrackType").getter(getter(CmfcSettings::audioTrackTypeAsString))
.setter(setter(Builder::audioTrackType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("audioTrackType").build()).build();
private static final SdkField DESCRIPTIVE_VIDEO_SERVICE_FLAG_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("DescriptiveVideoServiceFlag")
.getter(getter(CmfcSettings::descriptiveVideoServiceFlagAsString))
.setter(setter(Builder::descriptiveVideoServiceFlag))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("descriptiveVideoServiceFlag")
.build()).build();
private static final SdkField I_FRAME_ONLY_MANIFEST_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("IFrameOnlyManifest").getter(getter(CmfcSettings::iFrameOnlyManifestAsString))
.setter(setter(Builder::iFrameOnlyManifest))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("iFrameOnlyManifest").build())
.build();
private static final SdkField KLV_METADATA_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("KlvMetadata").getter(getter(CmfcSettings::klvMetadataAsString)).setter(setter(Builder::klvMetadata))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("klvMetadata").build()).build();
private static final SdkField MANIFEST_METADATA_SIGNALING_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ManifestMetadataSignaling").getter(getter(CmfcSettings::manifestMetadataSignalingAsString))
.setter(setter(Builder::manifestMetadataSignaling))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("manifestMetadataSignaling").build())
.build();
private static final SdkField SCTE35_ESAM_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Scte35Esam").getter(getter(CmfcSettings::scte35EsamAsString)).setter(setter(Builder::scte35Esam))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("scte35Esam").build()).build();
private static final SdkField SCTE35_SOURCE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Scte35Source").getter(getter(CmfcSettings::scte35SourceAsString)).setter(setter(Builder::scte35Source))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("scte35Source").build()).build();
private static final SdkField TIMED_METADATA_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TimedMetadata").getter(getter(CmfcSettings::timedMetadataAsString))
.setter(setter(Builder::timedMetadata))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("timedMetadata").build()).build();
private static final SdkField TIMED_METADATA_BOX_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TimedMetadataBoxVersion").getter(getter(CmfcSettings::timedMetadataBoxVersionAsString))
.setter(setter(Builder::timedMetadataBoxVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("timedMetadataBoxVersion").build())
.build();
private static final SdkField TIMED_METADATA_SCHEME_ID_URI_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TimedMetadataSchemeIdUri").getter(getter(CmfcSettings::timedMetadataSchemeIdUri))
.setter(setter(Builder::timedMetadataSchemeIdUri))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("timedMetadataSchemeIdUri").build())
.build();
private static final SdkField TIMED_METADATA_VALUE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TimedMetadataValue").getter(getter(CmfcSettings::timedMetadataValue))
.setter(setter(Builder::timedMetadataValue))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("timedMetadataValue").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(AUDIO_DURATION_FIELD,
AUDIO_GROUP_ID_FIELD, AUDIO_RENDITION_SETS_FIELD, AUDIO_TRACK_TYPE_FIELD, DESCRIPTIVE_VIDEO_SERVICE_FLAG_FIELD,
I_FRAME_ONLY_MANIFEST_FIELD, KLV_METADATA_FIELD, MANIFEST_METADATA_SIGNALING_FIELD, SCTE35_ESAM_FIELD,
SCTE35_SOURCE_FIELD, TIMED_METADATA_FIELD, TIMED_METADATA_BOX_VERSION_FIELD, TIMED_METADATA_SCHEME_ID_URI_FIELD,
TIMED_METADATA_VALUE_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final String audioDuration;
private final String audioGroupId;
private final String audioRenditionSets;
private final String audioTrackType;
private final String descriptiveVideoServiceFlag;
private final String iFrameOnlyManifest;
private final String klvMetadata;
private final String manifestMetadataSignaling;
private final String scte35Esam;
private final String scte35Source;
private final String timedMetadata;
private final String timedMetadataBoxVersion;
private final String timedMetadataSchemeIdUri;
private final String timedMetadataValue;
private CmfcSettings(BuilderImpl builder) {
this.audioDuration = builder.audioDuration;
this.audioGroupId = builder.audioGroupId;
this.audioRenditionSets = builder.audioRenditionSets;
this.audioTrackType = builder.audioTrackType;
this.descriptiveVideoServiceFlag = builder.descriptiveVideoServiceFlag;
this.iFrameOnlyManifest = builder.iFrameOnlyManifest;
this.klvMetadata = builder.klvMetadata;
this.manifestMetadataSignaling = builder.manifestMetadataSignaling;
this.scte35Esam = builder.scte35Esam;
this.scte35Source = builder.scte35Source;
this.timedMetadata = builder.timedMetadata;
this.timedMetadataBoxVersion = builder.timedMetadataBoxVersion;
this.timedMetadataSchemeIdUri = builder.timedMetadataSchemeIdUri;
this.timedMetadataValue = builder.timedMetadataValue;
}
/**
* Specify this setting only when your output will be consumed by a downstream repackaging workflow that is
* sensitive to very small duration differences between video and audio. For this situation, choose Match video
* duration. In all other cases, keep the default value, Default codec duration. When you choose Match video
* duration, MediaConvert pads the output audio streams with silence or trims them to ensure that the total duration
* of each audio stream is at least as long as the total duration of the video stream. After padding or trimming,
* the audio stream duration is no more than one frame longer than the video stream. MediaConvert applies audio
* padding or trimming only to the end of the last segment of the output. For unsegmented outputs, MediaConvert adds
* padding only to the end of the file. When you keep the default value, any minor discrepancies between audio and
* video duration will depend on your output audio codec.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #audioDuration}
* will return {@link CmfcAudioDuration#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #audioDurationAsString}.
*
*
* @return Specify this setting only when your output will be consumed by a downstream repackaging workflow that is
* sensitive to very small duration differences between video and audio. For this situation, choose Match
* video duration. In all other cases, keep the default value, Default codec duration. When you choose Match
* video duration, MediaConvert pads the output audio streams with silence or trims them to ensure that the
* total duration of each audio stream is at least as long as the total duration of the video stream. After
* padding or trimming, the audio stream duration is no more than one frame longer than the video stream.
* MediaConvert applies audio padding or trimming only to the end of the last segment of the output. For
* unsegmented outputs, MediaConvert adds padding only to the end of the file. When you keep the default
* value, any minor discrepancies between audio and video duration will depend on your output audio codec.
* @see CmfcAudioDuration
*/
public final CmfcAudioDuration audioDuration() {
return CmfcAudioDuration.fromValue(audioDuration);
}
/**
* Specify this setting only when your output will be consumed by a downstream repackaging workflow that is
* sensitive to very small duration differences between video and audio. For this situation, choose Match video
* duration. In all other cases, keep the default value, Default codec duration. When you choose Match video
* duration, MediaConvert pads the output audio streams with silence or trims them to ensure that the total duration
* of each audio stream is at least as long as the total duration of the video stream. After padding or trimming,
* the audio stream duration is no more than one frame longer than the video stream. MediaConvert applies audio
* padding or trimming only to the end of the last segment of the output. For unsegmented outputs, MediaConvert adds
* padding only to the end of the file. When you keep the default value, any minor discrepancies between audio and
* video duration will depend on your output audio codec.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #audioDuration}
* will return {@link CmfcAudioDuration#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #audioDurationAsString}.
*
*
* @return Specify this setting only when your output will be consumed by a downstream repackaging workflow that is
* sensitive to very small duration differences between video and audio. For this situation, choose Match
* video duration. In all other cases, keep the default value, Default codec duration. When you choose Match
* video duration, MediaConvert pads the output audio streams with silence or trims them to ensure that the
* total duration of each audio stream is at least as long as the total duration of the video stream. After
* padding or trimming, the audio stream duration is no more than one frame longer than the video stream.
* MediaConvert applies audio padding or trimming only to the end of the last segment of the output. For
* unsegmented outputs, MediaConvert adds padding only to the end of the file. When you keep the default
* value, any minor discrepancies between audio and video duration will depend on your output audio codec.
* @see CmfcAudioDuration
*/
public final String audioDurationAsString() {
return audioDuration;
}
/**
* Specify the audio rendition group for this audio rendition. Specify up to one value for each audio output in your
* output group. This value appears in your HLS parent manifest in the EXT-X-MEDIA tag of TYPE=AUDIO, as the value
* for the GROUP-ID attribute. For example, if you specify "audio_aac_1" for Audio group ID, it appears in your
* manifest like this: #EXT-X-MEDIA:TYPE=AUDIO,GROUP-ID="audio_aac_1". Related setting: To associate the rendition
* group that this audio track belongs to with a video rendition, include the same value that you provide here for
* that video output's setting Audio rendition sets.
*
* @return Specify the audio rendition group for this audio rendition. Specify up to one value for each audio output
* in your output group. This value appears in your HLS parent manifest in the EXT-X-MEDIA tag of
* TYPE=AUDIO, as the value for the GROUP-ID attribute. For example, if you specify "audio_aac_1" for Audio
* group ID, it appears in your manifest like this: #EXT-X-MEDIA:TYPE=AUDIO,GROUP-ID="audio_aac_1". Related
* setting: To associate the rendition group that this audio track belongs to with a video rendition,
* include the same value that you provide here for that video output's setting Audio rendition sets.
*/
public final String audioGroupId() {
return audioGroupId;
}
/**
* List the audio rendition groups that you want included with this video rendition. Use a comma-separated list. For
* example, say you want to include the audio rendition groups that have the audio group IDs "audio_aac_1" and
* "audio_dolby". Then you would specify this value: "audio_aac_1,audio_dolby". Related setting: The rendition
* groups that you include in your comma-separated list should all match values that you specify in the setting
* Audio group ID for audio renditions in the same output group as this video rendition. Default behavior: If you
* don't specify anything here and for Audio group ID, MediaConvert puts each audio variant in its own audio
* rendition group and associates it with every video variant. Each value in your list appears in your HLS parent
* manifest in the EXT-X-STREAM-INF tag as the value for the AUDIO attribute. To continue the previous example, say
* that the file name for the child manifest for your video rendition is "amazing_video_1.m3u8". Then, in your
* parent manifest, each value will appear on separate lines, like this: #EXT-X-STREAM-INF:AUDIO="audio_aac_1"...
* amazing_video_1.m3u8 #EXT-X-STREAM-INF:AUDIO="audio_dolby"... amazing_video_1.m3u8
*
* @return List the audio rendition groups that you want included with this video rendition. Use a comma-separated
* list. For example, say you want to include the audio rendition groups that have the audio group IDs
* "audio_aac_1" and "audio_dolby". Then you would specify this value: "audio_aac_1,audio_dolby". Related
* setting: The rendition groups that you include in your comma-separated list should all match values that
* you specify in the setting Audio group ID for audio renditions in the same output group as this video
* rendition. Default behavior: If you don't specify anything here and for Audio group ID, MediaConvert puts
* each audio variant in its own audio rendition group and associates it with every video variant. Each
* value in your list appears in your HLS parent manifest in the EXT-X-STREAM-INF tag as the value for the
* AUDIO attribute. To continue the previous example, say that the file name for the child manifest for your
* video rendition is "amazing_video_1.m3u8". Then, in your parent manifest, each value will appear on
* separate lines, like this: #EXT-X-STREAM-INF:AUDIO="audio_aac_1"... amazing_video_1.m3u8
* #EXT-X-STREAM-INF:AUDIO="audio_dolby"... amazing_video_1.m3u8
*/
public final String audioRenditionSets() {
return audioRenditionSets;
}
/**
* Use this setting to control the values that MediaConvert puts in your HLS parent playlist to control how the
* client player selects which audio track to play. Choose Audio-only variant stream (AUDIO_ONLY_VARIANT_STREAM) for
* any variant that you want to prohibit the client from playing with video. This causes MediaConvert to represent
* the variant as an EXT-X-STREAM-INF in the HLS manifest. The other options for this setting determine the values
* that MediaConvert writes for the DEFAULT and AUTOSELECT attributes of the EXT-X-MEDIA entry for the audio
* variant. For more information about these attributes, see the Apple documentation article
* https://developer.apple.com/documentation/http_live_streaming/example_playlists_for_http_live_streaming/
* adding_alternate_media_to_a_playlist. Choose Alternate audio, auto select, default to set DEFAULT=YES and
* AUTOSELECT=YES. Choose this value for only one variant in your output group. Choose Alternate audio, auto select,
* not default to set DEFAULT=NO and AUTOSELECT=YES. Choose Alternate Audio, Not Auto Select to set DEFAULT=NO and
* AUTOSELECT=NO. When you don't specify a value for this setting, MediaConvert defaults to Alternate audio, auto
* select, default. When there is more than one variant in your output group, you must explicitly choose a value for
* this setting.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #audioTrackType}
* will return {@link CmfcAudioTrackType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #audioTrackTypeAsString}.
*
*
* @return Use this setting to control the values that MediaConvert puts in your HLS parent playlist to control how
* the client player selects which audio track to play. Choose Audio-only variant stream
* (AUDIO_ONLY_VARIANT_STREAM) for any variant that you want to prohibit the client from playing with video.
* This causes MediaConvert to represent the variant as an EXT-X-STREAM-INF in the HLS manifest. The other
* options for this setting determine the values that MediaConvert writes for the DEFAULT and AUTOSELECT
* attributes of the EXT-X-MEDIA entry for the audio variant. For more information about these attributes,
* see the Apple documentation article
* https://developer.apple.com/documentation/http_live_streaming/example_playlists_for_http_live_streaming
* /adding_alternate_media_to_a_playlist. Choose Alternate audio, auto select, default to set DEFAULT=YES
* and AUTOSELECT=YES. Choose this value for only one variant in your output group. Choose Alternate audio,
* auto select, not default to set DEFAULT=NO and AUTOSELECT=YES. Choose Alternate Audio, Not Auto Select to
* set DEFAULT=NO and AUTOSELECT=NO. When you don't specify a value for this setting, MediaConvert defaults
* to Alternate audio, auto select, default. When there is more than one variant in your output group, you
* must explicitly choose a value for this setting.
* @see CmfcAudioTrackType
*/
public final CmfcAudioTrackType audioTrackType() {
return CmfcAudioTrackType.fromValue(audioTrackType);
}
/**
* Use this setting to control the values that MediaConvert puts in your HLS parent playlist to control how the
* client player selects which audio track to play. Choose Audio-only variant stream (AUDIO_ONLY_VARIANT_STREAM) for
* any variant that you want to prohibit the client from playing with video. This causes MediaConvert to represent
* the variant as an EXT-X-STREAM-INF in the HLS manifest. The other options for this setting determine the values
* that MediaConvert writes for the DEFAULT and AUTOSELECT attributes of the EXT-X-MEDIA entry for the audio
* variant. For more information about these attributes, see the Apple documentation article
* https://developer.apple.com/documentation/http_live_streaming/example_playlists_for_http_live_streaming/
* adding_alternate_media_to_a_playlist. Choose Alternate audio, auto select, default to set DEFAULT=YES and
* AUTOSELECT=YES. Choose this value for only one variant in your output group. Choose Alternate audio, auto select,
* not default to set DEFAULT=NO and AUTOSELECT=YES. Choose Alternate Audio, Not Auto Select to set DEFAULT=NO and
* AUTOSELECT=NO. When you don't specify a value for this setting, MediaConvert defaults to Alternate audio, auto
* select, default. When there is more than one variant in your output group, you must explicitly choose a value for
* this setting.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #audioTrackType}
* will return {@link CmfcAudioTrackType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #audioTrackTypeAsString}.
*
*
* @return Use this setting to control the values that MediaConvert puts in your HLS parent playlist to control how
* the client player selects which audio track to play. Choose Audio-only variant stream
* (AUDIO_ONLY_VARIANT_STREAM) for any variant that you want to prohibit the client from playing with video.
* This causes MediaConvert to represent the variant as an EXT-X-STREAM-INF in the HLS manifest. The other
* options for this setting determine the values that MediaConvert writes for the DEFAULT and AUTOSELECT
* attributes of the EXT-X-MEDIA entry for the audio variant. For more information about these attributes,
* see the Apple documentation article
* https://developer.apple.com/documentation/http_live_streaming/example_playlists_for_http_live_streaming
* /adding_alternate_media_to_a_playlist. Choose Alternate audio, auto select, default to set DEFAULT=YES
* and AUTOSELECT=YES. Choose this value for only one variant in your output group. Choose Alternate audio,
* auto select, not default to set DEFAULT=NO and AUTOSELECT=YES. Choose Alternate Audio, Not Auto Select to
* set DEFAULT=NO and AUTOSELECT=NO. When you don't specify a value for this setting, MediaConvert defaults
* to Alternate audio, auto select, default. When there is more than one variant in your output group, you
* must explicitly choose a value for this setting.
* @see CmfcAudioTrackType
*/
public final String audioTrackTypeAsString() {
return audioTrackType;
}
/**
* Specify whether to flag this audio track as descriptive video service (DVS) in your HLS parent manifest. When you
* choose Flag, MediaConvert includes the parameter CHARACTERISTICS="public.accessibility.describes-video" in the
* EXT-X-MEDIA entry for this track. When you keep the default choice, Don't flag, MediaConvert leaves this
* parameter out. The DVS flag can help with accessibility on Apple devices. For more information, see the Apple
* documentation.
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #descriptiveVideoServiceFlag} will return {@link CmfcDescriptiveVideoServiceFlag#UNKNOWN_TO_SDK_VERSION}.
* The raw value returned by the service is available from {@link #descriptiveVideoServiceFlagAsString}.
*
*
* @return Specify whether to flag this audio track as descriptive video service (DVS) in your HLS parent manifest.
* When you choose Flag, MediaConvert includes the parameter
* CHARACTERISTICS="public.accessibility.describes-video" in the EXT-X-MEDIA entry for this track. When you
* keep the default choice, Don't flag, MediaConvert leaves this parameter out. The DVS flag can help with
* accessibility on Apple devices. For more information, see the Apple documentation.
* @see CmfcDescriptiveVideoServiceFlag
*/
public final CmfcDescriptiveVideoServiceFlag descriptiveVideoServiceFlag() {
return CmfcDescriptiveVideoServiceFlag.fromValue(descriptiveVideoServiceFlag);
}
/**
* Specify whether to flag this audio track as descriptive video service (DVS) in your HLS parent manifest. When you
* choose Flag, MediaConvert includes the parameter CHARACTERISTICS="public.accessibility.describes-video" in the
* EXT-X-MEDIA entry for this track. When you keep the default choice, Don't flag, MediaConvert leaves this
* parameter out. The DVS flag can help with accessibility on Apple devices. For more information, see the Apple
* documentation.
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #descriptiveVideoServiceFlag} will return {@link CmfcDescriptiveVideoServiceFlag#UNKNOWN_TO_SDK_VERSION}.
* The raw value returned by the service is available from {@link #descriptiveVideoServiceFlagAsString}.
*
*
* @return Specify whether to flag this audio track as descriptive video service (DVS) in your HLS parent manifest.
* When you choose Flag, MediaConvert includes the parameter
* CHARACTERISTICS="public.accessibility.describes-video" in the EXT-X-MEDIA entry for this track. When you
* keep the default choice, Don't flag, MediaConvert leaves this parameter out. The DVS flag can help with
* accessibility on Apple devices. For more information, see the Apple documentation.
* @see CmfcDescriptiveVideoServiceFlag
*/
public final String descriptiveVideoServiceFlagAsString() {
return descriptiveVideoServiceFlag;
}
/**
* Choose Include to have MediaConvert generate an HLS child manifest that lists only the I-frames for this
* rendition, in addition to your regular manifest for this rendition. You might use this manifest as part of a
* workflow that creates preview functions for your video. MediaConvert adds both the I-frame only child manifest
* and the regular child manifest to the parent manifest. When you don't need the I-frame only child manifest, keep
* the default value Exclude.
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #iFrameOnlyManifest} will return {@link CmfcIFrameOnlyManifest#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #iFrameOnlyManifestAsString}.
*
*
* @return Choose Include to have MediaConvert generate an HLS child manifest that lists only the I-frames for this
* rendition, in addition to your regular manifest for this rendition. You might use this manifest as part
* of a workflow that creates preview functions for your video. MediaConvert adds both the I-frame only
* child manifest and the regular child manifest to the parent manifest. When you don't need the I-frame
* only child manifest, keep the default value Exclude.
* @see CmfcIFrameOnlyManifest
*/
public final CmfcIFrameOnlyManifest iFrameOnlyManifest() {
return CmfcIFrameOnlyManifest.fromValue(iFrameOnlyManifest);
}
/**
* Choose Include to have MediaConvert generate an HLS child manifest that lists only the I-frames for this
* rendition, in addition to your regular manifest for this rendition. You might use this manifest as part of a
* workflow that creates preview functions for your video. MediaConvert adds both the I-frame only child manifest
* and the regular child manifest to the parent manifest. When you don't need the I-frame only child manifest, keep
* the default value Exclude.
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #iFrameOnlyManifest} will return {@link CmfcIFrameOnlyManifest#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #iFrameOnlyManifestAsString}.
*
*
* @return Choose Include to have MediaConvert generate an HLS child manifest that lists only the I-frames for this
* rendition, in addition to your regular manifest for this rendition. You might use this manifest as part
* of a workflow that creates preview functions for your video. MediaConvert adds both the I-frame only
* child manifest and the regular child manifest to the parent manifest. When you don't need the I-frame
* only child manifest, keep the default value Exclude.
* @see CmfcIFrameOnlyManifest
*/
public final String iFrameOnlyManifestAsString() {
return iFrameOnlyManifest;
}
/**
* To include key-length-value metadata in this output: Set KLV metadata insertion to Passthrough. MediaConvert
* reads KLV metadata present in your input and writes each instance to a separate event message box in the output,
* according to MISB ST1910.1. To exclude this KLV metadata: Set KLV metadata insertion to None or leave blank.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #klvMetadata} will
* return {@link CmfcKlvMetadata#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #klvMetadataAsString}.
*
*
* @return To include key-length-value metadata in this output: Set KLV metadata insertion to Passthrough.
* MediaConvert reads KLV metadata present in your input and writes each instance to a separate event
* message box in the output, according to MISB ST1910.1. To exclude this KLV metadata: Set KLV metadata
* insertion to None or leave blank.
* @see CmfcKlvMetadata
*/
public final CmfcKlvMetadata klvMetadata() {
return CmfcKlvMetadata.fromValue(klvMetadata);
}
/**
* To include key-length-value metadata in this output: Set KLV metadata insertion to Passthrough. MediaConvert
* reads KLV metadata present in your input and writes each instance to a separate event message box in the output,
* according to MISB ST1910.1. To exclude this KLV metadata: Set KLV metadata insertion to None or leave blank.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #klvMetadata} will
* return {@link CmfcKlvMetadata#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #klvMetadataAsString}.
*
*
* @return To include key-length-value metadata in this output: Set KLV metadata insertion to Passthrough.
* MediaConvert reads KLV metadata present in your input and writes each instance to a separate event
* message box in the output, according to MISB ST1910.1. To exclude this KLV metadata: Set KLV metadata
* insertion to None or leave blank.
* @see CmfcKlvMetadata
*/
public final String klvMetadataAsString() {
return klvMetadata;
}
/**
* To add an InbandEventStream element in your output MPD manifest for each type of event message, set Manifest
* metadata signaling to Enabled. For ID3 event messages, the InbandEventStream element schemeIdUri will be same
* value that you specify for ID3 metadata scheme ID URI. For SCTE35 event messages, the InbandEventStream element
* schemeIdUri will be "urn:scte:scte35:2013:bin". To leave these elements out of your output MPD manifest, set
* Manifest metadata signaling to Disabled. To enable Manifest metadata signaling, you must also set SCTE-35 source
* to Passthrough, ESAM SCTE-35 to insert, or ID3 metadata to Passthrough.
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #manifestMetadataSignaling} will return {@link CmfcManifestMetadataSignaling#UNKNOWN_TO_SDK_VERSION}. The
* raw value returned by the service is available from {@link #manifestMetadataSignalingAsString}.
*
*
* @return To add an InbandEventStream element in your output MPD manifest for each type of event message, set
* Manifest metadata signaling to Enabled. For ID3 event messages, the InbandEventStream element schemeIdUri
* will be same value that you specify for ID3 metadata scheme ID URI. For SCTE35 event messages, the
* InbandEventStream element schemeIdUri will be "urn:scte:scte35:2013:bin". To leave these elements out of
* your output MPD manifest, set Manifest metadata signaling to Disabled. To enable Manifest metadata
* signaling, you must also set SCTE-35 source to Passthrough, ESAM SCTE-35 to insert, or ID3 metadata to
* Passthrough.
* @see CmfcManifestMetadataSignaling
*/
public final CmfcManifestMetadataSignaling manifestMetadataSignaling() {
return CmfcManifestMetadataSignaling.fromValue(manifestMetadataSignaling);
}
/**
* To add an InbandEventStream element in your output MPD manifest for each type of event message, set Manifest
* metadata signaling to Enabled. For ID3 event messages, the InbandEventStream element schemeIdUri will be same
* value that you specify for ID3 metadata scheme ID URI. For SCTE35 event messages, the InbandEventStream element
* schemeIdUri will be "urn:scte:scte35:2013:bin". To leave these elements out of your output MPD manifest, set
* Manifest metadata signaling to Disabled. To enable Manifest metadata signaling, you must also set SCTE-35 source
* to Passthrough, ESAM SCTE-35 to insert, or ID3 metadata to Passthrough.
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #manifestMetadataSignaling} will return {@link CmfcManifestMetadataSignaling#UNKNOWN_TO_SDK_VERSION}. The
* raw value returned by the service is available from {@link #manifestMetadataSignalingAsString}.
*
*
* @return To add an InbandEventStream element in your output MPD manifest for each type of event message, set
* Manifest metadata signaling to Enabled. For ID3 event messages, the InbandEventStream element schemeIdUri
* will be same value that you specify for ID3 metadata scheme ID URI. For SCTE35 event messages, the
* InbandEventStream element schemeIdUri will be "urn:scte:scte35:2013:bin". To leave these elements out of
* your output MPD manifest, set Manifest metadata signaling to Disabled. To enable Manifest metadata
* signaling, you must also set SCTE-35 source to Passthrough, ESAM SCTE-35 to insert, or ID3 metadata to
* Passthrough.
* @see CmfcManifestMetadataSignaling
*/
public final String manifestMetadataSignalingAsString() {
return manifestMetadataSignaling;
}
/**
* Use this setting only when you specify SCTE-35 markers from ESAM. Choose INSERT to put SCTE-35 markers in this
* output at the insertion points that you specify in an ESAM XML document. Provide the document in the setting SCC
* XML.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #scte35Esam} will
* return {@link CmfcScte35Esam#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #scte35EsamAsString}.
*
*
* @return Use this setting only when you specify SCTE-35 markers from ESAM. Choose INSERT to put SCTE-35 markers in
* this output at the insertion points that you specify in an ESAM XML document. Provide the document in the
* setting SCC XML.
* @see CmfcScte35Esam
*/
public final CmfcScte35Esam scte35Esam() {
return CmfcScte35Esam.fromValue(scte35Esam);
}
/**
* Use this setting only when you specify SCTE-35 markers from ESAM. Choose INSERT to put SCTE-35 markers in this
* output at the insertion points that you specify in an ESAM XML document. Provide the document in the setting SCC
* XML.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #scte35Esam} will
* return {@link CmfcScte35Esam#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #scte35EsamAsString}.
*
*
* @return Use this setting only when you specify SCTE-35 markers from ESAM. Choose INSERT to put SCTE-35 markers in
* this output at the insertion points that you specify in an ESAM XML document. Provide the document in the
* setting SCC XML.
* @see CmfcScte35Esam
*/
public final String scte35EsamAsString() {
return scte35Esam;
}
/**
* Ignore this setting unless you have SCTE-35 markers in your input video file. Choose Passthrough if you want
* SCTE-35 markers that appear in your input to also appear in this output. Choose None if you don't want those
* SCTE-35 markers in this output.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #scte35Source} will
* return {@link CmfcScte35Source#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #scte35SourceAsString}.
*
*
* @return Ignore this setting unless you have SCTE-35 markers in your input video file. Choose Passthrough if you
* want SCTE-35 markers that appear in your input to also appear in this output. Choose None if you don't
* want those SCTE-35 markers in this output.
* @see CmfcScte35Source
*/
public final CmfcScte35Source scte35Source() {
return CmfcScte35Source.fromValue(scte35Source);
}
/**
* Ignore this setting unless you have SCTE-35 markers in your input video file. Choose Passthrough if you want
* SCTE-35 markers that appear in your input to also appear in this output. Choose None if you don't want those
* SCTE-35 markers in this output.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #scte35Source} will
* return {@link CmfcScte35Source#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #scte35SourceAsString}.
*
*
* @return Ignore this setting unless you have SCTE-35 markers in your input video file. Choose Passthrough if you
* want SCTE-35 markers that appear in your input to also appear in this output. Choose None if you don't
* want those SCTE-35 markers in this output.
* @see CmfcScte35Source
*/
public final String scte35SourceAsString() {
return scte35Source;
}
/**
* To include ID3 metadata in this output: Set ID3 metadata to Passthrough. Specify this ID3 metadata in Custom ID3
* metadata inserter. MediaConvert writes each instance of ID3 metadata in a separate Event Message (eMSG) box. To
* exclude this ID3 metadata: Set ID3 metadata to None or leave blank.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #timedMetadata}
* will return {@link CmfcTimedMetadata#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #timedMetadataAsString}.
*
*
* @return To include ID3 metadata in this output: Set ID3 metadata to Passthrough. Specify this ID3 metadata in
* Custom ID3 metadata inserter. MediaConvert writes each instance of ID3 metadata in a separate Event
* Message (eMSG) box. To exclude this ID3 metadata: Set ID3 metadata to None or leave blank.
* @see CmfcTimedMetadata
*/
public final CmfcTimedMetadata timedMetadata() {
return CmfcTimedMetadata.fromValue(timedMetadata);
}
/**
* To include ID3 metadata in this output: Set ID3 metadata to Passthrough. Specify this ID3 metadata in Custom ID3
* metadata inserter. MediaConvert writes each instance of ID3 metadata in a separate Event Message (eMSG) box. To
* exclude this ID3 metadata: Set ID3 metadata to None or leave blank.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #timedMetadata}
* will return {@link CmfcTimedMetadata#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #timedMetadataAsString}.
*
*
* @return To include ID3 metadata in this output: Set ID3 metadata to Passthrough. Specify this ID3 metadata in
* Custom ID3 metadata inserter. MediaConvert writes each instance of ID3 metadata in a separate Event
* Message (eMSG) box. To exclude this ID3 metadata: Set ID3 metadata to None or leave blank.
* @see CmfcTimedMetadata
*/
public final String timedMetadataAsString() {
return timedMetadata;
}
/**
* Specify the event message box (eMSG) version for ID3 timed metadata in your output. For more information, see
* ISO/IEC 23009-1:2022 section 5.10.3.3.3 Syntax. Leave blank to use the default value Version 0. When you specify
* Version 1, you must also set ID3 metadata to Passthrough.
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #timedMetadataBoxVersion} will return {@link CmfcTimedMetadataBoxVersion#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #timedMetadataBoxVersionAsString}.
*
*
* @return Specify the event message box (eMSG) version for ID3 timed metadata in your output. For more information,
* see ISO/IEC 23009-1:2022 section 5.10.3.3.3 Syntax. Leave blank to use the default value Version 0. When
* you specify Version 1, you must also set ID3 metadata to Passthrough.
* @see CmfcTimedMetadataBoxVersion
*/
public final CmfcTimedMetadataBoxVersion timedMetadataBoxVersion() {
return CmfcTimedMetadataBoxVersion.fromValue(timedMetadataBoxVersion);
}
/**
* Specify the event message box (eMSG) version for ID3 timed metadata in your output. For more information, see
* ISO/IEC 23009-1:2022 section 5.10.3.3.3 Syntax. Leave blank to use the default value Version 0. When you specify
* Version 1, you must also set ID3 metadata to Passthrough.
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #timedMetadataBoxVersion} will return {@link CmfcTimedMetadataBoxVersion#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #timedMetadataBoxVersionAsString}.
*
*
* @return Specify the event message box (eMSG) version for ID3 timed metadata in your output. For more information,
* see ISO/IEC 23009-1:2022 section 5.10.3.3.3 Syntax. Leave blank to use the default value Version 0. When
* you specify Version 1, you must also set ID3 metadata to Passthrough.
* @see CmfcTimedMetadataBoxVersion
*/
public final String timedMetadataBoxVersionAsString() {
return timedMetadataBoxVersion;
}
/**
* Specify the event message box (eMSG) scheme ID URI for ID3 timed metadata in your output. For more information,
* see ISO/IEC 23009-1:2022 section 5.10.3.3.4 Semantics. Leave blank to use the default value:
* https://aomedia.org/emsg/ID3 When you specify a value for ID3 metadata scheme ID URI, you must also set ID3
* metadata to Passthrough.
*
* @return Specify the event message box (eMSG) scheme ID URI for ID3 timed metadata in your output. For more
* information, see ISO/IEC 23009-1:2022 section 5.10.3.3.4 Semantics. Leave blank to use the default value:
* https://aomedia.org/emsg/ID3 When you specify a value for ID3 metadata scheme ID URI, you must also set
* ID3 metadata to Passthrough.
*/
public final String timedMetadataSchemeIdUri() {
return timedMetadataSchemeIdUri;
}
/**
* Specify the event message box (eMSG) value for ID3 timed metadata in your output. For more information, see
* ISO/IEC 23009-1:2022 section 5.10.3.3.4 Semantics. When you specify a value for ID3 Metadata Value, you must also
* set ID3 metadata to Passthrough.
*
* @return Specify the event message box (eMSG) value for ID3 timed metadata in your output. For more information,
* see ISO/IEC 23009-1:2022 section 5.10.3.3.4 Semantics. When you specify a value for ID3 Metadata Value,
* you must also set ID3 metadata to Passthrough.
*/
public final String timedMetadataValue() {
return timedMetadataValue;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(audioDurationAsString());
hashCode = 31 * hashCode + Objects.hashCode(audioGroupId());
hashCode = 31 * hashCode + Objects.hashCode(audioRenditionSets());
hashCode = 31 * hashCode + Objects.hashCode(audioTrackTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(descriptiveVideoServiceFlagAsString());
hashCode = 31 * hashCode + Objects.hashCode(iFrameOnlyManifestAsString());
hashCode = 31 * hashCode + Objects.hashCode(klvMetadataAsString());
hashCode = 31 * hashCode + Objects.hashCode(manifestMetadataSignalingAsString());
hashCode = 31 * hashCode + Objects.hashCode(scte35EsamAsString());
hashCode = 31 * hashCode + Objects.hashCode(scte35SourceAsString());
hashCode = 31 * hashCode + Objects.hashCode(timedMetadataAsString());
hashCode = 31 * hashCode + Objects.hashCode(timedMetadataBoxVersionAsString());
hashCode = 31 * hashCode + Objects.hashCode(timedMetadataSchemeIdUri());
hashCode = 31 * hashCode + Objects.hashCode(timedMetadataValue());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CmfcSettings)) {
return false;
}
CmfcSettings other = (CmfcSettings) obj;
return Objects.equals(audioDurationAsString(), other.audioDurationAsString())
&& Objects.equals(audioGroupId(), other.audioGroupId())
&& Objects.equals(audioRenditionSets(), other.audioRenditionSets())
&& Objects.equals(audioTrackTypeAsString(), other.audioTrackTypeAsString())
&& Objects.equals(descriptiveVideoServiceFlagAsString(), other.descriptiveVideoServiceFlagAsString())
&& Objects.equals(iFrameOnlyManifestAsString(), other.iFrameOnlyManifestAsString())
&& Objects.equals(klvMetadataAsString(), other.klvMetadataAsString())
&& Objects.equals(manifestMetadataSignalingAsString(), other.manifestMetadataSignalingAsString())
&& Objects.equals(scte35EsamAsString(), other.scte35EsamAsString())
&& Objects.equals(scte35SourceAsString(), other.scte35SourceAsString())
&& Objects.equals(timedMetadataAsString(), other.timedMetadataAsString())
&& Objects.equals(timedMetadataBoxVersionAsString(), other.timedMetadataBoxVersionAsString())
&& Objects.equals(timedMetadataSchemeIdUri(), other.timedMetadataSchemeIdUri())
&& Objects.equals(timedMetadataValue(), other.timedMetadataValue());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CmfcSettings").add("AudioDuration", audioDurationAsString()).add("AudioGroupId", audioGroupId())
.add("AudioRenditionSets", audioRenditionSets()).add("AudioTrackType", audioTrackTypeAsString())
.add("DescriptiveVideoServiceFlag", descriptiveVideoServiceFlagAsString())
.add("IFrameOnlyManifest", iFrameOnlyManifestAsString()).add("KlvMetadata", klvMetadataAsString())
.add("ManifestMetadataSignaling", manifestMetadataSignalingAsString()).add("Scte35Esam", scte35EsamAsString())
.add("Scte35Source", scte35SourceAsString()).add("TimedMetadata", timedMetadataAsString())
.add("TimedMetadataBoxVersion", timedMetadataBoxVersionAsString())
.add("TimedMetadataSchemeIdUri", timedMetadataSchemeIdUri()).add("TimedMetadataValue", timedMetadataValue())
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AudioDuration":
return Optional.ofNullable(clazz.cast(audioDurationAsString()));
case "AudioGroupId":
return Optional.ofNullable(clazz.cast(audioGroupId()));
case "AudioRenditionSets":
return Optional.ofNullable(clazz.cast(audioRenditionSets()));
case "AudioTrackType":
return Optional.ofNullable(clazz.cast(audioTrackTypeAsString()));
case "DescriptiveVideoServiceFlag":
return Optional.ofNullable(clazz.cast(descriptiveVideoServiceFlagAsString()));
case "IFrameOnlyManifest":
return Optional.ofNullable(clazz.cast(iFrameOnlyManifestAsString()));
case "KlvMetadata":
return Optional.ofNullable(clazz.cast(klvMetadataAsString()));
case "ManifestMetadataSignaling":
return Optional.ofNullable(clazz.cast(manifestMetadataSignalingAsString()));
case "Scte35Esam":
return Optional.ofNullable(clazz.cast(scte35EsamAsString()));
case "Scte35Source":
return Optional.ofNullable(clazz.cast(scte35SourceAsString()));
case "TimedMetadata":
return Optional.ofNullable(clazz.cast(timedMetadataAsString()));
case "TimedMetadataBoxVersion":
return Optional.ofNullable(clazz.cast(timedMetadataBoxVersionAsString()));
case "TimedMetadataSchemeIdUri":
return Optional.ofNullable(clazz.cast(timedMetadataSchemeIdUri()));
case "TimedMetadataValue":
return Optional.ofNullable(clazz.cast(timedMetadataValue()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("audioDuration", AUDIO_DURATION_FIELD);
map.put("audioGroupId", AUDIO_GROUP_ID_FIELD);
map.put("audioRenditionSets", AUDIO_RENDITION_SETS_FIELD);
map.put("audioTrackType", AUDIO_TRACK_TYPE_FIELD);
map.put("descriptiveVideoServiceFlag", DESCRIPTIVE_VIDEO_SERVICE_FLAG_FIELD);
map.put("iFrameOnlyManifest", I_FRAME_ONLY_MANIFEST_FIELD);
map.put("klvMetadata", KLV_METADATA_FIELD);
map.put("manifestMetadataSignaling", MANIFEST_METADATA_SIGNALING_FIELD);
map.put("scte35Esam", SCTE35_ESAM_FIELD);
map.put("scte35Source", SCTE35_SOURCE_FIELD);
map.put("timedMetadata", TIMED_METADATA_FIELD);
map.put("timedMetadataBoxVersion", TIMED_METADATA_BOX_VERSION_FIELD);
map.put("timedMetadataSchemeIdUri", TIMED_METADATA_SCHEME_ID_URI_FIELD);
map.put("timedMetadataValue", TIMED_METADATA_VALUE_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function