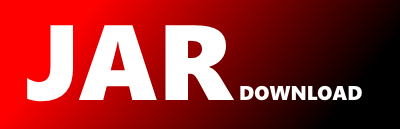
software.amazon.awssdk.services.medialive.model.AacSettings Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.medialive.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
* Aac Settings
*/
@Generated("software.amazon.awssdk:codegen")
public final class AacSettings implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField BITRATE_FIELD = SdkField. builder(MarshallingType.DOUBLE).memberName("Bitrate")
.getter(getter(AacSettings::bitrate)).setter(setter(Builder::bitrate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("bitrate").build()).build();
private static final SdkField CODING_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CodingMode").getter(getter(AacSettings::codingModeAsString)).setter(setter(Builder::codingMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("codingMode").build()).build();
private static final SdkField INPUT_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InputType").getter(getter(AacSettings::inputTypeAsString)).setter(setter(Builder::inputType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("inputType").build()).build();
private static final SdkField PROFILE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Profile")
.getter(getter(AacSettings::profileAsString)).setter(setter(Builder::profile))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("profile").build()).build();
private static final SdkField RATE_CONTROL_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RateControlMode").getter(getter(AacSettings::rateControlModeAsString))
.setter(setter(Builder::rateControlMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("rateControlMode").build()).build();
private static final SdkField RAW_FORMAT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RawFormat").getter(getter(AacSettings::rawFormatAsString)).setter(setter(Builder::rawFormat))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("rawFormat").build()).build();
private static final SdkField SAMPLE_RATE_FIELD = SdkField. builder(MarshallingType.DOUBLE)
.memberName("SampleRate").getter(getter(AacSettings::sampleRate)).setter(setter(Builder::sampleRate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("sampleRate").build()).build();
private static final SdkField SPEC_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Spec")
.getter(getter(AacSettings::specAsString)).setter(setter(Builder::spec))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("spec").build()).build();
private static final SdkField VBR_QUALITY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("VbrQuality").getter(getter(AacSettings::vbrQualityAsString)).setter(setter(Builder::vbrQuality))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("vbrQuality").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(BITRATE_FIELD,
CODING_MODE_FIELD, INPUT_TYPE_FIELD, PROFILE_FIELD, RATE_CONTROL_MODE_FIELD, RAW_FORMAT_FIELD, SAMPLE_RATE_FIELD,
SPEC_FIELD, VBR_QUALITY_FIELD));
private static final long serialVersionUID = 1L;
private final Double bitrate;
private final String codingMode;
private final String inputType;
private final String profile;
private final String rateControlMode;
private final String rawFormat;
private final Double sampleRate;
private final String spec;
private final String vbrQuality;
private AacSettings(BuilderImpl builder) {
this.bitrate = builder.bitrate;
this.codingMode = builder.codingMode;
this.inputType = builder.inputType;
this.profile = builder.profile;
this.rateControlMode = builder.rateControlMode;
this.rawFormat = builder.rawFormat;
this.sampleRate = builder.sampleRate;
this.spec = builder.spec;
this.vbrQuality = builder.vbrQuality;
}
/**
* Average bitrate in bits/second. Valid values depend on rate control mode and profile.
*
* @return Average bitrate in bits/second. Valid values depend on rate control mode and profile.
*/
public final Double bitrate() {
return bitrate;
}
/**
* Mono, Stereo, or 5.1 channel layout. Valid values depend on rate control mode and profile. The adReceiverMix
* setting receives a stereo description plus control track and emits a mono AAC encode of the description track,
* with control data emitted in the PES header as per ETSI TS 101 154 Annex E.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #codingMode} will
* return {@link AacCodingMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #codingModeAsString}.
*
*
* @return Mono, Stereo, or 5.1 channel layout. Valid values depend on rate control mode and profile. The
* adReceiverMix setting receives a stereo description plus control track and emits a mono AAC encode of the
* description track, with control data emitted in the PES header as per ETSI TS 101 154 Annex E.
* @see AacCodingMode
*/
public final AacCodingMode codingMode() {
return AacCodingMode.fromValue(codingMode);
}
/**
* Mono, Stereo, or 5.1 channel layout. Valid values depend on rate control mode and profile. The adReceiverMix
* setting receives a stereo description plus control track and emits a mono AAC encode of the description track,
* with control data emitted in the PES header as per ETSI TS 101 154 Annex E.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #codingMode} will
* return {@link AacCodingMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #codingModeAsString}.
*
*
* @return Mono, Stereo, or 5.1 channel layout. Valid values depend on rate control mode and profile. The
* adReceiverMix setting receives a stereo description plus control track and emits a mono AAC encode of the
* description track, with control data emitted in the PES header as per ETSI TS 101 154 Annex E.
* @see AacCodingMode
*/
public final String codingModeAsString() {
return codingMode;
}
/**
* Set to "broadcasterMixedAd" when input contains pre-mixed main audio + AD (narration) as a stereo pair. The Audio
* Type field (audioType) will be set to 3, which signals to downstream systems that this stream contains
* "broadcaster mixed AD". Note that the input received by the encoder must contain pre-mixed audio; the encoder
* does not perform the mixing. The values in audioTypeControl and audioType (in AudioDescription) are ignored when
* set to broadcasterMixedAd.
*
* Leave set to "normal" when input does not contain pre-mixed audio + AD.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #inputType} will
* return {@link AacInputType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #inputTypeAsString}.
*
*
* @return Set to "broadcasterMixedAd" when input contains pre-mixed main audio + AD (narration) as a stereo pair.
* The Audio Type field (audioType) will be set to 3, which signals to downstream systems that this stream
* contains "broadcaster mixed AD". Note that the input received by the encoder must contain pre-mixed
* audio; the encoder does not perform the mixing. The values in audioTypeControl and audioType (in
* AudioDescription) are ignored when set to broadcasterMixedAd.
*
* Leave set to "normal" when input does not contain pre-mixed audio + AD.
* @see AacInputType
*/
public final AacInputType inputType() {
return AacInputType.fromValue(inputType);
}
/**
* Set to "broadcasterMixedAd" when input contains pre-mixed main audio + AD (narration) as a stereo pair. The Audio
* Type field (audioType) will be set to 3, which signals to downstream systems that this stream contains
* "broadcaster mixed AD". Note that the input received by the encoder must contain pre-mixed audio; the encoder
* does not perform the mixing. The values in audioTypeControl and audioType (in AudioDescription) are ignored when
* set to broadcasterMixedAd.
*
* Leave set to "normal" when input does not contain pre-mixed audio + AD.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #inputType} will
* return {@link AacInputType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #inputTypeAsString}.
*
*
* @return Set to "broadcasterMixedAd" when input contains pre-mixed main audio + AD (narration) as a stereo pair.
* The Audio Type field (audioType) will be set to 3, which signals to downstream systems that this stream
* contains "broadcaster mixed AD". Note that the input received by the encoder must contain pre-mixed
* audio; the encoder does not perform the mixing. The values in audioTypeControl and audioType (in
* AudioDescription) are ignored when set to broadcasterMixedAd.
*
* Leave set to "normal" when input does not contain pre-mixed audio + AD.
* @see AacInputType
*/
public final String inputTypeAsString() {
return inputType;
}
/**
* AAC Profile.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #profile} will
* return {@link AacProfile#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #profileAsString}.
*
*
* @return AAC Profile.
* @see AacProfile
*/
public final AacProfile profile() {
return AacProfile.fromValue(profile);
}
/**
* AAC Profile.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #profile} will
* return {@link AacProfile#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #profileAsString}.
*
*
* @return AAC Profile.
* @see AacProfile
*/
public final String profileAsString() {
return profile;
}
/**
* Rate Control Mode.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #rateControlMode}
* will return {@link AacRateControlMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #rateControlModeAsString}.
*
*
* @return Rate Control Mode.
* @see AacRateControlMode
*/
public final AacRateControlMode rateControlMode() {
return AacRateControlMode.fromValue(rateControlMode);
}
/**
* Rate Control Mode.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #rateControlMode}
* will return {@link AacRateControlMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #rateControlModeAsString}.
*
*
* @return Rate Control Mode.
* @see AacRateControlMode
*/
public final String rateControlModeAsString() {
return rateControlMode;
}
/**
* Sets LATM / LOAS AAC output for raw containers.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #rawFormat} will
* return {@link AacRawFormat#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #rawFormatAsString}.
*
*
* @return Sets LATM / LOAS AAC output for raw containers.
* @see AacRawFormat
*/
public final AacRawFormat rawFormat() {
return AacRawFormat.fromValue(rawFormat);
}
/**
* Sets LATM / LOAS AAC output for raw containers.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #rawFormat} will
* return {@link AacRawFormat#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #rawFormatAsString}.
*
*
* @return Sets LATM / LOAS AAC output for raw containers.
* @see AacRawFormat
*/
public final String rawFormatAsString() {
return rawFormat;
}
/**
* Sample rate in Hz. Valid values depend on rate control mode and profile.
*
* @return Sample rate in Hz. Valid values depend on rate control mode and profile.
*/
public final Double sampleRate() {
return sampleRate;
}
/**
* Use MPEG-2 AAC audio instead of MPEG-4 AAC audio for raw or MPEG-2 Transport Stream containers.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #spec} will return
* {@link AacSpec#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #specAsString}.
*
*
* @return Use MPEG-2 AAC audio instead of MPEG-4 AAC audio for raw or MPEG-2 Transport Stream containers.
* @see AacSpec
*/
public final AacSpec spec() {
return AacSpec.fromValue(spec);
}
/**
* Use MPEG-2 AAC audio instead of MPEG-4 AAC audio for raw or MPEG-2 Transport Stream containers.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #spec} will return
* {@link AacSpec#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #specAsString}.
*
*
* @return Use MPEG-2 AAC audio instead of MPEG-4 AAC audio for raw or MPEG-2 Transport Stream containers.
* @see AacSpec
*/
public final String specAsString() {
return spec;
}
/**
* VBR Quality Level - Only used if rateControlMode is VBR.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #vbrQuality} will
* return {@link AacVbrQuality#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #vbrQualityAsString}.
*
*
* @return VBR Quality Level - Only used if rateControlMode is VBR.
* @see AacVbrQuality
*/
public final AacVbrQuality vbrQuality() {
return AacVbrQuality.fromValue(vbrQuality);
}
/**
* VBR Quality Level - Only used if rateControlMode is VBR.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #vbrQuality} will
* return {@link AacVbrQuality#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #vbrQualityAsString}.
*
*
* @return VBR Quality Level - Only used if rateControlMode is VBR.
* @see AacVbrQuality
*/
public final String vbrQualityAsString() {
return vbrQuality;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(bitrate());
hashCode = 31 * hashCode + Objects.hashCode(codingModeAsString());
hashCode = 31 * hashCode + Objects.hashCode(inputTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(profileAsString());
hashCode = 31 * hashCode + Objects.hashCode(rateControlModeAsString());
hashCode = 31 * hashCode + Objects.hashCode(rawFormatAsString());
hashCode = 31 * hashCode + Objects.hashCode(sampleRate());
hashCode = 31 * hashCode + Objects.hashCode(specAsString());
hashCode = 31 * hashCode + Objects.hashCode(vbrQualityAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof AacSettings)) {
return false;
}
AacSettings other = (AacSettings) obj;
return Objects.equals(bitrate(), other.bitrate()) && Objects.equals(codingModeAsString(), other.codingModeAsString())
&& Objects.equals(inputTypeAsString(), other.inputTypeAsString())
&& Objects.equals(profileAsString(), other.profileAsString())
&& Objects.equals(rateControlModeAsString(), other.rateControlModeAsString())
&& Objects.equals(rawFormatAsString(), other.rawFormatAsString())
&& Objects.equals(sampleRate(), other.sampleRate()) && Objects.equals(specAsString(), other.specAsString())
&& Objects.equals(vbrQualityAsString(), other.vbrQualityAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("AacSettings").add("Bitrate", bitrate()).add("CodingMode", codingModeAsString())
.add("InputType", inputTypeAsString()).add("Profile", profileAsString())
.add("RateControlMode", rateControlModeAsString()).add("RawFormat", rawFormatAsString())
.add("SampleRate", sampleRate()).add("Spec", specAsString()).add("VbrQuality", vbrQualityAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Bitrate":
return Optional.ofNullable(clazz.cast(bitrate()));
case "CodingMode":
return Optional.ofNullable(clazz.cast(codingModeAsString()));
case "InputType":
return Optional.ofNullable(clazz.cast(inputTypeAsString()));
case "Profile":
return Optional.ofNullable(clazz.cast(profileAsString()));
case "RateControlMode":
return Optional.ofNullable(clazz.cast(rateControlModeAsString()));
case "RawFormat":
return Optional.ofNullable(clazz.cast(rawFormatAsString()));
case "SampleRate":
return Optional.ofNullable(clazz.cast(sampleRate()));
case "Spec":
return Optional.ofNullable(clazz.cast(specAsString()));
case "VbrQuality":
return Optional.ofNullable(clazz.cast(vbrQualityAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function