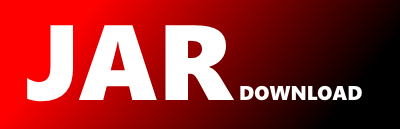
software.amazon.awssdk.services.medialive.model.FailoverConditionSettings Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of medialive Show documentation
Show all versions of medialive Show documentation
The AWS Java SDK for AWS Elemental MediaLive module holds the client classes that are used for
communicating
with AWS Elemental MediaLive Service
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.medialive.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
* Settings for one failover condition.
*/
@Generated("software.amazon.awssdk:codegen")
public final class FailoverConditionSettings implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField AUDIO_SILENCE_SETTINGS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("AudioSilenceSettings")
.getter(getter(FailoverConditionSettings::audioSilenceSettings)).setter(setter(Builder::audioSilenceSettings))
.constructor(AudioSilenceFailoverSettings::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("audioSilenceSettings").build())
.build();
private static final SdkField INPUT_LOSS_SETTINGS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("InputLossSettings")
.getter(getter(FailoverConditionSettings::inputLossSettings)).setter(setter(Builder::inputLossSettings))
.constructor(InputLossFailoverSettings::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("inputLossSettings").build()).build();
private static final SdkField VIDEO_BLACK_SETTINGS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("VideoBlackSettings")
.getter(getter(FailoverConditionSettings::videoBlackSettings)).setter(setter(Builder::videoBlackSettings))
.constructor(VideoBlackFailoverSettings::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("videoBlackSettings").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(AUDIO_SILENCE_SETTINGS_FIELD,
INPUT_LOSS_SETTINGS_FIELD, VIDEO_BLACK_SETTINGS_FIELD));
private static final long serialVersionUID = 1L;
private final AudioSilenceFailoverSettings audioSilenceSettings;
private final InputLossFailoverSettings inputLossSettings;
private final VideoBlackFailoverSettings videoBlackSettings;
private FailoverConditionSettings(BuilderImpl builder) {
this.audioSilenceSettings = builder.audioSilenceSettings;
this.inputLossSettings = builder.inputLossSettings;
this.videoBlackSettings = builder.videoBlackSettings;
}
/**
* MediaLive will perform a failover if the specified audio selector is silent for the specified period.
*
* @return MediaLive will perform a failover if the specified audio selector is silent for the specified period.
*/
public final AudioSilenceFailoverSettings audioSilenceSettings() {
return audioSilenceSettings;
}
/**
* MediaLive will perform a failover if content is not detected in this input for the specified period.
*
* @return MediaLive will perform a failover if content is not detected in this input for the specified period.
*/
public final InputLossFailoverSettings inputLossSettings() {
return inputLossSettings;
}
/**
* MediaLive will perform a failover if content is considered black for the specified period.
*
* @return MediaLive will perform a failover if content is considered black for the specified period.
*/
public final VideoBlackFailoverSettings videoBlackSettings() {
return videoBlackSettings;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(audioSilenceSettings());
hashCode = 31 * hashCode + Objects.hashCode(inputLossSettings());
hashCode = 31 * hashCode + Objects.hashCode(videoBlackSettings());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof FailoverConditionSettings)) {
return false;
}
FailoverConditionSettings other = (FailoverConditionSettings) obj;
return Objects.equals(audioSilenceSettings(), other.audioSilenceSettings())
&& Objects.equals(inputLossSettings(), other.inputLossSettings())
&& Objects.equals(videoBlackSettings(), other.videoBlackSettings());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("FailoverConditionSettings").add("AudioSilenceSettings", audioSilenceSettings())
.add("InputLossSettings", inputLossSettings()).add("VideoBlackSettings", videoBlackSettings()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AudioSilenceSettings":
return Optional.ofNullable(clazz.cast(audioSilenceSettings()));
case "InputLossSettings":
return Optional.ofNullable(clazz.cast(inputLossSettings()));
case "VideoBlackSettings":
return Optional.ofNullable(clazz.cast(videoBlackSettings()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy