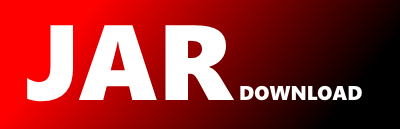
software.amazon.awssdk.services.medialive.model.FecOutputSettings Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of medialive Show documentation
Show all versions of medialive Show documentation
The AWS Java SDK for AWS Elemental MediaLive module holds the client classes that are used for
communicating
with AWS Elemental MediaLive Service
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.medialive.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
* Fec Output Settings
*/
@Generated("software.amazon.awssdk:codegen")
public final class FecOutputSettings implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField COLUMN_DEPTH_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("ColumnDepth").getter(getter(FecOutputSettings::columnDepth)).setter(setter(Builder::columnDepth))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("columnDepth").build()).build();
private static final SdkField INCLUDE_FEC_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("IncludeFec").getter(getter(FecOutputSettings::includeFecAsString)).setter(setter(Builder::includeFec))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("includeFec").build()).build();
private static final SdkField ROW_LENGTH_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("RowLength").getter(getter(FecOutputSettings::rowLength)).setter(setter(Builder::rowLength))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("rowLength").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(COLUMN_DEPTH_FIELD,
INCLUDE_FEC_FIELD, ROW_LENGTH_FIELD));
private static final long serialVersionUID = 1L;
private final Integer columnDepth;
private final String includeFec;
private final Integer rowLength;
private FecOutputSettings(BuilderImpl builder) {
this.columnDepth = builder.columnDepth;
this.includeFec = builder.includeFec;
this.rowLength = builder.rowLength;
}
/**
* Parameter D from SMPTE 2022-1. The height of the FEC protection matrix. The number of transport stream packets
* per column error correction packet. Must be between 4 and 20, inclusive.
*
* @return Parameter D from SMPTE 2022-1. The height of the FEC protection matrix. The number of transport stream
* packets per column error correction packet. Must be between 4 and 20, inclusive.
*/
public final Integer columnDepth() {
return columnDepth;
}
/**
* Enables column only or column and row based FEC
*
* If the service returns an enum value that is not available in the current SDK version, {@link #includeFec} will
* return {@link FecOutputIncludeFec#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #includeFecAsString}.
*
*
* @return Enables column only or column and row based FEC
* @see FecOutputIncludeFec
*/
public final FecOutputIncludeFec includeFec() {
return FecOutputIncludeFec.fromValue(includeFec);
}
/**
* Enables column only or column and row based FEC
*
* If the service returns an enum value that is not available in the current SDK version, {@link #includeFec} will
* return {@link FecOutputIncludeFec#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #includeFecAsString}.
*
*
* @return Enables column only or column and row based FEC
* @see FecOutputIncludeFec
*/
public final String includeFecAsString() {
return includeFec;
}
/**
* Parameter L from SMPTE 2022-1. The width of the FEC protection matrix. Must be between 1 and 20, inclusive. If
* only Column FEC is used, then larger values increase robustness. If Row FEC is used, then this is the number of
* transport stream packets per row error correction packet, and the value must be between 4 and 20, inclusive, if
* includeFec is columnAndRow. If includeFec is column, this value must be 1 to 20, inclusive.
*
* @return Parameter L from SMPTE 2022-1. The width of the FEC protection matrix. Must be between 1 and 20,
* inclusive. If only Column FEC is used, then larger values increase robustness. If Row FEC is used, then
* this is the number of transport stream packets per row error correction packet, and the value must be
* between 4 and 20, inclusive, if includeFec is columnAndRow. If includeFec is column, this value must be 1
* to 20, inclusive.
*/
public final Integer rowLength() {
return rowLength;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(columnDepth());
hashCode = 31 * hashCode + Objects.hashCode(includeFecAsString());
hashCode = 31 * hashCode + Objects.hashCode(rowLength());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof FecOutputSettings)) {
return false;
}
FecOutputSettings other = (FecOutputSettings) obj;
return Objects.equals(columnDepth(), other.columnDepth())
&& Objects.equals(includeFecAsString(), other.includeFecAsString())
&& Objects.equals(rowLength(), other.rowLength());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("FecOutputSettings").add("ColumnDepth", columnDepth()).add("IncludeFec", includeFecAsString())
.add("RowLength", rowLength()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ColumnDepth":
return Optional.ofNullable(clazz.cast(columnDepth()));
case "IncludeFec":
return Optional.ofNullable(clazz.cast(includeFecAsString()));
case "RowLength":
return Optional.ofNullable(clazz.cast(rowLength()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy