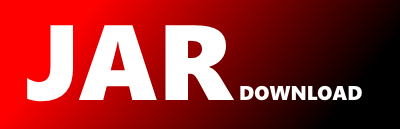
software.amazon.awssdk.services.mediapackage.MediaPackageClient Maven / Gradle / Ivy
Show all versions of mediapackage Show documentation
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.mediapackage;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.mediapackage.model.CreateChannelRequest;
import software.amazon.awssdk.services.mediapackage.model.CreateChannelResponse;
import software.amazon.awssdk.services.mediapackage.model.CreateOriginEndpointRequest;
import software.amazon.awssdk.services.mediapackage.model.CreateOriginEndpointResponse;
import software.amazon.awssdk.services.mediapackage.model.DeleteChannelRequest;
import software.amazon.awssdk.services.mediapackage.model.DeleteChannelResponse;
import software.amazon.awssdk.services.mediapackage.model.DeleteOriginEndpointRequest;
import software.amazon.awssdk.services.mediapackage.model.DeleteOriginEndpointResponse;
import software.amazon.awssdk.services.mediapackage.model.DescribeChannelRequest;
import software.amazon.awssdk.services.mediapackage.model.DescribeChannelResponse;
import software.amazon.awssdk.services.mediapackage.model.DescribeOriginEndpointRequest;
import software.amazon.awssdk.services.mediapackage.model.DescribeOriginEndpointResponse;
import software.amazon.awssdk.services.mediapackage.model.ForbiddenException;
import software.amazon.awssdk.services.mediapackage.model.InternalServerErrorException;
import software.amazon.awssdk.services.mediapackage.model.ListChannelsRequest;
import software.amazon.awssdk.services.mediapackage.model.ListChannelsResponse;
import software.amazon.awssdk.services.mediapackage.model.ListOriginEndpointsRequest;
import software.amazon.awssdk.services.mediapackage.model.ListOriginEndpointsResponse;
import software.amazon.awssdk.services.mediapackage.model.MediaPackageException;
import software.amazon.awssdk.services.mediapackage.model.NotFoundException;
import software.amazon.awssdk.services.mediapackage.model.RotateChannelCredentialsRequest;
import software.amazon.awssdk.services.mediapackage.model.RotateChannelCredentialsResponse;
import software.amazon.awssdk.services.mediapackage.model.ServiceUnavailableException;
import software.amazon.awssdk.services.mediapackage.model.TooManyRequestsException;
import software.amazon.awssdk.services.mediapackage.model.UnprocessableEntityException;
import software.amazon.awssdk.services.mediapackage.model.UpdateChannelRequest;
import software.amazon.awssdk.services.mediapackage.model.UpdateChannelResponse;
import software.amazon.awssdk.services.mediapackage.model.UpdateOriginEndpointRequest;
import software.amazon.awssdk.services.mediapackage.model.UpdateOriginEndpointResponse;
import software.amazon.awssdk.services.mediapackage.paginators.ListChannelsIterable;
import software.amazon.awssdk.services.mediapackage.paginators.ListOriginEndpointsIterable;
/**
* Service client for accessing MediaPackage. This can be created using the static {@link #builder()} method.
*
* AWS Elemental MediaPackage
*/
@Generated("software.amazon.awssdk:codegen")
public interface MediaPackageClient extends SdkClient {
String SERVICE_NAME = "mediapackage";
/**
* Create a {@link MediaPackageClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static MediaPackageClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link MediaPackageClient}.
*/
static MediaPackageClientBuilder builder() {
return new DefaultMediaPackageClientBuilder();
}
/**
* Creates a new Channel.
*
* @param createChannelRequest
* A new Channel configuration.
* @return Result of the CreateChannel operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.CreateChannel
* @see AWS API
* Documentation
*/
default CreateChannelResponse createChannel(CreateChannelRequest createChannelRequest) throws UnprocessableEntityException,
InternalServerErrorException, ForbiddenException, NotFoundException, ServiceUnavailableException,
TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
throw new UnsupportedOperationException();
}
/**
* Creates a new Channel.
*
* This is a convenience which creates an instance of the {@link CreateChannelRequest.Builder} avoiding the need to
* create one manually via {@link CreateChannelRequest#builder()}
*
*
* @param createChannelRequest
* A {@link Consumer} that will call methods on {@link CreateChannelRequest.Builder} to create a request. A
* new Channel configuration.
* @return Result of the CreateChannel operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.CreateChannel
* @see AWS API
* Documentation
*/
default CreateChannelResponse createChannel(Consumer createChannelRequest)
throws UnprocessableEntityException, InternalServerErrorException, ForbiddenException, NotFoundException,
ServiceUnavailableException, TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
return createChannel(CreateChannelRequest.builder().applyMutation(createChannelRequest).build());
}
/**
* Creates a new OriginEndpoint record.
*
* @param createOriginEndpointRequest
* Configuration parameters used to create a new OriginEndpoint.
* @return Result of the CreateOriginEndpoint operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.CreateOriginEndpoint
* @see AWS API Documentation
*/
default CreateOriginEndpointResponse createOriginEndpoint(CreateOriginEndpointRequest createOriginEndpointRequest)
throws UnprocessableEntityException, InternalServerErrorException, ForbiddenException, NotFoundException,
ServiceUnavailableException, TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
throw new UnsupportedOperationException();
}
/**
* Creates a new OriginEndpoint record.
*
* This is a convenience which creates an instance of the {@link CreateOriginEndpointRequest.Builder} avoiding the
* need to create one manually via {@link CreateOriginEndpointRequest#builder()}
*
*
* @param createOriginEndpointRequest
* A {@link Consumer} that will call methods on {@link CreateOriginEndpointRequest.Builder} to create a
* request. Configuration parameters used to create a new OriginEndpoint.
* @return Result of the CreateOriginEndpoint operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.CreateOriginEndpoint
* @see AWS API Documentation
*/
default CreateOriginEndpointResponse createOriginEndpoint(
Consumer createOriginEndpointRequest) throws UnprocessableEntityException,
InternalServerErrorException, ForbiddenException, NotFoundException, ServiceUnavailableException,
TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
return createOriginEndpoint(CreateOriginEndpointRequest.builder().applyMutation(createOriginEndpointRequest).build());
}
/**
* Deletes an existing Channel.
*
* @param deleteChannelRequest
* @return Result of the DeleteChannel operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.DeleteChannel
* @see AWS API
* Documentation
*/
default DeleteChannelResponse deleteChannel(DeleteChannelRequest deleteChannelRequest) throws UnprocessableEntityException,
InternalServerErrorException, ForbiddenException, NotFoundException, ServiceUnavailableException,
TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
throw new UnsupportedOperationException();
}
/**
* Deletes an existing Channel.
*
* This is a convenience which creates an instance of the {@link DeleteChannelRequest.Builder} avoiding the need to
* create one manually via {@link DeleteChannelRequest#builder()}
*
*
* @param deleteChannelRequest
* A {@link Consumer} that will call methods on {@link DeleteChannelRequest.Builder} to create a request.
* @return Result of the DeleteChannel operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.DeleteChannel
* @see AWS API
* Documentation
*/
default DeleteChannelResponse deleteChannel(Consumer deleteChannelRequest)
throws UnprocessableEntityException, InternalServerErrorException, ForbiddenException, NotFoundException,
ServiceUnavailableException, TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
return deleteChannel(DeleteChannelRequest.builder().applyMutation(deleteChannelRequest).build());
}
/**
* Deletes an existing OriginEndpoint.
*
* @param deleteOriginEndpointRequest
* @return Result of the DeleteOriginEndpoint operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.DeleteOriginEndpoint
* @see AWS API Documentation
*/
default DeleteOriginEndpointResponse deleteOriginEndpoint(DeleteOriginEndpointRequest deleteOriginEndpointRequest)
throws UnprocessableEntityException, InternalServerErrorException, ForbiddenException, NotFoundException,
ServiceUnavailableException, TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
throw new UnsupportedOperationException();
}
/**
* Deletes an existing OriginEndpoint.
*
* This is a convenience which creates an instance of the {@link DeleteOriginEndpointRequest.Builder} avoiding the
* need to create one manually via {@link DeleteOriginEndpointRequest#builder()}
*
*
* @param deleteOriginEndpointRequest
* A {@link Consumer} that will call methods on {@link DeleteOriginEndpointRequest.Builder} to create a
* request.
* @return Result of the DeleteOriginEndpoint operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.DeleteOriginEndpoint
* @see AWS API Documentation
*/
default DeleteOriginEndpointResponse deleteOriginEndpoint(
Consumer deleteOriginEndpointRequest) throws UnprocessableEntityException,
InternalServerErrorException, ForbiddenException, NotFoundException, ServiceUnavailableException,
TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
return deleteOriginEndpoint(DeleteOriginEndpointRequest.builder().applyMutation(deleteOriginEndpointRequest).build());
}
/**
* Gets details about a Channel.
*
* @param describeChannelRequest
* @return Result of the DescribeChannel operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.DescribeChannel
* @see AWS
* API Documentation
*/
default DescribeChannelResponse describeChannel(DescribeChannelRequest describeChannelRequest)
throws UnprocessableEntityException, InternalServerErrorException, ForbiddenException, NotFoundException,
ServiceUnavailableException, TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
throw new UnsupportedOperationException();
}
/**
* Gets details about a Channel.
*
* This is a convenience which creates an instance of the {@link DescribeChannelRequest.Builder} avoiding the need
* to create one manually via {@link DescribeChannelRequest#builder()}
*
*
* @param describeChannelRequest
* A {@link Consumer} that will call methods on {@link DescribeChannelRequest.Builder} to create a request.
* @return Result of the DescribeChannel operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.DescribeChannel
* @see AWS
* API Documentation
*/
default DescribeChannelResponse describeChannel(Consumer describeChannelRequest)
throws UnprocessableEntityException, InternalServerErrorException, ForbiddenException, NotFoundException,
ServiceUnavailableException, TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
return describeChannel(DescribeChannelRequest.builder().applyMutation(describeChannelRequest).build());
}
/**
* Gets details about an existing OriginEndpoint.
*
* @param describeOriginEndpointRequest
* @return Result of the DescribeOriginEndpoint operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.DescribeOriginEndpoint
* @see AWS API Documentation
*/
default DescribeOriginEndpointResponse describeOriginEndpoint(DescribeOriginEndpointRequest describeOriginEndpointRequest)
throws UnprocessableEntityException, InternalServerErrorException, ForbiddenException, NotFoundException,
ServiceUnavailableException, TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
throw new UnsupportedOperationException();
}
/**
* Gets details about an existing OriginEndpoint.
*
* This is a convenience which creates an instance of the {@link DescribeOriginEndpointRequest.Builder} avoiding the
* need to create one manually via {@link DescribeOriginEndpointRequest#builder()}
*
*
* @param describeOriginEndpointRequest
* A {@link Consumer} that will call methods on {@link DescribeOriginEndpointRequest.Builder} to create a
* request.
* @return Result of the DescribeOriginEndpoint operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.DescribeOriginEndpoint
* @see AWS API Documentation
*/
default DescribeOriginEndpointResponse describeOriginEndpoint(
Consumer describeOriginEndpointRequest) throws UnprocessableEntityException,
InternalServerErrorException, ForbiddenException, NotFoundException, ServiceUnavailableException,
TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
return describeOriginEndpoint(DescribeOriginEndpointRequest.builder().applyMutation(describeOriginEndpointRequest)
.build());
}
/**
* Returns a collection of Channels.
*
* @return Result of the ListChannels operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.ListChannels
* @see #listChannels(ListChannelsRequest)
* @see AWS API
* Documentation
*/
default ListChannelsResponse listChannels() throws UnprocessableEntityException, InternalServerErrorException,
ForbiddenException, NotFoundException, ServiceUnavailableException, TooManyRequestsException, AwsServiceException,
SdkClientException, MediaPackageException {
return listChannels(ListChannelsRequest.builder().build());
}
/**
* Returns a collection of Channels.
*
* @param listChannelsRequest
* @return Result of the ListChannels operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.ListChannels
* @see AWS API
* Documentation
*/
default ListChannelsResponse listChannels(ListChannelsRequest listChannelsRequest) throws UnprocessableEntityException,
InternalServerErrorException, ForbiddenException, NotFoundException, ServiceUnavailableException,
TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
throw new UnsupportedOperationException();
}
/**
* Returns a collection of Channels.
*
* This is a convenience which creates an instance of the {@link ListChannelsRequest.Builder} avoiding the need to
* create one manually via {@link ListChannelsRequest#builder()}
*
*
* @param listChannelsRequest
* A {@link Consumer} that will call methods on {@link ListChannelsRequest.Builder} to create a request.
* @return Result of the ListChannels operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.ListChannels
* @see AWS API
* Documentation
*/
default ListChannelsResponse listChannels(Consumer listChannelsRequest)
throws UnprocessableEntityException, InternalServerErrorException, ForbiddenException, NotFoundException,
ServiceUnavailableException, TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
return listChannels(ListChannelsRequest.builder().applyMutation(listChannelsRequest).build());
}
/**
* Returns a collection of Channels.
*
* This is a variant of
* {@link #listChannels(software.amazon.awssdk.services.mediapackage.model.ListChannelsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.mediapackage.paginators.ListChannelsIterable responses = client.listChannelsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.mediapackage.paginators.ListChannelsIterable responses = client
* .listChannelsPaginator(request);
* for (software.amazon.awssdk.services.mediapackage.model.ListChannelsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.mediapackage.paginators.ListChannelsIterable responses = client.listChannelsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listChannels(software.amazon.awssdk.services.mediapackage.model.ListChannelsRequest)} operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.ListChannels
* @see #listChannelsPaginator(ListChannelsRequest)
* @see AWS API
* Documentation
*/
default ListChannelsIterable listChannelsPaginator() throws UnprocessableEntityException, InternalServerErrorException,
ForbiddenException, NotFoundException, ServiceUnavailableException, TooManyRequestsException, AwsServiceException,
SdkClientException, MediaPackageException {
return listChannelsPaginator(ListChannelsRequest.builder().build());
}
/**
* Returns a collection of Channels.
*
* This is a variant of
* {@link #listChannels(software.amazon.awssdk.services.mediapackage.model.ListChannelsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.mediapackage.paginators.ListChannelsIterable responses = client.listChannelsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.mediapackage.paginators.ListChannelsIterable responses = client
* .listChannelsPaginator(request);
* for (software.amazon.awssdk.services.mediapackage.model.ListChannelsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.mediapackage.paginators.ListChannelsIterable responses = client.listChannelsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listChannels(software.amazon.awssdk.services.mediapackage.model.ListChannelsRequest)} operation.
*
*
* @param listChannelsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.ListChannels
* @see AWS API
* Documentation
*/
default ListChannelsIterable listChannelsPaginator(ListChannelsRequest listChannelsRequest)
throws UnprocessableEntityException, InternalServerErrorException, ForbiddenException, NotFoundException,
ServiceUnavailableException, TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
throw new UnsupportedOperationException();
}
/**
* Returns a collection of Channels.
*
* This is a variant of
* {@link #listChannels(software.amazon.awssdk.services.mediapackage.model.ListChannelsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.mediapackage.paginators.ListChannelsIterable responses = client.listChannelsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.mediapackage.paginators.ListChannelsIterable responses = client
* .listChannelsPaginator(request);
* for (software.amazon.awssdk.services.mediapackage.model.ListChannelsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.mediapackage.paginators.ListChannelsIterable responses = client.listChannelsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listChannels(software.amazon.awssdk.services.mediapackage.model.ListChannelsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListChannelsRequest.Builder} avoiding the need to
* create one manually via {@link ListChannelsRequest#builder()}
*
*
* @param listChannelsRequest
* A {@link Consumer} that will call methods on {@link ListChannelsRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.ListChannels
* @see AWS API
* Documentation
*/
default ListChannelsIterable listChannelsPaginator(Consumer listChannelsRequest)
throws UnprocessableEntityException, InternalServerErrorException, ForbiddenException, NotFoundException,
ServiceUnavailableException, TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
return listChannelsPaginator(ListChannelsRequest.builder().applyMutation(listChannelsRequest).build());
}
/**
* Returns a collection of OriginEndpoint records.
*
* @return Result of the ListOriginEndpoints operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.ListOriginEndpoints
* @see #listOriginEndpoints(ListOriginEndpointsRequest)
* @see AWS API Documentation
*/
default ListOriginEndpointsResponse listOriginEndpoints() throws UnprocessableEntityException, InternalServerErrorException,
ForbiddenException, NotFoundException, ServiceUnavailableException, TooManyRequestsException, AwsServiceException,
SdkClientException, MediaPackageException {
return listOriginEndpoints(ListOriginEndpointsRequest.builder().build());
}
/**
* Returns a collection of OriginEndpoint records.
*
* @param listOriginEndpointsRequest
* @return Result of the ListOriginEndpoints operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.ListOriginEndpoints
* @see AWS API Documentation
*/
default ListOriginEndpointsResponse listOriginEndpoints(ListOriginEndpointsRequest listOriginEndpointsRequest)
throws UnprocessableEntityException, InternalServerErrorException, ForbiddenException, NotFoundException,
ServiceUnavailableException, TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
throw new UnsupportedOperationException();
}
/**
* Returns a collection of OriginEndpoint records.
*
* This is a convenience which creates an instance of the {@link ListOriginEndpointsRequest.Builder} avoiding the
* need to create one manually via {@link ListOriginEndpointsRequest#builder()}
*
*
* @param listOriginEndpointsRequest
* A {@link Consumer} that will call methods on {@link ListOriginEndpointsRequest.Builder} to create a
* request.
* @return Result of the ListOriginEndpoints operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.ListOriginEndpoints
* @see AWS API Documentation
*/
default ListOriginEndpointsResponse listOriginEndpoints(
Consumer listOriginEndpointsRequest) throws UnprocessableEntityException,
InternalServerErrorException, ForbiddenException, NotFoundException, ServiceUnavailableException,
TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
return listOriginEndpoints(ListOriginEndpointsRequest.builder().applyMutation(listOriginEndpointsRequest).build());
}
/**
* Returns a collection of OriginEndpoint records.
*
* This is a variant of
* {@link #listOriginEndpoints(software.amazon.awssdk.services.mediapackage.model.ListOriginEndpointsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.mediapackage.paginators.ListOriginEndpointsIterable responses = client.listOriginEndpointsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.mediapackage.paginators.ListOriginEndpointsIterable responses = client
* .listOriginEndpointsPaginator(request);
* for (software.amazon.awssdk.services.mediapackage.model.ListOriginEndpointsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.mediapackage.paginators.ListOriginEndpointsIterable responses = client.listOriginEndpointsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listOriginEndpoints(software.amazon.awssdk.services.mediapackage.model.ListOriginEndpointsRequest)}
* operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.ListOriginEndpoints
* @see #listOriginEndpointsPaginator(ListOriginEndpointsRequest)
* @see AWS API Documentation
*/
default ListOriginEndpointsIterable listOriginEndpointsPaginator() throws UnprocessableEntityException,
InternalServerErrorException, ForbiddenException, NotFoundException, ServiceUnavailableException,
TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
return listOriginEndpointsPaginator(ListOriginEndpointsRequest.builder().build());
}
/**
* Returns a collection of OriginEndpoint records.
*
* This is a variant of
* {@link #listOriginEndpoints(software.amazon.awssdk.services.mediapackage.model.ListOriginEndpointsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.mediapackage.paginators.ListOriginEndpointsIterable responses = client.listOriginEndpointsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.mediapackage.paginators.ListOriginEndpointsIterable responses = client
* .listOriginEndpointsPaginator(request);
* for (software.amazon.awssdk.services.mediapackage.model.ListOriginEndpointsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.mediapackage.paginators.ListOriginEndpointsIterable responses = client.listOriginEndpointsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listOriginEndpoints(software.amazon.awssdk.services.mediapackage.model.ListOriginEndpointsRequest)}
* operation.
*
*
* @param listOriginEndpointsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.ListOriginEndpoints
* @see AWS API Documentation
*/
default ListOriginEndpointsIterable listOriginEndpointsPaginator(ListOriginEndpointsRequest listOriginEndpointsRequest)
throws UnprocessableEntityException, InternalServerErrorException, ForbiddenException, NotFoundException,
ServiceUnavailableException, TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
throw new UnsupportedOperationException();
}
/**
* Returns a collection of OriginEndpoint records.
*
* This is a variant of
* {@link #listOriginEndpoints(software.amazon.awssdk.services.mediapackage.model.ListOriginEndpointsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.mediapackage.paginators.ListOriginEndpointsIterable responses = client.listOriginEndpointsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.mediapackage.paginators.ListOriginEndpointsIterable responses = client
* .listOriginEndpointsPaginator(request);
* for (software.amazon.awssdk.services.mediapackage.model.ListOriginEndpointsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.mediapackage.paginators.ListOriginEndpointsIterable responses = client.listOriginEndpointsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listOriginEndpoints(software.amazon.awssdk.services.mediapackage.model.ListOriginEndpointsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListOriginEndpointsRequest.Builder} avoiding the
* need to create one manually via {@link ListOriginEndpointsRequest#builder()}
*
*
* @param listOriginEndpointsRequest
* A {@link Consumer} that will call methods on {@link ListOriginEndpointsRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.ListOriginEndpoints
* @see AWS API Documentation
*/
default ListOriginEndpointsIterable listOriginEndpointsPaginator(
Consumer listOriginEndpointsRequest) throws UnprocessableEntityException,
InternalServerErrorException, ForbiddenException, NotFoundException, ServiceUnavailableException,
TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
return listOriginEndpointsPaginator(ListOriginEndpointsRequest.builder().applyMutation(listOriginEndpointsRequest)
.build());
}
/**
* Changes the Channel ingest username and password.
*
* @param rotateChannelCredentialsRequest
* @return Result of the RotateChannelCredentials operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.RotateChannelCredentials
* @see AWS API Documentation
*/
default RotateChannelCredentialsResponse rotateChannelCredentials(
RotateChannelCredentialsRequest rotateChannelCredentialsRequest) throws UnprocessableEntityException,
InternalServerErrorException, ForbiddenException, NotFoundException, ServiceUnavailableException,
TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
throw new UnsupportedOperationException();
}
/**
* Changes the Channel ingest username and password.
*
* This is a convenience which creates an instance of the {@link RotateChannelCredentialsRequest.Builder} avoiding
* the need to create one manually via {@link RotateChannelCredentialsRequest#builder()}
*
*
* @param rotateChannelCredentialsRequest
* A {@link Consumer} that will call methods on {@link RotateChannelCredentialsRequest.Builder} to create a
* request.
* @return Result of the RotateChannelCredentials operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.RotateChannelCredentials
* @see AWS API Documentation
*/
default RotateChannelCredentialsResponse rotateChannelCredentials(
Consumer rotateChannelCredentialsRequest)
throws UnprocessableEntityException, InternalServerErrorException, ForbiddenException, NotFoundException,
ServiceUnavailableException, TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
return rotateChannelCredentials(RotateChannelCredentialsRequest.builder().applyMutation(rotateChannelCredentialsRequest)
.build());
}
/**
* Updates an existing Channel.
*
* @param updateChannelRequest
* Configuration parameters used to update the Channel.
* @return Result of the UpdateChannel operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.UpdateChannel
* @see AWS API
* Documentation
*/
default UpdateChannelResponse updateChannel(UpdateChannelRequest updateChannelRequest) throws UnprocessableEntityException,
InternalServerErrorException, ForbiddenException, NotFoundException, ServiceUnavailableException,
TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
throw new UnsupportedOperationException();
}
/**
* Updates an existing Channel.
*
* This is a convenience which creates an instance of the {@link UpdateChannelRequest.Builder} avoiding the need to
* create one manually via {@link UpdateChannelRequest#builder()}
*
*
* @param updateChannelRequest
* A {@link Consumer} that will call methods on {@link UpdateChannelRequest.Builder} to create a request.
* Configuration parameters used to update the Channel.
* @return Result of the UpdateChannel operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.UpdateChannel
* @see AWS API
* Documentation
*/
default UpdateChannelResponse updateChannel(Consumer updateChannelRequest)
throws UnprocessableEntityException, InternalServerErrorException, ForbiddenException, NotFoundException,
ServiceUnavailableException, TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
return updateChannel(UpdateChannelRequest.builder().applyMutation(updateChannelRequest).build());
}
/**
* Updates an existing OriginEndpoint.
*
* @param updateOriginEndpointRequest
* Configuration parameters used to update an existing OriginEndpoint.
* @return Result of the UpdateOriginEndpoint operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.UpdateOriginEndpoint
* @see AWS API Documentation
*/
default UpdateOriginEndpointResponse updateOriginEndpoint(UpdateOriginEndpointRequest updateOriginEndpointRequest)
throws UnprocessableEntityException, InternalServerErrorException, ForbiddenException, NotFoundException,
ServiceUnavailableException, TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
throw new UnsupportedOperationException();
}
/**
* Updates an existing OriginEndpoint.
*
* This is a convenience which creates an instance of the {@link UpdateOriginEndpointRequest.Builder} avoiding the
* need to create one manually via {@link UpdateOriginEndpointRequest#builder()}
*
*
* @param updateOriginEndpointRequest
* A {@link Consumer} that will call methods on {@link UpdateOriginEndpointRequest.Builder} to create a
* request. Configuration parameters used to update an existing OriginEndpoint.
* @return Result of the UpdateOriginEndpoint operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.UpdateOriginEndpoint
* @see AWS API Documentation
*/
default UpdateOriginEndpointResponse updateOriginEndpoint(
Consumer updateOriginEndpointRequest) throws UnprocessableEntityException,
InternalServerErrorException, ForbiddenException, NotFoundException, ServiceUnavailableException,
TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
return updateOriginEndpoint(UpdateOriginEndpointRequest.builder().applyMutation(updateOriginEndpointRequest).build());
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of("mediapackage");
}
}