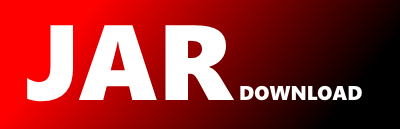
software.amazon.awssdk.services.mediapackage.model.CreateOriginEndpointResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mediapackage Show documentation
Show all versions of mediapackage Show documentation
The AWS Java SDK for AWS Elemental MediaPackage module holds the client classes that are used for
communicating
with AWS Elemental MediaPackage Service
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.mediapackage.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateOriginEndpointResponse extends MediaPackageResponse implements
ToCopyableBuilder {
private final String arn;
private final String channelId;
private final DashPackage dashPackage;
private final String description;
private final HlsPackage hlsPackage;
private final String id;
private final String manifestName;
private final MssPackage mssPackage;
private final Integer startoverWindowSeconds;
private final Integer timeDelaySeconds;
private final String url;
private final List whitelist;
private CreateOriginEndpointResponse(BuilderImpl builder) {
super(builder);
this.arn = builder.arn;
this.channelId = builder.channelId;
this.dashPackage = builder.dashPackage;
this.description = builder.description;
this.hlsPackage = builder.hlsPackage;
this.id = builder.id;
this.manifestName = builder.manifestName;
this.mssPackage = builder.mssPackage;
this.startoverWindowSeconds = builder.startoverWindowSeconds;
this.timeDelaySeconds = builder.timeDelaySeconds;
this.url = builder.url;
this.whitelist = builder.whitelist;
}
/**
* The Amazon Resource Name (ARN) assigned to the OriginEndpoint.
*
* @return The Amazon Resource Name (ARN) assigned to the OriginEndpoint.
*/
public String arn() {
return arn;
}
/**
* The ID of the Channel the OriginEndpoint is associated with.
*
* @return The ID of the Channel the OriginEndpoint is associated with.
*/
public String channelId() {
return channelId;
}
/**
* Returns the value of the DashPackage property for this object.
*
* @return The value of the DashPackage property for this object.
*/
public DashPackage dashPackage() {
return dashPackage;
}
/**
* A short text description of the OriginEndpoint.
*
* @return A short text description of the OriginEndpoint.
*/
public String description() {
return description;
}
/**
* Returns the value of the HlsPackage property for this object.
*
* @return The value of the HlsPackage property for this object.
*/
public HlsPackage hlsPackage() {
return hlsPackage;
}
/**
* The ID of the OriginEndpoint.
*
* @return The ID of the OriginEndpoint.
*/
public String id() {
return id;
}
/**
* A short string appended to the end of the OriginEndpoint URL.
*
* @return A short string appended to the end of the OriginEndpoint URL.
*/
public String manifestName() {
return manifestName;
}
/**
* Returns the value of the MssPackage property for this object.
*
* @return The value of the MssPackage property for this object.
*/
public MssPackage mssPackage() {
return mssPackage;
}
/**
* Maximum duration (seconds) of content to retain for startover playback. If not specified, startover playback will
* be disabled for the OriginEndpoint.
*
* @return Maximum duration (seconds) of content to retain for startover playback. If not specified, startover
* playback will be disabled for the OriginEndpoint.
*/
public Integer startoverWindowSeconds() {
return startoverWindowSeconds;
}
/**
* Amount of delay (seconds) to enforce on the playback of live content. If not specified, there will be no time
* delay in effect for the OriginEndpoint.
*
* @return Amount of delay (seconds) to enforce on the playback of live content. If not specified, there will be no
* time delay in effect for the OriginEndpoint.
*/
public Integer timeDelaySeconds() {
return timeDelaySeconds;
}
/**
* The URL of the packaged OriginEndpoint for consumption.
*
* @return The URL of the packaged OriginEndpoint for consumption.
*/
public String url() {
return url;
}
/**
* A list of source IP CIDR blocks that will be allowed to access the OriginEndpoint.
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return A list of source IP CIDR blocks that will be allowed to access the OriginEndpoint.
*/
public List whitelist() {
return whitelist;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(channelId());
hashCode = 31 * hashCode + Objects.hashCode(dashPackage());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(hlsPackage());
hashCode = 31 * hashCode + Objects.hashCode(id());
hashCode = 31 * hashCode + Objects.hashCode(manifestName());
hashCode = 31 * hashCode + Objects.hashCode(mssPackage());
hashCode = 31 * hashCode + Objects.hashCode(startoverWindowSeconds());
hashCode = 31 * hashCode + Objects.hashCode(timeDelaySeconds());
hashCode = 31 * hashCode + Objects.hashCode(url());
hashCode = 31 * hashCode + Objects.hashCode(whitelist());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateOriginEndpointResponse)) {
return false;
}
CreateOriginEndpointResponse other = (CreateOriginEndpointResponse) obj;
return Objects.equals(arn(), other.arn()) && Objects.equals(channelId(), other.channelId())
&& Objects.equals(dashPackage(), other.dashPackage()) && Objects.equals(description(), other.description())
&& Objects.equals(hlsPackage(), other.hlsPackage()) && Objects.equals(id(), other.id())
&& Objects.equals(manifestName(), other.manifestName()) && Objects.equals(mssPackage(), other.mssPackage())
&& Objects.equals(startoverWindowSeconds(), other.startoverWindowSeconds())
&& Objects.equals(timeDelaySeconds(), other.timeDelaySeconds()) && Objects.equals(url(), other.url())
&& Objects.equals(whitelist(), other.whitelist());
}
@Override
public String toString() {
return ToString.builder("CreateOriginEndpointResponse").add("Arn", arn()).add("ChannelId", channelId())
.add("DashPackage", dashPackage()).add("Description", description()).add("HlsPackage", hlsPackage())
.add("Id", id()).add("ManifestName", manifestName()).add("MssPackage", mssPackage())
.add("StartoverWindowSeconds", startoverWindowSeconds()).add("TimeDelaySeconds", timeDelaySeconds())
.add("Url", url()).add("Whitelist", whitelist()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Arn":
return Optional.ofNullable(clazz.cast(arn()));
case "ChannelId":
return Optional.ofNullable(clazz.cast(channelId()));
case "DashPackage":
return Optional.ofNullable(clazz.cast(dashPackage()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "HlsPackage":
return Optional.ofNullable(clazz.cast(hlsPackage()));
case "Id":
return Optional.ofNullable(clazz.cast(id()));
case "ManifestName":
return Optional.ofNullable(clazz.cast(manifestName()));
case "MssPackage":
return Optional.ofNullable(clazz.cast(mssPackage()));
case "StartoverWindowSeconds":
return Optional.ofNullable(clazz.cast(startoverWindowSeconds()));
case "TimeDelaySeconds":
return Optional.ofNullable(clazz.cast(timeDelaySeconds()));
case "Url":
return Optional.ofNullable(clazz.cast(url()));
case "Whitelist":
return Optional.ofNullable(clazz.cast(whitelist()));
default:
return Optional.empty();
}
}
public interface Builder extends MediaPackageResponse.Builder, CopyableBuilder {
/**
* The Amazon Resource Name (ARN) assigned to the OriginEndpoint.
*
* @param arn
* The Amazon Resource Name (ARN) assigned to the OriginEndpoint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder arn(String arn);
/**
* The ID of the Channel the OriginEndpoint is associated with.
*
* @param channelId
* The ID of the Channel the OriginEndpoint is associated with.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder channelId(String channelId);
/**
* Sets the value of the DashPackage property for this object.
*
* @param dashPackage
* The new value for the DashPackage property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder dashPackage(DashPackage dashPackage);
/**
* Sets the value of the DashPackage property for this object.
*
* This is a convenience that creates an instance of the {@link DashPackage.Builder} avoiding the need to create
* one manually via {@link DashPackage#builder()}.
*
* When the {@link Consumer} completes, {@link DashPackage.Builder#build()} is called immediately and its result
* is passed to {@link #dashPackage(DashPackage)}.
*
* @param dashPackage
* a consumer that will call methods on {@link DashPackage.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #dashPackage(DashPackage)
*/
default Builder dashPackage(Consumer dashPackage) {
return dashPackage(DashPackage.builder().applyMutation(dashPackage).build());
}
/**
* A short text description of the OriginEndpoint.
*
* @param description
* A short text description of the OriginEndpoint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder description(String description);
/**
* Sets the value of the HlsPackage property for this object.
*
* @param hlsPackage
* The new value for the HlsPackage property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder hlsPackage(HlsPackage hlsPackage);
/**
* Sets the value of the HlsPackage property for this object.
*
* This is a convenience that creates an instance of the {@link HlsPackage.Builder} avoiding the need to create
* one manually via {@link HlsPackage#builder()}.
*
* When the {@link Consumer} completes, {@link HlsPackage.Builder#build()} is called immediately and its result
* is passed to {@link #hlsPackage(HlsPackage)}.
*
* @param hlsPackage
* a consumer that will call methods on {@link HlsPackage.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #hlsPackage(HlsPackage)
*/
default Builder hlsPackage(Consumer hlsPackage) {
return hlsPackage(HlsPackage.builder().applyMutation(hlsPackage).build());
}
/**
* The ID of the OriginEndpoint.
*
* @param id
* The ID of the OriginEndpoint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder id(String id);
/**
* A short string appended to the end of the OriginEndpoint URL.
*
* @param manifestName
* A short string appended to the end of the OriginEndpoint URL.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder manifestName(String manifestName);
/**
* Sets the value of the MssPackage property for this object.
*
* @param mssPackage
* The new value for the MssPackage property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder mssPackage(MssPackage mssPackage);
/**
* Sets the value of the MssPackage property for this object.
*
* This is a convenience that creates an instance of the {@link MssPackage.Builder} avoiding the need to create
* one manually via {@link MssPackage#builder()}.
*
* When the {@link Consumer} completes, {@link MssPackage.Builder#build()} is called immediately and its result
* is passed to {@link #mssPackage(MssPackage)}.
*
* @param mssPackage
* a consumer that will call methods on {@link MssPackage.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #mssPackage(MssPackage)
*/
default Builder mssPackage(Consumer mssPackage) {
return mssPackage(MssPackage.builder().applyMutation(mssPackage).build());
}
/**
* Maximum duration (seconds) of content to retain for startover playback. If not specified, startover playback
* will be disabled for the OriginEndpoint.
*
* @param startoverWindowSeconds
* Maximum duration (seconds) of content to retain for startover playback. If not specified, startover
* playback will be disabled for the OriginEndpoint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder startoverWindowSeconds(Integer startoverWindowSeconds);
/**
* Amount of delay (seconds) to enforce on the playback of live content. If not specified, there will be no time
* delay in effect for the OriginEndpoint.
*
* @param timeDelaySeconds
* Amount of delay (seconds) to enforce on the playback of live content. If not specified, there will be
* no time delay in effect for the OriginEndpoint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder timeDelaySeconds(Integer timeDelaySeconds);
/**
* The URL of the packaged OriginEndpoint for consumption.
*
* @param url
* The URL of the packaged OriginEndpoint for consumption.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder url(String url);
/**
* A list of source IP CIDR blocks that will be allowed to access the OriginEndpoint.
*
* @param whitelist
* A list of source IP CIDR blocks that will be allowed to access the OriginEndpoint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder whitelist(Collection whitelist);
/**
* A list of source IP CIDR blocks that will be allowed to access the OriginEndpoint.
*
* @param whitelist
* A list of source IP CIDR blocks that will be allowed to access the OriginEndpoint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder whitelist(String... whitelist);
}
static final class BuilderImpl extends MediaPackageResponse.BuilderImpl implements Builder {
private String arn;
private String channelId;
private DashPackage dashPackage;
private String description;
private HlsPackage hlsPackage;
private String id;
private String manifestName;
private MssPackage mssPackage;
private Integer startoverWindowSeconds;
private Integer timeDelaySeconds;
private String url;
private List whitelist = DefaultSdkAutoConstructList.getInstance();
private BuilderImpl() {
}
private BuilderImpl(CreateOriginEndpointResponse model) {
super(model);
arn(model.arn);
channelId(model.channelId);
dashPackage(model.dashPackage);
description(model.description);
hlsPackage(model.hlsPackage);
id(model.id);
manifestName(model.manifestName);
mssPackage(model.mssPackage);
startoverWindowSeconds(model.startoverWindowSeconds);
timeDelaySeconds(model.timeDelaySeconds);
url(model.url);
whitelist(model.whitelist);
}
public final String getArn() {
return arn;
}
@Override
public final Builder arn(String arn) {
this.arn = arn;
return this;
}
public final void setArn(String arn) {
this.arn = arn;
}
public final String getChannelId() {
return channelId;
}
@Override
public final Builder channelId(String channelId) {
this.channelId = channelId;
return this;
}
public final void setChannelId(String channelId) {
this.channelId = channelId;
}
public final DashPackage.Builder getDashPackage() {
return dashPackage != null ? dashPackage.toBuilder() : null;
}
@Override
public final Builder dashPackage(DashPackage dashPackage) {
this.dashPackage = dashPackage;
return this;
}
public final void setDashPackage(DashPackage.BuilderImpl dashPackage) {
this.dashPackage = dashPackage != null ? dashPackage.build() : null;
}
public final String getDescription() {
return description;
}
@Override
public final Builder description(String description) {
this.description = description;
return this;
}
public final void setDescription(String description) {
this.description = description;
}
public final HlsPackage.Builder getHlsPackage() {
return hlsPackage != null ? hlsPackage.toBuilder() : null;
}
@Override
public final Builder hlsPackage(HlsPackage hlsPackage) {
this.hlsPackage = hlsPackage;
return this;
}
public final void setHlsPackage(HlsPackage.BuilderImpl hlsPackage) {
this.hlsPackage = hlsPackage != null ? hlsPackage.build() : null;
}
public final String getId() {
return id;
}
@Override
public final Builder id(String id) {
this.id = id;
return this;
}
public final void setId(String id) {
this.id = id;
}
public final String getManifestName() {
return manifestName;
}
@Override
public final Builder manifestName(String manifestName) {
this.manifestName = manifestName;
return this;
}
public final void setManifestName(String manifestName) {
this.manifestName = manifestName;
}
public final MssPackage.Builder getMssPackage() {
return mssPackage != null ? mssPackage.toBuilder() : null;
}
@Override
public final Builder mssPackage(MssPackage mssPackage) {
this.mssPackage = mssPackage;
return this;
}
public final void setMssPackage(MssPackage.BuilderImpl mssPackage) {
this.mssPackage = mssPackage != null ? mssPackage.build() : null;
}
public final Integer getStartoverWindowSeconds() {
return startoverWindowSeconds;
}
@Override
public final Builder startoverWindowSeconds(Integer startoverWindowSeconds) {
this.startoverWindowSeconds = startoverWindowSeconds;
return this;
}
public final void setStartoverWindowSeconds(Integer startoverWindowSeconds) {
this.startoverWindowSeconds = startoverWindowSeconds;
}
public final Integer getTimeDelaySeconds() {
return timeDelaySeconds;
}
@Override
public final Builder timeDelaySeconds(Integer timeDelaySeconds) {
this.timeDelaySeconds = timeDelaySeconds;
return this;
}
public final void setTimeDelaySeconds(Integer timeDelaySeconds) {
this.timeDelaySeconds = timeDelaySeconds;
}
public final String getUrl() {
return url;
}
@Override
public final Builder url(String url) {
this.url = url;
return this;
}
public final void setUrl(String url) {
this.url = url;
}
public final Collection getWhitelist() {
return whitelist;
}
@Override
public final Builder whitelist(Collection whitelist) {
this.whitelist = ListOf__stringCopier.copy(whitelist);
return this;
}
@Override
@SafeVarargs
public final Builder whitelist(String... whitelist) {
whitelist(Arrays.asList(whitelist));
return this;
}
public final void setWhitelist(Collection whitelist) {
this.whitelist = ListOf__stringCopier.copy(whitelist);
}
@Override
public CreateOriginEndpointResponse build() {
return new CreateOriginEndpointResponse(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy