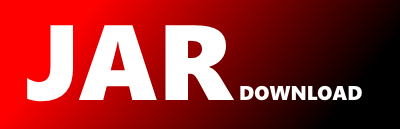
software.amazon.awssdk.services.mediapackage.model.UpdateChannelResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mediapackage Show documentation
Show all versions of mediapackage Show documentation
The AWS Java SDK for AWS Elemental MediaPackage module holds the client classes that are used for
communicating
with AWS Elemental MediaPackage Service
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.mediapackage.model;
import java.util.Objects;
import java.util.Optional;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class UpdateChannelResponse extends MediaPackageResponse implements
ToCopyableBuilder {
private final String arn;
private final String description;
private final HlsIngest hlsIngest;
private final String id;
private UpdateChannelResponse(BuilderImpl builder) {
super(builder);
this.arn = builder.arn;
this.description = builder.description;
this.hlsIngest = builder.hlsIngest;
this.id = builder.id;
}
/**
* The Amazon Resource Name (ARN) assigned to the Channel.
*
* @return The Amazon Resource Name (ARN) assigned to the Channel.
*/
public String arn() {
return arn;
}
/**
* A short text description of the Channel.
*
* @return A short text description of the Channel.
*/
public String description() {
return description;
}
/**
* Returns the value of the HlsIngest property for this object.
*
* @return The value of the HlsIngest property for this object.
*/
public HlsIngest hlsIngest() {
return hlsIngest;
}
/**
* The ID of the Channel.
*
* @return The ID of the Channel.
*/
public String id() {
return id;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(hlsIngest());
hashCode = 31 * hashCode + Objects.hashCode(id());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UpdateChannelResponse)) {
return false;
}
UpdateChannelResponse other = (UpdateChannelResponse) obj;
return Objects.equals(arn(), other.arn()) && Objects.equals(description(), other.description())
&& Objects.equals(hlsIngest(), other.hlsIngest()) && Objects.equals(id(), other.id());
}
@Override
public String toString() {
return ToString.builder("UpdateChannelResponse").add("Arn", arn()).add("Description", description())
.add("HlsIngest", hlsIngest()).add("Id", id()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Arn":
return Optional.ofNullable(clazz.cast(arn()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "HlsIngest":
return Optional.ofNullable(clazz.cast(hlsIngest()));
case "Id":
return Optional.ofNullable(clazz.cast(id()));
default:
return Optional.empty();
}
}
public interface Builder extends MediaPackageResponse.Builder, CopyableBuilder {
/**
* The Amazon Resource Name (ARN) assigned to the Channel.
*
* @param arn
* The Amazon Resource Name (ARN) assigned to the Channel.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder arn(String arn);
/**
* A short text description of the Channel.
*
* @param description
* A short text description of the Channel.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder description(String description);
/**
* Sets the value of the HlsIngest property for this object.
*
* @param hlsIngest
* The new value for the HlsIngest property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder hlsIngest(HlsIngest hlsIngest);
/**
* Sets the value of the HlsIngest property for this object.
*
* This is a convenience that creates an instance of the {@link HlsIngest.Builder} avoiding the need to create
* one manually via {@link HlsIngest#builder()}.
*
* When the {@link Consumer} completes, {@link HlsIngest.Builder#build()} is called immediately and its result
* is passed to {@link #hlsIngest(HlsIngest)}.
*
* @param hlsIngest
* a consumer that will call methods on {@link HlsIngest.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #hlsIngest(HlsIngest)
*/
default Builder hlsIngest(Consumer hlsIngest) {
return hlsIngest(HlsIngest.builder().applyMutation(hlsIngest).build());
}
/**
* The ID of the Channel.
*
* @param id
* The ID of the Channel.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder id(String id);
}
static final class BuilderImpl extends MediaPackageResponse.BuilderImpl implements Builder {
private String arn;
private String description;
private HlsIngest hlsIngest;
private String id;
private BuilderImpl() {
}
private BuilderImpl(UpdateChannelResponse model) {
super(model);
arn(model.arn);
description(model.description);
hlsIngest(model.hlsIngest);
id(model.id);
}
public final String getArn() {
return arn;
}
@Override
public final Builder arn(String arn) {
this.arn = arn;
return this;
}
public final void setArn(String arn) {
this.arn = arn;
}
public final String getDescription() {
return description;
}
@Override
public final Builder description(String description) {
this.description = description;
return this;
}
public final void setDescription(String description) {
this.description = description;
}
public final HlsIngest.Builder getHlsIngest() {
return hlsIngest != null ? hlsIngest.toBuilder() : null;
}
@Override
public final Builder hlsIngest(HlsIngest hlsIngest) {
this.hlsIngest = hlsIngest;
return this;
}
public final void setHlsIngest(HlsIngest.BuilderImpl hlsIngest) {
this.hlsIngest = hlsIngest != null ? hlsIngest.build() : null;
}
public final String getId() {
return id;
}
@Override
public final Builder id(String id) {
this.id = id;
return this;
}
public final void setId(String id) {
this.id = id;
}
@Override
public UpdateChannelResponse build() {
return new UpdateChannelResponse(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy