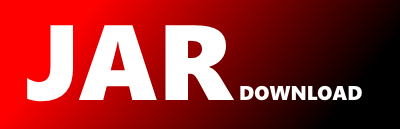
software.amazon.awssdk.services.mediapackage.DefaultMediaPackageClient Maven / Gradle / Ivy
Show all versions of mediapackage Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.mediapackage;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.awscore.client.handler.AwsSyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.ApiName;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.client.handler.SyncClientHandler;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.util.VersionInfo;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.services.mediapackage.model.CreateChannelRequest;
import software.amazon.awssdk.services.mediapackage.model.CreateChannelResponse;
import software.amazon.awssdk.services.mediapackage.model.CreateHarvestJobRequest;
import software.amazon.awssdk.services.mediapackage.model.CreateHarvestJobResponse;
import software.amazon.awssdk.services.mediapackage.model.CreateOriginEndpointRequest;
import software.amazon.awssdk.services.mediapackage.model.CreateOriginEndpointResponse;
import software.amazon.awssdk.services.mediapackage.model.DeleteChannelRequest;
import software.amazon.awssdk.services.mediapackage.model.DeleteChannelResponse;
import software.amazon.awssdk.services.mediapackage.model.DeleteOriginEndpointRequest;
import software.amazon.awssdk.services.mediapackage.model.DeleteOriginEndpointResponse;
import software.amazon.awssdk.services.mediapackage.model.DescribeChannelRequest;
import software.amazon.awssdk.services.mediapackage.model.DescribeChannelResponse;
import software.amazon.awssdk.services.mediapackage.model.DescribeHarvestJobRequest;
import software.amazon.awssdk.services.mediapackage.model.DescribeHarvestJobResponse;
import software.amazon.awssdk.services.mediapackage.model.DescribeOriginEndpointRequest;
import software.amazon.awssdk.services.mediapackage.model.DescribeOriginEndpointResponse;
import software.amazon.awssdk.services.mediapackage.model.ForbiddenException;
import software.amazon.awssdk.services.mediapackage.model.InternalServerErrorException;
import software.amazon.awssdk.services.mediapackage.model.ListChannelsRequest;
import software.amazon.awssdk.services.mediapackage.model.ListChannelsResponse;
import software.amazon.awssdk.services.mediapackage.model.ListHarvestJobsRequest;
import software.amazon.awssdk.services.mediapackage.model.ListHarvestJobsResponse;
import software.amazon.awssdk.services.mediapackage.model.ListOriginEndpointsRequest;
import software.amazon.awssdk.services.mediapackage.model.ListOriginEndpointsResponse;
import software.amazon.awssdk.services.mediapackage.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.mediapackage.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.mediapackage.model.MediaPackageException;
import software.amazon.awssdk.services.mediapackage.model.MediaPackageRequest;
import software.amazon.awssdk.services.mediapackage.model.NotFoundException;
import software.amazon.awssdk.services.mediapackage.model.RotateIngestEndpointCredentialsRequest;
import software.amazon.awssdk.services.mediapackage.model.RotateIngestEndpointCredentialsResponse;
import software.amazon.awssdk.services.mediapackage.model.ServiceUnavailableException;
import software.amazon.awssdk.services.mediapackage.model.TagResourceRequest;
import software.amazon.awssdk.services.mediapackage.model.TagResourceResponse;
import software.amazon.awssdk.services.mediapackage.model.TooManyRequestsException;
import software.amazon.awssdk.services.mediapackage.model.UnprocessableEntityException;
import software.amazon.awssdk.services.mediapackage.model.UntagResourceRequest;
import software.amazon.awssdk.services.mediapackage.model.UntagResourceResponse;
import software.amazon.awssdk.services.mediapackage.model.UpdateChannelRequest;
import software.amazon.awssdk.services.mediapackage.model.UpdateChannelResponse;
import software.amazon.awssdk.services.mediapackage.model.UpdateOriginEndpointRequest;
import software.amazon.awssdk.services.mediapackage.model.UpdateOriginEndpointResponse;
import software.amazon.awssdk.services.mediapackage.paginators.ListChannelsIterable;
import software.amazon.awssdk.services.mediapackage.paginators.ListHarvestJobsIterable;
import software.amazon.awssdk.services.mediapackage.paginators.ListOriginEndpointsIterable;
import software.amazon.awssdk.services.mediapackage.transform.CreateChannelRequestMarshaller;
import software.amazon.awssdk.services.mediapackage.transform.CreateHarvestJobRequestMarshaller;
import software.amazon.awssdk.services.mediapackage.transform.CreateOriginEndpointRequestMarshaller;
import software.amazon.awssdk.services.mediapackage.transform.DeleteChannelRequestMarshaller;
import software.amazon.awssdk.services.mediapackage.transform.DeleteOriginEndpointRequestMarshaller;
import software.amazon.awssdk.services.mediapackage.transform.DescribeChannelRequestMarshaller;
import software.amazon.awssdk.services.mediapackage.transform.DescribeHarvestJobRequestMarshaller;
import software.amazon.awssdk.services.mediapackage.transform.DescribeOriginEndpointRequestMarshaller;
import software.amazon.awssdk.services.mediapackage.transform.ListChannelsRequestMarshaller;
import software.amazon.awssdk.services.mediapackage.transform.ListHarvestJobsRequestMarshaller;
import software.amazon.awssdk.services.mediapackage.transform.ListOriginEndpointsRequestMarshaller;
import software.amazon.awssdk.services.mediapackage.transform.ListTagsForResourceRequestMarshaller;
import software.amazon.awssdk.services.mediapackage.transform.RotateIngestEndpointCredentialsRequestMarshaller;
import software.amazon.awssdk.services.mediapackage.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.mediapackage.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.mediapackage.transform.UpdateChannelRequestMarshaller;
import software.amazon.awssdk.services.mediapackage.transform.UpdateOriginEndpointRequestMarshaller;
/**
* Internal implementation of {@link MediaPackageClient}.
*
* @see MediaPackageClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultMediaPackageClient implements MediaPackageClient {
private final SyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultMediaPackageClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsSyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
* Creates a new Channel.
*
* @param createChannelRequest
* A new Channel configuration.
* @return Result of the CreateChannel operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.CreateChannel
* @see AWS API
* Documentation
*/
@Override
public CreateChannelResponse createChannel(CreateChannelRequest createChannelRequest) throws UnprocessableEntityException,
InternalServerErrorException, ForbiddenException, NotFoundException, ServiceUnavailableException,
TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateChannelResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateChannel").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createChannelRequest)
.withMarshaller(new CreateChannelRequestMarshaller(protocolFactory)));
}
/**
* Creates a new HarvestJob record.
*
* @param createHarvestJobRequest
* Configuration parameters used to create a new HarvestJob.
* @return Result of the CreateHarvestJob operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.CreateHarvestJob
* @see AWS
* API Documentation
*/
@Override
public CreateHarvestJobResponse createHarvestJob(CreateHarvestJobRequest createHarvestJobRequest)
throws UnprocessableEntityException, InternalServerErrorException, ForbiddenException, NotFoundException,
ServiceUnavailableException, TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateHarvestJobResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateHarvestJob").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createHarvestJobRequest)
.withMarshaller(new CreateHarvestJobRequestMarshaller(protocolFactory)));
}
/**
* Creates a new OriginEndpoint record.
*
* @param createOriginEndpointRequest
* Configuration parameters used to create a new OriginEndpoint.
* @return Result of the CreateOriginEndpoint operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.CreateOriginEndpoint
* @see AWS API Documentation
*/
@Override
public CreateOriginEndpointResponse createOriginEndpoint(CreateOriginEndpointRequest createOriginEndpointRequest)
throws UnprocessableEntityException, InternalServerErrorException, ForbiddenException, NotFoundException,
ServiceUnavailableException, TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateOriginEndpointResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateOriginEndpoint").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createOriginEndpointRequest)
.withMarshaller(new CreateOriginEndpointRequestMarshaller(protocolFactory)));
}
/**
* Deletes an existing Channel.
*
* @param deleteChannelRequest
* @return Result of the DeleteChannel operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.DeleteChannel
* @see AWS API
* Documentation
*/
@Override
public DeleteChannelResponse deleteChannel(DeleteChannelRequest deleteChannelRequest) throws UnprocessableEntityException,
InternalServerErrorException, ForbiddenException, NotFoundException, ServiceUnavailableException,
TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteChannelResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteChannel").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteChannelRequest)
.withMarshaller(new DeleteChannelRequestMarshaller(protocolFactory)));
}
/**
* Deletes an existing OriginEndpoint.
*
* @param deleteOriginEndpointRequest
* @return Result of the DeleteOriginEndpoint operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.DeleteOriginEndpoint
* @see AWS API Documentation
*/
@Override
public DeleteOriginEndpointResponse deleteOriginEndpoint(DeleteOriginEndpointRequest deleteOriginEndpointRequest)
throws UnprocessableEntityException, InternalServerErrorException, ForbiddenException, NotFoundException,
ServiceUnavailableException, TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteOriginEndpointResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteOriginEndpoint").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteOriginEndpointRequest)
.withMarshaller(new DeleteOriginEndpointRequestMarshaller(protocolFactory)));
}
/**
* Gets details about a Channel.
*
* @param describeChannelRequest
* @return Result of the DescribeChannel operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.DescribeChannel
* @see AWS
* API Documentation
*/
@Override
public DescribeChannelResponse describeChannel(DescribeChannelRequest describeChannelRequest)
throws UnprocessableEntityException, InternalServerErrorException, ForbiddenException, NotFoundException,
ServiceUnavailableException, TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeChannelResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeChannel").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeChannelRequest)
.withMarshaller(new DescribeChannelRequestMarshaller(protocolFactory)));
}
/**
* Gets details about an existing HarvestJob.
*
* @param describeHarvestJobRequest
* @return Result of the DescribeHarvestJob operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.DescribeHarvestJob
* @see AWS API Documentation
*/
@Override
public DescribeHarvestJobResponse describeHarvestJob(DescribeHarvestJobRequest describeHarvestJobRequest)
throws UnprocessableEntityException, InternalServerErrorException, ForbiddenException, NotFoundException,
ServiceUnavailableException, TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeHarvestJobResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeHarvestJob").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeHarvestJobRequest)
.withMarshaller(new DescribeHarvestJobRequestMarshaller(protocolFactory)));
}
/**
* Gets details about an existing OriginEndpoint.
*
* @param describeOriginEndpointRequest
* @return Result of the DescribeOriginEndpoint operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.DescribeOriginEndpoint
* @see AWS API Documentation
*/
@Override
public DescribeOriginEndpointResponse describeOriginEndpoint(DescribeOriginEndpointRequest describeOriginEndpointRequest)
throws UnprocessableEntityException, InternalServerErrorException, ForbiddenException, NotFoundException,
ServiceUnavailableException, TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeOriginEndpointResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeOriginEndpoint").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeOriginEndpointRequest)
.withMarshaller(new DescribeOriginEndpointRequestMarshaller(protocolFactory)));
}
/**
* Returns a collection of Channels.
*
* @param listChannelsRequest
* @return Result of the ListChannels operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.ListChannels
* @see AWS API
* Documentation
*/
@Override
public ListChannelsResponse listChannels(ListChannelsRequest listChannelsRequest) throws UnprocessableEntityException,
InternalServerErrorException, ForbiddenException, NotFoundException, ServiceUnavailableException,
TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListChannelsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListChannels").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listChannelsRequest)
.withMarshaller(new ListChannelsRequestMarshaller(protocolFactory)));
}
/**
* Returns a collection of Channels.
*
* This is a variant of
* {@link #listChannels(software.amazon.awssdk.services.mediapackage.model.ListChannelsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.mediapackage.paginators.ListChannelsIterable responses = client.listChannelsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.mediapackage.paginators.ListChannelsIterable responses = client
* .listChannelsPaginator(request);
* for (software.amazon.awssdk.services.mediapackage.model.ListChannelsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.mediapackage.paginators.ListChannelsIterable responses = client.listChannelsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listChannels(software.amazon.awssdk.services.mediapackage.model.ListChannelsRequest)} operation.
*
*
* @param listChannelsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.ListChannels
* @see AWS API
* Documentation
*/
@Override
public ListChannelsIterable listChannelsPaginator(ListChannelsRequest listChannelsRequest)
throws UnprocessableEntityException, InternalServerErrorException, ForbiddenException, NotFoundException,
ServiceUnavailableException, TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
return new ListChannelsIterable(this, applyPaginatorUserAgent(listChannelsRequest));
}
/**
* Returns a collection of HarvestJob records.
*
* @param listHarvestJobsRequest
* @return Result of the ListHarvestJobs operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.ListHarvestJobs
* @see AWS
* API Documentation
*/
@Override
public ListHarvestJobsResponse listHarvestJobs(ListHarvestJobsRequest listHarvestJobsRequest)
throws UnprocessableEntityException, InternalServerErrorException, ForbiddenException, NotFoundException,
ServiceUnavailableException, TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListHarvestJobsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListHarvestJobs").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listHarvestJobsRequest)
.withMarshaller(new ListHarvestJobsRequestMarshaller(protocolFactory)));
}
/**
* Returns a collection of HarvestJob records.
*
* This is a variant of
* {@link #listHarvestJobs(software.amazon.awssdk.services.mediapackage.model.ListHarvestJobsRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.mediapackage.paginators.ListHarvestJobsIterable responses = client.listHarvestJobsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.mediapackage.paginators.ListHarvestJobsIterable responses = client
* .listHarvestJobsPaginator(request);
* for (software.amazon.awssdk.services.mediapackage.model.ListHarvestJobsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.mediapackage.paginators.ListHarvestJobsIterable responses = client.listHarvestJobsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listHarvestJobs(software.amazon.awssdk.services.mediapackage.model.ListHarvestJobsRequest)}
* operation.
*
*
* @param listHarvestJobsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.ListHarvestJobs
* @see AWS
* API Documentation
*/
@Override
public ListHarvestJobsIterable listHarvestJobsPaginator(ListHarvestJobsRequest listHarvestJobsRequest)
throws UnprocessableEntityException, InternalServerErrorException, ForbiddenException, NotFoundException,
ServiceUnavailableException, TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
return new ListHarvestJobsIterable(this, applyPaginatorUserAgent(listHarvestJobsRequest));
}
/**
* Returns a collection of OriginEndpoint records.
*
* @param listOriginEndpointsRequest
* @return Result of the ListOriginEndpoints operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.ListOriginEndpoints
* @see AWS API Documentation
*/
@Override
public ListOriginEndpointsResponse listOriginEndpoints(ListOriginEndpointsRequest listOriginEndpointsRequest)
throws UnprocessableEntityException, InternalServerErrorException, ForbiddenException, NotFoundException,
ServiceUnavailableException, TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListOriginEndpointsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListOriginEndpoints").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listOriginEndpointsRequest)
.withMarshaller(new ListOriginEndpointsRequestMarshaller(protocolFactory)));
}
/**
* Returns a collection of OriginEndpoint records.
*
* This is a variant of
* {@link #listOriginEndpoints(software.amazon.awssdk.services.mediapackage.model.ListOriginEndpointsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.mediapackage.paginators.ListOriginEndpointsIterable responses = client.listOriginEndpointsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.mediapackage.paginators.ListOriginEndpointsIterable responses = client
* .listOriginEndpointsPaginator(request);
* for (software.amazon.awssdk.services.mediapackage.model.ListOriginEndpointsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.mediapackage.paginators.ListOriginEndpointsIterable responses = client.listOriginEndpointsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listOriginEndpoints(software.amazon.awssdk.services.mediapackage.model.ListOriginEndpointsRequest)}
* operation.
*
*
* @param listOriginEndpointsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.ListOriginEndpoints
* @see AWS API Documentation
*/
@Override
public ListOriginEndpointsIterable listOriginEndpointsPaginator(ListOriginEndpointsRequest listOriginEndpointsRequest)
throws UnprocessableEntityException, InternalServerErrorException, ForbiddenException, NotFoundException,
ServiceUnavailableException, TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
return new ListOriginEndpointsIterable(this, applyPaginatorUserAgent(listOriginEndpointsRequest));
}
/**
* Invokes the ListTagsForResource operation.
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.ListTagsForResource
* @see AWS API Documentation
*/
@Override
public ListTagsForResourceResponse listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest)
throws AwsServiceException, SdkClientException, MediaPackageException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListTagsForResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListTagsForResource").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listTagsForResourceRequest)
.withMarshaller(new ListTagsForResourceRequestMarshaller(protocolFactory)));
}
/**
* Rotate the IngestEndpoint's username and password, as specified by the IngestEndpoint's id.
*
* @param rotateIngestEndpointCredentialsRequest
* @return Result of the RotateIngestEndpointCredentials operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.RotateIngestEndpointCredentials
* @see AWS API Documentation
*/
@Override
public RotateIngestEndpointCredentialsResponse rotateIngestEndpointCredentials(
RotateIngestEndpointCredentialsRequest rotateIngestEndpointCredentialsRequest) throws UnprocessableEntityException,
InternalServerErrorException, ForbiddenException, NotFoundException, ServiceUnavailableException,
TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, RotateIngestEndpointCredentialsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("RotateIngestEndpointCredentials").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(rotateIngestEndpointCredentialsRequest)
.withMarshaller(new RotateIngestEndpointCredentialsRequestMarshaller(protocolFactory)));
}
/**
* Invokes the TagResource operation.
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.TagResource
* @see AWS API
* Documentation
*/
@Override
public TagResourceResponse tagResource(TagResourceRequest tagResourceRequest) throws AwsServiceException, SdkClientException,
MediaPackageException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
TagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("TagResource").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(tagResourceRequest)
.withMarshaller(new TagResourceRequestMarshaller(protocolFactory)));
}
/**
* Invokes the UntagResource operation.
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.UntagResource
* @see AWS API
* Documentation
*/
@Override
public UntagResourceResponse untagResource(UntagResourceRequest untagResourceRequest) throws AwsServiceException,
SdkClientException, MediaPackageException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UntagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UntagResource").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(untagResourceRequest)
.withMarshaller(new UntagResourceRequestMarshaller(protocolFactory)));
}
/**
* Updates an existing Channel.
*
* @param updateChannelRequest
* Configuration parameters used to update the Channel.
* @return Result of the UpdateChannel operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.UpdateChannel
* @see AWS API
* Documentation
*/
@Override
public UpdateChannelResponse updateChannel(UpdateChannelRequest updateChannelRequest) throws UnprocessableEntityException,
InternalServerErrorException, ForbiddenException, NotFoundException, ServiceUnavailableException,
TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UpdateChannelResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UpdateChannel").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(updateChannelRequest)
.withMarshaller(new UpdateChannelRequestMarshaller(protocolFactory)));
}
/**
* Updates an existing OriginEndpoint.
*
* @param updateOriginEndpointRequest
* Configuration parameters used to update an existing OriginEndpoint.
* @return Result of the UpdateOriginEndpoint operation returned by the service.
* @throws UnprocessableEntityException
* The parameters sent in the request are not valid.
* @throws InternalServerErrorException
* An unexpected error occurred.
* @throws ForbiddenException
* The client is not authorized to access the requested resource.
* @throws NotFoundException
* The requested resource does not exist.
* @throws ServiceUnavailableException
* An unexpected error occurred.
* @throws TooManyRequestsException
* The client has exceeded their resource or throttling limits.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaPackageException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaPackageClient.UpdateOriginEndpoint
* @see AWS API Documentation
*/
@Override
public UpdateOriginEndpointResponse updateOriginEndpoint(UpdateOriginEndpointRequest updateOriginEndpointRequest)
throws UnprocessableEntityException, InternalServerErrorException, ForbiddenException, NotFoundException,
ServiceUnavailableException, TooManyRequestsException, AwsServiceException, SdkClientException, MediaPackageException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, UpdateOriginEndpointResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UpdateOriginEndpoint").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(updateOriginEndpointRequest)
.withMarshaller(new UpdateOriginEndpointRequestMarshaller(protocolFactory)));
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata) {
return protocolFactory.createErrorResponseHandler(operationMetadata);
}
private > T init(T builder) {
return builder
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(MediaPackageException::builder)
.protocol(AwsJsonProtocol.REST_JSON)
.protocolVersion("1.1")
.registerModeledException(
ExceptionMetadata.builder().errorCode("UnprocessableEntityException")
.exceptionBuilderSupplier(UnprocessableEntityException::builder).httpStatusCode(422).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("NotFoundException")
.exceptionBuilderSupplier(NotFoundException::builder).httpStatusCode(404).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ServiceUnavailableException")
.exceptionBuilderSupplier(ServiceUnavailableException::builder).httpStatusCode(503).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ForbiddenException")
.exceptionBuilderSupplier(ForbiddenException::builder).httpStatusCode(403).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TooManyRequestsException")
.exceptionBuilderSupplier(TooManyRequestsException::builder).httpStatusCode(429).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InternalServerErrorException")
.exceptionBuilderSupplier(InternalServerErrorException::builder).httpStatusCode(500).build());
}
@Override
public void close() {
clientHandler.close();
}
private T applyPaginatorUserAgent(T request) {
Consumer userAgentApplier = b -> b.addApiName(ApiName.builder()
.version(VersionInfo.SDK_VERSION).name("PAGINATED").build());
AwsRequestOverrideConfiguration overrideConfiguration = request.overrideConfiguration()
.map(c -> c.toBuilder().applyMutation(userAgentApplier).build())
.orElse((AwsRequestOverrideConfiguration.builder().applyMutation(userAgentApplier).build()));
return (T) request.toBuilder().overrideConfiguration(overrideConfiguration).build();
}
}