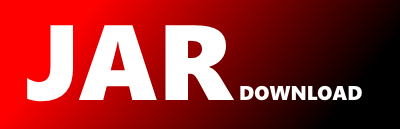
software.amazon.awssdk.services.mediapackage.model.UpdateOriginEndpointRequest Maven / Gradle / Ivy
Show all versions of mediapackage Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.mediapackage.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
* Configuration parameters used to update an existing OriginEndpoint.
*/
@Generated("software.amazon.awssdk:codegen")
public final class UpdateOriginEndpointRequest extends MediaPackageRequest implements
ToCopyableBuilder {
private static final SdkField AUTHORIZATION_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.getter(getter(UpdateOriginEndpointRequest::authorization)).setter(setter(Builder::authorization))
.constructor(Authorization::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("authorization").build()).build();
private static final SdkField CMAF_PACKAGE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.getter(getter(UpdateOriginEndpointRequest::cmafPackage)).setter(setter(Builder::cmafPackage))
.constructor(CmafPackageCreateOrUpdateParameters::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("cmafPackage").build()).build();
private static final SdkField DASH_PACKAGE_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.getter(getter(UpdateOriginEndpointRequest::dashPackage)).setter(setter(Builder::dashPackage))
.constructor(DashPackage::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("dashPackage").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(UpdateOriginEndpointRequest::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("description").build()).build();
private static final SdkField HLS_PACKAGE_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.getter(getter(UpdateOriginEndpointRequest::hlsPackage)).setter(setter(Builder::hlsPackage))
.constructor(HlsPackage::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("hlsPackage").build()).build();
private static final SdkField ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(UpdateOriginEndpointRequest::id)).setter(setter(Builder::id))
.traits(LocationTrait.builder().location(MarshallLocation.PATH).locationName("id").build()).build();
private static final SdkField MANIFEST_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(UpdateOriginEndpointRequest::manifestName)).setter(setter(Builder::manifestName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("manifestName").build()).build();
private static final SdkField MSS_PACKAGE_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.getter(getter(UpdateOriginEndpointRequest::mssPackage)).setter(setter(Builder::mssPackage))
.constructor(MssPackage::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("mssPackage").build()).build();
private static final SdkField ORIGINATION_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(UpdateOriginEndpointRequest::originationAsString)).setter(setter(Builder::origination))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("origination").build()).build();
private static final SdkField STARTOVER_WINDOW_SECONDS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.getter(getter(UpdateOriginEndpointRequest::startoverWindowSeconds)).setter(setter(Builder::startoverWindowSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("startoverWindowSeconds").build())
.build();
private static final SdkField TIME_DELAY_SECONDS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.getter(getter(UpdateOriginEndpointRequest::timeDelaySeconds)).setter(setter(Builder::timeDelaySeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("timeDelaySeconds").build()).build();
private static final SdkField> WHITELIST_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(UpdateOriginEndpointRequest::whitelist))
.setter(setter(Builder::whitelist))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("whitelist").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(AUTHORIZATION_FIELD,
CMAF_PACKAGE_FIELD, DASH_PACKAGE_FIELD, DESCRIPTION_FIELD, HLS_PACKAGE_FIELD, ID_FIELD, MANIFEST_NAME_FIELD,
MSS_PACKAGE_FIELD, ORIGINATION_FIELD, STARTOVER_WINDOW_SECONDS_FIELD, TIME_DELAY_SECONDS_FIELD, WHITELIST_FIELD));
private final Authorization authorization;
private final CmafPackageCreateOrUpdateParameters cmafPackage;
private final DashPackage dashPackage;
private final String description;
private final HlsPackage hlsPackage;
private final String id;
private final String manifestName;
private final MssPackage mssPackage;
private final String origination;
private final Integer startoverWindowSeconds;
private final Integer timeDelaySeconds;
private final List whitelist;
private UpdateOriginEndpointRequest(BuilderImpl builder) {
super(builder);
this.authorization = builder.authorization;
this.cmafPackage = builder.cmafPackage;
this.dashPackage = builder.dashPackage;
this.description = builder.description;
this.hlsPackage = builder.hlsPackage;
this.id = builder.id;
this.manifestName = builder.manifestName;
this.mssPackage = builder.mssPackage;
this.origination = builder.origination;
this.startoverWindowSeconds = builder.startoverWindowSeconds;
this.timeDelaySeconds = builder.timeDelaySeconds;
this.whitelist = builder.whitelist;
}
/**
* Returns the value of the Authorization property for this object.
*
* @return The value of the Authorization property for this object.
*/
public Authorization authorization() {
return authorization;
}
/**
* Returns the value of the CmafPackage property for this object.
*
* @return The value of the CmafPackage property for this object.
*/
public CmafPackageCreateOrUpdateParameters cmafPackage() {
return cmafPackage;
}
/**
* Returns the value of the DashPackage property for this object.
*
* @return The value of the DashPackage property for this object.
*/
public DashPackage dashPackage() {
return dashPackage;
}
/**
* A short text description of the OriginEndpoint.
*
* @return A short text description of the OriginEndpoint.
*/
public String description() {
return description;
}
/**
* Returns the value of the HlsPackage property for this object.
*
* @return The value of the HlsPackage property for this object.
*/
public HlsPackage hlsPackage() {
return hlsPackage;
}
/**
* The ID of the OriginEndpoint to update.
*
* @return The ID of the OriginEndpoint to update.
*/
public String id() {
return id;
}
/**
* A short string that will be appended to the end of the Endpoint URL.
*
* @return A short string that will be appended to the end of the Endpoint URL.
*/
public String manifestName() {
return manifestName;
}
/**
* Returns the value of the MssPackage property for this object.
*
* @return The value of the MssPackage property for this object.
*/
public MssPackage mssPackage() {
return mssPackage;
}
/**
* Control whether origination of video is allowed for this OriginEndpoint. If set to ALLOW, the OriginEndpoint may
* by requested, pursuant to any other form of access control. If set to DENY, the OriginEndpoint may not be
* requested. This can be helpful for Live to VOD harvesting, or for temporarily disabling origination
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #origination} will
* return {@link Origination#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #originationAsString}.
*
*
* @return Control whether origination of video is allowed for this OriginEndpoint. If set to ALLOW, the
* OriginEndpoint may by requested, pursuant to any other form of access control. If set to DENY, the
* OriginEndpoint may not be requested. This can be helpful for Live to VOD harvesting, or for temporarily
* disabling origination
* @see Origination
*/
public Origination origination() {
return Origination.fromValue(origination);
}
/**
* Control whether origination of video is allowed for this OriginEndpoint. If set to ALLOW, the OriginEndpoint may
* by requested, pursuant to any other form of access control. If set to DENY, the OriginEndpoint may not be
* requested. This can be helpful for Live to VOD harvesting, or for temporarily disabling origination
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #origination} will
* return {@link Origination#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #originationAsString}.
*
*
* @return Control whether origination of video is allowed for this OriginEndpoint. If set to ALLOW, the
* OriginEndpoint may by requested, pursuant to any other form of access control. If set to DENY, the
* OriginEndpoint may not be requested. This can be helpful for Live to VOD harvesting, or for temporarily
* disabling origination
* @see Origination
*/
public String originationAsString() {
return origination;
}
/**
* Maximum duration (in seconds) of content to retain for startover playback. If not specified, startover playback
* will be disabled for the OriginEndpoint.
*
* @return Maximum duration (in seconds) of content to retain for startover playback. If not specified, startover
* playback will be disabled for the OriginEndpoint.
*/
public Integer startoverWindowSeconds() {
return startoverWindowSeconds;
}
/**
* Amount of delay (in seconds) to enforce on the playback of live content. If not specified, there will be no time
* delay in effect for the OriginEndpoint.
*
* @return Amount of delay (in seconds) to enforce on the playback of live content. If not specified, there will be
* no time delay in effect for the OriginEndpoint.
*/
public Integer timeDelaySeconds() {
return timeDelaySeconds;
}
/**
* Returns true if the Whitelist property was specified by the sender (it may be empty), or false if the sender did
* not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public boolean hasWhitelist() {
return whitelist != null && !(whitelist instanceof SdkAutoConstructList);
}
/**
* A list of source IP CIDR blocks that will be allowed to access the OriginEndpoint.
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasWhitelist()} to see if a value was sent in this field.
*
*
* @return A list of source IP CIDR blocks that will be allowed to access the OriginEndpoint.
*/
public List whitelist() {
return whitelist;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(authorization());
hashCode = 31 * hashCode + Objects.hashCode(cmafPackage());
hashCode = 31 * hashCode + Objects.hashCode(dashPackage());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(hlsPackage());
hashCode = 31 * hashCode + Objects.hashCode(id());
hashCode = 31 * hashCode + Objects.hashCode(manifestName());
hashCode = 31 * hashCode + Objects.hashCode(mssPackage());
hashCode = 31 * hashCode + Objects.hashCode(originationAsString());
hashCode = 31 * hashCode + Objects.hashCode(startoverWindowSeconds());
hashCode = 31 * hashCode + Objects.hashCode(timeDelaySeconds());
hashCode = 31 * hashCode + Objects.hashCode(whitelist());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UpdateOriginEndpointRequest)) {
return false;
}
UpdateOriginEndpointRequest other = (UpdateOriginEndpointRequest) obj;
return Objects.equals(authorization(), other.authorization()) && Objects.equals(cmafPackage(), other.cmafPackage())
&& Objects.equals(dashPackage(), other.dashPackage()) && Objects.equals(description(), other.description())
&& Objects.equals(hlsPackage(), other.hlsPackage()) && Objects.equals(id(), other.id())
&& Objects.equals(manifestName(), other.manifestName()) && Objects.equals(mssPackage(), other.mssPackage())
&& Objects.equals(originationAsString(), other.originationAsString())
&& Objects.equals(startoverWindowSeconds(), other.startoverWindowSeconds())
&& Objects.equals(timeDelaySeconds(), other.timeDelaySeconds()) && Objects.equals(whitelist(), other.whitelist());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("UpdateOriginEndpointRequest").add("Authorization", authorization())
.add("CmafPackage", cmafPackage()).add("DashPackage", dashPackage()).add("Description", description())
.add("HlsPackage", hlsPackage()).add("Id", id()).add("ManifestName", manifestName())
.add("MssPackage", mssPackage()).add("Origination", originationAsString())
.add("StartoverWindowSeconds", startoverWindowSeconds()).add("TimeDelaySeconds", timeDelaySeconds())
.add("Whitelist", whitelist()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Authorization":
return Optional.ofNullable(clazz.cast(authorization()));
case "CmafPackage":
return Optional.ofNullable(clazz.cast(cmafPackage()));
case "DashPackage":
return Optional.ofNullable(clazz.cast(dashPackage()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "HlsPackage":
return Optional.ofNullable(clazz.cast(hlsPackage()));
case "Id":
return Optional.ofNullable(clazz.cast(id()));
case "ManifestName":
return Optional.ofNullable(clazz.cast(manifestName()));
case "MssPackage":
return Optional.ofNullable(clazz.cast(mssPackage()));
case "Origination":
return Optional.ofNullable(clazz.cast(originationAsString()));
case "StartoverWindowSeconds":
return Optional.ofNullable(clazz.cast(startoverWindowSeconds()));
case "TimeDelaySeconds":
return Optional.ofNullable(clazz.cast(timeDelaySeconds()));
case "Whitelist":
return Optional.ofNullable(clazz.cast(whitelist()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function