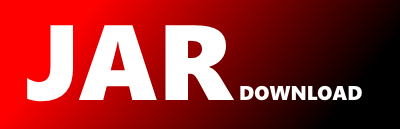
software.amazon.awssdk.services.mediapackage.model.SpekeKeyProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mediapackage Show documentation
Show all versions of mediapackage Show documentation
The AWS Java SDK for AWS Elemental MediaPackage module holds the client classes that are used for
communicating
with AWS Elemental MediaPackage Service
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.mediapackage.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
* A configuration for accessing an external Secure Packager and Encoder Key Exchange (SPEKE) service that will provide
* encryption keys.
*/
@Generated("software.amazon.awssdk:codegen")
public final class SpekeKeyProvider implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField CERTIFICATE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(SpekeKeyProvider::certificateArn)).setter(setter(Builder::certificateArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("certificateArn").build()).build();
private static final SdkField RESOURCE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(SpekeKeyProvider::resourceId)).setter(setter(Builder::resourceId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("resourceId").build()).build();
private static final SdkField ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(SpekeKeyProvider::roleArn)).setter(setter(Builder::roleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("roleArn").build()).build();
private static final SdkField> SYSTEM_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(SpekeKeyProvider::systemIds))
.setter(setter(Builder::systemIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("systemIds").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField URL_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(SpekeKeyProvider::url)).setter(setter(Builder::url))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("url").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CERTIFICATE_ARN_FIELD,
RESOURCE_ID_FIELD, ROLE_ARN_FIELD, SYSTEM_IDS_FIELD, URL_FIELD));
private static final long serialVersionUID = 1L;
private final String certificateArn;
private final String resourceId;
private final String roleArn;
private final List systemIds;
private final String url;
private SpekeKeyProvider(BuilderImpl builder) {
this.certificateArn = builder.certificateArn;
this.resourceId = builder.resourceId;
this.roleArn = builder.roleArn;
this.systemIds = builder.systemIds;
this.url = builder.url;
}
/**
* An Amazon Resource Name (ARN) of a Certificate Manager certificate that MediaPackage will use for enforcing
* secure end-to-end data transfer with the key provider service.
*
* @return An Amazon Resource Name (ARN) of a Certificate Manager certificate that MediaPackage will use for
* enforcing secure end-to-end data transfer with the key provider service.
*/
public String certificateArn() {
return certificateArn;
}
/**
* The resource ID to include in key requests.
*
* @return The resource ID to include in key requests.
*/
public String resourceId() {
return resourceId;
}
/**
* An Amazon Resource Name (ARN) of an IAM role that AWS Elemental MediaPackage will assume when accessing the key
* provider service.
*
* @return An Amazon Resource Name (ARN) of an IAM role that AWS Elemental MediaPackage will assume when accessing
* the key provider service.
*/
public String roleArn() {
return roleArn;
}
/**
* Returns true if the SystemIds property was specified by the sender (it may be empty), or false if the sender did
* not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public boolean hasSystemIds() {
return systemIds != null && !(systemIds instanceof SdkAutoConstructList);
}
/**
* The system IDs to include in key requests.
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasSystemIds()} to see if a value was sent in this field.
*
*
* @return The system IDs to include in key requests.
*/
public List systemIds() {
return systemIds;
}
/**
* The URL of the external key provider service.
*
* @return The URL of the external key provider service.
*/
public String url() {
return url;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(certificateArn());
hashCode = 31 * hashCode + Objects.hashCode(resourceId());
hashCode = 31 * hashCode + Objects.hashCode(roleArn());
hashCode = 31 * hashCode + Objects.hashCode(systemIds());
hashCode = 31 * hashCode + Objects.hashCode(url());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof SpekeKeyProvider)) {
return false;
}
SpekeKeyProvider other = (SpekeKeyProvider) obj;
return Objects.equals(certificateArn(), other.certificateArn()) && Objects.equals(resourceId(), other.resourceId())
&& Objects.equals(roleArn(), other.roleArn()) && Objects.equals(systemIds(), other.systemIds())
&& Objects.equals(url(), other.url());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("SpekeKeyProvider").add("CertificateArn", certificateArn()).add("ResourceId", resourceId())
.add("RoleArn", roleArn()).add("SystemIds", systemIds()).add("Url", url()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "CertificateArn":
return Optional.ofNullable(clazz.cast(certificateArn()));
case "ResourceId":
return Optional.ofNullable(clazz.cast(resourceId()));
case "RoleArn":
return Optional.ofNullable(clazz.cast(roleArn()));
case "SystemIds":
return Optional.ofNullable(clazz.cast(systemIds()));
case "Url":
return Optional.ofNullable(clazz.cast(url()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy