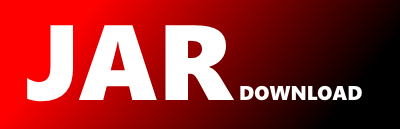
software.amazon.awssdk.services.mediapackage.model.HlsPackage Maven / Gradle / Ivy
Show all versions of mediapackage Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.mediapackage.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
* An HTTP Live Streaming (HLS) packaging configuration.
*/
@Generated("software.amazon.awssdk:codegen")
public final class HlsPackage implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField AD_MARKERS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AdMarkers").getter(getter(HlsPackage::adMarkersAsString)).setter(setter(Builder::adMarkers))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("adMarkers").build()).build();
private static final SdkField> AD_TRIGGERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("AdTriggers")
.getter(getter(HlsPackage::adTriggersAsStrings))
.setter(setter(Builder::adTriggersWithStrings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("adTriggers").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField ADS_ON_DELIVERY_RESTRICTIONS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AdsOnDeliveryRestrictions").getter(getter(HlsPackage::adsOnDeliveryRestrictionsAsString))
.setter(setter(Builder::adsOnDeliveryRestrictions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("adsOnDeliveryRestrictions").build())
.build();
private static final SdkField ENCRYPTION_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("Encryption").getter(getter(HlsPackage::encryption)).setter(setter(Builder::encryption))
.constructor(HlsEncryption::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("encryption").build()).build();
private static final SdkField INCLUDE_DVB_SUBTITLES_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("IncludeDvbSubtitles").getter(getter(HlsPackage::includeDvbSubtitles))
.setter(setter(Builder::includeDvbSubtitles))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("includeDvbSubtitles").build())
.build();
private static final SdkField INCLUDE_IFRAME_ONLY_STREAM_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("IncludeIframeOnlyStream").getter(getter(HlsPackage::includeIframeOnlyStream))
.setter(setter(Builder::includeIframeOnlyStream))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("includeIframeOnlyStream").build())
.build();
private static final SdkField PLAYLIST_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PlaylistType").getter(getter(HlsPackage::playlistTypeAsString)).setter(setter(Builder::playlistType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("playlistType").build()).build();
private static final SdkField PLAYLIST_WINDOW_SECONDS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("PlaylistWindowSeconds").getter(getter(HlsPackage::playlistWindowSeconds))
.setter(setter(Builder::playlistWindowSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("playlistWindowSeconds").build())
.build();
private static final SdkField PROGRAM_DATE_TIME_INTERVAL_SECONDS_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("ProgramDateTimeIntervalSeconds")
.getter(getter(HlsPackage::programDateTimeIntervalSeconds))
.setter(setter(Builder::programDateTimeIntervalSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("programDateTimeIntervalSeconds")
.build()).build();
private static final SdkField SEGMENT_DURATION_SECONDS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("SegmentDurationSeconds").getter(getter(HlsPackage::segmentDurationSeconds))
.setter(setter(Builder::segmentDurationSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("segmentDurationSeconds").build())
.build();
private static final SdkField STREAM_SELECTION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("StreamSelection")
.getter(getter(HlsPackage::streamSelection)).setter(setter(Builder::streamSelection))
.constructor(StreamSelection::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("streamSelection").build()).build();
private static final SdkField USE_AUDIO_RENDITION_GROUP_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("UseAudioRenditionGroup").getter(getter(HlsPackage::useAudioRenditionGroup))
.setter(setter(Builder::useAudioRenditionGroup))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("useAudioRenditionGroup").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(AD_MARKERS_FIELD,
AD_TRIGGERS_FIELD, ADS_ON_DELIVERY_RESTRICTIONS_FIELD, ENCRYPTION_FIELD, INCLUDE_DVB_SUBTITLES_FIELD,
INCLUDE_IFRAME_ONLY_STREAM_FIELD, PLAYLIST_TYPE_FIELD, PLAYLIST_WINDOW_SECONDS_FIELD,
PROGRAM_DATE_TIME_INTERVAL_SECONDS_FIELD, SEGMENT_DURATION_SECONDS_FIELD, STREAM_SELECTION_FIELD,
USE_AUDIO_RENDITION_GROUP_FIELD));
private static final long serialVersionUID = 1L;
private final String adMarkers;
private final List adTriggers;
private final String adsOnDeliveryRestrictions;
private final HlsEncryption encryption;
private final Boolean includeDvbSubtitles;
private final Boolean includeIframeOnlyStream;
private final String playlistType;
private final Integer playlistWindowSeconds;
private final Integer programDateTimeIntervalSeconds;
private final Integer segmentDurationSeconds;
private final StreamSelection streamSelection;
private final Boolean useAudioRenditionGroup;
private HlsPackage(BuilderImpl builder) {
this.adMarkers = builder.adMarkers;
this.adTriggers = builder.adTriggers;
this.adsOnDeliveryRestrictions = builder.adsOnDeliveryRestrictions;
this.encryption = builder.encryption;
this.includeDvbSubtitles = builder.includeDvbSubtitles;
this.includeIframeOnlyStream = builder.includeIframeOnlyStream;
this.playlistType = builder.playlistType;
this.playlistWindowSeconds = builder.playlistWindowSeconds;
this.programDateTimeIntervalSeconds = builder.programDateTimeIntervalSeconds;
this.segmentDurationSeconds = builder.segmentDurationSeconds;
this.streamSelection = builder.streamSelection;
this.useAudioRenditionGroup = builder.useAudioRenditionGroup;
}
/**
* This setting controls how ad markers are included in the packaged OriginEndpoint. "NONE" will omit all SCTE-35 ad
* markers from the output. "PASSTHROUGH" causes the manifest to contain a copy of the SCTE-35 ad markers (comments)
* taken directly from the input HTTP Live Streaming (HLS) manifest. "SCTE35_ENHANCED" generates ad markers and
* blackout tags based on SCTE-35 messages in the input source. "DATERANGE" inserts EXT-X-DATERANGE tags to signal
* ad and program transition events in HLS and CMAF manifests. For this option, you must set a
* programDateTimeIntervalSeconds value that is greater than 0.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #adMarkers} will
* return {@link AdMarkers#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #adMarkersAsString}.
*
*
* @return This setting controls how ad markers are included in the packaged OriginEndpoint. "NONE" will omit all
* SCTE-35 ad markers from the output. "PASSTHROUGH" causes the manifest to contain a copy of the SCTE-35 ad
* markers (comments) taken directly from the input HTTP Live Streaming (HLS) manifest. "SCTE35_ENHANCED"
* generates ad markers and blackout tags based on SCTE-35 messages in the input source. "DATERANGE" inserts
* EXT-X-DATERANGE tags to signal ad and program transition events in HLS and CMAF manifests. For this
* option, you must set a programDateTimeIntervalSeconds value that is greater than 0.
* @see AdMarkers
*/
public final AdMarkers adMarkers() {
return AdMarkers.fromValue(adMarkers);
}
/**
* This setting controls how ad markers are included in the packaged OriginEndpoint. "NONE" will omit all SCTE-35 ad
* markers from the output. "PASSTHROUGH" causes the manifest to contain a copy of the SCTE-35 ad markers (comments)
* taken directly from the input HTTP Live Streaming (HLS) manifest. "SCTE35_ENHANCED" generates ad markers and
* blackout tags based on SCTE-35 messages in the input source. "DATERANGE" inserts EXT-X-DATERANGE tags to signal
* ad and program transition events in HLS and CMAF manifests. For this option, you must set a
* programDateTimeIntervalSeconds value that is greater than 0.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #adMarkers} will
* return {@link AdMarkers#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #adMarkersAsString}.
*
*
* @return This setting controls how ad markers are included in the packaged OriginEndpoint. "NONE" will omit all
* SCTE-35 ad markers from the output. "PASSTHROUGH" causes the manifest to contain a copy of the SCTE-35 ad
* markers (comments) taken directly from the input HTTP Live Streaming (HLS) manifest. "SCTE35_ENHANCED"
* generates ad markers and blackout tags based on SCTE-35 messages in the input source. "DATERANGE" inserts
* EXT-X-DATERANGE tags to signal ad and program transition events in HLS and CMAF manifests. For this
* option, you must set a programDateTimeIntervalSeconds value that is greater than 0.
* @see AdMarkers
*/
public final String adMarkersAsString() {
return adMarkers;
}
/**
* Returns the value of the AdTriggers property for this object.
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAdTriggers} method.
*
*
* @return The value of the AdTriggers property for this object.
*/
public final List adTriggers() {
return AdTriggersCopier.copyStringToEnum(adTriggers);
}
/**
* For responses, this returns true if the service returned a value for the AdTriggers property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasAdTriggers() {
return adTriggers != null && !(adTriggers instanceof SdkAutoConstructList);
}
/**
* Returns the value of the AdTriggers property for this object.
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAdTriggers} method.
*
*
* @return The value of the AdTriggers property for this object.
*/
public final List adTriggersAsStrings() {
return adTriggers;
}
/**
* Returns the value of the AdsOnDeliveryRestrictions property for this object.
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #adsOnDeliveryRestrictions} will return {@link AdsOnDeliveryRestrictions#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #adsOnDeliveryRestrictionsAsString}.
*
*
* @return The value of the AdsOnDeliveryRestrictions property for this object.
* @see AdsOnDeliveryRestrictions
*/
public final AdsOnDeliveryRestrictions adsOnDeliveryRestrictions() {
return AdsOnDeliveryRestrictions.fromValue(adsOnDeliveryRestrictions);
}
/**
* Returns the value of the AdsOnDeliveryRestrictions property for this object.
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #adsOnDeliveryRestrictions} will return {@link AdsOnDeliveryRestrictions#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #adsOnDeliveryRestrictionsAsString}.
*
*
* @return The value of the AdsOnDeliveryRestrictions property for this object.
* @see AdsOnDeliveryRestrictions
*/
public final String adsOnDeliveryRestrictionsAsString() {
return adsOnDeliveryRestrictions;
}
/**
* Returns the value of the Encryption property for this object.
*
* @return The value of the Encryption property for this object.
*/
public final HlsEncryption encryption() {
return encryption;
}
/**
* When enabled, MediaPackage passes through digital video broadcasting (DVB) subtitles into the output.
*
* @return When enabled, MediaPackage passes through digital video broadcasting (DVB) subtitles into the output.
*/
public final Boolean includeDvbSubtitles() {
return includeDvbSubtitles;
}
/**
* When enabled, an I-Frame only stream will be included in the output.
*
* @return When enabled, an I-Frame only stream will be included in the output.
*/
public final Boolean includeIframeOnlyStream() {
return includeIframeOnlyStream;
}
/**
* The HTTP Live Streaming (HLS) playlist type. When either "EVENT" or "VOD" is specified, a corresponding
* EXT-X-PLAYLIST-TYPE entry will be included in the media playlist.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #playlistType} will
* return {@link PlaylistType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #playlistTypeAsString}.
*
*
* @return The HTTP Live Streaming (HLS) playlist type. When either "EVENT" or "VOD" is specified, a corresponding
* EXT-X-PLAYLIST-TYPE entry will be included in the media playlist.
* @see PlaylistType
*/
public final PlaylistType playlistType() {
return PlaylistType.fromValue(playlistType);
}
/**
* The HTTP Live Streaming (HLS) playlist type. When either "EVENT" or "VOD" is specified, a corresponding
* EXT-X-PLAYLIST-TYPE entry will be included in the media playlist.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #playlistType} will
* return {@link PlaylistType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #playlistTypeAsString}.
*
*
* @return The HTTP Live Streaming (HLS) playlist type. When either "EVENT" or "VOD" is specified, a corresponding
* EXT-X-PLAYLIST-TYPE entry will be included in the media playlist.
* @see PlaylistType
*/
public final String playlistTypeAsString() {
return playlistType;
}
/**
* Time window (in seconds) contained in each parent manifest.
*
* @return Time window (in seconds) contained in each parent manifest.
*/
public final Integer playlistWindowSeconds() {
return playlistWindowSeconds;
}
/**
* The interval (in seconds) between each EXT-X-PROGRAM-DATE-TIME tag inserted into manifests. Additionally, when an
* interval is specified ID3Timed Metadata messages will be generated every 5 seconds using the ingest time of the
* content. If the interval is not specified, or set to 0, then no EXT-X-PROGRAM-DATE-TIME tags will be inserted
* into manifests and no ID3Timed Metadata messages will be generated. Note that irrespective of this parameter, if
* any ID3 Timed Metadata is found in HTTP Live Streaming (HLS) input, it will be passed through to HLS output.
*
* @return The interval (in seconds) between each EXT-X-PROGRAM-DATE-TIME tag inserted into manifests. Additionally,
* when an interval is specified ID3Timed Metadata messages will be generated every 5 seconds using the
* ingest time of the content. If the interval is not specified, or set to 0, then no
* EXT-X-PROGRAM-DATE-TIME tags will be inserted into manifests and no ID3Timed Metadata messages will be
* generated. Note that irrespective of this parameter, if any ID3 Timed Metadata is found in HTTP Live
* Streaming (HLS) input, it will be passed through to HLS output.
*/
public final Integer programDateTimeIntervalSeconds() {
return programDateTimeIntervalSeconds;
}
/**
* Duration (in seconds) of each fragment. Actual fragments will be rounded to the nearest multiple of the source
* fragment duration.
*
* @return Duration (in seconds) of each fragment. Actual fragments will be rounded to the nearest multiple of the
* source fragment duration.
*/
public final Integer segmentDurationSeconds() {
return segmentDurationSeconds;
}
/**
* Returns the value of the StreamSelection property for this object.
*
* @return The value of the StreamSelection property for this object.
*/
public final StreamSelection streamSelection() {
return streamSelection;
}
/**
* When enabled, audio streams will be placed in rendition groups in the output.
*
* @return When enabled, audio streams will be placed in rendition groups in the output.
*/
public final Boolean useAudioRenditionGroup() {
return useAudioRenditionGroup;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(adMarkersAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasAdTriggers() ? adTriggersAsStrings() : null);
hashCode = 31 * hashCode + Objects.hashCode(adsOnDeliveryRestrictionsAsString());
hashCode = 31 * hashCode + Objects.hashCode(encryption());
hashCode = 31 * hashCode + Objects.hashCode(includeDvbSubtitles());
hashCode = 31 * hashCode + Objects.hashCode(includeIframeOnlyStream());
hashCode = 31 * hashCode + Objects.hashCode(playlistTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(playlistWindowSeconds());
hashCode = 31 * hashCode + Objects.hashCode(programDateTimeIntervalSeconds());
hashCode = 31 * hashCode + Objects.hashCode(segmentDurationSeconds());
hashCode = 31 * hashCode + Objects.hashCode(streamSelection());
hashCode = 31 * hashCode + Objects.hashCode(useAudioRenditionGroup());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof HlsPackage)) {
return false;
}
HlsPackage other = (HlsPackage) obj;
return Objects.equals(adMarkersAsString(), other.adMarkersAsString()) && hasAdTriggers() == other.hasAdTriggers()
&& Objects.equals(adTriggersAsStrings(), other.adTriggersAsStrings())
&& Objects.equals(adsOnDeliveryRestrictionsAsString(), other.adsOnDeliveryRestrictionsAsString())
&& Objects.equals(encryption(), other.encryption())
&& Objects.equals(includeDvbSubtitles(), other.includeDvbSubtitles())
&& Objects.equals(includeIframeOnlyStream(), other.includeIframeOnlyStream())
&& Objects.equals(playlistTypeAsString(), other.playlistTypeAsString())
&& Objects.equals(playlistWindowSeconds(), other.playlistWindowSeconds())
&& Objects.equals(programDateTimeIntervalSeconds(), other.programDateTimeIntervalSeconds())
&& Objects.equals(segmentDurationSeconds(), other.segmentDurationSeconds())
&& Objects.equals(streamSelection(), other.streamSelection())
&& Objects.equals(useAudioRenditionGroup(), other.useAudioRenditionGroup());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("HlsPackage").add("AdMarkers", adMarkersAsString())
.add("AdTriggers", hasAdTriggers() ? adTriggersAsStrings() : null)
.add("AdsOnDeliveryRestrictions", adsOnDeliveryRestrictionsAsString()).add("Encryption", encryption())
.add("IncludeDvbSubtitles", includeDvbSubtitles()).add("IncludeIframeOnlyStream", includeIframeOnlyStream())
.add("PlaylistType", playlistTypeAsString()).add("PlaylistWindowSeconds", playlistWindowSeconds())
.add("ProgramDateTimeIntervalSeconds", programDateTimeIntervalSeconds())
.add("SegmentDurationSeconds", segmentDurationSeconds()).add("StreamSelection", streamSelection())
.add("UseAudioRenditionGroup", useAudioRenditionGroup()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AdMarkers":
return Optional.ofNullable(clazz.cast(adMarkersAsString()));
case "AdTriggers":
return Optional.ofNullable(clazz.cast(adTriggersAsStrings()));
case "AdsOnDeliveryRestrictions":
return Optional.ofNullable(clazz.cast(adsOnDeliveryRestrictionsAsString()));
case "Encryption":
return Optional.ofNullable(clazz.cast(encryption()));
case "IncludeDvbSubtitles":
return Optional.ofNullable(clazz.cast(includeDvbSubtitles()));
case "IncludeIframeOnlyStream":
return Optional.ofNullable(clazz.cast(includeIframeOnlyStream()));
case "PlaylistType":
return Optional.ofNullable(clazz.cast(playlistTypeAsString()));
case "PlaylistWindowSeconds":
return Optional.ofNullable(clazz.cast(playlistWindowSeconds()));
case "ProgramDateTimeIntervalSeconds":
return Optional.ofNullable(clazz.cast(programDateTimeIntervalSeconds()));
case "SegmentDurationSeconds":
return Optional.ofNullable(clazz.cast(segmentDurationSeconds()));
case "StreamSelection":
return Optional.ofNullable(clazz.cast(streamSelection()));
case "UseAudioRenditionGroup":
return Optional.ofNullable(clazz.cast(useAudioRenditionGroup()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function