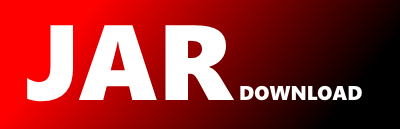
software.amazon.awssdk.services.mediapackage.DefaultMediaPackageAsyncClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mediapackage Show documentation
Show all versions of mediapackage Show documentation
The AWS Java SDK for AWS Elemental MediaPackage module holds the client classes that are used for
communicating
with AWS Elemental MediaPackage Service
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.mediapackage;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.AwsProtocolMetadata;
import software.amazon.awssdk.awscore.internal.AwsServiceProtocol;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkPlugin;
import software.amazon.awssdk.core.SdkRequest;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.services.mediapackage.internal.MediaPackageServiceClientConfigurationBuilder;
import software.amazon.awssdk.services.mediapackage.model.ConfigureLogsRequest;
import software.amazon.awssdk.services.mediapackage.model.ConfigureLogsResponse;
import software.amazon.awssdk.services.mediapackage.model.CreateChannelRequest;
import software.amazon.awssdk.services.mediapackage.model.CreateChannelResponse;
import software.amazon.awssdk.services.mediapackage.model.CreateHarvestJobRequest;
import software.amazon.awssdk.services.mediapackage.model.CreateHarvestJobResponse;
import software.amazon.awssdk.services.mediapackage.model.CreateOriginEndpointRequest;
import software.amazon.awssdk.services.mediapackage.model.CreateOriginEndpointResponse;
import software.amazon.awssdk.services.mediapackage.model.DeleteChannelRequest;
import software.amazon.awssdk.services.mediapackage.model.DeleteChannelResponse;
import software.amazon.awssdk.services.mediapackage.model.DeleteOriginEndpointRequest;
import software.amazon.awssdk.services.mediapackage.model.DeleteOriginEndpointResponse;
import software.amazon.awssdk.services.mediapackage.model.DescribeChannelRequest;
import software.amazon.awssdk.services.mediapackage.model.DescribeChannelResponse;
import software.amazon.awssdk.services.mediapackage.model.DescribeHarvestJobRequest;
import software.amazon.awssdk.services.mediapackage.model.DescribeHarvestJobResponse;
import software.amazon.awssdk.services.mediapackage.model.DescribeOriginEndpointRequest;
import software.amazon.awssdk.services.mediapackage.model.DescribeOriginEndpointResponse;
import software.amazon.awssdk.services.mediapackage.model.ForbiddenException;
import software.amazon.awssdk.services.mediapackage.model.InternalServerErrorException;
import software.amazon.awssdk.services.mediapackage.model.ListChannelsRequest;
import software.amazon.awssdk.services.mediapackage.model.ListChannelsResponse;
import software.amazon.awssdk.services.mediapackage.model.ListHarvestJobsRequest;
import software.amazon.awssdk.services.mediapackage.model.ListHarvestJobsResponse;
import software.amazon.awssdk.services.mediapackage.model.ListOriginEndpointsRequest;
import software.amazon.awssdk.services.mediapackage.model.ListOriginEndpointsResponse;
import software.amazon.awssdk.services.mediapackage.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.mediapackage.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.mediapackage.model.MediaPackageException;
import software.amazon.awssdk.services.mediapackage.model.NotFoundException;
import software.amazon.awssdk.services.mediapackage.model.RotateIngestEndpointCredentialsRequest;
import software.amazon.awssdk.services.mediapackage.model.RotateIngestEndpointCredentialsResponse;
import software.amazon.awssdk.services.mediapackage.model.ServiceUnavailableException;
import software.amazon.awssdk.services.mediapackage.model.TagResourceRequest;
import software.amazon.awssdk.services.mediapackage.model.TagResourceResponse;
import software.amazon.awssdk.services.mediapackage.model.TooManyRequestsException;
import software.amazon.awssdk.services.mediapackage.model.UnprocessableEntityException;
import software.amazon.awssdk.services.mediapackage.model.UntagResourceRequest;
import software.amazon.awssdk.services.mediapackage.model.UntagResourceResponse;
import software.amazon.awssdk.services.mediapackage.model.UpdateChannelRequest;
import software.amazon.awssdk.services.mediapackage.model.UpdateChannelResponse;
import software.amazon.awssdk.services.mediapackage.model.UpdateOriginEndpointRequest;
import software.amazon.awssdk.services.mediapackage.model.UpdateOriginEndpointResponse;
import software.amazon.awssdk.services.mediapackage.transform.ConfigureLogsRequestMarshaller;
import software.amazon.awssdk.services.mediapackage.transform.CreateChannelRequestMarshaller;
import software.amazon.awssdk.services.mediapackage.transform.CreateHarvestJobRequestMarshaller;
import software.amazon.awssdk.services.mediapackage.transform.CreateOriginEndpointRequestMarshaller;
import software.amazon.awssdk.services.mediapackage.transform.DeleteChannelRequestMarshaller;
import software.amazon.awssdk.services.mediapackage.transform.DeleteOriginEndpointRequestMarshaller;
import software.amazon.awssdk.services.mediapackage.transform.DescribeChannelRequestMarshaller;
import software.amazon.awssdk.services.mediapackage.transform.DescribeHarvestJobRequestMarshaller;
import software.amazon.awssdk.services.mediapackage.transform.DescribeOriginEndpointRequestMarshaller;
import software.amazon.awssdk.services.mediapackage.transform.ListChannelsRequestMarshaller;
import software.amazon.awssdk.services.mediapackage.transform.ListHarvestJobsRequestMarshaller;
import software.amazon.awssdk.services.mediapackage.transform.ListOriginEndpointsRequestMarshaller;
import software.amazon.awssdk.services.mediapackage.transform.ListTagsForResourceRequestMarshaller;
import software.amazon.awssdk.services.mediapackage.transform.RotateIngestEndpointCredentialsRequestMarshaller;
import software.amazon.awssdk.services.mediapackage.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.mediapackage.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.mediapackage.transform.UpdateChannelRequestMarshaller;
import software.amazon.awssdk.services.mediapackage.transform.UpdateOriginEndpointRequestMarshaller;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link MediaPackageAsyncClient}.
*
* @see MediaPackageAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultMediaPackageAsyncClient implements MediaPackageAsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultMediaPackageAsyncClient.class);
private static final AwsProtocolMetadata protocolMetadata = AwsProtocolMetadata.builder()
.serviceProtocol(AwsServiceProtocol.REST_JSON).build();
private final AsyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultMediaPackageAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration.toBuilder().option(SdkClientOption.SDK_CLIENT, this).build();
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
/**
* Changes the Channel's properities to configure log subscription
*
* @param configureLogsRequest
* the option to configure log subscription.
* @return A Java Future containing the result of the ConfigureLogs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.ConfigureLogs
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture configureLogs(ConfigureLogsRequest configureLogsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(configureLogsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, configureLogsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MediaPackage");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ConfigureLogs");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ConfigureLogsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ConfigureLogs").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ConfigureLogsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(configureLogsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
* Creates a new Channel.
*
* @param createChannelRequest
* A new Channel configuration.
* @return A Java Future containing the result of the CreateChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.CreateChannel
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture createChannel(CreateChannelRequest createChannelRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createChannelRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createChannelRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MediaPackage");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateChannel");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateChannelResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateChannel").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateChannelRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createChannelRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
* Creates a new HarvestJob record.
*
* @param createHarvestJobRequest
* Configuration parameters used to create a new HarvestJob.
* @return A Java Future containing the result of the CreateHarvestJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.CreateHarvestJob
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture createHarvestJob(CreateHarvestJobRequest createHarvestJobRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createHarvestJobRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createHarvestJobRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MediaPackage");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateHarvestJob");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateHarvestJobResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateHarvestJob").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateHarvestJobRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createHarvestJobRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
* Creates a new OriginEndpoint record.
*
* @param createOriginEndpointRequest
* Configuration parameters used to create a new OriginEndpoint.
* @return A Java Future containing the result of the CreateOriginEndpoint operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.CreateOriginEndpoint
* @see AWS API Documentation
*/
@Override
public CompletableFuture createOriginEndpoint(
CreateOriginEndpointRequest createOriginEndpointRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createOriginEndpointRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createOriginEndpointRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MediaPackage");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateOriginEndpoint");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateOriginEndpointResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateOriginEndpoint").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateOriginEndpointRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createOriginEndpointRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
* Deletes an existing Channel.
*
* @param deleteChannelRequest
* @return A Java Future containing the result of the DeleteChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.DeleteChannel
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteChannel(DeleteChannelRequest deleteChannelRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteChannelRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteChannelRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MediaPackage");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteChannel");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteChannelResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteChannel").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteChannelRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteChannelRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
* Deletes an existing OriginEndpoint.
*
* @param deleteOriginEndpointRequest
* @return A Java Future containing the result of the DeleteOriginEndpoint operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.DeleteOriginEndpoint
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteOriginEndpoint(
DeleteOriginEndpointRequest deleteOriginEndpointRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteOriginEndpointRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteOriginEndpointRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MediaPackage");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteOriginEndpoint");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteOriginEndpointResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteOriginEndpoint").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteOriginEndpointRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteOriginEndpointRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
* Gets details about a Channel.
*
* @param describeChannelRequest
* @return A Java Future containing the result of the DescribeChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.DescribeChannel
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describeChannel(DescribeChannelRequest describeChannelRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeChannelRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeChannelRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MediaPackage");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeChannel");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeChannelResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeChannel").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeChannelRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeChannelRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
* Gets details about an existing HarvestJob.
*
* @param describeHarvestJobRequest
* @return A Java Future containing the result of the DescribeHarvestJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.DescribeHarvestJob
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeHarvestJob(DescribeHarvestJobRequest describeHarvestJobRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeHarvestJobRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeHarvestJobRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MediaPackage");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeHarvestJob");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeHarvestJobResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeHarvestJob").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeHarvestJobRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeHarvestJobRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
* Gets details about an existing OriginEndpoint.
*
* @param describeOriginEndpointRequest
* @return A Java Future containing the result of the DescribeOriginEndpoint operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.DescribeOriginEndpoint
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeOriginEndpoint(
DescribeOriginEndpointRequest describeOriginEndpointRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeOriginEndpointRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeOriginEndpointRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MediaPackage");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeOriginEndpoint");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeOriginEndpointResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeOriginEndpoint").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeOriginEndpointRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeOriginEndpointRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
* Returns a collection of Channels.
*
* @param listChannelsRequest
* @return A Java Future containing the result of the ListChannels operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.ListChannels
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listChannels(ListChannelsRequest listChannelsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listChannelsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listChannelsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MediaPackage");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListChannels");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListChannelsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListChannels").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListChannelsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listChannelsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
* Returns a collection of HarvestJob records.
*
* @param listHarvestJobsRequest
* @return A Java Future containing the result of the ListHarvestJobs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.ListHarvestJobs
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listHarvestJobs(ListHarvestJobsRequest listHarvestJobsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listHarvestJobsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listHarvestJobsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MediaPackage");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListHarvestJobs");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListHarvestJobsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListHarvestJobs").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListHarvestJobsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listHarvestJobsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
* Returns a collection of OriginEndpoint records.
*
* @param listOriginEndpointsRequest
* @return A Java Future containing the result of the ListOriginEndpoints operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.ListOriginEndpoints
* @see AWS API Documentation
*/
@Override
public CompletableFuture listOriginEndpoints(
ListOriginEndpointsRequest listOriginEndpointsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listOriginEndpointsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listOriginEndpointsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MediaPackage");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListOriginEndpoints");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListOriginEndpointsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListOriginEndpoints").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListOriginEndpointsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listOriginEndpointsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
* Invokes the ListTagsForResource operation asynchronously.
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
@Override
public CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listTagsForResourceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listTagsForResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MediaPackage");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListTagsForResource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListTagsForResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListTagsForResource").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListTagsForResourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listTagsForResourceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
* Rotate the IngestEndpoint's username and password, as specified by the IngestEndpoint's id.
*
* @param rotateIngestEndpointCredentialsRequest
* @return A Java Future containing the result of the RotateIngestEndpointCredentials operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.RotateIngestEndpointCredentials
* @see AWS API Documentation
*/
@Override
public CompletableFuture rotateIngestEndpointCredentials(
RotateIngestEndpointCredentialsRequest rotateIngestEndpointCredentialsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(rotateIngestEndpointCredentialsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
rotateIngestEndpointCredentialsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MediaPackage");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RotateIngestEndpointCredentials");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, RotateIngestEndpointCredentialsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("RotateIngestEndpointCredentials").withProtocolMetadata(protocolMetadata)
.withMarshaller(new RotateIngestEndpointCredentialsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(rotateIngestEndpointCredentialsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
* Invokes the TagResource operation asynchronously.
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.TagResource
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(tagResourceRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, tagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MediaPackage");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "TagResource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
TagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("TagResource").withProtocolMetadata(protocolMetadata)
.withMarshaller(new TagResourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(tagResourceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
* Invokes the UntagResource operation asynchronously.
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.UntagResource
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(untagResourceRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, untagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MediaPackage");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UntagResource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UntagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("UntagResource").withProtocolMetadata(protocolMetadata)
.withMarshaller(new UntagResourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(untagResourceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
* Updates an existing Channel.
*
* @param updateChannelRequest
* Configuration parameters used to update the Channel.
* @return A Java Future containing the result of the UpdateChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.UpdateChannel
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture updateChannel(UpdateChannelRequest updateChannelRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(updateChannelRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateChannelRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MediaPackage");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateChannel");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UpdateChannelResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("UpdateChannel").withProtocolMetadata(protocolMetadata)
.withMarshaller(new UpdateChannelRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(updateChannelRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
* Updates an existing OriginEndpoint.
*
* @param updateOriginEndpointRequest
* Configuration parameters used to update an existing OriginEndpoint.
* @return A Java Future containing the result of the UpdateOriginEndpoint operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.UpdateOriginEndpoint
* @see AWS API Documentation
*/
@Override
public CompletableFuture updateOriginEndpoint(
UpdateOriginEndpointRequest updateOriginEndpointRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(updateOriginEndpointRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateOriginEndpointRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MediaPackage");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateOriginEndpoint");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, UpdateOriginEndpointResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("UpdateOriginEndpoint").withProtocolMetadata(protocolMetadata)
.withMarshaller(new UpdateOriginEndpointRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(updateOriginEndpointRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
@Override
public final MediaPackageServiceClientConfiguration serviceClientConfiguration() {
return new MediaPackageServiceClientConfigurationBuilder(this.clientConfiguration.toBuilder()).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
private > T init(T builder) {
return builder
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(MediaPackageException::builder)
.protocol(AwsJsonProtocol.REST_JSON)
.protocolVersion("1.1")
.registerModeledException(
ExceptionMetadata.builder().errorCode("UnprocessableEntityException")
.exceptionBuilderSupplier(UnprocessableEntityException::builder).httpStatusCode(422).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("NotFoundException")
.exceptionBuilderSupplier(NotFoundException::builder).httpStatusCode(404).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ServiceUnavailableException")
.exceptionBuilderSupplier(ServiceUnavailableException::builder).httpStatusCode(503).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ForbiddenException")
.exceptionBuilderSupplier(ForbiddenException::builder).httpStatusCode(403).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TooManyRequestsException")
.exceptionBuilderSupplier(TooManyRequestsException::builder).httpStatusCode(429).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InternalServerErrorException")
.exceptionBuilderSupplier(InternalServerErrorException::builder).httpStatusCode(500).build());
}
private static List resolveMetricPublishers(SdkClientConfiguration clientConfiguration,
RequestOverrideConfiguration requestOverrideConfiguration) {
List publishers = null;
if (requestOverrideConfiguration != null) {
publishers = requestOverrideConfiguration.metricPublishers();
}
if (publishers == null || publishers.isEmpty()) {
publishers = clientConfiguration.option(SdkClientOption.METRIC_PUBLISHERS);
}
if (publishers == null) {
publishers = Collections.emptyList();
}
return publishers;
}
private SdkClientConfiguration updateSdkClientConfiguration(SdkRequest request, SdkClientConfiguration clientConfiguration) {
List plugins = request.overrideConfiguration().map(c -> c.plugins()).orElse(Collections.emptyList());
SdkClientConfiguration.Builder configuration = clientConfiguration.toBuilder();
if (plugins.isEmpty()) {
return configuration.build();
}
MediaPackageServiceClientConfigurationBuilder serviceConfigBuilder = new MediaPackageServiceClientConfigurationBuilder(
configuration);
for (SdkPlugin plugin : plugins) {
plugin.configureClient(serviceConfigBuilder);
}
return configuration.build();
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata) {
return protocolFactory.createErrorResponseHandler(operationMetadata);
}
@Override
public void close() {
clientHandler.close();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy