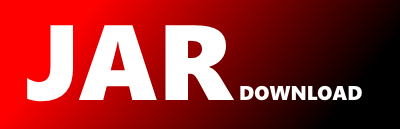
software.amazon.awssdk.services.mediapackage.model.CreateHarvestJobRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mediapackage Show documentation
Show all versions of mediapackage Show documentation
The AWS Java SDK for AWS Elemental MediaPackage module holds the client classes that are used for
communicating
with AWS Elemental MediaPackage Service
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.mediapackage.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
* Configuration parameters used to create a new HarvestJob.
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateHarvestJobRequest extends MediaPackageRequest implements
ToCopyableBuilder {
private static final SdkField END_TIME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EndTime").getter(getter(CreateHarvestJobRequest::endTime)).setter(setter(Builder::endTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("endTime").build()).build();
private static final SdkField ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Id")
.getter(getter(CreateHarvestJobRequest::id)).setter(setter(Builder::id))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("id").build()).build();
private static final SdkField ORIGIN_ENDPOINT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OriginEndpointId").getter(getter(CreateHarvestJobRequest::originEndpointId))
.setter(setter(Builder::originEndpointId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("originEndpointId").build()).build();
private static final SdkField S3_DESTINATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("S3Destination")
.getter(getter(CreateHarvestJobRequest::s3Destination)).setter(setter(Builder::s3Destination))
.constructor(S3Destination::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("s3Destination").build()).build();
private static final SdkField START_TIME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StartTime").getter(getter(CreateHarvestJobRequest::startTime)).setter(setter(Builder::startTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("startTime").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(END_TIME_FIELD, ID_FIELD,
ORIGIN_ENDPOINT_ID_FIELD, S3_DESTINATION_FIELD, START_TIME_FIELD));
private final String endTime;
private final String id;
private final String originEndpointId;
private final S3Destination s3Destination;
private final String startTime;
private CreateHarvestJobRequest(BuilderImpl builder) {
super(builder);
this.endTime = builder.endTime;
this.id = builder.id;
this.originEndpointId = builder.originEndpointId;
this.s3Destination = builder.s3Destination;
this.startTime = builder.startTime;
}
/**
* The end of the time-window which will be harvested
*
* @return The end of the time-window which will be harvested
*/
public final String endTime() {
return endTime;
}
/**
* The ID of the HarvestJob. The ID must be unique within the region and it cannot be changed after the HarvestJob
* is submitted
*
* @return The ID of the HarvestJob. The ID must be unique within the region and it cannot be changed after the
* HarvestJob is submitted
*/
public final String id() {
return id;
}
/**
* The ID of the OriginEndpoint that the HarvestJob will harvest from. This cannot be changed after the HarvestJob
* is submitted.
*
* @return The ID of the OriginEndpoint that the HarvestJob will harvest from. This cannot be changed after the
* HarvestJob is submitted.
*/
public final String originEndpointId() {
return originEndpointId;
}
/**
* Returns the value of the S3Destination property for this object.
*
* @return The value of the S3Destination property for this object.
*/
public final S3Destination s3Destination() {
return s3Destination;
}
/**
* The start of the time-window which will be harvested
*
* @return The start of the time-window which will be harvested
*/
public final String startTime() {
return startTime;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(endTime());
hashCode = 31 * hashCode + Objects.hashCode(id());
hashCode = 31 * hashCode + Objects.hashCode(originEndpointId());
hashCode = 31 * hashCode + Objects.hashCode(s3Destination());
hashCode = 31 * hashCode + Objects.hashCode(startTime());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateHarvestJobRequest)) {
return false;
}
CreateHarvestJobRequest other = (CreateHarvestJobRequest) obj;
return Objects.equals(endTime(), other.endTime()) && Objects.equals(id(), other.id())
&& Objects.equals(originEndpointId(), other.originEndpointId())
&& Objects.equals(s3Destination(), other.s3Destination()) && Objects.equals(startTime(), other.startTime());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateHarvestJobRequest").add("EndTime", endTime()).add("Id", id())
.add("OriginEndpointId", originEndpointId()).add("S3Destination", s3Destination()).add("StartTime", startTime())
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "EndTime":
return Optional.ofNullable(clazz.cast(endTime()));
case "Id":
return Optional.ofNullable(clazz.cast(id()));
case "OriginEndpointId":
return Optional.ofNullable(clazz.cast(originEndpointId()));
case "S3Destination":
return Optional.ofNullable(clazz.cast(s3Destination()));
case "StartTime":
return Optional.ofNullable(clazz.cast(startTime()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy