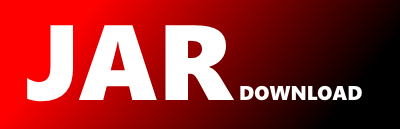
software.amazon.awssdk.services.mediapackage.MediaPackageAsyncClient Maven / Gradle / Ivy
Show all versions of mediapackage Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.mediapackage;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.services.mediapackage.model.ConfigureLogsRequest;
import software.amazon.awssdk.services.mediapackage.model.ConfigureLogsResponse;
import software.amazon.awssdk.services.mediapackage.model.CreateChannelRequest;
import software.amazon.awssdk.services.mediapackage.model.CreateChannelResponse;
import software.amazon.awssdk.services.mediapackage.model.CreateHarvestJobRequest;
import software.amazon.awssdk.services.mediapackage.model.CreateHarvestJobResponse;
import software.amazon.awssdk.services.mediapackage.model.CreateOriginEndpointRequest;
import software.amazon.awssdk.services.mediapackage.model.CreateOriginEndpointResponse;
import software.amazon.awssdk.services.mediapackage.model.DeleteChannelRequest;
import software.amazon.awssdk.services.mediapackage.model.DeleteChannelResponse;
import software.amazon.awssdk.services.mediapackage.model.DeleteOriginEndpointRequest;
import software.amazon.awssdk.services.mediapackage.model.DeleteOriginEndpointResponse;
import software.amazon.awssdk.services.mediapackage.model.DescribeChannelRequest;
import software.amazon.awssdk.services.mediapackage.model.DescribeChannelResponse;
import software.amazon.awssdk.services.mediapackage.model.DescribeHarvestJobRequest;
import software.amazon.awssdk.services.mediapackage.model.DescribeHarvestJobResponse;
import software.amazon.awssdk.services.mediapackage.model.DescribeOriginEndpointRequest;
import software.amazon.awssdk.services.mediapackage.model.DescribeOriginEndpointResponse;
import software.amazon.awssdk.services.mediapackage.model.ListChannelsRequest;
import software.amazon.awssdk.services.mediapackage.model.ListChannelsResponse;
import software.amazon.awssdk.services.mediapackage.model.ListHarvestJobsRequest;
import software.amazon.awssdk.services.mediapackage.model.ListHarvestJobsResponse;
import software.amazon.awssdk.services.mediapackage.model.ListOriginEndpointsRequest;
import software.amazon.awssdk.services.mediapackage.model.ListOriginEndpointsResponse;
import software.amazon.awssdk.services.mediapackage.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.mediapackage.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.mediapackage.model.RotateIngestEndpointCredentialsRequest;
import software.amazon.awssdk.services.mediapackage.model.RotateIngestEndpointCredentialsResponse;
import software.amazon.awssdk.services.mediapackage.model.TagResourceRequest;
import software.amazon.awssdk.services.mediapackage.model.TagResourceResponse;
import software.amazon.awssdk.services.mediapackage.model.UntagResourceRequest;
import software.amazon.awssdk.services.mediapackage.model.UntagResourceResponse;
import software.amazon.awssdk.services.mediapackage.model.UpdateChannelRequest;
import software.amazon.awssdk.services.mediapackage.model.UpdateChannelResponse;
import software.amazon.awssdk.services.mediapackage.model.UpdateOriginEndpointRequest;
import software.amazon.awssdk.services.mediapackage.model.UpdateOriginEndpointResponse;
import software.amazon.awssdk.services.mediapackage.paginators.ListChannelsPublisher;
import software.amazon.awssdk.services.mediapackage.paginators.ListHarvestJobsPublisher;
import software.amazon.awssdk.services.mediapackage.paginators.ListOriginEndpointsPublisher;
/**
* Service client for accessing MediaPackage asynchronously. This can be created using the static {@link #builder()}
* method.The asynchronous client performs non-blocking I/O when configured with any {@code SdkAsyncHttpClient}
* supported in the SDK. However, full non-blocking is not guaranteed as the async client may perform blocking calls in
* some cases such as credentials retrieval and endpoint discovery as part of the async API call.
*
* AWS Elemental MediaPackage
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface MediaPackageAsyncClient extends AwsClient {
String SERVICE_NAME = "mediapackage";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "mediapackage";
/**
* Changes the Channel's properities to configure log subscription
*
* @param configureLogsRequest
* the option to configure log subscription.
* @return A Java Future containing the result of the ConfigureLogs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.ConfigureLogs
* @see AWS
* API Documentation
*/
default CompletableFuture configureLogs(ConfigureLogsRequest configureLogsRequest) {
throw new UnsupportedOperationException();
}
/**
* Changes the Channel's properities to configure log subscription
*
* This is a convenience which creates an instance of the {@link ConfigureLogsRequest.Builder} avoiding the need to
* create one manually via {@link ConfigureLogsRequest#builder()}
*
*
* @param configureLogsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.mediapackage.model.ConfigureLogsRequest.Builder} to create a
* request. the option to configure log subscription.
* @return A Java Future containing the result of the ConfigureLogs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.ConfigureLogs
* @see AWS
* API Documentation
*/
default CompletableFuture configureLogs(Consumer configureLogsRequest) {
return configureLogs(ConfigureLogsRequest.builder().applyMutation(configureLogsRequest).build());
}
/**
* Creates a new Channel.
*
* @param createChannelRequest
* A new Channel configuration.
* @return A Java Future containing the result of the CreateChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.CreateChannel
* @see AWS
* API Documentation
*/
default CompletableFuture createChannel(CreateChannelRequest createChannelRequest) {
throw new UnsupportedOperationException();
}
/**
* Creates a new Channel.
*
* This is a convenience which creates an instance of the {@link CreateChannelRequest.Builder} avoiding the need to
* create one manually via {@link CreateChannelRequest#builder()}
*
*
* @param createChannelRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.mediapackage.model.CreateChannelRequest.Builder} to create a
* request. A new Channel configuration.
* @return A Java Future containing the result of the CreateChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.CreateChannel
* @see AWS
* API Documentation
*/
default CompletableFuture createChannel(Consumer createChannelRequest) {
return createChannel(CreateChannelRequest.builder().applyMutation(createChannelRequest).build());
}
/**
* Creates a new HarvestJob record.
*
* @param createHarvestJobRequest
* Configuration parameters used to create a new HarvestJob.
* @return A Java Future containing the result of the CreateHarvestJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.CreateHarvestJob
* @see AWS
* API Documentation
*/
default CompletableFuture createHarvestJob(CreateHarvestJobRequest createHarvestJobRequest) {
throw new UnsupportedOperationException();
}
/**
* Creates a new HarvestJob record.
*
* This is a convenience which creates an instance of the {@link CreateHarvestJobRequest.Builder} avoiding the need
* to create one manually via {@link CreateHarvestJobRequest#builder()}
*
*
* @param createHarvestJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.mediapackage.model.CreateHarvestJobRequest.Builder} to create a
* request. Configuration parameters used to create a new HarvestJob.
* @return A Java Future containing the result of the CreateHarvestJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.CreateHarvestJob
* @see AWS
* API Documentation
*/
default CompletableFuture createHarvestJob(
Consumer createHarvestJobRequest) {
return createHarvestJob(CreateHarvestJobRequest.builder().applyMutation(createHarvestJobRequest).build());
}
/**
* Creates a new OriginEndpoint record.
*
* @param createOriginEndpointRequest
* Configuration parameters used to create a new OriginEndpoint.
* @return A Java Future containing the result of the CreateOriginEndpoint operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.CreateOriginEndpoint
* @see AWS API Documentation
*/
default CompletableFuture createOriginEndpoint(
CreateOriginEndpointRequest createOriginEndpointRequest) {
throw new UnsupportedOperationException();
}
/**
* Creates a new OriginEndpoint record.
*
* This is a convenience which creates an instance of the {@link CreateOriginEndpointRequest.Builder} avoiding the
* need to create one manually via {@link CreateOriginEndpointRequest#builder()}
*
*
* @param createOriginEndpointRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.mediapackage.model.CreateOriginEndpointRequest.Builder} to create a
* request. Configuration parameters used to create a new OriginEndpoint.
* @return A Java Future containing the result of the CreateOriginEndpoint operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.CreateOriginEndpoint
* @see AWS API Documentation
*/
default CompletableFuture createOriginEndpoint(
Consumer createOriginEndpointRequest) {
return createOriginEndpoint(CreateOriginEndpointRequest.builder().applyMutation(createOriginEndpointRequest).build());
}
/**
* Deletes an existing Channel.
*
* @param deleteChannelRequest
* @return A Java Future containing the result of the DeleteChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.DeleteChannel
* @see AWS
* API Documentation
*/
default CompletableFuture deleteChannel(DeleteChannelRequest deleteChannelRequest) {
throw new UnsupportedOperationException();
}
/**
* Deletes an existing Channel.
*
* This is a convenience which creates an instance of the {@link DeleteChannelRequest.Builder} avoiding the need to
* create one manually via {@link DeleteChannelRequest#builder()}
*
*
* @param deleteChannelRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.mediapackage.model.DeleteChannelRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.DeleteChannel
* @see AWS
* API Documentation
*/
default CompletableFuture deleteChannel(Consumer deleteChannelRequest) {
return deleteChannel(DeleteChannelRequest.builder().applyMutation(deleteChannelRequest).build());
}
/**
* Deletes an existing OriginEndpoint.
*
* @param deleteOriginEndpointRequest
* @return A Java Future containing the result of the DeleteOriginEndpoint operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.DeleteOriginEndpoint
* @see AWS API Documentation
*/
default CompletableFuture deleteOriginEndpoint(
DeleteOriginEndpointRequest deleteOriginEndpointRequest) {
throw new UnsupportedOperationException();
}
/**
* Deletes an existing OriginEndpoint.
*
* This is a convenience which creates an instance of the {@link DeleteOriginEndpointRequest.Builder} avoiding the
* need to create one manually via {@link DeleteOriginEndpointRequest#builder()}
*
*
* @param deleteOriginEndpointRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.mediapackage.model.DeleteOriginEndpointRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteOriginEndpoint operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.DeleteOriginEndpoint
* @see AWS API Documentation
*/
default CompletableFuture deleteOriginEndpoint(
Consumer deleteOriginEndpointRequest) {
return deleteOriginEndpoint(DeleteOriginEndpointRequest.builder().applyMutation(deleteOriginEndpointRequest).build());
}
/**
* Gets details about a Channel.
*
* @param describeChannelRequest
* @return A Java Future containing the result of the DescribeChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.DescribeChannel
* @see AWS
* API Documentation
*/
default CompletableFuture describeChannel(DescribeChannelRequest describeChannelRequest) {
throw new UnsupportedOperationException();
}
/**
* Gets details about a Channel.
*
* This is a convenience which creates an instance of the {@link DescribeChannelRequest.Builder} avoiding the need
* to create one manually via {@link DescribeChannelRequest#builder()}
*
*
* @param describeChannelRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.mediapackage.model.DescribeChannelRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.DescribeChannel
* @see AWS
* API Documentation
*/
default CompletableFuture describeChannel(
Consumer describeChannelRequest) {
return describeChannel(DescribeChannelRequest.builder().applyMutation(describeChannelRequest).build());
}
/**
* Gets details about an existing HarvestJob.
*
* @param describeHarvestJobRequest
* @return A Java Future containing the result of the DescribeHarvestJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.DescribeHarvestJob
* @see AWS API Documentation
*/
default CompletableFuture describeHarvestJob(DescribeHarvestJobRequest describeHarvestJobRequest) {
throw new UnsupportedOperationException();
}
/**
* Gets details about an existing HarvestJob.
*
* This is a convenience which creates an instance of the {@link DescribeHarvestJobRequest.Builder} avoiding the
* need to create one manually via {@link DescribeHarvestJobRequest#builder()}
*
*
* @param describeHarvestJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.mediapackage.model.DescribeHarvestJobRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeHarvestJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.DescribeHarvestJob
* @see AWS API Documentation
*/
default CompletableFuture describeHarvestJob(
Consumer describeHarvestJobRequest) {
return describeHarvestJob(DescribeHarvestJobRequest.builder().applyMutation(describeHarvestJobRequest).build());
}
/**
* Gets details about an existing OriginEndpoint.
*
* @param describeOriginEndpointRequest
* @return A Java Future containing the result of the DescribeOriginEndpoint operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.DescribeOriginEndpoint
* @see AWS API Documentation
*/
default CompletableFuture describeOriginEndpoint(
DescribeOriginEndpointRequest describeOriginEndpointRequest) {
throw new UnsupportedOperationException();
}
/**
* Gets details about an existing OriginEndpoint.
*
* This is a convenience which creates an instance of the {@link DescribeOriginEndpointRequest.Builder} avoiding the
* need to create one manually via {@link DescribeOriginEndpointRequest#builder()}
*
*
* @param describeOriginEndpointRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.mediapackage.model.DescribeOriginEndpointRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the DescribeOriginEndpoint operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.DescribeOriginEndpoint
* @see AWS API Documentation
*/
default CompletableFuture describeOriginEndpoint(
Consumer describeOriginEndpointRequest) {
return describeOriginEndpoint(DescribeOriginEndpointRequest.builder().applyMutation(describeOriginEndpointRequest)
.build());
}
/**
* Returns a collection of Channels.
*
* @param listChannelsRequest
* @return A Java Future containing the result of the ListChannels operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.ListChannels
* @see AWS API
* Documentation
*/
default CompletableFuture listChannels(ListChannelsRequest listChannelsRequest) {
throw new UnsupportedOperationException();
}
/**
* Returns a collection of Channels.
*
* This is a convenience which creates an instance of the {@link ListChannelsRequest.Builder} avoiding the need to
* create one manually via {@link ListChannelsRequest#builder()}
*
*
* @param listChannelsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.mediapackage.model.ListChannelsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListChannels operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.ListChannels
* @see AWS API
* Documentation
*/
default CompletableFuture listChannels(Consumer listChannelsRequest) {
return listChannels(ListChannelsRequest.builder().applyMutation(listChannelsRequest).build());
}
/**
* Returns a collection of Channels.
*
* @return A Java Future containing the result of the ListChannels operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.ListChannels
* @see AWS API
* Documentation
*/
default CompletableFuture listChannels() {
return listChannels(ListChannelsRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #listChannels(software.amazon.awssdk.services.mediapackage.model.ListChannelsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.mediapackage.paginators.ListChannelsPublisher publisher = client.listChannelsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.mediapackage.paginators.ListChannelsPublisher publisher = client.listChannelsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.mediapackage.model.ListChannelsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listChannels(software.amazon.awssdk.services.mediapackage.model.ListChannelsRequest)} operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.ListChannels
* @see AWS API
* Documentation
*/
default ListChannelsPublisher listChannelsPaginator() {
return listChannelsPaginator(ListChannelsRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #listChannels(software.amazon.awssdk.services.mediapackage.model.ListChannelsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.mediapackage.paginators.ListChannelsPublisher publisher = client.listChannelsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.mediapackage.paginators.ListChannelsPublisher publisher = client.listChannelsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.mediapackage.model.ListChannelsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listChannels(software.amazon.awssdk.services.mediapackage.model.ListChannelsRequest)} operation.
*
*
* @param listChannelsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.ListChannels
* @see AWS API
* Documentation
*/
default ListChannelsPublisher listChannelsPaginator(ListChannelsRequest listChannelsRequest) {
return new ListChannelsPublisher(this, listChannelsRequest);
}
/**
*
* This is a variant of
* {@link #listChannels(software.amazon.awssdk.services.mediapackage.model.ListChannelsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.mediapackage.paginators.ListChannelsPublisher publisher = client.listChannelsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.mediapackage.paginators.ListChannelsPublisher publisher = client.listChannelsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.mediapackage.model.ListChannelsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listChannels(software.amazon.awssdk.services.mediapackage.model.ListChannelsRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListChannelsRequest.Builder} avoiding the need to
* create one manually via {@link ListChannelsRequest#builder()}
*
*
* @param listChannelsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.mediapackage.model.ListChannelsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.ListChannels
* @see AWS API
* Documentation
*/
default ListChannelsPublisher listChannelsPaginator(Consumer listChannelsRequest) {
return listChannelsPaginator(ListChannelsRequest.builder().applyMutation(listChannelsRequest).build());
}
/**
* Returns a collection of HarvestJob records.
*
* @param listHarvestJobsRequest
* @return A Java Future containing the result of the ListHarvestJobs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.ListHarvestJobs
* @see AWS
* API Documentation
*/
default CompletableFuture listHarvestJobs(ListHarvestJobsRequest listHarvestJobsRequest) {
throw new UnsupportedOperationException();
}
/**
* Returns a collection of HarvestJob records.
*
* This is a convenience which creates an instance of the {@link ListHarvestJobsRequest.Builder} avoiding the need
* to create one manually via {@link ListHarvestJobsRequest#builder()}
*
*
* @param listHarvestJobsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.mediapackage.model.ListHarvestJobsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListHarvestJobs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.ListHarvestJobs
* @see AWS
* API Documentation
*/
default CompletableFuture listHarvestJobs(
Consumer listHarvestJobsRequest) {
return listHarvestJobs(ListHarvestJobsRequest.builder().applyMutation(listHarvestJobsRequest).build());
}
/**
*
* This is a variant of
* {@link #listHarvestJobs(software.amazon.awssdk.services.mediapackage.model.ListHarvestJobsRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.mediapackage.paginators.ListHarvestJobsPublisher publisher = client.listHarvestJobsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.mediapackage.paginators.ListHarvestJobsPublisher publisher = client.listHarvestJobsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.mediapackage.model.ListHarvestJobsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listHarvestJobs(software.amazon.awssdk.services.mediapackage.model.ListHarvestJobsRequest)}
* operation.
*
*
* @param listHarvestJobsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.ListHarvestJobs
* @see AWS
* API Documentation
*/
default ListHarvestJobsPublisher listHarvestJobsPaginator(ListHarvestJobsRequest listHarvestJobsRequest) {
return new ListHarvestJobsPublisher(this, listHarvestJobsRequest);
}
/**
*
* This is a variant of
* {@link #listHarvestJobs(software.amazon.awssdk.services.mediapackage.model.ListHarvestJobsRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.mediapackage.paginators.ListHarvestJobsPublisher publisher = client.listHarvestJobsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.mediapackage.paginators.ListHarvestJobsPublisher publisher = client.listHarvestJobsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.mediapackage.model.ListHarvestJobsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listHarvestJobs(software.amazon.awssdk.services.mediapackage.model.ListHarvestJobsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListHarvestJobsRequest.Builder} avoiding the need
* to create one manually via {@link ListHarvestJobsRequest#builder()}
*
*
* @param listHarvestJobsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.mediapackage.model.ListHarvestJobsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.ListHarvestJobs
* @see AWS
* API Documentation
*/
default ListHarvestJobsPublisher listHarvestJobsPaginator(Consumer listHarvestJobsRequest) {
return listHarvestJobsPaginator(ListHarvestJobsRequest.builder().applyMutation(listHarvestJobsRequest).build());
}
/**
* Returns a collection of OriginEndpoint records.
*
* @param listOriginEndpointsRequest
* @return A Java Future containing the result of the ListOriginEndpoints operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.ListOriginEndpoints
* @see AWS API Documentation
*/
default CompletableFuture listOriginEndpoints(
ListOriginEndpointsRequest listOriginEndpointsRequest) {
throw new UnsupportedOperationException();
}
/**
* Returns a collection of OriginEndpoint records.
*
* This is a convenience which creates an instance of the {@link ListOriginEndpointsRequest.Builder} avoiding the
* need to create one manually via {@link ListOriginEndpointsRequest#builder()}
*
*
* @param listOriginEndpointsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.mediapackage.model.ListOriginEndpointsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListOriginEndpoints operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.ListOriginEndpoints
* @see AWS API Documentation
*/
default CompletableFuture listOriginEndpoints(
Consumer listOriginEndpointsRequest) {
return listOriginEndpoints(ListOriginEndpointsRequest.builder().applyMutation(listOriginEndpointsRequest).build());
}
/**
* Returns a collection of OriginEndpoint records.
*
* @return A Java Future containing the result of the ListOriginEndpoints operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.ListOriginEndpoints
* @see AWS API Documentation
*/
default CompletableFuture listOriginEndpoints() {
return listOriginEndpoints(ListOriginEndpointsRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #listOriginEndpoints(software.amazon.awssdk.services.mediapackage.model.ListOriginEndpointsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.mediapackage.paginators.ListOriginEndpointsPublisher publisher = client.listOriginEndpointsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.mediapackage.paginators.ListOriginEndpointsPublisher publisher = client.listOriginEndpointsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.mediapackage.model.ListOriginEndpointsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listOriginEndpoints(software.amazon.awssdk.services.mediapackage.model.ListOriginEndpointsRequest)}
* operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.ListOriginEndpoints
* @see AWS API Documentation
*/
default ListOriginEndpointsPublisher listOriginEndpointsPaginator() {
return listOriginEndpointsPaginator(ListOriginEndpointsRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #listOriginEndpoints(software.amazon.awssdk.services.mediapackage.model.ListOriginEndpointsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.mediapackage.paginators.ListOriginEndpointsPublisher publisher = client.listOriginEndpointsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.mediapackage.paginators.ListOriginEndpointsPublisher publisher = client.listOriginEndpointsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.mediapackage.model.ListOriginEndpointsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listOriginEndpoints(software.amazon.awssdk.services.mediapackage.model.ListOriginEndpointsRequest)}
* operation.
*
*
* @param listOriginEndpointsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.ListOriginEndpoints
* @see AWS API Documentation
*/
default ListOriginEndpointsPublisher listOriginEndpointsPaginator(ListOriginEndpointsRequest listOriginEndpointsRequest) {
return new ListOriginEndpointsPublisher(this, listOriginEndpointsRequest);
}
/**
*
* This is a variant of
* {@link #listOriginEndpoints(software.amazon.awssdk.services.mediapackage.model.ListOriginEndpointsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.mediapackage.paginators.ListOriginEndpointsPublisher publisher = client.listOriginEndpointsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.mediapackage.paginators.ListOriginEndpointsPublisher publisher = client.listOriginEndpointsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.mediapackage.model.ListOriginEndpointsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listOriginEndpoints(software.amazon.awssdk.services.mediapackage.model.ListOriginEndpointsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListOriginEndpointsRequest.Builder} avoiding the
* need to create one manually via {@link ListOriginEndpointsRequest#builder()}
*
*
* @param listOriginEndpointsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.mediapackage.model.ListOriginEndpointsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.ListOriginEndpoints
* @see AWS API Documentation
*/
default ListOriginEndpointsPublisher listOriginEndpointsPaginator(
Consumer listOriginEndpointsRequest) {
return listOriginEndpointsPaginator(ListOriginEndpointsRequest.builder().applyMutation(listOriginEndpointsRequest)
.build());
}
/**
* Invokes the ListTagsForResource operation asynchronously.
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
default CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
throw new UnsupportedOperationException();
}
/**
* Invokes the ListTagsForResource operation asynchronously.
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.mediapackage.model.ListTagsForResourceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
default CompletableFuture listTagsForResource(
Consumer listTagsForResourceRequest) {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
* Rotate the IngestEndpoint's username and password, as specified by the IngestEndpoint's id.
*
* @param rotateIngestEndpointCredentialsRequest
* @return A Java Future containing the result of the RotateIngestEndpointCredentials operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.RotateIngestEndpointCredentials
* @see AWS API Documentation
*/
default CompletableFuture rotateIngestEndpointCredentials(
RotateIngestEndpointCredentialsRequest rotateIngestEndpointCredentialsRequest) {
throw new UnsupportedOperationException();
}
/**
* Rotate the IngestEndpoint's username and password, as specified by the IngestEndpoint's id.
*
* This is a convenience which creates an instance of the {@link RotateIngestEndpointCredentialsRequest.Builder}
* avoiding the need to create one manually via {@link RotateIngestEndpointCredentialsRequest#builder()}
*
*
* @param rotateIngestEndpointCredentialsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.mediapackage.model.RotateIngestEndpointCredentialsRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the RotateIngestEndpointCredentials operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.RotateIngestEndpointCredentials
* @see AWS API Documentation
*/
default CompletableFuture rotateIngestEndpointCredentials(
Consumer rotateIngestEndpointCredentialsRequest) {
return rotateIngestEndpointCredentials(RotateIngestEndpointCredentialsRequest.builder()
.applyMutation(rotateIngestEndpointCredentialsRequest).build());
}
/**
* Invokes the TagResource operation asynchronously.
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
* Invokes the TagResource operation asynchronously.
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.mediapackage.model.TagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(Consumer tagResourceRequest) {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
* Invokes the UntagResource operation asynchronously.
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.UntagResource
* @see AWS
* API Documentation
*/
default CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
* Invokes the UntagResource operation asynchronously.
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.mediapackage.model.UntagResourceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.UntagResource
* @see AWS
* API Documentation
*/
default CompletableFuture untagResource(Consumer untagResourceRequest) {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
* Updates an existing Channel.
*
* @param updateChannelRequest
* Configuration parameters used to update the Channel.
* @return A Java Future containing the result of the UpdateChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.UpdateChannel
* @see AWS
* API Documentation
*/
default CompletableFuture updateChannel(UpdateChannelRequest updateChannelRequest) {
throw new UnsupportedOperationException();
}
/**
* Updates an existing Channel.
*
* This is a convenience which creates an instance of the {@link UpdateChannelRequest.Builder} avoiding the need to
* create one manually via {@link UpdateChannelRequest#builder()}
*
*
* @param updateChannelRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.mediapackage.model.UpdateChannelRequest.Builder} to create a
* request. Configuration parameters used to update the Channel.
* @return A Java Future containing the result of the UpdateChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.UpdateChannel
* @see AWS
* API Documentation
*/
default CompletableFuture updateChannel(Consumer updateChannelRequest) {
return updateChannel(UpdateChannelRequest.builder().applyMutation(updateChannelRequest).build());
}
/**
* Updates an existing OriginEndpoint.
*
* @param updateOriginEndpointRequest
* Configuration parameters used to update an existing OriginEndpoint.
* @return A Java Future containing the result of the UpdateOriginEndpoint operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.UpdateOriginEndpoint
* @see AWS API Documentation
*/
default CompletableFuture updateOriginEndpoint(
UpdateOriginEndpointRequest updateOriginEndpointRequest) {
throw new UnsupportedOperationException();
}
/**
* Updates an existing OriginEndpoint.
*
* This is a convenience which creates an instance of the {@link UpdateOriginEndpointRequest.Builder} avoiding the
* need to create one manually via {@link UpdateOriginEndpointRequest#builder()}
*
*
* @param updateOriginEndpointRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.mediapackage.model.UpdateOriginEndpointRequest.Builder} to create a
* request. Configuration parameters used to update an existing OriginEndpoint.
* @return A Java Future containing the result of the UpdateOriginEndpoint operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnprocessableEntityException The parameters sent in the request are not valid.
* - InternalServerErrorException An unexpected error occurred.
* - ForbiddenException The client is not authorized to access the requested resource.
* - NotFoundException The requested resource does not exist.
* - ServiceUnavailableException An unexpected error occurred.
* - TooManyRequestsException The client has exceeded their resource or throttling limits.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaPackageException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaPackageAsyncClient.UpdateOriginEndpoint
* @see AWS API Documentation
*/
default CompletableFuture updateOriginEndpoint(
Consumer updateOriginEndpointRequest) {
return updateOriginEndpoint(UpdateOriginEndpointRequest.builder().applyMutation(updateOriginEndpointRequest).build());
}
@Override
default MediaPackageServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
/**
* Create a {@link MediaPackageAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static MediaPackageAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link MediaPackageAsyncClient}.
*/
static MediaPackageAsyncClientBuilder builder() {
return new DefaultMediaPackageAsyncClientBuilder();
}
}