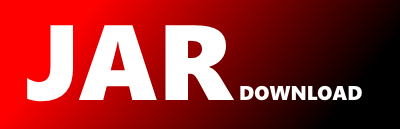
software.amazon.awssdk.services.mediapackage.model.DashPackage Maven / Gradle / Ivy
Show all versions of mediapackage Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.mediapackage.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
* A Dynamic Adaptive Streaming over HTTP (DASH) packaging configuration.
*/
@Generated("software.amazon.awssdk:codegen")
public final class DashPackage implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField> AD_TRIGGERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("AdTriggers")
.getter(getter(DashPackage::adTriggersAsStrings))
.setter(setter(Builder::adTriggersWithStrings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("adTriggers").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField ADS_ON_DELIVERY_RESTRICTIONS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AdsOnDeliveryRestrictions").getter(getter(DashPackage::adsOnDeliveryRestrictionsAsString))
.setter(setter(Builder::adsOnDeliveryRestrictions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("adsOnDeliveryRestrictions").build())
.build();
private static final SdkField ENCRYPTION_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("Encryption").getter(getter(DashPackage::encryption)).setter(setter(Builder::encryption))
.constructor(DashEncryption::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("encryption").build()).build();
private static final SdkField INCLUDE_IFRAME_ONLY_STREAM_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("IncludeIframeOnlyStream").getter(getter(DashPackage::includeIframeOnlyStream))
.setter(setter(Builder::includeIframeOnlyStream))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("includeIframeOnlyStream").build())
.build();
private static final SdkField MANIFEST_LAYOUT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ManifestLayout").getter(getter(DashPackage::manifestLayoutAsString))
.setter(setter(Builder::manifestLayout))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("manifestLayout").build()).build();
private static final SdkField MANIFEST_WINDOW_SECONDS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("ManifestWindowSeconds").getter(getter(DashPackage::manifestWindowSeconds))
.setter(setter(Builder::manifestWindowSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("manifestWindowSeconds").build())
.build();
private static final SdkField MIN_BUFFER_TIME_SECONDS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("MinBufferTimeSeconds").getter(getter(DashPackage::minBufferTimeSeconds))
.setter(setter(Builder::minBufferTimeSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("minBufferTimeSeconds").build())
.build();
private static final SdkField MIN_UPDATE_PERIOD_SECONDS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("MinUpdatePeriodSeconds").getter(getter(DashPackage::minUpdatePeriodSeconds))
.setter(setter(Builder::minUpdatePeriodSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("minUpdatePeriodSeconds").build())
.build();
private static final SdkField> PERIOD_TRIGGERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("PeriodTriggers")
.getter(getter(DashPackage::periodTriggersAsStrings))
.setter(setter(Builder::periodTriggersWithStrings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("periodTriggers").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField PROFILE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Profile")
.getter(getter(DashPackage::profileAsString)).setter(setter(Builder::profile))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("profile").build()).build();
private static final SdkField SEGMENT_DURATION_SECONDS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("SegmentDurationSeconds").getter(getter(DashPackage::segmentDurationSeconds))
.setter(setter(Builder::segmentDurationSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("segmentDurationSeconds").build())
.build();
private static final SdkField SEGMENT_TEMPLATE_FORMAT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SegmentTemplateFormat").getter(getter(DashPackage::segmentTemplateFormatAsString))
.setter(setter(Builder::segmentTemplateFormat))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("segmentTemplateFormat").build())
.build();
private static final SdkField STREAM_SELECTION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("StreamSelection")
.getter(getter(DashPackage::streamSelection)).setter(setter(Builder::streamSelection))
.constructor(StreamSelection::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("streamSelection").build()).build();
private static final SdkField SUGGESTED_PRESENTATION_DELAY_SECONDS_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("SuggestedPresentationDelaySeconds")
.getter(getter(DashPackage::suggestedPresentationDelaySeconds))
.setter(setter(Builder::suggestedPresentationDelaySeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("suggestedPresentationDelaySeconds")
.build()).build();
private static final SdkField UTC_TIMING_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("UtcTiming").getter(getter(DashPackage::utcTimingAsString)).setter(setter(Builder::utcTiming))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("utcTiming").build()).build();
private static final SdkField UTC_TIMING_URI_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("UtcTimingUri").getter(getter(DashPackage::utcTimingUri)).setter(setter(Builder::utcTimingUri))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("utcTimingUri").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(AD_TRIGGERS_FIELD,
ADS_ON_DELIVERY_RESTRICTIONS_FIELD, ENCRYPTION_FIELD, INCLUDE_IFRAME_ONLY_STREAM_FIELD, MANIFEST_LAYOUT_FIELD,
MANIFEST_WINDOW_SECONDS_FIELD, MIN_BUFFER_TIME_SECONDS_FIELD, MIN_UPDATE_PERIOD_SECONDS_FIELD, PERIOD_TRIGGERS_FIELD,
PROFILE_FIELD, SEGMENT_DURATION_SECONDS_FIELD, SEGMENT_TEMPLATE_FORMAT_FIELD, STREAM_SELECTION_FIELD,
SUGGESTED_PRESENTATION_DELAY_SECONDS_FIELD, UTC_TIMING_FIELD, UTC_TIMING_URI_FIELD));
private static final long serialVersionUID = 1L;
private final List adTriggers;
private final String adsOnDeliveryRestrictions;
private final DashEncryption encryption;
private final Boolean includeIframeOnlyStream;
private final String manifestLayout;
private final Integer manifestWindowSeconds;
private final Integer minBufferTimeSeconds;
private final Integer minUpdatePeriodSeconds;
private final List periodTriggers;
private final String profile;
private final Integer segmentDurationSeconds;
private final String segmentTemplateFormat;
private final StreamSelection streamSelection;
private final Integer suggestedPresentationDelaySeconds;
private final String utcTiming;
private final String utcTimingUri;
private DashPackage(BuilderImpl builder) {
this.adTriggers = builder.adTriggers;
this.adsOnDeliveryRestrictions = builder.adsOnDeliveryRestrictions;
this.encryption = builder.encryption;
this.includeIframeOnlyStream = builder.includeIframeOnlyStream;
this.manifestLayout = builder.manifestLayout;
this.manifestWindowSeconds = builder.manifestWindowSeconds;
this.minBufferTimeSeconds = builder.minBufferTimeSeconds;
this.minUpdatePeriodSeconds = builder.minUpdatePeriodSeconds;
this.periodTriggers = builder.periodTriggers;
this.profile = builder.profile;
this.segmentDurationSeconds = builder.segmentDurationSeconds;
this.segmentTemplateFormat = builder.segmentTemplateFormat;
this.streamSelection = builder.streamSelection;
this.suggestedPresentationDelaySeconds = builder.suggestedPresentationDelaySeconds;
this.utcTiming = builder.utcTiming;
this.utcTimingUri = builder.utcTimingUri;
}
/**
* Returns the value of the AdTriggers property for this object.
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAdTriggers} method.
*
*
* @return The value of the AdTriggers property for this object.
*/
public final List adTriggers() {
return AdTriggersCopier.copyStringToEnum(adTriggers);
}
/**
* For responses, this returns true if the service returned a value for the AdTriggers property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasAdTriggers() {
return adTriggers != null && !(adTriggers instanceof SdkAutoConstructList);
}
/**
* Returns the value of the AdTriggers property for this object.
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAdTriggers} method.
*
*
* @return The value of the AdTriggers property for this object.
*/
public final List adTriggersAsStrings() {
return adTriggers;
}
/**
* Returns the value of the AdsOnDeliveryRestrictions property for this object.
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #adsOnDeliveryRestrictions} will return {@link AdsOnDeliveryRestrictions#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #adsOnDeliveryRestrictionsAsString}.
*
*
* @return The value of the AdsOnDeliveryRestrictions property for this object.
* @see AdsOnDeliveryRestrictions
*/
public final AdsOnDeliveryRestrictions adsOnDeliveryRestrictions() {
return AdsOnDeliveryRestrictions.fromValue(adsOnDeliveryRestrictions);
}
/**
* Returns the value of the AdsOnDeliveryRestrictions property for this object.
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #adsOnDeliveryRestrictions} will return {@link AdsOnDeliveryRestrictions#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #adsOnDeliveryRestrictionsAsString}.
*
*
* @return The value of the AdsOnDeliveryRestrictions property for this object.
* @see AdsOnDeliveryRestrictions
*/
public final String adsOnDeliveryRestrictionsAsString() {
return adsOnDeliveryRestrictions;
}
/**
* Returns the value of the Encryption property for this object.
*
* @return The value of the Encryption property for this object.
*/
public final DashEncryption encryption() {
return encryption;
}
/**
* When enabled, an I-Frame only stream will be included in the output.
*
* @return When enabled, an I-Frame only stream will be included in the output.
*/
public final Boolean includeIframeOnlyStream() {
return includeIframeOnlyStream;
}
/**
* Determines the position of some tags in the Media Presentation Description (MPD). When set to FULL, elements like
* SegmentTemplate and ContentProtection are included in each Representation. When set to COMPACT, duplicate
* elements are combined and presented at the AdaptationSet level. When set to DRM_TOP_LEVEL_COMPACT, content
* protection elements are placed the MPD level and referenced at the AdaptationSet level.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #manifestLayout}
* will return {@link ManifestLayout#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #manifestLayoutAsString}.
*
*
* @return Determines the position of some tags in the Media Presentation Description (MPD). When set to FULL,
* elements like SegmentTemplate and ContentProtection are included in each Representation. When set to
* COMPACT, duplicate elements are combined and presented at the AdaptationSet level. When set to
* DRM_TOP_LEVEL_COMPACT, content protection elements are placed the MPD level and referenced at the
* AdaptationSet level.
* @see ManifestLayout
*/
public final ManifestLayout manifestLayout() {
return ManifestLayout.fromValue(manifestLayout);
}
/**
* Determines the position of some tags in the Media Presentation Description (MPD). When set to FULL, elements like
* SegmentTemplate and ContentProtection are included in each Representation. When set to COMPACT, duplicate
* elements are combined and presented at the AdaptationSet level. When set to DRM_TOP_LEVEL_COMPACT, content
* protection elements are placed the MPD level and referenced at the AdaptationSet level.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #manifestLayout}
* will return {@link ManifestLayout#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #manifestLayoutAsString}.
*
*
* @return Determines the position of some tags in the Media Presentation Description (MPD). When set to FULL,
* elements like SegmentTemplate and ContentProtection are included in each Representation. When set to
* COMPACT, duplicate elements are combined and presented at the AdaptationSet level. When set to
* DRM_TOP_LEVEL_COMPACT, content protection elements are placed the MPD level and referenced at the
* AdaptationSet level.
* @see ManifestLayout
*/
public final String manifestLayoutAsString() {
return manifestLayout;
}
/**
* Time window (in seconds) contained in each manifest.
*
* @return Time window (in seconds) contained in each manifest.
*/
public final Integer manifestWindowSeconds() {
return manifestWindowSeconds;
}
/**
* Minimum duration (in seconds) that a player will buffer media before starting the presentation.
*
* @return Minimum duration (in seconds) that a player will buffer media before starting the presentation.
*/
public final Integer minBufferTimeSeconds() {
return minBufferTimeSeconds;
}
/**
* Minimum duration (in seconds) between potential changes to the Dynamic Adaptive Streaming over HTTP (DASH) Media
* Presentation Description (MPD).
*
* @return Minimum duration (in seconds) between potential changes to the Dynamic Adaptive Streaming over HTTP
* (DASH) Media Presentation Description (MPD).
*/
public final Integer minUpdatePeriodSeconds() {
return minUpdatePeriodSeconds;
}
/**
* A list of triggers that controls when the outgoing Dynamic Adaptive Streaming over HTTP (DASH) Media Presentation
* Description (MPD) will be partitioned into multiple periods. If empty, the content will not be partitioned into
* more than one period. If the list contains "ADS", new periods will be created where the Channel source contains
* SCTE-35 ad markers.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasPeriodTriggers} method.
*
*
* @return A list of triggers that controls when the outgoing Dynamic Adaptive Streaming over HTTP (DASH) Media
* Presentation Description (MPD) will be partitioned into multiple periods. If empty, the content will not
* be partitioned into more than one period. If the list contains "ADS", new periods will be created where
* the Channel source contains SCTE-35 ad markers.
*/
public final List periodTriggers() {
return ___listOf__PeriodTriggersElementCopier.copyStringToEnum(periodTriggers);
}
/**
* For responses, this returns true if the service returned a value for the PeriodTriggers property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasPeriodTriggers() {
return periodTriggers != null && !(periodTriggers instanceof SdkAutoConstructList);
}
/**
* A list of triggers that controls when the outgoing Dynamic Adaptive Streaming over HTTP (DASH) Media Presentation
* Description (MPD) will be partitioned into multiple periods. If empty, the content will not be partitioned into
* more than one period. If the list contains "ADS", new periods will be created where the Channel source contains
* SCTE-35 ad markers.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasPeriodTriggers} method.
*
*
* @return A list of triggers that controls when the outgoing Dynamic Adaptive Streaming over HTTP (DASH) Media
* Presentation Description (MPD) will be partitioned into multiple periods. If empty, the content will not
* be partitioned into more than one period. If the list contains "ADS", new periods will be created where
* the Channel source contains SCTE-35 ad markers.
*/
public final List periodTriggersAsStrings() {
return periodTriggers;
}
/**
* The Dynamic Adaptive Streaming over HTTP (DASH) profile type. When set to "HBBTV_1_5", HbbTV 1.5 compliant output
* is enabled. When set to "DVB-DASH_2014", DVB-DASH 2014 compliant output is enabled.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #profile} will
* return {@link Profile#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #profileAsString}.
*
*
* @return The Dynamic Adaptive Streaming over HTTP (DASH) profile type. When set to "HBBTV_1_5", HbbTV 1.5
* compliant output is enabled. When set to "DVB-DASH_2014", DVB-DASH 2014 compliant output is enabled.
* @see Profile
*/
public final Profile profile() {
return Profile.fromValue(profile);
}
/**
* The Dynamic Adaptive Streaming over HTTP (DASH) profile type. When set to "HBBTV_1_5", HbbTV 1.5 compliant output
* is enabled. When set to "DVB-DASH_2014", DVB-DASH 2014 compliant output is enabled.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #profile} will
* return {@link Profile#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #profileAsString}.
*
*
* @return The Dynamic Adaptive Streaming over HTTP (DASH) profile type. When set to "HBBTV_1_5", HbbTV 1.5
* compliant output is enabled. When set to "DVB-DASH_2014", DVB-DASH 2014 compliant output is enabled.
* @see Profile
*/
public final String profileAsString() {
return profile;
}
/**
* Duration (in seconds) of each segment. Actual segments will be rounded to the nearest multiple of the source
* segment duration.
*
* @return Duration (in seconds) of each segment. Actual segments will be rounded to the nearest multiple of the
* source segment duration.
*/
public final Integer segmentDurationSeconds() {
return segmentDurationSeconds;
}
/**
* Determines the type of SegmentTemplate included in the Media Presentation Description (MPD). When set to
* NUMBER_WITH_TIMELINE, a full timeline is presented in each SegmentTemplate, with $Number$ media URLs. When set to
* TIME_WITH_TIMELINE, a full timeline is presented in each SegmentTemplate, with $Time$ media URLs. When set to
* NUMBER_WITH_DURATION, only a duration is included in each SegmentTemplate, with $Number$ media URLs.
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #segmentTemplateFormat} will return {@link SegmentTemplateFormat#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #segmentTemplateFormatAsString}.
*
*
* @return Determines the type of SegmentTemplate included in the Media Presentation Description (MPD). When set to
* NUMBER_WITH_TIMELINE, a full timeline is presented in each SegmentTemplate, with $Number$ media URLs.
* When set to TIME_WITH_TIMELINE, a full timeline is presented in each SegmentTemplate, with $Time$ media
* URLs. When set to NUMBER_WITH_DURATION, only a duration is included in each SegmentTemplate, with
* $Number$ media URLs.
* @see SegmentTemplateFormat
*/
public final SegmentTemplateFormat segmentTemplateFormat() {
return SegmentTemplateFormat.fromValue(segmentTemplateFormat);
}
/**
* Determines the type of SegmentTemplate included in the Media Presentation Description (MPD). When set to
* NUMBER_WITH_TIMELINE, a full timeline is presented in each SegmentTemplate, with $Number$ media URLs. When set to
* TIME_WITH_TIMELINE, a full timeline is presented in each SegmentTemplate, with $Time$ media URLs. When set to
* NUMBER_WITH_DURATION, only a duration is included in each SegmentTemplate, with $Number$ media URLs.
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #segmentTemplateFormat} will return {@link SegmentTemplateFormat#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #segmentTemplateFormatAsString}.
*
*
* @return Determines the type of SegmentTemplate included in the Media Presentation Description (MPD). When set to
* NUMBER_WITH_TIMELINE, a full timeline is presented in each SegmentTemplate, with $Number$ media URLs.
* When set to TIME_WITH_TIMELINE, a full timeline is presented in each SegmentTemplate, with $Time$ media
* URLs. When set to NUMBER_WITH_DURATION, only a duration is included in each SegmentTemplate, with
* $Number$ media URLs.
* @see SegmentTemplateFormat
*/
public final String segmentTemplateFormatAsString() {
return segmentTemplateFormat;
}
/**
* Returns the value of the StreamSelection property for this object.
*
* @return The value of the StreamSelection property for this object.
*/
public final StreamSelection streamSelection() {
return streamSelection;
}
/**
* Duration (in seconds) to delay live content before presentation.
*
* @return Duration (in seconds) to delay live content before presentation.
*/
public final Integer suggestedPresentationDelaySeconds() {
return suggestedPresentationDelaySeconds;
}
/**
* Determines the type of UTCTiming included in the Media Presentation Description (MPD)
*
* If the service returns an enum value that is not available in the current SDK version, {@link #utcTiming} will
* return {@link UtcTiming#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #utcTimingAsString}.
*
*
* @return Determines the type of UTCTiming included in the Media Presentation Description (MPD)
* @see UtcTiming
*/
public final UtcTiming utcTiming() {
return UtcTiming.fromValue(utcTiming);
}
/**
* Determines the type of UTCTiming included in the Media Presentation Description (MPD)
*
* If the service returns an enum value that is not available in the current SDK version, {@link #utcTiming} will
* return {@link UtcTiming#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #utcTimingAsString}.
*
*
* @return Determines the type of UTCTiming included in the Media Presentation Description (MPD)
* @see UtcTiming
*/
public final String utcTimingAsString() {
return utcTiming;
}
/**
* Specifies the value attribute of the UTCTiming field when utcTiming is set to HTTP-ISO, HTTP-HEAD or HTTP-XSDATE
*
* @return Specifies the value attribute of the UTCTiming field when utcTiming is set to HTTP-ISO, HTTP-HEAD or
* HTTP-XSDATE
*/
public final String utcTimingUri() {
return utcTimingUri;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(hasAdTriggers() ? adTriggersAsStrings() : null);
hashCode = 31 * hashCode + Objects.hashCode(adsOnDeliveryRestrictionsAsString());
hashCode = 31 * hashCode + Objects.hashCode(encryption());
hashCode = 31 * hashCode + Objects.hashCode(includeIframeOnlyStream());
hashCode = 31 * hashCode + Objects.hashCode(manifestLayoutAsString());
hashCode = 31 * hashCode + Objects.hashCode(manifestWindowSeconds());
hashCode = 31 * hashCode + Objects.hashCode(minBufferTimeSeconds());
hashCode = 31 * hashCode + Objects.hashCode(minUpdatePeriodSeconds());
hashCode = 31 * hashCode + Objects.hashCode(hasPeriodTriggers() ? periodTriggersAsStrings() : null);
hashCode = 31 * hashCode + Objects.hashCode(profileAsString());
hashCode = 31 * hashCode + Objects.hashCode(segmentDurationSeconds());
hashCode = 31 * hashCode + Objects.hashCode(segmentTemplateFormatAsString());
hashCode = 31 * hashCode + Objects.hashCode(streamSelection());
hashCode = 31 * hashCode + Objects.hashCode(suggestedPresentationDelaySeconds());
hashCode = 31 * hashCode + Objects.hashCode(utcTimingAsString());
hashCode = 31 * hashCode + Objects.hashCode(utcTimingUri());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DashPackage)) {
return false;
}
DashPackage other = (DashPackage) obj;
return hasAdTriggers() == other.hasAdTriggers() && Objects.equals(adTriggersAsStrings(), other.adTriggersAsStrings())
&& Objects.equals(adsOnDeliveryRestrictionsAsString(), other.adsOnDeliveryRestrictionsAsString())
&& Objects.equals(encryption(), other.encryption())
&& Objects.equals(includeIframeOnlyStream(), other.includeIframeOnlyStream())
&& Objects.equals(manifestLayoutAsString(), other.manifestLayoutAsString())
&& Objects.equals(manifestWindowSeconds(), other.manifestWindowSeconds())
&& Objects.equals(minBufferTimeSeconds(), other.minBufferTimeSeconds())
&& Objects.equals(minUpdatePeriodSeconds(), other.minUpdatePeriodSeconds())
&& hasPeriodTriggers() == other.hasPeriodTriggers()
&& Objects.equals(periodTriggersAsStrings(), other.periodTriggersAsStrings())
&& Objects.equals(profileAsString(), other.profileAsString())
&& Objects.equals(segmentDurationSeconds(), other.segmentDurationSeconds())
&& Objects.equals(segmentTemplateFormatAsString(), other.segmentTemplateFormatAsString())
&& Objects.equals(streamSelection(), other.streamSelection())
&& Objects.equals(suggestedPresentationDelaySeconds(), other.suggestedPresentationDelaySeconds())
&& Objects.equals(utcTimingAsString(), other.utcTimingAsString())
&& Objects.equals(utcTimingUri(), other.utcTimingUri());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DashPackage").add("AdTriggers", hasAdTriggers() ? adTriggersAsStrings() : null)
.add("AdsOnDeliveryRestrictions", adsOnDeliveryRestrictionsAsString()).add("Encryption", encryption())
.add("IncludeIframeOnlyStream", includeIframeOnlyStream()).add("ManifestLayout", manifestLayoutAsString())
.add("ManifestWindowSeconds", manifestWindowSeconds()).add("MinBufferTimeSeconds", minBufferTimeSeconds())
.add("MinUpdatePeriodSeconds", minUpdatePeriodSeconds())
.add("PeriodTriggers", hasPeriodTriggers() ? periodTriggersAsStrings() : null).add("Profile", profileAsString())
.add("SegmentDurationSeconds", segmentDurationSeconds())
.add("SegmentTemplateFormat", segmentTemplateFormatAsString()).add("StreamSelection", streamSelection())
.add("SuggestedPresentationDelaySeconds", suggestedPresentationDelaySeconds())
.add("UtcTiming", utcTimingAsString()).add("UtcTimingUri", utcTimingUri()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AdTriggers":
return Optional.ofNullable(clazz.cast(adTriggersAsStrings()));
case "AdsOnDeliveryRestrictions":
return Optional.ofNullable(clazz.cast(adsOnDeliveryRestrictionsAsString()));
case "Encryption":
return Optional.ofNullable(clazz.cast(encryption()));
case "IncludeIframeOnlyStream":
return Optional.ofNullable(clazz.cast(includeIframeOnlyStream()));
case "ManifestLayout":
return Optional.ofNullable(clazz.cast(manifestLayoutAsString()));
case "ManifestWindowSeconds":
return Optional.ofNullable(clazz.cast(manifestWindowSeconds()));
case "MinBufferTimeSeconds":
return Optional.ofNullable(clazz.cast(minBufferTimeSeconds()));
case "MinUpdatePeriodSeconds":
return Optional.ofNullable(clazz.cast(minUpdatePeriodSeconds()));
case "PeriodTriggers":
return Optional.ofNullable(clazz.cast(periodTriggersAsStrings()));
case "Profile":
return Optional.ofNullable(clazz.cast(profileAsString()));
case "SegmentDurationSeconds":
return Optional.ofNullable(clazz.cast(segmentDurationSeconds()));
case "SegmentTemplateFormat":
return Optional.ofNullable(clazz.cast(segmentTemplateFormatAsString()));
case "StreamSelection":
return Optional.ofNullable(clazz.cast(streamSelection()));
case "SuggestedPresentationDelaySeconds":
return Optional.ofNullable(clazz.cast(suggestedPresentationDelaySeconds()));
case "UtcTiming":
return Optional.ofNullable(clazz.cast(utcTimingAsString()));
case "UtcTimingUri":
return Optional.ofNullable(clazz.cast(utcTimingUri()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function