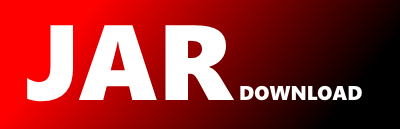
software.amazon.awssdk.services.mediastore.model.MetricPolicyRule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mediastore Show documentation
Show all versions of mediastore Show documentation
The AWS Java SDK for AWS Elemental MediaStore module holds the client classes that are used for
communicating
with AWS Elemental MediaStore Service
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.mediastore.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* A setting that enables metrics at the object level. Each rule contains an object group and an object group name. If
* the policy includes the MetricPolicyRules parameter, you must include at least one rule. Each metric policy can
* include up to five rules by default. You can also request a
* quota increase to allow up to 300 rules per policy.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class MetricPolicyRule implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField OBJECT_GROUP_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(MetricPolicyRule::objectGroup)).setter(setter(Builder::objectGroup))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ObjectGroup").build()).build();
private static final SdkField OBJECT_GROUP_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(MetricPolicyRule::objectGroupName)).setter(setter(Builder::objectGroupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ObjectGroupName").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(OBJECT_GROUP_FIELD,
OBJECT_GROUP_NAME_FIELD));
private static final long serialVersionUID = 1L;
private final String objectGroup;
private final String objectGroupName;
private MetricPolicyRule(BuilderImpl builder) {
this.objectGroup = builder.objectGroup;
this.objectGroupName = builder.objectGroupName;
}
/**
*
* A path or file name that defines which objects to include in the group. Wildcards (*) are acceptable.
*
*
* @return A path or file name that defines which objects to include in the group. Wildcards (*) are acceptable.
*/
public String objectGroup() {
return objectGroup;
}
/**
*
* A name that allows you to refer to the object group.
*
*
* @return A name that allows you to refer to the object group.
*/
public String objectGroupName() {
return objectGroupName;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(objectGroup());
hashCode = 31 * hashCode + Objects.hashCode(objectGroupName());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof MetricPolicyRule)) {
return false;
}
MetricPolicyRule other = (MetricPolicyRule) obj;
return Objects.equals(objectGroup(), other.objectGroup()) && Objects.equals(objectGroupName(), other.objectGroupName());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("MetricPolicyRule").add("ObjectGroup", objectGroup()).add("ObjectGroupName", objectGroupName())
.build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ObjectGroup":
return Optional.ofNullable(clazz.cast(objectGroup()));
case "ObjectGroupName":
return Optional.ofNullable(clazz.cast(objectGroupName()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy