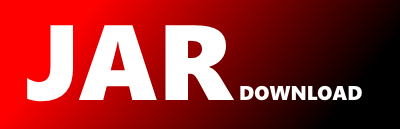
software.amazon.awssdk.services.mediastore.MediaStoreClient Maven / Gradle / Ivy
Show all versions of mediastore Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.mediastore;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.mediastore.model.ContainerInUseException;
import software.amazon.awssdk.services.mediastore.model.ContainerNotFoundException;
import software.amazon.awssdk.services.mediastore.model.CorsPolicyNotFoundException;
import software.amazon.awssdk.services.mediastore.model.CreateContainerRequest;
import software.amazon.awssdk.services.mediastore.model.CreateContainerResponse;
import software.amazon.awssdk.services.mediastore.model.DeleteContainerPolicyRequest;
import software.amazon.awssdk.services.mediastore.model.DeleteContainerPolicyResponse;
import software.amazon.awssdk.services.mediastore.model.DeleteContainerRequest;
import software.amazon.awssdk.services.mediastore.model.DeleteContainerResponse;
import software.amazon.awssdk.services.mediastore.model.DeleteCorsPolicyRequest;
import software.amazon.awssdk.services.mediastore.model.DeleteCorsPolicyResponse;
import software.amazon.awssdk.services.mediastore.model.DeleteLifecyclePolicyRequest;
import software.amazon.awssdk.services.mediastore.model.DeleteLifecyclePolicyResponse;
import software.amazon.awssdk.services.mediastore.model.DeleteMetricPolicyRequest;
import software.amazon.awssdk.services.mediastore.model.DeleteMetricPolicyResponse;
import software.amazon.awssdk.services.mediastore.model.DescribeContainerRequest;
import software.amazon.awssdk.services.mediastore.model.DescribeContainerResponse;
import software.amazon.awssdk.services.mediastore.model.GetContainerPolicyRequest;
import software.amazon.awssdk.services.mediastore.model.GetContainerPolicyResponse;
import software.amazon.awssdk.services.mediastore.model.GetCorsPolicyRequest;
import software.amazon.awssdk.services.mediastore.model.GetCorsPolicyResponse;
import software.amazon.awssdk.services.mediastore.model.GetLifecyclePolicyRequest;
import software.amazon.awssdk.services.mediastore.model.GetLifecyclePolicyResponse;
import software.amazon.awssdk.services.mediastore.model.GetMetricPolicyRequest;
import software.amazon.awssdk.services.mediastore.model.GetMetricPolicyResponse;
import software.amazon.awssdk.services.mediastore.model.InternalServerErrorException;
import software.amazon.awssdk.services.mediastore.model.LimitExceededException;
import software.amazon.awssdk.services.mediastore.model.ListContainersRequest;
import software.amazon.awssdk.services.mediastore.model.ListContainersResponse;
import software.amazon.awssdk.services.mediastore.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.mediastore.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.mediastore.model.MediaStoreException;
import software.amazon.awssdk.services.mediastore.model.PolicyNotFoundException;
import software.amazon.awssdk.services.mediastore.model.PutContainerPolicyRequest;
import software.amazon.awssdk.services.mediastore.model.PutContainerPolicyResponse;
import software.amazon.awssdk.services.mediastore.model.PutCorsPolicyRequest;
import software.amazon.awssdk.services.mediastore.model.PutCorsPolicyResponse;
import software.amazon.awssdk.services.mediastore.model.PutLifecyclePolicyRequest;
import software.amazon.awssdk.services.mediastore.model.PutLifecyclePolicyResponse;
import software.amazon.awssdk.services.mediastore.model.PutMetricPolicyRequest;
import software.amazon.awssdk.services.mediastore.model.PutMetricPolicyResponse;
import software.amazon.awssdk.services.mediastore.model.StartAccessLoggingRequest;
import software.amazon.awssdk.services.mediastore.model.StartAccessLoggingResponse;
import software.amazon.awssdk.services.mediastore.model.StopAccessLoggingRequest;
import software.amazon.awssdk.services.mediastore.model.StopAccessLoggingResponse;
import software.amazon.awssdk.services.mediastore.model.TagResourceRequest;
import software.amazon.awssdk.services.mediastore.model.TagResourceResponse;
import software.amazon.awssdk.services.mediastore.model.UntagResourceRequest;
import software.amazon.awssdk.services.mediastore.model.UntagResourceResponse;
import software.amazon.awssdk.services.mediastore.paginators.ListContainersIterable;
/**
* Service client for accessing MediaStore. This can be created using the static {@link #builder()} method.
*
*
* An AWS Elemental MediaStore container is a namespace that holds folders and objects. You use a container endpoint to
* create, read, and delete objects.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface MediaStoreClient extends SdkClient {
String SERVICE_NAME = "mediastore";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "mediastore";
/**
* Create a {@link MediaStoreClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static MediaStoreClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link MediaStoreClient}.
*/
static MediaStoreClientBuilder builder() {
return new DefaultMediaStoreClientBuilder();
}
/**
*
* Creates a storage container to hold objects. A container is similar to a bucket in the Amazon S3 service.
*
*
* @param createContainerRequest
* @return Result of the CreateContainer operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws LimitExceededException
* A service limit has been exceeded.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.CreateContainer
* @see AWS
* API Documentation
*/
default CreateContainerResponse createContainer(CreateContainerRequest createContainerRequest)
throws ContainerInUseException, LimitExceededException, InternalServerErrorException, AwsServiceException,
SdkClientException, MediaStoreException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a storage container to hold objects. A container is similar to a bucket in the Amazon S3 service.
*
*
*
* This is a convenience which creates an instance of the {@link CreateContainerRequest.Builder} avoiding the need
* to create one manually via {@link CreateContainerRequest#builder()}
*
*
* @param createContainerRequest
* A {@link Consumer} that will call methods on {@link CreateContainerInput.Builder} to create a request.
* @return Result of the CreateContainer operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws LimitExceededException
* A service limit has been exceeded.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.CreateContainer
* @see AWS
* API Documentation
*/
default CreateContainerResponse createContainer(Consumer createContainerRequest)
throws ContainerInUseException, LimitExceededException, InternalServerErrorException, AwsServiceException,
SdkClientException, MediaStoreException {
return createContainer(CreateContainerRequest.builder().applyMutation(createContainerRequest).build());
}
/**
*
* Deletes the specified container. Before you make a DeleteContainer
request, delete any objects in
* the container or in any folders in the container. You can delete only empty containers.
*
*
* @param deleteContainerRequest
* @return Result of the DeleteContainer operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.DeleteContainer
* @see AWS
* API Documentation
*/
default DeleteContainerResponse deleteContainer(DeleteContainerRequest deleteContainerRequest)
throws ContainerInUseException, ContainerNotFoundException, InternalServerErrorException, AwsServiceException,
SdkClientException, MediaStoreException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified container. Before you make a DeleteContainer
request, delete any objects in
* the container or in any folders in the container. You can delete only empty containers.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteContainerRequest.Builder} avoiding the need
* to create one manually via {@link DeleteContainerRequest#builder()}
*
*
* @param deleteContainerRequest
* A {@link Consumer} that will call methods on {@link DeleteContainerInput.Builder} to create a request.
* @return Result of the DeleteContainer operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.DeleteContainer
* @see AWS
* API Documentation
*/
default DeleteContainerResponse deleteContainer(Consumer deleteContainerRequest)
throws ContainerInUseException, ContainerNotFoundException, InternalServerErrorException, AwsServiceException,
SdkClientException, MediaStoreException {
return deleteContainer(DeleteContainerRequest.builder().applyMutation(deleteContainerRequest).build());
}
/**
*
* Deletes the access policy that is associated with the specified container.
*
*
* @param deleteContainerPolicyRequest
* @return Result of the DeleteContainerPolicy operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws PolicyNotFoundException
* The policy that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.DeleteContainerPolicy
* @see AWS API Documentation
*/
default DeleteContainerPolicyResponse deleteContainerPolicy(DeleteContainerPolicyRequest deleteContainerPolicyRequest)
throws ContainerInUseException, ContainerNotFoundException, PolicyNotFoundException, InternalServerErrorException,
AwsServiceException, SdkClientException, MediaStoreException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the access policy that is associated with the specified container.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteContainerPolicyRequest.Builder} avoiding the
* need to create one manually via {@link DeleteContainerPolicyRequest#builder()}
*
*
* @param deleteContainerPolicyRequest
* A {@link Consumer} that will call methods on {@link DeleteContainerPolicyInput.Builder} to create a
* request.
* @return Result of the DeleteContainerPolicy operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws PolicyNotFoundException
* The policy that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.DeleteContainerPolicy
* @see AWS API Documentation
*/
default DeleteContainerPolicyResponse deleteContainerPolicy(
Consumer deleteContainerPolicyRequest) throws ContainerInUseException,
ContainerNotFoundException, PolicyNotFoundException, InternalServerErrorException, AwsServiceException,
SdkClientException, MediaStoreException {
return deleteContainerPolicy(DeleteContainerPolicyRequest.builder().applyMutation(deleteContainerPolicyRequest).build());
}
/**
*
* Deletes the cross-origin resource sharing (CORS) configuration information that is set for the container.
*
*
* To use this operation, you must have permission to perform the MediaStore:DeleteCorsPolicy
action.
* The container owner has this permission by default and can grant this permission to others.
*
*
* @param deleteCorsPolicyRequest
* @return Result of the DeleteCorsPolicy operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws CorsPolicyNotFoundException
* The CORS policy that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.DeleteCorsPolicy
* @see AWS
* API Documentation
*/
default DeleteCorsPolicyResponse deleteCorsPolicy(DeleteCorsPolicyRequest deleteCorsPolicyRequest)
throws ContainerInUseException, ContainerNotFoundException, CorsPolicyNotFoundException,
InternalServerErrorException, AwsServiceException, SdkClientException, MediaStoreException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the cross-origin resource sharing (CORS) configuration information that is set for the container.
*
*
* To use this operation, you must have permission to perform the MediaStore:DeleteCorsPolicy
action.
* The container owner has this permission by default and can grant this permission to others.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteCorsPolicyRequest.Builder} avoiding the need
* to create one manually via {@link DeleteCorsPolicyRequest#builder()}
*
*
* @param deleteCorsPolicyRequest
* A {@link Consumer} that will call methods on {@link DeleteCorsPolicyInput.Builder} to create a request.
* @return Result of the DeleteCorsPolicy operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws CorsPolicyNotFoundException
* The CORS policy that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.DeleteCorsPolicy
* @see AWS
* API Documentation
*/
default DeleteCorsPolicyResponse deleteCorsPolicy(Consumer deleteCorsPolicyRequest)
throws ContainerInUseException, ContainerNotFoundException, CorsPolicyNotFoundException,
InternalServerErrorException, AwsServiceException, SdkClientException, MediaStoreException {
return deleteCorsPolicy(DeleteCorsPolicyRequest.builder().applyMutation(deleteCorsPolicyRequest).build());
}
/**
*
* Removes an object lifecycle policy from a container. It takes up to 20 minutes for the change to take effect.
*
*
* @param deleteLifecyclePolicyRequest
* @return Result of the DeleteLifecyclePolicy operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws PolicyNotFoundException
* The policy that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.DeleteLifecyclePolicy
* @see AWS API Documentation
*/
default DeleteLifecyclePolicyResponse deleteLifecyclePolicy(DeleteLifecyclePolicyRequest deleteLifecyclePolicyRequest)
throws ContainerInUseException, ContainerNotFoundException, PolicyNotFoundException, InternalServerErrorException,
AwsServiceException, SdkClientException, MediaStoreException {
throw new UnsupportedOperationException();
}
/**
*
* Removes an object lifecycle policy from a container. It takes up to 20 minutes for the change to take effect.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteLifecyclePolicyRequest.Builder} avoiding the
* need to create one manually via {@link DeleteLifecyclePolicyRequest#builder()}
*
*
* @param deleteLifecyclePolicyRequest
* A {@link Consumer} that will call methods on {@link DeleteLifecyclePolicyInput.Builder} to create a
* request.
* @return Result of the DeleteLifecyclePolicy operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws PolicyNotFoundException
* The policy that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.DeleteLifecyclePolicy
* @see AWS API Documentation
*/
default DeleteLifecyclePolicyResponse deleteLifecyclePolicy(
Consumer deleteLifecyclePolicyRequest) throws ContainerInUseException,
ContainerNotFoundException, PolicyNotFoundException, InternalServerErrorException, AwsServiceException,
SdkClientException, MediaStoreException {
return deleteLifecyclePolicy(DeleteLifecyclePolicyRequest.builder().applyMutation(deleteLifecyclePolicyRequest).build());
}
/**
*
* Deletes the metric policy that is associated with the specified container. If there is no metric policy
* associated with the container, MediaStore doesn't send metrics to CloudWatch.
*
*
* @param deleteMetricPolicyRequest
* @return Result of the DeleteMetricPolicy operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws PolicyNotFoundException
* The policy that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.DeleteMetricPolicy
* @see AWS
* API Documentation
*/
default DeleteMetricPolicyResponse deleteMetricPolicy(DeleteMetricPolicyRequest deleteMetricPolicyRequest)
throws ContainerInUseException, ContainerNotFoundException, PolicyNotFoundException, InternalServerErrorException,
AwsServiceException, SdkClientException, MediaStoreException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the metric policy that is associated with the specified container. If there is no metric policy
* associated with the container, MediaStore doesn't send metrics to CloudWatch.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteMetricPolicyRequest.Builder} avoiding the
* need to create one manually via {@link DeleteMetricPolicyRequest#builder()}
*
*
* @param deleteMetricPolicyRequest
* A {@link Consumer} that will call methods on {@link DeleteMetricPolicyInput.Builder} to create a request.
* @return Result of the DeleteMetricPolicy operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws PolicyNotFoundException
* The policy that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.DeleteMetricPolicy
* @see AWS
* API Documentation
*/
default DeleteMetricPolicyResponse deleteMetricPolicy(Consumer deleteMetricPolicyRequest)
throws ContainerInUseException, ContainerNotFoundException, PolicyNotFoundException, InternalServerErrorException,
AwsServiceException, SdkClientException, MediaStoreException {
return deleteMetricPolicy(DeleteMetricPolicyRequest.builder().applyMutation(deleteMetricPolicyRequest).build());
}
/**
*
* Retrieves the properties of the requested container. This request is commonly used to retrieve the endpoint of a
* container. An endpoint is a value assigned by the service when a new container is created. A container's endpoint
* does not change after it has been assigned. The DescribeContainer
request returns a single
* Container
object based on ContainerName
. To return all Container
objects
* that are associated with a specified AWS account, use ListContainers.
*
*
* @param describeContainerRequest
* @return Result of the DescribeContainer operation returned by the service.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.DescribeContainer
* @see AWS
* API Documentation
*/
default DescribeContainerResponse describeContainer(DescribeContainerRequest describeContainerRequest)
throws ContainerNotFoundException, InternalServerErrorException, AwsServiceException, SdkClientException,
MediaStoreException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the properties of the requested container. This request is commonly used to retrieve the endpoint of a
* container. An endpoint is a value assigned by the service when a new container is created. A container's endpoint
* does not change after it has been assigned. The DescribeContainer
request returns a single
* Container
object based on ContainerName
. To return all Container
objects
* that are associated with a specified AWS account, use ListContainers.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeContainerRequest.Builder} avoiding the need
* to create one manually via {@link DescribeContainerRequest#builder()}
*
*
* @param describeContainerRequest
* A {@link Consumer} that will call methods on {@link DescribeContainerInput.Builder} to create a request.
* @return Result of the DescribeContainer operation returned by the service.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.DescribeContainer
* @see AWS
* API Documentation
*/
default DescribeContainerResponse describeContainer(Consumer describeContainerRequest)
throws ContainerNotFoundException, InternalServerErrorException, AwsServiceException, SdkClientException,
MediaStoreException {
return describeContainer(DescribeContainerRequest.builder().applyMutation(describeContainerRequest).build());
}
/**
*
* Retrieves the access policy for the specified container. For information about the data that is included in an
* access policy, see the AWS Identity and Access Management
* User Guide.
*
*
* @param getContainerPolicyRequest
* @return Result of the GetContainerPolicy operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws PolicyNotFoundException
* The policy that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.GetContainerPolicy
* @see AWS
* API Documentation
*/
default GetContainerPolicyResponse getContainerPolicy(GetContainerPolicyRequest getContainerPolicyRequest)
throws ContainerInUseException, ContainerNotFoundException, PolicyNotFoundException, InternalServerErrorException,
AwsServiceException, SdkClientException, MediaStoreException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the access policy for the specified container. For information about the data that is included in an
* access policy, see the AWS Identity and Access Management
* User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link GetContainerPolicyRequest.Builder} avoiding the
* need to create one manually via {@link GetContainerPolicyRequest#builder()}
*
*
* @param getContainerPolicyRequest
* A {@link Consumer} that will call methods on {@link GetContainerPolicyInput.Builder} to create a request.
* @return Result of the GetContainerPolicy operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws PolicyNotFoundException
* The policy that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.GetContainerPolicy
* @see AWS
* API Documentation
*/
default GetContainerPolicyResponse getContainerPolicy(Consumer getContainerPolicyRequest)
throws ContainerInUseException, ContainerNotFoundException, PolicyNotFoundException, InternalServerErrorException,
AwsServiceException, SdkClientException, MediaStoreException {
return getContainerPolicy(GetContainerPolicyRequest.builder().applyMutation(getContainerPolicyRequest).build());
}
/**
*
* Returns the cross-origin resource sharing (CORS) configuration information that is set for the container.
*
*
* To use this operation, you must have permission to perform the MediaStore:GetCorsPolicy
action. By
* default, the container owner has this permission and can grant it to others.
*
*
* @param getCorsPolicyRequest
* @return Result of the GetCorsPolicy operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws CorsPolicyNotFoundException
* The CORS policy that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.GetCorsPolicy
* @see AWS API
* Documentation
*/
default GetCorsPolicyResponse getCorsPolicy(GetCorsPolicyRequest getCorsPolicyRequest) throws ContainerInUseException,
ContainerNotFoundException, CorsPolicyNotFoundException, InternalServerErrorException, AwsServiceException,
SdkClientException, MediaStoreException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the cross-origin resource sharing (CORS) configuration information that is set for the container.
*
*
* To use this operation, you must have permission to perform the MediaStore:GetCorsPolicy
action. By
* default, the container owner has this permission and can grant it to others.
*
*
*
* This is a convenience which creates an instance of the {@link GetCorsPolicyRequest.Builder} avoiding the need to
* create one manually via {@link GetCorsPolicyRequest#builder()}
*
*
* @param getCorsPolicyRequest
* A {@link Consumer} that will call methods on {@link GetCorsPolicyInput.Builder} to create a request.
* @return Result of the GetCorsPolicy operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws CorsPolicyNotFoundException
* The CORS policy that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.GetCorsPolicy
* @see AWS API
* Documentation
*/
default GetCorsPolicyResponse getCorsPolicy(Consumer getCorsPolicyRequest)
throws ContainerInUseException, ContainerNotFoundException, CorsPolicyNotFoundException,
InternalServerErrorException, AwsServiceException, SdkClientException, MediaStoreException {
return getCorsPolicy(GetCorsPolicyRequest.builder().applyMutation(getCorsPolicyRequest).build());
}
/**
*
* Retrieves the object lifecycle policy that is assigned to a container.
*
*
* @param getLifecyclePolicyRequest
* @return Result of the GetLifecyclePolicy operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws PolicyNotFoundException
* The policy that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.GetLifecyclePolicy
* @see AWS
* API Documentation
*/
default GetLifecyclePolicyResponse getLifecyclePolicy(GetLifecyclePolicyRequest getLifecyclePolicyRequest)
throws ContainerInUseException, ContainerNotFoundException, PolicyNotFoundException, InternalServerErrorException,
AwsServiceException, SdkClientException, MediaStoreException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the object lifecycle policy that is assigned to a container.
*
*
*
* This is a convenience which creates an instance of the {@link GetLifecyclePolicyRequest.Builder} avoiding the
* need to create one manually via {@link GetLifecyclePolicyRequest#builder()}
*
*
* @param getLifecyclePolicyRequest
* A {@link Consumer} that will call methods on {@link GetLifecyclePolicyInput.Builder} to create a request.
* @return Result of the GetLifecyclePolicy operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws PolicyNotFoundException
* The policy that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.GetLifecyclePolicy
* @see AWS
* API Documentation
*/
default GetLifecyclePolicyResponse getLifecyclePolicy(Consumer getLifecyclePolicyRequest)
throws ContainerInUseException, ContainerNotFoundException, PolicyNotFoundException, InternalServerErrorException,
AwsServiceException, SdkClientException, MediaStoreException {
return getLifecyclePolicy(GetLifecyclePolicyRequest.builder().applyMutation(getLifecyclePolicyRequest).build());
}
/**
*
* Returns the metric policy for the specified container.
*
*
* @param getMetricPolicyRequest
* @return Result of the GetMetricPolicy operation returned by the service.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws PolicyNotFoundException
* The policy that you specified in the request does not exist.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.GetMetricPolicy
* @see AWS
* API Documentation
*/
default GetMetricPolicyResponse getMetricPolicy(GetMetricPolicyRequest getMetricPolicyRequest)
throws ContainerNotFoundException, PolicyNotFoundException, ContainerInUseException, InternalServerErrorException,
AwsServiceException, SdkClientException, MediaStoreException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the metric policy for the specified container.
*
*
*
* This is a convenience which creates an instance of the {@link GetMetricPolicyRequest.Builder} avoiding the need
* to create one manually via {@link GetMetricPolicyRequest#builder()}
*
*
* @param getMetricPolicyRequest
* A {@link Consumer} that will call methods on {@link GetMetricPolicyInput.Builder} to create a request.
* @return Result of the GetMetricPolicy operation returned by the service.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws PolicyNotFoundException
* The policy that you specified in the request does not exist.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.GetMetricPolicy
* @see AWS
* API Documentation
*/
default GetMetricPolicyResponse getMetricPolicy(Consumer getMetricPolicyRequest)
throws ContainerNotFoundException, PolicyNotFoundException, ContainerInUseException, InternalServerErrorException,
AwsServiceException, SdkClientException, MediaStoreException {
return getMetricPolicy(GetMetricPolicyRequest.builder().applyMutation(getMetricPolicyRequest).build());
}
/**
*
* Lists the properties of all containers in AWS Elemental MediaStore.
*
*
* You can query to receive all the containers in one response. Or you can include the MaxResults
* parameter to receive a limited number of containers in each response. In this case, the response includes a
* token. To get the next set of containers, send the command again, this time with the NextToken
* parameter (with the returned token as its value). The next set of responses appears, with a token if there are
* still more containers to receive.
*
*
* See also DescribeContainer, which gets the properties of one container.
*
*
* @return Result of the ListContainers operation returned by the service.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.ListContainers
* @see #listContainers(ListContainersRequest)
* @see AWS API
* Documentation
*/
default ListContainersResponse listContainers() throws InternalServerErrorException, AwsServiceException, SdkClientException,
MediaStoreException {
return listContainers(ListContainersRequest.builder().build());
}
/**
*
* Lists the properties of all containers in AWS Elemental MediaStore.
*
*
* You can query to receive all the containers in one response. Or you can include the MaxResults
* parameter to receive a limited number of containers in each response. In this case, the response includes a
* token. To get the next set of containers, send the command again, this time with the NextToken
* parameter (with the returned token as its value). The next set of responses appears, with a token if there are
* still more containers to receive.
*
*
* See also DescribeContainer, which gets the properties of one container.
*
*
* @param listContainersRequest
* @return Result of the ListContainers operation returned by the service.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.ListContainers
* @see AWS API
* Documentation
*/
default ListContainersResponse listContainers(ListContainersRequest listContainersRequest)
throws InternalServerErrorException, AwsServiceException, SdkClientException, MediaStoreException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the properties of all containers in AWS Elemental MediaStore.
*
*
* You can query to receive all the containers in one response. Or you can include the MaxResults
* parameter to receive a limited number of containers in each response. In this case, the response includes a
* token. To get the next set of containers, send the command again, this time with the NextToken
* parameter (with the returned token as its value). The next set of responses appears, with a token if there are
* still more containers to receive.
*
*
* See also DescribeContainer, which gets the properties of one container.
*
*
*
* This is a convenience which creates an instance of the {@link ListContainersRequest.Builder} avoiding the need to
* create one manually via {@link ListContainersRequest#builder()}
*
*
* @param listContainersRequest
* A {@link Consumer} that will call methods on {@link ListContainersInput.Builder} to create a request.
* @return Result of the ListContainers operation returned by the service.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.ListContainers
* @see AWS API
* Documentation
*/
default ListContainersResponse listContainers(Consumer listContainersRequest)
throws InternalServerErrorException, AwsServiceException, SdkClientException, MediaStoreException {
return listContainers(ListContainersRequest.builder().applyMutation(listContainersRequest).build());
}
/**
*
* Lists the properties of all containers in AWS Elemental MediaStore.
*
*
* You can query to receive all the containers in one response. Or you can include the MaxResults
* parameter to receive a limited number of containers in each response. In this case, the response includes a
* token. To get the next set of containers, send the command again, this time with the NextToken
* parameter (with the returned token as its value). The next set of responses appears, with a token if there are
* still more containers to receive.
*
*
* See also DescribeContainer, which gets the properties of one container.
*
*
*
* This is a variant of
* {@link #listContainers(software.amazon.awssdk.services.mediastore.model.ListContainersRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.mediastore.paginators.ListContainersIterable responses = client.listContainersPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.mediastore.paginators.ListContainersIterable responses = client
* .listContainersPaginator(request);
* for (software.amazon.awssdk.services.mediastore.model.ListContainersResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.mediastore.paginators.ListContainersIterable responses = client.listContainersPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listContainers(software.amazon.awssdk.services.mediastore.model.ListContainersRequest)} operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.ListContainers
* @see #listContainersPaginator(ListContainersRequest)
* @see AWS API
* Documentation
*/
default ListContainersIterable listContainersPaginator() throws InternalServerErrorException, AwsServiceException,
SdkClientException, MediaStoreException {
return listContainersPaginator(ListContainersRequest.builder().build());
}
/**
*
* Lists the properties of all containers in AWS Elemental MediaStore.
*
*
* You can query to receive all the containers in one response. Or you can include the MaxResults
* parameter to receive a limited number of containers in each response. In this case, the response includes a
* token. To get the next set of containers, send the command again, this time with the NextToken
* parameter (with the returned token as its value). The next set of responses appears, with a token if there are
* still more containers to receive.
*
*
* See also DescribeContainer, which gets the properties of one container.
*
*
*
* This is a variant of
* {@link #listContainers(software.amazon.awssdk.services.mediastore.model.ListContainersRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.mediastore.paginators.ListContainersIterable responses = client.listContainersPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.mediastore.paginators.ListContainersIterable responses = client
* .listContainersPaginator(request);
* for (software.amazon.awssdk.services.mediastore.model.ListContainersResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.mediastore.paginators.ListContainersIterable responses = client.listContainersPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listContainers(software.amazon.awssdk.services.mediastore.model.ListContainersRequest)} operation.
*
*
* @param listContainersRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.ListContainers
* @see AWS API
* Documentation
*/
default ListContainersIterable listContainersPaginator(ListContainersRequest listContainersRequest)
throws InternalServerErrorException, AwsServiceException, SdkClientException, MediaStoreException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the properties of all containers in AWS Elemental MediaStore.
*
*
* You can query to receive all the containers in one response. Or you can include the MaxResults
* parameter to receive a limited number of containers in each response. In this case, the response includes a
* token. To get the next set of containers, send the command again, this time with the NextToken
* parameter (with the returned token as its value). The next set of responses appears, with a token if there are
* still more containers to receive.
*
*
* See also DescribeContainer, which gets the properties of one container.
*
*
*
* This is a variant of
* {@link #listContainers(software.amazon.awssdk.services.mediastore.model.ListContainersRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.mediastore.paginators.ListContainersIterable responses = client.listContainersPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.mediastore.paginators.ListContainersIterable responses = client
* .listContainersPaginator(request);
* for (software.amazon.awssdk.services.mediastore.model.ListContainersResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.mediastore.paginators.ListContainersIterable responses = client.listContainersPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listContainers(software.amazon.awssdk.services.mediastore.model.ListContainersRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListContainersRequest.Builder} avoiding the need to
* create one manually via {@link ListContainersRequest#builder()}
*
*
* @param listContainersRequest
* A {@link Consumer} that will call methods on {@link ListContainersInput.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.ListContainers
* @see AWS API
* Documentation
*/
default ListContainersIterable listContainersPaginator(Consumer listContainersRequest)
throws InternalServerErrorException, AwsServiceException, SdkClientException, MediaStoreException {
return listContainersPaginator(ListContainersRequest.builder().applyMutation(listContainersRequest).build());
}
/**
*
* Returns a list of the tags assigned to the specified container.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.ListTagsForResource
* @see AWS API Documentation
*/
default ListTagsForResourceResponse listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest)
throws ContainerInUseException, ContainerNotFoundException, InternalServerErrorException, AwsServiceException,
SdkClientException, MediaStoreException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of the tags assigned to the specified container.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on {@link ListTagsForResourceInput.Builder} to create a request.
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.ListTagsForResource
* @see AWS API Documentation
*/
default ListTagsForResourceResponse listTagsForResource(
Consumer listTagsForResourceRequest) throws ContainerInUseException,
ContainerNotFoundException, InternalServerErrorException, AwsServiceException, SdkClientException,
MediaStoreException {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Creates an access policy for the specified container to restrict the users and clients that can access it. For
* information about the data that is included in an access policy, see the AWS Identity and Access Management User Guide.
*
*
* For this release of the REST API, you can create only one policy for a container. If you enter
* PutContainerPolicy
twice, the second command modifies the existing policy.
*
*
* @param putContainerPolicyRequest
* @return Result of the PutContainerPolicy operation returned by the service.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.PutContainerPolicy
* @see AWS
* API Documentation
*/
default PutContainerPolicyResponse putContainerPolicy(PutContainerPolicyRequest putContainerPolicyRequest)
throws ContainerNotFoundException, ContainerInUseException, InternalServerErrorException, AwsServiceException,
SdkClientException, MediaStoreException {
throw new UnsupportedOperationException();
}
/**
*
* Creates an access policy for the specified container to restrict the users and clients that can access it. For
* information about the data that is included in an access policy, see the AWS Identity and Access Management User Guide.
*
*
* For this release of the REST API, you can create only one policy for a container. If you enter
* PutContainerPolicy
twice, the second command modifies the existing policy.
*
*
*
* This is a convenience which creates an instance of the {@link PutContainerPolicyRequest.Builder} avoiding the
* need to create one manually via {@link PutContainerPolicyRequest#builder()}
*
*
* @param putContainerPolicyRequest
* A {@link Consumer} that will call methods on {@link PutContainerPolicyInput.Builder} to create a request.
* @return Result of the PutContainerPolicy operation returned by the service.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.PutContainerPolicy
* @see AWS
* API Documentation
*/
default PutContainerPolicyResponse putContainerPolicy(Consumer putContainerPolicyRequest)
throws ContainerNotFoundException, ContainerInUseException, InternalServerErrorException, AwsServiceException,
SdkClientException, MediaStoreException {
return putContainerPolicy(PutContainerPolicyRequest.builder().applyMutation(putContainerPolicyRequest).build());
}
/**
*
* Sets the cross-origin resource sharing (CORS) configuration on a container so that the container can service
* cross-origin requests. For example, you might want to enable a request whose origin is http://www.example.com to
* access your AWS Elemental MediaStore container at my.example.container.com by using the browser's XMLHttpRequest
* capability.
*
*
* To enable CORS on a container, you attach a CORS policy to the container. In the CORS policy, you configure rules
* that identify origins and the HTTP methods that can be executed on your container. The policy can contain up to
* 398,000 characters. You can add up to 100 rules to a CORS policy. If more than one rule applies, the service uses
* the first applicable rule listed.
*
*
* To learn more about CORS, see Cross-Origin Resource Sharing (CORS) in
* AWS Elemental MediaStore.
*
*
* @param putCorsPolicyRequest
* @return Result of the PutCorsPolicy operation returned by the service.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.PutCorsPolicy
* @see AWS API
* Documentation
*/
default PutCorsPolicyResponse putCorsPolicy(PutCorsPolicyRequest putCorsPolicyRequest) throws ContainerNotFoundException,
ContainerInUseException, InternalServerErrorException, AwsServiceException, SdkClientException, MediaStoreException {
throw new UnsupportedOperationException();
}
/**
*
* Sets the cross-origin resource sharing (CORS) configuration on a container so that the container can service
* cross-origin requests. For example, you might want to enable a request whose origin is http://www.example.com to
* access your AWS Elemental MediaStore container at my.example.container.com by using the browser's XMLHttpRequest
* capability.
*
*
* To enable CORS on a container, you attach a CORS policy to the container. In the CORS policy, you configure rules
* that identify origins and the HTTP methods that can be executed on your container. The policy can contain up to
* 398,000 characters. You can add up to 100 rules to a CORS policy. If more than one rule applies, the service uses
* the first applicable rule listed.
*
*
* To learn more about CORS, see Cross-Origin Resource Sharing (CORS) in
* AWS Elemental MediaStore.
*
*
*
* This is a convenience which creates an instance of the {@link PutCorsPolicyRequest.Builder} avoiding the need to
* create one manually via {@link PutCorsPolicyRequest#builder()}
*
*
* @param putCorsPolicyRequest
* A {@link Consumer} that will call methods on {@link PutCorsPolicyInput.Builder} to create a request.
* @return Result of the PutCorsPolicy operation returned by the service.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.PutCorsPolicy
* @see AWS API
* Documentation
*/
default PutCorsPolicyResponse putCorsPolicy(Consumer putCorsPolicyRequest)
throws ContainerNotFoundException, ContainerInUseException, InternalServerErrorException, AwsServiceException,
SdkClientException, MediaStoreException {
return putCorsPolicy(PutCorsPolicyRequest.builder().applyMutation(putCorsPolicyRequest).build());
}
/**
*
* Writes an object lifecycle policy to a container. If the container already has an object lifecycle policy, the
* service replaces the existing policy with the new policy. It takes up to 20 minutes for the change to take
* effect.
*
*
* For information about how to construct an object lifecycle policy, see Components of
* an Object Lifecycle Policy.
*
*
* @param putLifecyclePolicyRequest
* @return Result of the PutLifecyclePolicy operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.PutLifecyclePolicy
* @see AWS
* API Documentation
*/
default PutLifecyclePolicyResponse putLifecyclePolicy(PutLifecyclePolicyRequest putLifecyclePolicyRequest)
throws ContainerInUseException, ContainerNotFoundException, InternalServerErrorException, AwsServiceException,
SdkClientException, MediaStoreException {
throw new UnsupportedOperationException();
}
/**
*
* Writes an object lifecycle policy to a container. If the container already has an object lifecycle policy, the
* service replaces the existing policy with the new policy. It takes up to 20 minutes for the change to take
* effect.
*
*
* For information about how to construct an object lifecycle policy, see Components of
* an Object Lifecycle Policy.
*
*
*
* This is a convenience which creates an instance of the {@link PutLifecyclePolicyRequest.Builder} avoiding the
* need to create one manually via {@link PutLifecyclePolicyRequest#builder()}
*
*
* @param putLifecyclePolicyRequest
* A {@link Consumer} that will call methods on {@link PutLifecyclePolicyInput.Builder} to create a request.
* @return Result of the PutLifecyclePolicy operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.PutLifecyclePolicy
* @see AWS
* API Documentation
*/
default PutLifecyclePolicyResponse putLifecyclePolicy(Consumer putLifecyclePolicyRequest)
throws ContainerInUseException, ContainerNotFoundException, InternalServerErrorException, AwsServiceException,
SdkClientException, MediaStoreException {
return putLifecyclePolicy(PutLifecyclePolicyRequest.builder().applyMutation(putLifecyclePolicyRequest).build());
}
/**
*
* The metric policy that you want to add to the container. A metric policy allows AWS Elemental MediaStore to send
* metrics to Amazon CloudWatch. It takes up to 20 minutes for the new policy to take effect.
*
*
* @param putMetricPolicyRequest
* @return Result of the PutMetricPolicy operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.PutMetricPolicy
* @see AWS
* API Documentation
*/
default PutMetricPolicyResponse putMetricPolicy(PutMetricPolicyRequest putMetricPolicyRequest)
throws ContainerInUseException, ContainerNotFoundException, InternalServerErrorException, AwsServiceException,
SdkClientException, MediaStoreException {
throw new UnsupportedOperationException();
}
/**
*
* The metric policy that you want to add to the container. A metric policy allows AWS Elemental MediaStore to send
* metrics to Amazon CloudWatch. It takes up to 20 minutes for the new policy to take effect.
*
*
*
* This is a convenience which creates an instance of the {@link PutMetricPolicyRequest.Builder} avoiding the need
* to create one manually via {@link PutMetricPolicyRequest#builder()}
*
*
* @param putMetricPolicyRequest
* A {@link Consumer} that will call methods on {@link PutMetricPolicyInput.Builder} to create a request.
* @return Result of the PutMetricPolicy operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.PutMetricPolicy
* @see AWS
* API Documentation
*/
default PutMetricPolicyResponse putMetricPolicy(Consumer putMetricPolicyRequest)
throws ContainerInUseException, ContainerNotFoundException, InternalServerErrorException, AwsServiceException,
SdkClientException, MediaStoreException {
return putMetricPolicy(PutMetricPolicyRequest.builder().applyMutation(putMetricPolicyRequest).build());
}
/**
*
* Starts access logging on the specified container. When you enable access logging on a container, MediaStore
* delivers access logs for objects stored in that container to Amazon CloudWatch Logs.
*
*
* @param startAccessLoggingRequest
* @return Result of the StartAccessLogging operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.StartAccessLogging
* @see AWS
* API Documentation
*/
default StartAccessLoggingResponse startAccessLogging(StartAccessLoggingRequest startAccessLoggingRequest)
throws ContainerInUseException, ContainerNotFoundException, InternalServerErrorException, AwsServiceException,
SdkClientException, MediaStoreException {
throw new UnsupportedOperationException();
}
/**
*
* Starts access logging on the specified container. When you enable access logging on a container, MediaStore
* delivers access logs for objects stored in that container to Amazon CloudWatch Logs.
*
*
*
* This is a convenience which creates an instance of the {@link StartAccessLoggingRequest.Builder} avoiding the
* need to create one manually via {@link StartAccessLoggingRequest#builder()}
*
*
* @param startAccessLoggingRequest
* A {@link Consumer} that will call methods on {@link StartAccessLoggingInput.Builder} to create a request.
* @return Result of the StartAccessLogging operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.StartAccessLogging
* @see AWS
* API Documentation
*/
default StartAccessLoggingResponse startAccessLogging(Consumer startAccessLoggingRequest)
throws ContainerInUseException, ContainerNotFoundException, InternalServerErrorException, AwsServiceException,
SdkClientException, MediaStoreException {
return startAccessLogging(StartAccessLoggingRequest.builder().applyMutation(startAccessLoggingRequest).build());
}
/**
*
* Stops access logging on the specified container. When you stop access logging on a container, MediaStore stops
* sending access logs to Amazon CloudWatch Logs. These access logs are not saved and are not retrievable.
*
*
* @param stopAccessLoggingRequest
* @return Result of the StopAccessLogging operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.StopAccessLogging
* @see AWS
* API Documentation
*/
default StopAccessLoggingResponse stopAccessLogging(StopAccessLoggingRequest stopAccessLoggingRequest)
throws ContainerInUseException, ContainerNotFoundException, InternalServerErrorException, AwsServiceException,
SdkClientException, MediaStoreException {
throw new UnsupportedOperationException();
}
/**
*
* Stops access logging on the specified container. When you stop access logging on a container, MediaStore stops
* sending access logs to Amazon CloudWatch Logs. These access logs are not saved and are not retrievable.
*
*
*
* This is a convenience which creates an instance of the {@link StopAccessLoggingRequest.Builder} avoiding the need
* to create one manually via {@link StopAccessLoggingRequest#builder()}
*
*
* @param stopAccessLoggingRequest
* A {@link Consumer} that will call methods on {@link StopAccessLoggingInput.Builder} to create a request.
* @return Result of the StopAccessLogging operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.StopAccessLogging
* @see AWS
* API Documentation
*/
default StopAccessLoggingResponse stopAccessLogging(Consumer stopAccessLoggingRequest)
throws ContainerInUseException, ContainerNotFoundException, InternalServerErrorException, AwsServiceException,
SdkClientException, MediaStoreException {
return stopAccessLogging(StopAccessLoggingRequest.builder().applyMutation(stopAccessLoggingRequest).build());
}
/**
*
* Adds tags to the specified AWS Elemental MediaStore container. Tags are key:value pairs that you can associate
* with AWS resources. For example, the tag key might be "customer" and the tag value might be "companyA." You can
* specify one or more tags to add to each container. You can add up to 50 tags to each container. For more
* information about tagging, including naming and usage conventions, see Tagging Resources in MediaStore.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.TagResource
* @see AWS API
* Documentation
*/
default TagResourceResponse tagResource(TagResourceRequest tagResourceRequest) throws ContainerInUseException,
ContainerNotFoundException, InternalServerErrorException, AwsServiceException, SdkClientException,
MediaStoreException {
throw new UnsupportedOperationException();
}
/**
*
* Adds tags to the specified AWS Elemental MediaStore container. Tags are key:value pairs that you can associate
* with AWS resources. For example, the tag key might be "customer" and the tag value might be "companyA." You can
* specify one or more tags to add to each container. You can add up to 50 tags to each container. For more
* information about tagging, including naming and usage conventions, see Tagging Resources in MediaStore.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on {@link TagResourceInput.Builder} to create a request.
* @return Result of the TagResource operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.TagResource
* @see AWS API
* Documentation
*/
default TagResourceResponse tagResource(Consumer tagResourceRequest)
throws ContainerInUseException, ContainerNotFoundException, InternalServerErrorException, AwsServiceException,
SdkClientException, MediaStoreException {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Removes tags from the specified container. You can specify one or more tags to remove.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.UntagResource
* @see AWS API
* Documentation
*/
default UntagResourceResponse untagResource(UntagResourceRequest untagResourceRequest) throws ContainerInUseException,
ContainerNotFoundException, InternalServerErrorException, AwsServiceException, SdkClientException,
MediaStoreException {
throw new UnsupportedOperationException();
}
/**
*
* Removes tags from the specified container. You can specify one or more tags to remove.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on {@link UntagResourceInput.Builder} to create a request.
* @return Result of the UntagResource operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreClient.UntagResource
* @see AWS API
* Documentation
*/
default UntagResourceResponse untagResource(Consumer untagResourceRequest)
throws ContainerInUseException, ContainerNotFoundException, InternalServerErrorException, AwsServiceException,
SdkClientException, MediaStoreException {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of(SERVICE_METADATA_ID);
}
}