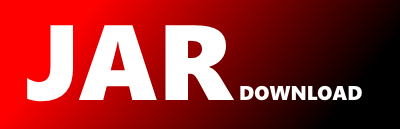
software.amazon.awssdk.services.mediastore.DefaultMediaStoreAsyncClient Maven / Gradle / Ivy
Show all versions of mediastore Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.mediastore;
import java.util.concurrent.CompletableFuture;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.services.mediastore.model.ContainerInUseException;
import software.amazon.awssdk.services.mediastore.model.ContainerNotFoundException;
import software.amazon.awssdk.services.mediastore.model.CorsPolicyNotFoundException;
import software.amazon.awssdk.services.mediastore.model.CreateContainerRequest;
import software.amazon.awssdk.services.mediastore.model.CreateContainerResponse;
import software.amazon.awssdk.services.mediastore.model.DeleteContainerPolicyRequest;
import software.amazon.awssdk.services.mediastore.model.DeleteContainerPolicyResponse;
import software.amazon.awssdk.services.mediastore.model.DeleteContainerRequest;
import software.amazon.awssdk.services.mediastore.model.DeleteContainerResponse;
import software.amazon.awssdk.services.mediastore.model.DeleteCorsPolicyRequest;
import software.amazon.awssdk.services.mediastore.model.DeleteCorsPolicyResponse;
import software.amazon.awssdk.services.mediastore.model.DeleteLifecyclePolicyRequest;
import software.amazon.awssdk.services.mediastore.model.DeleteLifecyclePolicyResponse;
import software.amazon.awssdk.services.mediastore.model.DescribeContainerRequest;
import software.amazon.awssdk.services.mediastore.model.DescribeContainerResponse;
import software.amazon.awssdk.services.mediastore.model.GetContainerPolicyRequest;
import software.amazon.awssdk.services.mediastore.model.GetContainerPolicyResponse;
import software.amazon.awssdk.services.mediastore.model.GetCorsPolicyRequest;
import software.amazon.awssdk.services.mediastore.model.GetCorsPolicyResponse;
import software.amazon.awssdk.services.mediastore.model.GetLifecyclePolicyRequest;
import software.amazon.awssdk.services.mediastore.model.GetLifecyclePolicyResponse;
import software.amazon.awssdk.services.mediastore.model.InternalServerErrorException;
import software.amazon.awssdk.services.mediastore.model.LimitExceededException;
import software.amazon.awssdk.services.mediastore.model.ListContainersRequest;
import software.amazon.awssdk.services.mediastore.model.ListContainersResponse;
import software.amazon.awssdk.services.mediastore.model.MediaStoreException;
import software.amazon.awssdk.services.mediastore.model.PolicyNotFoundException;
import software.amazon.awssdk.services.mediastore.model.PutContainerPolicyRequest;
import software.amazon.awssdk.services.mediastore.model.PutContainerPolicyResponse;
import software.amazon.awssdk.services.mediastore.model.PutCorsPolicyRequest;
import software.amazon.awssdk.services.mediastore.model.PutCorsPolicyResponse;
import software.amazon.awssdk.services.mediastore.model.PutLifecyclePolicyRequest;
import software.amazon.awssdk.services.mediastore.model.PutLifecyclePolicyResponse;
import software.amazon.awssdk.services.mediastore.model.StartAccessLoggingRequest;
import software.amazon.awssdk.services.mediastore.model.StartAccessLoggingResponse;
import software.amazon.awssdk.services.mediastore.model.StopAccessLoggingRequest;
import software.amazon.awssdk.services.mediastore.model.StopAccessLoggingResponse;
import software.amazon.awssdk.services.mediastore.transform.CreateContainerRequestMarshaller;
import software.amazon.awssdk.services.mediastore.transform.DeleteContainerPolicyRequestMarshaller;
import software.amazon.awssdk.services.mediastore.transform.DeleteContainerRequestMarshaller;
import software.amazon.awssdk.services.mediastore.transform.DeleteCorsPolicyRequestMarshaller;
import software.amazon.awssdk.services.mediastore.transform.DeleteLifecyclePolicyRequestMarshaller;
import software.amazon.awssdk.services.mediastore.transform.DescribeContainerRequestMarshaller;
import software.amazon.awssdk.services.mediastore.transform.GetContainerPolicyRequestMarshaller;
import software.amazon.awssdk.services.mediastore.transform.GetCorsPolicyRequestMarshaller;
import software.amazon.awssdk.services.mediastore.transform.GetLifecyclePolicyRequestMarshaller;
import software.amazon.awssdk.services.mediastore.transform.ListContainersRequestMarshaller;
import software.amazon.awssdk.services.mediastore.transform.PutContainerPolicyRequestMarshaller;
import software.amazon.awssdk.services.mediastore.transform.PutCorsPolicyRequestMarshaller;
import software.amazon.awssdk.services.mediastore.transform.PutLifecyclePolicyRequestMarshaller;
import software.amazon.awssdk.services.mediastore.transform.StartAccessLoggingRequestMarshaller;
import software.amazon.awssdk.services.mediastore.transform.StopAccessLoggingRequestMarshaller;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link MediaStoreAsyncClient}.
*
* @see MediaStoreAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultMediaStoreAsyncClient implements MediaStoreAsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultMediaStoreAsyncClient.class);
private final AsyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultMediaStoreAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
*
* Creates a storage container to hold objects. A container is similar to a bucket in the Amazon S3 service.
*
*
* @param createContainerRequest
* @return A Java Future containing the result of the CreateContainer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ContainerInUseException The container that you specified in the request already exists or is being
* updated.
* - LimitExceededException A service limit has been exceeded.
* - InternalServerErrorException The service is temporarily unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaStoreException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaStoreAsyncClient.CreateContainer
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createContainer(CreateContainerRequest createContainerRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateContainerResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateContainer")
.withMarshaller(new CreateContainerRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(createContainerRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified container. Before you make a DeleteContainer
request, delete any objects in
* the container or in any folders in the container. You can delete only empty containers.
*
*
* @param deleteContainerRequest
* @return A Java Future containing the result of the DeleteContainer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ContainerInUseException The container that you specified in the request already exists or is being
* updated.
* - ContainerNotFoundException The container that you specified in the request does not exist.
* - InternalServerErrorException The service is temporarily unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaStoreException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaStoreAsyncClient.DeleteContainer
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteContainer(DeleteContainerRequest deleteContainerRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteContainerResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteContainer")
.withMarshaller(new DeleteContainerRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(deleteContainerRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the access policy that is associated with the specified container.
*
*
* @param deleteContainerPolicyRequest
* @return A Java Future containing the result of the DeleteContainerPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ContainerInUseException The container that you specified in the request already exists or is being
* updated.
* - ContainerNotFoundException The container that you specified in the request does not exist.
* - PolicyNotFoundException The policy that you specified in the request does not exist.
* - InternalServerErrorException The service is temporarily unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaStoreException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaStoreAsyncClient.DeleteContainerPolicy
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteContainerPolicy(
DeleteContainerPolicyRequest deleteContainerPolicyRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteContainerPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteContainerPolicy")
.withMarshaller(new DeleteContainerPolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(deleteContainerPolicyRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the cross-origin resource sharing (CORS) configuration information that is set for the container.
*
*
* To use this operation, you must have permission to perform the MediaStore:DeleteCorsPolicy
action.
* The container owner has this permission by default and can grant this permission to others.
*
*
* @param deleteCorsPolicyRequest
* @return A Java Future containing the result of the DeleteCorsPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ContainerInUseException The container that you specified in the request already exists or is being
* updated.
* - ContainerNotFoundException The container that you specified in the request does not exist.
* - CorsPolicyNotFoundException The CORS policy that you specified in the request does not exist.
* - InternalServerErrorException The service is temporarily unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaStoreException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaStoreAsyncClient.DeleteCorsPolicy
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteCorsPolicy(DeleteCorsPolicyRequest deleteCorsPolicyRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteCorsPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteCorsPolicy")
.withMarshaller(new DeleteCorsPolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(deleteCorsPolicyRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Removes an object lifecycle policy from a container. It takes up to 20 minutes for the change to take effect.
*
*
* @param deleteLifecyclePolicyRequest
* @return A Java Future containing the result of the DeleteLifecyclePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ContainerInUseException The container that you specified in the request already exists or is being
* updated.
* - ContainerNotFoundException The container that you specified in the request does not exist.
* - PolicyNotFoundException The policy that you specified in the request does not exist.
* - InternalServerErrorException The service is temporarily unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaStoreException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaStoreAsyncClient.DeleteLifecyclePolicy
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteLifecyclePolicy(
DeleteLifecyclePolicyRequest deleteLifecyclePolicyRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteLifecyclePolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteLifecyclePolicy")
.withMarshaller(new DeleteLifecyclePolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(deleteLifecyclePolicyRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves the properties of the requested container. This request is commonly used to retrieve the endpoint of a
* container. An endpoint is a value assigned by the service when a new container is created. A container's endpoint
* does not change after it has been assigned. The DescribeContainer
request returns a single
* Container
object based on ContainerName
. To return all Container
objects
* that are associated with a specified AWS account, use ListContainers.
*
*
* @param describeContainerRequest
* @return A Java Future containing the result of the DescribeContainer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ContainerNotFoundException The container that you specified in the request does not exist.
* - InternalServerErrorException The service is temporarily unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaStoreException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaStoreAsyncClient.DescribeContainer
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describeContainer(DescribeContainerRequest describeContainerRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeContainerResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeContainer")
.withMarshaller(new DescribeContainerRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(describeContainerRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves the access policy for the specified container. For information about the data that is included in an
* access policy, see the AWS Identity and Access Management
* User Guide.
*
*
* @param getContainerPolicyRequest
* @return A Java Future containing the result of the GetContainerPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ContainerInUseException The container that you specified in the request already exists or is being
* updated.
* - ContainerNotFoundException The container that you specified in the request does not exist.
* - PolicyNotFoundException The policy that you specified in the request does not exist.
* - InternalServerErrorException The service is temporarily unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaStoreException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaStoreAsyncClient.GetContainerPolicy
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture getContainerPolicy(GetContainerPolicyRequest getContainerPolicyRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetContainerPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetContainerPolicy")
.withMarshaller(new GetContainerPolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getContainerPolicyRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the cross-origin resource sharing (CORS) configuration information that is set for the container.
*
*
* To use this operation, you must have permission to perform the MediaStore:GetCorsPolicy
action. By
* default, the container owner has this permission and can grant it to others.
*
*
* @param getCorsPolicyRequest
* @return A Java Future containing the result of the GetCorsPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ContainerInUseException The container that you specified in the request already exists or is being
* updated.
* - ContainerNotFoundException The container that you specified in the request does not exist.
* - CorsPolicyNotFoundException The CORS policy that you specified in the request does not exist.
* - InternalServerErrorException The service is temporarily unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaStoreException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaStoreAsyncClient.GetCorsPolicy
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getCorsPolicy(GetCorsPolicyRequest getCorsPolicyRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetCorsPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetCorsPolicy")
.withMarshaller(new GetCorsPolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getCorsPolicyRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves the object lifecycle policy that is assigned to a container.
*
*
* @param getLifecyclePolicyRequest
* @return A Java Future containing the result of the GetLifecyclePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ContainerInUseException The container that you specified in the request already exists or is being
* updated.
* - ContainerNotFoundException The container that you specified in the request does not exist.
* - PolicyNotFoundException The policy that you specified in the request does not exist.
* - InternalServerErrorException The service is temporarily unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaStoreException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaStoreAsyncClient.GetLifecyclePolicy
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture getLifecyclePolicy(GetLifecyclePolicyRequest getLifecyclePolicyRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetLifecyclePolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetLifecyclePolicy")
.withMarshaller(new GetLifecyclePolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getLifecyclePolicyRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the properties of all containers in AWS Elemental MediaStore.
*
*
* You can query to receive all the containers in one response. Or you can include the MaxResults
* parameter to receive a limited number of containers in each response. In this case, the response includes a
* token. To get the next set of containers, send the command again, this time with the NextToken
* parameter (with the returned token as its value). The next set of responses appears, with a token if there are
* still more containers to receive.
*
*
* See also DescribeContainer, which gets the properties of one container.
*
*
* @param listContainersRequest
* @return A Java Future containing the result of the ListContainers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerErrorException The service is temporarily unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaStoreException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaStoreAsyncClient.ListContainers
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listContainers(ListContainersRequest listContainersRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListContainersResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListContainers")
.withMarshaller(new ListContainersRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listContainersRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates an access policy for the specified container to restrict the users and clients that can access it. For
* information about the data that is included in an access policy, see the AWS Identity and Access Management User Guide.
*
*
* For this release of the REST API, you can create only one policy for a container. If you enter
* PutContainerPolicy
twice, the second command modifies the existing policy.
*
*
* @param putContainerPolicyRequest
* @return A Java Future containing the result of the PutContainerPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ContainerNotFoundException The container that you specified in the request does not exist.
* - ContainerInUseException The container that you specified in the request already exists or is being
* updated.
* - InternalServerErrorException The service is temporarily unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaStoreException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaStoreAsyncClient.PutContainerPolicy
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture putContainerPolicy(PutContainerPolicyRequest putContainerPolicyRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, PutContainerPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutContainerPolicy")
.withMarshaller(new PutContainerPolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(putContainerPolicyRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Sets the cross-origin resource sharing (CORS) configuration on a container so that the container can service
* cross-origin requests. For example, you might want to enable a request whose origin is http://www.example.com to
* access your AWS Elemental MediaStore container at my.example.container.com by using the browser's XMLHttpRequest
* capability.
*
*
* To enable CORS on a container, you attach a CORS policy to the container. In the CORS policy, you configure rules
* that identify origins and the HTTP methods that can be executed on your container. The policy can contain up to
* 398,000 characters. You can add up to 100 rules to a CORS policy. If more than one rule applies, the service uses
* the first applicable rule listed.
*
*
* To learn more about CORS, see Cross-Origin Resource Sharing (CORS) in
* AWS Elemental MediaStore.
*
*
* @param putCorsPolicyRequest
* @return A Java Future containing the result of the PutCorsPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ContainerNotFoundException The container that you specified in the request does not exist.
* - ContainerInUseException The container that you specified in the request already exists or is being
* updated.
* - InternalServerErrorException The service is temporarily unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaStoreException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaStoreAsyncClient.PutCorsPolicy
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture putCorsPolicy(PutCorsPolicyRequest putCorsPolicyRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
PutCorsPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutCorsPolicy")
.withMarshaller(new PutCorsPolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(putCorsPolicyRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Writes an object lifecycle policy to a container. If the container already has an object lifecycle policy, the
* service replaces the existing policy with the new policy. It takes up to 20 minutes for the change to take
* effect.
*
*
* For information about how to construct an object lifecycle policy, see Components of
* an Object Lifecycle Policy.
*
*
* @param putLifecyclePolicyRequest
* @return A Java Future containing the result of the PutLifecyclePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ContainerInUseException The container that you specified in the request already exists or is being
* updated.
* - ContainerNotFoundException The container that you specified in the request does not exist.
* - InternalServerErrorException The service is temporarily unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaStoreException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaStoreAsyncClient.PutLifecyclePolicy
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture putLifecyclePolicy(PutLifecyclePolicyRequest putLifecyclePolicyRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, PutLifecyclePolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutLifecyclePolicy")
.withMarshaller(new PutLifecyclePolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(putLifecyclePolicyRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Starts access logging on the specified container. When you enable access logging on a container, MediaStore
* delivers access logs for objects stored in that container to Amazon CloudWatch Logs.
*
*
* @param startAccessLoggingRequest
* @return A Java Future containing the result of the StartAccessLogging operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ContainerInUseException The container that you specified in the request already exists or is being
* updated.
* - ContainerNotFoundException The container that you specified in the request does not exist.
* - InternalServerErrorException The service is temporarily unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaStoreException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaStoreAsyncClient.StartAccessLogging
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture startAccessLogging(StartAccessLoggingRequest startAccessLoggingRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, StartAccessLoggingResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("StartAccessLogging")
.withMarshaller(new StartAccessLoggingRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(startAccessLoggingRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Stops access logging on the specified container. When you stop access logging on a container, MediaStore stops
* sending access logs to Amazon CloudWatch Logs. These access logs are not saved and are not retrievable.
*
*
* @param stopAccessLoggingRequest
* @return A Java Future containing the result of the StopAccessLogging operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ContainerInUseException The container that you specified in the request already exists or is being
* updated.
* - ContainerNotFoundException The container that you specified in the request does not exist.
* - InternalServerErrorException The service is temporarily unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaStoreException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MediaStoreAsyncClient.StopAccessLogging
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture stopAccessLogging(StopAccessLoggingRequest stopAccessLoggingRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, StopAccessLoggingResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("StopAccessLogging")
.withMarshaller(new StopAccessLoggingRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(stopAccessLoggingRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
@Override
public void close() {
clientHandler.close();
}
private > T init(T builder) {
return builder
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(MediaStoreException::builder)
.protocol(AwsJsonProtocol.AWS_JSON)
.protocolVersion("1.1")
.registerModeledException(
ExceptionMetadata.builder().errorCode("ContainerNotFoundException")
.exceptionBuilderSupplier(ContainerNotFoundException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ContainerInUseException")
.exceptionBuilderSupplier(ContainerInUseException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("PolicyNotFoundException")
.exceptionBuilderSupplier(PolicyNotFoundException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("CorsPolicyNotFoundException")
.exceptionBuilderSupplier(CorsPolicyNotFoundException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InternalServerError")
.exceptionBuilderSupplier(InternalServerErrorException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("LimitExceededException")
.exceptionBuilderSupplier(LimitExceededException::builder).build());
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata) {
return protocolFactory.createErrorResponseHandler(operationMetadata);
}
}