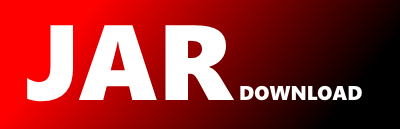
software.amazon.awssdk.services.mediastoredata.model.DescribeObjectResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mediastoredata Show documentation
Show all versions of mediastoredata Show documentation
The AWS Java SDK for AWS Elemental MediaStore Data module holds the client classes that are used for
communicating with AWS Elemental MediaStore Data Plane Service
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.mediastoredata.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class DescribeObjectResponse extends MediaStoreDataResponse implements
ToCopyableBuilder {
private static final SdkField E_TAG_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DescribeObjectResponse::eTag)).setter(setter(Builder::eTag))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("ETag").build()).build();
private static final SdkField CONTENT_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DescribeObjectResponse::contentType)).setter(setter(Builder::contentType))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("Content-Type").build()).build();
private static final SdkField CONTENT_LENGTH_FIELD = SdkField. builder(MarshallingType.LONG)
.getter(getter(DescribeObjectResponse::contentLength)).setter(setter(Builder::contentLength))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("Content-Length").build()).build();
private static final SdkField CACHE_CONTROL_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DescribeObjectResponse::cacheControl)).setter(setter(Builder::cacheControl))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("Cache-Control").build()).build();
private static final SdkField LAST_MODIFIED_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(DescribeObjectResponse::lastModified)).setter(setter(Builder::lastModified))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("Last-Modified").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(E_TAG_FIELD,
CONTENT_TYPE_FIELD, CONTENT_LENGTH_FIELD, CACHE_CONTROL_FIELD, LAST_MODIFIED_FIELD));
private final String eTag;
private final String contentType;
private final Long contentLength;
private final String cacheControl;
private final Instant lastModified;
private DescribeObjectResponse(BuilderImpl builder) {
super(builder);
this.eTag = builder.eTag;
this.contentType = builder.contentType;
this.contentLength = builder.contentLength;
this.cacheControl = builder.cacheControl;
this.lastModified = builder.lastModified;
}
/**
*
* The ETag that represents a unique instance of the object.
*
*
* @return The ETag that represents a unique instance of the object.
*/
public String eTag() {
return eTag;
}
/**
*
* The content type of the object.
*
*
* @return The content type of the object.
*/
public String contentType() {
return contentType;
}
/**
*
* The length of the object in bytes.
*
*
* @return The length of the object in bytes.
*/
public Long contentLength() {
return contentLength;
}
/**
*
* An optional CacheControl
header that allows the caller to control the object's cache behavior.
* Headers can be passed in as specified in the HTTP at https://www.w3.org/Protocols/rfc2616/rfc2616-sec14.html#sec14.9.
*
*
* Headers with a custom user-defined value are also accepted.
*
*
* @return An optional CacheControl
header that allows the caller to control the object's cache
* behavior. Headers can be passed in as specified in the HTTP at https://www.w3.org/Protocols/rfc2616/rfc2616-sec14.html#sec14.9.
*
* Headers with a custom user-defined value are also accepted.
*/
public String cacheControl() {
return cacheControl;
}
/**
*
* The date and time that the object was last modified.
*
*
* @return The date and time that the object was last modified.
*/
public Instant lastModified() {
return lastModified;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(eTag());
hashCode = 31 * hashCode + Objects.hashCode(contentType());
hashCode = 31 * hashCode + Objects.hashCode(contentLength());
hashCode = 31 * hashCode + Objects.hashCode(cacheControl());
hashCode = 31 * hashCode + Objects.hashCode(lastModified());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DescribeObjectResponse)) {
return false;
}
DescribeObjectResponse other = (DescribeObjectResponse) obj;
return Objects.equals(eTag(), other.eTag()) && Objects.equals(contentType(), other.contentType())
&& Objects.equals(contentLength(), other.contentLength()) && Objects.equals(cacheControl(), other.cacheControl())
&& Objects.equals(lastModified(), other.lastModified());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("DescribeObjectResponse").add("ETag", eTag()).add("ContentType", contentType())
.add("ContentLength", contentLength()).add("CacheControl", cacheControl()).add("LastModified", lastModified())
.build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ETag":
return Optional.ofNullable(clazz.cast(eTag()));
case "ContentType":
return Optional.ofNullable(clazz.cast(contentType()));
case "ContentLength":
return Optional.ofNullable(clazz.cast(contentLength()));
case "CacheControl":
return Optional.ofNullable(clazz.cast(cacheControl()));
case "LastModified":
return Optional.ofNullable(clazz.cast(lastModified()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy