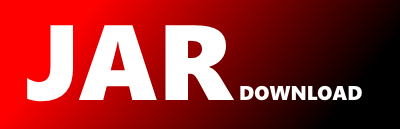
software.amazon.awssdk.services.mediastoredata.DefaultMediaStoreDataAsyncClient Maven / Gradle / Ivy
Show all versions of mediastoredata Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.mediastoredata;
import static software.amazon.awssdk.utils.FunctionalUtils.runAndLogError;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.auth.signer.Aws4UnsignedPayloadSigner;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.ApiName;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.async.AsyncRequestBody;
import software.amazon.awssdk.core.async.AsyncResponseTransformer;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.runtime.transform.AsyncStreamingRequestMarshaller;
import software.amazon.awssdk.core.signer.Signer;
import software.amazon.awssdk.core.util.VersionInfo;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.services.mediastoredata.model.ContainerNotFoundException;
import software.amazon.awssdk.services.mediastoredata.model.DeleteObjectRequest;
import software.amazon.awssdk.services.mediastoredata.model.DeleteObjectResponse;
import software.amazon.awssdk.services.mediastoredata.model.DescribeObjectRequest;
import software.amazon.awssdk.services.mediastoredata.model.DescribeObjectResponse;
import software.amazon.awssdk.services.mediastoredata.model.GetObjectRequest;
import software.amazon.awssdk.services.mediastoredata.model.GetObjectResponse;
import software.amazon.awssdk.services.mediastoredata.model.InternalServerErrorException;
import software.amazon.awssdk.services.mediastoredata.model.ListItemsRequest;
import software.amazon.awssdk.services.mediastoredata.model.ListItemsResponse;
import software.amazon.awssdk.services.mediastoredata.model.MediaStoreDataException;
import software.amazon.awssdk.services.mediastoredata.model.MediaStoreDataRequest;
import software.amazon.awssdk.services.mediastoredata.model.ObjectNotFoundException;
import software.amazon.awssdk.services.mediastoredata.model.PutObjectRequest;
import software.amazon.awssdk.services.mediastoredata.model.PutObjectResponse;
import software.amazon.awssdk.services.mediastoredata.model.RequestedRangeNotSatisfiableException;
import software.amazon.awssdk.services.mediastoredata.paginators.ListItemsPublisher;
import software.amazon.awssdk.services.mediastoredata.transform.DeleteObjectRequestMarshaller;
import software.amazon.awssdk.services.mediastoredata.transform.DescribeObjectRequestMarshaller;
import software.amazon.awssdk.services.mediastoredata.transform.GetObjectRequestMarshaller;
import software.amazon.awssdk.services.mediastoredata.transform.ListItemsRequestMarshaller;
import software.amazon.awssdk.services.mediastoredata.transform.PutObjectRequestMarshaller;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link MediaStoreDataAsyncClient}.
*
* @see MediaStoreDataAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultMediaStoreDataAsyncClient implements MediaStoreDataAsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultMediaStoreDataAsyncClient.class);
private final AsyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultMediaStoreDataAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
*
* Deletes an object at the specified path.
*
*
* @param deleteObjectRequest
* @return A Java Future containing the result of the DeleteObject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ContainerNotFoundException The specified container was not found for the specified account.
* - ObjectNotFoundException Could not perform an operation on an object that does not exist.
* - InternalServerErrorException The service is temporarily unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaStoreDataException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MediaStoreDataAsyncClient.DeleteObject
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteObject(DeleteObjectRequest deleteObjectRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteObjectRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MediaStore Data");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteObject");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteObjectResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteObject").withMarshaller(new DeleteObjectRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteObjectRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteObjectRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets the headers for an object at the specified path.
*
*
* @param describeObjectRequest
* @return A Java Future containing the result of the DescribeObject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ContainerNotFoundException The specified container was not found for the specified account.
* - ObjectNotFoundException Could not perform an operation on an object that does not exist.
* - InternalServerErrorException The service is temporarily unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaStoreDataException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MediaStoreDataAsyncClient.DescribeObject
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describeObject(DescribeObjectRequest describeObjectRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeObjectRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MediaStore Data");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeObject");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeObjectResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeObject")
.withMarshaller(new DescribeObjectRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeObjectRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = describeObjectRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Downloads the object at the specified path. If the object’s upload availability is set to streaming
,
* AWS Elemental MediaStore downloads the object even if it’s still uploading the object.
*
*
* @param getObjectRequest
* @param asyncResponseTransformer
* The response transformer for processing the streaming response in a non-blocking manner. See
* {@link AsyncResponseTransformer} for details on how this callback should be implemented and for links to
* precanned implementations for common scenarios like downloading to a file. The service documentation for
* the response content is as follows '
*
* The bytes of the object.
*
* '.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ContainerNotFoundException The specified container was not found for the specified account.
* - ObjectNotFoundException Could not perform an operation on an object that does not exist.
* - RequestedRangeNotSatisfiableException The requested content range is not valid.
* - InternalServerErrorException The service is temporarily unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaStoreDataException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MediaStoreDataAsyncClient.GetObject
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getObject(GetObjectRequest getObjectRequest,
AsyncResponseTransformer asyncResponseTransformer) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getObjectRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MediaStore Data");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetObject");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(true)
.isPayloadJson(false).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetObjectResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler.execute(
new ClientExecutionParams().withOperationName("GetObject")
.withMarshaller(new GetObjectRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withMetricCollector(apiCallMetricCollector)
.withInput(getObjectRequest), asyncResponseTransformer);
AwsRequestOverrideConfiguration requestOverrideConfig = getObjectRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
if (e != null) {
runAndLogError(log, "Exception thrown in exceptionOccurred callback, ignoring",
() -> asyncResponseTransformer.exceptionOccurred(e));
}
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
runAndLogError(log, "Exception thrown in exceptionOccurred callback, ignoring",
() -> asyncResponseTransformer.exceptionOccurred(t));
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides a list of metadata entries about folders and objects in the specified folder.
*
*
* @param listItemsRequest
* @return A Java Future containing the result of the ListItems operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ContainerNotFoundException The specified container was not found for the specified account.
* - InternalServerErrorException The service is temporarily unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaStoreDataException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MediaStoreDataAsyncClient.ListItems
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listItems(ListItemsRequest listItemsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listItemsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MediaStore Data");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListItems");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListItemsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("ListItems")
.withMarshaller(new ListItemsRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withMetricCollector(apiCallMetricCollector)
.withInput(listItemsRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = listItemsRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides a list of metadata entries about folders and objects in the specified folder.
*
*
*
* This is a variant of {@link #listItems(software.amazon.awssdk.services.mediastoredata.model.ListItemsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.mediastoredata.paginators.ListItemsPublisher publisher = client.listItemsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.mediastoredata.paginators.ListItemsPublisher publisher = client.listItemsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.mediastoredata.model.ListItemsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listItems(software.amazon.awssdk.services.mediastoredata.model.ListItemsRequest)} operation.
*
*
* @param listItemsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ContainerNotFoundException The specified container was not found for the specified account.
* - InternalServerErrorException The service is temporarily unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaStoreDataException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MediaStoreDataAsyncClient.ListItems
* @see AWS API
* Documentation
*/
public ListItemsPublisher listItemsPaginator(ListItemsRequest listItemsRequest) {
return new ListItemsPublisher(this, applyPaginatorUserAgent(listItemsRequest));
}
/**
*
* Uploads an object to the specified path. Object sizes are limited to 25 MB for standard upload availability and
* 10 MB for streaming upload availability.
*
*
* @param putObjectRequest
* @param requestBody
* Functional interface that can be implemented to produce the request content in a non-blocking manner. The
* size of the content is expected to be known up front. See {@link AsyncRequestBody} for specific details on
* implementing this interface as well as links to precanned implementations for common scenarios like
* uploading from a file. The service documentation for the request content is as follows '
*
* The bytes to be stored.
*
* '
* @return A Java Future containing the result of the PutObject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ContainerNotFoundException The specified container was not found for the specified account.
* - InternalServerErrorException The service is temporarily unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaStoreDataException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MediaStoreDataAsyncClient.PutObject
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture putObject(PutObjectRequest putObjectRequest, AsyncRequestBody requestBody) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, putObjectRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MediaStore Data");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutObject");
putObjectRequest = applySignerOverride(putObjectRequest, Aws4UnsignedPayloadSigner.create());
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
PutObjectResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutObject")
.withMarshaller(
AsyncStreamingRequestMarshaller.builder()
.delegateMarshaller(new PutObjectRequestMarshaller(protocolFactory))
.asyncRequestBody(requestBody).transferEncoding(true).build())
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withAsyncRequestBody(requestBody)
.withInput(putObjectRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = putObjectRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
@Override
public void close() {
clientHandler.close();
}
private > T init(T builder) {
return builder
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(MediaStoreDataException::builder)
.protocol(AwsJsonProtocol.REST_JSON)
.protocolVersion("1.1")
.registerModeledException(
ExceptionMetadata.builder().errorCode("RequestedRangeNotSatisfiableException")
.exceptionBuilderSupplier(RequestedRangeNotSatisfiableException::builder).httpStatusCode(416)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ObjectNotFoundException")
.exceptionBuilderSupplier(ObjectNotFoundException::builder).httpStatusCode(404).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InternalServerError")
.exceptionBuilderSupplier(InternalServerErrorException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ContainerNotFoundException")
.exceptionBuilderSupplier(ContainerNotFoundException::builder).httpStatusCode(404).build());
}
private static List resolveMetricPublishers(SdkClientConfiguration clientConfiguration,
RequestOverrideConfiguration requestOverrideConfiguration) {
List publishers = null;
if (requestOverrideConfiguration != null) {
publishers = requestOverrideConfiguration.metricPublishers();
}
if (publishers == null || publishers.isEmpty()) {
publishers = clientConfiguration.option(SdkClientOption.METRIC_PUBLISHERS);
}
if (publishers == null) {
publishers = Collections.emptyList();
}
return publishers;
}
private T applyPaginatorUserAgent(T request) {
Consumer userAgentApplier = b -> b.addApiName(ApiName.builder()
.version(VersionInfo.SDK_VERSION).name("PAGINATED").build());
AwsRequestOverrideConfiguration overrideConfiguration = request.overrideConfiguration()
.map(c -> c.toBuilder().applyMutation(userAgentApplier).build())
.orElse((AwsRequestOverrideConfiguration.builder().applyMutation(userAgentApplier).build()));
return (T) request.toBuilder().overrideConfiguration(overrideConfiguration).build();
}
private T applySignerOverride(T request, Signer signer) {
if (request.overrideConfiguration().flatMap(c -> c.signer()).isPresent()) {
return request;
}
Consumer signerOverride = b -> b.signer(signer).build();
AwsRequestOverrideConfiguration overrideConfiguration = request.overrideConfiguration()
.map(c -> c.toBuilder().applyMutation(signerOverride).build())
.orElse((AwsRequestOverrideConfiguration.builder().applyMutation(signerOverride).build()));
return (T) request.toBuilder().overrideConfiguration(overrideConfiguration).build();
}
private static boolean isSignerOverridden(SdkClientConfiguration clientConfiguration) {
return Boolean.TRUE.equals(clientConfiguration.option(SdkClientOption.SIGNER_OVERRIDDEN));
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata) {
return protocolFactory.createErrorResponseHandler(operationMetadata);
}
}