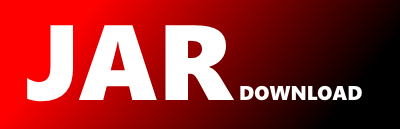
software.amazon.awssdk.services.mediastoredata.MediaStoreDataAsyncClient Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.mediastoredata;
import java.nio.file.Path;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.core.async.AsyncRequestBody;
import software.amazon.awssdk.core.async.AsyncResponseTransformer;
import software.amazon.awssdk.services.mediastoredata.model.DeleteObjectRequest;
import software.amazon.awssdk.services.mediastoredata.model.DeleteObjectResponse;
import software.amazon.awssdk.services.mediastoredata.model.DescribeObjectRequest;
import software.amazon.awssdk.services.mediastoredata.model.DescribeObjectResponse;
import software.amazon.awssdk.services.mediastoredata.model.GetObjectRequest;
import software.amazon.awssdk.services.mediastoredata.model.GetObjectResponse;
import software.amazon.awssdk.services.mediastoredata.model.ListItemsRequest;
import software.amazon.awssdk.services.mediastoredata.model.ListItemsResponse;
import software.amazon.awssdk.services.mediastoredata.model.PutObjectRequest;
import software.amazon.awssdk.services.mediastoredata.model.PutObjectResponse;
import software.amazon.awssdk.services.mediastoredata.paginators.ListItemsPublisher;
/**
* Service client for accessing MediaStore Data asynchronously. This can be created using the static {@link #builder()}
* method.
*
*
* An AWS Elemental MediaStore asset is an object, similar to an object in the Amazon S3 service. Objects are the
* fundamental entities that are stored in AWS Elemental MediaStore.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface MediaStoreDataAsyncClient extends AwsClient {
String SERVICE_NAME = "mediastore";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "data.mediastore";
/**
*
* Deletes an object at the specified path.
*
*
* @param deleteObjectRequest
* @return A Java Future containing the result of the DeleteObject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ContainerNotFoundException The specified container was not found for the specified account.
* - ObjectNotFoundException Could not perform an operation on an object that does not exist.
* - InternalServerErrorException The service is temporarily unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaStoreDataException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MediaStoreDataAsyncClient.DeleteObject
* @see AWS
* API Documentation
*/
default CompletableFuture deleteObject(DeleteObjectRequest deleteObjectRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an object at the specified path.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteObjectRequest.Builder} avoiding the need to
* create one manually via {@link DeleteObjectRequest#builder()}
*
*
* @param deleteObjectRequest
* A {@link Consumer} that will call methods on {@link DeleteObjectRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteObject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ContainerNotFoundException The specified container was not found for the specified account.
* - ObjectNotFoundException Could not perform an operation on an object that does not exist.
* - InternalServerErrorException The service is temporarily unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaStoreDataException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MediaStoreDataAsyncClient.DeleteObject
* @see AWS
* API Documentation
*/
default CompletableFuture deleteObject(Consumer deleteObjectRequest) {
return deleteObject(DeleteObjectRequest.builder().applyMutation(deleteObjectRequest).build());
}
/**
*
* Gets the headers for an object at the specified path.
*
*
* @param describeObjectRequest
* @return A Java Future containing the result of the DescribeObject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ContainerNotFoundException The specified container was not found for the specified account.
* - ObjectNotFoundException Could not perform an operation on an object that does not exist.
* - InternalServerErrorException The service is temporarily unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaStoreDataException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MediaStoreDataAsyncClient.DescribeObject
* @see AWS API Documentation
*/
default CompletableFuture describeObject(DescribeObjectRequest describeObjectRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the headers for an object at the specified path.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeObjectRequest.Builder} avoiding the need to
* create one manually via {@link DescribeObjectRequest#builder()}
*
*
* @param describeObjectRequest
* A {@link Consumer} that will call methods on {@link DescribeObjectRequest.Builder} to create a request.
* @return A Java Future containing the result of the DescribeObject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ContainerNotFoundException The specified container was not found for the specified account.
* - ObjectNotFoundException Could not perform an operation on an object that does not exist.
* - InternalServerErrorException The service is temporarily unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaStoreDataException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MediaStoreDataAsyncClient.DescribeObject
* @see AWS API Documentation
*/
default CompletableFuture describeObject(Consumer describeObjectRequest) {
return describeObject(DescribeObjectRequest.builder().applyMutation(describeObjectRequest).build());
}
/**
*
* Downloads the object at the specified path. If the object’s upload availability is set to streaming
,
* AWS Elemental MediaStore downloads the object even if it’s still uploading the object.
*
*
* @param getObjectRequest
* @param asyncResponseTransformer
* The response transformer for processing the streaming response in a non-blocking manner. See
* {@link AsyncResponseTransformer} for details on how this callback should be implemented and for links to
* precanned implementations for common scenarios like downloading to a file. The service documentation for
* the response content is as follows '
*
* The bytes of the object.
*
* '.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ContainerNotFoundException The specified container was not found for the specified account.
* - ObjectNotFoundException Could not perform an operation on an object that does not exist.
* - RequestedRangeNotSatisfiableException The requested content range is not valid.
* - InternalServerErrorException The service is temporarily unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaStoreDataException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MediaStoreDataAsyncClient.GetObject
* @see AWS API
* Documentation
*/
default CompletableFuture getObject(GetObjectRequest getObjectRequest,
AsyncResponseTransformer asyncResponseTransformer) {
throw new UnsupportedOperationException();
}
/**
*
* Downloads the object at the specified path. If the object’s upload availability is set to streaming
,
* AWS Elemental MediaStore downloads the object even if it’s still uploading the object.
*
*
*
* This is a convenience which creates an instance of the {@link GetObjectRequest.Builder} avoiding the need to
* create one manually via {@link GetObjectRequest#builder()}
*
*
* @param getObjectRequest
* A {@link Consumer} that will call methods on {@link GetObjectRequest.Builder} to create a request.
* @param asyncResponseTransformer
* The response transformer for processing the streaming response in a non-blocking manner. See
* {@link AsyncResponseTransformer} for details on how this callback should be implemented and for links to
* precanned implementations for common scenarios like downloading to a file. The service documentation for
* the response content is as follows '
*
* The bytes of the object.
*
* '.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ContainerNotFoundException The specified container was not found for the specified account.
* - ObjectNotFoundException Could not perform an operation on an object that does not exist.
* - RequestedRangeNotSatisfiableException The requested content range is not valid.
* - InternalServerErrorException The service is temporarily unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaStoreDataException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MediaStoreDataAsyncClient.GetObject
* @see AWS API
* Documentation
*/
default CompletableFuture getObject(Consumer getObjectRequest,
AsyncResponseTransformer asyncResponseTransformer) {
return getObject(GetObjectRequest.builder().applyMutation(getObjectRequest).build(), asyncResponseTransformer);
}
/**
*
* Downloads the object at the specified path. If the object’s upload availability is set to streaming
,
* AWS Elemental MediaStore downloads the object even if it’s still uploading the object.
*
*
* @param getObjectRequest
* @param destinationPath
* {@link Path} to file that response contents will be written to. The file must not exist or this method
* will throw an exception. If the file is not writable by the current user then an exception will be thrown.
* The service documentation for the response content is as follows '
*
* The bytes of the object.
*
* '.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ContainerNotFoundException The specified container was not found for the specified account.
* - ObjectNotFoundException Could not perform an operation on an object that does not exist.
* - RequestedRangeNotSatisfiableException The requested content range is not valid.
* - InternalServerErrorException The service is temporarily unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaStoreDataException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MediaStoreDataAsyncClient.GetObject
* @see AWS API
* Documentation
*/
default CompletableFuture getObject(GetObjectRequest getObjectRequest, Path destinationPath) {
return getObject(getObjectRequest, AsyncResponseTransformer.toFile(destinationPath));
}
/**
*
* Downloads the object at the specified path. If the object’s upload availability is set to streaming
,
* AWS Elemental MediaStore downloads the object even if it’s still uploading the object.
*
*
*
* This is a convenience which creates an instance of the {@link GetObjectRequest.Builder} avoiding the need to
* create one manually via {@link GetObjectRequest#builder()}
*
*
* @param getObjectRequest
* A {@link Consumer} that will call methods on {@link GetObjectRequest.Builder} to create a request.
* @param destinationPath
* {@link Path} to file that response contents will be written to. The file must not exist or this method
* will throw an exception. If the file is not writable by the current user then an exception will be thrown.
* The service documentation for the response content is as follows '
*
* The bytes of the object.
*
* '.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ContainerNotFoundException The specified container was not found for the specified account.
* - ObjectNotFoundException Could not perform an operation on an object that does not exist.
* - RequestedRangeNotSatisfiableException The requested content range is not valid.
* - InternalServerErrorException The service is temporarily unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaStoreDataException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MediaStoreDataAsyncClient.GetObject
* @see AWS API
* Documentation
*/
default CompletableFuture getObject(Consumer getObjectRequest,
Path destinationPath) {
return getObject(GetObjectRequest.builder().applyMutation(getObjectRequest).build(), destinationPath);
}
/**
*
* Provides a list of metadata entries about folders and objects in the specified folder.
*
*
* @param listItemsRequest
* @return A Java Future containing the result of the ListItems operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ContainerNotFoundException The specified container was not found for the specified account.
* - InternalServerErrorException The service is temporarily unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaStoreDataException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MediaStoreDataAsyncClient.ListItems
* @see AWS API
* Documentation
*/
default CompletableFuture listItems(ListItemsRequest listItemsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Provides a list of metadata entries about folders and objects in the specified folder.
*
*
*
* This is a convenience which creates an instance of the {@link ListItemsRequest.Builder} avoiding the need to
* create one manually via {@link ListItemsRequest#builder()}
*
*
* @param listItemsRequest
* A {@link Consumer} that will call methods on {@link ListItemsRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListItems operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ContainerNotFoundException The specified container was not found for the specified account.
* - InternalServerErrorException The service is temporarily unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaStoreDataException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MediaStoreDataAsyncClient.ListItems
* @see AWS API
* Documentation
*/
default CompletableFuture listItems(Consumer listItemsRequest) {
return listItems(ListItemsRequest.builder().applyMutation(listItemsRequest).build());
}
/**
*
* Provides a list of metadata entries about folders and objects in the specified folder.
*
*
*
* This is a variant of {@link #listItems(software.amazon.awssdk.services.mediastoredata.model.ListItemsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.mediastoredata.paginators.ListItemsPublisher publisher = client.listItemsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.mediastoredata.paginators.ListItemsPublisher publisher = client.listItemsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.mediastoredata.model.ListItemsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listItems(software.amazon.awssdk.services.mediastoredata.model.ListItemsRequest)} operation.
*
*
* @param listItemsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ContainerNotFoundException The specified container was not found for the specified account.
* - InternalServerErrorException The service is temporarily unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaStoreDataException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MediaStoreDataAsyncClient.ListItems
* @see AWS API
* Documentation
*/
default ListItemsPublisher listItemsPaginator(ListItemsRequest listItemsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Provides a list of metadata entries about folders and objects in the specified folder.
*
*
*
* This is a variant of {@link #listItems(software.amazon.awssdk.services.mediastoredata.model.ListItemsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.mediastoredata.paginators.ListItemsPublisher publisher = client.listItemsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.mediastoredata.paginators.ListItemsPublisher publisher = client.listItemsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.mediastoredata.model.ListItemsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listItems(software.amazon.awssdk.services.mediastoredata.model.ListItemsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListItemsRequest.Builder} avoiding the need to
* create one manually via {@link ListItemsRequest#builder()}
*
*
* @param listItemsRequest
* A {@link Consumer} that will call methods on {@link ListItemsRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ContainerNotFoundException The specified container was not found for the specified account.
* - InternalServerErrorException The service is temporarily unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaStoreDataException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MediaStoreDataAsyncClient.ListItems
* @see AWS API
* Documentation
*/
default ListItemsPublisher listItemsPaginator(Consumer listItemsRequest) {
return listItemsPaginator(ListItemsRequest.builder().applyMutation(listItemsRequest).build());
}
/**
*
* Uploads an object to the specified path. Object sizes are limited to 25 MB for standard upload availability and
* 10 MB for streaming upload availability.
*
*
* @param putObjectRequest
* @param requestBody
* Functional interface that can be implemented to produce the request content in a non-blocking manner. The
* size of the content is expected to be known up front. See {@link AsyncRequestBody} for specific details on
* implementing this interface as well as links to precanned implementations for common scenarios like
* uploading from a file. The service documentation for the request content is as follows '
*
* The bytes to be stored.
*
* '
* @return A Java Future containing the result of the PutObject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ContainerNotFoundException The specified container was not found for the specified account.
* - InternalServerErrorException The service is temporarily unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaStoreDataException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MediaStoreDataAsyncClient.PutObject
* @see AWS API
* Documentation
*/
default CompletableFuture putObject(PutObjectRequest putObjectRequest, AsyncRequestBody requestBody) {
throw new UnsupportedOperationException();
}
/**
*
* Uploads an object to the specified path. Object sizes are limited to 25 MB for standard upload availability and
* 10 MB for streaming upload availability.
*
*
*
* This is a convenience which creates an instance of the {@link PutObjectRequest.Builder} avoiding the need to
* create one manually via {@link PutObjectRequest#builder()}
*
*
* @param putObjectRequest
* A {@link Consumer} that will call methods on {@link PutObjectRequest.Builder} to create a request.
* @param requestBody
* Functional interface that can be implemented to produce the request content in a non-blocking manner. The
* size of the content is expected to be known up front. See {@link AsyncRequestBody} for specific details on
* implementing this interface as well as links to precanned implementations for common scenarios like
* uploading from a file. The service documentation for the request content is as follows '
*
* The bytes to be stored.
*
* '
* @return A Java Future containing the result of the PutObject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ContainerNotFoundException The specified container was not found for the specified account.
* - InternalServerErrorException The service is temporarily unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaStoreDataException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MediaStoreDataAsyncClient.PutObject
* @see AWS API
* Documentation
*/
default CompletableFuture putObject(Consumer putObjectRequest,
AsyncRequestBody requestBody) {
return putObject(PutObjectRequest.builder().applyMutation(putObjectRequest).build(), requestBody);
}
/**
*
* Uploads an object to the specified path. Object sizes are limited to 25 MB for standard upload availability and
* 10 MB for streaming upload availability.
*
*
* @param putObjectRequest
* @param sourcePath
* {@link Path} to file containing data to send to the service. File will be read entirely and may be read
* multiple times in the event of a retry. If the file does not exist or the current user does not have
* access to read it then an exception will be thrown. The service documentation for the request content is
* as follows '
*
* The bytes to be stored.
*
* '
* @return A Java Future containing the result of the PutObject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ContainerNotFoundException The specified container was not found for the specified account.
* - InternalServerErrorException The service is temporarily unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaStoreDataException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MediaStoreDataAsyncClient.PutObject
* @see AWS API
* Documentation
*/
default CompletableFuture putObject(PutObjectRequest putObjectRequest, Path sourcePath) {
return putObject(putObjectRequest, AsyncRequestBody.fromFile(sourcePath));
}
/**
*
* Uploads an object to the specified path. Object sizes are limited to 25 MB for standard upload availability and
* 10 MB for streaming upload availability.
*
*
*
* This is a convenience which creates an instance of the {@link PutObjectRequest.Builder} avoiding the need to
* create one manually via {@link PutObjectRequest#builder()}
*
*
* @param putObjectRequest
* A {@link Consumer} that will call methods on {@link PutObjectRequest.Builder} to create a request.
* @param sourcePath
* {@link Path} to file containing data to send to the service. File will be read entirely and may be read
* multiple times in the event of a retry. If the file does not exist or the current user does not have
* access to read it then an exception will be thrown. The service documentation for the request content is
* as follows '
*
* The bytes to be stored.
*
* '
* @return A Java Future containing the result of the PutObject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ContainerNotFoundException The specified container was not found for the specified account.
* - InternalServerErrorException The service is temporarily unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MediaStoreDataException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MediaStoreDataAsyncClient.PutObject
* @see AWS API
* Documentation
*/
default CompletableFuture putObject(Consumer putObjectRequest, Path sourcePath) {
return putObject(PutObjectRequest.builder().applyMutation(putObjectRequest).build(), sourcePath);
}
@Override
default MediaStoreDataServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
/**
* Create a {@link MediaStoreDataAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static MediaStoreDataAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link MediaStoreDataAsyncClient}.
*/
static MediaStoreDataAsyncClientBuilder builder() {
return new DefaultMediaStoreDataAsyncClientBuilder();
}
}