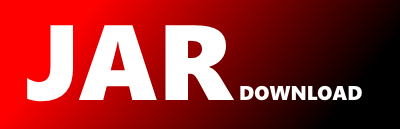
software.amazon.awssdk.services.mediastoredata.MediaStoreDataClient Maven / Gradle / Ivy
Show all versions of mediastoredata Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.mediastoredata;
import java.nio.file.Path;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.ResponseBytes;
import software.amazon.awssdk.core.ResponseInputStream;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.sync.RequestBody;
import software.amazon.awssdk.core.sync.ResponseTransformer;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.mediastoredata.model.ContainerNotFoundException;
import software.amazon.awssdk.services.mediastoredata.model.DeleteObjectRequest;
import software.amazon.awssdk.services.mediastoredata.model.DeleteObjectResponse;
import software.amazon.awssdk.services.mediastoredata.model.DescribeObjectRequest;
import software.amazon.awssdk.services.mediastoredata.model.DescribeObjectResponse;
import software.amazon.awssdk.services.mediastoredata.model.GetObjectRequest;
import software.amazon.awssdk.services.mediastoredata.model.GetObjectResponse;
import software.amazon.awssdk.services.mediastoredata.model.InternalServerErrorException;
import software.amazon.awssdk.services.mediastoredata.model.ListItemsRequest;
import software.amazon.awssdk.services.mediastoredata.model.ListItemsResponse;
import software.amazon.awssdk.services.mediastoredata.model.MediaStoreDataException;
import software.amazon.awssdk.services.mediastoredata.model.ObjectNotFoundException;
import software.amazon.awssdk.services.mediastoredata.model.PutObjectRequest;
import software.amazon.awssdk.services.mediastoredata.model.PutObjectResponse;
import software.amazon.awssdk.services.mediastoredata.model.RequestedRangeNotSatisfiableException;
import software.amazon.awssdk.services.mediastoredata.paginators.ListItemsIterable;
/**
* Service client for accessing MediaStore Data. This can be created using the static {@link #builder()} method.
*
*
* An AWS Elemental MediaStore asset is an object, similar to an object in the Amazon S3 service. Objects are the
* fundamental entities that are stored in AWS Elemental MediaStore.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface MediaStoreDataClient extends AwsClient {
String SERVICE_NAME = "mediastore";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "data.mediastore";
/**
*
* Deletes an object at the specified path.
*
*
* @param deleteObjectRequest
* @return Result of the DeleteObject operation returned by the service.
* @throws ContainerNotFoundException
* The specified container was not found for the specified account.
* @throws ObjectNotFoundException
* Could not perform an operation on an object that does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreDataClient.DeleteObject
* @see AWS
* API Documentation
*/
default DeleteObjectResponse deleteObject(DeleteObjectRequest deleteObjectRequest) throws ContainerNotFoundException,
ObjectNotFoundException, InternalServerErrorException, AwsServiceException, SdkClientException,
MediaStoreDataException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an object at the specified path.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteObjectRequest.Builder} avoiding the need to
* create one manually via {@link DeleteObjectRequest#builder()}
*
*
* @param deleteObjectRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.mediastoredata.model.DeleteObjectRequest.Builder} to create a
* request.
* @return Result of the DeleteObject operation returned by the service.
* @throws ContainerNotFoundException
* The specified container was not found for the specified account.
* @throws ObjectNotFoundException
* Could not perform an operation on an object that does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreDataClient.DeleteObject
* @see AWS
* API Documentation
*/
default DeleteObjectResponse deleteObject(Consumer deleteObjectRequest)
throws ContainerNotFoundException, ObjectNotFoundException, InternalServerErrorException, AwsServiceException,
SdkClientException, MediaStoreDataException {
return deleteObject(DeleteObjectRequest.builder().applyMutation(deleteObjectRequest).build());
}
/**
*
* Gets the headers for an object at the specified path.
*
*
* @param describeObjectRequest
* @return Result of the DescribeObject operation returned by the service.
* @throws ContainerNotFoundException
* The specified container was not found for the specified account.
* @throws ObjectNotFoundException
* Could not perform an operation on an object that does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreDataClient.DescribeObject
* @see AWS API Documentation
*/
default DescribeObjectResponse describeObject(DescribeObjectRequest describeObjectRequest) throws ContainerNotFoundException,
ObjectNotFoundException, InternalServerErrorException, AwsServiceException, SdkClientException,
MediaStoreDataException {
throw new UnsupportedOperationException();
}
/**
*
* Gets the headers for an object at the specified path.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeObjectRequest.Builder} avoiding the need to
* create one manually via {@link DescribeObjectRequest#builder()}
*
*
* @param describeObjectRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.mediastoredata.model.DescribeObjectRequest.Builder} to create a
* request.
* @return Result of the DescribeObject operation returned by the service.
* @throws ContainerNotFoundException
* The specified container was not found for the specified account.
* @throws ObjectNotFoundException
* Could not perform an operation on an object that does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreDataClient.DescribeObject
* @see AWS API Documentation
*/
default DescribeObjectResponse describeObject(Consumer describeObjectRequest)
throws ContainerNotFoundException, ObjectNotFoundException, InternalServerErrorException, AwsServiceException,
SdkClientException, MediaStoreDataException {
return describeObject(DescribeObjectRequest.builder().applyMutation(describeObjectRequest).build());
}
/**
*
* Downloads the object at the specified path. If the object’s upload availability is set to streaming
,
* AWS Elemental MediaStore downloads the object even if it’s still uploading the object.
*
*
* @param getObjectRequest
* @param responseTransformer
* Functional interface for processing the streamed response content. The unmarshalled GetObjectResponse and
* an InputStream to the response content are provided as parameters to the callback. The callback may return
* a transformed type which will be the return value of this method. See
* {@link software.amazon.awssdk.core.sync.ResponseTransformer} for details on implementing this interface
* and for links to pre-canned implementations for common scenarios like downloading to a file. The service
* documentation for the response content is as follows '
*
* The bytes of the object.
*
* '.
* @return The transformed result of the ResponseTransformer.
* @throws ContainerNotFoundException
* The specified container was not found for the specified account.
* @throws ObjectNotFoundException
* Could not perform an operation on an object that does not exist.
* @throws RequestedRangeNotSatisfiableException
* The requested content range is not valid.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreDataClient.GetObject
* @see AWS API
* Documentation
*/
default ReturnT getObject(GetObjectRequest getObjectRequest,
ResponseTransformer responseTransformer) throws ContainerNotFoundException,
ObjectNotFoundException, RequestedRangeNotSatisfiableException, InternalServerErrorException, AwsServiceException,
SdkClientException, MediaStoreDataException {
throw new UnsupportedOperationException();
}
/**
*
* Downloads the object at the specified path. If the object’s upload availability is set to streaming
,
* AWS Elemental MediaStore downloads the object even if it’s still uploading the object.
*
*
*
* This is a convenience which creates an instance of the {@link GetObjectRequest.Builder} avoiding the need to
* create one manually via {@link GetObjectRequest#builder()}
*
*
* @param getObjectRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.mediastoredata.model.GetObjectRequest.Builder} to create a request.
* @param responseTransformer
* Functional interface for processing the streamed response content. The unmarshalled GetObjectResponse and
* an InputStream to the response content are provided as parameters to the callback. The callback may return
* a transformed type which will be the return value of this method. See
* {@link software.amazon.awssdk.core.sync.ResponseTransformer} for details on implementing this interface
* and for links to pre-canned implementations for common scenarios like downloading to a file. The service
* documentation for the response content is as follows '
*
* The bytes of the object.
*
* '.
* @return The transformed result of the ResponseTransformer.
* @throws ContainerNotFoundException
* The specified container was not found for the specified account.
* @throws ObjectNotFoundException
* Could not perform an operation on an object that does not exist.
* @throws RequestedRangeNotSatisfiableException
* The requested content range is not valid.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreDataClient.GetObject
* @see AWS API
* Documentation
*/
default ReturnT getObject(Consumer getObjectRequest,
ResponseTransformer responseTransformer) throws ContainerNotFoundException,
ObjectNotFoundException, RequestedRangeNotSatisfiableException, InternalServerErrorException, AwsServiceException,
SdkClientException, MediaStoreDataException {
return getObject(GetObjectRequest.builder().applyMutation(getObjectRequest).build(), responseTransformer);
}
/**
*
* Downloads the object at the specified path. If the object’s upload availability is set to streaming
,
* AWS Elemental MediaStore downloads the object even if it’s still uploading the object.
*
*
* @param getObjectRequest
* @param destinationPath
* {@link Path} to file that response contents will be written to. The file must not exist or this method
* will throw an exception. If the file is not writable by the current user then an exception will be thrown.
* The service documentation for the response content is as follows '
*
* The bytes of the object.
*
* '.
* @return The transformed result of the ResponseTransformer.
* @throws ContainerNotFoundException
* The specified container was not found for the specified account.
* @throws ObjectNotFoundException
* Could not perform an operation on an object that does not exist.
* @throws RequestedRangeNotSatisfiableException
* The requested content range is not valid.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreDataClient.GetObject
* @see #getObject(GetObjectRequest, ResponseTransformer)
* @see AWS API
* Documentation
*/
default GetObjectResponse getObject(GetObjectRequest getObjectRequest, Path destinationPath)
throws ContainerNotFoundException, ObjectNotFoundException, RequestedRangeNotSatisfiableException,
InternalServerErrorException, AwsServiceException, SdkClientException, MediaStoreDataException {
return getObject(getObjectRequest, ResponseTransformer.toFile(destinationPath));
}
/**
*
* Downloads the object at the specified path. If the object’s upload availability is set to streaming
,
* AWS Elemental MediaStore downloads the object even if it’s still uploading the object.
*
*
*
* This is a convenience which creates an instance of the {@link GetObjectRequest.Builder} avoiding the need to
* create one manually via {@link GetObjectRequest#builder()}
*
*
* @param getObjectRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.mediastoredata.model.GetObjectRequest.Builder} to create a request.
* @param destinationPath
* {@link Path} to file that response contents will be written to. The file must not exist or this method
* will throw an exception. If the file is not writable by the current user then an exception will be thrown.
* The service documentation for the response content is as follows '
*
* The bytes of the object.
*
* '.
* @return The transformed result of the ResponseTransformer.
* @throws ContainerNotFoundException
* The specified container was not found for the specified account.
* @throws ObjectNotFoundException
* Could not perform an operation on an object that does not exist.
* @throws RequestedRangeNotSatisfiableException
* The requested content range is not valid.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreDataClient.GetObject
* @see #getObject(GetObjectRequest, ResponseTransformer)
* @see AWS API
* Documentation
*/
default GetObjectResponse getObject(Consumer getObjectRequest, Path destinationPath)
throws ContainerNotFoundException, ObjectNotFoundException, RequestedRangeNotSatisfiableException,
InternalServerErrorException, AwsServiceException, SdkClientException, MediaStoreDataException {
return getObject(GetObjectRequest.builder().applyMutation(getObjectRequest).build(), destinationPath);
}
/**
*
* Downloads the object at the specified path. If the object’s upload availability is set to streaming
,
* AWS Elemental MediaStore downloads the object even if it’s still uploading the object.
*
*
* @param getObjectRequest
* @return A {@link ResponseInputStream} containing data streamed from service. Note that this is an unmanaged
* reference to the underlying HTTP connection so great care must be taken to ensure all data if fully read
* from the input stream and that it is properly closed. Failure to do so may result in sub-optimal behavior
* and exhausting connections in the connection pool. The unmarshalled response object can be obtained via
* {@link ResponseInputStream#response()}. The service documentation for the response content is as follows
* '
*
* The bytes of the object.
*
* '.
* @throws ContainerNotFoundException
* The specified container was not found for the specified account.
* @throws ObjectNotFoundException
* Could not perform an operation on an object that does not exist.
* @throws RequestedRangeNotSatisfiableException
* The requested content range is not valid.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreDataClient.GetObject
* @see #getObject(getObject, ResponseTransformer)
* @see AWS API
* Documentation
*/
default ResponseInputStream getObject(GetObjectRequest getObjectRequest)
throws ContainerNotFoundException, ObjectNotFoundException, RequestedRangeNotSatisfiableException,
InternalServerErrorException, AwsServiceException, SdkClientException, MediaStoreDataException {
return getObject(getObjectRequest, ResponseTransformer.toInputStream());
}
/**
*
* Downloads the object at the specified path. If the object’s upload availability is set to streaming
,
* AWS Elemental MediaStore downloads the object even if it’s still uploading the object.
*
*
*
* This is a convenience which creates an instance of the {@link GetObjectRequest.Builder} avoiding the need to
* create one manually via {@link GetObjectRequest#builder()}
*
*
* @param getObjectRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.mediastoredata.model.GetObjectRequest.Builder} to create a request.
* @return A {@link ResponseInputStream} containing data streamed from service. Note that this is an unmanaged
* reference to the underlying HTTP connection so great care must be taken to ensure all data if fully read
* from the input stream and that it is properly closed. Failure to do so may result in sub-optimal behavior
* and exhausting connections in the connection pool. The unmarshalled response object can be obtained via
* {@link ResponseInputStream#response()}. The service documentation for the response content is as follows
* '
*
* The bytes of the object.
*
* '.
* @throws ContainerNotFoundException
* The specified container was not found for the specified account.
* @throws ObjectNotFoundException
* Could not perform an operation on an object that does not exist.
* @throws RequestedRangeNotSatisfiableException
* The requested content range is not valid.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreDataClient.GetObject
* @see #getObject(getObject, ResponseTransformer)
* @see AWS API
* Documentation
*/
default ResponseInputStream getObject(Consumer getObjectRequest)
throws ContainerNotFoundException, ObjectNotFoundException, RequestedRangeNotSatisfiableException,
InternalServerErrorException, AwsServiceException, SdkClientException, MediaStoreDataException {
return getObject(GetObjectRequest.builder().applyMutation(getObjectRequest).build());
}
/**
*
* Downloads the object at the specified path. If the object’s upload availability is set to streaming
,
* AWS Elemental MediaStore downloads the object even if it’s still uploading the object.
*
*
* @param getObjectRequest
* @return A {@link ResponseBytes} that loads the data streamed from the service into memory and exposes it in
* convenient in-memory representations like a byte buffer or string. The unmarshalled response object can
* be obtained via {@link ResponseBytes#response()}. The service documentation for the response content is
* as follows '
*
* The bytes of the object.
*
* '.
* @throws ContainerNotFoundException
* The specified container was not found for the specified account.
* @throws ObjectNotFoundException
* Could not perform an operation on an object that does not exist.
* @throws RequestedRangeNotSatisfiableException
* The requested content range is not valid.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreDataClient.GetObject
* @see #getObject(getObject, ResponseTransformer)
* @see AWS API
* Documentation
*/
default ResponseBytes getObjectAsBytes(GetObjectRequest getObjectRequest)
throws ContainerNotFoundException, ObjectNotFoundException, RequestedRangeNotSatisfiableException,
InternalServerErrorException, AwsServiceException, SdkClientException, MediaStoreDataException {
return getObject(getObjectRequest, ResponseTransformer.toBytes());
}
/**
*
* Downloads the object at the specified path. If the object’s upload availability is set to streaming
,
* AWS Elemental MediaStore downloads the object even if it’s still uploading the object.
*
*
*
* This is a convenience which creates an instance of the {@link GetObjectRequest.Builder} avoiding the need to
* create one manually via {@link GetObjectRequest#builder()}
*
*
* @param getObjectRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.mediastoredata.model.GetObjectRequest.Builder} to create a request.
* @return A {@link ResponseBytes} that loads the data streamed from the service into memory and exposes it in
* convenient in-memory representations like a byte buffer or string. The unmarshalled response object can
* be obtained via {@link ResponseBytes#response()}. The service documentation for the response content is
* as follows '
*
* The bytes of the object.
*
* '.
* @throws ContainerNotFoundException
* The specified container was not found for the specified account.
* @throws ObjectNotFoundException
* Could not perform an operation on an object that does not exist.
* @throws RequestedRangeNotSatisfiableException
* The requested content range is not valid.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreDataClient.GetObject
* @see #getObject(getObject, ResponseTransformer)
* @see AWS API
* Documentation
*/
default ResponseBytes getObjectAsBytes(Consumer getObjectRequest)
throws ContainerNotFoundException, ObjectNotFoundException, RequestedRangeNotSatisfiableException,
InternalServerErrorException, AwsServiceException, SdkClientException, MediaStoreDataException {
return getObjectAsBytes(GetObjectRequest.builder().applyMutation(getObjectRequest).build());
}
/**
*
* Provides a list of metadata entries about folders and objects in the specified folder.
*
*
* @param listItemsRequest
* @return Result of the ListItems operation returned by the service.
* @throws ContainerNotFoundException
* The specified container was not found for the specified account.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreDataClient.ListItems
* @see AWS API
* Documentation
*/
default ListItemsResponse listItems(ListItemsRequest listItemsRequest) throws ContainerNotFoundException,
InternalServerErrorException, AwsServiceException, SdkClientException, MediaStoreDataException {
throw new UnsupportedOperationException();
}
/**
*
* Provides a list of metadata entries about folders and objects in the specified folder.
*
*
*
* This is a convenience which creates an instance of the {@link ListItemsRequest.Builder} avoiding the need to
* create one manually via {@link ListItemsRequest#builder()}
*
*
* @param listItemsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.mediastoredata.model.ListItemsRequest.Builder} to create a request.
* @return Result of the ListItems operation returned by the service.
* @throws ContainerNotFoundException
* The specified container was not found for the specified account.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreDataClient.ListItems
* @see AWS API
* Documentation
*/
default ListItemsResponse listItems(Consumer listItemsRequest) throws ContainerNotFoundException,
InternalServerErrorException, AwsServiceException, SdkClientException, MediaStoreDataException {
return listItems(ListItemsRequest.builder().applyMutation(listItemsRequest).build());
}
/**
*
* This is a variant of {@link #listItems(software.amazon.awssdk.services.mediastoredata.model.ListItemsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.mediastoredata.paginators.ListItemsIterable responses = client.listItemsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.mediastoredata.paginators.ListItemsIterable responses = client.listItemsPaginator(request);
* for (software.amazon.awssdk.services.mediastoredata.model.ListItemsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.mediastoredata.paginators.ListItemsIterable responses = client.listItemsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listItems(software.amazon.awssdk.services.mediastoredata.model.ListItemsRequest)} operation.
*
*
* @param listItemsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ContainerNotFoundException
* The specified container was not found for the specified account.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreDataClient.ListItems
* @see AWS API
* Documentation
*/
default ListItemsIterable listItemsPaginator(ListItemsRequest listItemsRequest) throws ContainerNotFoundException,
InternalServerErrorException, AwsServiceException, SdkClientException, MediaStoreDataException {
return new ListItemsIterable(this, listItemsRequest);
}
/**
*
* This is a variant of {@link #listItems(software.amazon.awssdk.services.mediastoredata.model.ListItemsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.mediastoredata.paginators.ListItemsIterable responses = client.listItemsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.mediastoredata.paginators.ListItemsIterable responses = client.listItemsPaginator(request);
* for (software.amazon.awssdk.services.mediastoredata.model.ListItemsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.mediastoredata.paginators.ListItemsIterable responses = client.listItemsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listItems(software.amazon.awssdk.services.mediastoredata.model.ListItemsRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListItemsRequest.Builder} avoiding the need to
* create one manually via {@link ListItemsRequest#builder()}
*
*
* @param listItemsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.mediastoredata.model.ListItemsRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ContainerNotFoundException
* The specified container was not found for the specified account.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreDataClient.ListItems
* @see AWS API
* Documentation
*/
default ListItemsIterable listItemsPaginator(Consumer listItemsRequest)
throws ContainerNotFoundException, InternalServerErrorException, AwsServiceException, SdkClientException,
MediaStoreDataException {
return listItemsPaginator(ListItemsRequest.builder().applyMutation(listItemsRequest).build());
}
/**
*
* Uploads an object to the specified path. Object sizes are limited to 25 MB for standard upload availability and
* 10 MB for streaming upload availability.
*
*
* @param putObjectRequest
* @param requestBody
* The content to send to the service. A {@link RequestBody} can be created using one of several factory
* methods for various sources of data. For example, to create a request body from a file you can do the
* following.
*
*
* {@code RequestBody.fromFile(new File("myfile.txt"))}
*
*
* See documentation in {@link RequestBody} for additional details and which sources of data are supported.
* The service documentation for the request content is as follows '
*
* The bytes to be stored.
*
* '
* @return Result of the PutObject operation returned by the service.
* @throws ContainerNotFoundException
* The specified container was not found for the specified account.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreDataClient.PutObject
* @see AWS API
* Documentation
*/
default PutObjectResponse putObject(PutObjectRequest putObjectRequest, RequestBody requestBody)
throws ContainerNotFoundException, InternalServerErrorException, AwsServiceException, SdkClientException,
MediaStoreDataException {
throw new UnsupportedOperationException();
}
/**
*
* Uploads an object to the specified path. Object sizes are limited to 25 MB for standard upload availability and
* 10 MB for streaming upload availability.
*
*
*
* This is a convenience which creates an instance of the {@link PutObjectRequest.Builder} avoiding the need to
* create one manually via {@link PutObjectRequest#builder()}
*
*
* @param putObjectRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.mediastoredata.model.PutObjectRequest.Builder} to create a request.
* @param requestBody
* The content to send to the service. A {@link RequestBody} can be created using one of several factory
* methods for various sources of data. For example, to create a request body from a file you can do the
* following.
*
*
* {@code RequestBody.fromFile(new File("myfile.txt"))}
*
*
* See documentation in {@link RequestBody} for additional details and which sources of data are supported.
* The service documentation for the request content is as follows '
*
* The bytes to be stored.
*
* '
* @return Result of the PutObject operation returned by the service.
* @throws ContainerNotFoundException
* The specified container was not found for the specified account.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreDataClient.PutObject
* @see AWS API
* Documentation
*/
default PutObjectResponse putObject(Consumer putObjectRequest, RequestBody requestBody)
throws ContainerNotFoundException, InternalServerErrorException, AwsServiceException, SdkClientException,
MediaStoreDataException {
return putObject(PutObjectRequest.builder().applyMutation(putObjectRequest).build(), requestBody);
}
/**
*
* Uploads an object to the specified path. Object sizes are limited to 25 MB for standard upload availability and
* 10 MB for streaming upload availability.
*
*
* @param putObjectRequest
* @param sourcePath
* {@link Path} to file containing data to send to the service. File will be read entirely and may be read
* multiple times in the event of a retry. If the file does not exist or the current user does not have
* access to read it then an exception will be thrown. The service documentation for the request content is
* as follows '
*
* The bytes to be stored.
*
* '
* @return Result of the PutObject operation returned by the service.
* @throws ContainerNotFoundException
* The specified container was not found for the specified account.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreDataClient.PutObject
* @see #putObject(PutObjectRequest, RequestBody)
* @see AWS API
* Documentation
*/
default PutObjectResponse putObject(PutObjectRequest putObjectRequest, Path sourcePath) throws ContainerNotFoundException,
InternalServerErrorException, AwsServiceException, SdkClientException, MediaStoreDataException {
return putObject(putObjectRequest, RequestBody.fromFile(sourcePath));
}
/**
*
* Uploads an object to the specified path. Object sizes are limited to 25 MB for standard upload availability and
* 10 MB for streaming upload availability.
*
*
*
* This is a convenience which creates an instance of the {@link PutObjectRequest.Builder} avoiding the need to
* create one manually via {@link PutObjectRequest#builder()}
*
*
* @param putObjectRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.mediastoredata.model.PutObjectRequest.Builder} to create a request.
* @param sourcePath
* {@link Path} to file containing data to send to the service. File will be read entirely and may be read
* multiple times in the event of a retry. If the file does not exist or the current user does not have
* access to read it then an exception will be thrown. The service documentation for the request content is
* as follows '
*
* The bytes to be stored.
*
* '
* @return Result of the PutObject operation returned by the service.
* @throws ContainerNotFoundException
* The specified container was not found for the specified account.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MediaStoreDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MediaStoreDataClient.PutObject
* @see #putObject(PutObjectRequest, RequestBody)
* @see AWS API
* Documentation
*/
default PutObjectResponse putObject(Consumer putObjectRequest, Path sourcePath)
throws ContainerNotFoundException, InternalServerErrorException, AwsServiceException, SdkClientException,
MediaStoreDataException {
return putObject(PutObjectRequest.builder().applyMutation(putObjectRequest).build(), sourcePath);
}
/**
* Create a {@link MediaStoreDataClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static MediaStoreDataClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link MediaStoreDataClient}.
*/
static MediaStoreDataClientBuilder builder() {
return new DefaultMediaStoreDataClientBuilder();
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of(SERVICE_METADATA_ID);
}
@Override
default MediaStoreDataServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
}