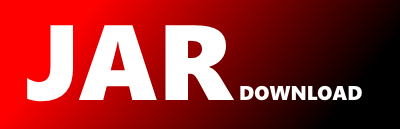
software.amazon.awssdk.services.mediastoredata.model.Item Maven / Gradle / Ivy
Show all versions of mediastoredata Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.mediastoredata.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* A metadata entry for a folder or object.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Item implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Name")
.getter(getter(Item::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Name").build()).build();
private static final SdkField TYPE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Type")
.getter(getter(Item::typeAsString)).setter(setter(Builder::type))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Type").build()).build();
private static final SdkField E_TAG_FIELD = SdkField. builder(MarshallingType.STRING).memberName("ETag")
.getter(getter(Item::eTag)).setter(setter(Builder::eTag))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ETag").build()).build();
private static final SdkField LAST_MODIFIED_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("LastModified").getter(getter(Item::lastModified)).setter(setter(Builder::lastModified))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastModified").build()).build();
private static final SdkField CONTENT_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ContentType").getter(getter(Item::contentType)).setter(setter(Builder::contentType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ContentType").build()).build();
private static final SdkField CONTENT_LENGTH_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("ContentLength").getter(getter(Item::contentLength)).setter(setter(Builder::contentLength))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ContentLength").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(NAME_FIELD, TYPE_FIELD,
E_TAG_FIELD, LAST_MODIFIED_FIELD, CONTENT_TYPE_FIELD, CONTENT_LENGTH_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final String name;
private final String type;
private final String eTag;
private final Instant lastModified;
private final String contentType;
private final Long contentLength;
private Item(BuilderImpl builder) {
this.name = builder.name;
this.type = builder.type;
this.eTag = builder.eTag;
this.lastModified = builder.lastModified;
this.contentType = builder.contentType;
this.contentLength = builder.contentLength;
}
/**
*
* The name of the item.
*
*
* @return The name of the item.
*/
public final String name() {
return name;
}
/**
*
* The item type (folder or object).
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link ItemType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The item type (folder or object).
* @see ItemType
*/
public final ItemType type() {
return ItemType.fromValue(type);
}
/**
*
* The item type (folder or object).
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link ItemType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The item type (folder or object).
* @see ItemType
*/
public final String typeAsString() {
return type;
}
/**
*
* The ETag that represents a unique instance of the item.
*
*
* @return The ETag that represents a unique instance of the item.
*/
public final String eTag() {
return eTag;
}
/**
*
* The date and time that the item was last modified.
*
*
* @return The date and time that the item was last modified.
*/
public final Instant lastModified() {
return lastModified;
}
/**
*
* The content type of the item.
*
*
* @return The content type of the item.
*/
public final String contentType() {
return contentType;
}
/**
*
* The length of the item in bytes.
*
*
* @return The length of the item in bytes.
*/
public final Long contentLength() {
return contentLength;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(typeAsString());
hashCode = 31 * hashCode + Objects.hashCode(eTag());
hashCode = 31 * hashCode + Objects.hashCode(lastModified());
hashCode = 31 * hashCode + Objects.hashCode(contentType());
hashCode = 31 * hashCode + Objects.hashCode(contentLength());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Item)) {
return false;
}
Item other = (Item) obj;
return Objects.equals(name(), other.name()) && Objects.equals(typeAsString(), other.typeAsString())
&& Objects.equals(eTag(), other.eTag()) && Objects.equals(lastModified(), other.lastModified())
&& Objects.equals(contentType(), other.contentType()) && Objects.equals(contentLength(), other.contentLength());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Item").add("Name", name()).add("Type", typeAsString()).add("ETag", eTag())
.add("LastModified", lastModified()).add("ContentType", contentType()).add("ContentLength", contentLength())
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Name":
return Optional.ofNullable(clazz.cast(name()));
case "Type":
return Optional.ofNullable(clazz.cast(typeAsString()));
case "ETag":
return Optional.ofNullable(clazz.cast(eTag()));
case "LastModified":
return Optional.ofNullable(clazz.cast(lastModified()));
case "ContentType":
return Optional.ofNullable(clazz.cast(contentType()));
case "ContentLength":
return Optional.ofNullable(clazz.cast(contentLength()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("Name", NAME_FIELD);
map.put("Type", TYPE_FIELD);
map.put("ETag", E_TAG_FIELD);
map.put("LastModified", LAST_MODIFIED_FIELD);
map.put("ContentType", CONTENT_TYPE_FIELD);
map.put("ContentLength", CONTENT_LENGTH_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function