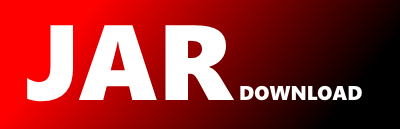
software.amazon.awssdk.services.medicalimaging.MedicalImagingClient Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.medicalimaging;
import java.nio.file.Path;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.ResponseBytes;
import software.amazon.awssdk.core.ResponseInputStream;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.sync.ResponseTransformer;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.medicalimaging.model.AccessDeniedException;
import software.amazon.awssdk.services.medicalimaging.model.ConflictException;
import software.amazon.awssdk.services.medicalimaging.model.CopyImageSetRequest;
import software.amazon.awssdk.services.medicalimaging.model.CopyImageSetResponse;
import software.amazon.awssdk.services.medicalimaging.model.CreateDatastoreRequest;
import software.amazon.awssdk.services.medicalimaging.model.CreateDatastoreResponse;
import software.amazon.awssdk.services.medicalimaging.model.DeleteDatastoreRequest;
import software.amazon.awssdk.services.medicalimaging.model.DeleteDatastoreResponse;
import software.amazon.awssdk.services.medicalimaging.model.DeleteImageSetRequest;
import software.amazon.awssdk.services.medicalimaging.model.DeleteImageSetResponse;
import software.amazon.awssdk.services.medicalimaging.model.GetDatastoreRequest;
import software.amazon.awssdk.services.medicalimaging.model.GetDatastoreResponse;
import software.amazon.awssdk.services.medicalimaging.model.GetDicomImportJobRequest;
import software.amazon.awssdk.services.medicalimaging.model.GetDicomImportJobResponse;
import software.amazon.awssdk.services.medicalimaging.model.GetImageFrameRequest;
import software.amazon.awssdk.services.medicalimaging.model.GetImageFrameResponse;
import software.amazon.awssdk.services.medicalimaging.model.GetImageSetMetadataRequest;
import software.amazon.awssdk.services.medicalimaging.model.GetImageSetMetadataResponse;
import software.amazon.awssdk.services.medicalimaging.model.GetImageSetRequest;
import software.amazon.awssdk.services.medicalimaging.model.GetImageSetResponse;
import software.amazon.awssdk.services.medicalimaging.model.InternalServerException;
import software.amazon.awssdk.services.medicalimaging.model.ListDatastoresRequest;
import software.amazon.awssdk.services.medicalimaging.model.ListDatastoresResponse;
import software.amazon.awssdk.services.medicalimaging.model.ListDicomImportJobsRequest;
import software.amazon.awssdk.services.medicalimaging.model.ListDicomImportJobsResponse;
import software.amazon.awssdk.services.medicalimaging.model.ListImageSetVersionsRequest;
import software.amazon.awssdk.services.medicalimaging.model.ListImageSetVersionsResponse;
import software.amazon.awssdk.services.medicalimaging.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.medicalimaging.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.medicalimaging.model.MedicalImagingException;
import software.amazon.awssdk.services.medicalimaging.model.ResourceNotFoundException;
import software.amazon.awssdk.services.medicalimaging.model.SearchImageSetsRequest;
import software.amazon.awssdk.services.medicalimaging.model.SearchImageSetsResponse;
import software.amazon.awssdk.services.medicalimaging.model.ServiceQuotaExceededException;
import software.amazon.awssdk.services.medicalimaging.model.StartDicomImportJobRequest;
import software.amazon.awssdk.services.medicalimaging.model.StartDicomImportJobResponse;
import software.amazon.awssdk.services.medicalimaging.model.TagResourceRequest;
import software.amazon.awssdk.services.medicalimaging.model.TagResourceResponse;
import software.amazon.awssdk.services.medicalimaging.model.ThrottlingException;
import software.amazon.awssdk.services.medicalimaging.model.UntagResourceRequest;
import software.amazon.awssdk.services.medicalimaging.model.UntagResourceResponse;
import software.amazon.awssdk.services.medicalimaging.model.UpdateImageSetMetadataRequest;
import software.amazon.awssdk.services.medicalimaging.model.UpdateImageSetMetadataResponse;
import software.amazon.awssdk.services.medicalimaging.model.ValidationException;
import software.amazon.awssdk.services.medicalimaging.paginators.ListDICOMImportJobsIterable;
import software.amazon.awssdk.services.medicalimaging.paginators.ListDatastoresIterable;
import software.amazon.awssdk.services.medicalimaging.paginators.ListImageSetVersionsIterable;
import software.amazon.awssdk.services.medicalimaging.paginators.SearchImageSetsIterable;
/**
* Service client for accessing AWS Health Imaging. This can be created using the static {@link #builder()} method.
*
*
* This is the AWS HealthImaging API Reference. AWS HealthImaging is an AWS service for storing, accessing, and
* analyzing medical images. For an introduction to the service, see the AWS HealthImaging Developer Guide .
*
*
*
* We recommend using one of the AWS Software Development Kits (SDKs) for your programming language, as they take care
* of request authentication, serialization, and connection management. For more information, see Tools to build on AWS.
*
*
* For information about using AWS HealthImaging API actions in one of the language-specific AWS SDKs, refer to the
* See Also link at the end of each section that describes an API action or data type.
*
*
*
* The following sections list AWS HealthImaging API actions categorized according to functionality. Links are provided
* to actions within this Reference, along with links back to corresponding sections in the AWS HealthImaging
* Developer Guide so you can view console procedures and CLI/SDK code examples.
*
*
* Data store actions
*
*
* -
*
* CreateDatastore –
* See Creating a data
* store.
*
*
* -
*
* GetDatastore –
* See Getting data store
* properties.
*
*
* -
*
* ListDatastores
* – See Listing data
* stores.
*
*
* -
*
* DeleteDatastore –
* See Deleting a data
* store.
*
*
*
*
* Import job actions
*
*
* -
*
*
* StartDICOMImportJob – See Starting an import
* job.
*
*
* -
*
* GetDICOMImportJob
* – See Getting
* import job properties.
*
*
* -
*
*
* ListDICOMImportJobs – See Listing import
* jobs.
*
*
*
*
* Image set access actions
*
*
* -
*
* SearchImageSets –
* See Searching image
* sets.
*
*
* -
*
* GetImageSet – See
* Getting image set
* properties.
*
*
* -
*
*
* GetImageSetMetadata – See Getting image set
* metadata.
*
*
* -
*
* GetImageFrame –
* See Getting image set
* pixel data.
*
*
*
*
* Image set modification actions
*
*
* -
*
*
* ListImageSetVersions – See Listing image set
* versions.
*
*
* -
*
*
* UpdateImageSetMetadata – See Updating image set
* metadata.
*
*
* -
*
* CopyImageSet –
* See Copying an image
* set.
*
*
* -
*
* DeleteImageSet
* – See Deleting an image
* set.
*
*
*
*
* Tagging actions
*
*
* -
*
* TagResource – See
* Tagging a data
* store and Tagging an image
* set.
*
*
* -
*
*
* ListTagsForResource – See Tagging a data
* store and Tagging an image
* set.
*
*
* -
*
* UntagResource –
* See Tagging a
* data store and Tagging an image
* set.
*
*
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface MedicalImagingClient extends AwsClient {
String SERVICE_NAME = "medical-imaging";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "medical-imaging";
/**
*
* Copy an image set.
*
*
* @param copyImageSetRequest
* @return Result of the CopyImageSet operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws ServiceQuotaExceededException
* The request caused a service quota to be exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.CopyImageSet
* @see AWS
* API Documentation
*/
default CopyImageSetResponse copyImageSet(CopyImageSetRequest copyImageSetRequest) throws ThrottlingException,
ConflictException, AccessDeniedException, ValidationException, InternalServerException, ResourceNotFoundException,
ServiceQuotaExceededException, AwsServiceException, SdkClientException, MedicalImagingException {
throw new UnsupportedOperationException();
}
/**
*
* Copy an image set.
*
*
*
* This is a convenience which creates an instance of the {@link CopyImageSetRequest.Builder} avoiding the need to
* create one manually via {@link CopyImageSetRequest#builder()}
*
*
* @param copyImageSetRequest
* A {@link Consumer} that will call methods on {@link CopyImageSetRequest.Builder} to create a request.
* @return Result of the CopyImageSet operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws ServiceQuotaExceededException
* The request caused a service quota to be exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.CopyImageSet
* @see AWS
* API Documentation
*/
default CopyImageSetResponse copyImageSet(Consumer copyImageSetRequest)
throws ThrottlingException, ConflictException, AccessDeniedException, ValidationException, InternalServerException,
ResourceNotFoundException, ServiceQuotaExceededException, AwsServiceException, SdkClientException,
MedicalImagingException {
return copyImageSet(CopyImageSetRequest.builder().applyMutation(copyImageSetRequest).build());
}
/**
*
* Create a data store.
*
*
* @param createDatastoreRequest
* @return Result of the CreateDatastore operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ServiceQuotaExceededException
* The request caused a service quota to be exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.CreateDatastore
* @see AWS API Documentation
*/
default CreateDatastoreResponse createDatastore(CreateDatastoreRequest createDatastoreRequest) throws ThrottlingException,
ConflictException, AccessDeniedException, ValidationException, InternalServerException,
ServiceQuotaExceededException, AwsServiceException, SdkClientException, MedicalImagingException {
throw new UnsupportedOperationException();
}
/**
*
* Create a data store.
*
*
*
* This is a convenience which creates an instance of the {@link CreateDatastoreRequest.Builder} avoiding the need
* to create one manually via {@link CreateDatastoreRequest#builder()}
*
*
* @param createDatastoreRequest
* A {@link Consumer} that will call methods on {@link CreateDatastoreRequest.Builder} to create a request.
* @return Result of the CreateDatastore operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ServiceQuotaExceededException
* The request caused a service quota to be exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.CreateDatastore
* @see AWS API Documentation
*/
default CreateDatastoreResponse createDatastore(Consumer createDatastoreRequest)
throws ThrottlingException, ConflictException, AccessDeniedException, ValidationException, InternalServerException,
ServiceQuotaExceededException, AwsServiceException, SdkClientException, MedicalImagingException {
return createDatastore(CreateDatastoreRequest.builder().applyMutation(createDatastoreRequest).build());
}
/**
*
* Delete a data store.
*
*
*
* Before a data store can be deleted, you must first delete all image sets within it.
*
*
*
* @param deleteDatastoreRequest
* @return Result of the DeleteDatastore operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.DeleteDatastore
* @see AWS API Documentation
*/
default DeleteDatastoreResponse deleteDatastore(DeleteDatastoreRequest deleteDatastoreRequest) throws ThrottlingException,
ConflictException, AccessDeniedException, ValidationException, InternalServerException, ResourceNotFoundException,
AwsServiceException, SdkClientException, MedicalImagingException {
throw new UnsupportedOperationException();
}
/**
*
* Delete a data store.
*
*
*
* Before a data store can be deleted, you must first delete all image sets within it.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteDatastoreRequest.Builder} avoiding the need
* to create one manually via {@link DeleteDatastoreRequest#builder()}
*
*
* @param deleteDatastoreRequest
* A {@link Consumer} that will call methods on {@link DeleteDatastoreRequest.Builder} to create a request.
* @return Result of the DeleteDatastore operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.DeleteDatastore
* @see AWS API Documentation
*/
default DeleteDatastoreResponse deleteDatastore(Consumer deleteDatastoreRequest)
throws ThrottlingException, ConflictException, AccessDeniedException, ValidationException, InternalServerException,
ResourceNotFoundException, AwsServiceException, SdkClientException, MedicalImagingException {
return deleteDatastore(DeleteDatastoreRequest.builder().applyMutation(deleteDatastoreRequest).build());
}
/**
*
* Delete an image set.
*
*
* @param deleteImageSetRequest
* @return Result of the DeleteImageSet operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.DeleteImageSet
* @see AWS API Documentation
*/
default DeleteImageSetResponse deleteImageSet(DeleteImageSetRequest deleteImageSetRequest) throws ThrottlingException,
ConflictException, AccessDeniedException, ValidationException, InternalServerException, ResourceNotFoundException,
AwsServiceException, SdkClientException, MedicalImagingException {
throw new UnsupportedOperationException();
}
/**
*
* Delete an image set.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteImageSetRequest.Builder} avoiding the need to
* create one manually via {@link DeleteImageSetRequest#builder()}
*
*
* @param deleteImageSetRequest
* A {@link Consumer} that will call methods on {@link DeleteImageSetRequest.Builder} to create a request.
* @return Result of the DeleteImageSet operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.DeleteImageSet
* @see AWS API Documentation
*/
default DeleteImageSetResponse deleteImageSet(Consumer deleteImageSetRequest)
throws ThrottlingException, ConflictException, AccessDeniedException, ValidationException, InternalServerException,
ResourceNotFoundException, AwsServiceException, SdkClientException, MedicalImagingException {
return deleteImageSet(DeleteImageSetRequest.builder().applyMutation(deleteImageSetRequest).build());
}
/**
*
* Get the import job properties to learn more about the job or job progress.
*
*
* @param getDicomImportJobRequest
* @return Result of the GetDICOMImportJob operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.GetDICOMImportJob
* @see AWS API Documentation
*/
default GetDicomImportJobResponse getDICOMImportJob(GetDicomImportJobRequest getDicomImportJobRequest)
throws ThrottlingException, ConflictException, AccessDeniedException, ValidationException, InternalServerException,
ResourceNotFoundException, AwsServiceException, SdkClientException, MedicalImagingException {
throw new UnsupportedOperationException();
}
/**
*
* Get the import job properties to learn more about the job or job progress.
*
*
*
* This is a convenience which creates an instance of the {@link GetDicomImportJobRequest.Builder} avoiding the need
* to create one manually via {@link GetDicomImportJobRequest#builder()}
*
*
* @param getDicomImportJobRequest
* A {@link Consumer} that will call methods on {@link GetDICOMImportJobRequest.Builder} to create a request.
* @return Result of the GetDICOMImportJob operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.GetDICOMImportJob
* @see AWS API Documentation
*/
default GetDicomImportJobResponse getDICOMImportJob(Consumer getDicomImportJobRequest)
throws ThrottlingException, ConflictException, AccessDeniedException, ValidationException, InternalServerException,
ResourceNotFoundException, AwsServiceException, SdkClientException, MedicalImagingException {
return getDICOMImportJob(GetDicomImportJobRequest.builder().applyMutation(getDicomImportJobRequest).build());
}
/**
*
* Get data store properties.
*
*
* @param getDatastoreRequest
* @return Result of the GetDatastore operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.GetDatastore
* @see AWS
* API Documentation
*/
default GetDatastoreResponse getDatastore(GetDatastoreRequest getDatastoreRequest) throws ThrottlingException,
AccessDeniedException, ValidationException, InternalServerException, ResourceNotFoundException, AwsServiceException,
SdkClientException, MedicalImagingException {
throw new UnsupportedOperationException();
}
/**
*
* Get data store properties.
*
*
*
* This is a convenience which creates an instance of the {@link GetDatastoreRequest.Builder} avoiding the need to
* create one manually via {@link GetDatastoreRequest#builder()}
*
*
* @param getDatastoreRequest
* A {@link Consumer} that will call methods on {@link GetDatastoreRequest.Builder} to create a request.
* @return Result of the GetDatastore operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.GetDatastore
* @see AWS
* API Documentation
*/
default GetDatastoreResponse getDatastore(Consumer getDatastoreRequest)
throws ThrottlingException, AccessDeniedException, ValidationException, InternalServerException,
ResourceNotFoundException, AwsServiceException, SdkClientException, MedicalImagingException {
return getDatastore(GetDatastoreRequest.builder().applyMutation(getDatastoreRequest).build());
}
/**
*
* Get an image frame (pixel data) for an image set.
*
*
* @param getImageFrameRequest
* @param responseTransformer
* Functional interface for processing the streamed response content. The unmarshalled GetImageFrameResponse
* and an InputStream to the response content are provided as parameters to the callback. The callback may
* return a transformed type which will be the return value of this method. See
* {@link software.amazon.awssdk.core.sync.ResponseTransformer} for details on implementing this interface
* and for links to pre-canned implementations for common scenarios like downloading to a file. The service
* documentation for the response content is as follows '
*
* The blob containing the aggregated image frame information.
*
* '.
* @return The transformed result of the ResponseTransformer.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.GetImageFrame
* @see AWS
* API Documentation
*/
default ReturnT getImageFrame(GetImageFrameRequest getImageFrameRequest,
ResponseTransformer responseTransformer) throws ThrottlingException,
ConflictException, AccessDeniedException, ValidationException, InternalServerException, ResourceNotFoundException,
AwsServiceException, SdkClientException, MedicalImagingException {
throw new UnsupportedOperationException();
}
/**
*
* Get an image frame (pixel data) for an image set.
*
*
*
* This is a convenience which creates an instance of the {@link GetImageFrameRequest.Builder} avoiding the need to
* create one manually via {@link GetImageFrameRequest#builder()}
*
*
* @param getImageFrameRequest
* A {@link Consumer} that will call methods on {@link GetImageFrameRequest.Builder} to create a request.
* @param responseTransformer
* Functional interface for processing the streamed response content. The unmarshalled GetImageFrameResponse
* and an InputStream to the response content are provided as parameters to the callback. The callback may
* return a transformed type which will be the return value of this method. See
* {@link software.amazon.awssdk.core.sync.ResponseTransformer} for details on implementing this interface
* and for links to pre-canned implementations for common scenarios like downloading to a file. The service
* documentation for the response content is as follows '
*
* The blob containing the aggregated image frame information.
*
* '.
* @return The transformed result of the ResponseTransformer.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.GetImageFrame
* @see AWS
* API Documentation
*/
default ReturnT getImageFrame(Consumer getImageFrameRequest,
ResponseTransformer responseTransformer) throws ThrottlingException,
ConflictException, AccessDeniedException, ValidationException, InternalServerException, ResourceNotFoundException,
AwsServiceException, SdkClientException, MedicalImagingException {
return getImageFrame(GetImageFrameRequest.builder().applyMutation(getImageFrameRequest).build(), responseTransformer);
}
/**
*
* Get an image frame (pixel data) for an image set.
*
*
* @param getImageFrameRequest
* @param destinationPath
* {@link Path} to file that response contents will be written to. The file must not exist or this method
* will throw an exception. If the file is not writable by the current user then an exception will be thrown.
* The service documentation for the response content is as follows '
*
* The blob containing the aggregated image frame information.
*
* '.
* @return The transformed result of the ResponseTransformer.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.GetImageFrame
* @see #getImageFrame(GetImageFrameRequest, ResponseTransformer)
* @see AWS
* API Documentation
*/
default GetImageFrameResponse getImageFrame(GetImageFrameRequest getImageFrameRequest, Path destinationPath)
throws ThrottlingException, ConflictException, AccessDeniedException, ValidationException, InternalServerException,
ResourceNotFoundException, AwsServiceException, SdkClientException, MedicalImagingException {
return getImageFrame(getImageFrameRequest, ResponseTransformer.toFile(destinationPath));
}
/**
*
* Get an image frame (pixel data) for an image set.
*
*
*
* This is a convenience which creates an instance of the {@link GetImageFrameRequest.Builder} avoiding the need to
* create one manually via {@link GetImageFrameRequest#builder()}
*
*
* @param getImageFrameRequest
* A {@link Consumer} that will call methods on {@link GetImageFrameRequest.Builder} to create a request.
* @param destinationPath
* {@link Path} to file that response contents will be written to. The file must not exist or this method
* will throw an exception. If the file is not writable by the current user then an exception will be thrown.
* The service documentation for the response content is as follows '
*
* The blob containing the aggregated image frame information.
*
* '.
* @return The transformed result of the ResponseTransformer.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.GetImageFrame
* @see #getImageFrame(GetImageFrameRequest, ResponseTransformer)
* @see AWS
* API Documentation
*/
default GetImageFrameResponse getImageFrame(Consumer getImageFrameRequest, Path destinationPath)
throws ThrottlingException, ConflictException, AccessDeniedException, ValidationException, InternalServerException,
ResourceNotFoundException, AwsServiceException, SdkClientException, MedicalImagingException {
return getImageFrame(GetImageFrameRequest.builder().applyMutation(getImageFrameRequest).build(), destinationPath);
}
/**
*
* Get an image frame (pixel data) for an image set.
*
*
* @param getImageFrameRequest
* @return A {@link ResponseInputStream} containing data streamed from service. Note that this is an unmanaged
* reference to the underlying HTTP connection so great care must be taken to ensure all data if fully read
* from the input stream and that it is properly closed. Failure to do so may result in sub-optimal behavior
* and exhausting connections in the connection pool. The unmarshalled response object can be obtained via
* {@link ResponseInputStream#response()}. The service documentation for the response content is as follows
* '
*
* The blob containing the aggregated image frame information.
*
* '.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.GetImageFrame
* @see #getObject(getImageFrame, ResponseTransformer)
* @see AWS
* API Documentation
*/
default ResponseInputStream getImageFrame(GetImageFrameRequest getImageFrameRequest)
throws ThrottlingException, ConflictException, AccessDeniedException, ValidationException, InternalServerException,
ResourceNotFoundException, AwsServiceException, SdkClientException, MedicalImagingException {
return getImageFrame(getImageFrameRequest, ResponseTransformer.toInputStream());
}
/**
*
* Get an image frame (pixel data) for an image set.
*
*
*
* This is a convenience which creates an instance of the {@link GetImageFrameRequest.Builder} avoiding the need to
* create one manually via {@link GetImageFrameRequest#builder()}
*
*
* @param getImageFrameRequest
* A {@link Consumer} that will call methods on {@link GetImageFrameRequest.Builder} to create a request.
* @return A {@link ResponseInputStream} containing data streamed from service. Note that this is an unmanaged
* reference to the underlying HTTP connection so great care must be taken to ensure all data if fully read
* from the input stream and that it is properly closed. Failure to do so may result in sub-optimal behavior
* and exhausting connections in the connection pool. The unmarshalled response object can be obtained via
* {@link ResponseInputStream#response()}. The service documentation for the response content is as follows
* '
*
* The blob containing the aggregated image frame information.
*
* '.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.GetImageFrame
* @see #getObject(getImageFrame, ResponseTransformer)
* @see AWS
* API Documentation
*/
default ResponseInputStream getImageFrame(Consumer getImageFrameRequest)
throws ThrottlingException, ConflictException, AccessDeniedException, ValidationException, InternalServerException,
ResourceNotFoundException, AwsServiceException, SdkClientException, MedicalImagingException {
return getImageFrame(GetImageFrameRequest.builder().applyMutation(getImageFrameRequest).build());
}
/**
*
* Get an image frame (pixel data) for an image set.
*
*
* @param getImageFrameRequest
* @return A {@link ResponseBytes} that loads the data streamed from the service into memory and exposes it in
* convenient in-memory representations like a byte buffer or string. The unmarshalled response object can
* be obtained via {@link ResponseBytes#response()}. The service documentation for the response content is
* as follows '
*
* The blob containing the aggregated image frame information.
*
* '.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.GetImageFrame
* @see #getObject(getImageFrame, ResponseTransformer)
* @see AWS
* API Documentation
*/
default ResponseBytes getImageFrameAsBytes(GetImageFrameRequest getImageFrameRequest)
throws ThrottlingException, ConflictException, AccessDeniedException, ValidationException, InternalServerException,
ResourceNotFoundException, AwsServiceException, SdkClientException, MedicalImagingException {
return getImageFrame(getImageFrameRequest, ResponseTransformer.toBytes());
}
/**
*
* Get an image frame (pixel data) for an image set.
*
*
*
* This is a convenience which creates an instance of the {@link GetImageFrameRequest.Builder} avoiding the need to
* create one manually via {@link GetImageFrameRequest#builder()}
*
*
* @param getImageFrameRequest
* A {@link Consumer} that will call methods on {@link GetImageFrameRequest.Builder} to create a request.
* @return A {@link ResponseBytes} that loads the data streamed from the service into memory and exposes it in
* convenient in-memory representations like a byte buffer or string. The unmarshalled response object can
* be obtained via {@link ResponseBytes#response()}. The service documentation for the response content is
* as follows '
*
* The blob containing the aggregated image frame information.
*
* '.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.GetImageFrame
* @see #getObject(getImageFrame, ResponseTransformer)
* @see AWS
* API Documentation
*/
default ResponseBytes getImageFrameAsBytes(Consumer getImageFrameRequest)
throws ThrottlingException, ConflictException, AccessDeniedException, ValidationException, InternalServerException,
ResourceNotFoundException, AwsServiceException, SdkClientException, MedicalImagingException {
return getImageFrameAsBytes(GetImageFrameRequest.builder().applyMutation(getImageFrameRequest).build());
}
/**
*
* Get image set properties.
*
*
* @param getImageSetRequest
* @return Result of the GetImageSet operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.GetImageSet
* @see AWS
* API Documentation
*/
default GetImageSetResponse getImageSet(GetImageSetRequest getImageSetRequest) throws ThrottlingException, ConflictException,
AccessDeniedException, ValidationException, InternalServerException, ResourceNotFoundException, AwsServiceException,
SdkClientException, MedicalImagingException {
throw new UnsupportedOperationException();
}
/**
*
* Get image set properties.
*
*
*
* This is a convenience which creates an instance of the {@link GetImageSetRequest.Builder} avoiding the need to
* create one manually via {@link GetImageSetRequest#builder()}
*
*
* @param getImageSetRequest
* A {@link Consumer} that will call methods on {@link GetImageSetRequest.Builder} to create a request.
* @return Result of the GetImageSet operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.GetImageSet
* @see AWS
* API Documentation
*/
default GetImageSetResponse getImageSet(Consumer getImageSetRequest) throws ThrottlingException,
ConflictException, AccessDeniedException, ValidationException, InternalServerException, ResourceNotFoundException,
AwsServiceException, SdkClientException, MedicalImagingException {
return getImageSet(GetImageSetRequest.builder().applyMutation(getImageSetRequest).build());
}
/**
*
* Get metadata attributes for an image set.
*
*
* @param getImageSetMetadataRequest
* @param responseTransformer
* Functional interface for processing the streamed response content. The unmarshalled
* GetImageSetMetadataResponse and an InputStream to the response content are provided as parameters to the
* callback. The callback may return a transformed type which will be the return value of this method. See
* {@link software.amazon.awssdk.core.sync.ResponseTransformer} for details on implementing this interface
* and for links to pre-canned implementations for common scenarios like downloading to a file. The service
* documentation for the response content is as follows '
*
* The blob containing the aggregated metadata information for the image set.
*
* '.
* @return The transformed result of the ResponseTransformer.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.GetImageSetMetadata
* @see AWS API Documentation
*/
default ReturnT getImageSetMetadata(GetImageSetMetadataRequest getImageSetMetadataRequest,
ResponseTransformer responseTransformer) throws ThrottlingException,
ConflictException, AccessDeniedException, ValidationException, InternalServerException, ResourceNotFoundException,
AwsServiceException, SdkClientException, MedicalImagingException {
throw new UnsupportedOperationException();
}
/**
*
* Get metadata attributes for an image set.
*
*
*
* This is a convenience which creates an instance of the {@link GetImageSetMetadataRequest.Builder} avoiding the
* need to create one manually via {@link GetImageSetMetadataRequest#builder()}
*
*
* @param getImageSetMetadataRequest
* A {@link Consumer} that will call methods on {@link GetImageSetMetadataRequest.Builder} to create a
* request.
* @param responseTransformer
* Functional interface for processing the streamed response content. The unmarshalled
* GetImageSetMetadataResponse and an InputStream to the response content are provided as parameters to the
* callback. The callback may return a transformed type which will be the return value of this method. See
* {@link software.amazon.awssdk.core.sync.ResponseTransformer} for details on implementing this interface
* and for links to pre-canned implementations for common scenarios like downloading to a file. The service
* documentation for the response content is as follows '
*
* The blob containing the aggregated metadata information for the image set.
*
* '.
* @return The transformed result of the ResponseTransformer.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.GetImageSetMetadata
* @see AWS API Documentation
*/
default ReturnT getImageSetMetadata(Consumer getImageSetMetadataRequest,
ResponseTransformer responseTransformer) throws ThrottlingException,
ConflictException, AccessDeniedException, ValidationException, InternalServerException, ResourceNotFoundException,
AwsServiceException, SdkClientException, MedicalImagingException {
return getImageSetMetadata(GetImageSetMetadataRequest.builder().applyMutation(getImageSetMetadataRequest).build(),
responseTransformer);
}
/**
*
* Get metadata attributes for an image set.
*
*
* @param getImageSetMetadataRequest
* @param destinationPath
* {@link Path} to file that response contents will be written to. The file must not exist or this method
* will throw an exception. If the file is not writable by the current user then an exception will be thrown.
* The service documentation for the response content is as follows '
*
* The blob containing the aggregated metadata information for the image set.
*
* '.
* @return The transformed result of the ResponseTransformer.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.GetImageSetMetadata
* @see #getImageSetMetadata(GetImageSetMetadataRequest, ResponseTransformer)
* @see AWS API Documentation
*/
default GetImageSetMetadataResponse getImageSetMetadata(GetImageSetMetadataRequest getImageSetMetadataRequest,
Path destinationPath) throws ThrottlingException, ConflictException, AccessDeniedException, ValidationException,
InternalServerException, ResourceNotFoundException, AwsServiceException, SdkClientException, MedicalImagingException {
return getImageSetMetadata(getImageSetMetadataRequest, ResponseTransformer.toFile(destinationPath));
}
/**
*
* Get metadata attributes for an image set.
*
*
*
* This is a convenience which creates an instance of the {@link GetImageSetMetadataRequest.Builder} avoiding the
* need to create one manually via {@link GetImageSetMetadataRequest#builder()}
*
*
* @param getImageSetMetadataRequest
* A {@link Consumer} that will call methods on {@link GetImageSetMetadataRequest.Builder} to create a
* request.
* @param destinationPath
* {@link Path} to file that response contents will be written to. The file must not exist or this method
* will throw an exception. If the file is not writable by the current user then an exception will be thrown.
* The service documentation for the response content is as follows '
*
* The blob containing the aggregated metadata information for the image set.
*
* '.
* @return The transformed result of the ResponseTransformer.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.GetImageSetMetadata
* @see #getImageSetMetadata(GetImageSetMetadataRequest, ResponseTransformer)
* @see AWS API Documentation
*/
default GetImageSetMetadataResponse getImageSetMetadata(
Consumer getImageSetMetadataRequest, Path destinationPath)
throws ThrottlingException, ConflictException, AccessDeniedException, ValidationException, InternalServerException,
ResourceNotFoundException, AwsServiceException, SdkClientException, MedicalImagingException {
return getImageSetMetadata(GetImageSetMetadataRequest.builder().applyMutation(getImageSetMetadataRequest).build(),
destinationPath);
}
/**
*
* Get metadata attributes for an image set.
*
*
* @param getImageSetMetadataRequest
* @return A {@link ResponseInputStream} containing data streamed from service. Note that this is an unmanaged
* reference to the underlying HTTP connection so great care must be taken to ensure all data if fully read
* from the input stream and that it is properly closed. Failure to do so may result in sub-optimal behavior
* and exhausting connections in the connection pool. The unmarshalled response object can be obtained via
* {@link ResponseInputStream#response()}. The service documentation for the response content is as follows
* '
*
* The blob containing the aggregated metadata information for the image set.
*
* '.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.GetImageSetMetadata
* @see #getObject(getImageSetMetadata, ResponseTransformer)
* @see AWS API Documentation
*/
default ResponseInputStream getImageSetMetadata(
GetImageSetMetadataRequest getImageSetMetadataRequest) throws ThrottlingException, ConflictException,
AccessDeniedException, ValidationException, InternalServerException, ResourceNotFoundException, AwsServiceException,
SdkClientException, MedicalImagingException {
return getImageSetMetadata(getImageSetMetadataRequest, ResponseTransformer.toInputStream());
}
/**
*
* Get metadata attributes for an image set.
*
*
*
* This is a convenience which creates an instance of the {@link GetImageSetMetadataRequest.Builder} avoiding the
* need to create one manually via {@link GetImageSetMetadataRequest#builder()}
*
*
* @param getImageSetMetadataRequest
* A {@link Consumer} that will call methods on {@link GetImageSetMetadataRequest.Builder} to create a
* request.
* @return A {@link ResponseInputStream} containing data streamed from service. Note that this is an unmanaged
* reference to the underlying HTTP connection so great care must be taken to ensure all data if fully read
* from the input stream and that it is properly closed. Failure to do so may result in sub-optimal behavior
* and exhausting connections in the connection pool. The unmarshalled response object can be obtained via
* {@link ResponseInputStream#response()}. The service documentation for the response content is as follows
* '
*
* The blob containing the aggregated metadata information for the image set.
*
* '.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.GetImageSetMetadata
* @see #getObject(getImageSetMetadata, ResponseTransformer)
* @see AWS API Documentation
*/
default ResponseInputStream getImageSetMetadata(
Consumer getImageSetMetadataRequest) throws ThrottlingException,
ConflictException, AccessDeniedException, ValidationException, InternalServerException, ResourceNotFoundException,
AwsServiceException, SdkClientException, MedicalImagingException {
return getImageSetMetadata(GetImageSetMetadataRequest.builder().applyMutation(getImageSetMetadataRequest).build());
}
/**
*
* Get metadata attributes for an image set.
*
*
* @param getImageSetMetadataRequest
* @return A {@link ResponseBytes} that loads the data streamed from the service into memory and exposes it in
* convenient in-memory representations like a byte buffer or string. The unmarshalled response object can
* be obtained via {@link ResponseBytes#response()}. The service documentation for the response content is
* as follows '
*
* The blob containing the aggregated metadata information for the image set.
*
* '.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.GetImageSetMetadata
* @see #getObject(getImageSetMetadata, ResponseTransformer)
* @see AWS API Documentation
*/
default ResponseBytes getImageSetMetadataAsBytes(
GetImageSetMetadataRequest getImageSetMetadataRequest) throws ThrottlingException, ConflictException,
AccessDeniedException, ValidationException, InternalServerException, ResourceNotFoundException, AwsServiceException,
SdkClientException, MedicalImagingException {
return getImageSetMetadata(getImageSetMetadataRequest, ResponseTransformer.toBytes());
}
/**
*
* Get metadata attributes for an image set.
*
*
*
* This is a convenience which creates an instance of the {@link GetImageSetMetadataRequest.Builder} avoiding the
* need to create one manually via {@link GetImageSetMetadataRequest#builder()}
*
*
* @param getImageSetMetadataRequest
* A {@link Consumer} that will call methods on {@link GetImageSetMetadataRequest.Builder} to create a
* request.
* @return A {@link ResponseBytes} that loads the data streamed from the service into memory and exposes it in
* convenient in-memory representations like a byte buffer or string. The unmarshalled response object can
* be obtained via {@link ResponseBytes#response()}. The service documentation for the response content is
* as follows '
*
* The blob containing the aggregated metadata information for the image set.
*
* '.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.GetImageSetMetadata
* @see #getObject(getImageSetMetadata, ResponseTransformer)
* @see AWS API Documentation
*/
default ResponseBytes getImageSetMetadataAsBytes(
Consumer getImageSetMetadataRequest) throws ThrottlingException,
ConflictException, AccessDeniedException, ValidationException, InternalServerException, ResourceNotFoundException,
AwsServiceException, SdkClientException, MedicalImagingException {
return getImageSetMetadataAsBytes(GetImageSetMetadataRequest.builder().applyMutation(getImageSetMetadataRequest).build());
}
/**
*
* List import jobs created by this AWS account for a specific data store.
*
*
* @param listDicomImportJobsRequest
* @return Result of the ListDICOMImportJobs operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.ListDICOMImportJobs
* @see AWS API Documentation
*/
default ListDicomImportJobsResponse listDICOMImportJobs(ListDicomImportJobsRequest listDicomImportJobsRequest)
throws ThrottlingException, ConflictException, AccessDeniedException, ValidationException, InternalServerException,
ResourceNotFoundException, AwsServiceException, SdkClientException, MedicalImagingException {
throw new UnsupportedOperationException();
}
/**
*
* List import jobs created by this AWS account for a specific data store.
*
*
*
* This is a convenience which creates an instance of the {@link ListDicomImportJobsRequest.Builder} avoiding the
* need to create one manually via {@link ListDicomImportJobsRequest#builder()}
*
*
* @param listDicomImportJobsRequest
* A {@link Consumer} that will call methods on {@link ListDICOMImportJobsRequest.Builder} to create a
* request.
* @return Result of the ListDICOMImportJobs operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.ListDICOMImportJobs
* @see AWS API Documentation
*/
default ListDicomImportJobsResponse listDICOMImportJobs(
Consumer listDicomImportJobsRequest) throws ThrottlingException,
ConflictException, AccessDeniedException, ValidationException, InternalServerException, ResourceNotFoundException,
AwsServiceException, SdkClientException, MedicalImagingException {
return listDICOMImportJobs(ListDicomImportJobsRequest.builder().applyMutation(listDicomImportJobsRequest).build());
}
/**
*
* List import jobs created by this AWS account for a specific data store.
*
*
*
* This is a variant of
* {@link #listDICOMImportJobs(software.amazon.awssdk.services.medicalimaging.model.ListDicomImportJobsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.medicalimaging.paginators.ListDICOMImportJobsIterable responses = client.listDICOMImportJobsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.medicalimaging.paginators.ListDICOMImportJobsIterable responses = client
* .listDICOMImportJobsPaginator(request);
* for (software.amazon.awssdk.services.medicalimaging.model.ListDicomImportJobsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.medicalimaging.paginators.ListDICOMImportJobsIterable responses = client.listDICOMImportJobsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDICOMImportJobs(software.amazon.awssdk.services.medicalimaging.model.ListDicomImportJobsRequest)}
* operation.
*
*
* @param listDicomImportJobsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.ListDICOMImportJobs
* @see AWS API Documentation
*/
default ListDICOMImportJobsIterable listDICOMImportJobsPaginator(ListDicomImportJobsRequest listDicomImportJobsRequest)
throws ThrottlingException, ConflictException, AccessDeniedException, ValidationException, InternalServerException,
ResourceNotFoundException, AwsServiceException, SdkClientException, MedicalImagingException {
return new ListDICOMImportJobsIterable(this, listDicomImportJobsRequest);
}
/**
*
* List import jobs created by this AWS account for a specific data store.
*
*
*
* This is a variant of
* {@link #listDICOMImportJobs(software.amazon.awssdk.services.medicalimaging.model.ListDicomImportJobsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.medicalimaging.paginators.ListDICOMImportJobsIterable responses = client.listDICOMImportJobsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.medicalimaging.paginators.ListDICOMImportJobsIterable responses = client
* .listDICOMImportJobsPaginator(request);
* for (software.amazon.awssdk.services.medicalimaging.model.ListDicomImportJobsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.medicalimaging.paginators.ListDICOMImportJobsIterable responses = client.listDICOMImportJobsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDICOMImportJobs(software.amazon.awssdk.services.medicalimaging.model.ListDicomImportJobsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListDicomImportJobsRequest.Builder} avoiding the
* need to create one manually via {@link ListDicomImportJobsRequest#builder()}
*
*
* @param listDicomImportJobsRequest
* A {@link Consumer} that will call methods on {@link ListDICOMImportJobsRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.ListDICOMImportJobs
* @see AWS API Documentation
*/
default ListDICOMImportJobsIterable listDICOMImportJobsPaginator(
Consumer listDicomImportJobsRequest) throws ThrottlingException,
ConflictException, AccessDeniedException, ValidationException, InternalServerException, ResourceNotFoundException,
AwsServiceException, SdkClientException, MedicalImagingException {
return listDICOMImportJobsPaginator(ListDicomImportJobsRequest.builder().applyMutation(listDicomImportJobsRequest)
.build());
}
/**
*
* List data stores created by this AWS account.
*
*
* @param listDatastoresRequest
* @return Result of the ListDatastores operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.ListDatastores
* @see AWS API Documentation
*/
default ListDatastoresResponse listDatastores(ListDatastoresRequest listDatastoresRequest) throws ThrottlingException,
AccessDeniedException, ValidationException, InternalServerException, AwsServiceException, SdkClientException,
MedicalImagingException {
throw new UnsupportedOperationException();
}
/**
*
* List data stores created by this AWS account.
*
*
*
* This is a convenience which creates an instance of the {@link ListDatastoresRequest.Builder} avoiding the need to
* create one manually via {@link ListDatastoresRequest#builder()}
*
*
* @param listDatastoresRequest
* A {@link Consumer} that will call methods on {@link ListDatastoresRequest.Builder} to create a request.
* @return Result of the ListDatastores operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.ListDatastores
* @see AWS API Documentation
*/
default ListDatastoresResponse listDatastores(Consumer listDatastoresRequest)
throws ThrottlingException, AccessDeniedException, ValidationException, InternalServerException, AwsServiceException,
SdkClientException, MedicalImagingException {
return listDatastores(ListDatastoresRequest.builder().applyMutation(listDatastoresRequest).build());
}
/**
*
* List data stores created by this AWS account.
*
*
*
* This is a variant of
* {@link #listDatastores(software.amazon.awssdk.services.medicalimaging.model.ListDatastoresRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.medicalimaging.paginators.ListDatastoresIterable responses = client.listDatastoresPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.medicalimaging.paginators.ListDatastoresIterable responses = client
* .listDatastoresPaginator(request);
* for (software.amazon.awssdk.services.medicalimaging.model.ListDatastoresResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.medicalimaging.paginators.ListDatastoresIterable responses = client.listDatastoresPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDatastores(software.amazon.awssdk.services.medicalimaging.model.ListDatastoresRequest)}
* operation.
*
*
* @param listDatastoresRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.ListDatastores
* @see AWS API Documentation
*/
default ListDatastoresIterable listDatastoresPaginator(ListDatastoresRequest listDatastoresRequest)
throws ThrottlingException, AccessDeniedException, ValidationException, InternalServerException, AwsServiceException,
SdkClientException, MedicalImagingException {
return new ListDatastoresIterable(this, listDatastoresRequest);
}
/**
*
* List data stores created by this AWS account.
*
*
*
* This is a variant of
* {@link #listDatastores(software.amazon.awssdk.services.medicalimaging.model.ListDatastoresRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.medicalimaging.paginators.ListDatastoresIterable responses = client.listDatastoresPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.medicalimaging.paginators.ListDatastoresIterable responses = client
* .listDatastoresPaginator(request);
* for (software.amazon.awssdk.services.medicalimaging.model.ListDatastoresResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.medicalimaging.paginators.ListDatastoresIterable responses = client.listDatastoresPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDatastores(software.amazon.awssdk.services.medicalimaging.model.ListDatastoresRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListDatastoresRequest.Builder} avoiding the need to
* create one manually via {@link ListDatastoresRequest#builder()}
*
*
* @param listDatastoresRequest
* A {@link Consumer} that will call methods on {@link ListDatastoresRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.ListDatastores
* @see AWS API Documentation
*/
default ListDatastoresIterable listDatastoresPaginator(Consumer listDatastoresRequest)
throws ThrottlingException, AccessDeniedException, ValidationException, InternalServerException, AwsServiceException,
SdkClientException, MedicalImagingException {
return listDatastoresPaginator(ListDatastoresRequest.builder().applyMutation(listDatastoresRequest).build());
}
/**
*
* List image set versions.
*
*
* @param listImageSetVersionsRequest
* @return Result of the ListImageSetVersions operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.ListImageSetVersions
* @see AWS API Documentation
*/
default ListImageSetVersionsResponse listImageSetVersions(ListImageSetVersionsRequest listImageSetVersionsRequest)
throws ThrottlingException, ConflictException, AccessDeniedException, ValidationException, InternalServerException,
ResourceNotFoundException, AwsServiceException, SdkClientException, MedicalImagingException {
throw new UnsupportedOperationException();
}
/**
*
* List image set versions.
*
*
*
* This is a convenience which creates an instance of the {@link ListImageSetVersionsRequest.Builder} avoiding the
* need to create one manually via {@link ListImageSetVersionsRequest#builder()}
*
*
* @param listImageSetVersionsRequest
* A {@link Consumer} that will call methods on {@link ListImageSetVersionsRequest.Builder} to create a
* request.
* @return Result of the ListImageSetVersions operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.ListImageSetVersions
* @see AWS API Documentation
*/
default ListImageSetVersionsResponse listImageSetVersions(
Consumer listImageSetVersionsRequest) throws ThrottlingException,
ConflictException, AccessDeniedException, ValidationException, InternalServerException, ResourceNotFoundException,
AwsServiceException, SdkClientException, MedicalImagingException {
return listImageSetVersions(ListImageSetVersionsRequest.builder().applyMutation(listImageSetVersionsRequest).build());
}
/**
*
* List image set versions.
*
*
*
* This is a variant of
* {@link #listImageSetVersions(software.amazon.awssdk.services.medicalimaging.model.ListImageSetVersionsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.medicalimaging.paginators.ListImageSetVersionsIterable responses = client.listImageSetVersionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.medicalimaging.paginators.ListImageSetVersionsIterable responses = client
* .listImageSetVersionsPaginator(request);
* for (software.amazon.awssdk.services.medicalimaging.model.ListImageSetVersionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.medicalimaging.paginators.ListImageSetVersionsIterable responses = client.listImageSetVersionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listImageSetVersions(software.amazon.awssdk.services.medicalimaging.model.ListImageSetVersionsRequest)}
* operation.
*
*
* @param listImageSetVersionsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.ListImageSetVersions
* @see AWS API Documentation
*/
default ListImageSetVersionsIterable listImageSetVersionsPaginator(ListImageSetVersionsRequest listImageSetVersionsRequest)
throws ThrottlingException, ConflictException, AccessDeniedException, ValidationException, InternalServerException,
ResourceNotFoundException, AwsServiceException, SdkClientException, MedicalImagingException {
return new ListImageSetVersionsIterable(this, listImageSetVersionsRequest);
}
/**
*
* List image set versions.
*
*
*
* This is a variant of
* {@link #listImageSetVersions(software.amazon.awssdk.services.medicalimaging.model.ListImageSetVersionsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.medicalimaging.paginators.ListImageSetVersionsIterable responses = client.listImageSetVersionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.medicalimaging.paginators.ListImageSetVersionsIterable responses = client
* .listImageSetVersionsPaginator(request);
* for (software.amazon.awssdk.services.medicalimaging.model.ListImageSetVersionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.medicalimaging.paginators.ListImageSetVersionsIterable responses = client.listImageSetVersionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listImageSetVersions(software.amazon.awssdk.services.medicalimaging.model.ListImageSetVersionsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListImageSetVersionsRequest.Builder} avoiding the
* need to create one manually via {@link ListImageSetVersionsRequest#builder()}
*
*
* @param listImageSetVersionsRequest
* A {@link Consumer} that will call methods on {@link ListImageSetVersionsRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.ListImageSetVersions
* @see AWS API Documentation
*/
default ListImageSetVersionsIterable listImageSetVersionsPaginator(
Consumer listImageSetVersionsRequest) throws ThrottlingException,
ConflictException, AccessDeniedException, ValidationException, InternalServerException, ResourceNotFoundException,
AwsServiceException, SdkClientException, MedicalImagingException {
return listImageSetVersionsPaginator(ListImageSetVersionsRequest.builder().applyMutation(listImageSetVersionsRequest)
.build());
}
/**
*
* Lists all tags associated with a medical imaging resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.ListTagsForResource
* @see AWS API Documentation
*/
default ListTagsForResourceResponse listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest)
throws ThrottlingException, AccessDeniedException, ValidationException, InternalServerException,
ResourceNotFoundException, AwsServiceException, SdkClientException, MedicalImagingException {
throw new UnsupportedOperationException();
}
/**
*
* Lists all tags associated with a medical imaging resource.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on {@link ListTagsForResourceRequest.Builder} to create a
* request.
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.ListTagsForResource
* @see AWS API Documentation
*/
default ListTagsForResourceResponse listTagsForResource(
Consumer listTagsForResourceRequest) throws ThrottlingException,
AccessDeniedException, ValidationException, InternalServerException, ResourceNotFoundException, AwsServiceException,
SdkClientException, MedicalImagingException {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Search image sets based on defined input attributes.
*
*
* @param searchImageSetsRequest
* @return Result of the SearchImageSets operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.SearchImageSets
* @see AWS API Documentation
*/
default SearchImageSetsResponse searchImageSets(SearchImageSetsRequest searchImageSetsRequest) throws ThrottlingException,
ConflictException, AccessDeniedException, ValidationException, InternalServerException, ResourceNotFoundException,
AwsServiceException, SdkClientException, MedicalImagingException {
throw new UnsupportedOperationException();
}
/**
*
* Search image sets based on defined input attributes.
*
*
*
* This is a convenience which creates an instance of the {@link SearchImageSetsRequest.Builder} avoiding the need
* to create one manually via {@link SearchImageSetsRequest#builder()}
*
*
* @param searchImageSetsRequest
* A {@link Consumer} that will call methods on {@link SearchImageSetsRequest.Builder} to create a request.
* @return Result of the SearchImageSets operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.SearchImageSets
* @see AWS API Documentation
*/
default SearchImageSetsResponse searchImageSets(Consumer searchImageSetsRequest)
throws ThrottlingException, ConflictException, AccessDeniedException, ValidationException, InternalServerException,
ResourceNotFoundException, AwsServiceException, SdkClientException, MedicalImagingException {
return searchImageSets(SearchImageSetsRequest.builder().applyMutation(searchImageSetsRequest).build());
}
/**
*
* Search image sets based on defined input attributes.
*
*
*
* This is a variant of
* {@link #searchImageSets(software.amazon.awssdk.services.medicalimaging.model.SearchImageSetsRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.medicalimaging.paginators.SearchImageSetsIterable responses = client.searchImageSetsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.medicalimaging.paginators.SearchImageSetsIterable responses = client
* .searchImageSetsPaginator(request);
* for (software.amazon.awssdk.services.medicalimaging.model.SearchImageSetsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.medicalimaging.paginators.SearchImageSetsIterable responses = client.searchImageSetsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #searchImageSets(software.amazon.awssdk.services.medicalimaging.model.SearchImageSetsRequest)}
* operation.
*
*
* @param searchImageSetsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.SearchImageSets
* @see AWS API Documentation
*/
default SearchImageSetsIterable searchImageSetsPaginator(SearchImageSetsRequest searchImageSetsRequest)
throws ThrottlingException, ConflictException, AccessDeniedException, ValidationException, InternalServerException,
ResourceNotFoundException, AwsServiceException, SdkClientException, MedicalImagingException {
return new SearchImageSetsIterable(this, searchImageSetsRequest);
}
/**
*
* Search image sets based on defined input attributes.
*
*
*
* This is a variant of
* {@link #searchImageSets(software.amazon.awssdk.services.medicalimaging.model.SearchImageSetsRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.medicalimaging.paginators.SearchImageSetsIterable responses = client.searchImageSetsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.medicalimaging.paginators.SearchImageSetsIterable responses = client
* .searchImageSetsPaginator(request);
* for (software.amazon.awssdk.services.medicalimaging.model.SearchImageSetsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.medicalimaging.paginators.SearchImageSetsIterable responses = client.searchImageSetsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #searchImageSets(software.amazon.awssdk.services.medicalimaging.model.SearchImageSetsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link SearchImageSetsRequest.Builder} avoiding the need
* to create one manually via {@link SearchImageSetsRequest#builder()}
*
*
* @param searchImageSetsRequest
* A {@link Consumer} that will call methods on {@link SearchImageSetsRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.SearchImageSets
* @see AWS API Documentation
*/
default SearchImageSetsIterable searchImageSetsPaginator(Consumer searchImageSetsRequest)
throws ThrottlingException, ConflictException, AccessDeniedException, ValidationException, InternalServerException,
ResourceNotFoundException, AwsServiceException, SdkClientException, MedicalImagingException {
return searchImageSetsPaginator(SearchImageSetsRequest.builder().applyMutation(searchImageSetsRequest).build());
}
/**
*
* Start importing bulk data into an ACTIVE
data store. The import job imports DICOM P10 files found in
* the S3 prefix specified by the inputS3Uri
parameter. The import job stores processing results in the
* file specified by the outputS3Uri
parameter.
*
*
* @param startDicomImportJobRequest
* @return Result of the StartDICOMImportJob operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws ServiceQuotaExceededException
* The request caused a service quota to be exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.StartDICOMImportJob
* @see AWS API Documentation
*/
default StartDicomImportJobResponse startDICOMImportJob(StartDicomImportJobRequest startDicomImportJobRequest)
throws ThrottlingException, ConflictException, AccessDeniedException, ValidationException, InternalServerException,
ResourceNotFoundException, ServiceQuotaExceededException, AwsServiceException, SdkClientException,
MedicalImagingException {
throw new UnsupportedOperationException();
}
/**
*
* Start importing bulk data into an ACTIVE
data store. The import job imports DICOM P10 files found in
* the S3 prefix specified by the inputS3Uri
parameter. The import job stores processing results in the
* file specified by the outputS3Uri
parameter.
*
*
*
* This is a convenience which creates an instance of the {@link StartDicomImportJobRequest.Builder} avoiding the
* need to create one manually via {@link StartDicomImportJobRequest#builder()}
*
*
* @param startDicomImportJobRequest
* A {@link Consumer} that will call methods on {@link StartDICOMImportJobRequest.Builder} to create a
* request.
* @return Result of the StartDICOMImportJob operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws ServiceQuotaExceededException
* The request caused a service quota to be exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.StartDICOMImportJob
* @see AWS API Documentation
*/
default StartDicomImportJobResponse startDICOMImportJob(
Consumer startDicomImportJobRequest) throws ThrottlingException,
ConflictException, AccessDeniedException, ValidationException, InternalServerException, ResourceNotFoundException,
ServiceQuotaExceededException, AwsServiceException, SdkClientException, MedicalImagingException {
return startDICOMImportJob(StartDicomImportJobRequest.builder().applyMutation(startDicomImportJobRequest).build());
}
/**
*
* Adds a user-specifed key and value tag to a medical imaging resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.TagResource
* @see AWS
* API Documentation
*/
default TagResourceResponse tagResource(TagResourceRequest tagResourceRequest) throws ThrottlingException,
AccessDeniedException, ValidationException, InternalServerException, ResourceNotFoundException, AwsServiceException,
SdkClientException, MedicalImagingException {
throw new UnsupportedOperationException();
}
/**
*
* Adds a user-specifed key and value tag to a medical imaging resource.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on {@link TagResourceRequest.Builder} to create a request.
* @return Result of the TagResource operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.TagResource
* @see AWS
* API Documentation
*/
default TagResourceResponse tagResource(Consumer tagResourceRequest) throws ThrottlingException,
AccessDeniedException, ValidationException, InternalServerException, ResourceNotFoundException, AwsServiceException,
SdkClientException, MedicalImagingException {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Removes tags from a medical imaging resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.UntagResource
* @see AWS
* API Documentation
*/
default UntagResourceResponse untagResource(UntagResourceRequest untagResourceRequest) throws ThrottlingException,
AccessDeniedException, ValidationException, InternalServerException, ResourceNotFoundException, AwsServiceException,
SdkClientException, MedicalImagingException {
throw new UnsupportedOperationException();
}
/**
*
* Removes tags from a medical imaging resource.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on {@link UntagResourceRequest.Builder} to create a request.
* @return Result of the UntagResource operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.UntagResource
* @see AWS
* API Documentation
*/
default UntagResourceResponse untagResource(Consumer untagResourceRequest)
throws ThrottlingException, AccessDeniedException, ValidationException, InternalServerException,
ResourceNotFoundException, AwsServiceException, SdkClientException, MedicalImagingException {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
*
* Update image set metadata attributes.
*
*
* @param updateImageSetMetadataRequest
* @return Result of the UpdateImageSetMetadata operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws ServiceQuotaExceededException
* The request caused a service quota to be exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.UpdateImageSetMetadata
* @see AWS API Documentation
*/
default UpdateImageSetMetadataResponse updateImageSetMetadata(UpdateImageSetMetadataRequest updateImageSetMetadataRequest)
throws ThrottlingException, ConflictException, AccessDeniedException, ValidationException, InternalServerException,
ResourceNotFoundException, ServiceQuotaExceededException, AwsServiceException, SdkClientException,
MedicalImagingException {
throw new UnsupportedOperationException();
}
/**
*
* Update image set metadata attributes.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateImageSetMetadataRequest.Builder} avoiding the
* need to create one manually via {@link UpdateImageSetMetadataRequest#builder()}
*
*
* @param updateImageSetMetadataRequest
* A {@link Consumer} that will call methods on {@link UpdateImageSetMetadataRequest.Builder} to create a
* request.
* @return Result of the UpdateImageSetMetadata operation returned by the service.
* @throws ThrottlingException
* The request was denied due to throttling.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws AccessDeniedException
* The user does not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws InternalServerException
* An unexpected error occurred during processing of the request.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws ServiceQuotaExceededException
* The request caused a service quota to be exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MedicalImagingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MedicalImagingClient.UpdateImageSetMetadata
* @see AWS API Documentation
*/
default UpdateImageSetMetadataResponse updateImageSetMetadata(
Consumer updateImageSetMetadataRequest) throws ThrottlingException,
ConflictException, AccessDeniedException, ValidationException, InternalServerException, ResourceNotFoundException,
ServiceQuotaExceededException, AwsServiceException, SdkClientException, MedicalImagingException {
return updateImageSetMetadata(UpdateImageSetMetadataRequest.builder().applyMutation(updateImageSetMetadataRequest)
.build());
}
/**
* Create a {@link MedicalImagingClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static MedicalImagingClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link MedicalImagingClient}.
*/
static MedicalImagingClientBuilder builder() {
return new DefaultMedicalImagingClientBuilder();
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of(SERVICE_METADATA_ID);
}
@Override
default MedicalImagingServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
}