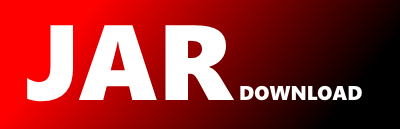
software.amazon.awssdk.services.medicalimaging.model.DICOMImportJobProperties Maven / Gradle / Ivy
Show all versions of medicalimaging Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.medicalimaging.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Properties of the import job.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DICOMImportJobProperties implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField JOB_ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("jobId")
.getter(getter(DICOMImportJobProperties::jobId)).setter(setter(Builder::jobId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("jobId").build()).build();
private static final SdkField JOB_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("jobName").getter(getter(DICOMImportJobProperties::jobName)).setter(setter(Builder::jobName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("jobName").build()).build();
private static final SdkField JOB_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("jobStatus").getter(getter(DICOMImportJobProperties::jobStatusAsString))
.setter(setter(Builder::jobStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("jobStatus").build()).build();
private static final SdkField DATASTORE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("datastoreId").getter(getter(DICOMImportJobProperties::datastoreId)).setter(setter(Builder::datastoreId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("datastoreId").build()).build();
private static final SdkField DATA_ACCESS_ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("dataAccessRoleArn").getter(getter(DICOMImportJobProperties::dataAccessRoleArn))
.setter(setter(Builder::dataAccessRoleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("dataAccessRoleArn").build()).build();
private static final SdkField ENDED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("endedAt").getter(getter(DICOMImportJobProperties::endedAt)).setter(setter(Builder::endedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("endedAt").build()).build();
private static final SdkField SUBMITTED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("submittedAt").getter(getter(DICOMImportJobProperties::submittedAt)).setter(setter(Builder::submittedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("submittedAt").build()).build();
private static final SdkField INPUT_S3_URI_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("inputS3Uri").getter(getter(DICOMImportJobProperties::inputS3Uri)).setter(setter(Builder::inputS3Uri))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("inputS3Uri").build()).build();
private static final SdkField OUTPUT_S3_URI_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("outputS3Uri").getter(getter(DICOMImportJobProperties::outputS3Uri)).setter(setter(Builder::outputS3Uri))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("outputS3Uri").build()).build();
private static final SdkField MESSAGE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("message")
.getter(getter(DICOMImportJobProperties::message)).setter(setter(Builder::message))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("message").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(JOB_ID_FIELD, JOB_NAME_FIELD,
JOB_STATUS_FIELD, DATASTORE_ID_FIELD, DATA_ACCESS_ROLE_ARN_FIELD, ENDED_AT_FIELD, SUBMITTED_AT_FIELD,
INPUT_S3_URI_FIELD, OUTPUT_S3_URI_FIELD, MESSAGE_FIELD));
private static final long serialVersionUID = 1L;
private final String jobId;
private final String jobName;
private final String jobStatus;
private final String datastoreId;
private final String dataAccessRoleArn;
private final Instant endedAt;
private final Instant submittedAt;
private final String inputS3Uri;
private final String outputS3Uri;
private final String message;
private DICOMImportJobProperties(BuilderImpl builder) {
this.jobId = builder.jobId;
this.jobName = builder.jobName;
this.jobStatus = builder.jobStatus;
this.datastoreId = builder.datastoreId;
this.dataAccessRoleArn = builder.dataAccessRoleArn;
this.endedAt = builder.endedAt;
this.submittedAt = builder.submittedAt;
this.inputS3Uri = builder.inputS3Uri;
this.outputS3Uri = builder.outputS3Uri;
this.message = builder.message;
}
/**
*
* The import job identifier.
*
*
* @return The import job identifier.
*/
public final String jobId() {
return jobId;
}
/**
*
* The import job name.
*
*
* @return The import job name.
*/
public final String jobName() {
return jobName;
}
/**
*
* The filters for listing import jobs based on status.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #jobStatus} will
* return {@link JobStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #jobStatusAsString}.
*
*
* @return The filters for listing import jobs based on status.
* @see JobStatus
*/
public final JobStatus jobStatus() {
return JobStatus.fromValue(jobStatus);
}
/**
*
* The filters for listing import jobs based on status.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #jobStatus} will
* return {@link JobStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #jobStatusAsString}.
*
*
* @return The filters for listing import jobs based on status.
* @see JobStatus
*/
public final String jobStatusAsString() {
return jobStatus;
}
/**
*
* The data store identifier.
*
*
* @return The data store identifier.
*/
public final String datastoreId() {
return datastoreId;
}
/**
*
* The Amazon Resource Name (ARN) that grants permissions to access medical imaging resources.
*
*
* @return The Amazon Resource Name (ARN) that grants permissions to access medical imaging resources.
*/
public final String dataAccessRoleArn() {
return dataAccessRoleArn;
}
/**
*
* The timestamp for when the import job was ended.
*
*
* @return The timestamp for when the import job was ended.
*/
public final Instant endedAt() {
return endedAt;
}
/**
*
* The timestamp for when the import job was submitted.
*
*
* @return The timestamp for when the import job was submitted.
*/
public final Instant submittedAt() {
return submittedAt;
}
/**
*
* The input prefix path for the S3 bucket that contains the DICOM P10 files to be imported.
*
*
* @return The input prefix path for the S3 bucket that contains the DICOM P10 files to be imported.
*/
public final String inputS3Uri() {
return inputS3Uri;
}
/**
*
* The output prefix of the S3 bucket to upload the results of the DICOM import job.
*
*
* @return The output prefix of the S3 bucket to upload the results of the DICOM import job.
*/
public final String outputS3Uri() {
return outputS3Uri;
}
/**
*
* The error message thrown if an import job fails.
*
*
* @return The error message thrown if an import job fails.
*/
public final String message() {
return message;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(jobId());
hashCode = 31 * hashCode + Objects.hashCode(jobName());
hashCode = 31 * hashCode + Objects.hashCode(jobStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(datastoreId());
hashCode = 31 * hashCode + Objects.hashCode(dataAccessRoleArn());
hashCode = 31 * hashCode + Objects.hashCode(endedAt());
hashCode = 31 * hashCode + Objects.hashCode(submittedAt());
hashCode = 31 * hashCode + Objects.hashCode(inputS3Uri());
hashCode = 31 * hashCode + Objects.hashCode(outputS3Uri());
hashCode = 31 * hashCode + Objects.hashCode(message());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DICOMImportJobProperties)) {
return false;
}
DICOMImportJobProperties other = (DICOMImportJobProperties) obj;
return Objects.equals(jobId(), other.jobId()) && Objects.equals(jobName(), other.jobName())
&& Objects.equals(jobStatusAsString(), other.jobStatusAsString())
&& Objects.equals(datastoreId(), other.datastoreId())
&& Objects.equals(dataAccessRoleArn(), other.dataAccessRoleArn()) && Objects.equals(endedAt(), other.endedAt())
&& Objects.equals(submittedAt(), other.submittedAt()) && Objects.equals(inputS3Uri(), other.inputS3Uri())
&& Objects.equals(outputS3Uri(), other.outputS3Uri()) && Objects.equals(message(), other.message());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DICOMImportJobProperties").add("JobId", jobId()).add("JobName", jobName())
.add("JobStatus", jobStatusAsString()).add("DatastoreId", datastoreId())
.add("DataAccessRoleArn", dataAccessRoleArn()).add("EndedAt", endedAt()).add("SubmittedAt", submittedAt())
.add("InputS3Uri", inputS3Uri()).add("OutputS3Uri", outputS3Uri()).add("Message", message()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "jobId":
return Optional.ofNullable(clazz.cast(jobId()));
case "jobName":
return Optional.ofNullable(clazz.cast(jobName()));
case "jobStatus":
return Optional.ofNullable(clazz.cast(jobStatusAsString()));
case "datastoreId":
return Optional.ofNullable(clazz.cast(datastoreId()));
case "dataAccessRoleArn":
return Optional.ofNullable(clazz.cast(dataAccessRoleArn()));
case "endedAt":
return Optional.ofNullable(clazz.cast(endedAt()));
case "submittedAt":
return Optional.ofNullable(clazz.cast(submittedAt()));
case "inputS3Uri":
return Optional.ofNullable(clazz.cast(inputS3Uri()));
case "outputS3Uri":
return Optional.ofNullable(clazz.cast(outputS3Uri()));
case "message":
return Optional.ofNullable(clazz.cast(message()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function