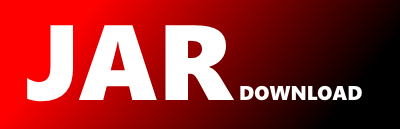
software.amazon.awssdk.services.medicalimaging.MedicalImagingAsyncClient Maven / Gradle / Ivy
Show all versions of medicalimaging Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.medicalimaging;
import java.nio.file.Path;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.core.async.AsyncResponseTransformer;
import software.amazon.awssdk.services.medicalimaging.model.CopyImageSetRequest;
import software.amazon.awssdk.services.medicalimaging.model.CopyImageSetResponse;
import software.amazon.awssdk.services.medicalimaging.model.CreateDatastoreRequest;
import software.amazon.awssdk.services.medicalimaging.model.CreateDatastoreResponse;
import software.amazon.awssdk.services.medicalimaging.model.DeleteDatastoreRequest;
import software.amazon.awssdk.services.medicalimaging.model.DeleteDatastoreResponse;
import software.amazon.awssdk.services.medicalimaging.model.DeleteImageSetRequest;
import software.amazon.awssdk.services.medicalimaging.model.DeleteImageSetResponse;
import software.amazon.awssdk.services.medicalimaging.model.GetDatastoreRequest;
import software.amazon.awssdk.services.medicalimaging.model.GetDatastoreResponse;
import software.amazon.awssdk.services.medicalimaging.model.GetDicomImportJobRequest;
import software.amazon.awssdk.services.medicalimaging.model.GetDicomImportJobResponse;
import software.amazon.awssdk.services.medicalimaging.model.GetImageFrameRequest;
import software.amazon.awssdk.services.medicalimaging.model.GetImageFrameResponse;
import software.amazon.awssdk.services.medicalimaging.model.GetImageSetMetadataRequest;
import software.amazon.awssdk.services.medicalimaging.model.GetImageSetMetadataResponse;
import software.amazon.awssdk.services.medicalimaging.model.GetImageSetRequest;
import software.amazon.awssdk.services.medicalimaging.model.GetImageSetResponse;
import software.amazon.awssdk.services.medicalimaging.model.ListDatastoresRequest;
import software.amazon.awssdk.services.medicalimaging.model.ListDatastoresResponse;
import software.amazon.awssdk.services.medicalimaging.model.ListDicomImportJobsRequest;
import software.amazon.awssdk.services.medicalimaging.model.ListDicomImportJobsResponse;
import software.amazon.awssdk.services.medicalimaging.model.ListImageSetVersionsRequest;
import software.amazon.awssdk.services.medicalimaging.model.ListImageSetVersionsResponse;
import software.amazon.awssdk.services.medicalimaging.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.medicalimaging.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.medicalimaging.model.SearchImageSetsRequest;
import software.amazon.awssdk.services.medicalimaging.model.SearchImageSetsResponse;
import software.amazon.awssdk.services.medicalimaging.model.StartDicomImportJobRequest;
import software.amazon.awssdk.services.medicalimaging.model.StartDicomImportJobResponse;
import software.amazon.awssdk.services.medicalimaging.model.TagResourceRequest;
import software.amazon.awssdk.services.medicalimaging.model.TagResourceResponse;
import software.amazon.awssdk.services.medicalimaging.model.UntagResourceRequest;
import software.amazon.awssdk.services.medicalimaging.model.UntagResourceResponse;
import software.amazon.awssdk.services.medicalimaging.model.UpdateImageSetMetadataRequest;
import software.amazon.awssdk.services.medicalimaging.model.UpdateImageSetMetadataResponse;
import software.amazon.awssdk.services.medicalimaging.paginators.ListDICOMImportJobsPublisher;
import software.amazon.awssdk.services.medicalimaging.paginators.ListDatastoresPublisher;
import software.amazon.awssdk.services.medicalimaging.paginators.ListImageSetVersionsPublisher;
import software.amazon.awssdk.services.medicalimaging.paginators.SearchImageSetsPublisher;
/**
* Service client for accessing AWS Health Imaging asynchronously. This can be created using the static
* {@link #builder()} method.The asynchronous client performs non-blocking I/O when configured with any
* {@code SdkAsyncHttpClient} supported in the SDK. However, full non-blocking is not guaranteed as the async client may
* perform blocking calls in some cases such as credentials retrieval and endpoint discovery as part of the async API
* call.
*
*
* This is the AWS HealthImaging API Reference. AWS HealthImaging is a HIPAA eligible service that empowers
* healthcare providers, life science organizations, and their software partners to store, analyze, and share medical
* images in the cloud at petabyte scale. For an introduction to the service, see the AWS HealthImaging Developer
* Guide .
*
*
*
* We recommend using one of the AWS Software Development Kits (SDKs) for your programming language, as they take care
* of request authentication, serialization, and connection management. For more information, see Tools to build on AWS.
*
*
*
* The following sections list AWS HealthImaging API actions categorized according to functionality. Links are provided
* to actions within this Reference, along with links back to corresponding sections in the AWS HealthImaging
* Developer Guide where you can view tested code examples.
*
*
* Data store actions
*
*
* -
*
* CreateDatastore
* – See Creating a data
* store.
*
*
* -
*
* GetDatastore – See
* Getting data store
* properties.
*
*
* -
*
* ListDatastores –
* See Listing data
* stores.
*
*
* -
*
* DeleteDatastore
* – See Deleting a data
* store.
*
*
*
*
* Import job actions
*
*
* -
*
*
* StartDICOMImportJob – See Starting an import
* job.
*
*
* -
*
* GetDICOMImportJob
* – See Getting import
* job properties.
*
*
* -
*
*
* ListDICOMImportJobs – See Listing import jobs.
*
*
*
*
* Image set access actions
*
*
* -
*
* SearchImageSets
* – See Searching image
* sets.
*
*
* -
*
* GetImageSet – See Getting image set
* properties.
*
*
* -
*
*
* GetImageSetMetadata – See Getting image set
* metadata.
*
*
* -
*
* GetImageFrame –
* See Getting image set pixel
* data.
*
*
*
*
* Image set modification actions
*
*
* -
*
*
* ListImageSetVersions – See Listing image set
* versions.
*
*
* -
*
*
* UpdateImageSetMetadata – See Updating image set
* metadata.
*
*
* -
*
* CopyImageSet – See
* Copying an image set.
*
*
* -
*
* DeleteImageSet –
* See Deleting an image
* set.
*
*
*
*
* Tagging actions
*
*
* -
*
* TagResource – See Tagging a resource.
*
*
* -
*
*
* ListTagsForResource – See Listing tags for a
* resource.
*
*
* -
*
* UntagResource –
* See Untagging a resource.
*
*
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface MedicalImagingAsyncClient extends AwsClient {
String SERVICE_NAME = "medical-imaging";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "medical-imaging";
/**
*
* Copy an image set.
*
*
* @param copyImageSetRequest
* @return A Java Future containing the result of the CopyImageSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - ServiceQuotaExceededException The request caused a service quota to be exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.CopyImageSet
* @see AWS
* API Documentation
*/
default CompletableFuture copyImageSet(CopyImageSetRequest copyImageSetRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Copy an image set.
*
*
*
* This is a convenience which creates an instance of the {@link CopyImageSetRequest.Builder} avoiding the need to
* create one manually via {@link CopyImageSetRequest#builder()}
*
*
* @param copyImageSetRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.medicalimaging.model.CopyImageSetRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CopyImageSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - ServiceQuotaExceededException The request caused a service quota to be exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.CopyImageSet
* @see AWS
* API Documentation
*/
default CompletableFuture copyImageSet(Consumer copyImageSetRequest) {
return copyImageSet(CopyImageSetRequest.builder().applyMutation(copyImageSetRequest).build());
}
/**
*
* Create a data store.
*
*
* @param createDatastoreRequest
* @return A Java Future containing the result of the CreateDatastore operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ServiceQuotaExceededException The request caused a service quota to be exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.CreateDatastore
* @see AWS API Documentation
*/
default CompletableFuture createDatastore(CreateDatastoreRequest createDatastoreRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Create a data store.
*
*
*
* This is a convenience which creates an instance of the {@link CreateDatastoreRequest.Builder} avoiding the need
* to create one manually via {@link CreateDatastoreRequest#builder()}
*
*
* @param createDatastoreRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.medicalimaging.model.CreateDatastoreRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateDatastore operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ServiceQuotaExceededException The request caused a service quota to be exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.CreateDatastore
* @see AWS API Documentation
*/
default CompletableFuture createDatastore(
Consumer createDatastoreRequest) {
return createDatastore(CreateDatastoreRequest.builder().applyMutation(createDatastoreRequest).build());
}
/**
*
* Delete a data store.
*
*
*
* Before a data store can be deleted, you must first delete all image sets within it.
*
*
*
* @param deleteDatastoreRequest
* @return A Java Future containing the result of the DeleteDatastore operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.DeleteDatastore
* @see AWS API Documentation
*/
default CompletableFuture deleteDatastore(DeleteDatastoreRequest deleteDatastoreRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Delete a data store.
*
*
*
* Before a data store can be deleted, you must first delete all image sets within it.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteDatastoreRequest.Builder} avoiding the need
* to create one manually via {@link DeleteDatastoreRequest#builder()}
*
*
* @param deleteDatastoreRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.medicalimaging.model.DeleteDatastoreRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteDatastore operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.DeleteDatastore
* @see AWS API Documentation
*/
default CompletableFuture deleteDatastore(
Consumer deleteDatastoreRequest) {
return deleteDatastore(DeleteDatastoreRequest.builder().applyMutation(deleteDatastoreRequest).build());
}
/**
*
* Delete an image set.
*
*
* @param deleteImageSetRequest
* @return A Java Future containing the result of the DeleteImageSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.DeleteImageSet
* @see AWS API Documentation
*/
default CompletableFuture deleteImageSet(DeleteImageSetRequest deleteImageSetRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Delete an image set.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteImageSetRequest.Builder} avoiding the need to
* create one manually via {@link DeleteImageSetRequest#builder()}
*
*
* @param deleteImageSetRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.medicalimaging.model.DeleteImageSetRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteImageSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.DeleteImageSet
* @see AWS API Documentation
*/
default CompletableFuture deleteImageSet(Consumer deleteImageSetRequest) {
return deleteImageSet(DeleteImageSetRequest.builder().applyMutation(deleteImageSetRequest).build());
}
/**
*
* Get the import job properties to learn more about the job or job progress.
*
*
*
* The jobStatus
refers to the execution of the import job. Therefore, an import job can return a
* jobStatus
as COMPLETED
even if validation issues are discovered during the import
* process. If a jobStatus
returns as COMPLETED
, we still recommend you review the output
* manifests written to S3, as they provide details on the success or failure of individual P10 object imports.
*
*
*
* @param getDicomImportJobRequest
* @return A Java Future containing the result of the GetDICOMImportJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.GetDICOMImportJob
* @see AWS API Documentation
*/
default CompletableFuture getDICOMImportJob(GetDicomImportJobRequest getDicomImportJobRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Get the import job properties to learn more about the job or job progress.
*
*
*
* The jobStatus
refers to the execution of the import job. Therefore, an import job can return a
* jobStatus
as COMPLETED
even if validation issues are discovered during the import
* process. If a jobStatus
returns as COMPLETED
, we still recommend you review the output
* manifests written to S3, as they provide details on the success or failure of individual P10 object imports.
*
*
*
* This is a convenience which creates an instance of the {@link GetDicomImportJobRequest.Builder} avoiding the need
* to create one manually via {@link GetDicomImportJobRequest#builder()}
*
*
* @param getDicomImportJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.medicalimaging.model.GetDicomImportJobRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetDICOMImportJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.GetDICOMImportJob
* @see AWS API Documentation
*/
default CompletableFuture getDICOMImportJob(
Consumer getDicomImportJobRequest) {
return getDICOMImportJob(GetDicomImportJobRequest.builder().applyMutation(getDicomImportJobRequest).build());
}
/**
*
* Get data store properties.
*
*
* @param getDatastoreRequest
* @return A Java Future containing the result of the GetDatastore operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.GetDatastore
* @see AWS
* API Documentation
*/
default CompletableFuture getDatastore(GetDatastoreRequest getDatastoreRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Get data store properties.
*
*
*
* This is a convenience which creates an instance of the {@link GetDatastoreRequest.Builder} avoiding the need to
* create one manually via {@link GetDatastoreRequest#builder()}
*
*
* @param getDatastoreRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.medicalimaging.model.GetDatastoreRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetDatastore operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.GetDatastore
* @see AWS
* API Documentation
*/
default CompletableFuture getDatastore(Consumer getDatastoreRequest) {
return getDatastore(GetDatastoreRequest.builder().applyMutation(getDatastoreRequest).build());
}
/**
*
* Get an image frame (pixel data) for an image set.
*
*
* @param getImageFrameRequest
* @param asyncResponseTransformer
* The response transformer for processing the streaming response in a non-blocking manner. See
* {@link AsyncResponseTransformer} for details on how this callback should be implemented and for links to
* precanned implementations for common scenarios like downloading to a file. The service documentation for
* the response content is as follows '
*
* The blob containing the aggregated image frame information.
*
* '.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.GetImageFrame
* @see AWS
* API Documentation
*/
default CompletableFuture getImageFrame(GetImageFrameRequest getImageFrameRequest,
AsyncResponseTransformer asyncResponseTransformer) {
throw new UnsupportedOperationException();
}
/**
*
* Get an image frame (pixel data) for an image set.
*
*
*
* This is a convenience which creates an instance of the {@link GetImageFrameRequest.Builder} avoiding the need to
* create one manually via {@link GetImageFrameRequest#builder()}
*
*
* @param getImageFrameRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.medicalimaging.model.GetImageFrameRequest.Builder} to create a
* request.
* @param asyncResponseTransformer
* The response transformer for processing the streaming response in a non-blocking manner. See
* {@link AsyncResponseTransformer} for details on how this callback should be implemented and for links to
* precanned implementations for common scenarios like downloading to a file. The service documentation for
* the response content is as follows '
*
* The blob containing the aggregated image frame information.
*
* '.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.GetImageFrame
* @see AWS
* API Documentation
*/
default CompletableFuture getImageFrame(Consumer getImageFrameRequest,
AsyncResponseTransformer asyncResponseTransformer) {
return getImageFrame(GetImageFrameRequest.builder().applyMutation(getImageFrameRequest).build(), asyncResponseTransformer);
}
/**
*
* Get an image frame (pixel data) for an image set.
*
*
* @param getImageFrameRequest
* @param destinationPath
* {@link Path} to file that response contents will be written to. The file must not exist or this method
* will throw an exception. If the file is not writable by the current user then an exception will be thrown.
* The service documentation for the response content is as follows '
*
* The blob containing the aggregated image frame information.
*
* '.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.GetImageFrame
* @see AWS
* API Documentation
*/
default CompletableFuture getImageFrame(GetImageFrameRequest getImageFrameRequest, Path destinationPath) {
return getImageFrame(getImageFrameRequest, AsyncResponseTransformer.toFile(destinationPath));
}
/**
*
* Get an image frame (pixel data) for an image set.
*
*
*
* This is a convenience which creates an instance of the {@link GetImageFrameRequest.Builder} avoiding the need to
* create one manually via {@link GetImageFrameRequest#builder()}
*
*
* @param getImageFrameRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.medicalimaging.model.GetImageFrameRequest.Builder} to create a
* request.
* @param destinationPath
* {@link Path} to file that response contents will be written to. The file must not exist or this method
* will throw an exception. If the file is not writable by the current user then an exception will be thrown.
* The service documentation for the response content is as follows '
*
* The blob containing the aggregated image frame information.
*
* '.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.GetImageFrame
* @see AWS
* API Documentation
*/
default CompletableFuture getImageFrame(Consumer getImageFrameRequest,
Path destinationPath) {
return getImageFrame(GetImageFrameRequest.builder().applyMutation(getImageFrameRequest).build(), destinationPath);
}
/**
*
* Get image set properties.
*
*
* @param getImageSetRequest
* @return A Java Future containing the result of the GetImageSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.GetImageSet
* @see AWS
* API Documentation
*/
default CompletableFuture getImageSet(GetImageSetRequest getImageSetRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Get image set properties.
*
*
*
* This is a convenience which creates an instance of the {@link GetImageSetRequest.Builder} avoiding the need to
* create one manually via {@link GetImageSetRequest#builder()}
*
*
* @param getImageSetRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.medicalimaging.model.GetImageSetRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetImageSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.GetImageSet
* @see AWS
* API Documentation
*/
default CompletableFuture getImageSet(Consumer getImageSetRequest) {
return getImageSet(GetImageSetRequest.builder().applyMutation(getImageSetRequest).build());
}
/**
*
* Get metadata attributes for an image set.
*
*
* @param getImageSetMetadataRequest
* @param asyncResponseTransformer
* The response transformer for processing the streaming response in a non-blocking manner. See
* {@link AsyncResponseTransformer} for details on how this callback should be implemented and for links to
* precanned implementations for common scenarios like downloading to a file. The service documentation for
* the response content is as follows '
*
* The blob containing the aggregated metadata information for the image set.
*
* '.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.GetImageSetMetadata
* @see AWS API Documentation
*/
default CompletableFuture getImageSetMetadata(GetImageSetMetadataRequest getImageSetMetadataRequest,
AsyncResponseTransformer asyncResponseTransformer) {
throw new UnsupportedOperationException();
}
/**
*
* Get metadata attributes for an image set.
*
*
*
* This is a convenience which creates an instance of the {@link GetImageSetMetadataRequest.Builder} avoiding the
* need to create one manually via {@link GetImageSetMetadataRequest#builder()}
*
*
* @param getImageSetMetadataRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.medicalimaging.model.GetImageSetMetadataRequest.Builder} to create
* a request.
* @param asyncResponseTransformer
* The response transformer for processing the streaming response in a non-blocking manner. See
* {@link AsyncResponseTransformer} for details on how this callback should be implemented and for links to
* precanned implementations for common scenarios like downloading to a file. The service documentation for
* the response content is as follows '
*
* The blob containing the aggregated metadata information for the image set.
*
* '.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.GetImageSetMetadata
* @see AWS API Documentation
*/
default CompletableFuture getImageSetMetadata(
Consumer getImageSetMetadataRequest,
AsyncResponseTransformer asyncResponseTransformer) {
return getImageSetMetadata(GetImageSetMetadataRequest.builder().applyMutation(getImageSetMetadataRequest).build(),
asyncResponseTransformer);
}
/**
*
* Get metadata attributes for an image set.
*
*
* @param getImageSetMetadataRequest
* @param destinationPath
* {@link Path} to file that response contents will be written to. The file must not exist or this method
* will throw an exception. If the file is not writable by the current user then an exception will be thrown.
* The service documentation for the response content is as follows '
*
* The blob containing the aggregated metadata information for the image set.
*
* '.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.GetImageSetMetadata
* @see AWS API Documentation
*/
default CompletableFuture getImageSetMetadata(
GetImageSetMetadataRequest getImageSetMetadataRequest, Path destinationPath) {
return getImageSetMetadata(getImageSetMetadataRequest, AsyncResponseTransformer.toFile(destinationPath));
}
/**
*
* Get metadata attributes for an image set.
*
*
*
* This is a convenience which creates an instance of the {@link GetImageSetMetadataRequest.Builder} avoiding the
* need to create one manually via {@link GetImageSetMetadataRequest#builder()}
*
*
* @param getImageSetMetadataRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.medicalimaging.model.GetImageSetMetadataRequest.Builder} to create
* a request.
* @param destinationPath
* {@link Path} to file that response contents will be written to. The file must not exist or this method
* will throw an exception. If the file is not writable by the current user then an exception will be thrown.
* The service documentation for the response content is as follows '
*
* The blob containing the aggregated metadata information for the image set.
*
* '.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.GetImageSetMetadata
* @see AWS API Documentation
*/
default CompletableFuture getImageSetMetadata(
Consumer getImageSetMetadataRequest, Path destinationPath) {
return getImageSetMetadata(GetImageSetMetadataRequest.builder().applyMutation(getImageSetMetadataRequest).build(),
destinationPath);
}
/**
*
* List import jobs created for a specific data store.
*
*
* @param listDicomImportJobsRequest
* @return A Java Future containing the result of the ListDICOMImportJobs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.ListDICOMImportJobs
* @see AWS API Documentation
*/
default CompletableFuture listDICOMImportJobs(
ListDicomImportJobsRequest listDicomImportJobsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* List import jobs created for a specific data store.
*
*
*
* This is a convenience which creates an instance of the {@link ListDicomImportJobsRequest.Builder} avoiding the
* need to create one manually via {@link ListDicomImportJobsRequest#builder()}
*
*
* @param listDicomImportJobsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.medicalimaging.model.ListDicomImportJobsRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the ListDICOMImportJobs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.ListDICOMImportJobs
* @see AWS API Documentation
*/
default CompletableFuture listDICOMImportJobs(
Consumer listDicomImportJobsRequest) {
return listDICOMImportJobs(ListDicomImportJobsRequest.builder().applyMutation(listDicomImportJobsRequest).build());
}
/**
*
* This is a variant of
* {@link #listDICOMImportJobs(software.amazon.awssdk.services.medicalimaging.model.ListDicomImportJobsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.medicalimaging.paginators.ListDICOMImportJobsPublisher publisher = client.listDICOMImportJobsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.medicalimaging.paginators.ListDICOMImportJobsPublisher publisher = client.listDICOMImportJobsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.medicalimaging.model.ListDicomImportJobsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDICOMImportJobs(software.amazon.awssdk.services.medicalimaging.model.ListDicomImportJobsRequest)}
* operation.
*
*
* @param listDicomImportJobsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.ListDICOMImportJobs
* @see AWS API Documentation
*/
default ListDICOMImportJobsPublisher listDICOMImportJobsPaginator(ListDicomImportJobsRequest listDicomImportJobsRequest) {
return new ListDICOMImportJobsPublisher(this, listDicomImportJobsRequest);
}
/**
*
* This is a variant of
* {@link #listDICOMImportJobs(software.amazon.awssdk.services.medicalimaging.model.ListDicomImportJobsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.medicalimaging.paginators.ListDICOMImportJobsPublisher publisher = client.listDICOMImportJobsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.medicalimaging.paginators.ListDICOMImportJobsPublisher publisher = client.listDICOMImportJobsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.medicalimaging.model.ListDicomImportJobsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDICOMImportJobs(software.amazon.awssdk.services.medicalimaging.model.ListDicomImportJobsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListDicomImportJobsRequest.Builder} avoiding the
* need to create one manually via {@link ListDicomImportJobsRequest#builder()}
*
*
* @param listDicomImportJobsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.medicalimaging.model.ListDicomImportJobsRequest.Builder} to create
* a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.ListDICOMImportJobs
* @see AWS API Documentation
*/
default ListDICOMImportJobsPublisher listDICOMImportJobsPaginator(
Consumer listDicomImportJobsRequest) {
return listDICOMImportJobsPaginator(ListDicomImportJobsRequest.builder().applyMutation(listDicomImportJobsRequest)
.build());
}
/**
*
* List data stores.
*
*
* @param listDatastoresRequest
* @return A Java Future containing the result of the ListDatastores operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.ListDatastores
* @see AWS API Documentation
*/
default CompletableFuture listDatastores(ListDatastoresRequest listDatastoresRequest) {
throw new UnsupportedOperationException();
}
/**
*
* List data stores.
*
*
*
* This is a convenience which creates an instance of the {@link ListDatastoresRequest.Builder} avoiding the need to
* create one manually via {@link ListDatastoresRequest#builder()}
*
*
* @param listDatastoresRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.medicalimaging.model.ListDatastoresRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListDatastores operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.ListDatastores
* @see AWS API Documentation
*/
default CompletableFuture listDatastores(Consumer listDatastoresRequest) {
return listDatastores(ListDatastoresRequest.builder().applyMutation(listDatastoresRequest).build());
}
/**
*
* This is a variant of
* {@link #listDatastores(software.amazon.awssdk.services.medicalimaging.model.ListDatastoresRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.medicalimaging.paginators.ListDatastoresPublisher publisher = client.listDatastoresPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.medicalimaging.paginators.ListDatastoresPublisher publisher = client.listDatastoresPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.medicalimaging.model.ListDatastoresResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDatastores(software.amazon.awssdk.services.medicalimaging.model.ListDatastoresRequest)}
* operation.
*
*
* @param listDatastoresRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.ListDatastores
* @see AWS API Documentation
*/
default ListDatastoresPublisher listDatastoresPaginator(ListDatastoresRequest listDatastoresRequest) {
return new ListDatastoresPublisher(this, listDatastoresRequest);
}
/**
*
* This is a variant of
* {@link #listDatastores(software.amazon.awssdk.services.medicalimaging.model.ListDatastoresRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.medicalimaging.paginators.ListDatastoresPublisher publisher = client.listDatastoresPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.medicalimaging.paginators.ListDatastoresPublisher publisher = client.listDatastoresPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.medicalimaging.model.ListDatastoresResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDatastores(software.amazon.awssdk.services.medicalimaging.model.ListDatastoresRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListDatastoresRequest.Builder} avoiding the need to
* create one manually via {@link ListDatastoresRequest#builder()}
*
*
* @param listDatastoresRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.medicalimaging.model.ListDatastoresRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.ListDatastores
* @see AWS API Documentation
*/
default ListDatastoresPublisher listDatastoresPaginator(Consumer listDatastoresRequest) {
return listDatastoresPaginator(ListDatastoresRequest.builder().applyMutation(listDatastoresRequest).build());
}
/**
*
* List image set versions.
*
*
* @param listImageSetVersionsRequest
* @return A Java Future containing the result of the ListImageSetVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.ListImageSetVersions
* @see AWS API Documentation
*/
default CompletableFuture listImageSetVersions(
ListImageSetVersionsRequest listImageSetVersionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* List image set versions.
*
*
*
* This is a convenience which creates an instance of the {@link ListImageSetVersionsRequest.Builder} avoiding the
* need to create one manually via {@link ListImageSetVersionsRequest#builder()}
*
*
* @param listImageSetVersionsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.medicalimaging.model.ListImageSetVersionsRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the ListImageSetVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.ListImageSetVersions
* @see AWS API Documentation
*/
default CompletableFuture listImageSetVersions(
Consumer listImageSetVersionsRequest) {
return listImageSetVersions(ListImageSetVersionsRequest.builder().applyMutation(listImageSetVersionsRequest).build());
}
/**
*
* This is a variant of
* {@link #listImageSetVersions(software.amazon.awssdk.services.medicalimaging.model.ListImageSetVersionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.medicalimaging.paginators.ListImageSetVersionsPublisher publisher = client.listImageSetVersionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.medicalimaging.paginators.ListImageSetVersionsPublisher publisher = client.listImageSetVersionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.medicalimaging.model.ListImageSetVersionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listImageSetVersions(software.amazon.awssdk.services.medicalimaging.model.ListImageSetVersionsRequest)}
* operation.
*
*
* @param listImageSetVersionsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.ListImageSetVersions
* @see AWS API Documentation
*/
default ListImageSetVersionsPublisher listImageSetVersionsPaginator(ListImageSetVersionsRequest listImageSetVersionsRequest) {
return new ListImageSetVersionsPublisher(this, listImageSetVersionsRequest);
}
/**
*
* This is a variant of
* {@link #listImageSetVersions(software.amazon.awssdk.services.medicalimaging.model.ListImageSetVersionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.medicalimaging.paginators.ListImageSetVersionsPublisher publisher = client.listImageSetVersionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.medicalimaging.paginators.ListImageSetVersionsPublisher publisher = client.listImageSetVersionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.medicalimaging.model.ListImageSetVersionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listImageSetVersions(software.amazon.awssdk.services.medicalimaging.model.ListImageSetVersionsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListImageSetVersionsRequest.Builder} avoiding the
* need to create one manually via {@link ListImageSetVersionsRequest#builder()}
*
*
* @param listImageSetVersionsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.medicalimaging.model.ListImageSetVersionsRequest.Builder} to create
* a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.ListImageSetVersions
* @see AWS API Documentation
*/
default ListImageSetVersionsPublisher listImageSetVersionsPaginator(
Consumer listImageSetVersionsRequest) {
return listImageSetVersionsPaginator(ListImageSetVersionsRequest.builder().applyMutation(listImageSetVersionsRequest)
.build());
}
/**
*
* Lists all tags associated with a medical imaging resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
default CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all tags associated with a medical imaging resource.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.medicalimaging.model.ListTagsForResourceRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
default CompletableFuture listTagsForResource(
Consumer listTagsForResourceRequest) {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Search image sets based on defined input attributes.
*
*
*
* SearchImageSets
accepts a single search query parameter and returns a paginated response of all
* image sets that have the matching criteria. All date range queries must be input as
* (lowerBound, upperBound)
.
*
*
* By default, SearchImageSets
uses the updatedAt
field for sorting in descending order
* from newest to oldest.
*
*
*
* @param searchImageSetsRequest
* @return A Java Future containing the result of the SearchImageSets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.SearchImageSets
* @see AWS API Documentation
*/
default CompletableFuture searchImageSets(SearchImageSetsRequest searchImageSetsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Search image sets based on defined input attributes.
*
*
*
* SearchImageSets
accepts a single search query parameter and returns a paginated response of all
* image sets that have the matching criteria. All date range queries must be input as
* (lowerBound, upperBound)
.
*
*
* By default, SearchImageSets
uses the updatedAt
field for sorting in descending order
* from newest to oldest.
*
*
*
* This is a convenience which creates an instance of the {@link SearchImageSetsRequest.Builder} avoiding the need
* to create one manually via {@link SearchImageSetsRequest#builder()}
*
*
* @param searchImageSetsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.medicalimaging.model.SearchImageSetsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the SearchImageSets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.SearchImageSets
* @see AWS API Documentation
*/
default CompletableFuture searchImageSets(
Consumer searchImageSetsRequest) {
return searchImageSets(SearchImageSetsRequest.builder().applyMutation(searchImageSetsRequest).build());
}
/**
*
* This is a variant of
* {@link #searchImageSets(software.amazon.awssdk.services.medicalimaging.model.SearchImageSetsRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.medicalimaging.paginators.SearchImageSetsPublisher publisher = client.searchImageSetsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.medicalimaging.paginators.SearchImageSetsPublisher publisher = client.searchImageSetsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.medicalimaging.model.SearchImageSetsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #searchImageSets(software.amazon.awssdk.services.medicalimaging.model.SearchImageSetsRequest)}
* operation.
*
*
* @param searchImageSetsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.SearchImageSets
* @see AWS API Documentation
*/
default SearchImageSetsPublisher searchImageSetsPaginator(SearchImageSetsRequest searchImageSetsRequest) {
return new SearchImageSetsPublisher(this, searchImageSetsRequest);
}
/**
*
* This is a variant of
* {@link #searchImageSets(software.amazon.awssdk.services.medicalimaging.model.SearchImageSetsRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.medicalimaging.paginators.SearchImageSetsPublisher publisher = client.searchImageSetsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.medicalimaging.paginators.SearchImageSetsPublisher publisher = client.searchImageSetsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.medicalimaging.model.SearchImageSetsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #searchImageSets(software.amazon.awssdk.services.medicalimaging.model.SearchImageSetsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link SearchImageSetsRequest.Builder} avoiding the need
* to create one manually via {@link SearchImageSetsRequest#builder()}
*
*
* @param searchImageSetsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.medicalimaging.model.SearchImageSetsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.SearchImageSets
* @see AWS API Documentation
*/
default SearchImageSetsPublisher searchImageSetsPaginator(Consumer searchImageSetsRequest) {
return searchImageSetsPaginator(SearchImageSetsRequest.builder().applyMutation(searchImageSetsRequest).build());
}
/**
*
* Start importing bulk data into an ACTIVE
data store. The import job imports DICOM P10 files found in
* the S3 prefix specified by the inputS3Uri
parameter. The import job stores processing results in the
* file specified by the outputS3Uri
parameter.
*
*
* @param startDicomImportJobRequest
* @return A Java Future containing the result of the StartDICOMImportJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - ServiceQuotaExceededException The request caused a service quota to be exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.StartDICOMImportJob
* @see AWS API Documentation
*/
default CompletableFuture startDICOMImportJob(
StartDicomImportJobRequest startDicomImportJobRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Start importing bulk data into an ACTIVE
data store. The import job imports DICOM P10 files found in
* the S3 prefix specified by the inputS3Uri
parameter. The import job stores processing results in the
* file specified by the outputS3Uri
parameter.
*
*
*
* This is a convenience which creates an instance of the {@link StartDicomImportJobRequest.Builder} avoiding the
* need to create one manually via {@link StartDicomImportJobRequest#builder()}
*
*
* @param startDicomImportJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.medicalimaging.model.StartDicomImportJobRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the StartDICOMImportJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - ServiceQuotaExceededException The request caused a service quota to be exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.StartDICOMImportJob
* @see AWS API Documentation
*/
default CompletableFuture startDICOMImportJob(
Consumer startDicomImportJobRequest) {
return startDICOMImportJob(StartDicomImportJobRequest.builder().applyMutation(startDicomImportJobRequest).build());
}
/**
*
* Adds a user-specifed key and value tag to a medical imaging resource.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.TagResource
* @see AWS
* API Documentation
*/
default CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds a user-specifed key and value tag to a medical imaging resource.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.medicalimaging.model.TagResourceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.TagResource
* @see AWS
* API Documentation
*/
default CompletableFuture tagResource(Consumer tagResourceRequest) {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Removes tags from a medical imaging resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.UntagResource
* @see AWS
* API Documentation
*/
default CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes tags from a medical imaging resource.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.medicalimaging.model.UntagResourceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.UntagResource
* @see AWS
* API Documentation
*/
default CompletableFuture untagResource(Consumer untagResourceRequest) {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
*
* Update image set metadata attributes.
*
*
* @param updateImageSetMetadataRequest
* @return A Java Future containing the result of the UpdateImageSetMetadata operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - ServiceQuotaExceededException The request caused a service quota to be exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.UpdateImageSetMetadata
* @see AWS API Documentation
*/
default CompletableFuture updateImageSetMetadata(
UpdateImageSetMetadataRequest updateImageSetMetadataRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Update image set metadata attributes.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateImageSetMetadataRequest.Builder} avoiding the
* need to create one manually via {@link UpdateImageSetMetadataRequest#builder()}
*
*
* @param updateImageSetMetadataRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.medicalimaging.model.UpdateImageSetMetadataRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the UpdateImageSetMetadata operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException The request was denied due to throttling.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - AccessDeniedException The user does not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints set by the service.
* - InternalServerException An unexpected error occurred during processing of the request.
* - ResourceNotFoundException The request references a resource which does not exist.
* - ServiceQuotaExceededException The request caused a service quota to be exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MedicalImagingException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample MedicalImagingAsyncClient.UpdateImageSetMetadata
* @see AWS API Documentation
*/
default CompletableFuture updateImageSetMetadata(
Consumer updateImageSetMetadataRequest) {
return updateImageSetMetadata(UpdateImageSetMetadataRequest.builder().applyMutation(updateImageSetMetadataRequest)
.build());
}
@Override
default MedicalImagingServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
/**
* Create a {@link MedicalImagingAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static MedicalImagingAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link MedicalImagingAsyncClient}.
*/
static MedicalImagingAsyncClientBuilder builder() {
return new DefaultMedicalImagingAsyncClientBuilder();
}
}